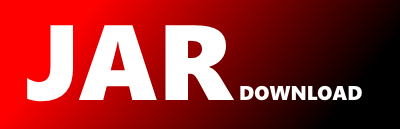
org.sql2o.Connection Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of anima Show documentation
Show all versions of anima Show documentation
Operate the database like a stream
package org.sql2o;
import lombok.extern.slf4j.Slf4j;
import org.sql2o.connectionsources.ConnectionSource;
import org.sql2o.converters.Converter;
import org.sql2o.converters.ConverterException;
import org.sql2o.quirks.Quirks;
import java.io.Closeable;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import static org.sql2o.converters.Convert.throwIfNull;
/**
* Represents a connection to the database with a transaction.
*/
@Slf4j
public class Connection implements AutoCloseable, Closeable {
private ConnectionSource connectionSource;
private java.sql.Connection jdbcConnection;
private Sql2o sql2o;
private Integer result = null;
private int[] batchResult = null;
private List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy