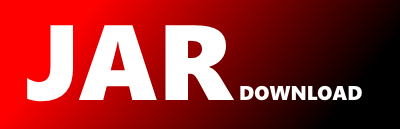
io.github.amayaframework.di.HashRepository Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of amaya-di Show documentation
Show all versions of amaya-di Show documentation
A framework responsible for monitoring and automating the dependency injection process.
package io.github.amayaframework.di;
import com.github.romanqed.jfunc.Function0;
import java.util.Collections;
import java.util.Map;
import java.util.Objects;
import java.util.concurrent.ConcurrentHashMap;
import java.util.function.Supplier;
/**
* A {@link Repository} implementation using a hash map.
*/
public class HashRepository implements Repository {
private final Map> body;
/**
* Constructs {@link HashRepository} instance with map instance, specified by the supplier.
*
* @param supplier the specified map supplier, must be non-null
*/
public HashRepository(Supplier
© 2015 - 2025 Weber Informatics LLC | Privacy Policy