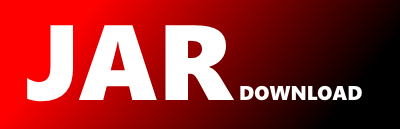
ca.gc.aafc.dina.mapper.DinaMapperV2 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dina-base-api Show documentation
Show all versions of dina-base-api Show documentation
Base DINA API package for Java built on SpringBoot and Crnk
The newest version!
package ca.gc.aafc.dina.mapper;
import java.util.Arrays;
import java.util.Set;
import java.util.UUID;
import org.apache.commons.lang3.StringUtils;
import org.mapstruct.Condition;
import org.mapstruct.Context;
import org.mapstruct.Named;
import org.mapstruct.SourcePropertyName;
import ca.gc.aafc.dina.dto.ExternalRelationDto;
/**
* MapStruct based mapper that supports provided property to prevent lazy-loading to be triggered.
*/
public interface DinaMapperV2 {
/**
* Map an entity to a new instance of a dto.
* @param entity
* @param provided provided properties so only those will be set
* @param scope used to check provided properties within nested properties
* @return dto instance
*/
D toDto(E entity, @Context Set provided, @Context String scope);
/**
* Map a dto to a new instance of an entity.
* @param dto
* @param provided provided properties so only those will be set
* @param scope used to check provided properties within nested properties
* @return entity instance
*/
E toEntity(D dto, @Context Set provided, @Context String scope);
/**
* Used to map the uuid of an {@link ExternalRelationDto}.
* To support legacy classes.
* @param er
* @return
*/
default UUID externalRelationToUUID(ExternalRelationDto er) {
if (er == null) {
return null;
}
return UUID.fromString(er.getId());
}
/**
* Used by MapStruct to map String arrays.
* @param arr
* @return
*/
default String[] nullSafeStringArrayCopy(String[] arr) {
if (arr == null) {
return null;
}
return Arrays.copyOf(arr, arr.length);
}
/**
* Used by MapStruct to control which field to set.
* @param sourcePropertyName
* @param provided
@param scope used to check provided properties within nested properties
* @return
*/
@Condition
default boolean isPropertyProvided(@SourcePropertyName String sourcePropertyName,
@Context Set provided, @Context String scope) {
if (StringUtils.isBlank(scope)) {
return provided.contains(sourcePropertyName);
}
// dealing with nested objects
return provided.contains(scope + "." + sourcePropertyName);
}
/**
* Used to map UUID of type person to {@link ExternalRelationDto} for all legacy classes.
* Usage: @Mapping(source = "agent", target = "agent", qualifiedByName = "uuidToPersonExternalRelation")
* @param personUUID
* @return
*/
@Named("uuidToPersonExternalRelation")
static ExternalRelationDto uuidToPersonExternalRelation(UUID personUUID) {
if (personUUID == null) {
return null;
}
return ExternalRelationDto.builder().id(personUUID.toString()).type("person").build();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy