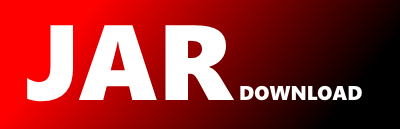
org.treesitter.TsLookAheadIterator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tree-sitter Show documentation
Show all versions of tree-sitter Show documentation
Next generation Tree Sitter Java binding
package org.treesitter;
public class TsLookAheadIterator {
private long ptr;
/**
* Create a new lookahead iterator for the given language and parse state.
*
* Repeatedly using {@link TsLookAheadIterator#next()} and
* {@link TsLookAheadIterator#currentSymbol()} will generate valid symbols in the
* given parse state. Newly created lookahead iterators will contain the ERROR
* symbol.
*
* Lookahead iterators can be useful to generate suggestions and improve syntax
* error diagnostics. To get symbols valid in an ERROR node, use the lookahead
* iterator on its first leaf node state. For MISSING nodes, a lookahead
* iterator created on the previous non-extra leaf node may be appropriate.
*
* @param language The language
* @param state Parser state
*
* @throws TSException If state is invalid for the language
*/
public TsLookAheadIterator(TSLanguage language, int state) {
this.ptr = TSParser.ts_lookahead_iterator_new(language.getPtr(), state);
if(this.ptr == 0){
throw new TSException("State is invalid.");
}
CleanerRunner.register(this, new TsLookAheadIteratorCleanAction(ptr));
}
/**
* Reset the lookahead iterator to another state.
*
* @param state New state
*
* @return true
if the iterator was reset to the given state and false
otherwise.
*/
public boolean resetState(int state){
return TSParser.ts_lookahead_iterator_reset_state(ptr, state);
}
/**
* Reset the lookahead iterator.
*
* @param language The language
* @param state New state
*
* @return true
if the language was set successfully and false
otherwise.
*/
public boolean reset(TSLanguage language, int state){
return TSParser.ts_lookahead_iterator_reset(ptr, language.getPtr(), state);
}
/**
* Get the current language of the lookahead iterator.
*
* @return The language of the lookahead iterator
*/
public TSLanguage getLanguage(){
return new AnonymousLanguage(TSParser.ts_lookahead_iterator_language(ptr));
}
/**
* Advance the lookahead iterator to the next symbol.
*
* @return true
if there is a new symbol and false
otherwise.
*/
public boolean next(){
return TSParser.ts_lookahead_iterator_next(ptr);
}
/**
* Get the current symbol of the lookahead iterator
*
* @return Current symbol
*/
public int currentSymbol(){
return TSParser.ts_lookahead_iterator_current_symbol(ptr);
}
/**
* Get the current symbol type of the lookahead iterator as a string.
*
* @return Current symbol name
*/
public String currentSymbolName(){
return TSParser.ts_lookahead_iterator_current_symbol_name(ptr);
}
protected long getPtr(){
return ptr;
}
public static class TsLookAheadIteratorCleanAction implements Runnable{
private long ptr;
public TsLookAheadIteratorCleanAction(long ptr) {
this.ptr = ptr;
}
@Override
public void run() {
TSParser.ts_lookahead_iterator_delete(ptr);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy