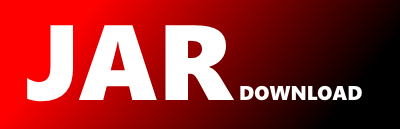
com.authzed.api.v1.Debug Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: authzed/api/v1/debug.proto
package com.authzed.api.v1;
public final class Debug {
private Debug() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
public interface DebugInformationOrBuilder extends
// @@protoc_insertion_point(interface_extends:authzed.api.v1.DebugInformation)
com.google.protobuf.MessageOrBuilder {
/**
*
* check holds debug information about a check request.
*
*
* .authzed.api.v1.CheckDebugTrace check = 1;
* @return Whether the check field is set.
*/
boolean hasCheck();
/**
*
* check holds debug information about a check request.
*
*
* .authzed.api.v1.CheckDebugTrace check = 1;
* @return The check.
*/
com.authzed.api.v1.Debug.CheckDebugTrace getCheck();
/**
*
* check holds debug information about a check request.
*
*
* .authzed.api.v1.CheckDebugTrace check = 1;
*/
com.authzed.api.v1.Debug.CheckDebugTraceOrBuilder getCheckOrBuilder();
/**
*
* schema_used holds the schema used for the request.
*
*
* string schema_used = 2;
* @return The schemaUsed.
*/
java.lang.String getSchemaUsed();
/**
*
* schema_used holds the schema used for the request.
*
*
* string schema_used = 2;
* @return The bytes for schemaUsed.
*/
com.google.protobuf.ByteString
getSchemaUsedBytes();
}
/**
*
* DebugInformation defines debug information returned by an API call in a footer when
* requested with a specific debugging header.
* The specific debug information returned will depend on the type of the API call made.
* See the github.com/authzed/authzed-go project for the specific header and footer names.
*
*
* Protobuf type {@code authzed.api.v1.DebugInformation}
*/
public static final class DebugInformation extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:authzed.api.v1.DebugInformation)
DebugInformationOrBuilder {
private static final long serialVersionUID = 0L;
// Use DebugInformation.newBuilder() to construct.
private DebugInformation(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private DebugInformation() {
schemaUsed_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new DebugInformation();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.Debug.internal_static_authzed_api_v1_DebugInformation_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.Debug.internal_static_authzed_api_v1_DebugInformation_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.Debug.DebugInformation.class, com.authzed.api.v1.Debug.DebugInformation.Builder.class);
}
public static final int CHECK_FIELD_NUMBER = 1;
private com.authzed.api.v1.Debug.CheckDebugTrace check_;
/**
*
* check holds debug information about a check request.
*
*
* .authzed.api.v1.CheckDebugTrace check = 1;
* @return Whether the check field is set.
*/
@java.lang.Override
public boolean hasCheck() {
return check_ != null;
}
/**
*
* check holds debug information about a check request.
*
*
* .authzed.api.v1.CheckDebugTrace check = 1;
* @return The check.
*/
@java.lang.Override
public com.authzed.api.v1.Debug.CheckDebugTrace getCheck() {
return check_ == null ? com.authzed.api.v1.Debug.CheckDebugTrace.getDefaultInstance() : check_;
}
/**
*
* check holds debug information about a check request.
*
*
* .authzed.api.v1.CheckDebugTrace check = 1;
*/
@java.lang.Override
public com.authzed.api.v1.Debug.CheckDebugTraceOrBuilder getCheckOrBuilder() {
return check_ == null ? com.authzed.api.v1.Debug.CheckDebugTrace.getDefaultInstance() : check_;
}
public static final int SCHEMA_USED_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object schemaUsed_ = "";
/**
*
* schema_used holds the schema used for the request.
*
*
* string schema_used = 2;
* @return The schemaUsed.
*/
@java.lang.Override
public java.lang.String getSchemaUsed() {
java.lang.Object ref = schemaUsed_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
schemaUsed_ = s;
return s;
}
}
/**
*
* schema_used holds the schema used for the request.
*
*
* string schema_used = 2;
* @return The bytes for schemaUsed.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getSchemaUsedBytes() {
java.lang.Object ref = schemaUsed_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
schemaUsed_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (check_ != null) {
output.writeMessage(1, getCheck());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(schemaUsed_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, schemaUsed_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (check_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getCheck());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(schemaUsed_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, schemaUsed_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.authzed.api.v1.Debug.DebugInformation)) {
return super.equals(obj);
}
com.authzed.api.v1.Debug.DebugInformation other = (com.authzed.api.v1.Debug.DebugInformation) obj;
if (hasCheck() != other.hasCheck()) return false;
if (hasCheck()) {
if (!getCheck()
.equals(other.getCheck())) return false;
}
if (!getSchemaUsed()
.equals(other.getSchemaUsed())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasCheck()) {
hash = (37 * hash) + CHECK_FIELD_NUMBER;
hash = (53 * hash) + getCheck().hashCode();
}
hash = (37 * hash) + SCHEMA_USED_FIELD_NUMBER;
hash = (53 * hash) + getSchemaUsed().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.authzed.api.v1.Debug.DebugInformation parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.Debug.DebugInformation parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.Debug.DebugInformation parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.Debug.DebugInformation parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.Debug.DebugInformation parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.Debug.DebugInformation parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.Debug.DebugInformation parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.Debug.DebugInformation parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.Debug.DebugInformation parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.authzed.api.v1.Debug.DebugInformation parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.Debug.DebugInformation parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.Debug.DebugInformation parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.authzed.api.v1.Debug.DebugInformation prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* DebugInformation defines debug information returned by an API call in a footer when
* requested with a specific debugging header.
* The specific debug information returned will depend on the type of the API call made.
* See the github.com/authzed/authzed-go project for the specific header and footer names.
*
*
* Protobuf type {@code authzed.api.v1.DebugInformation}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:authzed.api.v1.DebugInformation)
com.authzed.api.v1.Debug.DebugInformationOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.Debug.internal_static_authzed_api_v1_DebugInformation_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.Debug.internal_static_authzed_api_v1_DebugInformation_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.Debug.DebugInformation.class, com.authzed.api.v1.Debug.DebugInformation.Builder.class);
}
// Construct using com.authzed.api.v1.Debug.DebugInformation.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
check_ = null;
if (checkBuilder_ != null) {
checkBuilder_.dispose();
checkBuilder_ = null;
}
schemaUsed_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.authzed.api.v1.Debug.internal_static_authzed_api_v1_DebugInformation_descriptor;
}
@java.lang.Override
public com.authzed.api.v1.Debug.DebugInformation getDefaultInstanceForType() {
return com.authzed.api.v1.Debug.DebugInformation.getDefaultInstance();
}
@java.lang.Override
public com.authzed.api.v1.Debug.DebugInformation build() {
com.authzed.api.v1.Debug.DebugInformation result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.authzed.api.v1.Debug.DebugInformation buildPartial() {
com.authzed.api.v1.Debug.DebugInformation result = new com.authzed.api.v1.Debug.DebugInformation(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.authzed.api.v1.Debug.DebugInformation result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.check_ = checkBuilder_ == null
? check_
: checkBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.schemaUsed_ = schemaUsed_;
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.authzed.api.v1.Debug.DebugInformation) {
return mergeFrom((com.authzed.api.v1.Debug.DebugInformation)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.authzed.api.v1.Debug.DebugInformation other) {
if (other == com.authzed.api.v1.Debug.DebugInformation.getDefaultInstance()) return this;
if (other.hasCheck()) {
mergeCheck(other.getCheck());
}
if (!other.getSchemaUsed().isEmpty()) {
schemaUsed_ = other.schemaUsed_;
bitField0_ |= 0x00000002;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
input.readMessage(
getCheckFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
schemaUsed_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 18
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.authzed.api.v1.Debug.CheckDebugTrace check_;
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Debug.CheckDebugTrace, com.authzed.api.v1.Debug.CheckDebugTrace.Builder, com.authzed.api.v1.Debug.CheckDebugTraceOrBuilder> checkBuilder_;
/**
*
* check holds debug information about a check request.
*
*
* .authzed.api.v1.CheckDebugTrace check = 1;
* @return Whether the check field is set.
*/
public boolean hasCheck() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* check holds debug information about a check request.
*
*
* .authzed.api.v1.CheckDebugTrace check = 1;
* @return The check.
*/
public com.authzed.api.v1.Debug.CheckDebugTrace getCheck() {
if (checkBuilder_ == null) {
return check_ == null ? com.authzed.api.v1.Debug.CheckDebugTrace.getDefaultInstance() : check_;
} else {
return checkBuilder_.getMessage();
}
}
/**
*
* check holds debug information about a check request.
*
*
* .authzed.api.v1.CheckDebugTrace check = 1;
*/
public Builder setCheck(com.authzed.api.v1.Debug.CheckDebugTrace value) {
if (checkBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
check_ = value;
} else {
checkBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* check holds debug information about a check request.
*
*
* .authzed.api.v1.CheckDebugTrace check = 1;
*/
public Builder setCheck(
com.authzed.api.v1.Debug.CheckDebugTrace.Builder builderForValue) {
if (checkBuilder_ == null) {
check_ = builderForValue.build();
} else {
checkBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* check holds debug information about a check request.
*
*
* .authzed.api.v1.CheckDebugTrace check = 1;
*/
public Builder mergeCheck(com.authzed.api.v1.Debug.CheckDebugTrace value) {
if (checkBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0) &&
check_ != null &&
check_ != com.authzed.api.v1.Debug.CheckDebugTrace.getDefaultInstance()) {
getCheckBuilder().mergeFrom(value);
} else {
check_ = value;
}
} else {
checkBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* check holds debug information about a check request.
*
*
* .authzed.api.v1.CheckDebugTrace check = 1;
*/
public Builder clearCheck() {
bitField0_ = (bitField0_ & ~0x00000001);
check_ = null;
if (checkBuilder_ != null) {
checkBuilder_.dispose();
checkBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* check holds debug information about a check request.
*
*
* .authzed.api.v1.CheckDebugTrace check = 1;
*/
public com.authzed.api.v1.Debug.CheckDebugTrace.Builder getCheckBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getCheckFieldBuilder().getBuilder();
}
/**
*
* check holds debug information about a check request.
*
*
* .authzed.api.v1.CheckDebugTrace check = 1;
*/
public com.authzed.api.v1.Debug.CheckDebugTraceOrBuilder getCheckOrBuilder() {
if (checkBuilder_ != null) {
return checkBuilder_.getMessageOrBuilder();
} else {
return check_ == null ?
com.authzed.api.v1.Debug.CheckDebugTrace.getDefaultInstance() : check_;
}
}
/**
*
* check holds debug information about a check request.
*
*
* .authzed.api.v1.CheckDebugTrace check = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Debug.CheckDebugTrace, com.authzed.api.v1.Debug.CheckDebugTrace.Builder, com.authzed.api.v1.Debug.CheckDebugTraceOrBuilder>
getCheckFieldBuilder() {
if (checkBuilder_ == null) {
checkBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Debug.CheckDebugTrace, com.authzed.api.v1.Debug.CheckDebugTrace.Builder, com.authzed.api.v1.Debug.CheckDebugTraceOrBuilder>(
getCheck(),
getParentForChildren(),
isClean());
check_ = null;
}
return checkBuilder_;
}
private java.lang.Object schemaUsed_ = "";
/**
*
* schema_used holds the schema used for the request.
*
*
* string schema_used = 2;
* @return The schemaUsed.
*/
public java.lang.String getSchemaUsed() {
java.lang.Object ref = schemaUsed_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
schemaUsed_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* schema_used holds the schema used for the request.
*
*
* string schema_used = 2;
* @return The bytes for schemaUsed.
*/
public com.google.protobuf.ByteString
getSchemaUsedBytes() {
java.lang.Object ref = schemaUsed_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
schemaUsed_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* schema_used holds the schema used for the request.
*
*
* string schema_used = 2;
* @param value The schemaUsed to set.
* @return This builder for chaining.
*/
public Builder setSchemaUsed(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
schemaUsed_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* schema_used holds the schema used for the request.
*
*
* string schema_used = 2;
* @return This builder for chaining.
*/
public Builder clearSchemaUsed() {
schemaUsed_ = getDefaultInstance().getSchemaUsed();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
* schema_used holds the schema used for the request.
*
*
* string schema_used = 2;
* @param value The bytes for schemaUsed to set.
* @return This builder for chaining.
*/
public Builder setSchemaUsedBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
schemaUsed_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:authzed.api.v1.DebugInformation)
}
// @@protoc_insertion_point(class_scope:authzed.api.v1.DebugInformation)
private static final com.authzed.api.v1.Debug.DebugInformation DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.authzed.api.v1.Debug.DebugInformation();
}
public static com.authzed.api.v1.Debug.DebugInformation getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public DebugInformation parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.authzed.api.v1.Debug.DebugInformation getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CheckDebugTraceOrBuilder extends
// @@protoc_insertion_point(interface_extends:authzed.api.v1.CheckDebugTrace)
com.google.protobuf.MessageOrBuilder {
/**
*
* resource holds the resource on which the Check was performed.
*
*
* .authzed.api.v1.ObjectReference resource = 1 [(.validate.rules) = { ... }
* @return Whether the resource field is set.
*/
boolean hasResource();
/**
*
* resource holds the resource on which the Check was performed.
*
*
* .authzed.api.v1.ObjectReference resource = 1 [(.validate.rules) = { ... }
* @return The resource.
*/
com.authzed.api.v1.Core.ObjectReference getResource();
/**
*
* resource holds the resource on which the Check was performed.
*
*
* .authzed.api.v1.ObjectReference resource = 1 [(.validate.rules) = { ... }
*/
com.authzed.api.v1.Core.ObjectReferenceOrBuilder getResourceOrBuilder();
/**
*
* permission holds the name of the permission or relation on which the Check was performed.
*
*
* string permission = 2;
* @return The permission.
*/
java.lang.String getPermission();
/**
*
* permission holds the name of the permission or relation on which the Check was performed.
*
*
* string permission = 2;
* @return The bytes for permission.
*/
com.google.protobuf.ByteString
getPermissionBytes();
/**
*
* permission_type holds information indicating whether it was a permission or relation.
*
*
* .authzed.api.v1.CheckDebugTrace.PermissionType permission_type = 3 [(.validate.rules) = { ... }
* @return The enum numeric value on the wire for permissionType.
*/
int getPermissionTypeValue();
/**
*
* permission_type holds information indicating whether it was a permission or relation.
*
*
* .authzed.api.v1.CheckDebugTrace.PermissionType permission_type = 3 [(.validate.rules) = { ... }
* @return The permissionType.
*/
com.authzed.api.v1.Debug.CheckDebugTrace.PermissionType getPermissionType();
/**
*
* subject holds the subject on which the Check was performed. This will be static across all calls within
* the same Check tree.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
* @return Whether the subject field is set.
*/
boolean hasSubject();
/**
*
* subject holds the subject on which the Check was performed. This will be static across all calls within
* the same Check tree.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
* @return The subject.
*/
com.authzed.api.v1.Core.SubjectReference getSubject();
/**
*
* subject holds the subject on which the Check was performed. This will be static across all calls within
* the same Check tree.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
*/
com.authzed.api.v1.Core.SubjectReferenceOrBuilder getSubjectOrBuilder();
/**
*
* result holds the result of the Check call.
*
*
* .authzed.api.v1.CheckDebugTrace.Permissionship result = 5 [(.validate.rules) = { ... }
* @return The enum numeric value on the wire for result.
*/
int getResultValue();
/**
*
* result holds the result of the Check call.
*
*
* .authzed.api.v1.CheckDebugTrace.Permissionship result = 5 [(.validate.rules) = { ... }
* @return The result.
*/
com.authzed.api.v1.Debug.CheckDebugTrace.Permissionship getResult();
/**
*
* caveat_evaluation_info holds information about the caveat evaluated for this step of the trace.
*
*
* .authzed.api.v1.CaveatEvalInfo caveat_evaluation_info = 8;
* @return Whether the caveatEvaluationInfo field is set.
*/
boolean hasCaveatEvaluationInfo();
/**
*
* caveat_evaluation_info holds information about the caveat evaluated for this step of the trace.
*
*
* .authzed.api.v1.CaveatEvalInfo caveat_evaluation_info = 8;
* @return The caveatEvaluationInfo.
*/
com.authzed.api.v1.Debug.CaveatEvalInfo getCaveatEvaluationInfo();
/**
*
* caveat_evaluation_info holds information about the caveat evaluated for this step of the trace.
*
*
* .authzed.api.v1.CaveatEvalInfo caveat_evaluation_info = 8;
*/
com.authzed.api.v1.Debug.CaveatEvalInfoOrBuilder getCaveatEvaluationInfoOrBuilder();
/**
*
* was_cached_result, if true, indicates that the result was found in the cache and returned directly.
*
*
* bool was_cached_result = 6;
* @return Whether the wasCachedResult field is set.
*/
boolean hasWasCachedResult();
/**
*
* was_cached_result, if true, indicates that the result was found in the cache and returned directly.
*
*
* bool was_cached_result = 6;
* @return The wasCachedResult.
*/
boolean getWasCachedResult();
/**
*
* sub_problems holds the sub problems that were executed to resolve the answer to this Check. An empty list
* and a permissionship of PERMISSIONSHIP_HAS_PERMISSION indicates the subject was found within this relation.
*
*
* .authzed.api.v1.CheckDebugTrace.SubProblems sub_problems = 7;
* @return Whether the subProblems field is set.
*/
boolean hasSubProblems();
/**
*
* sub_problems holds the sub problems that were executed to resolve the answer to this Check. An empty list
* and a permissionship of PERMISSIONSHIP_HAS_PERMISSION indicates the subject was found within this relation.
*
*
* .authzed.api.v1.CheckDebugTrace.SubProblems sub_problems = 7;
* @return The subProblems.
*/
com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems getSubProblems();
/**
*
* sub_problems holds the sub problems that were executed to resolve the answer to this Check. An empty list
* and a permissionship of PERMISSIONSHIP_HAS_PERMISSION indicates the subject was found within this relation.
*
*
* .authzed.api.v1.CheckDebugTrace.SubProblems sub_problems = 7;
*/
com.authzed.api.v1.Debug.CheckDebugTrace.SubProblemsOrBuilder getSubProblemsOrBuilder();
public com.authzed.api.v1.Debug.CheckDebugTrace.ResolutionCase getResolutionCase();
}
/**
*
* CheckDebugTrace is a recursive trace of the requests made for resolving a CheckPermission
* API call.
*
*
* Protobuf type {@code authzed.api.v1.CheckDebugTrace}
*/
public static final class CheckDebugTrace extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:authzed.api.v1.CheckDebugTrace)
CheckDebugTraceOrBuilder {
private static final long serialVersionUID = 0L;
// Use CheckDebugTrace.newBuilder() to construct.
private CheckDebugTrace(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CheckDebugTrace() {
permission_ = "";
permissionType_ = 0;
result_ = 0;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CheckDebugTrace();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.Debug.internal_static_authzed_api_v1_CheckDebugTrace_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.Debug.internal_static_authzed_api_v1_CheckDebugTrace_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.Debug.CheckDebugTrace.class, com.authzed.api.v1.Debug.CheckDebugTrace.Builder.class);
}
/**
* Protobuf enum {@code authzed.api.v1.CheckDebugTrace.PermissionType}
*/
public enum PermissionType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* PERMISSION_TYPE_UNSPECIFIED = 0;
*/
PERMISSION_TYPE_UNSPECIFIED(0),
/**
* PERMISSION_TYPE_RELATION = 1;
*/
PERMISSION_TYPE_RELATION(1),
/**
* PERMISSION_TYPE_PERMISSION = 2;
*/
PERMISSION_TYPE_PERMISSION(2),
UNRECOGNIZED(-1),
;
/**
* PERMISSION_TYPE_UNSPECIFIED = 0;
*/
public static final int PERMISSION_TYPE_UNSPECIFIED_VALUE = 0;
/**
* PERMISSION_TYPE_RELATION = 1;
*/
public static final int PERMISSION_TYPE_RELATION_VALUE = 1;
/**
* PERMISSION_TYPE_PERMISSION = 2;
*/
public static final int PERMISSION_TYPE_PERMISSION_VALUE = 2;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static PermissionType valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static PermissionType forNumber(int value) {
switch (value) {
case 0: return PERMISSION_TYPE_UNSPECIFIED;
case 1: return PERMISSION_TYPE_RELATION;
case 2: return PERMISSION_TYPE_PERMISSION;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
PermissionType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public PermissionType findValueByNumber(int number) {
return PermissionType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.authzed.api.v1.Debug.CheckDebugTrace.getDescriptor().getEnumTypes().get(0);
}
private static final PermissionType[] VALUES = values();
public static PermissionType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private PermissionType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:authzed.api.v1.CheckDebugTrace.PermissionType)
}
/**
* Protobuf enum {@code authzed.api.v1.CheckDebugTrace.Permissionship}
*/
public enum Permissionship
implements com.google.protobuf.ProtocolMessageEnum {
/**
* PERMISSIONSHIP_UNSPECIFIED = 0;
*/
PERMISSIONSHIP_UNSPECIFIED(0),
/**
* PERMISSIONSHIP_NO_PERMISSION = 1;
*/
PERMISSIONSHIP_NO_PERMISSION(1),
/**
* PERMISSIONSHIP_HAS_PERMISSION = 2;
*/
PERMISSIONSHIP_HAS_PERMISSION(2),
/**
* PERMISSIONSHIP_CONDITIONAL_PERMISSION = 3;
*/
PERMISSIONSHIP_CONDITIONAL_PERMISSION(3),
UNRECOGNIZED(-1),
;
/**
* PERMISSIONSHIP_UNSPECIFIED = 0;
*/
public static final int PERMISSIONSHIP_UNSPECIFIED_VALUE = 0;
/**
* PERMISSIONSHIP_NO_PERMISSION = 1;
*/
public static final int PERMISSIONSHIP_NO_PERMISSION_VALUE = 1;
/**
* PERMISSIONSHIP_HAS_PERMISSION = 2;
*/
public static final int PERMISSIONSHIP_HAS_PERMISSION_VALUE = 2;
/**
* PERMISSIONSHIP_CONDITIONAL_PERMISSION = 3;
*/
public static final int PERMISSIONSHIP_CONDITIONAL_PERMISSION_VALUE = 3;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Permissionship valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static Permissionship forNumber(int value) {
switch (value) {
case 0: return PERMISSIONSHIP_UNSPECIFIED;
case 1: return PERMISSIONSHIP_NO_PERMISSION;
case 2: return PERMISSIONSHIP_HAS_PERMISSION;
case 3: return PERMISSIONSHIP_CONDITIONAL_PERMISSION;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
Permissionship> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Permissionship findValueByNumber(int number) {
return Permissionship.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.authzed.api.v1.Debug.CheckDebugTrace.getDescriptor().getEnumTypes().get(1);
}
private static final Permissionship[] VALUES = values();
public static Permissionship valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private Permissionship(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:authzed.api.v1.CheckDebugTrace.Permissionship)
}
public interface SubProblemsOrBuilder extends
// @@protoc_insertion_point(interface_extends:authzed.api.v1.CheckDebugTrace.SubProblems)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .authzed.api.v1.CheckDebugTrace traces = 1;
*/
java.util.List
getTracesList();
/**
* repeated .authzed.api.v1.CheckDebugTrace traces = 1;
*/
com.authzed.api.v1.Debug.CheckDebugTrace getTraces(int index);
/**
* repeated .authzed.api.v1.CheckDebugTrace traces = 1;
*/
int getTracesCount();
/**
* repeated .authzed.api.v1.CheckDebugTrace traces = 1;
*/
java.util.List extends com.authzed.api.v1.Debug.CheckDebugTraceOrBuilder>
getTracesOrBuilderList();
/**
* repeated .authzed.api.v1.CheckDebugTrace traces = 1;
*/
com.authzed.api.v1.Debug.CheckDebugTraceOrBuilder getTracesOrBuilder(
int index);
}
/**
* Protobuf type {@code authzed.api.v1.CheckDebugTrace.SubProblems}
*/
public static final class SubProblems extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:authzed.api.v1.CheckDebugTrace.SubProblems)
SubProblemsOrBuilder {
private static final long serialVersionUID = 0L;
// Use SubProblems.newBuilder() to construct.
private SubProblems(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private SubProblems() {
traces_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new SubProblems();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.Debug.internal_static_authzed_api_v1_CheckDebugTrace_SubProblems_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.Debug.internal_static_authzed_api_v1_CheckDebugTrace_SubProblems_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems.class, com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems.Builder.class);
}
public static final int TRACES_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private java.util.List traces_;
/**
* repeated .authzed.api.v1.CheckDebugTrace traces = 1;
*/
@java.lang.Override
public java.util.List getTracesList() {
return traces_;
}
/**
* repeated .authzed.api.v1.CheckDebugTrace traces = 1;
*/
@java.lang.Override
public java.util.List extends com.authzed.api.v1.Debug.CheckDebugTraceOrBuilder>
getTracesOrBuilderList() {
return traces_;
}
/**
* repeated .authzed.api.v1.CheckDebugTrace traces = 1;
*/
@java.lang.Override
public int getTracesCount() {
return traces_.size();
}
/**
* repeated .authzed.api.v1.CheckDebugTrace traces = 1;
*/
@java.lang.Override
public com.authzed.api.v1.Debug.CheckDebugTrace getTraces(int index) {
return traces_.get(index);
}
/**
* repeated .authzed.api.v1.CheckDebugTrace traces = 1;
*/
@java.lang.Override
public com.authzed.api.v1.Debug.CheckDebugTraceOrBuilder getTracesOrBuilder(
int index) {
return traces_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < traces_.size(); i++) {
output.writeMessage(1, traces_.get(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < traces_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, traces_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems)) {
return super.equals(obj);
}
com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems other = (com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems) obj;
if (!getTracesList()
.equals(other.getTracesList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getTracesCount() > 0) {
hash = (37 * hash) + TRACES_FIELD_NUMBER;
hash = (53 * hash) + getTracesList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code authzed.api.v1.CheckDebugTrace.SubProblems}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:authzed.api.v1.CheckDebugTrace.SubProblems)
com.authzed.api.v1.Debug.CheckDebugTrace.SubProblemsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.Debug.internal_static_authzed_api_v1_CheckDebugTrace_SubProblems_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.Debug.internal_static_authzed_api_v1_CheckDebugTrace_SubProblems_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems.class, com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems.Builder.class);
}
// Construct using com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
if (tracesBuilder_ == null) {
traces_ = java.util.Collections.emptyList();
} else {
traces_ = null;
tracesBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.authzed.api.v1.Debug.internal_static_authzed_api_v1_CheckDebugTrace_SubProblems_descriptor;
}
@java.lang.Override
public com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems getDefaultInstanceForType() {
return com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems.getDefaultInstance();
}
@java.lang.Override
public com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems build() {
com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems buildPartial() {
com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems result = new com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems result) {
if (tracesBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
traces_ = java.util.Collections.unmodifiableList(traces_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.traces_ = traces_;
} else {
result.traces_ = tracesBuilder_.build();
}
}
private void buildPartial0(com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems result) {
int from_bitField0_ = bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems) {
return mergeFrom((com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems other) {
if (other == com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems.getDefaultInstance()) return this;
if (tracesBuilder_ == null) {
if (!other.traces_.isEmpty()) {
if (traces_.isEmpty()) {
traces_ = other.traces_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureTracesIsMutable();
traces_.addAll(other.traces_);
}
onChanged();
}
} else {
if (!other.traces_.isEmpty()) {
if (tracesBuilder_.isEmpty()) {
tracesBuilder_.dispose();
tracesBuilder_ = null;
traces_ = other.traces_;
bitField0_ = (bitField0_ & ~0x00000001);
tracesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getTracesFieldBuilder() : null;
} else {
tracesBuilder_.addAllMessages(other.traces_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.authzed.api.v1.Debug.CheckDebugTrace m =
input.readMessage(
com.authzed.api.v1.Debug.CheckDebugTrace.parser(),
extensionRegistry);
if (tracesBuilder_ == null) {
ensureTracesIsMutable();
traces_.add(m);
} else {
tracesBuilder_.addMessage(m);
}
break;
} // case 10
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.util.List traces_ =
java.util.Collections.emptyList();
private void ensureTracesIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
traces_ = new java.util.ArrayList(traces_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.authzed.api.v1.Debug.CheckDebugTrace, com.authzed.api.v1.Debug.CheckDebugTrace.Builder, com.authzed.api.v1.Debug.CheckDebugTraceOrBuilder> tracesBuilder_;
/**
* repeated .authzed.api.v1.CheckDebugTrace traces = 1;
*/
public java.util.List getTracesList() {
if (tracesBuilder_ == null) {
return java.util.Collections.unmodifiableList(traces_);
} else {
return tracesBuilder_.getMessageList();
}
}
/**
* repeated .authzed.api.v1.CheckDebugTrace traces = 1;
*/
public int getTracesCount() {
if (tracesBuilder_ == null) {
return traces_.size();
} else {
return tracesBuilder_.getCount();
}
}
/**
* repeated .authzed.api.v1.CheckDebugTrace traces = 1;
*/
public com.authzed.api.v1.Debug.CheckDebugTrace getTraces(int index) {
if (tracesBuilder_ == null) {
return traces_.get(index);
} else {
return tracesBuilder_.getMessage(index);
}
}
/**
* repeated .authzed.api.v1.CheckDebugTrace traces = 1;
*/
public Builder setTraces(
int index, com.authzed.api.v1.Debug.CheckDebugTrace value) {
if (tracesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTracesIsMutable();
traces_.set(index, value);
onChanged();
} else {
tracesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .authzed.api.v1.CheckDebugTrace traces = 1;
*/
public Builder setTraces(
int index, com.authzed.api.v1.Debug.CheckDebugTrace.Builder builderForValue) {
if (tracesBuilder_ == null) {
ensureTracesIsMutable();
traces_.set(index, builderForValue.build());
onChanged();
} else {
tracesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .authzed.api.v1.CheckDebugTrace traces = 1;
*/
public Builder addTraces(com.authzed.api.v1.Debug.CheckDebugTrace value) {
if (tracesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTracesIsMutable();
traces_.add(value);
onChanged();
} else {
tracesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .authzed.api.v1.CheckDebugTrace traces = 1;
*/
public Builder addTraces(
int index, com.authzed.api.v1.Debug.CheckDebugTrace value) {
if (tracesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTracesIsMutable();
traces_.add(index, value);
onChanged();
} else {
tracesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .authzed.api.v1.CheckDebugTrace traces = 1;
*/
public Builder addTraces(
com.authzed.api.v1.Debug.CheckDebugTrace.Builder builderForValue) {
if (tracesBuilder_ == null) {
ensureTracesIsMutable();
traces_.add(builderForValue.build());
onChanged();
} else {
tracesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .authzed.api.v1.CheckDebugTrace traces = 1;
*/
public Builder addTraces(
int index, com.authzed.api.v1.Debug.CheckDebugTrace.Builder builderForValue) {
if (tracesBuilder_ == null) {
ensureTracesIsMutable();
traces_.add(index, builderForValue.build());
onChanged();
} else {
tracesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .authzed.api.v1.CheckDebugTrace traces = 1;
*/
public Builder addAllTraces(
java.lang.Iterable extends com.authzed.api.v1.Debug.CheckDebugTrace> values) {
if (tracesBuilder_ == null) {
ensureTracesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, traces_);
onChanged();
} else {
tracesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .authzed.api.v1.CheckDebugTrace traces = 1;
*/
public Builder clearTraces() {
if (tracesBuilder_ == null) {
traces_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
tracesBuilder_.clear();
}
return this;
}
/**
* repeated .authzed.api.v1.CheckDebugTrace traces = 1;
*/
public Builder removeTraces(int index) {
if (tracesBuilder_ == null) {
ensureTracesIsMutable();
traces_.remove(index);
onChanged();
} else {
tracesBuilder_.remove(index);
}
return this;
}
/**
* repeated .authzed.api.v1.CheckDebugTrace traces = 1;
*/
public com.authzed.api.v1.Debug.CheckDebugTrace.Builder getTracesBuilder(
int index) {
return getTracesFieldBuilder().getBuilder(index);
}
/**
* repeated .authzed.api.v1.CheckDebugTrace traces = 1;
*/
public com.authzed.api.v1.Debug.CheckDebugTraceOrBuilder getTracesOrBuilder(
int index) {
if (tracesBuilder_ == null) {
return traces_.get(index); } else {
return tracesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .authzed.api.v1.CheckDebugTrace traces = 1;
*/
public java.util.List extends com.authzed.api.v1.Debug.CheckDebugTraceOrBuilder>
getTracesOrBuilderList() {
if (tracesBuilder_ != null) {
return tracesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(traces_);
}
}
/**
* repeated .authzed.api.v1.CheckDebugTrace traces = 1;
*/
public com.authzed.api.v1.Debug.CheckDebugTrace.Builder addTracesBuilder() {
return getTracesFieldBuilder().addBuilder(
com.authzed.api.v1.Debug.CheckDebugTrace.getDefaultInstance());
}
/**
* repeated .authzed.api.v1.CheckDebugTrace traces = 1;
*/
public com.authzed.api.v1.Debug.CheckDebugTrace.Builder addTracesBuilder(
int index) {
return getTracesFieldBuilder().addBuilder(
index, com.authzed.api.v1.Debug.CheckDebugTrace.getDefaultInstance());
}
/**
* repeated .authzed.api.v1.CheckDebugTrace traces = 1;
*/
public java.util.List
getTracesBuilderList() {
return getTracesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.authzed.api.v1.Debug.CheckDebugTrace, com.authzed.api.v1.Debug.CheckDebugTrace.Builder, com.authzed.api.v1.Debug.CheckDebugTraceOrBuilder>
getTracesFieldBuilder() {
if (tracesBuilder_ == null) {
tracesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.authzed.api.v1.Debug.CheckDebugTrace, com.authzed.api.v1.Debug.CheckDebugTrace.Builder, com.authzed.api.v1.Debug.CheckDebugTraceOrBuilder>(
traces_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
traces_ = null;
}
return tracesBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:authzed.api.v1.CheckDebugTrace.SubProblems)
}
// @@protoc_insertion_point(class_scope:authzed.api.v1.CheckDebugTrace.SubProblems)
private static final com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems();
}
public static com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public SubProblems parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int resolutionCase_ = 0;
private java.lang.Object resolution_;
public enum ResolutionCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
WAS_CACHED_RESULT(6),
SUB_PROBLEMS(7),
RESOLUTION_NOT_SET(0);
private final int value;
private ResolutionCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ResolutionCase valueOf(int value) {
return forNumber(value);
}
public static ResolutionCase forNumber(int value) {
switch (value) {
case 6: return WAS_CACHED_RESULT;
case 7: return SUB_PROBLEMS;
case 0: return RESOLUTION_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public ResolutionCase
getResolutionCase() {
return ResolutionCase.forNumber(
resolutionCase_);
}
public static final int RESOURCE_FIELD_NUMBER = 1;
private com.authzed.api.v1.Core.ObjectReference resource_;
/**
*
* resource holds the resource on which the Check was performed.
*
*
* .authzed.api.v1.ObjectReference resource = 1 [(.validate.rules) = { ... }
* @return Whether the resource field is set.
*/
@java.lang.Override
public boolean hasResource() {
return resource_ != null;
}
/**
*
* resource holds the resource on which the Check was performed.
*
*
* .authzed.api.v1.ObjectReference resource = 1 [(.validate.rules) = { ... }
* @return The resource.
*/
@java.lang.Override
public com.authzed.api.v1.Core.ObjectReference getResource() {
return resource_ == null ? com.authzed.api.v1.Core.ObjectReference.getDefaultInstance() : resource_;
}
/**
*
* resource holds the resource on which the Check was performed.
*
*
* .authzed.api.v1.ObjectReference resource = 1 [(.validate.rules) = { ... }
*/
@java.lang.Override
public com.authzed.api.v1.Core.ObjectReferenceOrBuilder getResourceOrBuilder() {
return resource_ == null ? com.authzed.api.v1.Core.ObjectReference.getDefaultInstance() : resource_;
}
public static final int PERMISSION_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object permission_ = "";
/**
*
* permission holds the name of the permission or relation on which the Check was performed.
*
*
* string permission = 2;
* @return The permission.
*/
@java.lang.Override
public java.lang.String getPermission() {
java.lang.Object ref = permission_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
permission_ = s;
return s;
}
}
/**
*
* permission holds the name of the permission or relation on which the Check was performed.
*
*
* string permission = 2;
* @return The bytes for permission.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getPermissionBytes() {
java.lang.Object ref = permission_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
permission_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PERMISSION_TYPE_FIELD_NUMBER = 3;
private int permissionType_ = 0;
/**
*
* permission_type holds information indicating whether it was a permission or relation.
*
*
* .authzed.api.v1.CheckDebugTrace.PermissionType permission_type = 3 [(.validate.rules) = { ... }
* @return The enum numeric value on the wire for permissionType.
*/
@java.lang.Override public int getPermissionTypeValue() {
return permissionType_;
}
/**
*
* permission_type holds information indicating whether it was a permission or relation.
*
*
* .authzed.api.v1.CheckDebugTrace.PermissionType permission_type = 3 [(.validate.rules) = { ... }
* @return The permissionType.
*/
@java.lang.Override public com.authzed.api.v1.Debug.CheckDebugTrace.PermissionType getPermissionType() {
com.authzed.api.v1.Debug.CheckDebugTrace.PermissionType result = com.authzed.api.v1.Debug.CheckDebugTrace.PermissionType.forNumber(permissionType_);
return result == null ? com.authzed.api.v1.Debug.CheckDebugTrace.PermissionType.UNRECOGNIZED : result;
}
public static final int SUBJECT_FIELD_NUMBER = 4;
private com.authzed.api.v1.Core.SubjectReference subject_;
/**
*
* subject holds the subject on which the Check was performed. This will be static across all calls within
* the same Check tree.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
* @return Whether the subject field is set.
*/
@java.lang.Override
public boolean hasSubject() {
return subject_ != null;
}
/**
*
* subject holds the subject on which the Check was performed. This will be static across all calls within
* the same Check tree.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
* @return The subject.
*/
@java.lang.Override
public com.authzed.api.v1.Core.SubjectReference getSubject() {
return subject_ == null ? com.authzed.api.v1.Core.SubjectReference.getDefaultInstance() : subject_;
}
/**
*
* subject holds the subject on which the Check was performed. This will be static across all calls within
* the same Check tree.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
*/
@java.lang.Override
public com.authzed.api.v1.Core.SubjectReferenceOrBuilder getSubjectOrBuilder() {
return subject_ == null ? com.authzed.api.v1.Core.SubjectReference.getDefaultInstance() : subject_;
}
public static final int RESULT_FIELD_NUMBER = 5;
private int result_ = 0;
/**
*
* result holds the result of the Check call.
*
*
* .authzed.api.v1.CheckDebugTrace.Permissionship result = 5 [(.validate.rules) = { ... }
* @return The enum numeric value on the wire for result.
*/
@java.lang.Override public int getResultValue() {
return result_;
}
/**
*
* result holds the result of the Check call.
*
*
* .authzed.api.v1.CheckDebugTrace.Permissionship result = 5 [(.validate.rules) = { ... }
* @return The result.
*/
@java.lang.Override public com.authzed.api.v1.Debug.CheckDebugTrace.Permissionship getResult() {
com.authzed.api.v1.Debug.CheckDebugTrace.Permissionship result = com.authzed.api.v1.Debug.CheckDebugTrace.Permissionship.forNumber(result_);
return result == null ? com.authzed.api.v1.Debug.CheckDebugTrace.Permissionship.UNRECOGNIZED : result;
}
public static final int CAVEAT_EVALUATION_INFO_FIELD_NUMBER = 8;
private com.authzed.api.v1.Debug.CaveatEvalInfo caveatEvaluationInfo_;
/**
*
* caveat_evaluation_info holds information about the caveat evaluated for this step of the trace.
*
*
* .authzed.api.v1.CaveatEvalInfo caveat_evaluation_info = 8;
* @return Whether the caveatEvaluationInfo field is set.
*/
@java.lang.Override
public boolean hasCaveatEvaluationInfo() {
return caveatEvaluationInfo_ != null;
}
/**
*
* caveat_evaluation_info holds information about the caveat evaluated for this step of the trace.
*
*
* .authzed.api.v1.CaveatEvalInfo caveat_evaluation_info = 8;
* @return The caveatEvaluationInfo.
*/
@java.lang.Override
public com.authzed.api.v1.Debug.CaveatEvalInfo getCaveatEvaluationInfo() {
return caveatEvaluationInfo_ == null ? com.authzed.api.v1.Debug.CaveatEvalInfo.getDefaultInstance() : caveatEvaluationInfo_;
}
/**
*
* caveat_evaluation_info holds information about the caveat evaluated for this step of the trace.
*
*
* .authzed.api.v1.CaveatEvalInfo caveat_evaluation_info = 8;
*/
@java.lang.Override
public com.authzed.api.v1.Debug.CaveatEvalInfoOrBuilder getCaveatEvaluationInfoOrBuilder() {
return caveatEvaluationInfo_ == null ? com.authzed.api.v1.Debug.CaveatEvalInfo.getDefaultInstance() : caveatEvaluationInfo_;
}
public static final int WAS_CACHED_RESULT_FIELD_NUMBER = 6;
/**
*
* was_cached_result, if true, indicates that the result was found in the cache and returned directly.
*
*
* bool was_cached_result = 6;
* @return Whether the wasCachedResult field is set.
*/
@java.lang.Override
public boolean hasWasCachedResult() {
return resolutionCase_ == 6;
}
/**
*
* was_cached_result, if true, indicates that the result was found in the cache and returned directly.
*
*
* bool was_cached_result = 6;
* @return The wasCachedResult.
*/
@java.lang.Override
public boolean getWasCachedResult() {
if (resolutionCase_ == 6) {
return (java.lang.Boolean) resolution_;
}
return false;
}
public static final int SUB_PROBLEMS_FIELD_NUMBER = 7;
/**
*
* sub_problems holds the sub problems that were executed to resolve the answer to this Check. An empty list
* and a permissionship of PERMISSIONSHIP_HAS_PERMISSION indicates the subject was found within this relation.
*
*
* .authzed.api.v1.CheckDebugTrace.SubProblems sub_problems = 7;
* @return Whether the subProblems field is set.
*/
@java.lang.Override
public boolean hasSubProblems() {
return resolutionCase_ == 7;
}
/**
*
* sub_problems holds the sub problems that were executed to resolve the answer to this Check. An empty list
* and a permissionship of PERMISSIONSHIP_HAS_PERMISSION indicates the subject was found within this relation.
*
*
* .authzed.api.v1.CheckDebugTrace.SubProblems sub_problems = 7;
* @return The subProblems.
*/
@java.lang.Override
public com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems getSubProblems() {
if (resolutionCase_ == 7) {
return (com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems) resolution_;
}
return com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems.getDefaultInstance();
}
/**
*
* sub_problems holds the sub problems that were executed to resolve the answer to this Check. An empty list
* and a permissionship of PERMISSIONSHIP_HAS_PERMISSION indicates the subject was found within this relation.
*
*
* .authzed.api.v1.CheckDebugTrace.SubProblems sub_problems = 7;
*/
@java.lang.Override
public com.authzed.api.v1.Debug.CheckDebugTrace.SubProblemsOrBuilder getSubProblemsOrBuilder() {
if (resolutionCase_ == 7) {
return (com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems) resolution_;
}
return com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (resource_ != null) {
output.writeMessage(1, getResource());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(permission_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, permission_);
}
if (permissionType_ != com.authzed.api.v1.Debug.CheckDebugTrace.PermissionType.PERMISSION_TYPE_UNSPECIFIED.getNumber()) {
output.writeEnum(3, permissionType_);
}
if (subject_ != null) {
output.writeMessage(4, getSubject());
}
if (result_ != com.authzed.api.v1.Debug.CheckDebugTrace.Permissionship.PERMISSIONSHIP_UNSPECIFIED.getNumber()) {
output.writeEnum(5, result_);
}
if (resolutionCase_ == 6) {
output.writeBool(
6, (boolean)((java.lang.Boolean) resolution_));
}
if (resolutionCase_ == 7) {
output.writeMessage(7, (com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems) resolution_);
}
if (caveatEvaluationInfo_ != null) {
output.writeMessage(8, getCaveatEvaluationInfo());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (resource_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getResource());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(permission_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, permission_);
}
if (permissionType_ != com.authzed.api.v1.Debug.CheckDebugTrace.PermissionType.PERMISSION_TYPE_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(3, permissionType_);
}
if (subject_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getSubject());
}
if (result_ != com.authzed.api.v1.Debug.CheckDebugTrace.Permissionship.PERMISSIONSHIP_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(5, result_);
}
if (resolutionCase_ == 6) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(
6, (boolean)((java.lang.Boolean) resolution_));
}
if (resolutionCase_ == 7) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, (com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems) resolution_);
}
if (caveatEvaluationInfo_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, getCaveatEvaluationInfo());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.authzed.api.v1.Debug.CheckDebugTrace)) {
return super.equals(obj);
}
com.authzed.api.v1.Debug.CheckDebugTrace other = (com.authzed.api.v1.Debug.CheckDebugTrace) obj;
if (hasResource() != other.hasResource()) return false;
if (hasResource()) {
if (!getResource()
.equals(other.getResource())) return false;
}
if (!getPermission()
.equals(other.getPermission())) return false;
if (permissionType_ != other.permissionType_) return false;
if (hasSubject() != other.hasSubject()) return false;
if (hasSubject()) {
if (!getSubject()
.equals(other.getSubject())) return false;
}
if (result_ != other.result_) return false;
if (hasCaveatEvaluationInfo() != other.hasCaveatEvaluationInfo()) return false;
if (hasCaveatEvaluationInfo()) {
if (!getCaveatEvaluationInfo()
.equals(other.getCaveatEvaluationInfo())) return false;
}
if (!getResolutionCase().equals(other.getResolutionCase())) return false;
switch (resolutionCase_) {
case 6:
if (getWasCachedResult()
!= other.getWasCachedResult()) return false;
break;
case 7:
if (!getSubProblems()
.equals(other.getSubProblems())) return false;
break;
case 0:
default:
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasResource()) {
hash = (37 * hash) + RESOURCE_FIELD_NUMBER;
hash = (53 * hash) + getResource().hashCode();
}
hash = (37 * hash) + PERMISSION_FIELD_NUMBER;
hash = (53 * hash) + getPermission().hashCode();
hash = (37 * hash) + PERMISSION_TYPE_FIELD_NUMBER;
hash = (53 * hash) + permissionType_;
if (hasSubject()) {
hash = (37 * hash) + SUBJECT_FIELD_NUMBER;
hash = (53 * hash) + getSubject().hashCode();
}
hash = (37 * hash) + RESULT_FIELD_NUMBER;
hash = (53 * hash) + result_;
if (hasCaveatEvaluationInfo()) {
hash = (37 * hash) + CAVEAT_EVALUATION_INFO_FIELD_NUMBER;
hash = (53 * hash) + getCaveatEvaluationInfo().hashCode();
}
switch (resolutionCase_) {
case 6:
hash = (37 * hash) + WAS_CACHED_RESULT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getWasCachedResult());
break;
case 7:
hash = (37 * hash) + SUB_PROBLEMS_FIELD_NUMBER;
hash = (53 * hash) + getSubProblems().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.authzed.api.v1.Debug.CheckDebugTrace parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.Debug.CheckDebugTrace parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.Debug.CheckDebugTrace parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.Debug.CheckDebugTrace parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.Debug.CheckDebugTrace parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.Debug.CheckDebugTrace parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.Debug.CheckDebugTrace parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.Debug.CheckDebugTrace parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.Debug.CheckDebugTrace parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.authzed.api.v1.Debug.CheckDebugTrace parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.Debug.CheckDebugTrace parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.Debug.CheckDebugTrace parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.authzed.api.v1.Debug.CheckDebugTrace prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* CheckDebugTrace is a recursive trace of the requests made for resolving a CheckPermission
* API call.
*
*
* Protobuf type {@code authzed.api.v1.CheckDebugTrace}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:authzed.api.v1.CheckDebugTrace)
com.authzed.api.v1.Debug.CheckDebugTraceOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.Debug.internal_static_authzed_api_v1_CheckDebugTrace_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.Debug.internal_static_authzed_api_v1_CheckDebugTrace_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.Debug.CheckDebugTrace.class, com.authzed.api.v1.Debug.CheckDebugTrace.Builder.class);
}
// Construct using com.authzed.api.v1.Debug.CheckDebugTrace.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
resource_ = null;
if (resourceBuilder_ != null) {
resourceBuilder_.dispose();
resourceBuilder_ = null;
}
permission_ = "";
permissionType_ = 0;
subject_ = null;
if (subjectBuilder_ != null) {
subjectBuilder_.dispose();
subjectBuilder_ = null;
}
result_ = 0;
caveatEvaluationInfo_ = null;
if (caveatEvaluationInfoBuilder_ != null) {
caveatEvaluationInfoBuilder_.dispose();
caveatEvaluationInfoBuilder_ = null;
}
if (subProblemsBuilder_ != null) {
subProblemsBuilder_.clear();
}
resolutionCase_ = 0;
resolution_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.authzed.api.v1.Debug.internal_static_authzed_api_v1_CheckDebugTrace_descriptor;
}
@java.lang.Override
public com.authzed.api.v1.Debug.CheckDebugTrace getDefaultInstanceForType() {
return com.authzed.api.v1.Debug.CheckDebugTrace.getDefaultInstance();
}
@java.lang.Override
public com.authzed.api.v1.Debug.CheckDebugTrace build() {
com.authzed.api.v1.Debug.CheckDebugTrace result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.authzed.api.v1.Debug.CheckDebugTrace buildPartial() {
com.authzed.api.v1.Debug.CheckDebugTrace result = new com.authzed.api.v1.Debug.CheckDebugTrace(this);
if (bitField0_ != 0) { buildPartial0(result); }
buildPartialOneofs(result);
onBuilt();
return result;
}
private void buildPartial0(com.authzed.api.v1.Debug.CheckDebugTrace result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.resource_ = resourceBuilder_ == null
? resource_
: resourceBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.permission_ = permission_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.permissionType_ = permissionType_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.subject_ = subjectBuilder_ == null
? subject_
: subjectBuilder_.build();
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.result_ = result_;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.caveatEvaluationInfo_ = caveatEvaluationInfoBuilder_ == null
? caveatEvaluationInfo_
: caveatEvaluationInfoBuilder_.build();
}
}
private void buildPartialOneofs(com.authzed.api.v1.Debug.CheckDebugTrace result) {
result.resolutionCase_ = resolutionCase_;
result.resolution_ = this.resolution_;
if (resolutionCase_ == 7 &&
subProblemsBuilder_ != null) {
result.resolution_ = subProblemsBuilder_.build();
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.authzed.api.v1.Debug.CheckDebugTrace) {
return mergeFrom((com.authzed.api.v1.Debug.CheckDebugTrace)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.authzed.api.v1.Debug.CheckDebugTrace other) {
if (other == com.authzed.api.v1.Debug.CheckDebugTrace.getDefaultInstance()) return this;
if (other.hasResource()) {
mergeResource(other.getResource());
}
if (!other.getPermission().isEmpty()) {
permission_ = other.permission_;
bitField0_ |= 0x00000002;
onChanged();
}
if (other.permissionType_ != 0) {
setPermissionTypeValue(other.getPermissionTypeValue());
}
if (other.hasSubject()) {
mergeSubject(other.getSubject());
}
if (other.result_ != 0) {
setResultValue(other.getResultValue());
}
if (other.hasCaveatEvaluationInfo()) {
mergeCaveatEvaluationInfo(other.getCaveatEvaluationInfo());
}
switch (other.getResolutionCase()) {
case WAS_CACHED_RESULT: {
setWasCachedResult(other.getWasCachedResult());
break;
}
case SUB_PROBLEMS: {
mergeSubProblems(other.getSubProblems());
break;
}
case RESOLUTION_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
input.readMessage(
getResourceFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
permission_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 18
case 24: {
permissionType_ = input.readEnum();
bitField0_ |= 0x00000004;
break;
} // case 24
case 34: {
input.readMessage(
getSubjectFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000008;
break;
} // case 34
case 40: {
result_ = input.readEnum();
bitField0_ |= 0x00000010;
break;
} // case 40
case 48: {
resolution_ = input.readBool();
resolutionCase_ = 6;
break;
} // case 48
case 58: {
input.readMessage(
getSubProblemsFieldBuilder().getBuilder(),
extensionRegistry);
resolutionCase_ = 7;
break;
} // case 58
case 66: {
input.readMessage(
getCaveatEvaluationInfoFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000020;
break;
} // case 66
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int resolutionCase_ = 0;
private java.lang.Object resolution_;
public ResolutionCase
getResolutionCase() {
return ResolutionCase.forNumber(
resolutionCase_);
}
public Builder clearResolution() {
resolutionCase_ = 0;
resolution_ = null;
onChanged();
return this;
}
private int bitField0_;
private com.authzed.api.v1.Core.ObjectReference resource_;
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.ObjectReference, com.authzed.api.v1.Core.ObjectReference.Builder, com.authzed.api.v1.Core.ObjectReferenceOrBuilder> resourceBuilder_;
/**
*
* resource holds the resource on which the Check was performed.
*
*
* .authzed.api.v1.ObjectReference resource = 1 [(.validate.rules) = { ... }
* @return Whether the resource field is set.
*/
public boolean hasResource() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* resource holds the resource on which the Check was performed.
*
*
* .authzed.api.v1.ObjectReference resource = 1 [(.validate.rules) = { ... }
* @return The resource.
*/
public com.authzed.api.v1.Core.ObjectReference getResource() {
if (resourceBuilder_ == null) {
return resource_ == null ? com.authzed.api.v1.Core.ObjectReference.getDefaultInstance() : resource_;
} else {
return resourceBuilder_.getMessage();
}
}
/**
*
* resource holds the resource on which the Check was performed.
*
*
* .authzed.api.v1.ObjectReference resource = 1 [(.validate.rules) = { ... }
*/
public Builder setResource(com.authzed.api.v1.Core.ObjectReference value) {
if (resourceBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
resource_ = value;
} else {
resourceBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* resource holds the resource on which the Check was performed.
*
*
* .authzed.api.v1.ObjectReference resource = 1 [(.validate.rules) = { ... }
*/
public Builder setResource(
com.authzed.api.v1.Core.ObjectReference.Builder builderForValue) {
if (resourceBuilder_ == null) {
resource_ = builderForValue.build();
} else {
resourceBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* resource holds the resource on which the Check was performed.
*
*
* .authzed.api.v1.ObjectReference resource = 1 [(.validate.rules) = { ... }
*/
public Builder mergeResource(com.authzed.api.v1.Core.ObjectReference value) {
if (resourceBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0) &&
resource_ != null &&
resource_ != com.authzed.api.v1.Core.ObjectReference.getDefaultInstance()) {
getResourceBuilder().mergeFrom(value);
} else {
resource_ = value;
}
} else {
resourceBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* resource holds the resource on which the Check was performed.
*
*
* .authzed.api.v1.ObjectReference resource = 1 [(.validate.rules) = { ... }
*/
public Builder clearResource() {
bitField0_ = (bitField0_ & ~0x00000001);
resource_ = null;
if (resourceBuilder_ != null) {
resourceBuilder_.dispose();
resourceBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* resource holds the resource on which the Check was performed.
*
*
* .authzed.api.v1.ObjectReference resource = 1 [(.validate.rules) = { ... }
*/
public com.authzed.api.v1.Core.ObjectReference.Builder getResourceBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getResourceFieldBuilder().getBuilder();
}
/**
*
* resource holds the resource on which the Check was performed.
*
*
* .authzed.api.v1.ObjectReference resource = 1 [(.validate.rules) = { ... }
*/
public com.authzed.api.v1.Core.ObjectReferenceOrBuilder getResourceOrBuilder() {
if (resourceBuilder_ != null) {
return resourceBuilder_.getMessageOrBuilder();
} else {
return resource_ == null ?
com.authzed.api.v1.Core.ObjectReference.getDefaultInstance() : resource_;
}
}
/**
*
* resource holds the resource on which the Check was performed.
*
*
* .authzed.api.v1.ObjectReference resource = 1 [(.validate.rules) = { ... }
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.ObjectReference, com.authzed.api.v1.Core.ObjectReference.Builder, com.authzed.api.v1.Core.ObjectReferenceOrBuilder>
getResourceFieldBuilder() {
if (resourceBuilder_ == null) {
resourceBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.ObjectReference, com.authzed.api.v1.Core.ObjectReference.Builder, com.authzed.api.v1.Core.ObjectReferenceOrBuilder>(
getResource(),
getParentForChildren(),
isClean());
resource_ = null;
}
return resourceBuilder_;
}
private java.lang.Object permission_ = "";
/**
*
* permission holds the name of the permission or relation on which the Check was performed.
*
*
* string permission = 2;
* @return The permission.
*/
public java.lang.String getPermission() {
java.lang.Object ref = permission_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
permission_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* permission holds the name of the permission or relation on which the Check was performed.
*
*
* string permission = 2;
* @return The bytes for permission.
*/
public com.google.protobuf.ByteString
getPermissionBytes() {
java.lang.Object ref = permission_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
permission_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* permission holds the name of the permission or relation on which the Check was performed.
*
*
* string permission = 2;
* @param value The permission to set.
* @return This builder for chaining.
*/
public Builder setPermission(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
permission_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* permission holds the name of the permission or relation on which the Check was performed.
*
*
* string permission = 2;
* @return This builder for chaining.
*/
public Builder clearPermission() {
permission_ = getDefaultInstance().getPermission();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
* permission holds the name of the permission or relation on which the Check was performed.
*
*
* string permission = 2;
* @param value The bytes for permission to set.
* @return This builder for chaining.
*/
public Builder setPermissionBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
permission_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private int permissionType_ = 0;
/**
*
* permission_type holds information indicating whether it was a permission or relation.
*
*
* .authzed.api.v1.CheckDebugTrace.PermissionType permission_type = 3 [(.validate.rules) = { ... }
* @return The enum numeric value on the wire for permissionType.
*/
@java.lang.Override public int getPermissionTypeValue() {
return permissionType_;
}
/**
*
* permission_type holds information indicating whether it was a permission or relation.
*
*
* .authzed.api.v1.CheckDebugTrace.PermissionType permission_type = 3 [(.validate.rules) = { ... }
* @param value The enum numeric value on the wire for permissionType to set.
* @return This builder for chaining.
*/
public Builder setPermissionTypeValue(int value) {
permissionType_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* permission_type holds information indicating whether it was a permission or relation.
*
*
* .authzed.api.v1.CheckDebugTrace.PermissionType permission_type = 3 [(.validate.rules) = { ... }
* @return The permissionType.
*/
@java.lang.Override
public com.authzed.api.v1.Debug.CheckDebugTrace.PermissionType getPermissionType() {
com.authzed.api.v1.Debug.CheckDebugTrace.PermissionType result = com.authzed.api.v1.Debug.CheckDebugTrace.PermissionType.forNumber(permissionType_);
return result == null ? com.authzed.api.v1.Debug.CheckDebugTrace.PermissionType.UNRECOGNIZED : result;
}
/**
*
* permission_type holds information indicating whether it was a permission or relation.
*
*
* .authzed.api.v1.CheckDebugTrace.PermissionType permission_type = 3 [(.validate.rules) = { ... }
* @param value The permissionType to set.
* @return This builder for chaining.
*/
public Builder setPermissionType(com.authzed.api.v1.Debug.CheckDebugTrace.PermissionType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
permissionType_ = value.getNumber();
onChanged();
return this;
}
/**
*
* permission_type holds information indicating whether it was a permission or relation.
*
*
* .authzed.api.v1.CheckDebugTrace.PermissionType permission_type = 3 [(.validate.rules) = { ... }
* @return This builder for chaining.
*/
public Builder clearPermissionType() {
bitField0_ = (bitField0_ & ~0x00000004);
permissionType_ = 0;
onChanged();
return this;
}
private com.authzed.api.v1.Core.SubjectReference subject_;
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.SubjectReference, com.authzed.api.v1.Core.SubjectReference.Builder, com.authzed.api.v1.Core.SubjectReferenceOrBuilder> subjectBuilder_;
/**
*
* subject holds the subject on which the Check was performed. This will be static across all calls within
* the same Check tree.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
* @return Whether the subject field is set.
*/
public boolean hasSubject() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* subject holds the subject on which the Check was performed. This will be static across all calls within
* the same Check tree.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
* @return The subject.
*/
public com.authzed.api.v1.Core.SubjectReference getSubject() {
if (subjectBuilder_ == null) {
return subject_ == null ? com.authzed.api.v1.Core.SubjectReference.getDefaultInstance() : subject_;
} else {
return subjectBuilder_.getMessage();
}
}
/**
*
* subject holds the subject on which the Check was performed. This will be static across all calls within
* the same Check tree.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
*/
public Builder setSubject(com.authzed.api.v1.Core.SubjectReference value) {
if (subjectBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
subject_ = value;
} else {
subjectBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* subject holds the subject on which the Check was performed. This will be static across all calls within
* the same Check tree.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
*/
public Builder setSubject(
com.authzed.api.v1.Core.SubjectReference.Builder builderForValue) {
if (subjectBuilder_ == null) {
subject_ = builderForValue.build();
} else {
subjectBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* subject holds the subject on which the Check was performed. This will be static across all calls within
* the same Check tree.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
*/
public Builder mergeSubject(com.authzed.api.v1.Core.SubjectReference value) {
if (subjectBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0) &&
subject_ != null &&
subject_ != com.authzed.api.v1.Core.SubjectReference.getDefaultInstance()) {
getSubjectBuilder().mergeFrom(value);
} else {
subject_ = value;
}
} else {
subjectBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* subject holds the subject on which the Check was performed. This will be static across all calls within
* the same Check tree.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
*/
public Builder clearSubject() {
bitField0_ = (bitField0_ & ~0x00000008);
subject_ = null;
if (subjectBuilder_ != null) {
subjectBuilder_.dispose();
subjectBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* subject holds the subject on which the Check was performed. This will be static across all calls within
* the same Check tree.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
*/
public com.authzed.api.v1.Core.SubjectReference.Builder getSubjectBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getSubjectFieldBuilder().getBuilder();
}
/**
*
* subject holds the subject on which the Check was performed. This will be static across all calls within
* the same Check tree.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
*/
public com.authzed.api.v1.Core.SubjectReferenceOrBuilder getSubjectOrBuilder() {
if (subjectBuilder_ != null) {
return subjectBuilder_.getMessageOrBuilder();
} else {
return subject_ == null ?
com.authzed.api.v1.Core.SubjectReference.getDefaultInstance() : subject_;
}
}
/**
*
* subject holds the subject on which the Check was performed. This will be static across all calls within
* the same Check tree.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.SubjectReference, com.authzed.api.v1.Core.SubjectReference.Builder, com.authzed.api.v1.Core.SubjectReferenceOrBuilder>
getSubjectFieldBuilder() {
if (subjectBuilder_ == null) {
subjectBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.SubjectReference, com.authzed.api.v1.Core.SubjectReference.Builder, com.authzed.api.v1.Core.SubjectReferenceOrBuilder>(
getSubject(),
getParentForChildren(),
isClean());
subject_ = null;
}
return subjectBuilder_;
}
private int result_ = 0;
/**
*
* result holds the result of the Check call.
*
*
* .authzed.api.v1.CheckDebugTrace.Permissionship result = 5 [(.validate.rules) = { ... }
* @return The enum numeric value on the wire for result.
*/
@java.lang.Override public int getResultValue() {
return result_;
}
/**
*
* result holds the result of the Check call.
*
*
* .authzed.api.v1.CheckDebugTrace.Permissionship result = 5 [(.validate.rules) = { ... }
* @param value The enum numeric value on the wire for result to set.
* @return This builder for chaining.
*/
public Builder setResultValue(int value) {
result_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
* result holds the result of the Check call.
*
*
* .authzed.api.v1.CheckDebugTrace.Permissionship result = 5 [(.validate.rules) = { ... }
* @return The result.
*/
@java.lang.Override
public com.authzed.api.v1.Debug.CheckDebugTrace.Permissionship getResult() {
com.authzed.api.v1.Debug.CheckDebugTrace.Permissionship result = com.authzed.api.v1.Debug.CheckDebugTrace.Permissionship.forNumber(result_);
return result == null ? com.authzed.api.v1.Debug.CheckDebugTrace.Permissionship.UNRECOGNIZED : result;
}
/**
*
* result holds the result of the Check call.
*
*
* .authzed.api.v1.CheckDebugTrace.Permissionship result = 5 [(.validate.rules) = { ... }
* @param value The result to set.
* @return This builder for chaining.
*/
public Builder setResult(com.authzed.api.v1.Debug.CheckDebugTrace.Permissionship value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
result_ = value.getNumber();
onChanged();
return this;
}
/**
*
* result holds the result of the Check call.
*
*
* .authzed.api.v1.CheckDebugTrace.Permissionship result = 5 [(.validate.rules) = { ... }
* @return This builder for chaining.
*/
public Builder clearResult() {
bitField0_ = (bitField0_ & ~0x00000010);
result_ = 0;
onChanged();
return this;
}
private com.authzed.api.v1.Debug.CaveatEvalInfo caveatEvaluationInfo_;
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Debug.CaveatEvalInfo, com.authzed.api.v1.Debug.CaveatEvalInfo.Builder, com.authzed.api.v1.Debug.CaveatEvalInfoOrBuilder> caveatEvaluationInfoBuilder_;
/**
*
* caveat_evaluation_info holds information about the caveat evaluated for this step of the trace.
*
*
* .authzed.api.v1.CaveatEvalInfo caveat_evaluation_info = 8;
* @return Whether the caveatEvaluationInfo field is set.
*/
public boolean hasCaveatEvaluationInfo() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* caveat_evaluation_info holds information about the caveat evaluated for this step of the trace.
*
*
* .authzed.api.v1.CaveatEvalInfo caveat_evaluation_info = 8;
* @return The caveatEvaluationInfo.
*/
public com.authzed.api.v1.Debug.CaveatEvalInfo getCaveatEvaluationInfo() {
if (caveatEvaluationInfoBuilder_ == null) {
return caveatEvaluationInfo_ == null ? com.authzed.api.v1.Debug.CaveatEvalInfo.getDefaultInstance() : caveatEvaluationInfo_;
} else {
return caveatEvaluationInfoBuilder_.getMessage();
}
}
/**
*
* caveat_evaluation_info holds information about the caveat evaluated for this step of the trace.
*
*
* .authzed.api.v1.CaveatEvalInfo caveat_evaluation_info = 8;
*/
public Builder setCaveatEvaluationInfo(com.authzed.api.v1.Debug.CaveatEvalInfo value) {
if (caveatEvaluationInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
caveatEvaluationInfo_ = value;
} else {
caveatEvaluationInfoBuilder_.setMessage(value);
}
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
* caveat_evaluation_info holds information about the caveat evaluated for this step of the trace.
*
*
* .authzed.api.v1.CaveatEvalInfo caveat_evaluation_info = 8;
*/
public Builder setCaveatEvaluationInfo(
com.authzed.api.v1.Debug.CaveatEvalInfo.Builder builderForValue) {
if (caveatEvaluationInfoBuilder_ == null) {
caveatEvaluationInfo_ = builderForValue.build();
} else {
caveatEvaluationInfoBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
* caveat_evaluation_info holds information about the caveat evaluated for this step of the trace.
*
*
* .authzed.api.v1.CaveatEvalInfo caveat_evaluation_info = 8;
*/
public Builder mergeCaveatEvaluationInfo(com.authzed.api.v1.Debug.CaveatEvalInfo value) {
if (caveatEvaluationInfoBuilder_ == null) {
if (((bitField0_ & 0x00000020) != 0) &&
caveatEvaluationInfo_ != null &&
caveatEvaluationInfo_ != com.authzed.api.v1.Debug.CaveatEvalInfo.getDefaultInstance()) {
getCaveatEvaluationInfoBuilder().mergeFrom(value);
} else {
caveatEvaluationInfo_ = value;
}
} else {
caveatEvaluationInfoBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
* caveat_evaluation_info holds information about the caveat evaluated for this step of the trace.
*
*
* .authzed.api.v1.CaveatEvalInfo caveat_evaluation_info = 8;
*/
public Builder clearCaveatEvaluationInfo() {
bitField0_ = (bitField0_ & ~0x00000020);
caveatEvaluationInfo_ = null;
if (caveatEvaluationInfoBuilder_ != null) {
caveatEvaluationInfoBuilder_.dispose();
caveatEvaluationInfoBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* caveat_evaluation_info holds information about the caveat evaluated for this step of the trace.
*
*
* .authzed.api.v1.CaveatEvalInfo caveat_evaluation_info = 8;
*/
public com.authzed.api.v1.Debug.CaveatEvalInfo.Builder getCaveatEvaluationInfoBuilder() {
bitField0_ |= 0x00000020;
onChanged();
return getCaveatEvaluationInfoFieldBuilder().getBuilder();
}
/**
*
* caveat_evaluation_info holds information about the caveat evaluated for this step of the trace.
*
*
* .authzed.api.v1.CaveatEvalInfo caveat_evaluation_info = 8;
*/
public com.authzed.api.v1.Debug.CaveatEvalInfoOrBuilder getCaveatEvaluationInfoOrBuilder() {
if (caveatEvaluationInfoBuilder_ != null) {
return caveatEvaluationInfoBuilder_.getMessageOrBuilder();
} else {
return caveatEvaluationInfo_ == null ?
com.authzed.api.v1.Debug.CaveatEvalInfo.getDefaultInstance() : caveatEvaluationInfo_;
}
}
/**
*
* caveat_evaluation_info holds information about the caveat evaluated for this step of the trace.
*
*
* .authzed.api.v1.CaveatEvalInfo caveat_evaluation_info = 8;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Debug.CaveatEvalInfo, com.authzed.api.v1.Debug.CaveatEvalInfo.Builder, com.authzed.api.v1.Debug.CaveatEvalInfoOrBuilder>
getCaveatEvaluationInfoFieldBuilder() {
if (caveatEvaluationInfoBuilder_ == null) {
caveatEvaluationInfoBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Debug.CaveatEvalInfo, com.authzed.api.v1.Debug.CaveatEvalInfo.Builder, com.authzed.api.v1.Debug.CaveatEvalInfoOrBuilder>(
getCaveatEvaluationInfo(),
getParentForChildren(),
isClean());
caveatEvaluationInfo_ = null;
}
return caveatEvaluationInfoBuilder_;
}
/**
*
* was_cached_result, if true, indicates that the result was found in the cache and returned directly.
*
*
* bool was_cached_result = 6;
* @return Whether the wasCachedResult field is set.
*/
public boolean hasWasCachedResult() {
return resolutionCase_ == 6;
}
/**
*
* was_cached_result, if true, indicates that the result was found in the cache and returned directly.
*
*
* bool was_cached_result = 6;
* @return The wasCachedResult.
*/
public boolean getWasCachedResult() {
if (resolutionCase_ == 6) {
return (java.lang.Boolean) resolution_;
}
return false;
}
/**
*
* was_cached_result, if true, indicates that the result was found in the cache and returned directly.
*
*
* bool was_cached_result = 6;
* @param value The wasCachedResult to set.
* @return This builder for chaining.
*/
public Builder setWasCachedResult(boolean value) {
resolutionCase_ = 6;
resolution_ = value;
onChanged();
return this;
}
/**
*
* was_cached_result, if true, indicates that the result was found in the cache and returned directly.
*
*
* bool was_cached_result = 6;
* @return This builder for chaining.
*/
public Builder clearWasCachedResult() {
if (resolutionCase_ == 6) {
resolutionCase_ = 0;
resolution_ = null;
onChanged();
}
return this;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems, com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems.Builder, com.authzed.api.v1.Debug.CheckDebugTrace.SubProblemsOrBuilder> subProblemsBuilder_;
/**
*
* sub_problems holds the sub problems that were executed to resolve the answer to this Check. An empty list
* and a permissionship of PERMISSIONSHIP_HAS_PERMISSION indicates the subject was found within this relation.
*
*
* .authzed.api.v1.CheckDebugTrace.SubProblems sub_problems = 7;
* @return Whether the subProblems field is set.
*/
@java.lang.Override
public boolean hasSubProblems() {
return resolutionCase_ == 7;
}
/**
*
* sub_problems holds the sub problems that were executed to resolve the answer to this Check. An empty list
* and a permissionship of PERMISSIONSHIP_HAS_PERMISSION indicates the subject was found within this relation.
*
*
* .authzed.api.v1.CheckDebugTrace.SubProblems sub_problems = 7;
* @return The subProblems.
*/
@java.lang.Override
public com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems getSubProblems() {
if (subProblemsBuilder_ == null) {
if (resolutionCase_ == 7) {
return (com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems) resolution_;
}
return com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems.getDefaultInstance();
} else {
if (resolutionCase_ == 7) {
return subProblemsBuilder_.getMessage();
}
return com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems.getDefaultInstance();
}
}
/**
*
* sub_problems holds the sub problems that were executed to resolve the answer to this Check. An empty list
* and a permissionship of PERMISSIONSHIP_HAS_PERMISSION indicates the subject was found within this relation.
*
*
* .authzed.api.v1.CheckDebugTrace.SubProblems sub_problems = 7;
*/
public Builder setSubProblems(com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems value) {
if (subProblemsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
resolution_ = value;
onChanged();
} else {
subProblemsBuilder_.setMessage(value);
}
resolutionCase_ = 7;
return this;
}
/**
*
* sub_problems holds the sub problems that were executed to resolve the answer to this Check. An empty list
* and a permissionship of PERMISSIONSHIP_HAS_PERMISSION indicates the subject was found within this relation.
*
*
* .authzed.api.v1.CheckDebugTrace.SubProblems sub_problems = 7;
*/
public Builder setSubProblems(
com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems.Builder builderForValue) {
if (subProblemsBuilder_ == null) {
resolution_ = builderForValue.build();
onChanged();
} else {
subProblemsBuilder_.setMessage(builderForValue.build());
}
resolutionCase_ = 7;
return this;
}
/**
*
* sub_problems holds the sub problems that were executed to resolve the answer to this Check. An empty list
* and a permissionship of PERMISSIONSHIP_HAS_PERMISSION indicates the subject was found within this relation.
*
*
* .authzed.api.v1.CheckDebugTrace.SubProblems sub_problems = 7;
*/
public Builder mergeSubProblems(com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems value) {
if (subProblemsBuilder_ == null) {
if (resolutionCase_ == 7 &&
resolution_ != com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems.getDefaultInstance()) {
resolution_ = com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems.newBuilder((com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems) resolution_)
.mergeFrom(value).buildPartial();
} else {
resolution_ = value;
}
onChanged();
} else {
if (resolutionCase_ == 7) {
subProblemsBuilder_.mergeFrom(value);
} else {
subProblemsBuilder_.setMessage(value);
}
}
resolutionCase_ = 7;
return this;
}
/**
*
* sub_problems holds the sub problems that were executed to resolve the answer to this Check. An empty list
* and a permissionship of PERMISSIONSHIP_HAS_PERMISSION indicates the subject was found within this relation.
*
*
* .authzed.api.v1.CheckDebugTrace.SubProblems sub_problems = 7;
*/
public Builder clearSubProblems() {
if (subProblemsBuilder_ == null) {
if (resolutionCase_ == 7) {
resolutionCase_ = 0;
resolution_ = null;
onChanged();
}
} else {
if (resolutionCase_ == 7) {
resolutionCase_ = 0;
resolution_ = null;
}
subProblemsBuilder_.clear();
}
return this;
}
/**
*
* sub_problems holds the sub problems that were executed to resolve the answer to this Check. An empty list
* and a permissionship of PERMISSIONSHIP_HAS_PERMISSION indicates the subject was found within this relation.
*
*
* .authzed.api.v1.CheckDebugTrace.SubProblems sub_problems = 7;
*/
public com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems.Builder getSubProblemsBuilder() {
return getSubProblemsFieldBuilder().getBuilder();
}
/**
*
* sub_problems holds the sub problems that were executed to resolve the answer to this Check. An empty list
* and a permissionship of PERMISSIONSHIP_HAS_PERMISSION indicates the subject was found within this relation.
*
*
* .authzed.api.v1.CheckDebugTrace.SubProblems sub_problems = 7;
*/
@java.lang.Override
public com.authzed.api.v1.Debug.CheckDebugTrace.SubProblemsOrBuilder getSubProblemsOrBuilder() {
if ((resolutionCase_ == 7) && (subProblemsBuilder_ != null)) {
return subProblemsBuilder_.getMessageOrBuilder();
} else {
if (resolutionCase_ == 7) {
return (com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems) resolution_;
}
return com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems.getDefaultInstance();
}
}
/**
*
* sub_problems holds the sub problems that were executed to resolve the answer to this Check. An empty list
* and a permissionship of PERMISSIONSHIP_HAS_PERMISSION indicates the subject was found within this relation.
*
*
* .authzed.api.v1.CheckDebugTrace.SubProblems sub_problems = 7;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems, com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems.Builder, com.authzed.api.v1.Debug.CheckDebugTrace.SubProblemsOrBuilder>
getSubProblemsFieldBuilder() {
if (subProblemsBuilder_ == null) {
if (!(resolutionCase_ == 7)) {
resolution_ = com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems.getDefaultInstance();
}
subProblemsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems, com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems.Builder, com.authzed.api.v1.Debug.CheckDebugTrace.SubProblemsOrBuilder>(
(com.authzed.api.v1.Debug.CheckDebugTrace.SubProblems) resolution_,
getParentForChildren(),
isClean());
resolution_ = null;
}
resolutionCase_ = 7;
onChanged();
return subProblemsBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:authzed.api.v1.CheckDebugTrace)
}
// @@protoc_insertion_point(class_scope:authzed.api.v1.CheckDebugTrace)
private static final com.authzed.api.v1.Debug.CheckDebugTrace DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.authzed.api.v1.Debug.CheckDebugTrace();
}
public static com.authzed.api.v1.Debug.CheckDebugTrace getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CheckDebugTrace parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.authzed.api.v1.Debug.CheckDebugTrace getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CaveatEvalInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:authzed.api.v1.CaveatEvalInfo)
com.google.protobuf.MessageOrBuilder {
/**
*
* expression is the expression that was evaluated.
*
*
* string expression = 1;
* @return The expression.
*/
java.lang.String getExpression();
/**
*
* expression is the expression that was evaluated.
*
*
* string expression = 1;
* @return The bytes for expression.
*/
com.google.protobuf.ByteString
getExpressionBytes();
/**
*
* result is the result of the evaluation.
*
*
* .authzed.api.v1.CaveatEvalInfo.Result result = 2;
* @return The enum numeric value on the wire for result.
*/
int getResultValue();
/**
*
* result is the result of the evaluation.
*
*
* .authzed.api.v1.CaveatEvalInfo.Result result = 2;
* @return The result.
*/
com.authzed.api.v1.Debug.CaveatEvalInfo.Result getResult();
/**
*
* context consists of any named values that were used for evaluating the caveat expression.
*
*
* .google.protobuf.Struct context = 3;
* @return Whether the context field is set.
*/
boolean hasContext();
/**
*
* context consists of any named values that were used for evaluating the caveat expression.
*
*
* .google.protobuf.Struct context = 3;
* @return The context.
*/
com.google.protobuf.Struct getContext();
/**
*
* context consists of any named values that were used for evaluating the caveat expression.
*
*
* .google.protobuf.Struct context = 3;
*/
com.google.protobuf.StructOrBuilder getContextOrBuilder();
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response, if applicable.
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 4;
* @return Whether the partialCaveatInfo field is set.
*/
boolean hasPartialCaveatInfo();
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response, if applicable.
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 4;
* @return The partialCaveatInfo.
*/
com.authzed.api.v1.Core.PartialCaveatInfo getPartialCaveatInfo();
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response, if applicable.
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 4;
*/
com.authzed.api.v1.Core.PartialCaveatInfoOrBuilder getPartialCaveatInfoOrBuilder();
/**
*
* caveat_name is the name of the caveat that was executed, if applicable.
*
*
* string caveat_name = 5;
* @return The caveatName.
*/
java.lang.String getCaveatName();
/**
*
* caveat_name is the name of the caveat that was executed, if applicable.
*
*
* string caveat_name = 5;
* @return The bytes for caveatName.
*/
com.google.protobuf.ByteString
getCaveatNameBytes();
}
/**
*
* CaveatEvalInfo holds information about a caveat expression that was evaluated.
*
*
* Protobuf type {@code authzed.api.v1.CaveatEvalInfo}
*/
public static final class CaveatEvalInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:authzed.api.v1.CaveatEvalInfo)
CaveatEvalInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use CaveatEvalInfo.newBuilder() to construct.
private CaveatEvalInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CaveatEvalInfo() {
expression_ = "";
result_ = 0;
caveatName_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CaveatEvalInfo();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.Debug.internal_static_authzed_api_v1_CaveatEvalInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.Debug.internal_static_authzed_api_v1_CaveatEvalInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.Debug.CaveatEvalInfo.class, com.authzed.api.v1.Debug.CaveatEvalInfo.Builder.class);
}
/**
* Protobuf enum {@code authzed.api.v1.CaveatEvalInfo.Result}
*/
public enum Result
implements com.google.protobuf.ProtocolMessageEnum {
/**
* RESULT_UNSPECIFIED = 0;
*/
RESULT_UNSPECIFIED(0),
/**
* RESULT_UNEVALUATED = 1;
*/
RESULT_UNEVALUATED(1),
/**
* RESULT_FALSE = 2;
*/
RESULT_FALSE(2),
/**
* RESULT_TRUE = 3;
*/
RESULT_TRUE(3),
/**
* RESULT_MISSING_SOME_CONTEXT = 4;
*/
RESULT_MISSING_SOME_CONTEXT(4),
UNRECOGNIZED(-1),
;
/**
* RESULT_UNSPECIFIED = 0;
*/
public static final int RESULT_UNSPECIFIED_VALUE = 0;
/**
* RESULT_UNEVALUATED = 1;
*/
public static final int RESULT_UNEVALUATED_VALUE = 1;
/**
* RESULT_FALSE = 2;
*/
public static final int RESULT_FALSE_VALUE = 2;
/**
* RESULT_TRUE = 3;
*/
public static final int RESULT_TRUE_VALUE = 3;
/**
* RESULT_MISSING_SOME_CONTEXT = 4;
*/
public static final int RESULT_MISSING_SOME_CONTEXT_VALUE = 4;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Result valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static Result forNumber(int value) {
switch (value) {
case 0: return RESULT_UNSPECIFIED;
case 1: return RESULT_UNEVALUATED;
case 2: return RESULT_FALSE;
case 3: return RESULT_TRUE;
case 4: return RESULT_MISSING_SOME_CONTEXT;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
Result> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Result findValueByNumber(int number) {
return Result.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.authzed.api.v1.Debug.CaveatEvalInfo.getDescriptor().getEnumTypes().get(0);
}
private static final Result[] VALUES = values();
public static Result valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private Result(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:authzed.api.v1.CaveatEvalInfo.Result)
}
public static final int EXPRESSION_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object expression_ = "";
/**
*
* expression is the expression that was evaluated.
*
*
* string expression = 1;
* @return The expression.
*/
@java.lang.Override
public java.lang.String getExpression() {
java.lang.Object ref = expression_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
expression_ = s;
return s;
}
}
/**
*
* expression is the expression that was evaluated.
*
*
* string expression = 1;
* @return The bytes for expression.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getExpressionBytes() {
java.lang.Object ref = expression_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
expression_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int RESULT_FIELD_NUMBER = 2;
private int result_ = 0;
/**
*
* result is the result of the evaluation.
*
*
* .authzed.api.v1.CaveatEvalInfo.Result result = 2;
* @return The enum numeric value on the wire for result.
*/
@java.lang.Override public int getResultValue() {
return result_;
}
/**
*
* result is the result of the evaluation.
*
*
* .authzed.api.v1.CaveatEvalInfo.Result result = 2;
* @return The result.
*/
@java.lang.Override public com.authzed.api.v1.Debug.CaveatEvalInfo.Result getResult() {
com.authzed.api.v1.Debug.CaveatEvalInfo.Result result = com.authzed.api.v1.Debug.CaveatEvalInfo.Result.forNumber(result_);
return result == null ? com.authzed.api.v1.Debug.CaveatEvalInfo.Result.UNRECOGNIZED : result;
}
public static final int CONTEXT_FIELD_NUMBER = 3;
private com.google.protobuf.Struct context_;
/**
*
* context consists of any named values that were used for evaluating the caveat expression.
*
*
* .google.protobuf.Struct context = 3;
* @return Whether the context field is set.
*/
@java.lang.Override
public boolean hasContext() {
return context_ != null;
}
/**
*
* context consists of any named values that were used for evaluating the caveat expression.
*
*
* .google.protobuf.Struct context = 3;
* @return The context.
*/
@java.lang.Override
public com.google.protobuf.Struct getContext() {
return context_ == null ? com.google.protobuf.Struct.getDefaultInstance() : context_;
}
/**
*
* context consists of any named values that were used for evaluating the caveat expression.
*
*
* .google.protobuf.Struct context = 3;
*/
@java.lang.Override
public com.google.protobuf.StructOrBuilder getContextOrBuilder() {
return context_ == null ? com.google.protobuf.Struct.getDefaultInstance() : context_;
}
public static final int PARTIAL_CAVEAT_INFO_FIELD_NUMBER = 4;
private com.authzed.api.v1.Core.PartialCaveatInfo partialCaveatInfo_;
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response, if applicable.
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 4;
* @return Whether the partialCaveatInfo field is set.
*/
@java.lang.Override
public boolean hasPartialCaveatInfo() {
return partialCaveatInfo_ != null;
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response, if applicable.
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 4;
* @return The partialCaveatInfo.
*/
@java.lang.Override
public com.authzed.api.v1.Core.PartialCaveatInfo getPartialCaveatInfo() {
return partialCaveatInfo_ == null ? com.authzed.api.v1.Core.PartialCaveatInfo.getDefaultInstance() : partialCaveatInfo_;
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response, if applicable.
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 4;
*/
@java.lang.Override
public com.authzed.api.v1.Core.PartialCaveatInfoOrBuilder getPartialCaveatInfoOrBuilder() {
return partialCaveatInfo_ == null ? com.authzed.api.v1.Core.PartialCaveatInfo.getDefaultInstance() : partialCaveatInfo_;
}
public static final int CAVEAT_NAME_FIELD_NUMBER = 5;
@SuppressWarnings("serial")
private volatile java.lang.Object caveatName_ = "";
/**
*
* caveat_name is the name of the caveat that was executed, if applicable.
*
*
* string caveat_name = 5;
* @return The caveatName.
*/
@java.lang.Override
public java.lang.String getCaveatName() {
java.lang.Object ref = caveatName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
caveatName_ = s;
return s;
}
}
/**
*
* caveat_name is the name of the caveat that was executed, if applicable.
*
*
* string caveat_name = 5;
* @return The bytes for caveatName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getCaveatNameBytes() {
java.lang.Object ref = caveatName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
caveatName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(expression_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, expression_);
}
if (result_ != com.authzed.api.v1.Debug.CaveatEvalInfo.Result.RESULT_UNSPECIFIED.getNumber()) {
output.writeEnum(2, result_);
}
if (context_ != null) {
output.writeMessage(3, getContext());
}
if (partialCaveatInfo_ != null) {
output.writeMessage(4, getPartialCaveatInfo());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(caveatName_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, caveatName_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(expression_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, expression_);
}
if (result_ != com.authzed.api.v1.Debug.CaveatEvalInfo.Result.RESULT_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, result_);
}
if (context_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getContext());
}
if (partialCaveatInfo_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getPartialCaveatInfo());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(caveatName_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, caveatName_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.authzed.api.v1.Debug.CaveatEvalInfo)) {
return super.equals(obj);
}
com.authzed.api.v1.Debug.CaveatEvalInfo other = (com.authzed.api.v1.Debug.CaveatEvalInfo) obj;
if (!getExpression()
.equals(other.getExpression())) return false;
if (result_ != other.result_) return false;
if (hasContext() != other.hasContext()) return false;
if (hasContext()) {
if (!getContext()
.equals(other.getContext())) return false;
}
if (hasPartialCaveatInfo() != other.hasPartialCaveatInfo()) return false;
if (hasPartialCaveatInfo()) {
if (!getPartialCaveatInfo()
.equals(other.getPartialCaveatInfo())) return false;
}
if (!getCaveatName()
.equals(other.getCaveatName())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + EXPRESSION_FIELD_NUMBER;
hash = (53 * hash) + getExpression().hashCode();
hash = (37 * hash) + RESULT_FIELD_NUMBER;
hash = (53 * hash) + result_;
if (hasContext()) {
hash = (37 * hash) + CONTEXT_FIELD_NUMBER;
hash = (53 * hash) + getContext().hashCode();
}
if (hasPartialCaveatInfo()) {
hash = (37 * hash) + PARTIAL_CAVEAT_INFO_FIELD_NUMBER;
hash = (53 * hash) + getPartialCaveatInfo().hashCode();
}
hash = (37 * hash) + CAVEAT_NAME_FIELD_NUMBER;
hash = (53 * hash) + getCaveatName().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.authzed.api.v1.Debug.CaveatEvalInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.Debug.CaveatEvalInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.Debug.CaveatEvalInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.Debug.CaveatEvalInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.Debug.CaveatEvalInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.Debug.CaveatEvalInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.Debug.CaveatEvalInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.Debug.CaveatEvalInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.Debug.CaveatEvalInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.authzed.api.v1.Debug.CaveatEvalInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.Debug.CaveatEvalInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.Debug.CaveatEvalInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.authzed.api.v1.Debug.CaveatEvalInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* CaveatEvalInfo holds information about a caveat expression that was evaluated.
*
*
* Protobuf type {@code authzed.api.v1.CaveatEvalInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:authzed.api.v1.CaveatEvalInfo)
com.authzed.api.v1.Debug.CaveatEvalInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.Debug.internal_static_authzed_api_v1_CaveatEvalInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.Debug.internal_static_authzed_api_v1_CaveatEvalInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.Debug.CaveatEvalInfo.class, com.authzed.api.v1.Debug.CaveatEvalInfo.Builder.class);
}
// Construct using com.authzed.api.v1.Debug.CaveatEvalInfo.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
expression_ = "";
result_ = 0;
context_ = null;
if (contextBuilder_ != null) {
contextBuilder_.dispose();
contextBuilder_ = null;
}
partialCaveatInfo_ = null;
if (partialCaveatInfoBuilder_ != null) {
partialCaveatInfoBuilder_.dispose();
partialCaveatInfoBuilder_ = null;
}
caveatName_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.authzed.api.v1.Debug.internal_static_authzed_api_v1_CaveatEvalInfo_descriptor;
}
@java.lang.Override
public com.authzed.api.v1.Debug.CaveatEvalInfo getDefaultInstanceForType() {
return com.authzed.api.v1.Debug.CaveatEvalInfo.getDefaultInstance();
}
@java.lang.Override
public com.authzed.api.v1.Debug.CaveatEvalInfo build() {
com.authzed.api.v1.Debug.CaveatEvalInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.authzed.api.v1.Debug.CaveatEvalInfo buildPartial() {
com.authzed.api.v1.Debug.CaveatEvalInfo result = new com.authzed.api.v1.Debug.CaveatEvalInfo(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.authzed.api.v1.Debug.CaveatEvalInfo result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.expression_ = expression_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.result_ = result_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.context_ = contextBuilder_ == null
? context_
: contextBuilder_.build();
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.partialCaveatInfo_ = partialCaveatInfoBuilder_ == null
? partialCaveatInfo_
: partialCaveatInfoBuilder_.build();
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.caveatName_ = caveatName_;
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.authzed.api.v1.Debug.CaveatEvalInfo) {
return mergeFrom((com.authzed.api.v1.Debug.CaveatEvalInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.authzed.api.v1.Debug.CaveatEvalInfo other) {
if (other == com.authzed.api.v1.Debug.CaveatEvalInfo.getDefaultInstance()) return this;
if (!other.getExpression().isEmpty()) {
expression_ = other.expression_;
bitField0_ |= 0x00000001;
onChanged();
}
if (other.result_ != 0) {
setResultValue(other.getResultValue());
}
if (other.hasContext()) {
mergeContext(other.getContext());
}
if (other.hasPartialCaveatInfo()) {
mergePartialCaveatInfo(other.getPartialCaveatInfo());
}
if (!other.getCaveatName().isEmpty()) {
caveatName_ = other.caveatName_;
bitField0_ |= 0x00000010;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
expression_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 10
case 16: {
result_ = input.readEnum();
bitField0_ |= 0x00000002;
break;
} // case 16
case 26: {
input.readMessage(
getContextFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000004;
break;
} // case 26
case 34: {
input.readMessage(
getPartialCaveatInfoFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000008;
break;
} // case 34
case 42: {
caveatName_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000010;
break;
} // case 42
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object expression_ = "";
/**
*
* expression is the expression that was evaluated.
*
*
* string expression = 1;
* @return The expression.
*/
public java.lang.String getExpression() {
java.lang.Object ref = expression_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
expression_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* expression is the expression that was evaluated.
*
*
* string expression = 1;
* @return The bytes for expression.
*/
public com.google.protobuf.ByteString
getExpressionBytes() {
java.lang.Object ref = expression_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
expression_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* expression is the expression that was evaluated.
*
*
* string expression = 1;
* @param value The expression to set.
* @return This builder for chaining.
*/
public Builder setExpression(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
expression_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* expression is the expression that was evaluated.
*
*
* string expression = 1;
* @return This builder for chaining.
*/
public Builder clearExpression() {
expression_ = getDefaultInstance().getExpression();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
* expression is the expression that was evaluated.
*
*
* string expression = 1;
* @param value The bytes for expression to set.
* @return This builder for chaining.
*/
public Builder setExpressionBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
expression_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private int result_ = 0;
/**
*
* result is the result of the evaluation.
*
*
* .authzed.api.v1.CaveatEvalInfo.Result result = 2;
* @return The enum numeric value on the wire for result.
*/
@java.lang.Override public int getResultValue() {
return result_;
}
/**
*
* result is the result of the evaluation.
*
*
* .authzed.api.v1.CaveatEvalInfo.Result result = 2;
* @param value The enum numeric value on the wire for result to set.
* @return This builder for chaining.
*/
public Builder setResultValue(int value) {
result_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* result is the result of the evaluation.
*
*
* .authzed.api.v1.CaveatEvalInfo.Result result = 2;
* @return The result.
*/
@java.lang.Override
public com.authzed.api.v1.Debug.CaveatEvalInfo.Result getResult() {
com.authzed.api.v1.Debug.CaveatEvalInfo.Result result = com.authzed.api.v1.Debug.CaveatEvalInfo.Result.forNumber(result_);
return result == null ? com.authzed.api.v1.Debug.CaveatEvalInfo.Result.UNRECOGNIZED : result;
}
/**
*
* result is the result of the evaluation.
*
*
* .authzed.api.v1.CaveatEvalInfo.Result result = 2;
* @param value The result to set.
* @return This builder for chaining.
*/
public Builder setResult(com.authzed.api.v1.Debug.CaveatEvalInfo.Result value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
result_ = value.getNumber();
onChanged();
return this;
}
/**
*
* result is the result of the evaluation.
*
*
* .authzed.api.v1.CaveatEvalInfo.Result result = 2;
* @return This builder for chaining.
*/
public Builder clearResult() {
bitField0_ = (bitField0_ & ~0x00000002);
result_ = 0;
onChanged();
return this;
}
private com.google.protobuf.Struct context_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Struct, com.google.protobuf.Struct.Builder, com.google.protobuf.StructOrBuilder> contextBuilder_;
/**
*
* context consists of any named values that were used for evaluating the caveat expression.
*
*
* .google.protobuf.Struct context = 3;
* @return Whether the context field is set.
*/
public boolean hasContext() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* context consists of any named values that were used for evaluating the caveat expression.
*
*
* .google.protobuf.Struct context = 3;
* @return The context.
*/
public com.google.protobuf.Struct getContext() {
if (contextBuilder_ == null) {
return context_ == null ? com.google.protobuf.Struct.getDefaultInstance() : context_;
} else {
return contextBuilder_.getMessage();
}
}
/**
*
* context consists of any named values that were used for evaluating the caveat expression.
*
*
* .google.protobuf.Struct context = 3;
*/
public Builder setContext(com.google.protobuf.Struct value) {
if (contextBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
context_ = value;
} else {
contextBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* context consists of any named values that were used for evaluating the caveat expression.
*
*
* .google.protobuf.Struct context = 3;
*/
public Builder setContext(
com.google.protobuf.Struct.Builder builderForValue) {
if (contextBuilder_ == null) {
context_ = builderForValue.build();
} else {
contextBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* context consists of any named values that were used for evaluating the caveat expression.
*
*
* .google.protobuf.Struct context = 3;
*/
public Builder mergeContext(com.google.protobuf.Struct value) {
if (contextBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0) &&
context_ != null &&
context_ != com.google.protobuf.Struct.getDefaultInstance()) {
getContextBuilder().mergeFrom(value);
} else {
context_ = value;
}
} else {
contextBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* context consists of any named values that were used for evaluating the caveat expression.
*
*
* .google.protobuf.Struct context = 3;
*/
public Builder clearContext() {
bitField0_ = (bitField0_ & ~0x00000004);
context_ = null;
if (contextBuilder_ != null) {
contextBuilder_.dispose();
contextBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* context consists of any named values that were used for evaluating the caveat expression.
*
*
* .google.protobuf.Struct context = 3;
*/
public com.google.protobuf.Struct.Builder getContextBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getContextFieldBuilder().getBuilder();
}
/**
*
* context consists of any named values that were used for evaluating the caveat expression.
*
*
* .google.protobuf.Struct context = 3;
*/
public com.google.protobuf.StructOrBuilder getContextOrBuilder() {
if (contextBuilder_ != null) {
return contextBuilder_.getMessageOrBuilder();
} else {
return context_ == null ?
com.google.protobuf.Struct.getDefaultInstance() : context_;
}
}
/**
*
* context consists of any named values that were used for evaluating the caveat expression.
*
*
* .google.protobuf.Struct context = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Struct, com.google.protobuf.Struct.Builder, com.google.protobuf.StructOrBuilder>
getContextFieldBuilder() {
if (contextBuilder_ == null) {
contextBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Struct, com.google.protobuf.Struct.Builder, com.google.protobuf.StructOrBuilder>(
getContext(),
getParentForChildren(),
isClean());
context_ = null;
}
return contextBuilder_;
}
private com.authzed.api.v1.Core.PartialCaveatInfo partialCaveatInfo_;
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.PartialCaveatInfo, com.authzed.api.v1.Core.PartialCaveatInfo.Builder, com.authzed.api.v1.Core.PartialCaveatInfoOrBuilder> partialCaveatInfoBuilder_;
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response, if applicable.
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 4;
* @return Whether the partialCaveatInfo field is set.
*/
public boolean hasPartialCaveatInfo() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response, if applicable.
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 4;
* @return The partialCaveatInfo.
*/
public com.authzed.api.v1.Core.PartialCaveatInfo getPartialCaveatInfo() {
if (partialCaveatInfoBuilder_ == null) {
return partialCaveatInfo_ == null ? com.authzed.api.v1.Core.PartialCaveatInfo.getDefaultInstance() : partialCaveatInfo_;
} else {
return partialCaveatInfoBuilder_.getMessage();
}
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response, if applicable.
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 4;
*/
public Builder setPartialCaveatInfo(com.authzed.api.v1.Core.PartialCaveatInfo value) {
if (partialCaveatInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
partialCaveatInfo_ = value;
} else {
partialCaveatInfoBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response, if applicable.
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 4;
*/
public Builder setPartialCaveatInfo(
com.authzed.api.v1.Core.PartialCaveatInfo.Builder builderForValue) {
if (partialCaveatInfoBuilder_ == null) {
partialCaveatInfo_ = builderForValue.build();
} else {
partialCaveatInfoBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response, if applicable.
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 4;
*/
public Builder mergePartialCaveatInfo(com.authzed.api.v1.Core.PartialCaveatInfo value) {
if (partialCaveatInfoBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0) &&
partialCaveatInfo_ != null &&
partialCaveatInfo_ != com.authzed.api.v1.Core.PartialCaveatInfo.getDefaultInstance()) {
getPartialCaveatInfoBuilder().mergeFrom(value);
} else {
partialCaveatInfo_ = value;
}
} else {
partialCaveatInfoBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response, if applicable.
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 4;
*/
public Builder clearPartialCaveatInfo() {
bitField0_ = (bitField0_ & ~0x00000008);
partialCaveatInfo_ = null;
if (partialCaveatInfoBuilder_ != null) {
partialCaveatInfoBuilder_.dispose();
partialCaveatInfoBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response, if applicable.
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 4;
*/
public com.authzed.api.v1.Core.PartialCaveatInfo.Builder getPartialCaveatInfoBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getPartialCaveatInfoFieldBuilder().getBuilder();
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response, if applicable.
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 4;
*/
public com.authzed.api.v1.Core.PartialCaveatInfoOrBuilder getPartialCaveatInfoOrBuilder() {
if (partialCaveatInfoBuilder_ != null) {
return partialCaveatInfoBuilder_.getMessageOrBuilder();
} else {
return partialCaveatInfo_ == null ?
com.authzed.api.v1.Core.PartialCaveatInfo.getDefaultInstance() : partialCaveatInfo_;
}
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response, if applicable.
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.PartialCaveatInfo, com.authzed.api.v1.Core.PartialCaveatInfo.Builder, com.authzed.api.v1.Core.PartialCaveatInfoOrBuilder>
getPartialCaveatInfoFieldBuilder() {
if (partialCaveatInfoBuilder_ == null) {
partialCaveatInfoBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.PartialCaveatInfo, com.authzed.api.v1.Core.PartialCaveatInfo.Builder, com.authzed.api.v1.Core.PartialCaveatInfoOrBuilder>(
getPartialCaveatInfo(),
getParentForChildren(),
isClean());
partialCaveatInfo_ = null;
}
return partialCaveatInfoBuilder_;
}
private java.lang.Object caveatName_ = "";
/**
*
* caveat_name is the name of the caveat that was executed, if applicable.
*
*
* string caveat_name = 5;
* @return The caveatName.
*/
public java.lang.String getCaveatName() {
java.lang.Object ref = caveatName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
caveatName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* caveat_name is the name of the caveat that was executed, if applicable.
*
*
* string caveat_name = 5;
* @return The bytes for caveatName.
*/
public com.google.protobuf.ByteString
getCaveatNameBytes() {
java.lang.Object ref = caveatName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
caveatName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* caveat_name is the name of the caveat that was executed, if applicable.
*
*
* string caveat_name = 5;
* @param value The caveatName to set.
* @return This builder for chaining.
*/
public Builder setCaveatName(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
caveatName_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
* caveat_name is the name of the caveat that was executed, if applicable.
*
*
* string caveat_name = 5;
* @return This builder for chaining.
*/
public Builder clearCaveatName() {
caveatName_ = getDefaultInstance().getCaveatName();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
return this;
}
/**
*
* caveat_name is the name of the caveat that was executed, if applicable.
*
*
* string caveat_name = 5;
* @param value The bytes for caveatName to set.
* @return This builder for chaining.
*/
public Builder setCaveatNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
caveatName_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:authzed.api.v1.CaveatEvalInfo)
}
// @@protoc_insertion_point(class_scope:authzed.api.v1.CaveatEvalInfo)
private static final com.authzed.api.v1.Debug.CaveatEvalInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.authzed.api.v1.Debug.CaveatEvalInfo();
}
public static com.authzed.api.v1.Debug.CaveatEvalInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CaveatEvalInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.authzed.api.v1.Debug.CaveatEvalInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_authzed_api_v1_DebugInformation_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_authzed_api_v1_DebugInformation_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_authzed_api_v1_CheckDebugTrace_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_authzed_api_v1_CheckDebugTrace_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_authzed_api_v1_CheckDebugTrace_SubProblems_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_authzed_api_v1_CheckDebugTrace_SubProblems_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_authzed_api_v1_CaveatEvalInfo_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_authzed_api_v1_CaveatEvalInfo_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\032authzed/api/v1/debug.proto\022\016authzed.ap" +
"i.v1\032\031authzed/api/v1/core.proto\032\027validat" +
"e/validate.proto\032\034google/protobuf/struct" +
".proto\"W\n\020DebugInformation\022.\n\005check\030\001 \001(" +
"\0132\037.authzed.api.v1.CheckDebugTrace\022\023\n\013sc" +
"hema_used\030\002 \001(\t\"\311\006\n\017CheckDebugTrace\022;\n\010r" +
"esource\030\001 \001(\0132\037.authzed.api.v1.ObjectRef" +
"erenceB\010\372B\005\212\001\002\020\001\022\022\n\npermission\030\002 \001(\t\022S\n\017" +
"permission_type\030\003 \001(\0162..authzed.api.v1.C" +
"heckDebugTrace.PermissionTypeB\n\372B\007\202\001\004\020\001 " +
"\000\022;\n\007subject\030\004 \001(\0132 .authzed.api.v1.Subj" +
"ectReferenceB\010\372B\005\212\001\002\020\001\022J\n\006result\030\005 \001(\0162." +
".authzed.api.v1.CheckDebugTrace.Permissi" +
"onshipB\n\372B\007\202\001\004\020\001 \000\022>\n\026caveat_evaluation_" +
"info\030\010 \001(\0132\036.authzed.api.v1.CaveatEvalIn" +
"fo\022\033\n\021was_cached_result\030\006 \001(\010H\000\022C\n\014sub_p" +
"roblems\030\007 \001(\0132+.authzed.api.v1.CheckDebu" +
"gTrace.SubProblemsH\000\032>\n\013SubProblems\022/\n\006t" +
"races\030\001 \003(\0132\037.authzed.api.v1.CheckDebugT" +
"race\"o\n\016PermissionType\022\037\n\033PERMISSION_TYP" +
"E_UNSPECIFIED\020\000\022\034\n\030PERMISSION_TYPE_RELAT" +
"ION\020\001\022\036\n\032PERMISSION_TYPE_PERMISSION\020\002\"\240\001" +
"\n\016Permissionship\022\036\n\032PERMISSIONSHIP_UNSPE" +
"CIFIED\020\000\022 \n\034PERMISSIONSHIP_NO_PERMISSION" +
"\020\001\022!\n\035PERMISSIONSHIP_HAS_PERMISSION\020\002\022)\n" +
"%PERMISSIONSHIP_CONDITIONAL_PERMISSION\020\003" +
"B\021\n\nresolution\022\003\370B\001\"\330\002\n\016CaveatEvalInfo\022\022" +
"\n\nexpression\030\001 \001(\t\0225\n\006result\030\002 \001(\0162%.aut" +
"hzed.api.v1.CaveatEvalInfo.Result\022(\n\007con" +
"text\030\003 \001(\0132\027.google.protobuf.Struct\022>\n\023p" +
"artial_caveat_info\030\004 \001(\0132!.authzed.api.v" +
"1.PartialCaveatInfo\022\023\n\013caveat_name\030\005 \001(\t" +
"\"|\n\006Result\022\026\n\022RESULT_UNSPECIFIED\020\000\022\026\n\022RE" +
"SULT_UNEVALUATED\020\001\022\020\n\014RESULT_FALSE\020\002\022\017\n\013" +
"RESULT_TRUE\020\003\022\037\n\033RESULT_MISSING_SOME_CON" +
"TEXT\020\004BH\n\022com.authzed.api.v1Z2github.com" +
"/authzed/authzed-go/proto/authzed/api/v1" +
"b\006proto3"
};
descriptor = com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
com.authzed.api.v1.Core.getDescriptor(),
io.envoyproxy.pgv.validate.Validate.getDescriptor(),
com.google.protobuf.StructProto.getDescriptor(),
});
internal_static_authzed_api_v1_DebugInformation_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_authzed_api_v1_DebugInformation_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_authzed_api_v1_DebugInformation_descriptor,
new java.lang.String[] { "Check", "SchemaUsed", });
internal_static_authzed_api_v1_CheckDebugTrace_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_authzed_api_v1_CheckDebugTrace_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_authzed_api_v1_CheckDebugTrace_descriptor,
new java.lang.String[] { "Resource", "Permission", "PermissionType", "Subject", "Result", "CaveatEvaluationInfo", "WasCachedResult", "SubProblems", "Resolution", });
internal_static_authzed_api_v1_CheckDebugTrace_SubProblems_descriptor =
internal_static_authzed_api_v1_CheckDebugTrace_descriptor.getNestedTypes().get(0);
internal_static_authzed_api_v1_CheckDebugTrace_SubProblems_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_authzed_api_v1_CheckDebugTrace_SubProblems_descriptor,
new java.lang.String[] { "Traces", });
internal_static_authzed_api_v1_CaveatEvalInfo_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_authzed_api_v1_CaveatEvalInfo_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_authzed_api_v1_CaveatEvalInfo_descriptor,
new java.lang.String[] { "Expression", "Result", "Context", "PartialCaveatInfo", "CaveatName", });
com.google.protobuf.ExtensionRegistry registry =
com.google.protobuf.ExtensionRegistry.newInstance();
registry.add(io.envoyproxy.pgv.validate.Validate.required);
registry.add(io.envoyproxy.pgv.validate.Validate.rules);
com.google.protobuf.Descriptors.FileDescriptor
.internalUpdateFileDescriptor(descriptor, registry);
com.authzed.api.v1.Core.getDescriptor();
io.envoyproxy.pgv.validate.Validate.getDescriptor();
com.google.protobuf.StructProto.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy