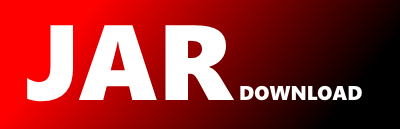
io.github.albertus82.jface.sysinfo.SystemInformationExporter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jface-utils Show documentation
Show all versions of jface-utils Show documentation
Java SWT/JFace Utility Library including a Preferences Framework, Lightweight HTTP Server and macOS support.
package io.github.albertus82.jface.sysinfo;
import java.io.BufferedWriter;
import java.io.FileWriter;
import java.io.IOException;
import java.lang.reflect.InvocationTargetException;
import java.text.DateFormat;
import java.util.Date;
import java.util.Locale;
import java.util.Map;
import java.util.Map.Entry;
import org.eclipse.core.runtime.IProgressMonitor;
import org.eclipse.jface.operation.IRunnableWithProgress;
import io.github.albertus82.jface.JFaceMessages;
import io.github.albertus82.util.IOUtils;
public class SystemInformationExporter implements IRunnableWithProgress {
private static final String TASK_NAME = "Exporting system information";
private static final String LBL_SYSTEM_INFO_EXPORT_TITLE = "lbl.system.info.export.title";
private static final char KEY_VALUE_SEPARATOR = '=';
private final String fileName;
private final Map> maps;
private final Map> iterables;
public SystemInformationExporter(final String fileName, final Map> maps, final Map> iterables) {
this.fileName = fileName;
this.maps = maps;
this.iterables = iterables;
}
@Override
public void run(final IProgressMonitor monitor) throws InvocationTargetException {
monitor.beginTask(TASK_NAME, maps.size() + iterables.size());
FileWriter fw = null;
BufferedWriter bw = null;
try {
fw = new FileWriter(fileName);
bw = new BufferedWriter(fw);
bw.write(JFaceMessages.get(LBL_SYSTEM_INFO_EXPORT_TITLE, DateFormat.getDateTimeInstance(DateFormat.FULL, DateFormat.FULL, new Locale(JFaceMessages.getLanguage())).format(new Date())));
bw.newLine();
for (final Entry> e1 : maps.entrySet()) {
bw.newLine();
bw.write(e1.getKey());
bw.newLine();
for (final Entry e2 : e1.getValue().entrySet()) {
bw.append(e2.getKey()).append(KEY_VALUE_SEPARATOR).append(e2.getValue());
bw.newLine();
}
monitor.worked(1);
}
for (final Entry> entry : iterables.entrySet()) {
bw.newLine();
bw.write(entry.getKey());
bw.newLine();
for (final String str : entry.getValue()) {
bw.write(str);
bw.newLine();
}
monitor.worked(1);
}
}
catch (final IOException e) {
throw new InvocationTargetException(e);
}
finally {
IOUtils.closeQuietly(bw, fw);
}
monitor.done();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy