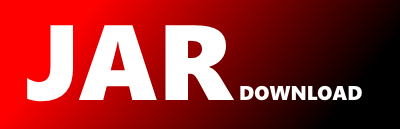
cloud.chain.git.api.service.impl.CompositeApiServiceImpl Maven / Gradle / Ivy
The newest version!
package cloud.chain.git.api.service.impl;
import cloud.chain.git.api.dto.*;
import cloud.chain.git.api.service.GitApiService;
import org.springframework.context.annotation.Primary;
import org.springframework.stereotype.Service;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.Objects;
/**
* wuXiaoMing
* 2023/2/10 13:40
*/
@Primary
@Service
public class CompositeApiServiceImpl implements GitApiService {
private static final List MEMBERS = new ArrayList<>();
public CompositeApiServiceImpl(List list) {
MEMBERS.addAll(list);
}
@Override
public Res getRepositoryContent(ReposInfo reposInfo) {
GitApiService apiService = MEMBERS.stream().findFirst().orElse(null);
if (Objects.nonNull(apiService)) {
return apiService.getRepositoryContent(reposInfo);
}
return null;
}
@Override
public Res getRepositoryContent(ReposInfo reposInfo, Map headers) {
GitApiService apiService = MEMBERS.stream().findFirst().orElse(null);
if (Objects.nonNull(apiService)) {
return apiService.getRepositoryContent(reposInfo, headers);
}
return null;
}
@Override
public Res createARepositoryForTheAuthenticatedUser(Repos repos) {
GitApiService apiService = MEMBERS.stream().findFirst().orElse(null);
if (Objects.nonNull(apiService)) {
return apiService.createARepositoryForTheAuthenticatedUser(repos);
}
return null;
}
@Override
public Res createOrUpdateFileContents(ReposFileInfo reposFileInfo, ReposInfo reposInfo) {
GitApiService apiService = MEMBERS.stream().findFirst().orElse(null);
if (Objects.nonNull(apiService)) {
return apiService.createOrUpdateFileContents(reposFileInfo, reposInfo);
}
return null;
}
@Override
public Res deleteAFile(ReposDeleteInfo reposDeleteInfo, ReposInfo reposInfo) {
GitApiService apiService = MEMBERS.stream().findFirst().orElse(null);
if (Objects.nonNull(apiService)) {
return apiService.deleteAFile(reposDeleteInfo, reposInfo);
}
return null;
}
@Override
public void proxy(String path, HttpServletRequest request, HttpServletResponse response) {
GitApiService apiService = MEMBERS.stream().findFirst().orElse(null);
if (Objects.nonNull(apiService)) {
apiService.proxy(path, request, response);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy