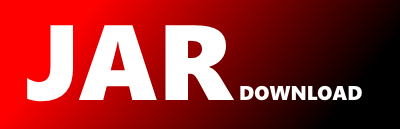
io.github.amayaframework.di.scheme.ClassScheme Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of amaya-di Show documentation
Show all versions of amaya-di Show documentation
A framework responsible for monitoring and automating the dependency injection process.
package io.github.amayaframework.di.scheme;
import io.github.amayaframework.di.Artifact;
import java.util.Collections;
import java.util.HashSet;
import java.util.Objects;
import java.util.Set;
/**
* A scheme that defines the correspondence between set of artifacts and class.
*/
public final class ClassScheme extends AbstractScheme> {
private final Set methodSchemes;
private final Set fieldSchemes;
private final ConstructorScheme constructorScheme;
private final Set artifacts;
/**
* Constructs class scheme for specified class and schemes for its members.
*
* @param clazz the specified class, must be non-null
* @param constructorScheme the constructor scheme, may be null
* @param fieldSchemes the set of field schemes, must be non-null
* @param methodSchemes the set of method schemes, must be non-null
*/
public ClassScheme(Class> clazz,
ConstructorScheme constructorScheme,
Set fieldSchemes,
Set methodSchemes) {
super(clazz);
this.constructorScheme = Objects.requireNonNull(constructorScheme);
this.fieldSchemes = Collections.unmodifiableSet(Objects.requireNonNull(fieldSchemes));
this.methodSchemes = Collections.unmodifiableSet(Objects.requireNonNull(methodSchemes));
this.artifacts = Collections.unmodifiableSet(collectArtifacts());
}
private Set collectArtifacts() {
var ret = new HashSet<>(constructorScheme.artifacts);
for (var scheme : fieldSchemes) {
ret.add(scheme.artifact);
}
for (var scheme : methodSchemes) {
ret.addAll(scheme.artifacts);
}
return ret;
}
/**
* Returns the constructor scheme for class constructor.
*
* @return the constructor scheme.
*/
public ConstructorScheme getConstructorScheme() {
return constructorScheme;
}
/**
* Returns the set of field schemes for class fields.
*
* @return the set of field schemes
*/
public Set getFieldSchemes() {
return fieldSchemes;
}
/**
* Returns the set of method schemes for class methods.
*
* @return the set of method schemes
*/
public Set getMethodSchemes() {
return methodSchemes;
}
/**
* Returns all artifacts that class members depend on.
*
* @return the set of artifacts
*/
@Override
public Set getArtifacts() {
return artifacts;
}
@Override
public String toString() {
return "ClassScheme{" +
"methodSchemes=" + methodSchemes +
", fieldSchemes=" + fieldSchemes +
", constructorScheme=" + constructorScheme +
", target=" + target +
'}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy