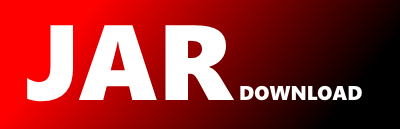
io.github.airiot.sdk.client.http.clients.HttpProjectAuthorizationClientImpl Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.github.airiot.sdk.client.http.clients;
import feign.FeignException;
import io.github.airiot.sdk.client.dto.ResponseDTO;
import io.github.airiot.sdk.client.dto.Token;
import io.github.airiot.sdk.client.exception.AuthorizationException;
import io.github.airiot.sdk.client.properties.AuthorizationProperties;
import io.github.airiot.sdk.client.service.AuthorizationClient;
import io.github.airiot.sdk.client.service.core.AppClient;
import java.time.Duration;
import java.util.concurrent.atomic.AtomicReference;
public class HttpProjectAuthorizationClientImpl implements AuthorizationClient {
private final AtomicReference holder = new AtomicReference<>();
private final AppClient appClient;
private final AuthorizationProperties properties;
public HttpProjectAuthorizationClientImpl(AppClient appClient, AuthorizationProperties properties) {
this.appClient = appClient;
this.properties = properties;
}
@Override
public synchronized Token getToken() throws AuthorizationException {
TokenHolder token = this.holder.get();
if (token != null && token.equals(this.properties) && !token.getToken().isExpired(Duration.ofSeconds(60))) {
return token.getToken();
}
try {
ResponseDTO response = this.appClient.getToken(this.properties.getAppKey(), this.properties.getAppSecret());
Token t = response.unwrap(() -> new AuthorizationException(response.getCode(), response.getMessage(), response.getDetail()));
this.holder.set(new TokenHolder(this.properties.getAppKey(), this.properties.getAppSecret(), t));
return t;
} catch (FeignException.NotFound e) {
throw new AuthorizationException(404, "获取平台 token 失败", "请检查平台地址及端口是否正确", e);
} catch (FeignException e) {
throw new AuthorizationException(e.status(), e.getMessage(), e.contentUTF8());
} catch (Exception e) {
throw new AuthorizationException(500, "获取平台 token 失败", e.getMessage(), e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy