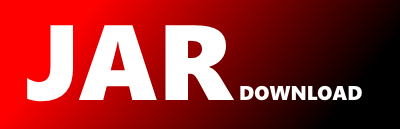
scalapb.com.google.rpc.Code.scala Maven / Gradle / Ivy
The newest version!
// Generated by the Scala Plugin for the Protocol Buffer Compiler.
// Do not edit!
//
// Protofile syntax: PROTO3
package com.google.rpc
/** The canonical error codes for gRPC APIs.
*
*
* Sometimes multiple error codes may apply. Services should return
* the most specific error code that applies. For example, prefer
* `OUT_OF_RANGE` over `FAILED_PRECONDITION` if both codes apply.
* Similarly prefer `NOT_FOUND` or `ALREADY_EXISTS` over `FAILED_PRECONDITION`.
*/
sealed abstract class Code(val value: _root_.scala.Int) extends _root_.scalapb.GeneratedEnum {
type EnumType = com.google.rpc.Code
type RecognizedType = com.google.rpc.Code.Recognized
def isOk: _root_.scala.Boolean = false
def isCancelled: _root_.scala.Boolean = false
def isUnknown: _root_.scala.Boolean = false
def isInvalidArgument: _root_.scala.Boolean = false
def isDeadlineExceeded: _root_.scala.Boolean = false
def isNotFound: _root_.scala.Boolean = false
def isAlreadyExists: _root_.scala.Boolean = false
def isPermissionDenied: _root_.scala.Boolean = false
def isUnauthenticated: _root_.scala.Boolean = false
def isResourceExhausted: _root_.scala.Boolean = false
def isFailedPrecondition: _root_.scala.Boolean = false
def isAborted: _root_.scala.Boolean = false
def isOutOfRange: _root_.scala.Boolean = false
def isUnimplemented: _root_.scala.Boolean = false
def isInternal: _root_.scala.Boolean = false
def isUnavailable: _root_.scala.Boolean = false
def isDataLoss: _root_.scala.Boolean = false
def companion: _root_.scalapb.GeneratedEnumCompanion[Code] = com.google.rpc.Code
final def asRecognized: _root_.scala.Option[com.google.rpc.Code.Recognized] = if (isUnrecognized) _root_.scala.None else _root_.scala.Some(this.asInstanceOf[com.google.rpc.Code.Recognized])
}
object Code extends _root_.scalapb.GeneratedEnumCompanion[Code] {
sealed trait Recognized extends Code
implicit def enumCompanion: _root_.scalapb.GeneratedEnumCompanion[Code] = this
/** Not an error; returned on success
*
* HTTP Mapping: 200 OK
*/
@SerialVersionUID(0L)
case object OK extends Code(0) with Code.Recognized {
val index = 0
val name = "OK"
override def isOk: _root_.scala.Boolean = true
}
/** The operation was cancelled, typically by the caller.
*
* HTTP Mapping: 499 Client Closed Request
*/
@SerialVersionUID(0L)
case object CANCELLED extends Code(1) with Code.Recognized {
val index = 1
val name = "CANCELLED"
override def isCancelled: _root_.scala.Boolean = true
}
/** Unknown error. For example, this error may be returned when
* a `Status` value received from another address space belongs to
* an error space that is not known in this address space. Also
* errors raised by APIs that do not return enough error information
* may be converted to this error.
*
* HTTP Mapping: 500 Internal Server Error
*/
@SerialVersionUID(0L)
case object UNKNOWN extends Code(2) with Code.Recognized {
val index = 2
val name = "UNKNOWN"
override def isUnknown: _root_.scala.Boolean = true
}
/** The client specified an invalid argument. Note that this differs
* from `FAILED_PRECONDITION`. `INVALID_ARGUMENT` indicates arguments
* that are problematic regardless of the state of the system
* (e.g., a malformed file name).
*
* HTTP Mapping: 400 Bad Request
*/
@SerialVersionUID(0L)
case object INVALID_ARGUMENT extends Code(3) with Code.Recognized {
val index = 3
val name = "INVALID_ARGUMENT"
override def isInvalidArgument: _root_.scala.Boolean = true
}
/** The deadline expired before the operation could complete. For operations
* that change the state of the system, this error may be returned
* even if the operation has completed successfully. For example, a
* successful response from a server could have been delayed long
* enough for the deadline to expire.
*
* HTTP Mapping: 504 Gateway Timeout
*/
@SerialVersionUID(0L)
case object DEADLINE_EXCEEDED extends Code(4) with Code.Recognized {
val index = 4
val name = "DEADLINE_EXCEEDED"
override def isDeadlineExceeded: _root_.scala.Boolean = true
}
/** Some requested entity (e.g., file or directory) was not found.
*
* Note to server developers: if a request is denied for an entire class
* of users, such as gradual feature rollout or undocumented whitelist,
* `NOT_FOUND` may be used. If a request is denied for some users within
* a class of users, such as user-based access control, `PERMISSION_DENIED`
* must be used.
*
* HTTP Mapping: 404 Not Found
*/
@SerialVersionUID(0L)
case object NOT_FOUND extends Code(5) with Code.Recognized {
val index = 5
val name = "NOT_FOUND"
override def isNotFound: _root_.scala.Boolean = true
}
/** The entity that a client attempted to create (e.g., file or directory)
* already exists.
*
* HTTP Mapping: 409 Conflict
*/
@SerialVersionUID(0L)
case object ALREADY_EXISTS extends Code(6) with Code.Recognized {
val index = 6
val name = "ALREADY_EXISTS"
override def isAlreadyExists: _root_.scala.Boolean = true
}
/** The caller does not have permission to execute the specified
* operation. `PERMISSION_DENIED` must not be used for rejections
* caused by exhausting some resource (use `RESOURCE_EXHAUSTED`
* instead for those errors). `PERMISSION_DENIED` must not be
* used if the caller can not be identified (use `UNAUTHENTICATED`
* instead for those errors). This error code does not imply the
* request is valid or the requested entity exists or satisfies
* other pre-conditions.
*
* HTTP Mapping: 403 Forbidden
*/
@SerialVersionUID(0L)
case object PERMISSION_DENIED extends Code(7) with Code.Recognized {
val index = 7
val name = "PERMISSION_DENIED"
override def isPermissionDenied: _root_.scala.Boolean = true
}
/** The request does not have valid authentication credentials for the
* operation.
*
* HTTP Mapping: 401 Unauthorized
*/
@SerialVersionUID(0L)
case object UNAUTHENTICATED extends Code(16) with Code.Recognized {
val index = 8
val name = "UNAUTHENTICATED"
override def isUnauthenticated: _root_.scala.Boolean = true
}
/** Some resource has been exhausted, perhaps a per-user quota, or
* perhaps the entire file system is out of space.
*
* HTTP Mapping: 429 Too Many Requests
*/
@SerialVersionUID(0L)
case object RESOURCE_EXHAUSTED extends Code(8) with Code.Recognized {
val index = 9
val name = "RESOURCE_EXHAUSTED"
override def isResourceExhausted: _root_.scala.Boolean = true
}
/** The operation was rejected because the system is not in a state
* required for the operation's execution. For example, the directory
* to be deleted is non-empty, an rmdir operation is applied to
* a non-directory, etc.
*
* Service implementors can use the following guidelines to decide
* between `FAILED_PRECONDITION`, `ABORTED`, and `UNAVAILABLE`:
* (a) Use `UNAVAILABLE` if the client can retry just the failing call.
* (b) Use `ABORTED` if the client should retry at a higher level
* (e.g., when a client-specified test-and-set fails, indicating the
* client should restart a read-modify-write sequence).
* (c) Use `FAILED_PRECONDITION` if the client should not retry until
* the system state has been explicitly fixed. E.g., if an "rmdir"
* fails because the directory is non-empty, `FAILED_PRECONDITION`
* should be returned since the client should not retry unless
* the files are deleted from the directory.
*
* HTTP Mapping: 400 Bad Request
*/
@SerialVersionUID(0L)
case object FAILED_PRECONDITION extends Code(9) with Code.Recognized {
val index = 10
val name = "FAILED_PRECONDITION"
override def isFailedPrecondition: _root_.scala.Boolean = true
}
/** The operation was aborted, typically due to a concurrency issue such as
* a sequencer check failure or transaction abort.
*
* See the guidelines above for deciding between `FAILED_PRECONDITION`,
* `ABORTED`, and `UNAVAILABLE`.
*
* HTTP Mapping: 409 Conflict
*/
@SerialVersionUID(0L)
case object ABORTED extends Code(10) with Code.Recognized {
val index = 11
val name = "ABORTED"
override def isAborted: _root_.scala.Boolean = true
}
/** The operation was attempted past the valid range. E.g., seeking or
* reading past end-of-file.
*
* Unlike `INVALID_ARGUMENT`, this error indicates a problem that may
* be fixed if the system state changes. For example, a 32-bit file
* system will generate `INVALID_ARGUMENT` if asked to read at an
* offset that is not in the range [0,2^32-1], but it will generate
* `OUT_OF_RANGE` if asked to read from an offset past the current
* file size.
*
* There is a fair bit of overlap between `FAILED_PRECONDITION` and
* `OUT_OF_RANGE`. We recommend using `OUT_OF_RANGE` (the more specific
* error) when it applies so that callers who are iterating through
* a space can easily look for an `OUT_OF_RANGE` error to detect when
* they are done.
*
* HTTP Mapping: 400 Bad Request
*/
@SerialVersionUID(0L)
case object OUT_OF_RANGE extends Code(11) with Code.Recognized {
val index = 12
val name = "OUT_OF_RANGE"
override def isOutOfRange: _root_.scala.Boolean = true
}
/** The operation is not implemented or is not supported/enabled in this
* service.
*
* HTTP Mapping: 501 Not Implemented
*/
@SerialVersionUID(0L)
case object UNIMPLEMENTED extends Code(12) with Code.Recognized {
val index = 13
val name = "UNIMPLEMENTED"
override def isUnimplemented: _root_.scala.Boolean = true
}
/** Internal errors. This means that some invariants expected by the
* underlying system have been broken. This error code is reserved
* for serious errors.
*
* HTTP Mapping: 500 Internal Server Error
*/
@SerialVersionUID(0L)
case object INTERNAL extends Code(13) with Code.Recognized {
val index = 14
val name = "INTERNAL"
override def isInternal: _root_.scala.Boolean = true
}
/** The service is currently unavailable. This is most likely a
* transient condition, which can be corrected by retrying with
* a backoff. Note that it is not always safe to retry
* non-idempotent operations.
*
* See the guidelines above for deciding between `FAILED_PRECONDITION`,
* `ABORTED`, and `UNAVAILABLE`.
*
* HTTP Mapping: 503 Service Unavailable
*/
@SerialVersionUID(0L)
case object UNAVAILABLE extends Code(14) with Code.Recognized {
val index = 15
val name = "UNAVAILABLE"
override def isUnavailable: _root_.scala.Boolean = true
}
/** Unrecoverable data loss or corruption.
*
* HTTP Mapping: 500 Internal Server Error
*/
@SerialVersionUID(0L)
case object DATA_LOSS extends Code(15) with Code.Recognized {
val index = 16
val name = "DATA_LOSS"
override def isDataLoss: _root_.scala.Boolean = true
}
@SerialVersionUID(0L)
final case class Unrecognized(unrecognizedValue: _root_.scala.Int) extends Code(unrecognizedValue) with _root_.scalapb.UnrecognizedEnum
lazy val values: scala.collection.immutable.Seq[ValueType] = scala.collection.immutable.Seq(OK, CANCELLED, UNKNOWN, INVALID_ARGUMENT, DEADLINE_EXCEEDED, NOT_FOUND, ALREADY_EXISTS, PERMISSION_DENIED, UNAUTHENTICATED, RESOURCE_EXHAUSTED, FAILED_PRECONDITION, ABORTED, OUT_OF_RANGE, UNIMPLEMENTED, INTERNAL, UNAVAILABLE, DATA_LOSS)
def fromValue(__value: _root_.scala.Int): Code = __value match {
case 0 => OK
case 1 => CANCELLED
case 2 => UNKNOWN
case 3 => INVALID_ARGUMENT
case 4 => DEADLINE_EXCEEDED
case 5 => NOT_FOUND
case 6 => ALREADY_EXISTS
case 7 => PERMISSION_DENIED
case 8 => RESOURCE_EXHAUSTED
case 9 => FAILED_PRECONDITION
case 10 => ABORTED
case 11 => OUT_OF_RANGE
case 12 => UNIMPLEMENTED
case 13 => INTERNAL
case 14 => UNAVAILABLE
case 15 => DATA_LOSS
case 16 => UNAUTHENTICATED
case __other => Unrecognized(__other)
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.EnumDescriptor = CodeProto.javaDescriptor.getEnumTypes().get(0)
def scalaDescriptor: _root_.scalapb.descriptors.EnumDescriptor = CodeProto.scalaDescriptor.enums(0)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy