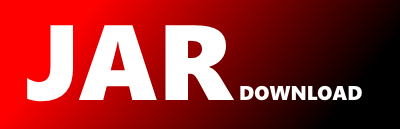
com.version1.webdriver.utils.WebDriverUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of selenium-java-test-automation Show documentation
Show all versions of selenium-java-test-automation Show documentation
A simple Selenium framework offering externalised configuration, a good selection of libraries for supporting
test data, simple WebDriver browser binary resolution and an opinionated approach for WebDriver test design.
The newest version!
package com.version1.webdriver.utils;
import java.awt.Toolkit;
import java.awt.datatransfer.StringSelection;
import java.util.List;
import java.util.NoSuchElementException;
import java.util.concurrent.TimeoutException;
import org.openqa.selenium.By;
import org.openqa.selenium.JavascriptExecutor;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.interactions.Actions;
import org.openqa.selenium.support.events.EventFiringWebDriver;
import org.openqa.selenium.support.ui.ExpectedCondition;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* WebDriver Utility class with helper methods for web element locators
* WebDriver explicit waits
*/
public class WebDriverUtils {
private static final long DRIVER_WAIT_TIME = 10;
private static final Logger LOG = LoggerFactory.getLogger(WebDriverUtils.class);
protected WebDriverWait Wait(EventFiringWebDriver webDriver){
WebDriverWait wait = new WebDriverWait(webDriver, DRIVER_WAIT_TIME);
return wait;
}
/**
* Gets the current URL
* @param webDriver used to execute the test cases
* @return URL, Returns the current URL
*/
public String getCurrentUrl(EventFiringWebDriver webDriver) {
return webDriver.getCurrentUrl();
}
/**
* Gets the current page title
* @param webDriver used to execute the test cases
* @return page title, Returns the current page title
*/
public String getCurrentPageTitle(EventFiringWebDriver webDriver) {
return webDriver.getTitle();
}
/**
* Check and matches the page title
* @param title, title to be matched with
* @param webDriver used to execute the test cases
* @return boolean matching the title
*/
public boolean checkPageTitle(String title,EventFiringWebDriver webDriver) {
return new WebDriverWait(webDriver, DRIVER_WAIT_TIME).until(ExpectedConditions.titleIs(title));
}
/**
* checking that the title of a page contains a case-sensitive substring
* @param title, title to be matched with
* @param webDriver used to execute the test cases
* @return boolean matching the title of a page
*/
public boolean checkPageTitleContains(String title,EventFiringWebDriver webDriver) {
return new WebDriverWait(webDriver, DRIVER_WAIT_TIME).until(ExpectedConditions.titleContains(title));
}
/**
* checking for the URL of the current page to be a specific URL
* @param url, url to be matched with
* @param webDriver used to execute the test cases
* @return boolean matching the url of a current page
*/
public boolean checkPageUrlToBe(String url,EventFiringWebDriver webDriver) {
return new WebDriverWait(webDriver, DRIVER_WAIT_TIME).until(ExpectedConditions.urlToBe(url));
}
/**
* checking for fraction of the url that the page should be on
* @param fraction, fraction of the url to be matched with
* @param webDriver used to execute the test cases
* @return boolean matching the fraction of the url that the page should be on
*/
public boolean checkPageUrlContains(String fraction,EventFiringWebDriver webDriver) {
return new WebDriverWait(webDriver, DRIVER_WAIT_TIME).until(ExpectedConditions.urlContains(fraction));
}
/**
* checking for regular expression that the URL should match
* @param regex, regular expression of the url to be matched with
* @param webDriver used to execute the test cases
* @return boolean matching regular expression that the URL should match
*/
public boolean checkPageUrlMatches(String regex,EventFiringWebDriver webDriver) {
return new WebDriverWait(webDriver, DRIVER_WAIT_TIME).until(ExpectedConditions.urlMatches(regex));
}
/**
* Waits for the expected element to be visible
* @param by which locates elements by the value of the attribute.
* @param webDriver used to execute the test cases
* @return WebElement after the visibility of the Element
*/
protected WebElement waitForExpectedElement(final By by,EventFiringWebDriver webDriver) {
return Wait(webDriver).until(visibilityOfElementLocated(by,webDriver));
}
/**
* Waits for the expected element to be visible till provided wait time in seconds
* @param by which locates elements by the value of the attribute
* @param waitTimeInSeconds, time to wait for the visibility of the Element
* @param webDriver used to execute the test cases
* @return WebElement after the visibility of the Element
*/
public WebElement waitForExpectedElement(final By by, long waitTimeInSeconds,EventFiringWebDriver webDriver) throws TimeoutException {
try {
WebDriverWait wait = new WebDriverWait(webDriver, waitTimeInSeconds);
return wait.until(visibilityOfElementLocated(by,webDriver));
} catch (NoSuchElementException e) {
LOG.info(e.getMessage());
return null;
}
}
/**
* Checks the referenced element is displayed on the web page or not
* @param by which locates elements by the value of the attribute
* @param webDriver used to execute the test cases
* @return WebElement after the Element is displayed
*/
private ExpectedCondition visibilityOfElementLocated(final By by,EventFiringWebDriver webDriver) throws NoSuchElementException {
return driver -> {
try {
Thread.sleep(500);
} catch (InterruptedException e) {
LOG.error(e.getMessage());
}
WebElement element = webDriver.findElement(by);
return element.isDisplayed() ? element : null;
};
}
/**
* Checks the text to be present in the Element
* @param element,Element in which the presence of text needs to be verified
* @param text to be verified
* @param webDriver used to execute the test cases
* @return boolean summarising the presence of the text
*/
public boolean textToBePresentInElement(WebElement element, String text,EventFiringWebDriver webDriver) {
return new WebDriverWait(webDriver, DRIVER_WAIT_TIME).until(ExpectedConditions.textToBePresentInElement(element, text));
}
/**
* Checks if the given text is present in the element that matches the given locator
* @param by which locates elements by the value of the attribute
* @param text to be verified
* @param webDriver used to execute the test cases
* @return boolean summarising the presence of the text that matches the given locator
*/
public boolean textToBePresentInElementLocated(final By by, final String text,EventFiringWebDriver webDriver) {
return new WebDriverWait(webDriver, DRIVER_WAIT_TIME).until(ExpectedConditions.textToBePresentInElementLocated(by, text));
}
/**
* Checks if the given text is present in the specified elements value attribute
* @param element,Element in which the presence of text needs to be verified
* @param text to be verified
* @param webDriver used to execute the test cases
* @return boolean summarising the presence of the text in the specified elements value attribute
*/
public boolean textToBePresentInElementValue(final WebElement element, final String text,EventFiringWebDriver webDriver) {
return new WebDriverWait(webDriver, DRIVER_WAIT_TIME).until(ExpectedConditions.textToBePresentInElementValue(element, text));
}
/**
* Checks if the given text is present in the specified elements value attribute
* @param by which locates elements by the value of the attribute
* @param text to be verified
* @param webDriver used to execute the test cases
* @return boolean summarising the presence of the text in the specified elements value attribute
*/
public boolean textToBePresentInElementValue(final By by, final String text,EventFiringWebDriver webDriver) {
return new WebDriverWait(webDriver, DRIVER_WAIT_TIME).until(ExpectedConditions.textToBePresentInElementValue(by, text));
}
/**
* Checks whether the given frame is available to switch to
* If the frame is available it switches the given driver to the specified frame.
* @param frameLocator,Locator to the frame
* @param webDriver used to execute the test cases
* @return WebDriver after the frame is available
*/
public WebDriver frameToBeAvailableAndSwitchToIt(final String frameLocator,EventFiringWebDriver webDriver) {
return new WebDriverWait(webDriver, DRIVER_WAIT_TIME).until(ExpectedConditions.frameToBeAvailableAndSwitchToIt(frameLocator));
}
/**
* Checks whether the given frame is available to switch to
* If the frame is available it switches the given driver to the specified frame.
* @param by which locates elements by the value of the attribute
* @param webDriver used to execute the test cases
* @return WebDriver after the frame is available
*/
public WebDriver frameToBeAvailableAndSwitchToIt(final By by,EventFiringWebDriver webDriver) {
return new WebDriverWait(webDriver, DRIVER_WAIT_TIME).until(ExpectedConditions.frameToBeAvailableAndSwitchToIt(by));
}
/**
* Waits for the expected element to be invisible
* @param by which locates elements by the value of the attribute.
* @param webDriver used to execute the test cases
* @return boolean after the invisibility of the Element
*/
public boolean invisibilityOfElementLocated(By by,EventFiringWebDriver webDriver) {
return (new WebDriverWait(webDriver, DRIVER_WAIT_TIME)).until(ExpectedConditions.invisibilityOfElementLocated(by));
}
/**
* Waits for the expected element with text to be invisible
* @param by which locates elements by the value of the attribute.
* @param text to be invisible.
* @param webDriver used to execute the test cases
* @return boolean after the invisibility of the Element
*/
public boolean invisibilityOfElementWithText(final By by, final String text,EventFiringWebDriver webDriver) {
return (new WebDriverWait(webDriver, DRIVER_WAIT_TIME)).until(ExpectedConditions.invisibilityOfElementWithText(by, text));
}
/**
* Waits for the expected element to be clickable
* @param by which locates elements by the value of the attribute.
* @param webDriver used to execute the test cases
* @return WebElement after the element is clickable
*/
public WebElement elementToBeClickable(By by,EventFiringWebDriver webDriver) {
return (new WebDriverWait(webDriver, DRIVER_WAIT_TIME)).until(ExpectedConditions.elementToBeClickable(by));
}
/**
* Waits for the expected element to be clickable
* @param element,Element to be clickable
* @param webDriver used to execute the test cases
* @return WebElement after the element is clickable
*/
public WebElement elementToBeClickable(final WebElement element,EventFiringWebDriver webDriver) {
return (new WebDriverWait(webDriver, DRIVER_WAIT_TIME)).until(ExpectedConditions.elementToBeClickable(element));
}
/**
* Wait until an element is no longer attached to the DOM
* @param element,Element to be clickable
* @param webDriver used to execute the test cases
* @return boolean summarising the staleness of the element
*/
public boolean stalenessOf(final WebElement element,EventFiringWebDriver webDriver) {
return (new WebDriverWait(webDriver, DRIVER_WAIT_TIME)).until(ExpectedConditions.stalenessOf(element));
}
/**
* Checks if the given element is selected.
* @param by which locates elements by the value of the attribute.
* @param webDriver used to execute the test cases
* @return boolean summarising the selection of the element
*/
public boolean elementToBeSelected(final By by,EventFiringWebDriver webDriver) {
return (new WebDriverWait(webDriver, DRIVER_WAIT_TIME)).until(ExpectedConditions.elementToBeSelected(by));
}
/**
* Checks if the given element is selected.
* @param element,Element to be selected
* @param webDriver used to execute the test cases
* @return boolean summarising the selection of the element
*/
public boolean elementToBeSelected(final WebElement element,EventFiringWebDriver webDriver) {
return (new WebDriverWait(webDriver, DRIVER_WAIT_TIME)).until(ExpectedConditions.elementToBeSelected(element));
}
/**
* Checks if the given element is selected.
* @param element,Element to be selected
* @param selected, Boolean summarising the selected status
* @param webDriver used to execute the test cases
* @return boolean summarising the selection of the element
*/
public boolean elementSelectionStateToBe(final WebElement element, final boolean selected,EventFiringWebDriver webDriver) {
return (new WebDriverWait(webDriver, DRIVER_WAIT_TIME)).until(ExpectedConditions.elementSelectionStateToBe(element, selected));
}
/**
* Checks if the given element is selected.
* @param by which locates elements by the value of the attribute.
* @param selected, Boolean summarising the selected status
* @param webDriver used to execute the test cases
* @return boolean summarising the selection of the element
*/
public boolean elementSelectionStateToBe(final By by,
final boolean selected,EventFiringWebDriver webDriver) {
return (new WebDriverWait(webDriver, DRIVER_WAIT_TIME)).until(ExpectedConditions.elementSelectionStateToBe(by, selected));
}
/**
* Checks and waits for the presence of alert
* @param webDriver used to execute the test cases
*/
public void waitForAlert(EventFiringWebDriver webDriver) {
(new WebDriverWait(webDriver, DRIVER_WAIT_TIME)).until(ExpectedConditions.alertIsPresent());
}
/**
* Waits for all expected elements to be visible
* @param by which locates elements by the value of the attribute.
* @param webDriver used to execute the test cases
* @return List of WebElement after the visibility of the Elements
*/
public List visibilityOfAllElementsLocatedBy(final By by,EventFiringWebDriver webDriver) {
return (new WebDriverWait(webDriver, DRIVER_WAIT_TIME)).until(ExpectedConditions.visibilityOfAllElementsLocatedBy(by));
}
/**
* Waits for all expected elements to be visible
* @param elements, List of WebElement
* @param webDriver used to execute the test cases
* @return List of WebElement after the visibility of the Elements
*/
public List visibilityOfAllElements(final List elements,EventFiringWebDriver webDriver) {
return (new WebDriverWait(webDriver, DRIVER_WAIT_TIME)).until(ExpectedConditions.visibilityOfAllElements(elements));
}
/**
* Checks the presence of all elements
* @param by which locates elements by the value of the attribute
* @param webDriver used to execute the test cases
* @return List of WebElement after the presence of the Elements
*/
public List presenceOfAllElementsLocatedBy(final By by,EventFiringWebDriver webDriver) {
return (new WebDriverWait(webDriver, DRIVER_WAIT_TIME)).until(ExpectedConditions.presenceOfAllElementsLocatedBy(by));
}
/**
* Checks the visibility of elements
* @param element,Element whose visibility is to be verified
* @param webDriver used to execute the test cases
* @return WebElement after the visibility of the Element
*/
public WebElement visibilityOf(final WebElement element,EventFiringWebDriver webDriver) {
return (new WebDriverWait(webDriver, DRIVER_WAIT_TIME)).until(ExpectedConditions.visibilityOf(element));
}
/**
* Checks the presence of element
* @param by which locates elements by the value of the attribute
* @param webDriver used to execute the test cases
* @return boolean summarising the presence of element
*/
public boolean isElementPresent(final By by,EventFiringWebDriver webDriver) {
new WebDriverWait(webDriver, DRIVER_WAIT_TIME).until(ExpectedConditions.presenceOfElementLocated(by));
return true;
}
/**
* Navigates to the previous page using browser back button
* @param webDriver used to execute the test cases
*/
public void navigateToPreviousPageUsingBrowserBackButton(EventFiringWebDriver webDriver) {
webDriver.navigate().back();
}
/**
* Clicks on the element with x,y coordinates.
* @param webElement,Element which needs to be clicked
* @param x, Coordinate x
* @param y, Coordinate y
* @param webDriver used to execute the test cases
*/
public void clickWithinElementWithXYCoordinates(WebElement webElement, int x, int y,EventFiringWebDriver webDriver) {
Actions builder = new Actions(webDriver);
builder.moveToElement(webElement, x, y);
builder.click();
builder.perform();
}
/**
* Using JS Executor finds the element by tag name.
* @param tagName,Name of the tag
* @param webDriver used to execute the test cases
* @return String representation of the object
*/
public String getElementByTagNameWithJSExecutor(String tagName,EventFiringWebDriver webDriver) {
return ((JavascriptExecutor) webDriver).executeScript("return window.getComputedStyle(document.getElementsByTagName('" + tagName + "')").toString();
}
/**
* Using JS Executor finds the element by document query selector.
* @param cssSelector,Name of Cascading Style Sheets
* @param webDriver used to execute the test cases
* @return String representation of the object
*/
public String getElementByQueryJSExecutor(String cssSelector,EventFiringWebDriver webDriver) {
return ((JavascriptExecutor) webDriver).executeScript("return window.getComputedStyle(document.querySelector('" + cssSelector + "')").toString();
}
/**
* Finds an element on the web page
* @param by which locates elements by the value of the attribute
* @param webDriver used to execute the test cases
* @return WebElement after locating the element
*/
protected WebElement element(final By by,EventFiringWebDriver webDriver) {
return webDriver.findElement(by);
}
/**
* Clears the text field and then enters the text
* @param by which locates elements by the value of the attribute
* @param inputText, Text to be entered in the text field
* @param webDriver used to execute the test cases
*/
protected void clearEnterText(By by, String inputText,EventFiringWebDriver webDriver) {
element(by,webDriver).clear();
element(by,webDriver).sendKeys(inputText);
}
/**
* Waits before clearing the text field and then enters the text
* @param by which locates elements by the value of the attribute
* @param inputText, Text to be entered in the text field
* @param webDriver used to execute the test cases
*/
protected void waitClearEnterText(By by, String inputText,EventFiringWebDriver webDriver) {
waitForExpectedElement(by,webDriver).clear();
element(by,webDriver).sendKeys(inputText);
}
/**
* Sets the Clip board data
* @param string, String to be set in the clip board
*/
public static void setClipboardData(String string) {
StringSelection stringSelection = new StringSelection(string);
Toolkit.getDefaultToolkit().getSystemClipboard().setContents(stringSelection, null);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy