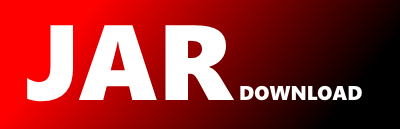
io.github.amayaframework.router.Path Maven / Gradle / Ivy
package io.github.amayaframework.router;
import java.util.List;
/**
* A class that represents a universal uri path descriptor.
*/
public final class Path {
final String path;
final List segments;
final boolean dynamic;
PathData data;
/**
* Constructs a {@link Path} instance with given normalized string representation, path segments and dynamic flag.
*
* @param path the specified normalized path representation, must be non-null
* @param segments the specified path segments, must be non-null
* @param dynamic the specified dynamic flag
*/
public Path(String path, List segments, boolean dynamic) {
this.path = path;
this.segments = segments;
this.dynamic = dynamic;
}
/**
* Gets normalized path representation.
*
* @return the normalized path representation
*/
public String getPath() {
return path;
}
/**
* Gets path segments.
*
* @return the {@link List} containing path segments
*/
public List getSegments() {
return segments;
}
/**
* Checks if this path is dynamic.
*
* @return true if this path is dynamic, false otherwise
*/
public boolean isDynamic() {
return dynamic;
}
/**
* Gets path data.
*
* @return the {@link PathData} instance
*/
public PathData getData() {
return data;
}
/**
* Sets path data.
*
* @param data the {@link PathData} instance
*/
public void setData(PathData data) {
this.data = data;
}
@Override
public boolean equals(Object object) {
if (this == object) return true;
if (object == null || getClass() != object.getClass()) return false;
var that = (Path) object;
return path.equals(that.path);
}
@Override
public int hashCode() {
return path.hashCode();
}
@Override
public String toString() {
return path;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy