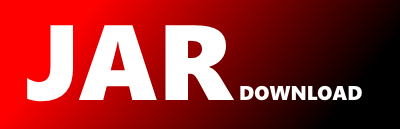
io.github.WeronikaJargielo.protein_interaction_finder.HydrophobicInteractionsFinder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of protein-interaction-finder Show documentation
Show all versions of protein-interaction-finder Show documentation
Library for finding possible interactions in proteins.
The newest version!
package io.github.WeronikaJargielo.protein_interaction_finder;
import org.biojava.nbio.structure.Atom;
import org.biojava.nbio.structure.Calc;
import java.util.*;
final class HydrophobicInteractionsFinder {
private final PdbStructureParser pdbStructureParser;
private final List nonPolarAminoAcids = Arrays.asList(AminoAcidAbbreviations.ALA,
AminoAcidAbbreviations.CYS,
AminoAcidAbbreviations.GLY,
AminoAcidAbbreviations.ILE,
AminoAcidAbbreviations.LEU,
AminoAcidAbbreviations.MET,
AminoAcidAbbreviations.PHE,
AminoAcidAbbreviations.PRO,
AminoAcidAbbreviations.TRP,
AminoAcidAbbreviations.TYR,
AminoAcidAbbreviations.VAL
);
private final String[] desiredAtoms = new String[] {"CA"};
public HydrophobicInteractionsFinder(PdbStructureParser pdbStructureParser) {
this.pdbStructureParser = pdbStructureParser;
}
List findHydrophobicInteractions(HydrophobicInteractionCriteria criteria) {
ArrayList CAsAtoms = pdbStructureParser.getAtoms(desiredAtoms, nonPolarAminoAcids);
ArrayList foundHydrophobicInteractions = new ArrayList<>();
final int CAsAtomsLen = CAsAtoms.size();
for (int i = 0; i < CAsAtomsLen; ++i) {
for (int j = i + 1; j < CAsAtomsLen; ++j) {
final HydrophobicInteraction hydrophobicInteraction = this.obtainHydrophobicInteraction(CAsAtoms.get(i),
CAsAtoms.get(j),
criteria);
if (hydrophobicInteraction != null) {
foundHydrophobicInteractions.add(hydrophobicInteraction);
}
}
}
return foundHydrophobicInteractions;
}
private HydrophobicInteraction obtainHydrophobicInteraction(Atom firstAtom, Atom secondAtom, HydrophobicInteractionCriteria criteria) {
final double distCAs = Calc.getDistance(firstAtom, secondAtom);
if (distCAs >= criteria.getMinDistanceCAs() && distCAs <= criteria.getMaxDistanceCAs()) {
return new HydrophobicInteraction(new AminoAcid(firstAtom.getGroup()),
new AminoAcid(secondAtom.getGroup()),
distCAs);
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy