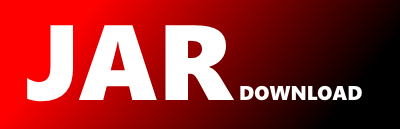
org.openapitools.codegen.languages.JavaPKMSTServerCodegen Maven / Gradle / Ivy
/*
* Copyright 2018 OpenAPI-Generator Contributors (https://openapi-generator.tech)
* Copyright 2018 SmartBear Software
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.openapitools.codegen.languages;
import io.swagger.v3.oas.models.OpenAPI;
import io.swagger.v3.oas.models.Operation;
import io.swagger.v3.oas.models.PathItem;
import io.swagger.v3.oas.models.tags.Tag;
import org.openapitools.codegen.*;
import org.openapitools.codegen.meta.features.DocumentationFeature;
import org.openapitools.codegen.utils.URLPathUtils;
import java.io.File;
import java.net.URL;
import java.util.*;
import static org.openapitools.codegen.utils.StringUtils.camelize;
/**
* Created by prokarma on 04/09/17.
*/
public class JavaPKMSTServerCodegen extends AbstractJavaCodegen {
public static final String CONFIG_PACKAGE = "configPackage";
public static final String BASE_PACKAGE = "basePackage";
public static final String TITLE = "title";
public static final String EUREKA_URI = "eurekaUri";
public static final String ZIPKIN_URI = "zipkinUri";
public static final String SPRINGADMIN_URI = "springBootAdminUri";
protected String basePackage = "com.prokarma.pkmst";
protected String serviceName = "Pkmst";
protected String configPackage = "com.prokarma.pkmst.config";
protected boolean implicitHeaders = false;
protected String title;
protected String eurekaUri;
protected String zipkinUri;
protected String springBootAdminUri;
public JavaPKMSTServerCodegen() {
super();
modifyFeatureSet(features -> features.includeDocumentationFeatures(DocumentationFeature.Readme));
groupId = "com.prokarma";
artifactId = "pkmst-microservice";
embeddedTemplateDir = templateDir = "java-pkmst";
apiPackage = "com.prokarma.pkmst.controller";
modelPackage = "com.prokarma.pkmst.model";
invokerPackage = "com.prokarma.pkmst.controller";
// clioOptions default redifinition need to be updated
updateOption(CodegenConstants.GROUP_ID, this.getGroupId());
updateOption(CodegenConstants.INVOKER_PACKAGE, this.getInvokerPackage());
updateOption(CodegenConstants.ARTIFACT_ID, this.getArtifactId());
updateOption(CodegenConstants.API_PACKAGE, apiPackage);
updateOption(CodegenConstants.MODEL_PACKAGE, modelPackage);
additionalProperties.put("jackson", "true");
this.cliOptions.add(new CliOption("basePackage", "base package for java source code"));
this.cliOptions.add(new CliOption("serviceName", "Service Name"));
this.cliOptions.add(new CliOption(TITLE, "server title name or client service name"));
this.cliOptions.add(new CliOption("eurekaUri", "Eureka URI"));
this.cliOptions.add(new CliOption("zipkinUri", "Zipkin URI"));
this.cliOptions.add(new CliOption("springBootAdminUri", "Spring-Boot URI"));
// Middleware config
this.cliOptions.add(new CliOption("pkmstInterceptor", "PKMST Interceptor"));
this.apiTestTemplateFiles.put("api_test.mustache", ".java");
if (".md".equals(this.modelDocTemplateFiles.get("model_doc.mustache"))) {
this.modelDocTemplateFiles.remove("model_doc.mustache");
}
if (".md".equals(this.apiDocTemplateFiles.get("api_doc.mustache"))) {
this.apiDocTemplateFiles.remove("api_doc.mustache");
}
}
public CodegenType getTag() {
return CodegenType.SERVER;
}
public String getName() {
return "java-pkmst";
}
public String getHelp() {
return "Generates a PKMST SpringBoot Server application using the SpringFox integration."
+ " Also enables EurekaServerClient / Zipkin / Spring-Boot admin";
}
public void processOpts() {
super.processOpts();
if (this.additionalProperties.containsKey("basePackage")) {
this.setBasePackage((String) this.additionalProperties.get("basePackage"));
this.setInvokerPackage(this.getBasePackage());
this.apiPackage = this.getBasePackage() + ".controller";
this.modelPackage = this.getBasePackage() + ".model";
this.setConfigPackage(this.getBasePackage() + ".config");
} else {
this.additionalProperties.put(BASE_PACKAGE, basePackage);
this.additionalProperties.put(CONFIG_PACKAGE, this.getConfigPackage());
this.additionalProperties.put(CodegenConstants.API_PACKAGE, apiPackage);
this.additionalProperties.put(CodegenConstants.MODEL_PACKAGE, modelPackage);
this.additionalProperties.put(CodegenConstants.INVOKER_PACKAGE, invokerPackage);
}
if (this.additionalProperties.containsKey("groupId")) {
this.setGroupId((String) this.additionalProperties.get("groupId"));
} else {
// not set, use to be passed to template
additionalProperties.put(CodegenConstants.GROUP_ID, groupId);
}
if (this.additionalProperties.containsKey("artifactId")) {
this.setArtifactId((String) this.additionalProperties.get("artifactId"));
} else {
// not set, use to be passed to template
additionalProperties.put(CodegenConstants.ARTIFACT_ID, artifactId);
}
if (this.additionalProperties.containsKey("artifactVersion")) {
this.setArtifactVersion((String) this.additionalProperties.get("artifactVersion"));
} else {
// not set, use to be passed to template
additionalProperties.put(CodegenConstants.ARTIFACT_VERSION, artifactVersion);
}
if (this.additionalProperties.containsKey("serviceName")) {
this.setServiceName((String) this.additionalProperties.get("serviceName"));
} else {
// not set, use to be passed to template
additionalProperties.put("serviceName", serviceName);
}
if (this.additionalProperties.containsKey(CodegenConstants.SERIALIZE_BIG_DECIMAL_AS_STRING)) {
this.setSerializeBigDecimalAsString(Boolean.valueOf(
this.additionalProperties.get(CodegenConstants.SERIALIZE_BIG_DECIMAL_AS_STRING).toString()));
}
if (this.additionalProperties.containsKey(CodegenConstants.SERIALIZABLE_MODEL)) {
this.setSerializableModel(
Boolean.valueOf(this.additionalProperties.get(CodegenConstants.SERIALIZABLE_MODEL).toString()));
}
if (this.additionalProperties.containsKey(TITLE)) {
this.setTitle((String) this.additionalProperties.get(TITLE));
}
this.additionalProperties.put(CodegenConstants.SERIALIZABLE_MODEL, serializableModel);
if (this.additionalProperties.containsKey(FULL_JAVA_UTIL)) {
this.setFullJavaUtil(Boolean.valueOf(this.additionalProperties.get(FULL_JAVA_UTIL).toString()));
}
if (this.additionalProperties.containsKey(EUREKA_URI)) {
this.setEurekaUri((String) this.additionalProperties.get(EUREKA_URI));
}
if (this.additionalProperties.containsKey(ZIPKIN_URI)) {
this.setZipkinUri((String) this.additionalProperties.get(ZIPKIN_URI));
}
if (this.additionalProperties.containsKey(SPRINGADMIN_URI)) {
this.setSpringBootAdminUri((String) this.additionalProperties.get(SPRINGADMIN_URI));
}
if (fullJavaUtil) {
javaUtilPrefix = "java.util.";
}
this.additionalProperties.put(FULL_JAVA_UTIL, fullJavaUtil);
this.additionalProperties.put("javaUtilPrefix", javaUtilPrefix);
this.additionalProperties.put(SUPPORT_JAVA6, false);
this.additionalProperties.put("java8", true);
if (this.additionalProperties.containsKey(WITH_XML)) {
this.setWithXml(Boolean.valueOf(additionalProperties.get(WITH_XML).toString()));
}
this.additionalProperties.put(WITH_XML, withXml);
this.apiTemplateFiles.put("api.mustache", ".java");
this.apiTemplateFiles.put("apiController.mustache", "Controller.java");
this.modelTemplateFiles.put("model.mustache", ".java");
this.supportingFiles.add(new SupportingFile("SpringBootApplication.mustache",
(this.getSourceFolder() + File.separator + this.getBasePackage()).replace(".", File.separator),
this.getServiceName() + "Application" + ".java"));
this.supportingFiles
.add(new SupportingFile("config" + File.separator + "openapiDocumentationConfig.mustache",
(this.sourceFolder + File.separator + this.getConfigPackage()).replace(".",
java.io.File.separator) + File.separator + "swagger",
"OpenAPIDocumentationConfig.java"));
this.supportingFiles.add(new SupportingFile("config" + File.separator + "pkmstproperties.mustache",
(this.sourceFolder + File.separator + this.getConfigPackage()).replace(".", java.io.File.separator)
+ File.separator + "swagger",
"PkmstProperties.java"));
this.supportingFiles.add(new SupportingFile("config" + File.separator + "appconfig.mustache",
(this.sourceFolder + File.separator + this.getConfigPackage()).replace(".", java.io.File.separator)
+ File.separator,
"AppConfig.java"));
// Security
this.supportingFiles
.add(new SupportingFile("security" + File.separator + "authorizationServerConfiguration.mustache",
(this.sourceFolder + File.separator + this.basePackage).replace(".", File.separator)
+ File.separator + "security",
"AuthorizationServerConfiguration.java"));
this.supportingFiles
.add(new SupportingFile("security" + File.separator + "oAuth2SecurityConfiguration.mustache",
(this.sourceFolder + File.separator + this.basePackage).replace(".", File.separator)
+ File.separator + "security",
"OAuth2SecurityConfiguration.java"));
this.supportingFiles
.add(new SupportingFile("security" + File.separator + "resourceServerConfiguration.mustache",
(this.sourceFolder + File.separator + this.basePackage).replace(".", File.separator)
+ File.separator + "security",
"ResourceServerConfiguration.java"));
// logging
this.supportingFiles.add(new SupportingFile("logging" + File.separator + "httpLoggingFilter.mustache",
(this.sourceFolder + File.separator + this.basePackage).replace(".", File.separator) + File.separator
+ "logging",
"HttpLoggingFilter.java"));
// Resources
this.supportingFiles.add(new SupportingFile("resources" + File.separator + "application-local.mustache",
("src.main.resources").replace(".", java.io.File.separator), "application-local.yml"));
this.supportingFiles.add(new SupportingFile("resources" + File.separator + "application-dev.mustache",
("src.main.resources").replace(".", java.io.File.separator), "application-dev.yml"));
this.supportingFiles.add(new SupportingFile("resources" + File.separator + "application-dev-config.mustache",
("src.main.resources").replace(".", java.io.File.separator), "application-dev-config.yml"));
this.supportingFiles.add(new SupportingFile("resources" + File.separator + "bootstrap.mustache",
("src.main.resources").replace(".", java.io.File.separator), "bootstrap.yml"));
// POM
this.supportingFiles.add(new SupportingFile("pom.mustache", "", "pom.xml"));
// Readme
this.supportingFiles.add(new SupportingFile("readme.mustache", "", "Readme.md"));
// manifest
this.supportingFiles.add(new SupportingFile("manifest.mustache", "", "manifest.yml"));
// docker
this.supportingFiles.add(new SupportingFile("docker.mustache", "", "Dockerfile"));
// logstash
this.supportingFiles.add(new SupportingFile("logstash.mustache", "", "logstash.conf"));
// Cucumber
this.supportingFiles.add(new SupportingFile("cucumber" + File.separator + "executeReport.mustache",
this.testFolder + File.separator + this.basePackage.replace(".", File.separator) + File.separator
+ "cucumber" + File.separator + "report",
"ExecuteReport.java"));
this.supportingFiles.add(new SupportingFile(
"cucumber" + File.separator + "cucumberTest.mustache", this.testFolder + File.separator
+ this.basePackage.replace(".", File.separator) + File.separator + "cucumber",
serviceName + "Test.java"));
this.supportingFiles.add(new SupportingFile(
"cucumber" + File.separator + "cucumberSteps.mustache", this.testFolder + File.separator
+ this.basePackage.replace(".", File.separator) + File.separator + "cucumber",
serviceName + "Steps.java"));
this.supportingFiles.add(new SupportingFile(
"cucumber" + File.separator + "package.mustache", this.testFolder + File.separator
+ this.basePackage.replace(".", File.separator) + File.separator + "cucumber",
serviceName + "package-info.java"));
// test resources
this.supportingFiles.add(new SupportingFile("cucumber" + File.separator + "cucumber.mustache",
(("src.test.resources") + File.separator + this.basePackage).replace(".", File.separator)
+ File.separator + "cucumber",
serviceName + ".feature"));
this.supportingFiles.add(new SupportingFile("testresources" + File.separator + "bootstrap.mustache",
("src.test.resources").replace(".", java.io.File.separator), "bootstrap.yml"));
this.supportingFiles.add(new SupportingFile("testresources" + File.separator + "application.mustache",
("src.test.resources").replace(".", java.io.File.separator), "application.properties"));
this.supportingFiles.add(new SupportingFile("testresources" + File.separator + "application-test.mustache",
("src.test.resources").replace(".", java.io.File.separator), "application-test.properties"));
// Gatling
this.supportingFiles.add(new SupportingFile("gatling" + File.separator + "gatling.mustache",
("src.test.resources").replace(".", java.io.File.separator), "gatling.conf"));
this.supportingFiles.add(new SupportingFile("gatling" + File.separator + "application.mustache",
("src.test.resources").replace(".", java.io.File.separator), "application.conf"));
this.supportingFiles.add(new SupportingFile(
"gatling" + File.separator + "testapi.mustache", ("src") + File.separator + ("test") + File.separator
+ ("scala") + File.separator + ("scalaFiles").replace(".", java.io.File.separator),
"testapi.scala"));
// adding class for integration test
this.supportingFiles.add(new SupportingFile(
"integration" + File.separator + "integrationtest.mustache", this.testFolder + File.separator
+ this.basePackage.replace(".", File.separator) + File.separator + "controller",
serviceName + "IT.java"));
}
@SuppressWarnings("unchecked")
@Override
public Map postProcessOperationsWithModels(Map objs, List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy