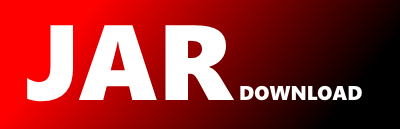
io.github.albertus82.jface.i18n.LocalizedWidgets Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jface-utils Show documentation
Show all versions of jface-utils Show documentation
Java SWT/JFace Utility Library including a Preferences Framework, Lightweight HTTP Server and macOS support.
package io.github.albertus82.jface.i18n;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.AbstractMap.SimpleEntry;
import java.util.Collection;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
import org.eclipse.swt.widgets.Widget;
import io.github.albertus82.util.ISupplier;
public class LocalizedWidgets implements Map> {
private static final Map, Method> setTextMethods = new HashMap<>();
private final Map> wrappedMap;
/** @see HashMap#HashMap() */
public LocalizedWidgets() {
wrappedMap = new HashMap<>();
}
/**
* @param initialCapacity the initial capacity.
*
* @see HashMap#HashMap(int)
*/
public LocalizedWidgets(final int initialCapacity) {
wrappedMap = new HashMap<>(initialCapacity);
}
@Override
public ISupplier put(final Widget widget, final ISupplier textSupplier) {
final Class extends Widget> widgetClass = widget.getClass();
if (!setTextMethods.containsKey(widgetClass)) {
try {
setTextMethods.put(widgetClass, widgetClass.getMethod("setText", String.class));
}
catch (final NoSuchMethodException e) {
throw new IllegalArgumentException(String.valueOf(widget), e);
}
}
setText(widget, textSupplier);
return wrappedMap.put(widget, textSupplier);
}
@Override
public void putAll(final Map extends Widget, ? extends ISupplier> m) {
for (final Entry extends Widget, ? extends ISupplier> entry : m.entrySet()) {
put(entry.getKey(), entry.getValue());
}
}
@Override
public int size() {
return wrappedMap.size();
}
@Override
public boolean isEmpty() {
return wrappedMap.isEmpty();
}
@Override
public boolean containsKey(final Object widget) {
return wrappedMap.containsKey(widget);
}
@Override
public boolean containsValue(final Object textSupplier) {
return wrappedMap.containsValue(textSupplier);
}
@Override
public ISupplier get(final Object widget) {
return wrappedMap.get(widget);
}
@Override
public ISupplier remove(final Object widget) {
return wrappedMap.remove(widget);
}
@Override
public void clear() {
wrappedMap.clear();
}
@Override
public Set keySet() {
return wrappedMap.keySet();
}
@Override
public Collection> values() {
return wrappedMap.values();
}
@Override
public Set>> entrySet() {
return wrappedMap.entrySet();
}
public Entry> putAndReturn(final T widget, final ISupplier textSupplier) {
put(widget, textSupplier);
return new SimpleEntry<>(widget, textSupplier);
}
public void resetText(final Widget widget) {
setText(widget, wrappedMap.get(widget));
}
public void resetAllTexts() {
for (final Entry> entry : wrappedMap.entrySet()) {
setText(entry.getKey(), entry.getValue());
}
}
private static void setText(final Widget widget, final ISupplier textSupplier) {
if (textSupplier != null && widget != null && !widget.isDisposed()) {
try {
setTextMethods.get(widget.getClass()).invoke(widget, String.valueOf(textSupplier.get()));
}
catch (final IllegalAccessException e) {
throw new IllegalStateException(e);
}
catch (final InvocationTargetException e) {
throw new IllegalStateException(e);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy