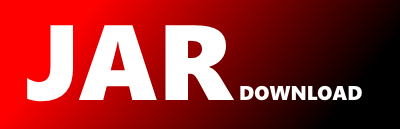
io.github.benas.randombeans.util.CharacterUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of random-beans Show documentation
Show all versions of random-beans Show documentation
Random Beans core implementation
package io.github.benas.randombeans.util;
import lombok.experimental.UtilityClass;
import java.nio.charset.Charset;
import java.util.ArrayList;
import java.util.List;
import static java.util.stream.Collectors.toList;
@UtilityClass
public class CharacterUtils {
/**
* Returns a list of all printable charaters of the given charset.
*
* @param charset
* Charset to use
* @return list of printable characters
*/
public static List collectPrintableCharactersOf(Charset charset) {
List chars = new ArrayList<>();
for (int i = Character.MIN_VALUE; i < Character.MAX_VALUE; i++) {
char character = (char) i;
if (isPrintable(character)) {
String characterAsString = Character.toString(character);
byte[] encoded = characterAsString.getBytes(charset);
String decoded = new String(encoded, charset);
if (characterAsString.equals(decoded)) {
chars.add(character);
}
}
}
return chars;
}
/**
* Keep only letters from a list of characters.
* @param characters to filter
* @return only letters
*/
public static List filterLetters(List characters) {
return characters.stream().filter(Character::isLetter).collect(toList());
}
private static boolean isPrintable(char character) {
Character.UnicodeBlock block = Character.UnicodeBlock.of(character);
return (!Character.isISOControl(character)) && block != null && block != Character.UnicodeBlock.SPECIALS;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy