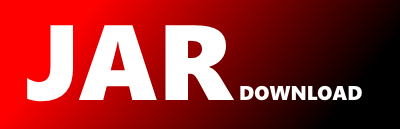
org.treesitter.TSLanguage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tree-sitter Show documentation
Show all versions of tree-sitter Show documentation
Next generation Tree Sitter Java binding
The newest version!
package org.treesitter;
public abstract class TSLanguage {
private long ptr;
protected TSLanguage(long ptr){
this.ptr = ptr;
CleanerRunner.register(this, new TSLanguageCleanAction(ptr));
}
protected long getPtr(){
return this.ptr;
}
/**
* Get the ABI version number for this language. This version number is used
* to ensure that languages were generated by a compatible version of
* Tree-sitter.
*
* See also {@link TSParser#setLanguage(TSLanguage) TSParser#setLanguage}.
*
* @return The language version number.
*/
public int version(){
return TSParser.ts_language_version(this.getPtr());
}
/**
* Get the number of distinct field names in the language.
*
* @return The number of fields.
*/
public int fieldCount(){
return TSParser.ts_language_field_count(this.getPtr());
}
/**
* Get the field name string for the given numerical id.
*
* @param id the field id.
*
* @return The field name string.
*/
public String fieldNameForId(int id){
return TSParser.ts_language_field_name_for_id(this.getPtr(), id);
}
/**
* Get the numerical id for the given field name string.
*
* @param name the field name string.
*
* @return The field id.
*/
public int fieldIdForName(String name){
return TSParser.ts_language_field_id_for_name(this.getPtr(), name);
}
/**
* Check whether the given node type id belongs to named nodes, anonymous nodes,
* or a hidden nodes.
*
* See also {@link TSNode#isNamed()}. Hidden nodes are never returned from the API.
*
* @param symbol the node type id.
*
* @return The symbol type.
*/
public TSSymbolType symbolType(int symbol){
int type = TSParser.ts_language_symbol_type(this.getPtr(), symbol);
switch (type){
case 0: return TSSymbolType.TSSymbolTypeRegular;
case 1: return TSSymbolType.TSSymbolTypeAnonymous;
case 2: return TSSymbolType.TSSymbolTypeSupertype;
case 3: return TSSymbolType.TSSymbolTypeAuxiliary;
default: throw new TSException("Can't handle symbol type: %d" + type);
}
}
/**
* Get the number of distinct node types in the language.
*
* @return the number of symbols.
*/
public int symbolCount(){
return TSParser.ts_language_symbol_count(this.getPtr());
}
/**
* Get a node type string for the given numerical id.
*
* @param symbol the node type id.
*
* @return the node type string.
*/
public String symbolName(int symbol){
return TSParser.ts_language_symbol_name(this.getPtr(), symbol);
}
/**
* Get the numerical id for the given node type string.
*
* @param name the node type string.
* @param isNamed whether the node type is a named node.
*
* @return the node type id.
*/
public int symbolForName(String name, boolean isNamed){
return TSParser.ts_language_symbol_for_name(this.getPtr(), name, isNamed);
}
/**
* Get another reference to the given language.
*
* @return Another reference to the given language
*/
public abstract TSLanguage copy();
protected long copyPtr(){
return TSParser.ts_language_copy(getPtr());
}
public static class TSLanguageCleanAction implements Runnable{
private long ptr;
public TSLanguageCleanAction(long ptr) {
this.ptr = ptr;
}
@Override
public void run() {
TSParser.ts_language_delete(ptr);
}
}
/**
* Get the next parse state. Combine this with lookahead iterators to generate
* completion suggestions or valid symbols in error nodes. Use
* {@link TSNode#getGrammarSymbol()} for valid symbols.
*
* @param state Current state
* @param symbol Symbol id
* @return Next state
*/
public int nextState(int state, int symbol){
return TSParser.ts_language_next_state(ptr, state, symbol);
}
/**
* Get the number of valid states in this language.
*
* @return Valid states counts
*/
public int stateCount(){
return TSParser.ts_language_state_count(ptr);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy