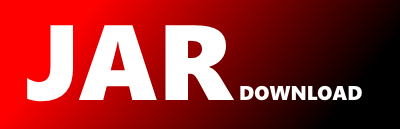
io.github.atkawa7.kannel.sms.clients.XmlSmsSender Maven / Gradle / Ivy
package io.github.atkawa7.kannel.sms.clients;
import io.github.atkawa7.kannel.sms.SendStatus;
import io.github.atkawa7.kannel.sms.Sms;
import io.github.atkawa7.kannel.sms.xml.*;
import javax.xml.bind.JAXBContext;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.Marshaller;
import java.io.StringWriter;
import java.net.URL;
/**
* Send SMS to the smsbox by POSTing XML via HTTP.
*
* @author Garth Patil {@literal }
*/
public class XmlSmsSender extends SmsSender {
private final Marshaller marshaller;
public XmlSmsSender(URL smsbox, SmsClient smsClient) throws Exception {
super(smsbox, smsClient);
JAXBContext jaxbContext = JAXBContext.newInstance(Message.class);
this.marshaller = jaxbContext.createMarshaller();
}
public SendStatus send(Sms sms) throws Exception {
StringWriter sw = new StringWriter();
marshaller.marshal( buildXml(sms), sw);
return smsClient.sendXml(smsbox, sw.toString());
}
private JAXBElement buildXml(Sms sms) {
ObjectFactory factory = new ObjectFactory();
Message message = new Message();
Submit submit = factory.createSubmit();
message.setSubmit(submit);
if (f(sms.getUsername()) && f(sms.getPassword())) {
From from = factory.createFrom();
from.setUsername(sms.getUsername());
from.setPassword(sms.getPassword());
submit.setFrom(from);
if (f(sms.getAccount())) {
from.setAccount(sms.getAccount());
}
}
if (f(sms.getSmsc())) {
submit.setTo(sms.getSmsc());
}
if (f(sms.getFrom())) {
Address oa = factory.createAddress();
oa.setNumber(sms.getFrom());
submit.setOa(oa);
}
if (f(sms.getTo())) {
Address da = factory.createAddress();
da.setNumber(sms.getTo());
submit.setDa(da);
}
if (f(sms.getText())) {
submit.setUd(sms.getText());
}
// if (sms.getUdh().length > 0) {
// submit.setUdh(new String(sms.getUdh()));
// }
if (sms.getDlrMask() != null && sms.getDlrUrl() != null) {
Statusrequest statusrequest = factory.createStatusrequest();
statusrequest.setDlrMask(sms.getDlrMask());
statusrequest.setDlrUrl(sms.getDlrUrl().toString());
submit.setStatusrequest(statusrequest);
}
if (sms.getPid() != null) {
submit.setPid(sms.getPid().intValue());
}
if (sms.getRpi() != null) {
submit.setRpi(sms.getRpi());
}
if (sms.getValidity() != null) {
Time vp = factory.createTime();
vp.setDelay(sms.getValidity());
submit.setVp(vp);
}
if (sms.getDeferred() != null) {
Time timing = factory.createTime();
timing.setDelay(sms.getDeferred());
submit.setTiming(timing);
}
if (sms.getMclass() != null ||
sms.getMwi() != null ||
sms.getCoding() != null ||
sms.getCompress() != null ||
sms.getAltDcs() != null) {
Dcs dcs = factory.createDcs();
if (sms.getMclass() != null) dcs.setMclass(sms.getMclass());
if (sms.getMwi() != null) dcs.setMwi(sms.getMwi());
if (sms.getCompress() != null) dcs.setCompress(sms.getCompress());
if (sms.getCoding() != null) dcs.setCoding(sms.getCoding());
if (sms.getAltDcs() != null) dcs.setAltDcs(sms.getAltDcs());
submit.setDcs(dcs);
}
return factory.createMessage(message);
}
private boolean e(String s) {
return (s == null || s.trim().equals(""));
}
private boolean f(String s) {
return (s != null && !s.trim().equals(""));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy