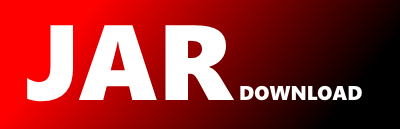
amk.sdk.deeplink.entity.model.AMKDLValidation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java Show documentation
Show all versions of java Show documentation
The AMK Deeplink SDK is a library for AMK's merchant that allow them to be able generate deeplink for their user to pay via AMK Bank app.
package amk.sdk.deeplink.entity.model;
import amk.sdk.deeplink.exception.DeeplinkException;
import amk.sdk.deeplink.utils.StringUtils;
import java.math.BigDecimal;
import java.util.regex.Pattern;
import static amk.sdk.deeplink.exception.DeeplinkException.throwCustomerException;
import static amk.sdk.deeplink.entity.model.AMKDLErrorCode.*;
import static amk.sdk.deeplink.utils.StringUtils.isBlank;
public class AMKDLValidation {
private static boolean isAmountInvalid(Double amount, AMKDLCurrency AMKDLCurrency) {
if (amount != null) {
BigDecimal bd = BigDecimal.valueOf(amount);
String valueAmount = bd.stripTrailingZeros().toPlainString();
int index = valueAmount.indexOf(".");
int decimalCount = index < 0 ? 0 : valueAmount.length() - index - 1;
int AMOUNT_LENGTH = 13;
if (amount < 0 || valueAmount.length() > AMOUNT_LENGTH) {
return true;
}
if (AMKDLCurrency == AMKDLCurrency.USD) {
//0.##
return decimalCount >= 3;
} else {
//0
return decimalCount != 0;
}
} else return false;
}
public static String getReceiverAccountId(String accountId) throws DeeplinkException {
int ACCOUNT_ID_LENGTH = 32;
String FORMAT_ACCOUNT_ID = "^[^@]+[@][^@]+$";
if (isBlank(accountId)) {
throwCustomerException(ACCOUNT_ID_REQUIRED);
} else if (accountId.length() > ACCOUNT_ID_LENGTH) {
throwCustomerException(ACCOUNT_ID_LENGTH_INVALID);
} else if (!Pattern.matches(FORMAT_ACCOUNT_ID, accountId)) {
throwCustomerException(ACCOUNT_ID_INVALID);
}
return accountId;
}
public static String getMerchantName(String merchantName) throws DeeplinkException {
int MERCHANT_NAME_LENGTH = 25;
if (isBlank(merchantName)) {
throwCustomerException(MERCHANT_NAME_REQUIRED);
} else if (merchantName.length() > MERCHANT_NAME_LENGTH) {
throwCustomerException(MERCHANT_NAME_LENGTH_INVALID);
}
return merchantName;
}
public static String getMerchantId(String merchantId) throws DeeplinkException {
int MERCHANT_ID_LENGTH = 25;
if (isBlank(merchantId)) {
throwCustomerException(MERCHANT_ID_REQUIRED);
} else if (merchantId.length() > MERCHANT_ID_LENGTH) {
throwCustomerException(MERCHANT_ID_LENGTH_INVALID);
}
return merchantId;
}
public static AMKDLCurrency getCurrencyType(AMKDLCurrency AMKDLCurrency) throws DeeplinkException {
if (AMKDLCurrency == null) {
throwCustomerException(CURRENCY_TYPE_REQUIRED);
}
return AMKDLCurrency;
}
public static Double getAmount(Double amount, AMKDLCurrency AMKDLCurrency) throws DeeplinkException {
if (isAmountInvalid(amount, AMKDLCurrency)) {
throwCustomerException(AMOUNT_INVALID);
}
return amount;
}
public static String getMobileNumber(String mobileNumber) throws DeeplinkException {
int MOBILE_NUMBER_LENGTH = 12;
if (mobileNumber != null && mobileNumber.length() > MOBILE_NUMBER_LENGTH) {
throwCustomerException(MOBILE_NUMBER_LENGTH_INVALID);
}
return mobileNumber;
}
public static String getBillNumber(String billNumber) throws DeeplinkException {
int BILL_NUMBER_LENGTH = 25;
if (billNumber != null && billNumber.length() > BILL_NUMBER_LENGTH) {
throwCustomerException(AMKDLErrorCode.BILL_NUMBER_LENGTH_INVALID);
}
return billNumber;
}
public static String getStoreLabel(String storeLabel) throws DeeplinkException {
int STORE_LABEL_LENGTH = 25;
if (storeLabel != null && storeLabel.length() > STORE_LABEL_LENGTH) {
throwCustomerException(STORE_LABEL_LENGTH_INVALID);
}
return storeLabel;
}
public static String getTerminalLabel(String terminalLabel) throws DeeplinkException {
int TERMINAL_LABEL_LENGTH = 25;
if (terminalLabel != null && terminalLabel.length() > TERMINAL_LABEL_LENGTH) {
throwCustomerException(TERMINAL_LABEL_LENGTH_INVALID);
}
return terminalLabel;
}
public static SourceInfo getSourceInfo(SourceInfo SourceInfo) throws DeeplinkException {
if (SourceInfo != null){
String appName = SourceInfo.getAppName();
String appDeepLinkCallback = SourceInfo.getAppDeeplinkCallback();
String appIconUrl = SourceInfo.getAppIconUrl();
if (isBlank(appName) || isBlank(appDeepLinkCallback) || isBlank(appIconUrl))
throwCustomerException(SOURCE_INFO_INVALID);
}
return SourceInfo;
}
public static String getAccountInformation(String accountInformation) throws DeeplinkException {
int ACCOUNT_INFORMATION_LENGTH = 32;
if (accountInformation != null && accountInformation.length() > ACCOUNT_INFORMATION_LENGTH) {
throwCustomerException(ACCOUNT_INFORMATION_LENGTH_INVALID);
}
return accountInformation;
}
public static String getReferenceId(String referenceId, String id) {
if (isBlank(referenceId)){
return Md5Config.getDefaultHash(id);
} else return referenceId;
}
public static String getAcquiringBankForMerchant(String acquiringBank) throws DeeplinkException {
int ACQUIRING_BANK_LENGTH = 32;
if (StringUtils.isBlank(acquiringBank)) {
throwCustomerException(ACQUIRING_BANK_REQUIRED);
} else if (acquiringBank.length() > ACQUIRING_BANK_LENGTH) {
throwCustomerException(ACQUIRING_BANK_LENGTH_INVALID);
}
return acquiringBank;
}
public static String getAcquiringBankForIndividual(String acquiringBank) throws DeeplinkException {
int ACQUIRING_BANK_LENGTH = 32;
if (acquiringBank != null && acquiringBank.length() > ACQUIRING_BANK_LENGTH) {
throwCustomerException(ACQUIRING_BANK_LENGTH_INVALID);
}
return acquiringBank;
}
public static String getMerchantCity(String merchantCity) throws DeeplinkException {
int MERCHANT_CITY_LENGTH = 15;
if (merchantCity.length() > MERCHANT_CITY_LENGTH) {
throwCustomerException(CITY_LENGTH_INVALID);
}
return merchantCity;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy