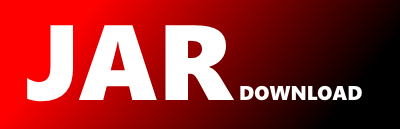
org.openapitools.codegen.languages.ProtobufSchemaCodegen Maven / Gradle / Ivy
/*
* Copyright 2019 OpenAPI-Generator Contributors (https://openapi-generator.tech)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.openapitools.codegen.languages;
import io.swagger.v3.oas.models.media.ArraySchema;
import io.swagger.v3.oas.models.media.Schema;
import org.openapitools.codegen.CodegenConfig;
import org.openapitools.codegen.CodegenConstants;
import org.openapitools.codegen.CodegenType;
import org.openapitools.codegen.*;
import org.openapitools.codegen.meta.GeneratorMetadata;
import org.openapitools.codegen.meta.Stability;
import org.openapitools.codegen.meta.features.DocumentationFeature;
import org.openapitools.codegen.meta.features.SecurityFeature;
import org.openapitools.codegen.meta.features.WireFormatFeature;
import org.openapitools.codegen.utils.ProcessUtils;
import org.openapitools.codegen.utils.ModelUtils;
import org.apache.commons.lang3.StringUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.File;
import java.util.*;
import java.util.regex.Pattern;
import static org.openapitools.codegen.utils.StringUtils.camelize;
import static org.openapitools.codegen.utils.StringUtils.underscore;
public class ProtobufSchemaCodegen extends DefaultCodegen implements CodegenConfig {
private static final Logger LOGGER = LoggerFactory.getLogger(ProtobufSchemaCodegen.class);
protected String packageName = "openapitools";
@Override
public CodegenType getTag() {
return CodegenType.CONFIG;
}
public String getName() {
return "protobuf-schema";
}
public String getHelp() {
return "Generates gRPC and protocol buffer schema files (beta)";
}
public ProtobufSchemaCodegen() {
super();
generatorMetadata = GeneratorMetadata.newBuilder(generatorMetadata)
.stability(Stability.BETA)
.build();
modifyFeatureSet(features -> features
.includeDocumentationFeatures(DocumentationFeature.Readme)
.includeWireFormatFeatures(WireFormatFeature.PROTOBUF)
.wireFormatFeatures(EnumSet.of(WireFormatFeature.PROTOBUF))
.securityFeatures(EnumSet.noneOf(SecurityFeature.class))
);
outputFolder = "generated-code/protobuf-schema";
modelTemplateFiles.put("model.mustache", ".proto");
apiTemplateFiles.put("api.mustache", ".proto");
embeddedTemplateDir = templateDir = "protobuf-schema";
hideGenerationTimestamp = Boolean.TRUE;
modelPackage = "messages";
apiPackage = "services";
/*setReservedWordsLowerCase(
Arrays.asList(
// data type
"nil", "string", "boolean", "number", "userdata", "thread",
"table",
// reserved words: http://www.lua.org/manual/5.1/manual.html#2.1
"and", "break", "do", "else", "elseif",
"end", "false", "for", "function", "if",
"in", "local", "nil", "not", "or",
"repeat", "return", "then", "true", "until", "while"
)
);*/
defaultIncludes = new HashSet(
Arrays.asList(
"map",
"array")
);
languageSpecificPrimitives = new HashSet(
Arrays.asList(
"map",
"array",
"bool",
"bytes",
"string",
"int32",
"int64",
"uint32",
"uint64",
"sint32",
"sint64",
"fixed32",
"fixed64",
"sfixed32",
"sfixed64",
"float",
"double")
);
instantiationTypes.clear();
instantiationTypes.put("array", "repeat");
//instantiationTypes.put("map", "map");
// ref: https://developers.google.com/protocol-buffers/docs/proto
typeMapping.clear();
typeMapping.put("array", "array");
typeMapping.put("map", "map");
typeMapping.put("integer", "int32");
typeMapping.put("long", "int64");
typeMapping.put("number", "float");
typeMapping.put("float", "float");
typeMapping.put("double", "double");
typeMapping.put("boolean", "bool");
typeMapping.put("string", "string");
typeMapping.put("UUID", "string");
typeMapping.put("URI", "string");
typeMapping.put("date", "string");
typeMapping.put("DateTime", "string");
typeMapping.put("password", "string");
// TODO fix file mapping
typeMapping.put("file", "string");
typeMapping.put("binary", "string");
typeMapping.put("ByteArray", "bytes");
typeMapping.put("object", "TODO_OBJECT_MAPPING");
importMapping.clear();
/*
importMapping = new HashMap();
importMapping.put("time.Time", "time");
importMapping.put("*os.File", "os");
importMapping.put("os", "io/ioutil");
*/
modelDocTemplateFiles.put("model_doc.mustache", ".md");
apiDocTemplateFiles.put("api_doc.mustache", ".md");
cliOptions.clear();
/*cliOptions.add(new CliOption(CodegenConstants.PACKAGE_NAME, "GraphQL package name (convention: lowercase).")
.defaultValue("openapi2graphql"));
cliOptions.add(new CliOption(CodegenConstants.PACKAGE_VERSION, "GraphQL package version.")
.defaultValue("1.0.0"));
cliOptions.add(new CliOption(CodegenConstants.HIDE_GENERATION_TIMESTAMP, CodegenConstants.HIDE_GENERATION_TIMESTAMP_DESC)
.defaultValue(Boolean.TRUE.toString()));*/
}
@Override
public void processOpts() {
super.processOpts();
//apiTestTemplateFiles.put("api_test.mustache", ".proto");
//modelTestTemplateFiles.put("model_test.mustache", ".proto");
apiDocTemplateFiles.clear(); // TODO: add api doc template
modelDocTemplateFiles.clear(); // TODO: add model doc template
modelPackage = "models";
apiPackage = "services";
if (additionalProperties.containsKey(CodegenConstants.PACKAGE_NAME)) {
setPackageName((String) additionalProperties.get(CodegenConstants.PACKAGE_NAME));
}
supportingFiles.add(new SupportingFile("README.mustache", "", "README.md"));
//supportingFiles.add(new SupportingFile("root.mustache", "", packageName + ".proto"));
//supportingFiles.add(new SupportingFile("git_push.sh.mustache", "", "git_push.sh"));
//supportingFiles.add(new SupportingFile("gitignore.mustache", "", ".gitignore"))
//supportingFiles.add(new SupportingFile(".travis.yml", "", ".travis.yml"));
}
@Override
public String toOperationId(String operationId) {
// throw exception if method name is empty (should not occur as an auto-generated method name will be used)
if (StringUtils.isEmpty(operationId)) {
throw new RuntimeException("Empty method name (operationId) not allowed");
}
// method name cannot use reserved keyword, e.g. return
if (isReservedWord(operationId)) {
LOGGER.warn(operationId + " (reserved word) cannot be used as method name. Renamed to " + camelize(sanitizeName("call_" + operationId)));
operationId = "call_" + operationId;
}
return camelize(sanitizeName(operationId));
}
@Override
public Map postProcessModels(Map objs) {
objs = postProcessModelsEnum(objs);
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy