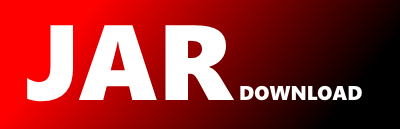
amk.sdk.deeplink.presenter.JwtTokenConfigPresenter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java Show documentation
Show all versions of java Show documentation
The AMK Deeplink SDK is a library for AMK's merchant that allow them to be able generate deeplink for their user to pay via AMK Bank app.
package amk.sdk.deeplink.presenter;
import amk.sdk.deeplink.entity.model.AMKDLErrorCode;
import io.jsonwebtoken.Jwts;
import io.jsonwebtoken.SignatureAlgorithm;
import java.io.File;
import java.nio.charset.StandardCharsets;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.security.*;
import java.security.spec.EncodedKeySpec;
import java.security.spec.PKCS8EncodedKeySpec;
import java.util.Base64;
import java.util.Date;
import static amk.sdk.deeplink.exception.DeeplinkException.throwCustomerException;
public class JwtTokenConfigPresenter {
private static final long JWT_TOKEN_VALIDITY = 300000;
public static String generateJwtToken(String clientId, String privateKeyPath, String jwtAudience) throws Exception {
long nowMillis = System.currentTimeMillis();
Date now = new Date(nowMillis);
long expired = nowMillis + JWT_TOKEN_VALIDITY;
Date exp = new Date(expired);
return Jwts.builder()
.setIssuer(clientId)
.setAudience(jwtAudience)
.setIssuedAt(now)
.setExpiration(exp)
// RS256 with privateKey
.signWith(SignatureAlgorithm.RS256, getPrivateKey(privateKeyPath))
.compact();
}
private static PrivateKey getPrivateKey(String fileName) throws Exception {
File file = new File(fileName);
if (!file.exists()) throwCustomerException(AMKDLErrorCode.FILE_NOT_EXIST);
String privateKeyString = new String(Files.readAllBytes(Paths.get(fileName)));
String replacePrivateKeyString = privateKeyString
.replaceAll(System.lineSeparator(), "")
.replace("-----BEGIN RSA PRIVATE KEY-----", "")
.replace("-----END RSA PRIVATE KEY-----", "")
.replace("-----BEGIN PRIVATE KEY-----", "")
.replace("-----END PRIVATE KEY-----", "");
KeyFactory fact = KeyFactory.getInstance("RSA");
byte[] bytes = Base64.getDecoder().decode(replacePrivateKeyString.getBytes(StandardCharsets.UTF_8));
EncodedKeySpec keySpec = new PKCS8EncodedKeySpec(bytes);
return fact.generatePrivate(keySpec);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy