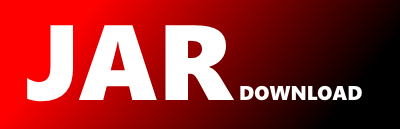
fs2-grpc.com.eventstore.dbclient.proto.streams.StreamsFs2GrpcTrailers.scala Maven / Gradle / Ivy
The newest version!
package com.eventstore.dbclient.proto.streams
import _root_.cats.syntax.all.*
trait StreamsFs2GrpcTrailers[F[_], A] {
def read(request: com.eventstore.dbclient.proto.streams.ReadReq, ctx: A): _root_.fs2.Stream[F, com.eventstore.dbclient.proto.streams.ReadResp]
def append(request: _root_.fs2.Stream[F, com.eventstore.dbclient.proto.streams.AppendReq], ctx: A): F[(com.eventstore.dbclient.proto.streams.AppendResp, _root_.io.grpc.Metadata)]
def delete(request: com.eventstore.dbclient.proto.streams.DeleteReq, ctx: A): F[(com.eventstore.dbclient.proto.streams.DeleteResp, _root_.io.grpc.Metadata)]
def tombstone(request: com.eventstore.dbclient.proto.streams.TombstoneReq, ctx: A): F[(com.eventstore.dbclient.proto.streams.TombstoneResp, _root_.io.grpc.Metadata)]
def batchAppend(request: _root_.fs2.Stream[F, com.eventstore.dbclient.proto.streams.BatchAppendReq], ctx: A): _root_.fs2.Stream[F, com.eventstore.dbclient.proto.streams.BatchAppendResp]
}
object StreamsFs2GrpcTrailers extends _root_.fs2.grpc.GeneratedCompanion[StreamsFs2GrpcTrailers] {
def serviceDescriptor: _root_.io.grpc.ServiceDescriptor = com.eventstore.dbclient.proto.streams.StreamsGrpc.SERVICE
def mkClient[F[_]: _root_.cats.effect.Async, A](dispatcher: _root_.cats.effect.std.Dispatcher[F], channel: _root_.io.grpc.Channel, mkMetadata: A => F[_root_.io.grpc.Metadata], clientOptions: _root_.fs2.grpc.client.ClientOptions): StreamsFs2GrpcTrailers[F, A] = new StreamsFs2GrpcTrailers[F, A] {
def read(request: com.eventstore.dbclient.proto.streams.ReadReq, ctx: A): _root_.fs2.Stream[F, com.eventstore.dbclient.proto.streams.ReadResp] = {
_root_.fs2.Stream.eval(mkMetadata(ctx)).flatMap { m =>
_root_.fs2.Stream.eval(_root_.fs2.grpc.client.Fs2ClientCall[F](channel, com.eventstore.dbclient.proto.streams.StreamsGrpc.METHOD_READ, dispatcher, clientOptions)).flatMap(_.unaryToStreamingCall(request, m))
}
}
def append(request: _root_.fs2.Stream[F, com.eventstore.dbclient.proto.streams.AppendReq], ctx: A): F[(com.eventstore.dbclient.proto.streams.AppendResp, _root_.io.grpc.Metadata)] = {
mkMetadata(ctx).flatMap { m =>
_root_.fs2.grpc.client.Fs2ClientCall[F](channel, com.eventstore.dbclient.proto.streams.StreamsGrpc.METHOD_APPEND, dispatcher, clientOptions).flatMap(_.streamingToUnaryCallTrailers(request, m))
}
}
def delete(request: com.eventstore.dbclient.proto.streams.DeleteReq, ctx: A): F[(com.eventstore.dbclient.proto.streams.DeleteResp, _root_.io.grpc.Metadata)] = {
mkMetadata(ctx).flatMap { m =>
_root_.fs2.grpc.client.Fs2ClientCall[F](channel, com.eventstore.dbclient.proto.streams.StreamsGrpc.METHOD_DELETE, dispatcher, clientOptions).flatMap(_.unaryToUnaryCallTrailers(request, m))
}
}
def tombstone(request: com.eventstore.dbclient.proto.streams.TombstoneReq, ctx: A): F[(com.eventstore.dbclient.proto.streams.TombstoneResp, _root_.io.grpc.Metadata)] = {
mkMetadata(ctx).flatMap { m =>
_root_.fs2.grpc.client.Fs2ClientCall[F](channel, com.eventstore.dbclient.proto.streams.StreamsGrpc.METHOD_TOMBSTONE, dispatcher, clientOptions).flatMap(_.unaryToUnaryCallTrailers(request, m))
}
}
def batchAppend(request: _root_.fs2.Stream[F, com.eventstore.dbclient.proto.streams.BatchAppendReq], ctx: A): _root_.fs2.Stream[F, com.eventstore.dbclient.proto.streams.BatchAppendResp] = {
_root_.fs2.Stream.eval(mkMetadata(ctx)).flatMap { m =>
_root_.fs2.Stream.eval(_root_.fs2.grpc.client.Fs2ClientCall[F](channel, com.eventstore.dbclient.proto.streams.StreamsGrpc.METHOD_BATCH_APPEND, dispatcher, clientOptions)).flatMap(_.streamingToStreamingCall(request, m))
}
}
}
protected def serviceBinding[F[_]: _root_.cats.effect.Async, A](dispatcher: _root_.cats.effect.std.Dispatcher[F], serviceImpl: StreamsFs2GrpcTrailers[F, A], mkCtx: _root_.io.grpc.Metadata => F[A], serverOptions: _root_.fs2.grpc.server.ServerOptions): _root_.io.grpc.ServerServiceDefinition = {
_root_.io.grpc.ServerServiceDefinition
.builder(com.eventstore.dbclient.proto.streams.StreamsGrpc.SERVICE)
.addMethod(com.eventstore.dbclient.proto.streams.StreamsGrpc.METHOD_READ, _root_.fs2.grpc.server.Fs2ServerCallHandler[F](dispatcher, serverOptions).unaryToStreamingCall[com.eventstore.dbclient.proto.streams.ReadReq, com.eventstore.dbclient.proto.streams.ReadResp]((r, m) => _root_.fs2.Stream.eval(mkCtx(m)).flatMap(serviceImpl.read(r, _))))
.addMethod(com.eventstore.dbclient.proto.streams.StreamsGrpc.METHOD_APPEND, _root_.fs2.grpc.server.Fs2ServerCallHandler[F](dispatcher, serverOptions).streamingToUnaryCallTrailers[com.eventstore.dbclient.proto.streams.AppendReq, com.eventstore.dbclient.proto.streams.AppendResp]((r, m) => mkCtx(m).flatMap(serviceImpl.append(r, _))))
.addMethod(com.eventstore.dbclient.proto.streams.StreamsGrpc.METHOD_DELETE, _root_.fs2.grpc.server.Fs2ServerCallHandler[F](dispatcher, serverOptions).unaryToUnaryCallTrailers[com.eventstore.dbclient.proto.streams.DeleteReq, com.eventstore.dbclient.proto.streams.DeleteResp]((r, m) => mkCtx(m).flatMap(serviceImpl.delete(r, _))))
.addMethod(com.eventstore.dbclient.proto.streams.StreamsGrpc.METHOD_TOMBSTONE, _root_.fs2.grpc.server.Fs2ServerCallHandler[F](dispatcher, serverOptions).unaryToUnaryCallTrailers[com.eventstore.dbclient.proto.streams.TombstoneReq, com.eventstore.dbclient.proto.streams.TombstoneResp]((r, m) => mkCtx(m).flatMap(serviceImpl.tombstone(r, _))))
.addMethod(com.eventstore.dbclient.proto.streams.StreamsGrpc.METHOD_BATCH_APPEND, _root_.fs2.grpc.server.Fs2ServerCallHandler[F](dispatcher, serverOptions).streamingToStreamingCall[com.eventstore.dbclient.proto.streams.BatchAppendReq, com.eventstore.dbclient.proto.streams.BatchAppendResp]((r, m) => _root_.fs2.Stream.eval(mkCtx(m)).flatMap(serviceImpl.batchAppend(r, _))))
.build()
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy