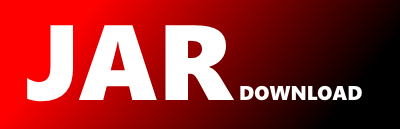
com.authzed.api.v1.ErrorReasonOuterClass Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: authzed/api/v1/error_reason.proto
package com.authzed.api.v1;
public final class ErrorReasonOuterClass {
private ErrorReasonOuterClass() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
/**
*
* Defines the supported values for `google.rpc.ErrorInfo.reason` for the
* `authzed.com` error domain.
*
*
* Protobuf enum {@code authzed.api.v1.ErrorReason}
*/
public enum ErrorReason
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* Do not use this default value.
*
*
* ERROR_REASON_UNSPECIFIED = 0;
*/
ERROR_REASON_UNSPECIFIED(0),
/**
*
* The request gave a schema that could not be parsed.
* Example of an ErrorInfo:
* {
* "reason": "ERROR_REASON_SCHEMA_PARSE_ERROR",
* "domain": "authzed.com",
* "metadata": {
* "start_line_number": "1",
* "start_column_position": "19",
* "end_line_number": "1",
* "end_column_position": "19",
* "source_code": "somedefinition",
* }
* }
* The line numbers and column positions are 0-indexed and may not be present.
*
*
* ERROR_REASON_SCHEMA_PARSE_ERROR = 1;
*/
ERROR_REASON_SCHEMA_PARSE_ERROR(1),
/**
*
* The request contains a schema with a type error.
* Example of an ErrorInfo:
* {
* "reason": "ERROR_REASON_SCHEMA_TYPE_ERROR",
* "domain": "authzed.com",
* "metadata": {
* "definition_name": "somedefinition",
* ... additional keys based on the kind of type error ...
* }
* }
*
*
* ERROR_REASON_SCHEMA_TYPE_ERROR = 2;
*/
ERROR_REASON_SCHEMA_TYPE_ERROR(2),
/**
*
* The request referenced an unknown object definition in the schema.
* Example of an ErrorInfo:
* {
* "reason": "ERROR_REASON_UNKNOWN_DEFINITION",
* "domain": "authzed.com",
* "metadata": {
* "definition_name": "somedefinition"
* }
* }
*
*
* ERROR_REASON_UNKNOWN_DEFINITION = 3;
*/
ERROR_REASON_UNKNOWN_DEFINITION(3),
/**
*
* The request referenced an unknown relation or permission under a definition in the schema.
* Example of an ErrorInfo:
* {
* "reason": "ERROR_REASON_UNKNOWN_RELATION_OR_PERMISSION",
* "domain": "authzed.com",
* "metadata": {
* "definition_name": "somedefinition",
* "relation_or_permission_name": "somepermission"
* }
* }
*
*
* ERROR_REASON_UNKNOWN_RELATION_OR_PERMISSION = 4;
*/
ERROR_REASON_UNKNOWN_RELATION_OR_PERMISSION(4),
/**
*
* The WriteRelationships request contained more updates than the maximum configured.
* Example of an ErrorInfo:
* { "reason": "ERROR_REASON_TOO_MANY_UPDATES_IN_REQUEST",
* "domain": "authzed.com",
* "metadata": {
* "update_count": "525",
* "maximum_updates_allowed": "500",
* }
* }
*
*
* ERROR_REASON_TOO_MANY_UPDATES_IN_REQUEST = 5;
*/
ERROR_REASON_TOO_MANY_UPDATES_IN_REQUEST(5),
/**
*
* The request contained more preconditions than the maximum configured.
* Example of an ErrorInfo:
* {
* "reason": "ERROR_REASON_TOO_MANY_PRECONDITIONS_IN_REQUEST",
* "domain": "authzed.com",
* "metadata": {
* "precondition_count": "525",
* "maximum_preconditions_allowed": "500",
* }
* }
*
*
* ERROR_REASON_TOO_MANY_PRECONDITIONS_IN_REQUEST = 6;
*/
ERROR_REASON_TOO_MANY_PRECONDITIONS_IN_REQUEST(6),
/**
*
* The request contained a precondition that failed.
* Example of an ErrorInfo:
* {
* "reason": "ERROR_REASON_WRITE_OR_DELETE_PRECONDITION_FAILURE",
* "domain": "authzed.com",
* "metadata": {
* "precondition_resource_type": "document",
* ... other fields for the filter ...
* "precondition_operation": "MUST_EXIST",
* }
* }
*
*
* ERROR_REASON_WRITE_OR_DELETE_PRECONDITION_FAILURE = 7;
*/
ERROR_REASON_WRITE_OR_DELETE_PRECONDITION_FAILURE(7),
/**
*
* A write or delete request was made to an instance that is deployed in read-only mode.
* Example of an ErrorInfo:
* {
* "reason": "ERROR_REASON_SERVICE_READ_ONLY",
* "domain": "authzed.com"
* }
*
*
* ERROR_REASON_SERVICE_READ_ONLY = 8;
*/
ERROR_REASON_SERVICE_READ_ONLY(8),
/**
*
* The request referenced an unknown caveat in the schema.
* Example of an ErrorInfo:
* {
* "reason": "ERROR_REASON_UNKNOWN_CAVEAT",
* "domain": "authzed.com",
* "metadata": {
* "caveat_name": "somecaveat"
* }
* }
*
*
* ERROR_REASON_UNKNOWN_CAVEAT = 9;
*/
ERROR_REASON_UNKNOWN_CAVEAT(9),
/**
*
* The request tries to use a subject type that was not valid for a relation.
* Example of an ErrorInfo:
* {
* "reason": "ERROR_REASON_INVALID_SUBJECT_TYPE",
* "domain": "authzed.com",
* "metadata": {
* "definition_name": "somedefinition",
* "relation_name": "somerelation",
* "subject_type": "user:*"
* }
* }
*
*
* ERROR_REASON_INVALID_SUBJECT_TYPE = 10;
*/
ERROR_REASON_INVALID_SUBJECT_TYPE(10),
/**
*
* The request tries to specify a caveat parameter value with the wrong type.
* Example of an ErrorInfo:
* {
* "reason": "ERROR_REASON_CAVEAT_PARAMETER_TYPE_ERROR",
* "domain": "authzed.com",
* "metadata": {
* "definition_name": "somedefinition",
* "relation_name": "somerelation",
* "caveat_name": "somecaveat",
* "parameter_name": "someparameter",
* "expected_type": "int",
* }
* }
*
*
* ERROR_REASON_CAVEAT_PARAMETER_TYPE_ERROR = 11;
*/
ERROR_REASON_CAVEAT_PARAMETER_TYPE_ERROR(11),
/**
*
* The request tries to perform two or more updates on the same relationship in the same WriteRelationships call.
* Example of an ErrorInfo:
* {
* "reason": "ERROR_REASON_UPDATES_ON_SAME_RELATIONSHIP",
* "domain": "authzed.com",
* "metadata": {
* "definition_name": "somedefinition",
* "relationship": "somerelationship",
* }
* }
*
*
* ERROR_REASON_UPDATES_ON_SAME_RELATIONSHIP = 12;
*/
ERROR_REASON_UPDATES_ON_SAME_RELATIONSHIP(12),
/**
*
* The request tries to write a relationship on a permission instead of a relation.
* Example of an ErrorInfo:
* {
* "reason": "ERROR_REASON_CANNOT_UPDATE_PERMISSION",
* "domain": "authzed.com",
* "metadata": {
* "definition_name": "somedefinition",
* "permission_name": "somerelation",
* }
* }
*
*
* ERROR_REASON_CANNOT_UPDATE_PERMISSION = 13;
*/
ERROR_REASON_CANNOT_UPDATE_PERMISSION(13),
/**
*
* The request failed to evaluate a caveat expression due to an error.
* Example of an ErrorInfo:
* {
* "reason": "ERROR_REASON_CAVEAT_EVALUATION_ERROR",
* "domain": "authzed.com",
* "metadata": {
* "caveat_name": "somecaveat",
* }
* }
*
*
* ERROR_REASON_CAVEAT_EVALUATION_ERROR = 14;
*/
ERROR_REASON_CAVEAT_EVALUATION_ERROR(14),
UNRECOGNIZED(-1),
;
/**
*
* Do not use this default value.
*
*
* ERROR_REASON_UNSPECIFIED = 0;
*/
public static final int ERROR_REASON_UNSPECIFIED_VALUE = 0;
/**
*
* The request gave a schema that could not be parsed.
* Example of an ErrorInfo:
* {
* "reason": "ERROR_REASON_SCHEMA_PARSE_ERROR",
* "domain": "authzed.com",
* "metadata": {
* "start_line_number": "1",
* "start_column_position": "19",
* "end_line_number": "1",
* "end_column_position": "19",
* "source_code": "somedefinition",
* }
* }
* The line numbers and column positions are 0-indexed and may not be present.
*
*
* ERROR_REASON_SCHEMA_PARSE_ERROR = 1;
*/
public static final int ERROR_REASON_SCHEMA_PARSE_ERROR_VALUE = 1;
/**
*
* The request contains a schema with a type error.
* Example of an ErrorInfo:
* {
* "reason": "ERROR_REASON_SCHEMA_TYPE_ERROR",
* "domain": "authzed.com",
* "metadata": {
* "definition_name": "somedefinition",
* ... additional keys based on the kind of type error ...
* }
* }
*
*
* ERROR_REASON_SCHEMA_TYPE_ERROR = 2;
*/
public static final int ERROR_REASON_SCHEMA_TYPE_ERROR_VALUE = 2;
/**
*
* The request referenced an unknown object definition in the schema.
* Example of an ErrorInfo:
* {
* "reason": "ERROR_REASON_UNKNOWN_DEFINITION",
* "domain": "authzed.com",
* "metadata": {
* "definition_name": "somedefinition"
* }
* }
*
*
* ERROR_REASON_UNKNOWN_DEFINITION = 3;
*/
public static final int ERROR_REASON_UNKNOWN_DEFINITION_VALUE = 3;
/**
*
* The request referenced an unknown relation or permission under a definition in the schema.
* Example of an ErrorInfo:
* {
* "reason": "ERROR_REASON_UNKNOWN_RELATION_OR_PERMISSION",
* "domain": "authzed.com",
* "metadata": {
* "definition_name": "somedefinition",
* "relation_or_permission_name": "somepermission"
* }
* }
*
*
* ERROR_REASON_UNKNOWN_RELATION_OR_PERMISSION = 4;
*/
public static final int ERROR_REASON_UNKNOWN_RELATION_OR_PERMISSION_VALUE = 4;
/**
*
* The WriteRelationships request contained more updates than the maximum configured.
* Example of an ErrorInfo:
* { "reason": "ERROR_REASON_TOO_MANY_UPDATES_IN_REQUEST",
* "domain": "authzed.com",
* "metadata": {
* "update_count": "525",
* "maximum_updates_allowed": "500",
* }
* }
*
*
* ERROR_REASON_TOO_MANY_UPDATES_IN_REQUEST = 5;
*/
public static final int ERROR_REASON_TOO_MANY_UPDATES_IN_REQUEST_VALUE = 5;
/**
*
* The request contained more preconditions than the maximum configured.
* Example of an ErrorInfo:
* {
* "reason": "ERROR_REASON_TOO_MANY_PRECONDITIONS_IN_REQUEST",
* "domain": "authzed.com",
* "metadata": {
* "precondition_count": "525",
* "maximum_preconditions_allowed": "500",
* }
* }
*
*
* ERROR_REASON_TOO_MANY_PRECONDITIONS_IN_REQUEST = 6;
*/
public static final int ERROR_REASON_TOO_MANY_PRECONDITIONS_IN_REQUEST_VALUE = 6;
/**
*
* The request contained a precondition that failed.
* Example of an ErrorInfo:
* {
* "reason": "ERROR_REASON_WRITE_OR_DELETE_PRECONDITION_FAILURE",
* "domain": "authzed.com",
* "metadata": {
* "precondition_resource_type": "document",
* ... other fields for the filter ...
* "precondition_operation": "MUST_EXIST",
* }
* }
*
*
* ERROR_REASON_WRITE_OR_DELETE_PRECONDITION_FAILURE = 7;
*/
public static final int ERROR_REASON_WRITE_OR_DELETE_PRECONDITION_FAILURE_VALUE = 7;
/**
*
* A write or delete request was made to an instance that is deployed in read-only mode.
* Example of an ErrorInfo:
* {
* "reason": "ERROR_REASON_SERVICE_READ_ONLY",
* "domain": "authzed.com"
* }
*
*
* ERROR_REASON_SERVICE_READ_ONLY = 8;
*/
public static final int ERROR_REASON_SERVICE_READ_ONLY_VALUE = 8;
/**
*
* The request referenced an unknown caveat in the schema.
* Example of an ErrorInfo:
* {
* "reason": "ERROR_REASON_UNKNOWN_CAVEAT",
* "domain": "authzed.com",
* "metadata": {
* "caveat_name": "somecaveat"
* }
* }
*
*
* ERROR_REASON_UNKNOWN_CAVEAT = 9;
*/
public static final int ERROR_REASON_UNKNOWN_CAVEAT_VALUE = 9;
/**
*
* The request tries to use a subject type that was not valid for a relation.
* Example of an ErrorInfo:
* {
* "reason": "ERROR_REASON_INVALID_SUBJECT_TYPE",
* "domain": "authzed.com",
* "metadata": {
* "definition_name": "somedefinition",
* "relation_name": "somerelation",
* "subject_type": "user:*"
* }
* }
*
*
* ERROR_REASON_INVALID_SUBJECT_TYPE = 10;
*/
public static final int ERROR_REASON_INVALID_SUBJECT_TYPE_VALUE = 10;
/**
*
* The request tries to specify a caveat parameter value with the wrong type.
* Example of an ErrorInfo:
* {
* "reason": "ERROR_REASON_CAVEAT_PARAMETER_TYPE_ERROR",
* "domain": "authzed.com",
* "metadata": {
* "definition_name": "somedefinition",
* "relation_name": "somerelation",
* "caveat_name": "somecaveat",
* "parameter_name": "someparameter",
* "expected_type": "int",
* }
* }
*
*
* ERROR_REASON_CAVEAT_PARAMETER_TYPE_ERROR = 11;
*/
public static final int ERROR_REASON_CAVEAT_PARAMETER_TYPE_ERROR_VALUE = 11;
/**
*
* The request tries to perform two or more updates on the same relationship in the same WriteRelationships call.
* Example of an ErrorInfo:
* {
* "reason": "ERROR_REASON_UPDATES_ON_SAME_RELATIONSHIP",
* "domain": "authzed.com",
* "metadata": {
* "definition_name": "somedefinition",
* "relationship": "somerelationship",
* }
* }
*
*
* ERROR_REASON_UPDATES_ON_SAME_RELATIONSHIP = 12;
*/
public static final int ERROR_REASON_UPDATES_ON_SAME_RELATIONSHIP_VALUE = 12;
/**
*
* The request tries to write a relationship on a permission instead of a relation.
* Example of an ErrorInfo:
* {
* "reason": "ERROR_REASON_CANNOT_UPDATE_PERMISSION",
* "domain": "authzed.com",
* "metadata": {
* "definition_name": "somedefinition",
* "permission_name": "somerelation",
* }
* }
*
*
* ERROR_REASON_CANNOT_UPDATE_PERMISSION = 13;
*/
public static final int ERROR_REASON_CANNOT_UPDATE_PERMISSION_VALUE = 13;
/**
*
* The request failed to evaluate a caveat expression due to an error.
* Example of an ErrorInfo:
* {
* "reason": "ERROR_REASON_CAVEAT_EVALUATION_ERROR",
* "domain": "authzed.com",
* "metadata": {
* "caveat_name": "somecaveat",
* }
* }
*
*
* ERROR_REASON_CAVEAT_EVALUATION_ERROR = 14;
*/
public static final int ERROR_REASON_CAVEAT_EVALUATION_ERROR_VALUE = 14;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ErrorReason valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static ErrorReason forNumber(int value) {
switch (value) {
case 0: return ERROR_REASON_UNSPECIFIED;
case 1: return ERROR_REASON_SCHEMA_PARSE_ERROR;
case 2: return ERROR_REASON_SCHEMA_TYPE_ERROR;
case 3: return ERROR_REASON_UNKNOWN_DEFINITION;
case 4: return ERROR_REASON_UNKNOWN_RELATION_OR_PERMISSION;
case 5: return ERROR_REASON_TOO_MANY_UPDATES_IN_REQUEST;
case 6: return ERROR_REASON_TOO_MANY_PRECONDITIONS_IN_REQUEST;
case 7: return ERROR_REASON_WRITE_OR_DELETE_PRECONDITION_FAILURE;
case 8: return ERROR_REASON_SERVICE_READ_ONLY;
case 9: return ERROR_REASON_UNKNOWN_CAVEAT;
case 10: return ERROR_REASON_INVALID_SUBJECT_TYPE;
case 11: return ERROR_REASON_CAVEAT_PARAMETER_TYPE_ERROR;
case 12: return ERROR_REASON_UPDATES_ON_SAME_RELATIONSHIP;
case 13: return ERROR_REASON_CANNOT_UPDATE_PERMISSION;
case 14: return ERROR_REASON_CAVEAT_EVALUATION_ERROR;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
ErrorReason> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public ErrorReason findValueByNumber(int number) {
return ErrorReason.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.authzed.api.v1.ErrorReasonOuterClass.getDescriptor().getEnumTypes().get(0);
}
private static final ErrorReason[] VALUES = values();
public static ErrorReason valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private ErrorReason(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:authzed.api.v1.ErrorReason)
}
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n!authzed/api/v1/error_reason.proto\022\016aut" +
"hzed.api.v1*\201\005\n\013ErrorReason\022\034\n\030ERROR_REA" +
"SON_UNSPECIFIED\020\000\022#\n\037ERROR_REASON_SCHEMA" +
"_PARSE_ERROR\020\001\022\"\n\036ERROR_REASON_SCHEMA_TY" +
"PE_ERROR\020\002\022#\n\037ERROR_REASON_UNKNOWN_DEFIN" +
"ITION\020\003\022/\n+ERROR_REASON_UNKNOWN_RELATION" +
"_OR_PERMISSION\020\004\022,\n(ERROR_REASON_TOO_MAN" +
"Y_UPDATES_IN_REQUEST\020\005\0222\n.ERROR_REASON_T" +
"OO_MANY_PRECONDITIONS_IN_REQUEST\020\006\0225\n1ER" +
"ROR_REASON_WRITE_OR_DELETE_PRECONDITION_" +
"FAILURE\020\007\022\"\n\036ERROR_REASON_SERVICE_READ_O" +
"NLY\020\010\022\037\n\033ERROR_REASON_UNKNOWN_CAVEAT\020\t\022%" +
"\n!ERROR_REASON_INVALID_SUBJECT_TYPE\020\n\022,\n" +
"(ERROR_REASON_CAVEAT_PARAMETER_TYPE_ERRO" +
"R\020\013\022-\n)ERROR_REASON_UPDATES_ON_SAME_RELA" +
"TIONSHIP\020\014\022)\n%ERROR_REASON_CANNOT_UPDATE" +
"_PERMISSION\020\r\022(\n$ERROR_REASON_CAVEAT_EVA" +
"LUATION_ERROR\020\016BH\n\022com.authzed.api.v1Z2g" +
"ithub.com/authzed/authzed-go/proto/authz" +
"ed/api/v1b\006proto3"
};
descriptor = com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
});
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy