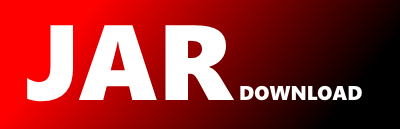
io.github.albertus82.jface.maps.MapBounds Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jface-utils Show documentation
Show all versions of jface-utils Show documentation
Java SWT/JFace Utility Library including a Preferences Framework, Lightweight HTTP Server and macOS support.
package io.github.albertus82.jface.maps;
import java.io.Serializable;
public class MapBounds implements Serializable {
private static final long serialVersionUID = -3330484387877221810L;
public static final int LATITUDE_MIN_VALUE = -90;
public static final int LATITUDE_MAX_VALUE = 90;
public static final int LONGITUDE_MIN_VALUE = -180;
public static final int LONGITUDE_MAX_VALUE = 180;
private final Double northEastLat;
private final Double southWestLat;
private final Double northEastLng;
private final Double southWestLng;
public MapBounds() {
this(null, null, null, null);
}
public MapBounds(final Double northEastLat, final Double southWestLat, final Double northEastLng, final Double southWestLng) {
this.northEastLat = northEastLat;
this.southWestLat = southWestLat;
this.northEastLng = northEastLng;
this.southWestLng = southWestLng;
}
public Double getNorthEastLat() {
return northEastLat;
}
public Double getSouthWestLat() {
return southWestLat;
}
public Double getNorthEastLng() {
return northEastLng;
}
public Double getSouthWestLng() {
return southWestLng;
}
@Override
public String toString() {
return "MapBounds [northEastLat=" + northEastLat + ", southWestLat=" + southWestLat + ", northEastLng=" + northEastLng + ", southWestLng=" + southWestLng + "]";
}
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + ((northEastLat == null) ? 0 : northEastLat.hashCode());
result = prime * result + ((northEastLng == null) ? 0 : northEastLng.hashCode());
result = prime * result + ((southWestLat == null) ? 0 : southWestLat.hashCode());
result = prime * result + ((southWestLng == null) ? 0 : southWestLng.hashCode());
return result;
}
@Override
public boolean equals(final Object obj) { // NOSONAR Autogenerated method
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof MapBounds)) {
return false;
}
MapBounds other = (MapBounds) obj;
if (northEastLat == null) {
if (other.northEastLat != null) {
return false;
}
}
else if (!northEastLat.equals(other.northEastLat)) {
return false;
}
if (northEastLng == null) {
if (other.northEastLng != null) {
return false;
}
}
else if (!northEastLng.equals(other.northEastLng)) {
return false;
}
if (southWestLat == null) {
if (other.southWestLat != null) {
return false;
}
}
else if (!southWestLat.equals(other.southWestLat)) {
return false;
}
if (southWestLng == null) {
if (other.southWestLng != null) {
return false;
}
}
else if (!southWestLng.equals(other.southWestLng)) {
return false;
}
return true;
}
public static MapBounds normalize(final MapBounds bounds) {
Double southWestLat = null;
Double northEastLat = null;
Double southWestLng = null;
Double northEastLng = null;
// Latitude
if (bounds.getSouthWestLat() != null && bounds.getNorthEastLat() != null) {
southWestLat = Math.max(MapBounds.LATITUDE_MIN_VALUE, bounds.getSouthWestLat());
northEastLat = Math.min(MapBounds.LATITUDE_MAX_VALUE, bounds.getNorthEastLat());
}
// Longitude
if (bounds.getSouthWestLng() != null && bounds.getNorthEastLng() != null) {
if (Math.abs(bounds.getSouthWestLng() - bounds.getNorthEastLng()) >= MapBounds.LONGITUDE_MAX_VALUE * 2) {
southWestLng = Double.valueOf(MapBounds.LONGITUDE_MIN_VALUE);
northEastLng = Double.valueOf(MapBounds.LONGITUDE_MAX_VALUE);
}
else {
double sw = bounds.getSouthWestLng();
while (Math.abs(sw) > MapBounds.LONGITUDE_MAX_VALUE) {
sw -= Math.signum(sw) * MapBounds.LONGITUDE_MAX_VALUE * 2;
}
southWestLng = Math.max(MapBounds.LONGITUDE_MIN_VALUE, sw);
double ne = bounds.getNorthEastLng();
while (Math.abs(ne) > MapBounds.LONGITUDE_MAX_VALUE) {
ne -= Math.signum(ne) * MapBounds.LONGITUDE_MAX_VALUE * 2;
}
northEastLng = Math.min(MapBounds.LONGITUDE_MAX_VALUE, ne);
}
}
return new MapBounds(northEastLat, southWestLat, northEastLng, southWestLng);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy