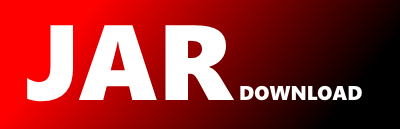
commonMain.ru.alexgladkov.odyssey.compose.animations.Transitions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odyssey-compose Show documentation
Show all versions of odyssey-compose Show documentation
Lightweight multiplatform navigation library (jvm, android, ios)
package ru.alexgladkov.odyssey.compose.animations
import androidx.compose.animation.*
import androidx.compose.animation.core.tween
/**
* Provide presentation transition
*
* @param T - transition target state
* @param isOpen - animation direction (true for forward, false for backward)
* @param transitionTime - animation duration
*/
@OptIn(ExperimentalAnimationApi::class)
fun providePresentationTransition(
isOpen: Boolean,
transitionTime: Int
): AnimatedContentScope.() -> ContentTransform = {
if (isOpen) {
// Forward animation
(slideInVertically(
animationSpec = tween(transitionTime),
initialOffsetY = { height -> height })
+ fadeIn(animationSpec = tween(transitionTime)) with
slideOutVertically(
animationSpec = tween(transitionTime),
targetOffsetY = { height -> -(height / 8) })
+ fadeOut(animationSpec = tween(transitionTime)))
.using(
SizeTransform(clip = false)
)
} else {
(slideInVertically(
animationSpec = tween(transitionTime),
initialOffsetY = { height -> -(height / 8) })
+ fadeIn(animationSpec = tween(transitionTime)) with
slideOutVertically(
animationSpec = tween(transitionTime), targetOffsetY = { height -> height })
+ fadeOut(animationSpec = tween(transitionTime))
)
.using(
SizeTransform(clip = false)
)
}
}
/**
* Provide push transition
*
* @param T - transition target state
* @param isForward - animation direction (true for forward, false for backward)
* @param transitionTime - animation duration
*/
@OptIn(ExperimentalAnimationApi::class)
fun providePushTransition(isForward: Boolean, transitionTime: Int): AnimatedContentScope.() -> ContentTransform =
{
if (isForward) {
// Forward animation
(slideInHorizontally(
animationSpec = tween(transitionTime),
initialOffsetX = { width -> width })
+ fadeIn(animationSpec = tween(transitionTime)) with
slideOutHorizontally(
animationSpec = tween(transitionTime),
targetOffsetX = { width -> -width })
+ fadeOut(animationSpec = tween(transitionTime)))
.using(
SizeTransform(clip = false)
)
} else {
(slideInHorizontally(animationSpec = tween(transitionTime), initialOffsetX = { width -> -width })
+ fadeIn(animationSpec = tween(transitionTime)) with
slideOutHorizontally(
animationSpec = tween(transitionTime), targetOffsetX = { width -> width })
+ fadeOut(animationSpec = tween(transitionTime))
)
.using(
SizeTransform(clip = false)
)
}
}
@OptIn(ExperimentalAnimationApi::class)
fun provideCrossFadeTransition(transitionTime: Int): AnimatedContentScope.() -> ContentTransform = {
(fadeIn(animationSpec = tween(transitionTime)) with fadeOut(animationSpec = tween(transitionTime)))
.using(SizeTransform(clip = false))
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy