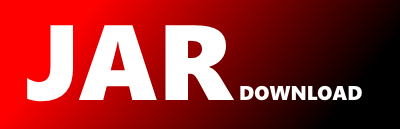
io.github.zhyshko.core.util.MethodUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of BotIO Show documentation
Show all versions of BotIO Show documentation
A framework for easy Telegram Bot creation
package io.github.zhyshko.core.util;
import io.github.zhyshko.core.annotation.*;
import io.github.zhyshko.core.router.Route;
import java.lang.reflect.Method;
import java.util.Map;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class MethodUtil {
public static Method getMethodForMessage(Route route, String message) {
Map methodMappings = Stream.of(route.getClass().getMethods())
.filter(m -> m.isAnnotationPresent(MessageMapping.class))
.collect(Collectors.toMap(m -> m.getAnnotation(MessageMapping.class).value(), Function.identity()));
Method defaultMethod = methodMappings.get("*");
Method methodToHandle = methodMappings.get(message);
if (methodToHandle == null && defaultMethod == null) {
throw new IllegalArgumentException("There is no suitable method mapping for route: " + route + " and message: " + message);
}
if (methodToHandle == null) {
methodToHandle = defaultMethod;
}
return methodToHandle;
}
public static Method getMethodForCallback(Route route, String callbackData) {
Map methodMappings = Stream.of(route.getClass().getMethods())
.filter(m -> m.isAnnotationPresent(CallbackMapping.class))
.collect(Collectors.toMap(m -> m.getAnnotation(CallbackMapping.class).value(), Function.identity()));
Method defaultMethod = methodMappings.get("*");
Method methodToHandle = methodMappings.get(callbackData);
if (methodToHandle == null && defaultMethod == null) {
throw new IllegalArgumentException("There is no suitable method mapping for route: " + route.getClass().getSimpleName() + " and message: " + callbackData);
}
if (methodToHandle == null) {
methodToHandle = defaultMethod;
}
return methodToHandle;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy