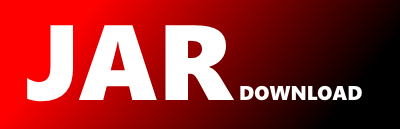
com.binance.connector.client.impl.spot.BSwap Maven / Gradle / Ivy
package com.binance.connector.client.impl.spot;
import com.binance.connector.client.enums.HttpMethod;
import com.binance.connector.client.utils.HmacSignatureGenerator;
import com.binance.connector.client.utils.ParameterChecker;
import com.binance.connector.client.utils.RequestHandler;
import com.binance.connector.client.utils.SignatureGenerator;
import java.util.LinkedHashMap;
/**
* BSwap Endpoints
* All endpoints under the
* BSwap Endpoint
* section of the API documentation will be implemented in this class.
*
* Response will be returned in String format.
*/
public class BSwap {
private final String baseUrl;
private final RequestHandler requestHandler;
private final boolean showLimitUsage;
public BSwap(String baseUrl, String apiKey, String secretKey, boolean showLimitUsage) {
this.baseUrl = baseUrl;
this.requestHandler = new RequestHandler(apiKey, new HmacSignatureGenerator(secretKey));
this.showLimitUsage = showLimitUsage;
}
public BSwap(String baseUrl, String apiKey, SignatureGenerator signatureGenerator, boolean showLimitUsage) {
this.baseUrl = baseUrl;
this.requestHandler = new RequestHandler(apiKey, signatureGenerator);
this.showLimitUsage = showLimitUsage;
}
private final String SWAP_POOLS = "/sapi/v1/bswap/pools";
/**
* Get metadata about all swap pools.
*
* GET /sapi/v1/bswap/pools
*
* @return String
* @see
* https://binance-docs.github.io/apidocs/spot/en/#list-all-swap-pools-market_data
*/
public String swapPools() {
return requestHandler.sendWithApiKeyRequest(baseUrl, SWAP_POOLS, null, HttpMethod.GET, showLimitUsage);
}
private final String LIQUIDITY = "/sapi/v1/bswap/liquidity";
/**
* Get liquidity information and user share of a pool.
*
* GET /sapi/v1/bswap/liquidity
*
* @param
* parameters LinkedHashedMap of String,Object pair
* where String is the name of the parameter and Object is the value of the parameter
*
* poolId -- optional/long
* recvWindow -- optional/long
* @return String
* @see
* https://binance-docs.github.io/apidocs/spot/en/#subscribe-blvt-user_data
*/
public String liquidity(LinkedHashMap parameters) {
return requestHandler.sendSignedRequest(baseUrl, LIQUIDITY, parameters, HttpMethod.GET, showLimitUsage);
}
private final String LIQUIDITY_ADD = "/sapi/v1/bswap/liquidityAdd";
/**
* Add liquidity to a pool.
*
* POST /sapi/v1/bswap/liquidityAdd
*
* @param
* parameters LinkedHashedMap of String,Object pair
* where String is the name of the parameter and Object is the value of the parameter
*
* poolId -- mandatory/long
* type -- optional/string -- "Single" to add a single token; "Combination" to add dual tokens. Default "Single"
* asset -- mandatory/string
* quantity -- mandatory/decimal
* recvWindow -- optional/long
* @return String
* @see
* https://binance-docs.github.io/apidocs/spot/en/#add-liquidity-trade
*/
public String liquidityAdd(LinkedHashMap parameters) {
ParameterChecker.checkParameter(parameters, "poolId", Long.class);
ParameterChecker.checkParameter(parameters, "asset", String.class);
ParameterChecker.checkRequiredParameter(parameters, "quantity");
return requestHandler.sendSignedRequest(baseUrl, LIQUIDITY_ADD, parameters, HttpMethod.POST, showLimitUsage);
}
private final String LIQUIDITY_REMOVE = "/sapi/v1/bswap/liquidityRemove";
/**
* Remove liquidity from a pool, type include SINGLE and COMBINATION, asset is mandatory for single asset removal.
*
* POST /sapi/v1/bswap/liquidityRemove
*
* @param
* parameters LinkedHashedMap of String,Object pair
* where String is the name of the parameter and Object is the value of the parameter
*
* poolId -- mandatory/long
* type -- mandatory/string -- SINGLE for single asset removal, COMBINATION for combination of all coins removal
* asset -- optional/string
* shareAmount -- mandatory/decimal
* recvWindow -- optional/long
* @return String
* @see
* https://binance-docs.github.io/apidocs/spot/en/#remove-liquidity-trade
*/
public String liquidityRemove(LinkedHashMap parameters) {
ParameterChecker.checkParameter(parameters, "poolId", Long.class);
ParameterChecker.checkParameter(parameters, "type", String.class);
ParameterChecker.checkRequiredParameter(parameters, "shareAmount");
return requestHandler.sendSignedRequest(baseUrl, LIQUIDITY_REMOVE, parameters, HttpMethod.POST, showLimitUsage);
}
private final String LIQUIDITY_OPS = "/sapi/v1/bswap/liquidityOps";
/**
* Get liquidity operation (add/remove) records.
*
* GET /sapi/v1/bswap/liquidityOps
*
* @param
* parameters LinkedHashedMap of String,Object pair
* where String is the name of the parameter and Object is the value of the parameter
*
* operationId -- optional/long
* poolId -- optional/long
* operation -- optional/enum -- ADD or REMOVE
* startTime -- optional/long
* endTime -- optional/long
* limit -- optional/long -- default 3, max 100
* recvWindow -- optional/long
* @return String
* @see
* https://binance-docs.github.io/apidocs/spot/en/#get-liquidity-operation-record-user_data
*/
public String liquidityOps(LinkedHashMap parameters) {
return requestHandler.sendSignedRequest(baseUrl, LIQUIDITY_OPS, parameters, HttpMethod.GET, showLimitUsage);
}
private final String QUOTE = "/sapi/v1/bswap/quote";
/**
* Request a quote for swap quote asset (selling asset) for base asset (buying asset), essentially price/exchange rates.
* quoteQty is quantity of quote asset (to sell).
* Please be noted the quote is for reference only, the actual price will change as the liquidity changes, it's recommended to swap immediate after request a quote for slippage prevention.
*
* GET /sapi/v1/bswap/quote
*
* @param
* parameters LinkedHashedMap of String,Object pair
* where String is the name of the parameter and Object is the value of the parameter
*
* quoteAsset -- mandatory/string
* baseAsset -- mandatory/string
* quoteQty -- mandatory/decimal
* recvWindow -- optional/long
* @return String
* @see
* https://binance-docs.github.io/apidocs/spot/en/#get-liquidity-operation-record-user_data
*/
public String quote(LinkedHashMap parameters) {
ParameterChecker.checkParameter(parameters, "quoteAsset", String.class);
ParameterChecker.checkParameter(parameters, "baseAsset", String.class);
ParameterChecker.checkRequiredParameter(parameters, "quoteQty");
return requestHandler.sendSignedRequest(baseUrl, QUOTE, parameters, HttpMethod.GET, showLimitUsage);
}
private final String SWAP = "/sapi/v1/bswap/swap";
/**
* Swap quoteAsset for baseAsset.
*
* POST /sapi/v1/bswap/swap
*
* @param
* parameters LinkedHashedMap of String,Object pair
* where String is the name of the parameter and Object is the value of the parameter
*
* quoteAsset -- mandatory/string
* baseAsset -- mandatory/string
* quoteQty -- mandatory/decimal
* recvWindow -- optional/long
* @return String
* @see
* https://binance-docs.github.io/apidocs/spot/en/#swap-trade
*/
public String swap(LinkedHashMap parameters) {
ParameterChecker.checkParameter(parameters, "quoteAsset", String.class);
ParameterChecker.checkParameter(parameters, "baseAsset", String.class);
ParameterChecker.checkRequiredParameter(parameters, "quoteQty");
return requestHandler.sendSignedRequest(baseUrl, SWAP, parameters, HttpMethod.POST, showLimitUsage);
}
/**
* Get swap history.
*
* GET /sapi/v1/bswap/swap
*
* @param
* parameters LinkedHashedMap of String,Object pair
* where String is the name of the parameter and Object is the value of the parameter
*
* swapId -- optional/long
* startTime -- optional/long
* endTime -- optional/long
* status -- optional/int -- 0: pending for swap, 1: success, 2: failed
* quoteAsset -- optional/string
* baseAsset -- optional/string
* limit -- optional/long -- default 3, max 100
* recvWindow -- optional/long
* @return String
* @see
* https://binance-docs.github.io/apidocs/spot/en/#get-swap-history-user_data
*/
public String swapHistory(LinkedHashMap parameters) {
return requestHandler.sendSignedRequest(baseUrl, SWAP, parameters, HttpMethod.GET, showLimitUsage);
}
private final String POOL_CONFIGURE = "/sapi/v1/bswap/poolConfigure";
/**
* GET /sapi/v1/bswap/poolConfigure
*
* @param
* parameters LinkedHashedMap of String,Object pair
* where String is the name of the parameter and Object is the value of the parameter
*
* poolId -- optional/long
* recvWindow -- optional/long
* @return String
* @see
* https://binance-docs.github.io/apidocs/spot/en/#get-pool-configure-user_data
*/
public String poolConfigure(LinkedHashMap parameters) {
return requestHandler.sendSignedRequest(baseUrl, POOL_CONFIGURE, parameters, HttpMethod.GET, showLimitUsage);
}
private final String ADD_LIQUIDITY_PREVIEW = "/sapi/v1/bswap/addLiquidityPreview";
/**
* Calculate expected share amount for adding liquidity in single or dual token.
*
* GET /sapi/v1/bswap/addLiquidityPreview
*
* @param
* parameters LinkedHashedMap of String,Object pair
* where String is the name of the parameter and Object is the value of the parameter
*
* poolId -- mandatory/long
* type -- mandatory/string -- "SINGLE" for adding a single token;"COMBINATION" for adding dual tokens
* quoteAsset -- mandatory/string
* quoteQty -- mandatory/decimal
* recvWindow -- optional/long
* @return String
* @see
* https://binance-docs.github.io/apidocs/spot/en/#add-liquidity-preview-user_data
*/
public String addLiquidityPreview(LinkedHashMap parameters) {
ParameterChecker.checkParameter(parameters, "poolId", Long.class);
ParameterChecker.checkParameter(parameters, "type", String.class);
ParameterChecker.checkParameter(parameters, "quoteAsset", String.class);
ParameterChecker.checkRequiredParameter(parameters, "quoteQty");
return requestHandler.sendSignedRequest(baseUrl, ADD_LIQUIDITY_PREVIEW, parameters, HttpMethod.GET, showLimitUsage);
}
private final String REMOVE_LIQUIDITY_PREVIEW = "/sapi/v1/bswap/removeLiquidityPreview";
/**
* Calculate the expected asset amount of single token redemption or dual token redemption.
*
* GET /sapi/v1/bswap/removeLiquidityPreview
*
* @param
* parameters LinkedHashedMap of String,Object pair
* where String is the name of the parameter and Object is the value of the parameter
*
* poolId -- mandatory/long
* type -- mandatory/string -- "SINGLE" for adding a single token;"COMBINATION" for adding dual tokens
* quoteAsset -- mandatory/string
* shareAmount -- mandatory/decimal
* recvWindow -- optional/long
* @return String
* @see
* https://binance-docs.github.io/apidocs/spot/en/#remove-liquidity-preview-user_data
*/
public String removeLiquidityPreview(LinkedHashMap parameters) {
ParameterChecker.checkParameter(parameters, "poolId", Long.class);
ParameterChecker.checkParameter(parameters, "type", String.class);
ParameterChecker.checkParameter(parameters, "quoteAsset", String.class);
ParameterChecker.checkRequiredParameter(parameters, "shareAmount");
return requestHandler.sendSignedRequest(baseUrl, REMOVE_LIQUIDITY_PREVIEW, parameters, HttpMethod.GET, showLimitUsage);
}
private final String UNCLAIMED_REWARDS = "/sapi/v1/bswap/unclaimedRewards";
/**
* Get unclaimed rewards record.
*
* GET /sapi/v1/bswap/unclaimedRewards
*
* @param
* parameters LinkedHashedMap of String,Object pair
* where String is the name of the parameter and Object is the value of the parameter
*
* type -- optional/int -- 0: Swap rewards,1:Liquidity rewards, default to 0
* recvWindow -- optional/long
* @return String
* @see
* https://binance-docs.github.io/apidocs/spot/en/#get-unclaimed-rewards-record-user_data
*/
public String unclaimedRewards(LinkedHashMap parameters) {
return requestHandler.sendSignedRequest(baseUrl, UNCLAIMED_REWARDS, parameters, HttpMethod.GET, showLimitUsage);
}
private final String CLAIM_REWARDS = "/sapi/v1/bswap/claimRewards";
/**
* Claim swap rewards or liquidity rewards
*
* POST /sapi/v1/bswap/claimRewards
*
* @param
* parameters LinkedHashedMap of String,Object pair
* where String is the name of the parameter and Object is the value of the parameter
*
* type -- optional/int -- 0: Swap rewards,1:Liquidity rewards, default to 0
* recvWindow -- optional/long
* @return String
* @see
* https://binance-docs.github.io/apidocs/spot/en/#claim-rewards-trade
*/
public String claimRewards(LinkedHashMap parameters) {
return requestHandler.sendSignedRequest(baseUrl, CLAIM_REWARDS, parameters, HttpMethod.POST, showLimitUsage);
}
private final String CLAIM_HISTORY = "/sapi/v1/bswap/claimedHistory";
/**
* Claim swap rewards or liquidity rewards
*
* GET /sapi/v1/bswap/claimedHistory
*
* @param
* parameters LinkedHashedMap of String,Object pair
* where String is the name of the parameter and Object is the value of the parameter
*
* poolId -- optional/long
* assetRewards -- optional/string
* type -- optional/int -- 0: Swap rewards,1:Liquidity rewards, default to 0
* startTime -- optional/long
* endTime -- optional/long
* limit -- optional/long -- Default 3, max 100
* recvWindow -- optional/long
* @return String
* @see
* https://binance-docs.github.io/apidocs/spot/en/#get-claimed-history-user_data
*/
public String claimedHistory(LinkedHashMap parameters) {
return requestHandler.sendSignedRequest(baseUrl, CLAIM_HISTORY, parameters, HttpMethod.GET, showLimitUsage);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy