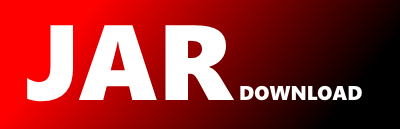
amk.sdk.deeplink.presenter.DeeplinkPresenter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java Show documentation
Show all versions of java Show documentation
The AMK Deeplink SDK is a library for AMK's merchant that allow them to be able generate deeplink for their user to pay via AMK Bank app.
package amk.sdk.deeplink.presenter;
import amk.sdk.deeplink.entity.model.*;
import amk.sdk.deeplink.exception.DeeplinkException;
import amk.sdk.deeplink.entity.request.IndividualRequest;
import amk.sdk.deeplink.entity.request.MerchantRequest;
import amk.sdk.deeplink.entity.response.AMKDLApiResponse;
import amk.sdk.deeplink.entity.response.AMKDLResponse;
import amk.sdk.deeplink.utils.StringUtils;
import com.google.gson.Gson;
import okhttp3.*;
import java.net.ConnectException;
import java.net.SocketTimeoutException;
import java.net.UnknownHostException;
import static amk.sdk.deeplink.exception.DeeplinkException.throwCustomerException;
import static amk.sdk.deeplink.entity.model.ApiConstant.BASE_URL;
public class DeeplinkPresenter {
private static final String SUCCESS_CODE = "00";
private static String mClientId;
private static String mPrivateKeyPath;
private static String mJwtAudience;
private static String referenceId;
public static void preConfig(String clientId, String privateKeyPath, String jwtAudience) {
mClientId = clientId;
mPrivateKeyPath = privateKeyPath;
mJwtAudience = jwtAudience;
}
public static AMKDLResponse generateIndividualDeeplink(AMKDLIndividual amkdlIndividual) throws DeeplinkException {
return okHttpClientGenerator(amkdlIndividual, true);
}
public static AMKDLResponse generateMerchantDeeplink(AMKDLMerchant amkdlMerchant) throws DeeplinkException {
return okHttpClientGenerator(amkdlMerchant, false);
}
private static AMKDLResponse okHttpClientGenerator(T request, boolean isIndividual) throws DeeplinkException {
String endpoint;
if (isIndividual) {
endpoint = "/deep-link/generate/individual";
} else endpoint = "/deep-link/generate/merchant";
String accessToken = null;
try {
accessToken = AuthenticationPresenter.getAccessToken(mClientId, mPrivateKeyPath, mJwtAudience);
} catch (Exception e) {
handleException(e);
}
if (accessToken == null) throwCustomerException(AMKDLErrorCode.WRONG_CONFIG);
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
String requestBody;
Gson gson = new Gson();
if (request instanceof AMKDLIndividual) {
requestBody = gson.toJson(getIndividualRequest((AMKDLIndividual) request));
} else {
requestBody = gson.toJson(getMerchantRequest((AMKDLMerchant) request));
}
MediaType mediaType = MediaType.parse("application/json");
RequestBody body = RequestBody.create(mediaType, requestBody);
Request apiRequest = new Request.Builder()
.url(BASE_URL + endpoint)
.method("POST", body)
.addHeader("Authorization", "Bearer " + accessToken)
.build();
try {
Response response = client.newCall(apiRequest).execute();
return convertToResponseBody(response);
} catch (Exception e) {
if (e instanceof ConnectException || e instanceof SocketTimeoutException || e instanceof UnknownHostException) {
throwCustomerException(AMKDLErrorCode.NO_INTERNET_CONNECTION);
} else {
//default error
throwCustomerException(AMKDLErrorCode.SOMETHING_WENT_WRONG);
}
}
return null;
}
private static void handleException(Exception e) throws DeeplinkException {
if (e instanceof DeeplinkException) {
throwCustomerException(((DeeplinkException) e).getErrorCode());
} else {
throwCustomerException(AMKDLErrorCode.SOMETHING_WENT_WRONG);
}
}
private static AMKDLResponse convertToResponseBody(Response response) throws Exception {
Gson gson = new Gson();
AMKDLApiResponse baseResponse;
ResponseBody responseBody = response.body();
if (responseBody != null) {
String json = responseBody.string();
baseResponse = gson.fromJson(
json,
AMKDLApiResponse.class
);
return responseParser(baseResponse);
}
return null;
}
private static AMKDLResponse responseParser(AMKDLApiResponse response) {
AMKDLResponse baseResponse;
switch (response.getResponseCode()) {
case SUCCESS_CODE: {
baseResponse = responseMapper(response);
break;
}
// other cases
default: {
baseResponse = responseMapper(response);
}
}
return baseResponse;
}
private static AMKDLResponse responseMapper(AMKDLApiResponse response) {
AMKDLResponse baseResponse = new AMKDLResponse<>();
if (response != null) {
AMKDLStatus amkdlStatus = new AMKDLStatus();
amkdlStatus.setCode(0);
amkdlStatus.setErrorCode(Integer.parseInt(response.getResponseCode()));
amkdlStatus.setMessage(response.getMessage());
baseResponse.setStatus(amkdlStatus);
if (response.getResult() != null && StringUtils.isNoneBlank(response.getResult().getShortLink())) {
AMKDeeplinkData amkDeeplinkData = new AMKDeeplinkData();
amkDeeplinkData.setShortLink(response.getResult().getShortLink());
amkDeeplinkData.setReferenceId(referenceId);
baseResponse.setData(amkDeeplinkData);
}
}
return baseResponse;
}
private static MerchantRequest getMerchantRequest(AMKDLMerchant amkdlMerchant) {
referenceId = amkdlMerchant.getReferenceId();
return new MerchantRequest(
"default@amkb",
amkdlMerchant.getReferenceId(),
amkdlMerchant.getMerchantId(),
amkdlMerchant.getAcquiringBank(),
amkdlMerchant.getCurrency().toString(),
amkdlMerchant.getAmount(),
amkdlMerchant.getMerchantName(),
amkdlMerchant.getMerchantCity(),
amkdlMerchant.getBillNumber(),
amkdlMerchant.getMobileNumber(),
amkdlMerchant.getStoreLabel(),
amkdlMerchant.getTerminalLabel(),
amkdlMerchant.getSourceInfo()
);
}
private static IndividualRequest getIndividualRequest(AMKDLIndividual amkdlIndividual) {
referenceId = amkdlIndividual.getReferenceId();
return new IndividualRequest(
amkdlIndividual.getAccountId(),
amkdlIndividual.getReferenceId(),
amkdlIndividual.getAccountInformation(),
amkdlIndividual.getAcquiringBank(),
amkdlIndividual.getCurrency().toString(),
amkdlIndividual.getAmount(),
amkdlIndividual.getUsername(),
amkdlIndividual.getCity(),
amkdlIndividual.getBillNumber(),
amkdlIndividual.getMobileNumber(),
amkdlIndividual.getStoreLabel(),
amkdlIndividual.getTerminalLabel()
);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy