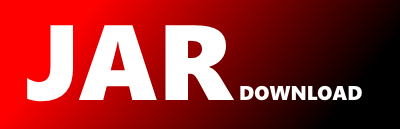
christophedelory.playlist.package.html Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lizzy Show documentation
Show all versions of lizzy Show documentation
Multimedia playlist parser, supporting a wide range of playlist file formats.
The home of the playlist management API.
You should begin here with {@link christophedelory.playlist.SpecificPlaylistFactory}.
You will find below the main steps in order to properly use the API for parsing an existing playlist (exception catching is not performed):
-
Let's begin with a file in your local file system.
{@link java.io.File} file = new {@link java.io.File}("a_playlist_file");
{@link christophedelory.playlist.SpecificPlaylist} specificPlaylist = SpecificPlaylistFactory.getInstance().{@link christophedelory.playlist.SpecificPlaylistFactory#readFrom(java.net.URL) readFrom}(file);
-
If the method didn't throw an exception, the playlist format was recognized.
And then, two cases: if the handle is null, the playlist is malformed:
if (specificPlaylist == null)
{
throw new Exception("Invalid playlist format");
}
-
Or you have now a valid specific playlist descriptor.
You can work directly with it if you know its type (i.e. class).
You can also switch to a "generic" playlist, which is the preferred way if you intend to be format-agnostic,
or if you want to convert this "specific" playlist to another format.
{@link christophedelory.playlist.Playlist} genericPlaylist = specificPlaylist.{@link christophedelory.playlist.SpecificPlaylist#toPlaylist() toPlaylist}();
-
Now work with your "normalized" playlist.
The "generic" playlist hierarchy is the following:

Now you want to save your playlist in a given format, let's say "ASX".
First you have to retrieve the associated playlist provider.
Two methods are available for that purpose:
- You know the "internal" identifier of this provider ("asx" in this case, see the diagram below): use the following code:
{@link christophedelory.playlist.SpecificPlaylistProvider} provider = SpecificPlaylistFactory.getInstance().{@link christophedelory.playlist.SpecificPlaylistFactory#findProviderById(String) findProviderById}("asx");
- Or use the typical file name extension for this type of playlist:
{@link christophedelory.playlist.SpecificPlaylistProvider} provider = SpecificPlaylistFactory.getInstance().{@link christophedelory.playlist.SpecificPlaylistFactory#findProviderByExtension(String) findProviderByExtension}(".asx");
Note: if you prefer to keep the same format as for the source, simply use the input playlist's provider, like this:
{@link christophedelory.playlist.SpecificPlaylistProvider} provider = specificPlaylist.{@link christophedelory.playlist.SpecificPlaylist#getProvider() getProvider}();
The "specific" playlist providers hierarchy is the following:

Now convert your generic playlist description to the chosen format:
{@link christophedelory.playlist.SpecificPlaylist} newSpecificPlaylist = provider.{@link christophedelory.playlist.SpecificPlaylistProvider#toSpecificPlaylist(christophedelory.playlist.Playlist) toSpecificPlaylist}(genericPlaylist);
If everything ran well, you can now flush the result to any output stream:
newSpecificPlaylist.{@link christophedelory.playlist.SpecificPlaylist#writeTo(java.io.OutputStream,String) writeTo}(System.out, null);
Enjoy!
© 2015 - 2025 Weber Informatics LLC | Privacy Policy