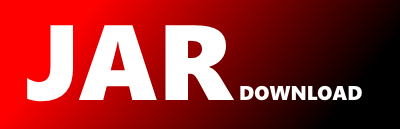
io.github.amayaframework.di.ManualProviderBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of amaya-di Show documentation
Show all versions of amaya-di Show documentation
A framework responsible for monitoring and automating the dependency injection process.
package io.github.amayaframework.di;
import com.github.romanqed.jfunc.Function0;
import com.github.romanqed.jfunc.Function1;
/**
* An interface describing an abstract {@link ServiceProvider} builder with
* the ability to manually build dependencies.
*/
public interface ManualProviderBuilder extends ServiceProviderBuilder {
/**
* Adds an artifact implementation provided by the specified function.
*
* IMPORTANT: the result of a function call is not checked,
* if a critical situation occurs during its operation, it will not be processed in any way
* (i.e., the function itself must control the absence of artifacts, cyclic dependencies, etc.).
*
* Use this function only if you know what you are doing!
*
* @param artifact the specified artifact, must be non-null
* @param function the specified function, must be non-null
* @return this {@link ManualProviderBuilder} instance
*/
ManualProviderBuilder addManual(Artifact artifact, Function1> function);
/**
* Adds a type implementation provided by the specified function.
*
* IMPORTANT: the result of a function call is not checked,
* if a critical situation occurs during its operation, it will not be processed in any way
* (i.e., the function itself must control the absence of artifacts, cyclic dependencies, etc.).
*
* Use this function only if you know what you are doing!
*
* @param type the specified type, must be non-null
* @param function the specified function, must be non-null
* @param the service type
* @return this {@link ManualProviderBuilder} instance
*/
ManualProviderBuilder addManual(Class type, Function1> function);
// Override parent methods to provide proper flow api
@Override
ManualProviderBuilder setRepository(Repository repository);
@Override
ManualProviderBuilder addService(Artifact artifact,
Class extends T> implementation,
Function1, Function0> wrapper);
@Override
ManualProviderBuilder addSingleton(Artifact artifact, Class> implementation);
@Override
ManualProviderBuilder addTransient(Artifact artifact, Class> implementation);
@Override
ManualProviderBuilder addService(Class type,
Class extends T> implementation,
Function1, Function0> wrapper);
@Override
ManualProviderBuilder addSingleton(Class type, Class extends T> implementation);
@Override
ManualProviderBuilder addTransient(Class type, Class extends T> implementation);
@Override
ManualProviderBuilder addService(Class type, Function1, Function0> wrapper);
@Override
ManualProviderBuilder addSingleton(Class> type);
@Override
ManualProviderBuilder addTransient(Class> type);
@Override
ManualProviderBuilder addService(Artifact artifact, Function0> supplier);
@Override
ManualProviderBuilder addService(Class type, Function0 supplier);
@Override
ManualProviderBuilder removeService(Artifact artifact);
@Override
ManualProviderBuilder removeService(Class> type);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy