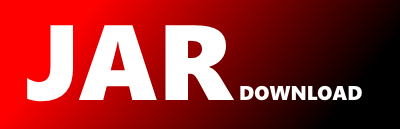
io.github.astrapi69.collections.map.MapFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of silly-collections Show documentation
Show all versions of silly-collections Show documentation
Utility library for collections, comparators and iterator classes
/**
* The MIT License
*
* Copyright (C) 2015 Asterios Raptis
*
* Permission is hereby granted, free of charge, to any person obtaining
* a copy of this software and associated documentation files (the
* "Software"), to deal in the Software without restriction, including
* without limitation the rights to use, copy, modify, merge, publish,
* distribute, sublicense, and/or sell copies of the Software, and to
* permit persons to whom the Software is furnished to do so, subject to
* the following conditions:
*
* The above copyright notice and this permission notice shall be
* included in all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
*/
package io.github.astrapi69.collections.map;
import java.util.Collection;
import java.util.Comparator;
import java.util.HashMap;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.Objects;
import java.util.TreeMap;
import java.util.concurrent.ConcurrentHashMap;
import org.apache.commons.collections4.functors.InstantiateFactory;
import org.apache.commons.collections4.map.LazyMap;
import io.github.astrapi69.check.Argument;
import io.github.astrapi69.collections.list.ListFactory;
import io.github.astrapi69.collections.pairs.KeyValuePair;
/**
* The factory class {@link MapFactory} provides factory methods for create new {@link Map} objects
*
* @version 1.0
* @author Asterios Raptis
*/
public final class MapFactory
{
private MapFactory()
{
}
/**
* Factory method for {@link java.util.Map} that acts like a javascript associative array.
*
* This Map is the counterpart for the associative array in js.
*
* For instance: in js you can create and fetch associative arrays like this:
*
*
* $arrayObj[0]['firstName'] = 'Albert';
* $arrayObj[0]['lastName'] = 'Einstein';
* $arrayObj[1]['firstName'] = 'Neil';
* $arrayObj[0]['lastName'] = 'Armstrong';
*
*
* With this method you can do the same like this:
*
*
* final Map<Integer, Map<String, String>> arrayMap = MapExtensions.newAssosiativeArrayMap();
*
* arrayMap.get(0).put("firstName", "Albert");
* arrayMap.get(0).put("lastName", "Einstein");
* arrayMap.get(1).put("firstName", "Neil");
* arrayMap.get(1).put("lastName", "Armstrong");
*
*
* @return The {@link java.util.Map} that acts like a javascript associative array
*/
public static Map> newAssosiativeArrayMap()
{
return newLazyMap();
}
/**
* Factory method for create a new {@link ConcurrentHashMap}
*
* @param
* the generic type of the key
* @param
* the generic type of the value
*
* @return The new {@link ConcurrentHashMap}
*/
public static ConcurrentHashMap newConcurrentHashMap()
{
return new ConcurrentHashMap<>();
}
/**
* Factory method for create a new {@link ConcurrentHashMap}
*
* @param
* the generic type of the key
* @param
* the generic type of the value
* @param initialCapacity
* the initial capacity
* @return The new {@link ConcurrentHashMap}
*/
public static ConcurrentHashMap newConcurrentHashMap(final int initialCapacity)
{
return new ConcurrentHashMap<>(initialCapacity);
}
/**
* Factory method for create a new {@link ConcurrentHashMap}
*
* @param
* the generic type of the key
* @param
* the generic type of the value
* @param map
* the map
* @return The new {@link ConcurrentHashMap}
*/
public static ConcurrentHashMap newConcurrentHashMap(final Map map)
{
Argument.notNull(map, "map");
return new ConcurrentHashMap<>(map);
}
/**
* Factory method for create a map for counting elements of the given collection
*
* @param
* the generic type of the elements
* @param elements
* the elements
* @return the new map ready to count elements
*/
public static Map newCounterMap(final Collection elements)
{
return newCounterMap(elements, true);
}
/**
* Factory method for create a map for counting elements of the given collection
*
* @param
* the generic type of the elements
* @param elements
* the elements
* @param startByZero
* if this flag is true the first element will start with 0 count
* @return the new map ready to count elements
*/
public static Map newCounterMap(final Collection elements,
boolean startByZero)
{
Objects.requireNonNull(elements);
return newCounterMap(MapFactory.newHashMap(), elements, startByZero);
}
/**
* Factory method for create a map for and count elements of the given collection
*
* @param
* the generic type of the elements
* @param counterMap
* the counter Map
* @param elements
* the elements
* @return the new map ready to count elements
*/
public static Map newCounterMap(Map counterMap,
Collection elements)
{
return newCounterMap(counterMap, elements, true);
}
/**
* Factory method for create a map for and count elements of the given collection
*
* @param
* the generic type of the elements
* @param counterMap
* the counter Map
* @param elements
* the elements
* @param startByZero
* if this flag is true the first element will start with 0 count
* @return the new map ready to count elements
*/
public static Map newCounterMap(Map counterMap,
Collection elements, boolean startByZero)
{
Objects.requireNonNull(counterMap);
for (K element : elements)
{
if (counterMap.containsKey(element))
{
counterMap.merge(element, 1, Integer::sum);
continue;
}
if (startByZero)
{
counterMap.put(element, 0);
}
else
{
counterMap.put(element, 1);
}
}
return counterMap;
}
/**
* Factory method for create a new {@link HashMap}
*
* @param
* the generic type of the key
* @param
* the generic type of the value
*
* @return The new {@link HashMap}
*/
public static Map newHashMap()
{
return new HashMap<>();
}
/**
* Factory method for create a new {@link HashMap}
*
* @param
* the generic type of the key
* @param
* the generic type of the value
* @param keyValuePairs
* the collection with the key value pairs
* @return The new {@link HashMap}
*/
public static Map newHashMap(final Collection> keyValuePairs)
{
Argument.notNull(keyValuePairs, "keyValuePairs");
return newHashMap(KeyValuePair.toMap(keyValuePairs));
}
/**
* Factory method for create a new {@link HashMap}
*
* @param
* the generic type of the key
* @param
* the generic type of the value
* @param initialCapacity
* the initial capacity
* @return The new {@link HashMap}
*/
public static Map newHashMap(final int initialCapacity)
{
return new HashMap<>(initialCapacity);
}
/**
* Factory method for create a new {@link HashMap}
*
* @param
* the generic type of the key
* @param
* the generic type of the value
* @param keyValuePairs
* the key value pairs to add in the new {@link HashMap}
* @return The new {@link HashMap}
*/
@SafeVarargs
public static Map newHashMap(final KeyValuePair... keyValuePairs)
{
Argument.notNull(keyValuePairs, "keyValuePairs");
return newHashMap(ListFactory.newArrayList(keyValuePairs));
}
/**
* Factory method for create a new {@link HashMap}
*
* @param
* the generic type of the key
* @param
* the generic type of the value
* @param map
* the map
* @return The new {@link HashMap}
*/
public static Map newHashMap(final Map map)
{
Argument.notNull(map, "map");
return new HashMap<>(map);
}
/**
* Factory method for create a new {@link InsertionOrderMap}
*
* @param
* the generic type of the key
* @param
* the generic type of the value
*
* @return The new {@link InsertionOrderMap}
*/
public static Map newInsertionOrderMap()
{
return new InsertionOrderMap<>();
}
/**
* Factory method for create a new {@link InsertionOrderMap}
*
* @param
* the generic type of the key
* @param
* the generic type of the value
* @param keyValuePairs
* the collection with the key value pairs
* @return The new {@link InsertionOrderMap}
*/
public static Map newInsertionOrderMap(
final Collection> keyValuePairs)
{
Argument.notNull(keyValuePairs, "keyValuePairs");
return newInsertionOrderMap(KeyValuePair.toMap(keyValuePairs));
}
/**
* Factory method for create a new {@link InsertionOrderMap}
*
* @param
* the generic type of the key
* @param
* the generic type of the value
* @param initialCapacity
* the initial capacity
*
* @return The new {@link InsertionOrderMap}
*/
public static Map newInsertionOrderMap(final int initialCapacity)
{
return new InsertionOrderMap<>(initialCapacity);
}
/**
* Factory method for create a new {@link InsertionOrderMap}
*
* @param
* the generic type of the key
* @param
* the generic type of the value
* @param keyValuePairs
* the key value pairs to add in the new {@link InsertionOrderMap}
* @return The new {@link InsertionOrderMap}
*/
@SafeVarargs
public static Map newInsertionOrderMap(final KeyValuePair... keyValuePairs)
{
Argument.notNull(keyValuePairs, "keyValuePairs");
return newInsertionOrderMap(ListFactory.newArrayList(keyValuePairs));
}
/**
* Factory method for create a new {@link InsertionOrderMap}
*
* @param
* the generic type of the key
* @param
* the generic type of the value
* @param map
* the map
* @return The new {@link InsertionOrderMap}
*/
public static Map newInsertionOrderMap(final Map map)
{
Argument.notNull(map, "map");
return new InsertionOrderMap<>(map);
}
/**
* Factory method for create a new {@link LazyMap} from commons-collections4 that encapsulates a
* {@link HashMap}
*
* @param
* the generic type of the key
* @param
* the generic type of the value
*
* @return The new {@link LazyMap}
*/
public static Map newLazyHashMap()
{
return newLazyHashMap(new HashMap<>());
}
/**
* Factory method for create a new {@link LazyMap} from commons-collections4 that encapsulates a
* {@link HashMap}
*
* @param
* the generic type of the key
* @param
* the generic type of the value
* @param map
* the map to initialize with it
* @return The new {@link LazyMap}
*/
@SuppressWarnings({ "unchecked", "rawtypes" })
public static Map newLazyHashMap(HashMap map)
{
return LazyMap.lazyMap(map, new InstantiateFactory(HashMap.class));
}
/**
* Factory method for create a new {@link LazyMap} from commons-collections4 that encapsulates a
* {@link HashMap}
*
* @param
* the generic type of the key
* @param
* the generic type of the value
*
* @return The new {@link LazyMap}
*/
public static Map newLazyMap()
{
return newLazyHashMap();
}
/**
* Factory method for create a new {@link LazyMap} from commons-collections4 that encapsulates a
* {@link TreeMap}
*
* @param
* the generic type of the key
* @param
* the generic type of the value
*
* @return The new {@link LazyMap}
*/
public static Map newLazyTreeMap()
{
return newLazyTreeMap(new TreeMap<>());
}
/**
* Factory method for create a new {@link LazyMap} from commons-collections4 that encapsulates a
* {@link TreeMap}
*
* @param
* the generic type of the key
* @param
* the generic type of the value
* @param comparator
* the comparator
*
* @return The new {@link LazyMap}
*/
@SuppressWarnings({ "unchecked", "rawtypes" })
public static Map newLazyTreeMap(final Comparator super K> comparator)
{
Argument.notNull(comparator, "comparator");
return LazyMap.lazyMap(new TreeMap(comparator),
new InstantiateFactory(TreeMap.class));
}
/**
* Factory method for create a new {@link LazyMap} from commons-collections4 that encapsulates a
* {@link TreeMap}
*
* @param
* the generic type of the key
* @param
* the generic type of the value
* @param map
* the map
* @return The new {@link LazyMap}
*/
@SuppressWarnings({ "unchecked", "rawtypes" })
public static Map newLazyTreeMap(final TreeMap map)
{
Argument.notNull(map, "map");
return LazyMap.lazyMap(map, new InstantiateFactory(TreeMap.class));
}
/**
* Factory method for create a new {@link LinkedHashMap}
*
* @param
* the generic type of the key
* @param
* the generic type of the value
*
* @return The new {@link LinkedHashMap}
*/
public static Map newLinkedHashMap()
{
return new LinkedHashMap<>();
}
/**
* Factory method for create a new {@link LinkedHashMap}
*
* @param
* the generic type of the key
* @param
* the generic type of the value
* @param keyValuePairs
* the collection with the key value pairs
* @return The new {@link LinkedHashMap}
*/
public static Map newLinkedHashMap(
final Collection> keyValuePairs)
{
Argument.notNull(keyValuePairs, "keyValuePairs");
return newLinkedHashMap(KeyValuePair.toMap(keyValuePairs));
}
/**
* Factory method for create a new {@link LinkedHashMap}
*
* @param
* the generic type of the key
* @param
* the generic type of the value
* @param initialCapacity
* the initial capacity
*
* @return The new {@link LinkedHashMap}
*/
public static Map newLinkedHashMap(final int initialCapacity)
{
return new LinkedHashMap<>(initialCapacity);
}
/**
* Factory method for create a new {@link LinkedHashMap}
*
* @param
* the generic type of the key
* @param
* the generic type of the value
* @param keyValuePairs
* the key value pairs to add in the new {@link LinkedHashMap}
* @return The new {@link LinkedHashMap}
*/
@SafeVarargs
public static Map newLinkedHashMap(final KeyValuePair... keyValuePairs)
{
Argument.notNull(keyValuePairs, "keyValuePairs");
return newLinkedHashMap(ListFactory.newArrayList(keyValuePairs));
}
/**
* Factory method for create a new {@link LinkedHashMap}
*
* @param
* the generic type of the key
* @param
* the generic type of the value
* @param map
* the map
* @return The new {@link LinkedHashMap}
*/
public static Map newLinkedHashMap(final Map map)
{
Argument.notNull(map, "map");
return new LinkedHashMap<>(map);
}
/**
* Factory method for create a map for count drawn numbers
*
* @param minVolume
* the min volume
* @param maxVolume
* the max volume
* @return the new map with the initial values
*/
public static Map newNumberCounterMap(int minVolume, int maxVolume)
{
return MapFactory.newCounterMap(ListFactory.newRangeList(minVolume, maxVolume));
}
/**
* Factory method for create a map for count drawn numbers and will be summarized with the given
* Map
*
* @param minVolume
* the min volume
* @param maxVolume
* the max volume
* @param numberCounterMap
* the Map that will be summarized
* @return the new map with the initial values
*/
public static Map newNumberCounterMap(int minVolume, int maxVolume,
Map numberCounterMap)
{
Argument.notNull(numberCounterMap, "numberCounterMap");
return MapExtensions.mergeAndSummarize(newNumberCounterMap(minVolume, maxVolume),
numberCounterMap);
}
/**
* Factory method for create a new {@link TreeMap}
*
* @param
* the generic type of the key
* @param
* the generic type of the value
*
* @return The new {@link TreeMap}
*/
public static Map newTreeMap()
{
return new TreeMap<>();
}
/**
* Factory method for create a new {@link TreeMap}
*
* @param
* the generic type of the key
* @param
* the generic type of the value
* @param keyValuePairs
* the collection with the key value pairs
* @return The new {@link TreeMap}
*/
public static Map newTreeMap(final Collection> keyValuePairs)
{
Argument.notNull(keyValuePairs, "keyValuePairs");
return newTreeMap(KeyValuePair.toMap(keyValuePairs));
}
/**
* Factory method for create a new {@link TreeMap}
*
* @param
* the generic type of the key
* @param
* the generic type of the value
* @param comparator
* the comparator
* @return The new {@link TreeMap}
*/
public static Map newTreeMap(final Comparator super K> comparator)
{
Argument.notNull(comparator, "comparator");
return new TreeMap<>(comparator);
}
/**
* Factory method for create a new {@link TreeMap}
*
* @param
* the generic type of the key
* @param
* the generic type of the value
* @param comparator
* the comparator
* @param keyValuePairs
* the key value pairs
* @return The new {@link TreeMap}
*/
public static Map newTreeMap(final Comparator super K> comparator,
final Collection> keyValuePairs)
{
Argument.notNull(comparator, "comparator");
Argument.notNull(keyValuePairs, "keyValuePairs");
TreeMap treeMap = new TreeMap<>(comparator);
treeMap.putAll(newTreeMap(keyValuePairs));
return treeMap;
}
/**
* Factory method for create a new {@link TreeMap}
*
* @param
* the generic type of the key
* @param
* the generic type of the value
* @param comparator
* the comparator
* @param keyValuePairs
* the key value pairs to add in the new {@link TreeMap}
* @return The new {@link TreeMap}
*/
@SafeVarargs
public static Map newTreeMap(final Comparator super K> comparator,
final KeyValuePair... keyValuePairs)
{
Argument.notNull(comparator, "comparator");
Argument.notNull(keyValuePairs, "keyValuePairs");
TreeMap treeMap = new TreeMap<>(comparator);
treeMap.putAll(newTreeMap(keyValuePairs));
return treeMap;
}
/**
* Factory method for create a new {@link TreeMap}
*
* @param
* the generic type of the key
* @param
* the generic type of the value
* @param keyValuePairs
* the key value pairs to add in the new {@link TreeMap}
* @return The new {@link TreeMap}
*/
@SafeVarargs
public static Map newTreeMap(final KeyValuePair... keyValuePairs)
{
Argument.notNull(keyValuePairs, "keyValuePairs");
return newTreeMap(ListFactory.newArrayList(keyValuePairs));
}
/**
* Factory method for create a new {@link TreeMap}
*
* @param
* the generic type of the key
* @param
* the generic type of the value
* @param map
* the map
* @return The new {@link TreeMap}
*/
public static Map newTreeMap(final Map map)
{
Argument.notNull(map, "map");
return new TreeMap<>(map);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy