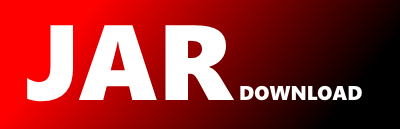
javastrava.util.PagingForkJoinTask Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of javastrava-api Show documentation
Show all versions of javastrava-api Show documentation
Java implementation of the Strava API
package javastrava.util;
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.RecursiveTask;
import javastrava.api.v3.service.exception.BadRequestException;
import javastrava.api.v3.service.exception.NotFoundException;
/**
* @author Dan Shannon
*
* @param Class of objects which will be returned in a list
*/
public class PagingForkJoinTask extends RecursiveTask> {
/**
* Default
*/
private static final long serialVersionUID = 1L;
/**
* Callback which returns a page of data from the Strava API
*/
private final PagingCallback callback;
/**
* List of pages to get from the Strava API
*/
private final List pages;
/**
* @param callback The callback which will be used to get a page of data from the Strava API
* @param pages The list of paging instructions
*/
public PagingForkJoinTask(final PagingCallback callback, final List pages) {
this.callback = callback;
this.pages = pages;
}
/**
* @see java.util.concurrent.RecursiveTask#compute()
*/
@Override
protected List compute() {
if (this.pages.size() == 0) {
return null;
}
if (this.pages.size() == 1) {
try {
final Paging pagingInstruction = this.pages.get(0);
List pageOfData = this.callback.getPageOfData(pagingInstruction);
pageOfData = PagingUtils.ignoreLastN(pageOfData, pagingInstruction.getIgnoreLastN());
pageOfData = PagingUtils.ignoreFirstN(pageOfData, pagingInstruction.getIgnoreFirstN());
return pageOfData;
} catch (final NotFoundException e) {
return null;
} catch (final BadRequestException e) {
return null;
}
}
final int middle = this.pages.size() / 2;
final List leftPages = this.pages.subList(0, middle);
final PagingForkJoinTask leftTask = new PagingForkJoinTask(this.callback, leftPages);
leftTask.fork();
final List rightPages = this.pages.subList(middle, this.pages.size());
final PagingForkJoinTask rightTask = new PagingForkJoinTask(this.callback, rightPages);
final List rightResult = rightTask.compute();
final List leftResult = leftTask.join();
final List result = new ArrayList<>();
if ((leftResult == null) && (rightResult == null)) {
return null;
}
if (leftResult != null) {
result.addAll(leftResult);
}
if (rightResult != null) {
result.addAll(rightResult);
}
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy