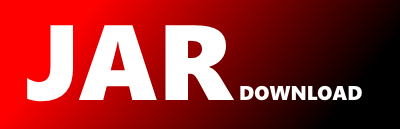
javastrava.api.v3.rest.util.RetrofitClientResponseInterceptor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of javastrava-api Show documentation
Show all versions of javastrava-api Show documentation
Java implementation of the Strava API
package javastrava.api.v3.rest.util;
import java.io.IOException;
import java.util.StringTokenizer;
import com.squareup.okhttp.OkHttpClient;
import javastrava.api.v3.service.impl.StravaServiceImpl;
import javastrava.config.StravaConfig;
import retrofit.client.Header;
import retrofit.client.OkClient;
import retrofit.client.Request;
import retrofit.client.Response;
/**
*
* Overrides the OkHttp client in order to intercept the rate limit data returned by the API in headers
*
*
* @author Dan Shannon
*
*/
public class RetrofitClientResponseInterceptor extends OkClient {
/**
* No-args constructor
*/
public RetrofitClientResponseInterceptor() {
super();
}
/**
* @param client The client to use
*/
public RetrofitClientResponseInterceptor(final OkHttpClient client) {
super(client);
}
/**
*
* Gets and stores the values of the rate limit information headers returned by Strava with each response
*
*
* @see retrofit.client.OkClient#execute(retrofit.client.Request)
*/
@Override
public Response execute(final Request request) throws IOException {
Response response = super.execute(request);
for (Header header : response.getHeaders()) {
if (header.getName().equals(StravaConfig.string("strava.rate-limit-usage-header-name"))) { //$NON-NLS-1$
String values = header.getValue();
StringTokenizer tokenizer = new StringTokenizer(values, ","); //$NON-NLS-1$
StravaServiceImpl.requestRate = Integer.valueOf(tokenizer.nextToken()).intValue();
StravaServiceImpl.requestRateDaily = Integer.valueOf(tokenizer.nextToken()).intValue();
StravaServiceImpl.requestRatePercentage();
}
if (header.getName().equals(StravaConfig.string("strava.rate-limit-limit-header-name"))) { //$NON-NLS-1$
String values = header.getValue();
StringTokenizer tokenizer = new StringTokenizer(values, ","); //$NON-NLS-1$
StravaConfig.RATE_LIMIT = Integer.valueOf(tokenizer.nextToken()).intValue();
StravaConfig.RATE_LIMIT_DAILY = Integer.valueOf(tokenizer.nextToken()).intValue();
StravaServiceImpl.requestRateDailyPercentage();
}
}
return response;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy