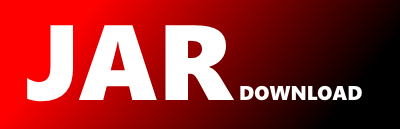
org.treesitter.TSLanguage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tree-sitter Show documentation
Show all versions of tree-sitter Show documentation
Next generation Tree Sitter Java binding
package org.treesitter;
public interface TSLanguage {
long getPtr();
/**
* Get the ABI version number for this language. This version number is used
* to ensure that languages were generated by a compatible version of
* Tree-sitter.
*
* See also {@link TSParser#setLanguage(TSLanguage) TSParser#setLanguage}.
*
* @return The language version number.
*/
default int version(){
return TSParser.ts_language_version(this.getPtr());
}
/**
* Get the number of distinct field names in the language.
*
* @return The number of fields.
*/
default int fieldCount(){
return TSParser.ts_language_field_count(this.getPtr());
}
/**
* Get the field name string for the given numerical id.
*
* @param id the field id.
*
* @return The field name string.
*/
default String fieldNameForId(int id){
return TSParser.ts_language_field_name_for_id(this.getPtr(), id);
}
/**
* Get the numerical id for the given field name string.
*
* @param name the field name string.
*
* @return The field id.
*/
default int fieldIdForName(String name){
return TSParser.ts_language_field_id_for_name(this.getPtr(), name);
}
/**
* Check whether the given node type id belongs to named nodes, anonymous nodes,
* or a hidden nodes.
*
* See also {@link TSNode#isNamed()}. Hidden nodes are never returned from the API.
*
* @param symbol the node type id.
*
* @return The symbol type.
*/
default TSSymbolType symbolType(int symbol){
int type = TSParser.ts_language_symbol_type(this.getPtr(), symbol);
switch (type){
case 0: return TSSymbolType.TSSymbolTypeRegular;
case 1: return TSSymbolType.TSSymbolTypeAnonymous;
case 2: return TSSymbolType.TSSymbolTypeAuxiliary;
default: throw new TSException("Can't handle symbol type: %d" + type);
}
}
/**
* Get the number of distinct node types in the language.
*
* @return the number of symbols.
*/
default int symbolCount(){
return TSParser.ts_language_symbol_count(this.getPtr());
}
/**
* Get a node type string for the given numerical id.
*
* @param symbol the node type id.
*
* @return the node type string.
*/
default String symbolName(int symbol){
return TSParser.ts_language_symbol_name(this.getPtr(), symbol);
}
/**
* Get the numerical id for the given node type string.
*
* @param name the node type string.
* @param isNamed whether the node type is a named node.
*
* @return the node type id.
*/
default int symbolForName(String name, boolean isNamed){
return TSParser.ts_language_symbol_for_name(this.getPtr(), name, isNamed);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy