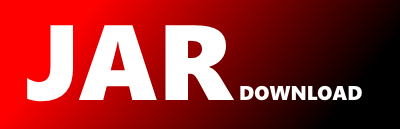
com.authzed.api.v1.LookupSubjectsResponseKt.kt Maven / Gradle / Ivy
The newest version!
//Generated by the protocol buffer compiler. DO NOT EDIT!
// source: authzed/api/v1/permission_service.proto
package com.authzed.api.v1;
@kotlin.jvm.JvmName("-initializelookupSubjectsResponse")
public inline fun lookupSubjectsResponse(block: com.authzed.api.v1.LookupSubjectsResponseKt.Dsl.() -> kotlin.Unit): com.authzed.api.v1.PermissionService.LookupSubjectsResponse =
com.authzed.api.v1.LookupSubjectsResponseKt.Dsl._create(com.authzed.api.v1.PermissionService.LookupSubjectsResponse.newBuilder()).apply { block() }._build()
public object LookupSubjectsResponseKt {
@kotlin.OptIn(com.google.protobuf.kotlin.OnlyForUseByGeneratedProtoCode::class)
@com.google.protobuf.kotlin.ProtoDslMarker
public class Dsl private constructor(
private val _builder: com.authzed.api.v1.PermissionService.LookupSubjectsResponse.Builder
) {
public companion object {
@kotlin.jvm.JvmSynthetic
@kotlin.PublishedApi
internal fun _create(builder: com.authzed.api.v1.PermissionService.LookupSubjectsResponse.Builder): Dsl = Dsl(builder)
}
@kotlin.jvm.JvmSynthetic
@kotlin.PublishedApi
internal fun _build(): com.authzed.api.v1.PermissionService.LookupSubjectsResponse = _builder.build()
/**
* .authzed.api.v1.ZedToken looked_up_at = 1;
*/
public var lookedUpAt: com.authzed.api.v1.Core.ZedToken
@JvmName("getLookedUpAt")
get() = _builder.getLookedUpAt()
@JvmName("setLookedUpAt")
set(value) {
_builder.setLookedUpAt(value)
}
/**
* .authzed.api.v1.ZedToken looked_up_at = 1;
*/
public fun clearLookedUpAt() {
_builder.clearLookedUpAt()
}
/**
* .authzed.api.v1.ZedToken looked_up_at = 1;
* @return Whether the lookedUpAt field is set.
*/
public fun hasLookedUpAt(): kotlin.Boolean {
return _builder.hasLookedUpAt()
}
/**
*
* subject_object_id is the Object ID of the subject found. May be a `*` if
* a wildcard was found.
* deprecated: use `subject`
*
*
* string subject_object_id = 2 [deprecated = true];
*/
@kotlin.Deprecated(message = "Field subjectObjectId is deprecated") public var subjectObjectId: kotlin.String
@JvmName("getSubjectObjectId")
get() = _builder.getSubjectObjectId()
@JvmName("setSubjectObjectId")
set(value) {
_builder.setSubjectObjectId(value)
}
/**
*
* subject_object_id is the Object ID of the subject found. May be a `*` if
* a wildcard was found.
* deprecated: use `subject`
*
*
* string subject_object_id = 2 [deprecated = true];
* @deprecated authzed.api.v1.LookupSubjectsResponse.subject_object_id is deprecated.
* See authzed/api/v1/permission_service.proto;l=401
*/
public fun clearSubjectObjectId() {
_builder.clearSubjectObjectId()
}
/**
* An uninstantiable, behaviorless type to represent the field in
* generics.
*/
@kotlin.OptIn(com.google.protobuf.kotlin.OnlyForUseByGeneratedProtoCode::class)
public class ExcludedSubjectIdsProxy private constructor() : com.google.protobuf.kotlin.DslProxy()
/**
*
* excluded_subject_ids are the Object IDs of the subjects excluded. This list
* will only contain object IDs if `subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
* deprecated: use `excluded_subjects`
*
*
* repeated string excluded_subject_ids = 3 [deprecated = true];
* @deprecated authzed.api.v1.LookupSubjectsResponse.excluded_subject_ids is deprecated.
* See authzed/api/v1/permission_service.proto;l=407
* @return A list containing the excludedSubjectIds.
*/
@kotlin.Deprecated(message = "Field excludedSubjectIds is deprecated") public val excludedSubjectIds: com.google.protobuf.kotlin.DslList
@kotlin.jvm.JvmSynthetic
get() = com.google.protobuf.kotlin.DslList(
_builder.getExcludedSubjectIdsList()
)
/**
*
* excluded_subject_ids are the Object IDs of the subjects excluded. This list
* will only contain object IDs if `subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
* deprecated: use `excluded_subjects`
*
*
* repeated string excluded_subject_ids = 3 [deprecated = true];
* @deprecated authzed.api.v1.LookupSubjectsResponse.excluded_subject_ids is deprecated.
* See authzed/api/v1/permission_service.proto;l=407
* @param value The excludedSubjectIds to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("addExcludedSubjectIds")
public fun com.google.protobuf.kotlin.DslList.add(value: kotlin.String) {
_builder.addExcludedSubjectIds(value)
}
/**
*
* excluded_subject_ids are the Object IDs of the subjects excluded. This list
* will only contain object IDs if `subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
* deprecated: use `excluded_subjects`
*
*
* repeated string excluded_subject_ids = 3 [deprecated = true];
* @deprecated authzed.api.v1.LookupSubjectsResponse.excluded_subject_ids is deprecated.
* See authzed/api/v1/permission_service.proto;l=407
* @param value The excludedSubjectIds to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("plusAssignExcludedSubjectIds")
@Suppress("NOTHING_TO_INLINE")
public inline operator fun com.google.protobuf.kotlin.DslList.plusAssign(value: kotlin.String) {
add(value)
}
/**
*
* excluded_subject_ids are the Object IDs of the subjects excluded. This list
* will only contain object IDs if `subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
* deprecated: use `excluded_subjects`
*
*
* repeated string excluded_subject_ids = 3 [deprecated = true];
* @deprecated authzed.api.v1.LookupSubjectsResponse.excluded_subject_ids is deprecated.
* See authzed/api/v1/permission_service.proto;l=407
* @param values The excludedSubjectIds to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("addAllExcludedSubjectIds")
public fun com.google.protobuf.kotlin.DslList.addAll(values: kotlin.collections.Iterable) {
_builder.addAllExcludedSubjectIds(values)
}
/**
*
* excluded_subject_ids are the Object IDs of the subjects excluded. This list
* will only contain object IDs if `subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
* deprecated: use `excluded_subjects`
*
*
* repeated string excluded_subject_ids = 3 [deprecated = true];
* @deprecated authzed.api.v1.LookupSubjectsResponse.excluded_subject_ids is deprecated.
* See authzed/api/v1/permission_service.proto;l=407
* @param values The excludedSubjectIds to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("plusAssignAllExcludedSubjectIds")
@Suppress("NOTHING_TO_INLINE")
public inline operator fun com.google.protobuf.kotlin.DslList.plusAssign(values: kotlin.collections.Iterable) {
addAll(values)
}
/**
*
* excluded_subject_ids are the Object IDs of the subjects excluded. This list
* will only contain object IDs if `subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
* deprecated: use `excluded_subjects`
*
*
* repeated string excluded_subject_ids = 3 [deprecated = true];
* @deprecated authzed.api.v1.LookupSubjectsResponse.excluded_subject_ids is deprecated.
* See authzed/api/v1/permission_service.proto;l=407
* @param index The index to set the value at.
* @param value The excludedSubjectIds to set.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("setExcludedSubjectIds")
public operator fun com.google.protobuf.kotlin.DslList.set(index: kotlin.Int, value: kotlin.String) {
_builder.setExcludedSubjectIds(index, value)
}/**
*
* excluded_subject_ids are the Object IDs of the subjects excluded. This list
* will only contain object IDs if `subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
* deprecated: use `excluded_subjects`
*
*
* repeated string excluded_subject_ids = 3 [deprecated = true];
* @deprecated authzed.api.v1.LookupSubjectsResponse.excluded_subject_ids is deprecated.
* See authzed/api/v1/permission_service.proto;l=407
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("clearExcludedSubjectIds")
public fun com.google.protobuf.kotlin.DslList.clear() {
_builder.clearExcludedSubjectIds()
}
/**
*
* permissionship indicates whether the response was partially evaluated or not
* deprecated: use `subject.permissionship`
*
*
* .authzed.api.v1.LookupPermissionship permissionship = 4 [deprecated = true, (.validate.rules) = { ... }
*/
@kotlin.Deprecated(message = "Field permissionship is deprecated") public var permissionship: com.authzed.api.v1.PermissionService.LookupPermissionship
@JvmName("getPermissionship")
get() = _builder.getPermissionship()
@JvmName("setPermissionship")
set(value) {
_builder.setPermissionship(value)
}
/**
*
* permissionship indicates whether the response was partially evaluated or not
* deprecated: use `subject.permissionship`
*
*
* .authzed.api.v1.LookupPermissionship permissionship = 4 [deprecated = true, (.validate.rules) = { ... }
* @deprecated authzed.api.v1.LookupSubjectsResponse.permissionship is deprecated.
* See authzed/api/v1/permission_service.proto;l=411
*/
public fun clearPermissionship() {
_builder.clearPermissionship()
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
* deprecated: use `subject.partial_caveat_info`
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 5 [deprecated = true, (.validate.rules) = { ... }
*/
@kotlin.Deprecated(message = "Field partialCaveatInfo is deprecated") public var partialCaveatInfo: com.authzed.api.v1.Core.PartialCaveatInfo
@JvmName("getPartialCaveatInfo")
get() = _builder.getPartialCaveatInfo()
@JvmName("setPartialCaveatInfo")
set(value) {
_builder.setPartialCaveatInfo(value)
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
* deprecated: use `subject.partial_caveat_info`
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 5 [deprecated = true, (.validate.rules) = { ... }
* @deprecated authzed.api.v1.LookupSubjectsResponse.partial_caveat_info is deprecated.
* See authzed/api/v1/permission_service.proto;l=415
*/
public fun clearPartialCaveatInfo() {
_builder.clearPartialCaveatInfo()
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
* deprecated: use `subject.partial_caveat_info`
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 5 [deprecated = true, (.validate.rules) = { ... }
* @deprecated authzed.api.v1.LookupSubjectsResponse.partial_caveat_info is deprecated.
* See authzed/api/v1/permission_service.proto;l=415
* @return Whether the partialCaveatInfo field is set.
*/
public fun hasPartialCaveatInfo(): kotlin.Boolean {
return _builder.hasPartialCaveatInfo()
}
/**
*
* subject is the subject found, along with its permissionship.
*
*
* .authzed.api.v1.ResolvedSubject subject = 6;
*/
public var subject: com.authzed.api.v1.PermissionService.ResolvedSubject
@JvmName("getSubject")
get() = _builder.getSubject()
@JvmName("setSubject")
set(value) {
_builder.setSubject(value)
}
/**
*
* subject is the subject found, along with its permissionship.
*
*
* .authzed.api.v1.ResolvedSubject subject = 6;
*/
public fun clearSubject() {
_builder.clearSubject()
}
/**
*
* subject is the subject found, along with its permissionship.
*
*
* .authzed.api.v1.ResolvedSubject subject = 6;
* @return Whether the subject field is set.
*/
public fun hasSubject(): kotlin.Boolean {
return _builder.hasSubject()
}
/**
* An uninstantiable, behaviorless type to represent the field in
* generics.
*/
@kotlin.OptIn(com.google.protobuf.kotlin.OnlyForUseByGeneratedProtoCode::class)
public class ExcludedSubjectsProxy private constructor() : com.google.protobuf.kotlin.DslProxy()
/**
*
* excluded_subjects are the subjects excluded. This list
* will only contain subjects if `subject.subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
*
*
* repeated .authzed.api.v1.ResolvedSubject excluded_subjects = 7;
*/
public val excludedSubjects: com.google.protobuf.kotlin.DslList
@kotlin.jvm.JvmSynthetic
get() = com.google.protobuf.kotlin.DslList(
_builder.getExcludedSubjectsList()
)
/**
*
* excluded_subjects are the subjects excluded. This list
* will only contain subjects if `subject.subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
*
*
* repeated .authzed.api.v1.ResolvedSubject excluded_subjects = 7;
* @param value The excludedSubjects to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("addExcludedSubjects")
public fun com.google.protobuf.kotlin.DslList.add(value: com.authzed.api.v1.PermissionService.ResolvedSubject) {
_builder.addExcludedSubjects(value)
}
/**
*
* excluded_subjects are the subjects excluded. This list
* will only contain subjects if `subject.subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
*
*
* repeated .authzed.api.v1.ResolvedSubject excluded_subjects = 7;
* @param value The excludedSubjects to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("plusAssignExcludedSubjects")
@Suppress("NOTHING_TO_INLINE")
public inline operator fun com.google.protobuf.kotlin.DslList.plusAssign(value: com.authzed.api.v1.PermissionService.ResolvedSubject) {
add(value)
}
/**
*
* excluded_subjects are the subjects excluded. This list
* will only contain subjects if `subject.subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
*
*
* repeated .authzed.api.v1.ResolvedSubject excluded_subjects = 7;
* @param values The excludedSubjects to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("addAllExcludedSubjects")
public fun com.google.protobuf.kotlin.DslList.addAll(values: kotlin.collections.Iterable) {
_builder.addAllExcludedSubjects(values)
}
/**
*
* excluded_subjects are the subjects excluded. This list
* will only contain subjects if `subject.subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
*
*
* repeated .authzed.api.v1.ResolvedSubject excluded_subjects = 7;
* @param values The excludedSubjects to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("plusAssignAllExcludedSubjects")
@Suppress("NOTHING_TO_INLINE")
public inline operator fun com.google.protobuf.kotlin.DslList.plusAssign(values: kotlin.collections.Iterable) {
addAll(values)
}
/**
*
* excluded_subjects are the subjects excluded. This list
* will only contain subjects if `subject.subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
*
*
* repeated .authzed.api.v1.ResolvedSubject excluded_subjects = 7;
* @param index The index to set the value at.
* @param value The excludedSubjects to set.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("setExcludedSubjects")
public operator fun com.google.protobuf.kotlin.DslList.set(index: kotlin.Int, value: com.authzed.api.v1.PermissionService.ResolvedSubject) {
_builder.setExcludedSubjects(index, value)
}
/**
*
* excluded_subjects are the subjects excluded. This list
* will only contain subjects if `subject.subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
*
*
* repeated .authzed.api.v1.ResolvedSubject excluded_subjects = 7;
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("clearExcludedSubjects")
public fun com.google.protobuf.kotlin.DslList.clear() {
_builder.clearExcludedSubjects()
}
}
}
@kotlin.jvm.JvmSynthetic
public inline fun com.authzed.api.v1.PermissionService.LookupSubjectsResponse.copy(block: com.authzed.api.v1.LookupSubjectsResponseKt.Dsl.() -> kotlin.Unit): com.authzed.api.v1.PermissionService.LookupSubjectsResponse =
com.authzed.api.v1.LookupSubjectsResponseKt.Dsl._create(this.toBuilder()).apply { block() }._build()
public val com.authzed.api.v1.PermissionService.LookupSubjectsResponseOrBuilder.lookedUpAtOrNull: com.authzed.api.v1.Core.ZedToken?
get() = if (hasLookedUpAt()) getLookedUpAt() else null
public val com.authzed.api.v1.PermissionService.LookupSubjectsResponseOrBuilder.partialCaveatInfoOrNull: com.authzed.api.v1.Core.PartialCaveatInfo?
get() = if (hasPartialCaveatInfo()) getPartialCaveatInfo() else null
public val com.authzed.api.v1.PermissionService.LookupSubjectsResponseOrBuilder.subjectOrNull: com.authzed.api.v1.PermissionService.ResolvedSubject?
get() = if (hasSubject()) getSubject() else null
© 2015 - 2025 Weber Informatics LLC | Privacy Policy