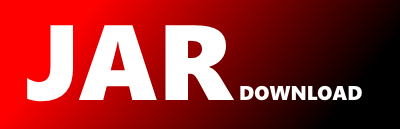
com.wm.df.sdk.component.AbstractComponent Maven / Gradle / Ivy
package com.wm.df.sdk.component;
import com.wm.df.sdk.component.config.*;
import com.wm.df.sdk.component.yaml.ComponentYamlReader;
import java.io.IOException;
import java.io.InputStream;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.logging.Level;
import java.util.logging.Logger;
/**
* 数据融合平台抽象组件
*
* @author wangjinnan
*/
public abstract class AbstractComponent implements IComponent {
public static final Logger logger = Logger.getLogger(AbstractComponent.class.getName());
/**
* 配置文件名称
*/
private static final String CONFIG_FILE_NAME = "application";
/**
* 组件参数配置
*/
private ComponentParameterConfig parameterConfig;
/**
* 组件输出配置
*/
private ComponentOutputConfig outputConfig;
/**
* 组件动态输出配置
*/
private ComponentOutputConfig dynamicOutputConfig;
/**
* 映射配置
*/
private ComponentMappingConfig mappingConfig;
public AbstractComponent() {
this.parameterConfig = new ComponentParameterConfig();
this.outputConfig = new ComponentOutputConfig();
this.dynamicOutputConfig = new ComponentOutputConfig();
this.mappingConfig = new ComponentMappingConfig();
}
/**
* 获取抽象组件参数配置.
*
* @return 参数配置对象.
*/
@Override
public final ComponentParameterConfig parameterConfig() {
return this.parameterConfig;
}
/**
* 获取组件结构化输出配置.
*
* @return 结构化输出配置对象.
*/
@Override
public final ComponentOutputConfig outputConfig() {
return this.outputConfig;
}
/**
* 获取组件动态输出配置.
*
* @return 结构化输出配置对象.
*/
@Override
public final ComponentOutputConfig dynamicOutputConfig() {
return this.dynamicOutputConfig;
}
/**
* 添加单个连接信息相关参数配置.
*
* @param item 单个参数配置对象.
*/
public final void addConnectionParameterConfigItem(ParameterConfigItem item) {
this.parameterConfig.addConnectionItem(item);
}
/**
* 添加单个高级信息相关参数配置.
*
* @param item 单个参数配置对象.
*/
public final void addAdvanceParameterConfigItem(ParameterConfigItem item) {
this.parameterConfig.addAdvanceItem(item);
}
/**
* 添加单个基础信息相关参数配置.
*
* @param item 单个参数配置对象.
*/
public final void addBaseParameterConfigItem(ParameterConfigItem item) {
this.parameterConfig.addBaseItem(item);
}
/**
* 通过参数key设置参数值.
*
* @param key 参数配置key.
* @param value 参数值.
*/
public final void setParameterValue(String key, String value) {
this.parameterConfig.setParameterValue(key, value);
}
/**
* 获取字符串类型参数值
*
* @param key 参数KEY
* @return 参数值,没有配置会返回null
*/
public final String getStringParameterValue(String key) {
return this.parameterConfig.getStringParameterValue(key);
}
/**
* 获取字符串类型参数值,没有设置则返回默认值.
*
* @param key 参数KEY
* @param defaultValue 参数默认值
* @return 参数值,没有配置会返回参数defaultValue
*/
public final String getStringParameterValueOrDefault(String key, String defaultValue) {
return this.parameterConfig.getStringParameterValueOrDefault(key, defaultValue);
}
/**
* 获取整数类型参数值
*
* @param key 参数KEY
* @return 参数值,没有配置会返回null
*/
public final Integer getIntParameterValue(String key) {
return this.parameterConfig.getIntParameterValue(key);
}
/**
* 获取整数类型参数值,没有设置则返回默认值.
*
* @param key 参数KEY
* @param defaultValue 参数默认值
* @return 参数值,没有配置会返回参数defaultValue
*/
public final Integer getIntParameterValueOrDefault(String key, Integer defaultValue) {
return this.parameterConfig.getIntParameterValueOrDefault(key, defaultValue);
}
/**
* 获取长整数类型参数值
*
* @param key 参数KEY
* @return 参数值,没有配置会返回null
*/
public final Long getLongParameterValue(String key) {
return this.parameterConfig.getLongParameterValue(key);
}
/**
* 获取长整数类型参数值,没有设置则返回默认值.
*
* @param key 参数KEY
* @param defaultValue 参数默认值
* @return 参数值,没有配置会返回参数defaultValue
*/
public final Long getLongParameterValueOrDefault(String key, Long defaultValue) {
return this.parameterConfig.getLongParameterValueOrDefault(key, defaultValue);
}
/**
* 获取浮点类型参数值
*
* @param key 参数KEY
* @return 参数值,没有配置会返回null
*/
public final Float getFloatParameterValue(String key) {
return this.parameterConfig.getFloatParameterValue(key);
}
/**
* 获取浮点类型参数值,没有设置则返回默认值.
*
* @param key 参数KEY
* @param defaultValue 参数默认值
* @return 参数值,没有配置会返回参数defaultValue
*/
public final Float getFloatParameterValueOrDefault(String key, Float defaultValue) {
return this.parameterConfig.getFloatParameterValueOrDefault(key, defaultValue);
}
/**
* 获取double类型参数值.
*
* @param key 参数KEY
* @return 参数值,没有配置会返回null
*/
public final Double getDoubleParameterValue(String key) {
return this.parameterConfig.getDoubleParameterValue(key);
}
/**
* 获取double类型参数值,没有设置则返回默认值.
*
* @param key 参数KEY
* @param defaultValue 参数默认值
* @return 参数值,没有配置会返回参数defaultValue
*/
public final Double getDoubleParameterValueOrDefault(String key, Double defaultValue) {
return this.parameterConfig.getDoubleParameterValueOrDefault(key, defaultValue);
}
/**
* 获取LIST类型参数值.
*
* @param key 参数配置key.
* @param clazz 参数对象.
* @param 参数对象泛型.
* @return 参数值.
*/
@SuppressWarnings("unchecked")
public List getListValue(final String key, Class clazz) {
return this.parameterConfig.getListValue(key, clazz);
}
/**
* 添加单个输出配置,
*
* @param outputConfigItem 单个输出配置对象.
*/
public final void addOutputConfigItem(OutputConfigItem outputConfigItem) {
this.outputConfig.addItem(outputConfigItem);
}
/**
* 添加输出配置集合,用于一次添加多个输出配置的场景.
*
* @param itemCollection 输出配置集合.
*/
public final void addOutputConfigItem(Collection itemCollection) {
if (Objects.nonNull(itemCollection)) {
for (OutputConfigItem configItem : itemCollection) {
this.outputConfig.addItem(configItem);
}
}
}
/**
* 添加单个动态输出配置,
*
* @param outputConfigItem 单个输出配置对象.
*/
public final void addDynamicOutputConfigItem(OutputConfigItem outputConfigItem) {
this.dynamicOutputConfig.addItem(outputConfigItem);
}
/**
* 添加动态输出配置集合,用于一次添加多个输出配置的场景.
*
* @param itemCollection 输出配置集合.
*/
public final void addDynamicOutputConfigItem(Collection itemCollection) {
if (Objects.nonNull(itemCollection)) {
for (OutputConfigItem configItem : itemCollection) {
this.dynamicOutputConfig.addItem(configItem);
}
}
}
/**
* 追加key是null的组件输出数据,追加多个会产生多行输出.
*
* @param data 组件输出的数据
*/
public final void appendOutputData(Object data) {
this.appendOutputData(null, data);
}
/**
* 追加格式化输出对象
*
* @param key 组件格式化输出的key
* @param data 组件格式化输出对象值
*/
public final void appendOutputData(String key, Object data) {
this.outputConfig.appendOutputData(key, data);
}
/**
* 获取组件所有结构化输出数据.
*
* @return 结构化输出数据集合.
*/
public final List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy