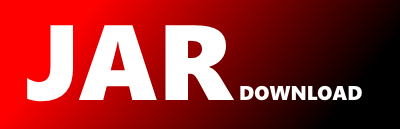
com.version1.testdata.BankHolidays Maven / Gradle / Ivy
package com.version1.testdata;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.JsonNode;
import com.version1.webdriver.utils.JsonUtils;
import java.util.List;
/**
* A simple representation class to calculate the bank holiday for different regions
*/
@JsonIgnoreProperties(ignoreUnknown = true)
public class BankHolidays {
private Locale locale;
private List englandAndWalesBankHolidays;
private List scotlandBankHolidays;
private List northernIrelandBankHolidays;
/**
*
* @return locale, Location to calculate the bank holiday
*/
public Locale getLocale() {
return locale;
}
/**
*
* @param locale, Location for the bank holiday
*/
public void setLocale(String locale) {
this.locale = Locale.valueOf(locale.replace("-", "_").toUpperCase());
}
/**
*
* @param jsonNode, Location for england-and-wales
*/
@JsonProperty("england-and-wales")
public void setEnglandAndWalesHolidays(JsonNode jsonNode) throws JsonProcessingException {
this.englandAndWalesBankHolidays = JsonUtils.fromString(jsonNode.get("events").toString(), new TypeReference<>() {
});
}
/**
*
* @param jsonNode, Location for scotland
*/
@JsonProperty("scotland")
public void scotlandHolidays(JsonNode jsonNode) throws JsonProcessingException {
this.scotlandBankHolidays = JsonUtils.fromString(jsonNode.get("events").toString(), new TypeReference<>() {});
}
/**
*
* @param jsonNode, Location for northern-ireland
*/
@JsonProperty("northern-ireland")
public void northernIrelandHolidays(JsonNode jsonNode) throws JsonProcessingException {
this.northernIrelandBankHolidays = JsonUtils.fromString(jsonNode.get("events").toString(), new TypeReference<>() {});
}
/**
* @param locale, Location for the bank holiday
* @return List, List of bank holidays based on location
*/
public List get(Locale locale) {
switch(locale) {
case ENGLAND_AND_WALES:
return this.englandAndWalesBankHolidays;
case SCOTLAND:
return this.scotlandBankHolidays;
case NORTHERN_IRELAND:
return this.northernIrelandBankHolidays;
default:
throw new IllegalArgumentException("Locale must be ENGLAND_AND_WALES, SCOTLAND or NORTHERN_IRELAND");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy