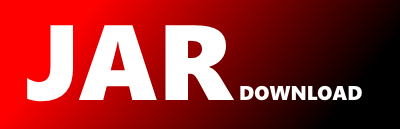
io.github.amayaframework.router.MachineRouterFactory Maven / Gradle / Ivy
package io.github.amayaframework.router;
import com.github.romanqed.jsm.StateMachine;
import com.github.romanqed.jsm.StateMachineFactory;
import com.github.romanqed.jsm.model.MachineModelBuilder;
import io.github.amayaframework.tokenize.Tokenizer;
import io.github.amayaframework.tokenize.Tokenizers;
import java.util.*;
/**
* Implementation of {@link RouterFactory} that uses state machines for dynamic routing.
*/
public final class MachineRouterFactory implements RouterFactory {
private static final String INITIAL_STATE = "I";
private static final String EXIT_STATE = "E";
private final StateMachineFactory factory;
private final Tokenizer tokenizer;
/**
* Constructs a {@link MachineRouterFactory} instance with given {@link StateMachineFactory} and {@link Tokenizer}.
*
* @param factory the specified {@link StateMachineFactory} instance, must be non-null
* @param tokenizer the specified {@link Tokenizer} instance, must be non-null
*/
public MachineRouterFactory(StateMachineFactory factory, Tokenizer tokenizer) {
this.factory = Objects.requireNonNull(factory);
this.tokenizer = Objects.requireNonNull(tokenizer);
}
/**
* Constructs a {@link MachineRouterFactory} instance with given {@link StateMachineFactory} and
* {@link io.github.amayaframework.tokenize.PlainTokenizer}.
*
* @param factory the specified {@link StateMachineFactory} instance, must be non-null
*/
public MachineRouterFactory(StateMachineFactory factory) {
this.factory = Objects.requireNonNull(factory);
this.tokenizer = Tokenizers.PLAIN_TOKENIZER;
}
private static void add(MachineModelBuilder
© 2015 - 2025 Weber Informatics LLC | Privacy Policy