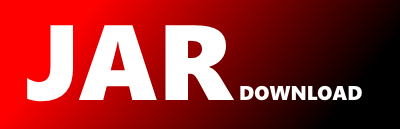
com.authzed.api.v1.CheckDebugTraceKt.kt Maven / Gradle / Ivy
The newest version!
//Generated by the protocol buffer compiler. DO NOT EDIT!
// source: authzed/api/v1/debug.proto
package com.authzed.api.v1;
@kotlin.jvm.JvmName("-initializecheckDebugTrace")
public inline fun checkDebugTrace(block: com.authzed.api.v1.CheckDebugTraceKt.Dsl.() -> kotlin.Unit): com.authzed.api.v1.Debug.CheckDebugTrace =
com.authzed.api.v1.CheckDebugTraceKt.Dsl._create(com.authzed.api.v1.Debug.CheckDebugTrace.newBuilder()).apply { block() }._build()
public object CheckDebugTraceKt {
@kotlin.OptIn(com.google.protobuf.kotlin.OnlyForUseByGeneratedProtoCode::class)
@com.google.protobuf.kotlin.ProtoDslMarker
public class Dsl private constructor(
private val _builder: com.authzed.api.v1.Debug.CheckDebugTrace.Builder
) {
public companion object {
@kotlin.jvm.JvmSynthetic
@kotlin.PublishedApi
internal fun _create(builder: com.authzed.api.v1.Debug.CheckDebugTrace.Builder): Dsl = Dsl(builder)
}
@kotlin.jvm.JvmSynthetic
@kotlin.PublishedApi
internal fun _build(): com.authzed.api.v1.Debug.CheckDebugTrace = _builder.build()
/**
*
* resource holds the resource on which the Check was performed.
*
*
* .authzed.api.v1.ObjectReference resource = 1 [(.validate.rules) = { ... }
*/
public var resource: com.authzed.api.v1.Core.ObjectReference
@JvmName("getResource")
get() = _builder.getResource()
@JvmName("setResource")
set(value) {
_builder.setResource(value)
}
/**
*
* resource holds the resource on which the Check was performed.
*
*
* .authzed.api.v1.ObjectReference resource = 1 [(.validate.rules) = { ... }
*/
public fun clearResource() {
_builder.clearResource()
}
/**
*
* resource holds the resource on which the Check was performed.
*
*
* .authzed.api.v1.ObjectReference resource = 1 [(.validate.rules) = { ... }
* @return Whether the resource field is set.
*/
public fun hasResource(): kotlin.Boolean {
return _builder.hasResource()
}
/**
*
* permission holds the name of the permission or relation on which the Check was performed.
*
*
* string permission = 2;
*/
public var permission: kotlin.String
@JvmName("getPermission")
get() = _builder.getPermission()
@JvmName("setPermission")
set(value) {
_builder.setPermission(value)
}
/**
*
* permission holds the name of the permission or relation on which the Check was performed.
*
*
* string permission = 2;
*/
public fun clearPermission() {
_builder.clearPermission()
}
/**
*
* permission_type holds information indicating whether it was a permission or relation.
*
*
* .authzed.api.v1.CheckDebugTrace.PermissionType permission_type = 3 [(.validate.rules) = { ... }
*/
public var permissionType: com.authzed.api.v1.Debug.CheckDebugTrace.PermissionType
@JvmName("getPermissionType")
get() = _builder.getPermissionType()
@JvmName("setPermissionType")
set(value) {
_builder.setPermissionType(value)
}
/**
*
* permission_type holds information indicating whether it was a permission or relation.
*
*
* .authzed.api.v1.CheckDebugTrace.PermissionType permission_type = 3 [(.validate.rules) = { ... }
*/
public fun clearPermissionType() {
_builder.clearPermissionType()
}
/**
*
* subject holds the subject on which the Check was performed. This will be static across all calls within
* the same Check tree.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
*/
public var subject: com.authzed.api.v1.Core.SubjectReference
@JvmName("getSubject")
get() = _builder.getSubject()
@JvmName("setSubject")
set(value) {
_builder.setSubject(value)
}
/**
*
* subject holds the subject on which the Check was performed. This will be static across all calls within
* the same Check tree.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
*/
public fun clearSubject() {
_builder.clearSubject()
}
/**
*
* subject holds the subject on which the Check was performed. This will be static across all calls within
* the same Check tree.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
* @return Whether the subject field is set.
*/
public fun hasSubject(): kotlin.Boolean {
return _builder.hasSubject()
}
/**
*
* result holds the result of the Check call.
*
*
* .authzed.api.v1.CheckDebugTrace.Permissionship result = 5 [(.validate.rules) = { ... }
*/
public var result: com.authzed.api.v1.Debug.CheckDebugTrace.Permissionship
@JvmName("getResult")
get() = _builder.getResult()
@JvmName("setResult")
set(value) {
_builder.setResult(value)
}
/**
*
* result holds the result of the Check call.
*
*
* .authzed.api.v1.CheckDebugTrace.Permissionship result = 5 [(.validate.rules) = { ... }
*/
public fun clearResult() {
_builder.clearResult()
}
/**
*
* caveat_evaluation_info holds information about the caveat evaluated for this step of the trace.
*
*
* .authzed.api.v1.CaveatEvalInfo caveat_evaluation_info = 8;
*/
public var caveatEvaluationInfo: com.authzed.api.v1.Debug.CaveatEvalInfo
@JvmName("getCaveatEvaluationInfo")
get() = _builder.getCaveatEvaluationInfo()
@JvmName("setCaveatEvaluationInfo")
set(value) {
_builder.setCaveatEvaluationInfo(value)
}
/**
*
* caveat_evaluation_info holds information about the caveat evaluated for this step of the trace.
*
*
* .authzed.api.v1.CaveatEvalInfo caveat_evaluation_info = 8;
*/
public fun clearCaveatEvaluationInfo() {
_builder.clearCaveatEvaluationInfo()
}
/**
*
* caveat_evaluation_info holds information about the caveat evaluated for this step of the trace.
*
*
* .authzed.api.v1.CaveatEvalInfo caveat_evaluation_info = 8;
* @return Whether the caveatEvaluationInfo field is set.
*/
public fun hasCaveatEvaluationInfo(): kotlin.Boolean {
return _builder.hasCaveatEvaluationInfo()
}
/**
*
* was_cached_result, if true, indicates that the result was found in the cache and returned directly.
*
*
* bool was_cached_result = 6;
*/
public var wasCachedResult: kotlin.Boolean
@JvmName("getWasCachedResult")
get() = _builder.getWasCachedResult()
@JvmName("setWasCachedResult")
set(value) {
_builder.setWasCachedResult(value)
}
/**
*
* was_cached_result, if true, indicates that the result was found in the cache and returned directly.
*
*
* bool was_cached_result = 6;
*/
public fun clearWasCachedResult() {
_builder.clearWasCachedResult()
}
/**
*
* was_cached_result, if true, indicates that the result was found in the cache and returned directly.
*
*
* bool was_cached_result = 6;
* @return Whether the wasCachedResult field is set.
*/
public fun hasWasCachedResult(): kotlin.Boolean {
return _builder.hasWasCachedResult()
}
/**
*
* sub_problems holds the sub problems that were executed to resolve the answer to this Check. An empty list
* and a permissionship of PERMISSIONSHIP_HAS_PERMISSION indicates the subject was found within this relation.
*
*
* .authzed.api.v1.CheckDebugTrace.SubProblems sub_problems = 7;
*/
public var subProblems: com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems
@JvmName("getSubProblems")
get() = _builder.getSubProblems()
@JvmName("setSubProblems")
set(value) {
_builder.setSubProblems(value)
}
/**
*
* sub_problems holds the sub problems that were executed to resolve the answer to this Check. An empty list
* and a permissionship of PERMISSIONSHIP_HAS_PERMISSION indicates the subject was found within this relation.
*
*
* .authzed.api.v1.CheckDebugTrace.SubProblems sub_problems = 7;
*/
public fun clearSubProblems() {
_builder.clearSubProblems()
}
/**
*
* sub_problems holds the sub problems that were executed to resolve the answer to this Check. An empty list
* and a permissionship of PERMISSIONSHIP_HAS_PERMISSION indicates the subject was found within this relation.
*
*
* .authzed.api.v1.CheckDebugTrace.SubProblems sub_problems = 7;
* @return Whether the subProblems field is set.
*/
public fun hasSubProblems(): kotlin.Boolean {
return _builder.hasSubProblems()
}
public val resolutionCase: com.authzed.api.v1.Debug.CheckDebugTrace.ResolutionCase
@JvmName("getResolutionCase")
get() = _builder.getResolutionCase()
public fun clearResolution() {
_builder.clearResolution()
}
}
@kotlin.jvm.JvmName("-initializesubProblems")
public inline fun subProblems(block: com.authzed.api.v1.CheckDebugTraceKt.SubProblemsKt.Dsl.() -> kotlin.Unit): com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems =
com.authzed.api.v1.CheckDebugTraceKt.SubProblemsKt.Dsl._create(com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems.newBuilder()).apply { block() }._build()
public object SubProblemsKt {
@kotlin.OptIn(com.google.protobuf.kotlin.OnlyForUseByGeneratedProtoCode::class)
@com.google.protobuf.kotlin.ProtoDslMarker
public class Dsl private constructor(
private val _builder: com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems.Builder
) {
public companion object {
@kotlin.jvm.JvmSynthetic
@kotlin.PublishedApi
internal fun _create(builder: com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems.Builder): Dsl = Dsl(builder)
}
@kotlin.jvm.JvmSynthetic
@kotlin.PublishedApi
internal fun _build(): com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems = _builder.build()
/**
* An uninstantiable, behaviorless type to represent the field in
* generics.
*/
@kotlin.OptIn(com.google.protobuf.kotlin.OnlyForUseByGeneratedProtoCode::class)
public class TracesProxy private constructor() : com.google.protobuf.kotlin.DslProxy()
/**
* repeated .authzed.api.v1.CheckDebugTrace traces = 1;
*/
public val traces: com.google.protobuf.kotlin.DslList
@kotlin.jvm.JvmSynthetic
get() = com.google.protobuf.kotlin.DslList(
_builder.getTracesList()
)
/**
* repeated .authzed.api.v1.CheckDebugTrace traces = 1;
* @param value The traces to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("addTraces")
public fun com.google.protobuf.kotlin.DslList.add(value: com.authzed.api.v1.Debug.CheckDebugTrace) {
_builder.addTraces(value)
}
/**
* repeated .authzed.api.v1.CheckDebugTrace traces = 1;
* @param value The traces to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("plusAssignTraces")
@Suppress("NOTHING_TO_INLINE")
public inline operator fun com.google.protobuf.kotlin.DslList.plusAssign(value: com.authzed.api.v1.Debug.CheckDebugTrace) {
add(value)
}
/**
* repeated .authzed.api.v1.CheckDebugTrace traces = 1;
* @param values The traces to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("addAllTraces")
public fun com.google.protobuf.kotlin.DslList.addAll(values: kotlin.collections.Iterable) {
_builder.addAllTraces(values)
}
/**
* repeated .authzed.api.v1.CheckDebugTrace traces = 1;
* @param values The traces to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("plusAssignAllTraces")
@Suppress("NOTHING_TO_INLINE")
public inline operator fun com.google.protobuf.kotlin.DslList.plusAssign(values: kotlin.collections.Iterable) {
addAll(values)
}
/**
* repeated .authzed.api.v1.CheckDebugTrace traces = 1;
* @param index The index to set the value at.
* @param value The traces to set.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("setTraces")
public operator fun com.google.protobuf.kotlin.DslList.set(index: kotlin.Int, value: com.authzed.api.v1.Debug.CheckDebugTrace) {
_builder.setTraces(index, value)
}
/**
* repeated .authzed.api.v1.CheckDebugTrace traces = 1;
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("clearTraces")
public fun com.google.protobuf.kotlin.DslList.clear() {
_builder.clearTraces()
}
}
}
}
@kotlin.jvm.JvmSynthetic
public inline fun com.authzed.api.v1.Debug.CheckDebugTrace.copy(block: com.authzed.api.v1.CheckDebugTraceKt.Dsl.() -> kotlin.Unit): com.authzed.api.v1.Debug.CheckDebugTrace =
com.authzed.api.v1.CheckDebugTraceKt.Dsl._create(this.toBuilder()).apply { block() }._build()
@kotlin.jvm.JvmSynthetic
public inline fun com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems.copy(block: com.authzed.api.v1.CheckDebugTraceKt.SubProblemsKt.Dsl.() -> kotlin.Unit): com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems =
com.authzed.api.v1.CheckDebugTraceKt.SubProblemsKt.Dsl._create(this.toBuilder()).apply { block() }._build()
public val com.authzed.api.v1.Debug.CheckDebugTraceOrBuilder.resourceOrNull: com.authzed.api.v1.Core.ObjectReference?
get() = if (hasResource()) getResource() else null
public val com.authzed.api.v1.Debug.CheckDebugTraceOrBuilder.subjectOrNull: com.authzed.api.v1.Core.SubjectReference?
get() = if (hasSubject()) getSubject() else null
public val com.authzed.api.v1.Debug.CheckDebugTraceOrBuilder.caveatEvaluationInfoOrNull: com.authzed.api.v1.Debug.CaveatEvalInfo?
get() = if (hasCaveatEvaluationInfo()) getCaveatEvaluationInfo() else null
public val com.authzed.api.v1.Debug.CheckDebugTraceOrBuilder.subProblemsOrNull: com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems?
get() = if (hasSubProblems()) getSubProblems() else null
© 2015 - 2025 Weber Informatics LLC | Privacy Policy