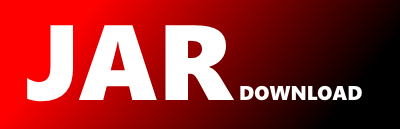
com.authzed.api.v1.LookupSubjectsRequestKt.kt Maven / Gradle / Ivy
The newest version!
//Generated by the protocol buffer compiler. DO NOT EDIT!
// source: authzed/api/v1/permission_service.proto
package com.authzed.api.v1;
@kotlin.jvm.JvmName("-initializelookupSubjectsRequest")
public inline fun lookupSubjectsRequest(block: com.authzed.api.v1.LookupSubjectsRequestKt.Dsl.() -> kotlin.Unit): com.authzed.api.v1.PermissionService.LookupSubjectsRequest =
com.authzed.api.v1.LookupSubjectsRequestKt.Dsl._create(com.authzed.api.v1.PermissionService.LookupSubjectsRequest.newBuilder()).apply { block() }._build()
public object LookupSubjectsRequestKt {
@kotlin.OptIn(com.google.protobuf.kotlin.OnlyForUseByGeneratedProtoCode::class)
@com.google.protobuf.kotlin.ProtoDslMarker
public class Dsl private constructor(
private val _builder: com.authzed.api.v1.PermissionService.LookupSubjectsRequest.Builder
) {
public companion object {
@kotlin.jvm.JvmSynthetic
@kotlin.PublishedApi
internal fun _create(builder: com.authzed.api.v1.PermissionService.LookupSubjectsRequest.Builder): Dsl = Dsl(builder)
}
@kotlin.jvm.JvmSynthetic
@kotlin.PublishedApi
internal fun _build(): com.authzed.api.v1.PermissionService.LookupSubjectsRequest = _builder.build()
/**
* .authzed.api.v1.Consistency consistency = 1;
*/
public var consistency: com.authzed.api.v1.PermissionService.Consistency
@JvmName("getConsistency")
get() = _builder.getConsistency()
@JvmName("setConsistency")
set(value) {
_builder.setConsistency(value)
}
/**
* .authzed.api.v1.Consistency consistency = 1;
*/
public fun clearConsistency() {
_builder.clearConsistency()
}
/**
* .authzed.api.v1.Consistency consistency = 1;
* @return Whether the consistency field is set.
*/
public fun hasConsistency(): kotlin.Boolean {
return _builder.hasConsistency()
}
/**
*
* resource is the resource for which all matching subjects for the permission
* or relation will be returned.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
*/
public var resource: com.authzed.api.v1.Core.ObjectReference
@JvmName("getResource")
get() = _builder.getResource()
@JvmName("setResource")
set(value) {
_builder.setResource(value)
}
/**
*
* resource is the resource for which all matching subjects for the permission
* or relation will be returned.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
*/
public fun clearResource() {
_builder.clearResource()
}
/**
*
* resource is the resource for which all matching subjects for the permission
* or relation will be returned.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
* @return Whether the resource field is set.
*/
public fun hasResource(): kotlin.Boolean {
return _builder.hasResource()
}
/**
*
* permission is the name of the permission (or relation) for which to find
* the subjects.
*
*
* string permission = 3 [(.validate.rules) = { ... }
*/
public var permission: kotlin.String
@JvmName("getPermission")
get() = _builder.getPermission()
@JvmName("setPermission")
set(value) {
_builder.setPermission(value)
}
/**
*
* permission is the name of the permission (or relation) for which to find
* the subjects.
*
*
* string permission = 3 [(.validate.rules) = { ... }
*/
public fun clearPermission() {
_builder.clearPermission()
}
/**
*
* subject_object_type is the type of subject object for which the IDs will
* be returned.
*
*
* string subject_object_type = 4 [(.validate.rules) = { ... }
*/
public var subjectObjectType: kotlin.String
@JvmName("getSubjectObjectType")
get() = _builder.getSubjectObjectType()
@JvmName("setSubjectObjectType")
set(value) {
_builder.setSubjectObjectType(value)
}
/**
*
* subject_object_type is the type of subject object for which the IDs will
* be returned.
*
*
* string subject_object_type = 4 [(.validate.rules) = { ... }
*/
public fun clearSubjectObjectType() {
_builder.clearSubjectObjectType()
}
/**
*
* optional_subject_relation is the optional relation for the subject.
*
*
* string optional_subject_relation = 5 [(.validate.rules) = { ... }
*/
public var optionalSubjectRelation: kotlin.String
@JvmName("getOptionalSubjectRelation")
get() = _builder.getOptionalSubjectRelation()
@JvmName("setOptionalSubjectRelation")
set(value) {
_builder.setOptionalSubjectRelation(value)
}
/**
*
* optional_subject_relation is the optional relation for the subject.
*
*
* string optional_subject_relation = 5 [(.validate.rules) = { ... }
*/
public fun clearOptionalSubjectRelation() {
_builder.clearOptionalSubjectRelation()
}
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 6 [(.validate.rules) = { ... }
*/
public var context: com.google.protobuf.Struct
@JvmName("getContext")
get() = _builder.getContext()
@JvmName("setContext")
set(value) {
_builder.setContext(value)
}
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 6 [(.validate.rules) = { ... }
*/
public fun clearContext() {
_builder.clearContext()
}
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 6 [(.validate.rules) = { ... }
* @return Whether the context field is set.
*/
public fun hasContext(): kotlin.Boolean {
return _builder.hasContext()
}
}
}
@kotlin.jvm.JvmSynthetic
public inline fun com.authzed.api.v1.PermissionService.LookupSubjectsRequest.copy(block: com.authzed.api.v1.LookupSubjectsRequestKt.Dsl.() -> kotlin.Unit): com.authzed.api.v1.PermissionService.LookupSubjectsRequest =
com.authzed.api.v1.LookupSubjectsRequestKt.Dsl._create(this.toBuilder()).apply { block() }._build()
public val com.authzed.api.v1.PermissionService.LookupSubjectsRequestOrBuilder.consistencyOrNull: com.authzed.api.v1.PermissionService.Consistency?
get() = if (hasConsistency()) getConsistency() else null
public val com.authzed.api.v1.PermissionService.LookupSubjectsRequestOrBuilder.resourceOrNull: com.authzed.api.v1.Core.ObjectReference?
get() = if (hasResource()) getResource() else null
public val com.authzed.api.v1.PermissionService.LookupSubjectsRequestOrBuilder.contextOrNull: com.google.protobuf.Struct?
get() = if (hasContext()) getContext() else null
© 2015 - 2025 Weber Informatics LLC | Privacy Policy