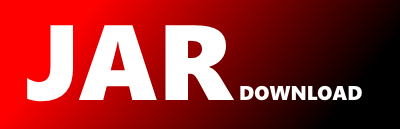
com.authzed.api.v1.PermissionService Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: authzed/api/v1/permission_service.proto
package com.authzed.api.v1;
public final class PermissionService {
private PermissionService() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
/**
*
* LookupPermissionship represents whether a Lookup response was partially evaluated or not
*
*
* Protobuf enum {@code authzed.api.v1.LookupPermissionship}
*/
public enum LookupPermissionship
implements com.google.protobuf.ProtocolMessageEnum {
/**
* LOOKUP_PERMISSIONSHIP_UNSPECIFIED = 0;
*/
LOOKUP_PERMISSIONSHIP_UNSPECIFIED(0),
/**
* LOOKUP_PERMISSIONSHIP_HAS_PERMISSION = 1;
*/
LOOKUP_PERMISSIONSHIP_HAS_PERMISSION(1),
/**
* LOOKUP_PERMISSIONSHIP_CONDITIONAL_PERMISSION = 2;
*/
LOOKUP_PERMISSIONSHIP_CONDITIONAL_PERMISSION(2),
UNRECOGNIZED(-1),
;
/**
* LOOKUP_PERMISSIONSHIP_UNSPECIFIED = 0;
*/
public static final int LOOKUP_PERMISSIONSHIP_UNSPECIFIED_VALUE = 0;
/**
* LOOKUP_PERMISSIONSHIP_HAS_PERMISSION = 1;
*/
public static final int LOOKUP_PERMISSIONSHIP_HAS_PERMISSION_VALUE = 1;
/**
* LOOKUP_PERMISSIONSHIP_CONDITIONAL_PERMISSION = 2;
*/
public static final int LOOKUP_PERMISSIONSHIP_CONDITIONAL_PERMISSION_VALUE = 2;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static LookupPermissionship valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static LookupPermissionship forNumber(int value) {
switch (value) {
case 0: return LOOKUP_PERMISSIONSHIP_UNSPECIFIED;
case 1: return LOOKUP_PERMISSIONSHIP_HAS_PERMISSION;
case 2: return LOOKUP_PERMISSIONSHIP_CONDITIONAL_PERMISSION;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
LookupPermissionship> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public LookupPermissionship findValueByNumber(int number) {
return LookupPermissionship.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.authzed.api.v1.PermissionService.getDescriptor().getEnumTypes().get(0);
}
private static final LookupPermissionship[] VALUES = values();
public static LookupPermissionship valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private LookupPermissionship(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:authzed.api.v1.LookupPermissionship)
}
public interface ConsistencyOrBuilder extends
// @@protoc_insertion_point(interface_extends:authzed.api.v1.Consistency)
com.google.protobuf.MessageOrBuilder {
/**
*
* minimize_latency indicates that the latency for the call should be
* minimized by having the system select the fastest snapshot available.
*
*
* bool minimize_latency = 1 [(.validate.rules) = { ... }
* @return Whether the minimizeLatency field is set.
*/
boolean hasMinimizeLatency();
/**
*
* minimize_latency indicates that the latency for the call should be
* minimized by having the system select the fastest snapshot available.
*
*
* bool minimize_latency = 1 [(.validate.rules) = { ... }
* @return The minimizeLatency.
*/
boolean getMinimizeLatency();
/**
*
* at_least_as_fresh indicates that all data used in the API call must be
* *at least as fresh* as that found in the ZedToken; more recent data might
* be used if available or faster.
*
*
* .authzed.api.v1.ZedToken at_least_as_fresh = 2;
* @return Whether the atLeastAsFresh field is set.
*/
boolean hasAtLeastAsFresh();
/**
*
* at_least_as_fresh indicates that all data used in the API call must be
* *at least as fresh* as that found in the ZedToken; more recent data might
* be used if available or faster.
*
*
* .authzed.api.v1.ZedToken at_least_as_fresh = 2;
* @return The atLeastAsFresh.
*/
com.authzed.api.v1.Core.ZedToken getAtLeastAsFresh();
/**
*
* at_least_as_fresh indicates that all data used in the API call must be
* *at least as fresh* as that found in the ZedToken; more recent data might
* be used if available or faster.
*
*
* .authzed.api.v1.ZedToken at_least_as_fresh = 2;
*/
com.authzed.api.v1.Core.ZedTokenOrBuilder getAtLeastAsFreshOrBuilder();
/**
*
* at_exact_snapshot indicates that all data used in the API call must be
* *at the given* snapshot in time; if the snapshot is no longer available,
* an error will be returned to the caller.
*
*
* .authzed.api.v1.ZedToken at_exact_snapshot = 3;
* @return Whether the atExactSnapshot field is set.
*/
boolean hasAtExactSnapshot();
/**
*
* at_exact_snapshot indicates that all data used in the API call must be
* *at the given* snapshot in time; if the snapshot is no longer available,
* an error will be returned to the caller.
*
*
* .authzed.api.v1.ZedToken at_exact_snapshot = 3;
* @return The atExactSnapshot.
*/
com.authzed.api.v1.Core.ZedToken getAtExactSnapshot();
/**
*
* at_exact_snapshot indicates that all data used in the API call must be
* *at the given* snapshot in time; if the snapshot is no longer available,
* an error will be returned to the caller.
*
*
* .authzed.api.v1.ZedToken at_exact_snapshot = 3;
*/
com.authzed.api.v1.Core.ZedTokenOrBuilder getAtExactSnapshotOrBuilder();
/**
*
* fully_consistent indicates that all data used in the API call *must* be
* at the most recent snapshot found.
* NOTE: using this method can be *quite slow*, so unless there is a need to
* do so, it is recommended to use `at_least_as_fresh` with a stored
* ZedToken.
*
*
* bool fully_consistent = 4 [(.validate.rules) = { ... }
* @return Whether the fullyConsistent field is set.
*/
boolean hasFullyConsistent();
/**
*
* fully_consistent indicates that all data used in the API call *must* be
* at the most recent snapshot found.
* NOTE: using this method can be *quite slow*, so unless there is a need to
* do so, it is recommended to use `at_least_as_fresh` with a stored
* ZedToken.
*
*
* bool fully_consistent = 4 [(.validate.rules) = { ... }
* @return The fullyConsistent.
*/
boolean getFullyConsistent();
public com.authzed.api.v1.PermissionService.Consistency.RequirementCase getRequirementCase();
}
/**
*
* Consistency will define how a request is handled by the backend.
* By defining a consistency requirement, and a token at which those
* requirements should be applied, where applicable.
*
*
* Protobuf type {@code authzed.api.v1.Consistency}
*/
public static final class Consistency extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:authzed.api.v1.Consistency)
ConsistencyOrBuilder {
private static final long serialVersionUID = 0L;
// Use Consistency.newBuilder() to construct.
private Consistency(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Consistency() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Consistency();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_Consistency_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_Consistency_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.PermissionService.Consistency.class, com.authzed.api.v1.PermissionService.Consistency.Builder.class);
}
private int requirementCase_ = 0;
private java.lang.Object requirement_;
public enum RequirementCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
MINIMIZE_LATENCY(1),
AT_LEAST_AS_FRESH(2),
AT_EXACT_SNAPSHOT(3),
FULLY_CONSISTENT(4),
REQUIREMENT_NOT_SET(0);
private final int value;
private RequirementCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static RequirementCase valueOf(int value) {
return forNumber(value);
}
public static RequirementCase forNumber(int value) {
switch (value) {
case 1: return MINIMIZE_LATENCY;
case 2: return AT_LEAST_AS_FRESH;
case 3: return AT_EXACT_SNAPSHOT;
case 4: return FULLY_CONSISTENT;
case 0: return REQUIREMENT_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public RequirementCase
getRequirementCase() {
return RequirementCase.forNumber(
requirementCase_);
}
public static final int MINIMIZE_LATENCY_FIELD_NUMBER = 1;
/**
*
* minimize_latency indicates that the latency for the call should be
* minimized by having the system select the fastest snapshot available.
*
*
* bool minimize_latency = 1 [(.validate.rules) = { ... }
* @return Whether the minimizeLatency field is set.
*/
@java.lang.Override
public boolean hasMinimizeLatency() {
return requirementCase_ == 1;
}
/**
*
* minimize_latency indicates that the latency for the call should be
* minimized by having the system select the fastest snapshot available.
*
*
* bool minimize_latency = 1 [(.validate.rules) = { ... }
* @return The minimizeLatency.
*/
@java.lang.Override
public boolean getMinimizeLatency() {
if (requirementCase_ == 1) {
return (java.lang.Boolean) requirement_;
}
return false;
}
public static final int AT_LEAST_AS_FRESH_FIELD_NUMBER = 2;
/**
*
* at_least_as_fresh indicates that all data used in the API call must be
* *at least as fresh* as that found in the ZedToken; more recent data might
* be used if available or faster.
*
*
* .authzed.api.v1.ZedToken at_least_as_fresh = 2;
* @return Whether the atLeastAsFresh field is set.
*/
@java.lang.Override
public boolean hasAtLeastAsFresh() {
return requirementCase_ == 2;
}
/**
*
* at_least_as_fresh indicates that all data used in the API call must be
* *at least as fresh* as that found in the ZedToken; more recent data might
* be used if available or faster.
*
*
* .authzed.api.v1.ZedToken at_least_as_fresh = 2;
* @return The atLeastAsFresh.
*/
@java.lang.Override
public com.authzed.api.v1.Core.ZedToken getAtLeastAsFresh() {
if (requirementCase_ == 2) {
return (com.authzed.api.v1.Core.ZedToken) requirement_;
}
return com.authzed.api.v1.Core.ZedToken.getDefaultInstance();
}
/**
*
* at_least_as_fresh indicates that all data used in the API call must be
* *at least as fresh* as that found in the ZedToken; more recent data might
* be used if available or faster.
*
*
* .authzed.api.v1.ZedToken at_least_as_fresh = 2;
*/
@java.lang.Override
public com.authzed.api.v1.Core.ZedTokenOrBuilder getAtLeastAsFreshOrBuilder() {
if (requirementCase_ == 2) {
return (com.authzed.api.v1.Core.ZedToken) requirement_;
}
return com.authzed.api.v1.Core.ZedToken.getDefaultInstance();
}
public static final int AT_EXACT_SNAPSHOT_FIELD_NUMBER = 3;
/**
*
* at_exact_snapshot indicates that all data used in the API call must be
* *at the given* snapshot in time; if the snapshot is no longer available,
* an error will be returned to the caller.
*
*
* .authzed.api.v1.ZedToken at_exact_snapshot = 3;
* @return Whether the atExactSnapshot field is set.
*/
@java.lang.Override
public boolean hasAtExactSnapshot() {
return requirementCase_ == 3;
}
/**
*
* at_exact_snapshot indicates that all data used in the API call must be
* *at the given* snapshot in time; if the snapshot is no longer available,
* an error will be returned to the caller.
*
*
* .authzed.api.v1.ZedToken at_exact_snapshot = 3;
* @return The atExactSnapshot.
*/
@java.lang.Override
public com.authzed.api.v1.Core.ZedToken getAtExactSnapshot() {
if (requirementCase_ == 3) {
return (com.authzed.api.v1.Core.ZedToken) requirement_;
}
return com.authzed.api.v1.Core.ZedToken.getDefaultInstance();
}
/**
*
* at_exact_snapshot indicates that all data used in the API call must be
* *at the given* snapshot in time; if the snapshot is no longer available,
* an error will be returned to the caller.
*
*
* .authzed.api.v1.ZedToken at_exact_snapshot = 3;
*/
@java.lang.Override
public com.authzed.api.v1.Core.ZedTokenOrBuilder getAtExactSnapshotOrBuilder() {
if (requirementCase_ == 3) {
return (com.authzed.api.v1.Core.ZedToken) requirement_;
}
return com.authzed.api.v1.Core.ZedToken.getDefaultInstance();
}
public static final int FULLY_CONSISTENT_FIELD_NUMBER = 4;
/**
*
* fully_consistent indicates that all data used in the API call *must* be
* at the most recent snapshot found.
* NOTE: using this method can be *quite slow*, so unless there is a need to
* do so, it is recommended to use `at_least_as_fresh` with a stored
* ZedToken.
*
*
* bool fully_consistent = 4 [(.validate.rules) = { ... }
* @return Whether the fullyConsistent field is set.
*/
@java.lang.Override
public boolean hasFullyConsistent() {
return requirementCase_ == 4;
}
/**
*
* fully_consistent indicates that all data used in the API call *must* be
* at the most recent snapshot found.
* NOTE: using this method can be *quite slow*, so unless there is a need to
* do so, it is recommended to use `at_least_as_fresh` with a stored
* ZedToken.
*
*
* bool fully_consistent = 4 [(.validate.rules) = { ... }
* @return The fullyConsistent.
*/
@java.lang.Override
public boolean getFullyConsistent() {
if (requirementCase_ == 4) {
return (java.lang.Boolean) requirement_;
}
return false;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (requirementCase_ == 1) {
output.writeBool(
1, (boolean)((java.lang.Boolean) requirement_));
}
if (requirementCase_ == 2) {
output.writeMessage(2, (com.authzed.api.v1.Core.ZedToken) requirement_);
}
if (requirementCase_ == 3) {
output.writeMessage(3, (com.authzed.api.v1.Core.ZedToken) requirement_);
}
if (requirementCase_ == 4) {
output.writeBool(
4, (boolean)((java.lang.Boolean) requirement_));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (requirementCase_ == 1) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(
1, (boolean)((java.lang.Boolean) requirement_));
}
if (requirementCase_ == 2) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, (com.authzed.api.v1.Core.ZedToken) requirement_);
}
if (requirementCase_ == 3) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, (com.authzed.api.v1.Core.ZedToken) requirement_);
}
if (requirementCase_ == 4) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(
4, (boolean)((java.lang.Boolean) requirement_));
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.authzed.api.v1.PermissionService.Consistency)) {
return super.equals(obj);
}
com.authzed.api.v1.PermissionService.Consistency other = (com.authzed.api.v1.PermissionService.Consistency) obj;
if (!getRequirementCase().equals(other.getRequirementCase())) return false;
switch (requirementCase_) {
case 1:
if (getMinimizeLatency()
!= other.getMinimizeLatency()) return false;
break;
case 2:
if (!getAtLeastAsFresh()
.equals(other.getAtLeastAsFresh())) return false;
break;
case 3:
if (!getAtExactSnapshot()
.equals(other.getAtExactSnapshot())) return false;
break;
case 4:
if (getFullyConsistent()
!= other.getFullyConsistent()) return false;
break;
case 0:
default:
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
switch (requirementCase_) {
case 1:
hash = (37 * hash) + MINIMIZE_LATENCY_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getMinimizeLatency());
break;
case 2:
hash = (37 * hash) + AT_LEAST_AS_FRESH_FIELD_NUMBER;
hash = (53 * hash) + getAtLeastAsFresh().hashCode();
break;
case 3:
hash = (37 * hash) + AT_EXACT_SNAPSHOT_FIELD_NUMBER;
hash = (53 * hash) + getAtExactSnapshot().hashCode();
break;
case 4:
hash = (37 * hash) + FULLY_CONSISTENT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getFullyConsistent());
break;
case 0:
default:
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.authzed.api.v1.PermissionService.Consistency parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.Consistency parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.Consistency parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.Consistency parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.Consistency parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.Consistency parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.Consistency parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.Consistency parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.Consistency parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.Consistency parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.Consistency parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.Consistency parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.authzed.api.v1.PermissionService.Consistency prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Consistency will define how a request is handled by the backend.
* By defining a consistency requirement, and a token at which those
* requirements should be applied, where applicable.
*
*
* Protobuf type {@code authzed.api.v1.Consistency}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:authzed.api.v1.Consistency)
com.authzed.api.v1.PermissionService.ConsistencyOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_Consistency_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_Consistency_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.PermissionService.Consistency.class, com.authzed.api.v1.PermissionService.Consistency.Builder.class);
}
// Construct using com.authzed.api.v1.PermissionService.Consistency.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
if (atLeastAsFreshBuilder_ != null) {
atLeastAsFreshBuilder_.clear();
}
if (atExactSnapshotBuilder_ != null) {
atExactSnapshotBuilder_.clear();
}
requirementCase_ = 0;
requirement_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_Consistency_descriptor;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.Consistency getDefaultInstanceForType() {
return com.authzed.api.v1.PermissionService.Consistency.getDefaultInstance();
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.Consistency build() {
com.authzed.api.v1.PermissionService.Consistency result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.Consistency buildPartial() {
com.authzed.api.v1.PermissionService.Consistency result = new com.authzed.api.v1.PermissionService.Consistency(this);
if (bitField0_ != 0) { buildPartial0(result); }
buildPartialOneofs(result);
onBuilt();
return result;
}
private void buildPartial0(com.authzed.api.v1.PermissionService.Consistency result) {
int from_bitField0_ = bitField0_;
}
private void buildPartialOneofs(com.authzed.api.v1.PermissionService.Consistency result) {
result.requirementCase_ = requirementCase_;
result.requirement_ = this.requirement_;
if (requirementCase_ == 2 &&
atLeastAsFreshBuilder_ != null) {
result.requirement_ = atLeastAsFreshBuilder_.build();
}
if (requirementCase_ == 3 &&
atExactSnapshotBuilder_ != null) {
result.requirement_ = atExactSnapshotBuilder_.build();
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.authzed.api.v1.PermissionService.Consistency) {
return mergeFrom((com.authzed.api.v1.PermissionService.Consistency)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.authzed.api.v1.PermissionService.Consistency other) {
if (other == com.authzed.api.v1.PermissionService.Consistency.getDefaultInstance()) return this;
switch (other.getRequirementCase()) {
case MINIMIZE_LATENCY: {
setMinimizeLatency(other.getMinimizeLatency());
break;
}
case AT_LEAST_AS_FRESH: {
mergeAtLeastAsFresh(other.getAtLeastAsFresh());
break;
}
case AT_EXACT_SNAPSHOT: {
mergeAtExactSnapshot(other.getAtExactSnapshot());
break;
}
case FULLY_CONSISTENT: {
setFullyConsistent(other.getFullyConsistent());
break;
}
case REQUIREMENT_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
requirement_ = input.readBool();
requirementCase_ = 1;
break;
} // case 8
case 18: {
input.readMessage(
getAtLeastAsFreshFieldBuilder().getBuilder(),
extensionRegistry);
requirementCase_ = 2;
break;
} // case 18
case 26: {
input.readMessage(
getAtExactSnapshotFieldBuilder().getBuilder(),
extensionRegistry);
requirementCase_ = 3;
break;
} // case 26
case 32: {
requirement_ = input.readBool();
requirementCase_ = 4;
break;
} // case 32
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int requirementCase_ = 0;
private java.lang.Object requirement_;
public RequirementCase
getRequirementCase() {
return RequirementCase.forNumber(
requirementCase_);
}
public Builder clearRequirement() {
requirementCase_ = 0;
requirement_ = null;
onChanged();
return this;
}
private int bitField0_;
/**
*
* minimize_latency indicates that the latency for the call should be
* minimized by having the system select the fastest snapshot available.
*
*
* bool minimize_latency = 1 [(.validate.rules) = { ... }
* @return Whether the minimizeLatency field is set.
*/
public boolean hasMinimizeLatency() {
return requirementCase_ == 1;
}
/**
*
* minimize_latency indicates that the latency for the call should be
* minimized by having the system select the fastest snapshot available.
*
*
* bool minimize_latency = 1 [(.validate.rules) = { ... }
* @return The minimizeLatency.
*/
public boolean getMinimizeLatency() {
if (requirementCase_ == 1) {
return (java.lang.Boolean) requirement_;
}
return false;
}
/**
*
* minimize_latency indicates that the latency for the call should be
* minimized by having the system select the fastest snapshot available.
*
*
* bool minimize_latency = 1 [(.validate.rules) = { ... }
* @param value The minimizeLatency to set.
* @return This builder for chaining.
*/
public Builder setMinimizeLatency(boolean value) {
requirementCase_ = 1;
requirement_ = value;
onChanged();
return this;
}
/**
*
* minimize_latency indicates that the latency for the call should be
* minimized by having the system select the fastest snapshot available.
*
*
* bool minimize_latency = 1 [(.validate.rules) = { ... }
* @return This builder for chaining.
*/
public Builder clearMinimizeLatency() {
if (requirementCase_ == 1) {
requirementCase_ = 0;
requirement_ = null;
onChanged();
}
return this;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.ZedToken, com.authzed.api.v1.Core.ZedToken.Builder, com.authzed.api.v1.Core.ZedTokenOrBuilder> atLeastAsFreshBuilder_;
/**
*
* at_least_as_fresh indicates that all data used in the API call must be
* *at least as fresh* as that found in the ZedToken; more recent data might
* be used if available or faster.
*
*
* .authzed.api.v1.ZedToken at_least_as_fresh = 2;
* @return Whether the atLeastAsFresh field is set.
*/
@java.lang.Override
public boolean hasAtLeastAsFresh() {
return requirementCase_ == 2;
}
/**
*
* at_least_as_fresh indicates that all data used in the API call must be
* *at least as fresh* as that found in the ZedToken; more recent data might
* be used if available or faster.
*
*
* .authzed.api.v1.ZedToken at_least_as_fresh = 2;
* @return The atLeastAsFresh.
*/
@java.lang.Override
public com.authzed.api.v1.Core.ZedToken getAtLeastAsFresh() {
if (atLeastAsFreshBuilder_ == null) {
if (requirementCase_ == 2) {
return (com.authzed.api.v1.Core.ZedToken) requirement_;
}
return com.authzed.api.v1.Core.ZedToken.getDefaultInstance();
} else {
if (requirementCase_ == 2) {
return atLeastAsFreshBuilder_.getMessage();
}
return com.authzed.api.v1.Core.ZedToken.getDefaultInstance();
}
}
/**
*
* at_least_as_fresh indicates that all data used in the API call must be
* *at least as fresh* as that found in the ZedToken; more recent data might
* be used if available or faster.
*
*
* .authzed.api.v1.ZedToken at_least_as_fresh = 2;
*/
public Builder setAtLeastAsFresh(com.authzed.api.v1.Core.ZedToken value) {
if (atLeastAsFreshBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
requirement_ = value;
onChanged();
} else {
atLeastAsFreshBuilder_.setMessage(value);
}
requirementCase_ = 2;
return this;
}
/**
*
* at_least_as_fresh indicates that all data used in the API call must be
* *at least as fresh* as that found in the ZedToken; more recent data might
* be used if available or faster.
*
*
* .authzed.api.v1.ZedToken at_least_as_fresh = 2;
*/
public Builder setAtLeastAsFresh(
com.authzed.api.v1.Core.ZedToken.Builder builderForValue) {
if (atLeastAsFreshBuilder_ == null) {
requirement_ = builderForValue.build();
onChanged();
} else {
atLeastAsFreshBuilder_.setMessage(builderForValue.build());
}
requirementCase_ = 2;
return this;
}
/**
*
* at_least_as_fresh indicates that all data used in the API call must be
* *at least as fresh* as that found in the ZedToken; more recent data might
* be used if available or faster.
*
*
* .authzed.api.v1.ZedToken at_least_as_fresh = 2;
*/
public Builder mergeAtLeastAsFresh(com.authzed.api.v1.Core.ZedToken value) {
if (atLeastAsFreshBuilder_ == null) {
if (requirementCase_ == 2 &&
requirement_ != com.authzed.api.v1.Core.ZedToken.getDefaultInstance()) {
requirement_ = com.authzed.api.v1.Core.ZedToken.newBuilder((com.authzed.api.v1.Core.ZedToken) requirement_)
.mergeFrom(value).buildPartial();
} else {
requirement_ = value;
}
onChanged();
} else {
if (requirementCase_ == 2) {
atLeastAsFreshBuilder_.mergeFrom(value);
} else {
atLeastAsFreshBuilder_.setMessage(value);
}
}
requirementCase_ = 2;
return this;
}
/**
*
* at_least_as_fresh indicates that all data used in the API call must be
* *at least as fresh* as that found in the ZedToken; more recent data might
* be used if available or faster.
*
*
* .authzed.api.v1.ZedToken at_least_as_fresh = 2;
*/
public Builder clearAtLeastAsFresh() {
if (atLeastAsFreshBuilder_ == null) {
if (requirementCase_ == 2) {
requirementCase_ = 0;
requirement_ = null;
onChanged();
}
} else {
if (requirementCase_ == 2) {
requirementCase_ = 0;
requirement_ = null;
}
atLeastAsFreshBuilder_.clear();
}
return this;
}
/**
*
* at_least_as_fresh indicates that all data used in the API call must be
* *at least as fresh* as that found in the ZedToken; more recent data might
* be used if available or faster.
*
*
* .authzed.api.v1.ZedToken at_least_as_fresh = 2;
*/
public com.authzed.api.v1.Core.ZedToken.Builder getAtLeastAsFreshBuilder() {
return getAtLeastAsFreshFieldBuilder().getBuilder();
}
/**
*
* at_least_as_fresh indicates that all data used in the API call must be
* *at least as fresh* as that found in the ZedToken; more recent data might
* be used if available or faster.
*
*
* .authzed.api.v1.ZedToken at_least_as_fresh = 2;
*/
@java.lang.Override
public com.authzed.api.v1.Core.ZedTokenOrBuilder getAtLeastAsFreshOrBuilder() {
if ((requirementCase_ == 2) && (atLeastAsFreshBuilder_ != null)) {
return atLeastAsFreshBuilder_.getMessageOrBuilder();
} else {
if (requirementCase_ == 2) {
return (com.authzed.api.v1.Core.ZedToken) requirement_;
}
return com.authzed.api.v1.Core.ZedToken.getDefaultInstance();
}
}
/**
*
* at_least_as_fresh indicates that all data used in the API call must be
* *at least as fresh* as that found in the ZedToken; more recent data might
* be used if available or faster.
*
*
* .authzed.api.v1.ZedToken at_least_as_fresh = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.ZedToken, com.authzed.api.v1.Core.ZedToken.Builder, com.authzed.api.v1.Core.ZedTokenOrBuilder>
getAtLeastAsFreshFieldBuilder() {
if (atLeastAsFreshBuilder_ == null) {
if (!(requirementCase_ == 2)) {
requirement_ = com.authzed.api.v1.Core.ZedToken.getDefaultInstance();
}
atLeastAsFreshBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.ZedToken, com.authzed.api.v1.Core.ZedToken.Builder, com.authzed.api.v1.Core.ZedTokenOrBuilder>(
(com.authzed.api.v1.Core.ZedToken) requirement_,
getParentForChildren(),
isClean());
requirement_ = null;
}
requirementCase_ = 2;
onChanged();
return atLeastAsFreshBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.ZedToken, com.authzed.api.v1.Core.ZedToken.Builder, com.authzed.api.v1.Core.ZedTokenOrBuilder> atExactSnapshotBuilder_;
/**
*
* at_exact_snapshot indicates that all data used in the API call must be
* *at the given* snapshot in time; if the snapshot is no longer available,
* an error will be returned to the caller.
*
*
* .authzed.api.v1.ZedToken at_exact_snapshot = 3;
* @return Whether the atExactSnapshot field is set.
*/
@java.lang.Override
public boolean hasAtExactSnapshot() {
return requirementCase_ == 3;
}
/**
*
* at_exact_snapshot indicates that all data used in the API call must be
* *at the given* snapshot in time; if the snapshot is no longer available,
* an error will be returned to the caller.
*
*
* .authzed.api.v1.ZedToken at_exact_snapshot = 3;
* @return The atExactSnapshot.
*/
@java.lang.Override
public com.authzed.api.v1.Core.ZedToken getAtExactSnapshot() {
if (atExactSnapshotBuilder_ == null) {
if (requirementCase_ == 3) {
return (com.authzed.api.v1.Core.ZedToken) requirement_;
}
return com.authzed.api.v1.Core.ZedToken.getDefaultInstance();
} else {
if (requirementCase_ == 3) {
return atExactSnapshotBuilder_.getMessage();
}
return com.authzed.api.v1.Core.ZedToken.getDefaultInstance();
}
}
/**
*
* at_exact_snapshot indicates that all data used in the API call must be
* *at the given* snapshot in time; if the snapshot is no longer available,
* an error will be returned to the caller.
*
*
* .authzed.api.v1.ZedToken at_exact_snapshot = 3;
*/
public Builder setAtExactSnapshot(com.authzed.api.v1.Core.ZedToken value) {
if (atExactSnapshotBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
requirement_ = value;
onChanged();
} else {
atExactSnapshotBuilder_.setMessage(value);
}
requirementCase_ = 3;
return this;
}
/**
*
* at_exact_snapshot indicates that all data used in the API call must be
* *at the given* snapshot in time; if the snapshot is no longer available,
* an error will be returned to the caller.
*
*
* .authzed.api.v1.ZedToken at_exact_snapshot = 3;
*/
public Builder setAtExactSnapshot(
com.authzed.api.v1.Core.ZedToken.Builder builderForValue) {
if (atExactSnapshotBuilder_ == null) {
requirement_ = builderForValue.build();
onChanged();
} else {
atExactSnapshotBuilder_.setMessage(builderForValue.build());
}
requirementCase_ = 3;
return this;
}
/**
*
* at_exact_snapshot indicates that all data used in the API call must be
* *at the given* snapshot in time; if the snapshot is no longer available,
* an error will be returned to the caller.
*
*
* .authzed.api.v1.ZedToken at_exact_snapshot = 3;
*/
public Builder mergeAtExactSnapshot(com.authzed.api.v1.Core.ZedToken value) {
if (atExactSnapshotBuilder_ == null) {
if (requirementCase_ == 3 &&
requirement_ != com.authzed.api.v1.Core.ZedToken.getDefaultInstance()) {
requirement_ = com.authzed.api.v1.Core.ZedToken.newBuilder((com.authzed.api.v1.Core.ZedToken) requirement_)
.mergeFrom(value).buildPartial();
} else {
requirement_ = value;
}
onChanged();
} else {
if (requirementCase_ == 3) {
atExactSnapshotBuilder_.mergeFrom(value);
} else {
atExactSnapshotBuilder_.setMessage(value);
}
}
requirementCase_ = 3;
return this;
}
/**
*
* at_exact_snapshot indicates that all data used in the API call must be
* *at the given* snapshot in time; if the snapshot is no longer available,
* an error will be returned to the caller.
*
*
* .authzed.api.v1.ZedToken at_exact_snapshot = 3;
*/
public Builder clearAtExactSnapshot() {
if (atExactSnapshotBuilder_ == null) {
if (requirementCase_ == 3) {
requirementCase_ = 0;
requirement_ = null;
onChanged();
}
} else {
if (requirementCase_ == 3) {
requirementCase_ = 0;
requirement_ = null;
}
atExactSnapshotBuilder_.clear();
}
return this;
}
/**
*
* at_exact_snapshot indicates that all data used in the API call must be
* *at the given* snapshot in time; if the snapshot is no longer available,
* an error will be returned to the caller.
*
*
* .authzed.api.v1.ZedToken at_exact_snapshot = 3;
*/
public com.authzed.api.v1.Core.ZedToken.Builder getAtExactSnapshotBuilder() {
return getAtExactSnapshotFieldBuilder().getBuilder();
}
/**
*
* at_exact_snapshot indicates that all data used in the API call must be
* *at the given* snapshot in time; if the snapshot is no longer available,
* an error will be returned to the caller.
*
*
* .authzed.api.v1.ZedToken at_exact_snapshot = 3;
*/
@java.lang.Override
public com.authzed.api.v1.Core.ZedTokenOrBuilder getAtExactSnapshotOrBuilder() {
if ((requirementCase_ == 3) && (atExactSnapshotBuilder_ != null)) {
return atExactSnapshotBuilder_.getMessageOrBuilder();
} else {
if (requirementCase_ == 3) {
return (com.authzed.api.v1.Core.ZedToken) requirement_;
}
return com.authzed.api.v1.Core.ZedToken.getDefaultInstance();
}
}
/**
*
* at_exact_snapshot indicates that all data used in the API call must be
* *at the given* snapshot in time; if the snapshot is no longer available,
* an error will be returned to the caller.
*
*
* .authzed.api.v1.ZedToken at_exact_snapshot = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.ZedToken, com.authzed.api.v1.Core.ZedToken.Builder, com.authzed.api.v1.Core.ZedTokenOrBuilder>
getAtExactSnapshotFieldBuilder() {
if (atExactSnapshotBuilder_ == null) {
if (!(requirementCase_ == 3)) {
requirement_ = com.authzed.api.v1.Core.ZedToken.getDefaultInstance();
}
atExactSnapshotBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.ZedToken, com.authzed.api.v1.Core.ZedToken.Builder, com.authzed.api.v1.Core.ZedTokenOrBuilder>(
(com.authzed.api.v1.Core.ZedToken) requirement_,
getParentForChildren(),
isClean());
requirement_ = null;
}
requirementCase_ = 3;
onChanged();
return atExactSnapshotBuilder_;
}
/**
*
* fully_consistent indicates that all data used in the API call *must* be
* at the most recent snapshot found.
* NOTE: using this method can be *quite slow*, so unless there is a need to
* do so, it is recommended to use `at_least_as_fresh` with a stored
* ZedToken.
*
*
* bool fully_consistent = 4 [(.validate.rules) = { ... }
* @return Whether the fullyConsistent field is set.
*/
public boolean hasFullyConsistent() {
return requirementCase_ == 4;
}
/**
*
* fully_consistent indicates that all data used in the API call *must* be
* at the most recent snapshot found.
* NOTE: using this method can be *quite slow*, so unless there is a need to
* do so, it is recommended to use `at_least_as_fresh` with a stored
* ZedToken.
*
*
* bool fully_consistent = 4 [(.validate.rules) = { ... }
* @return The fullyConsistent.
*/
public boolean getFullyConsistent() {
if (requirementCase_ == 4) {
return (java.lang.Boolean) requirement_;
}
return false;
}
/**
*
* fully_consistent indicates that all data used in the API call *must* be
* at the most recent snapshot found.
* NOTE: using this method can be *quite slow*, so unless there is a need to
* do so, it is recommended to use `at_least_as_fresh` with a stored
* ZedToken.
*
*
* bool fully_consistent = 4 [(.validate.rules) = { ... }
* @param value The fullyConsistent to set.
* @return This builder for chaining.
*/
public Builder setFullyConsistent(boolean value) {
requirementCase_ = 4;
requirement_ = value;
onChanged();
return this;
}
/**
*
* fully_consistent indicates that all data used in the API call *must* be
* at the most recent snapshot found.
* NOTE: using this method can be *quite slow*, so unless there is a need to
* do so, it is recommended to use `at_least_as_fresh` with a stored
* ZedToken.
*
*
* bool fully_consistent = 4 [(.validate.rules) = { ... }
* @return This builder for chaining.
*/
public Builder clearFullyConsistent() {
if (requirementCase_ == 4) {
requirementCase_ = 0;
requirement_ = null;
onChanged();
}
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:authzed.api.v1.Consistency)
}
// @@protoc_insertion_point(class_scope:authzed.api.v1.Consistency)
private static final com.authzed.api.v1.PermissionService.Consistency DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.authzed.api.v1.PermissionService.Consistency();
}
public static com.authzed.api.v1.PermissionService.Consistency getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Consistency parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.Consistency getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RelationshipFilterOrBuilder extends
// @@protoc_insertion_point(interface_extends:authzed.api.v1.RelationshipFilter)
com.google.protobuf.MessageOrBuilder {
/**
* string resource_type = 1 [(.validate.rules) = { ... }
* @return The resourceType.
*/
java.lang.String getResourceType();
/**
* string resource_type = 1 [(.validate.rules) = { ... }
* @return The bytes for resourceType.
*/
com.google.protobuf.ByteString
getResourceTypeBytes();
/**
* string optional_resource_id = 2 [(.validate.rules) = { ... }
* @return The optionalResourceId.
*/
java.lang.String getOptionalResourceId();
/**
* string optional_resource_id = 2 [(.validate.rules) = { ... }
* @return The bytes for optionalResourceId.
*/
com.google.protobuf.ByteString
getOptionalResourceIdBytes();
/**
* string optional_relation = 3 [(.validate.rules) = { ... }
* @return The optionalRelation.
*/
java.lang.String getOptionalRelation();
/**
* string optional_relation = 3 [(.validate.rules) = { ... }
* @return The bytes for optionalRelation.
*/
com.google.protobuf.ByteString
getOptionalRelationBytes();
/**
* .authzed.api.v1.SubjectFilter optional_subject_filter = 4;
* @return Whether the optionalSubjectFilter field is set.
*/
boolean hasOptionalSubjectFilter();
/**
* .authzed.api.v1.SubjectFilter optional_subject_filter = 4;
* @return The optionalSubjectFilter.
*/
com.authzed.api.v1.PermissionService.SubjectFilter getOptionalSubjectFilter();
/**
* .authzed.api.v1.SubjectFilter optional_subject_filter = 4;
*/
com.authzed.api.v1.PermissionService.SubjectFilterOrBuilder getOptionalSubjectFilterOrBuilder();
}
/**
*
* RelationshipFilter is a collection of filters which when applied to a
* relationship will return relationships that have exactly matching fields.
* resource_type is required. All other fields are optional and if left
* unspecified will not filter relationships.
*
*
* Protobuf type {@code authzed.api.v1.RelationshipFilter}
*/
public static final class RelationshipFilter extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:authzed.api.v1.RelationshipFilter)
RelationshipFilterOrBuilder {
private static final long serialVersionUID = 0L;
// Use RelationshipFilter.newBuilder() to construct.
private RelationshipFilter(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RelationshipFilter() {
resourceType_ = "";
optionalResourceId_ = "";
optionalRelation_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new RelationshipFilter();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_RelationshipFilter_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_RelationshipFilter_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.PermissionService.RelationshipFilter.class, com.authzed.api.v1.PermissionService.RelationshipFilter.Builder.class);
}
public static final int RESOURCE_TYPE_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object resourceType_ = "";
/**
* string resource_type = 1 [(.validate.rules) = { ... }
* @return The resourceType.
*/
@java.lang.Override
public java.lang.String getResourceType() {
java.lang.Object ref = resourceType_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
resourceType_ = s;
return s;
}
}
/**
* string resource_type = 1 [(.validate.rules) = { ... }
* @return The bytes for resourceType.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getResourceTypeBytes() {
java.lang.Object ref = resourceType_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
resourceType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int OPTIONAL_RESOURCE_ID_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object optionalResourceId_ = "";
/**
* string optional_resource_id = 2 [(.validate.rules) = { ... }
* @return The optionalResourceId.
*/
@java.lang.Override
public java.lang.String getOptionalResourceId() {
java.lang.Object ref = optionalResourceId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
optionalResourceId_ = s;
return s;
}
}
/**
* string optional_resource_id = 2 [(.validate.rules) = { ... }
* @return The bytes for optionalResourceId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getOptionalResourceIdBytes() {
java.lang.Object ref = optionalResourceId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
optionalResourceId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int OPTIONAL_RELATION_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private volatile java.lang.Object optionalRelation_ = "";
/**
* string optional_relation = 3 [(.validate.rules) = { ... }
* @return The optionalRelation.
*/
@java.lang.Override
public java.lang.String getOptionalRelation() {
java.lang.Object ref = optionalRelation_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
optionalRelation_ = s;
return s;
}
}
/**
* string optional_relation = 3 [(.validate.rules) = { ... }
* @return The bytes for optionalRelation.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getOptionalRelationBytes() {
java.lang.Object ref = optionalRelation_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
optionalRelation_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int OPTIONAL_SUBJECT_FILTER_FIELD_NUMBER = 4;
private com.authzed.api.v1.PermissionService.SubjectFilter optionalSubjectFilter_;
/**
* .authzed.api.v1.SubjectFilter optional_subject_filter = 4;
* @return Whether the optionalSubjectFilter field is set.
*/
@java.lang.Override
public boolean hasOptionalSubjectFilter() {
return optionalSubjectFilter_ != null;
}
/**
* .authzed.api.v1.SubjectFilter optional_subject_filter = 4;
* @return The optionalSubjectFilter.
*/
@java.lang.Override
public com.authzed.api.v1.PermissionService.SubjectFilter getOptionalSubjectFilter() {
return optionalSubjectFilter_ == null ? com.authzed.api.v1.PermissionService.SubjectFilter.getDefaultInstance() : optionalSubjectFilter_;
}
/**
* .authzed.api.v1.SubjectFilter optional_subject_filter = 4;
*/
@java.lang.Override
public com.authzed.api.v1.PermissionService.SubjectFilterOrBuilder getOptionalSubjectFilterOrBuilder() {
return optionalSubjectFilter_ == null ? com.authzed.api.v1.PermissionService.SubjectFilter.getDefaultInstance() : optionalSubjectFilter_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(resourceType_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, resourceType_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(optionalResourceId_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, optionalResourceId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(optionalRelation_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, optionalRelation_);
}
if (optionalSubjectFilter_ != null) {
output.writeMessage(4, getOptionalSubjectFilter());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(resourceType_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, resourceType_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(optionalResourceId_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, optionalResourceId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(optionalRelation_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, optionalRelation_);
}
if (optionalSubjectFilter_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getOptionalSubjectFilter());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.authzed.api.v1.PermissionService.RelationshipFilter)) {
return super.equals(obj);
}
com.authzed.api.v1.PermissionService.RelationshipFilter other = (com.authzed.api.v1.PermissionService.RelationshipFilter) obj;
if (!getResourceType()
.equals(other.getResourceType())) return false;
if (!getOptionalResourceId()
.equals(other.getOptionalResourceId())) return false;
if (!getOptionalRelation()
.equals(other.getOptionalRelation())) return false;
if (hasOptionalSubjectFilter() != other.hasOptionalSubjectFilter()) return false;
if (hasOptionalSubjectFilter()) {
if (!getOptionalSubjectFilter()
.equals(other.getOptionalSubjectFilter())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + RESOURCE_TYPE_FIELD_NUMBER;
hash = (53 * hash) + getResourceType().hashCode();
hash = (37 * hash) + OPTIONAL_RESOURCE_ID_FIELD_NUMBER;
hash = (53 * hash) + getOptionalResourceId().hashCode();
hash = (37 * hash) + OPTIONAL_RELATION_FIELD_NUMBER;
hash = (53 * hash) + getOptionalRelation().hashCode();
if (hasOptionalSubjectFilter()) {
hash = (37 * hash) + OPTIONAL_SUBJECT_FILTER_FIELD_NUMBER;
hash = (53 * hash) + getOptionalSubjectFilter().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.authzed.api.v1.PermissionService.RelationshipFilter parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.RelationshipFilter parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.RelationshipFilter parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.RelationshipFilter parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.RelationshipFilter parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.RelationshipFilter parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.RelationshipFilter parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.RelationshipFilter parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.RelationshipFilter parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.RelationshipFilter parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.RelationshipFilter parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.RelationshipFilter parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.authzed.api.v1.PermissionService.RelationshipFilter prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* RelationshipFilter is a collection of filters which when applied to a
* relationship will return relationships that have exactly matching fields.
* resource_type is required. All other fields are optional and if left
* unspecified will not filter relationships.
*
*
* Protobuf type {@code authzed.api.v1.RelationshipFilter}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:authzed.api.v1.RelationshipFilter)
com.authzed.api.v1.PermissionService.RelationshipFilterOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_RelationshipFilter_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_RelationshipFilter_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.PermissionService.RelationshipFilter.class, com.authzed.api.v1.PermissionService.RelationshipFilter.Builder.class);
}
// Construct using com.authzed.api.v1.PermissionService.RelationshipFilter.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
resourceType_ = "";
optionalResourceId_ = "";
optionalRelation_ = "";
optionalSubjectFilter_ = null;
if (optionalSubjectFilterBuilder_ != null) {
optionalSubjectFilterBuilder_.dispose();
optionalSubjectFilterBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_RelationshipFilter_descriptor;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.RelationshipFilter getDefaultInstanceForType() {
return com.authzed.api.v1.PermissionService.RelationshipFilter.getDefaultInstance();
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.RelationshipFilter build() {
com.authzed.api.v1.PermissionService.RelationshipFilter result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.RelationshipFilter buildPartial() {
com.authzed.api.v1.PermissionService.RelationshipFilter result = new com.authzed.api.v1.PermissionService.RelationshipFilter(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.authzed.api.v1.PermissionService.RelationshipFilter result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.resourceType_ = resourceType_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.optionalResourceId_ = optionalResourceId_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.optionalRelation_ = optionalRelation_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.optionalSubjectFilter_ = optionalSubjectFilterBuilder_ == null
? optionalSubjectFilter_
: optionalSubjectFilterBuilder_.build();
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.authzed.api.v1.PermissionService.RelationshipFilter) {
return mergeFrom((com.authzed.api.v1.PermissionService.RelationshipFilter)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.authzed.api.v1.PermissionService.RelationshipFilter other) {
if (other == com.authzed.api.v1.PermissionService.RelationshipFilter.getDefaultInstance()) return this;
if (!other.getResourceType().isEmpty()) {
resourceType_ = other.resourceType_;
bitField0_ |= 0x00000001;
onChanged();
}
if (!other.getOptionalResourceId().isEmpty()) {
optionalResourceId_ = other.optionalResourceId_;
bitField0_ |= 0x00000002;
onChanged();
}
if (!other.getOptionalRelation().isEmpty()) {
optionalRelation_ = other.optionalRelation_;
bitField0_ |= 0x00000004;
onChanged();
}
if (other.hasOptionalSubjectFilter()) {
mergeOptionalSubjectFilter(other.getOptionalSubjectFilter());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
resourceType_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
optionalResourceId_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 18
case 26: {
optionalRelation_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000004;
break;
} // case 26
case 34: {
input.readMessage(
getOptionalSubjectFilterFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000008;
break;
} // case 34
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object resourceType_ = "";
/**
* string resource_type = 1 [(.validate.rules) = { ... }
* @return The resourceType.
*/
public java.lang.String getResourceType() {
java.lang.Object ref = resourceType_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
resourceType_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string resource_type = 1 [(.validate.rules) = { ... }
* @return The bytes for resourceType.
*/
public com.google.protobuf.ByteString
getResourceTypeBytes() {
java.lang.Object ref = resourceType_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
resourceType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string resource_type = 1 [(.validate.rules) = { ... }
* @param value The resourceType to set.
* @return This builder for chaining.
*/
public Builder setResourceType(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
resourceType_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* string resource_type = 1 [(.validate.rules) = { ... }
* @return This builder for chaining.
*/
public Builder clearResourceType() {
resourceType_ = getDefaultInstance().getResourceType();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* string resource_type = 1 [(.validate.rules) = { ... }
* @param value The bytes for resourceType to set.
* @return This builder for chaining.
*/
public Builder setResourceTypeBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
resourceType_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private java.lang.Object optionalResourceId_ = "";
/**
* string optional_resource_id = 2 [(.validate.rules) = { ... }
* @return The optionalResourceId.
*/
public java.lang.String getOptionalResourceId() {
java.lang.Object ref = optionalResourceId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
optionalResourceId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string optional_resource_id = 2 [(.validate.rules) = { ... }
* @return The bytes for optionalResourceId.
*/
public com.google.protobuf.ByteString
getOptionalResourceIdBytes() {
java.lang.Object ref = optionalResourceId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
optionalResourceId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string optional_resource_id = 2 [(.validate.rules) = { ... }
* @param value The optionalResourceId to set.
* @return This builder for chaining.
*/
public Builder setOptionalResourceId(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
optionalResourceId_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* string optional_resource_id = 2 [(.validate.rules) = { ... }
* @return This builder for chaining.
*/
public Builder clearOptionalResourceId() {
optionalResourceId_ = getDefaultInstance().getOptionalResourceId();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
* string optional_resource_id = 2 [(.validate.rules) = { ... }
* @param value The bytes for optionalResourceId to set.
* @return This builder for chaining.
*/
public Builder setOptionalResourceIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
optionalResourceId_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private java.lang.Object optionalRelation_ = "";
/**
* string optional_relation = 3 [(.validate.rules) = { ... }
* @return The optionalRelation.
*/
public java.lang.String getOptionalRelation() {
java.lang.Object ref = optionalRelation_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
optionalRelation_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string optional_relation = 3 [(.validate.rules) = { ... }
* @return The bytes for optionalRelation.
*/
public com.google.protobuf.ByteString
getOptionalRelationBytes() {
java.lang.Object ref = optionalRelation_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
optionalRelation_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string optional_relation = 3 [(.validate.rules) = { ... }
* @param value The optionalRelation to set.
* @return This builder for chaining.
*/
public Builder setOptionalRelation(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
optionalRelation_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* string optional_relation = 3 [(.validate.rules) = { ... }
* @return This builder for chaining.
*/
public Builder clearOptionalRelation() {
optionalRelation_ = getDefaultInstance().getOptionalRelation();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
* string optional_relation = 3 [(.validate.rules) = { ... }
* @param value The bytes for optionalRelation to set.
* @return This builder for chaining.
*/
public Builder setOptionalRelationBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
optionalRelation_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
private com.authzed.api.v1.PermissionService.SubjectFilter optionalSubjectFilter_;
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.PermissionService.SubjectFilter, com.authzed.api.v1.PermissionService.SubjectFilter.Builder, com.authzed.api.v1.PermissionService.SubjectFilterOrBuilder> optionalSubjectFilterBuilder_;
/**
* .authzed.api.v1.SubjectFilter optional_subject_filter = 4;
* @return Whether the optionalSubjectFilter field is set.
*/
public boolean hasOptionalSubjectFilter() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* .authzed.api.v1.SubjectFilter optional_subject_filter = 4;
* @return The optionalSubjectFilter.
*/
public com.authzed.api.v1.PermissionService.SubjectFilter getOptionalSubjectFilter() {
if (optionalSubjectFilterBuilder_ == null) {
return optionalSubjectFilter_ == null ? com.authzed.api.v1.PermissionService.SubjectFilter.getDefaultInstance() : optionalSubjectFilter_;
} else {
return optionalSubjectFilterBuilder_.getMessage();
}
}
/**
* .authzed.api.v1.SubjectFilter optional_subject_filter = 4;
*/
public Builder setOptionalSubjectFilter(com.authzed.api.v1.PermissionService.SubjectFilter value) {
if (optionalSubjectFilterBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
optionalSubjectFilter_ = value;
} else {
optionalSubjectFilterBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* .authzed.api.v1.SubjectFilter optional_subject_filter = 4;
*/
public Builder setOptionalSubjectFilter(
com.authzed.api.v1.PermissionService.SubjectFilter.Builder builderForValue) {
if (optionalSubjectFilterBuilder_ == null) {
optionalSubjectFilter_ = builderForValue.build();
} else {
optionalSubjectFilterBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* .authzed.api.v1.SubjectFilter optional_subject_filter = 4;
*/
public Builder mergeOptionalSubjectFilter(com.authzed.api.v1.PermissionService.SubjectFilter value) {
if (optionalSubjectFilterBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0) &&
optionalSubjectFilter_ != null &&
optionalSubjectFilter_ != com.authzed.api.v1.PermissionService.SubjectFilter.getDefaultInstance()) {
getOptionalSubjectFilterBuilder().mergeFrom(value);
} else {
optionalSubjectFilter_ = value;
}
} else {
optionalSubjectFilterBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* .authzed.api.v1.SubjectFilter optional_subject_filter = 4;
*/
public Builder clearOptionalSubjectFilter() {
bitField0_ = (bitField0_ & ~0x00000008);
optionalSubjectFilter_ = null;
if (optionalSubjectFilterBuilder_ != null) {
optionalSubjectFilterBuilder_.dispose();
optionalSubjectFilterBuilder_ = null;
}
onChanged();
return this;
}
/**
* .authzed.api.v1.SubjectFilter optional_subject_filter = 4;
*/
public com.authzed.api.v1.PermissionService.SubjectFilter.Builder getOptionalSubjectFilterBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getOptionalSubjectFilterFieldBuilder().getBuilder();
}
/**
* .authzed.api.v1.SubjectFilter optional_subject_filter = 4;
*/
public com.authzed.api.v1.PermissionService.SubjectFilterOrBuilder getOptionalSubjectFilterOrBuilder() {
if (optionalSubjectFilterBuilder_ != null) {
return optionalSubjectFilterBuilder_.getMessageOrBuilder();
} else {
return optionalSubjectFilter_ == null ?
com.authzed.api.v1.PermissionService.SubjectFilter.getDefaultInstance() : optionalSubjectFilter_;
}
}
/**
* .authzed.api.v1.SubjectFilter optional_subject_filter = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.PermissionService.SubjectFilter, com.authzed.api.v1.PermissionService.SubjectFilter.Builder, com.authzed.api.v1.PermissionService.SubjectFilterOrBuilder>
getOptionalSubjectFilterFieldBuilder() {
if (optionalSubjectFilterBuilder_ == null) {
optionalSubjectFilterBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.PermissionService.SubjectFilter, com.authzed.api.v1.PermissionService.SubjectFilter.Builder, com.authzed.api.v1.PermissionService.SubjectFilterOrBuilder>(
getOptionalSubjectFilter(),
getParentForChildren(),
isClean());
optionalSubjectFilter_ = null;
}
return optionalSubjectFilterBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:authzed.api.v1.RelationshipFilter)
}
// @@protoc_insertion_point(class_scope:authzed.api.v1.RelationshipFilter)
private static final com.authzed.api.v1.PermissionService.RelationshipFilter DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.authzed.api.v1.PermissionService.RelationshipFilter();
}
public static com.authzed.api.v1.PermissionService.RelationshipFilter getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public RelationshipFilter parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.RelationshipFilter getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface SubjectFilterOrBuilder extends
// @@protoc_insertion_point(interface_extends:authzed.api.v1.SubjectFilter)
com.google.protobuf.MessageOrBuilder {
/**
* string subject_type = 1 [(.validate.rules) = { ... }
* @return The subjectType.
*/
java.lang.String getSubjectType();
/**
* string subject_type = 1 [(.validate.rules) = { ... }
* @return The bytes for subjectType.
*/
com.google.protobuf.ByteString
getSubjectTypeBytes();
/**
* string optional_subject_id = 2 [(.validate.rules) = { ... }
* @return The optionalSubjectId.
*/
java.lang.String getOptionalSubjectId();
/**
* string optional_subject_id = 2 [(.validate.rules) = { ... }
* @return The bytes for optionalSubjectId.
*/
com.google.protobuf.ByteString
getOptionalSubjectIdBytes();
/**
* .authzed.api.v1.SubjectFilter.RelationFilter optional_relation = 3;
* @return Whether the optionalRelation field is set.
*/
boolean hasOptionalRelation();
/**
* .authzed.api.v1.SubjectFilter.RelationFilter optional_relation = 3;
* @return The optionalRelation.
*/
com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter getOptionalRelation();
/**
* .authzed.api.v1.SubjectFilter.RelationFilter optional_relation = 3;
*/
com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilterOrBuilder getOptionalRelationOrBuilder();
}
/**
*
* SubjectFilter specifies a filter on the subject of a relationship.
* subject_type is required and all other fields are optional, and will not
* impose any additional requirements if left unspecified.
*
*
* Protobuf type {@code authzed.api.v1.SubjectFilter}
*/
public static final class SubjectFilter extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:authzed.api.v1.SubjectFilter)
SubjectFilterOrBuilder {
private static final long serialVersionUID = 0L;
// Use SubjectFilter.newBuilder() to construct.
private SubjectFilter(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private SubjectFilter() {
subjectType_ = "";
optionalSubjectId_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new SubjectFilter();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_SubjectFilter_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_SubjectFilter_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.PermissionService.SubjectFilter.class, com.authzed.api.v1.PermissionService.SubjectFilter.Builder.class);
}
public interface RelationFilterOrBuilder extends
// @@protoc_insertion_point(interface_extends:authzed.api.v1.SubjectFilter.RelationFilter)
com.google.protobuf.MessageOrBuilder {
/**
* string relation = 1 [(.validate.rules) = { ... }
* @return The relation.
*/
java.lang.String getRelation();
/**
* string relation = 1 [(.validate.rules) = { ... }
* @return The bytes for relation.
*/
com.google.protobuf.ByteString
getRelationBytes();
}
/**
* Protobuf type {@code authzed.api.v1.SubjectFilter.RelationFilter}
*/
public static final class RelationFilter extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:authzed.api.v1.SubjectFilter.RelationFilter)
RelationFilterOrBuilder {
private static final long serialVersionUID = 0L;
// Use RelationFilter.newBuilder() to construct.
private RelationFilter(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RelationFilter() {
relation_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new RelationFilter();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_SubjectFilter_RelationFilter_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_SubjectFilter_RelationFilter_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter.class, com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter.Builder.class);
}
public static final int RELATION_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object relation_ = "";
/**
* string relation = 1 [(.validate.rules) = { ... }
* @return The relation.
*/
@java.lang.Override
public java.lang.String getRelation() {
java.lang.Object ref = relation_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
relation_ = s;
return s;
}
}
/**
* string relation = 1 [(.validate.rules) = { ... }
* @return The bytes for relation.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getRelationBytes() {
java.lang.Object ref = relation_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
relation_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(relation_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, relation_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(relation_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, relation_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter)) {
return super.equals(obj);
}
com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter other = (com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter) obj;
if (!getRelation()
.equals(other.getRelation())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + RELATION_FIELD_NUMBER;
hash = (53 * hash) + getRelation().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code authzed.api.v1.SubjectFilter.RelationFilter}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:authzed.api.v1.SubjectFilter.RelationFilter)
com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilterOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_SubjectFilter_RelationFilter_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_SubjectFilter_RelationFilter_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter.class, com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter.Builder.class);
}
// Construct using com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
relation_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_SubjectFilter_RelationFilter_descriptor;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter getDefaultInstanceForType() {
return com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter.getDefaultInstance();
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter build() {
com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter buildPartial() {
com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter result = new com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.relation_ = relation_;
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter) {
return mergeFrom((com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter other) {
if (other == com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter.getDefaultInstance()) return this;
if (!other.getRelation().isEmpty()) {
relation_ = other.relation_;
bitField0_ |= 0x00000001;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
relation_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 10
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object relation_ = "";
/**
* string relation = 1 [(.validate.rules) = { ... }
* @return The relation.
*/
public java.lang.String getRelation() {
java.lang.Object ref = relation_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
relation_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string relation = 1 [(.validate.rules) = { ... }
* @return The bytes for relation.
*/
public com.google.protobuf.ByteString
getRelationBytes() {
java.lang.Object ref = relation_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
relation_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string relation = 1 [(.validate.rules) = { ... }
* @param value The relation to set.
* @return This builder for chaining.
*/
public Builder setRelation(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
relation_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* string relation = 1 [(.validate.rules) = { ... }
* @return This builder for chaining.
*/
public Builder clearRelation() {
relation_ = getDefaultInstance().getRelation();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* string relation = 1 [(.validate.rules) = { ... }
* @param value The bytes for relation to set.
* @return This builder for chaining.
*/
public Builder setRelationBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
relation_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:authzed.api.v1.SubjectFilter.RelationFilter)
}
// @@protoc_insertion_point(class_scope:authzed.api.v1.SubjectFilter.RelationFilter)
private static final com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter();
}
public static com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public RelationFilter parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public static final int SUBJECT_TYPE_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object subjectType_ = "";
/**
* string subject_type = 1 [(.validate.rules) = { ... }
* @return The subjectType.
*/
@java.lang.Override
public java.lang.String getSubjectType() {
java.lang.Object ref = subjectType_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
subjectType_ = s;
return s;
}
}
/**
* string subject_type = 1 [(.validate.rules) = { ... }
* @return The bytes for subjectType.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getSubjectTypeBytes() {
java.lang.Object ref = subjectType_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
subjectType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int OPTIONAL_SUBJECT_ID_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object optionalSubjectId_ = "";
/**
* string optional_subject_id = 2 [(.validate.rules) = { ... }
* @return The optionalSubjectId.
*/
@java.lang.Override
public java.lang.String getOptionalSubjectId() {
java.lang.Object ref = optionalSubjectId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
optionalSubjectId_ = s;
return s;
}
}
/**
* string optional_subject_id = 2 [(.validate.rules) = { ... }
* @return The bytes for optionalSubjectId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getOptionalSubjectIdBytes() {
java.lang.Object ref = optionalSubjectId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
optionalSubjectId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int OPTIONAL_RELATION_FIELD_NUMBER = 3;
private com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter optionalRelation_;
/**
* .authzed.api.v1.SubjectFilter.RelationFilter optional_relation = 3;
* @return Whether the optionalRelation field is set.
*/
@java.lang.Override
public boolean hasOptionalRelation() {
return optionalRelation_ != null;
}
/**
* .authzed.api.v1.SubjectFilter.RelationFilter optional_relation = 3;
* @return The optionalRelation.
*/
@java.lang.Override
public com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter getOptionalRelation() {
return optionalRelation_ == null ? com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter.getDefaultInstance() : optionalRelation_;
}
/**
* .authzed.api.v1.SubjectFilter.RelationFilter optional_relation = 3;
*/
@java.lang.Override
public com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilterOrBuilder getOptionalRelationOrBuilder() {
return optionalRelation_ == null ? com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter.getDefaultInstance() : optionalRelation_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(subjectType_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, subjectType_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(optionalSubjectId_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, optionalSubjectId_);
}
if (optionalRelation_ != null) {
output.writeMessage(3, getOptionalRelation());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(subjectType_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, subjectType_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(optionalSubjectId_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, optionalSubjectId_);
}
if (optionalRelation_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getOptionalRelation());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.authzed.api.v1.PermissionService.SubjectFilter)) {
return super.equals(obj);
}
com.authzed.api.v1.PermissionService.SubjectFilter other = (com.authzed.api.v1.PermissionService.SubjectFilter) obj;
if (!getSubjectType()
.equals(other.getSubjectType())) return false;
if (!getOptionalSubjectId()
.equals(other.getOptionalSubjectId())) return false;
if (hasOptionalRelation() != other.hasOptionalRelation()) return false;
if (hasOptionalRelation()) {
if (!getOptionalRelation()
.equals(other.getOptionalRelation())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + SUBJECT_TYPE_FIELD_NUMBER;
hash = (53 * hash) + getSubjectType().hashCode();
hash = (37 * hash) + OPTIONAL_SUBJECT_ID_FIELD_NUMBER;
hash = (53 * hash) + getOptionalSubjectId().hashCode();
if (hasOptionalRelation()) {
hash = (37 * hash) + OPTIONAL_RELATION_FIELD_NUMBER;
hash = (53 * hash) + getOptionalRelation().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.authzed.api.v1.PermissionService.SubjectFilter parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.SubjectFilter parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.SubjectFilter parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.SubjectFilter parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.SubjectFilter parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.SubjectFilter parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.SubjectFilter parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.SubjectFilter parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.SubjectFilter parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.SubjectFilter parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.SubjectFilter parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.SubjectFilter parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.authzed.api.v1.PermissionService.SubjectFilter prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* SubjectFilter specifies a filter on the subject of a relationship.
* subject_type is required and all other fields are optional, and will not
* impose any additional requirements if left unspecified.
*
*
* Protobuf type {@code authzed.api.v1.SubjectFilter}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:authzed.api.v1.SubjectFilter)
com.authzed.api.v1.PermissionService.SubjectFilterOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_SubjectFilter_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_SubjectFilter_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.PermissionService.SubjectFilter.class, com.authzed.api.v1.PermissionService.SubjectFilter.Builder.class);
}
// Construct using com.authzed.api.v1.PermissionService.SubjectFilter.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
subjectType_ = "";
optionalSubjectId_ = "";
optionalRelation_ = null;
if (optionalRelationBuilder_ != null) {
optionalRelationBuilder_.dispose();
optionalRelationBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_SubjectFilter_descriptor;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.SubjectFilter getDefaultInstanceForType() {
return com.authzed.api.v1.PermissionService.SubjectFilter.getDefaultInstance();
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.SubjectFilter build() {
com.authzed.api.v1.PermissionService.SubjectFilter result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.SubjectFilter buildPartial() {
com.authzed.api.v1.PermissionService.SubjectFilter result = new com.authzed.api.v1.PermissionService.SubjectFilter(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.authzed.api.v1.PermissionService.SubjectFilter result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.subjectType_ = subjectType_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.optionalSubjectId_ = optionalSubjectId_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.optionalRelation_ = optionalRelationBuilder_ == null
? optionalRelation_
: optionalRelationBuilder_.build();
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.authzed.api.v1.PermissionService.SubjectFilter) {
return mergeFrom((com.authzed.api.v1.PermissionService.SubjectFilter)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.authzed.api.v1.PermissionService.SubjectFilter other) {
if (other == com.authzed.api.v1.PermissionService.SubjectFilter.getDefaultInstance()) return this;
if (!other.getSubjectType().isEmpty()) {
subjectType_ = other.subjectType_;
bitField0_ |= 0x00000001;
onChanged();
}
if (!other.getOptionalSubjectId().isEmpty()) {
optionalSubjectId_ = other.optionalSubjectId_;
bitField0_ |= 0x00000002;
onChanged();
}
if (other.hasOptionalRelation()) {
mergeOptionalRelation(other.getOptionalRelation());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
subjectType_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
optionalSubjectId_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 18
case 26: {
input.readMessage(
getOptionalRelationFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000004;
break;
} // case 26
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object subjectType_ = "";
/**
* string subject_type = 1 [(.validate.rules) = { ... }
* @return The subjectType.
*/
public java.lang.String getSubjectType() {
java.lang.Object ref = subjectType_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
subjectType_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string subject_type = 1 [(.validate.rules) = { ... }
* @return The bytes for subjectType.
*/
public com.google.protobuf.ByteString
getSubjectTypeBytes() {
java.lang.Object ref = subjectType_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
subjectType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string subject_type = 1 [(.validate.rules) = { ... }
* @param value The subjectType to set.
* @return This builder for chaining.
*/
public Builder setSubjectType(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
subjectType_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* string subject_type = 1 [(.validate.rules) = { ... }
* @return This builder for chaining.
*/
public Builder clearSubjectType() {
subjectType_ = getDefaultInstance().getSubjectType();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* string subject_type = 1 [(.validate.rules) = { ... }
* @param value The bytes for subjectType to set.
* @return This builder for chaining.
*/
public Builder setSubjectTypeBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
subjectType_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private java.lang.Object optionalSubjectId_ = "";
/**
* string optional_subject_id = 2 [(.validate.rules) = { ... }
* @return The optionalSubjectId.
*/
public java.lang.String getOptionalSubjectId() {
java.lang.Object ref = optionalSubjectId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
optionalSubjectId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string optional_subject_id = 2 [(.validate.rules) = { ... }
* @return The bytes for optionalSubjectId.
*/
public com.google.protobuf.ByteString
getOptionalSubjectIdBytes() {
java.lang.Object ref = optionalSubjectId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
optionalSubjectId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string optional_subject_id = 2 [(.validate.rules) = { ... }
* @param value The optionalSubjectId to set.
* @return This builder for chaining.
*/
public Builder setOptionalSubjectId(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
optionalSubjectId_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* string optional_subject_id = 2 [(.validate.rules) = { ... }
* @return This builder for chaining.
*/
public Builder clearOptionalSubjectId() {
optionalSubjectId_ = getDefaultInstance().getOptionalSubjectId();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
* string optional_subject_id = 2 [(.validate.rules) = { ... }
* @param value The bytes for optionalSubjectId to set.
* @return This builder for chaining.
*/
public Builder setOptionalSubjectIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
optionalSubjectId_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter optionalRelation_;
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter, com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter.Builder, com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilterOrBuilder> optionalRelationBuilder_;
/**
* .authzed.api.v1.SubjectFilter.RelationFilter optional_relation = 3;
* @return Whether the optionalRelation field is set.
*/
public boolean hasOptionalRelation() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* .authzed.api.v1.SubjectFilter.RelationFilter optional_relation = 3;
* @return The optionalRelation.
*/
public com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter getOptionalRelation() {
if (optionalRelationBuilder_ == null) {
return optionalRelation_ == null ? com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter.getDefaultInstance() : optionalRelation_;
} else {
return optionalRelationBuilder_.getMessage();
}
}
/**
* .authzed.api.v1.SubjectFilter.RelationFilter optional_relation = 3;
*/
public Builder setOptionalRelation(com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter value) {
if (optionalRelationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
optionalRelation_ = value;
} else {
optionalRelationBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* .authzed.api.v1.SubjectFilter.RelationFilter optional_relation = 3;
*/
public Builder setOptionalRelation(
com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter.Builder builderForValue) {
if (optionalRelationBuilder_ == null) {
optionalRelation_ = builderForValue.build();
} else {
optionalRelationBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* .authzed.api.v1.SubjectFilter.RelationFilter optional_relation = 3;
*/
public Builder mergeOptionalRelation(com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter value) {
if (optionalRelationBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0) &&
optionalRelation_ != null &&
optionalRelation_ != com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter.getDefaultInstance()) {
getOptionalRelationBuilder().mergeFrom(value);
} else {
optionalRelation_ = value;
}
} else {
optionalRelationBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* .authzed.api.v1.SubjectFilter.RelationFilter optional_relation = 3;
*/
public Builder clearOptionalRelation() {
bitField0_ = (bitField0_ & ~0x00000004);
optionalRelation_ = null;
if (optionalRelationBuilder_ != null) {
optionalRelationBuilder_.dispose();
optionalRelationBuilder_ = null;
}
onChanged();
return this;
}
/**
* .authzed.api.v1.SubjectFilter.RelationFilter optional_relation = 3;
*/
public com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter.Builder getOptionalRelationBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getOptionalRelationFieldBuilder().getBuilder();
}
/**
* .authzed.api.v1.SubjectFilter.RelationFilter optional_relation = 3;
*/
public com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilterOrBuilder getOptionalRelationOrBuilder() {
if (optionalRelationBuilder_ != null) {
return optionalRelationBuilder_.getMessageOrBuilder();
} else {
return optionalRelation_ == null ?
com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter.getDefaultInstance() : optionalRelation_;
}
}
/**
* .authzed.api.v1.SubjectFilter.RelationFilter optional_relation = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter, com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter.Builder, com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilterOrBuilder>
getOptionalRelationFieldBuilder() {
if (optionalRelationBuilder_ == null) {
optionalRelationBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter, com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilter.Builder, com.authzed.api.v1.PermissionService.SubjectFilter.RelationFilterOrBuilder>(
getOptionalRelation(),
getParentForChildren(),
isClean());
optionalRelation_ = null;
}
return optionalRelationBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:authzed.api.v1.SubjectFilter)
}
// @@protoc_insertion_point(class_scope:authzed.api.v1.SubjectFilter)
private static final com.authzed.api.v1.PermissionService.SubjectFilter DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.authzed.api.v1.PermissionService.SubjectFilter();
}
public static com.authzed.api.v1.PermissionService.SubjectFilter getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public SubjectFilter parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.SubjectFilter getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ReadRelationshipsRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:authzed.api.v1.ReadRelationshipsRequest)
com.google.protobuf.MessageOrBuilder {
/**
* .authzed.api.v1.Consistency consistency = 1;
* @return Whether the consistency field is set.
*/
boolean hasConsistency();
/**
* .authzed.api.v1.Consistency consistency = 1;
* @return The consistency.
*/
com.authzed.api.v1.PermissionService.Consistency getConsistency();
/**
* .authzed.api.v1.Consistency consistency = 1;
*/
com.authzed.api.v1.PermissionService.ConsistencyOrBuilder getConsistencyOrBuilder();
/**
* .authzed.api.v1.RelationshipFilter relationship_filter = 2 [(.validate.rules) = { ... }
* @return Whether the relationshipFilter field is set.
*/
boolean hasRelationshipFilter();
/**
* .authzed.api.v1.RelationshipFilter relationship_filter = 2 [(.validate.rules) = { ... }
* @return The relationshipFilter.
*/
com.authzed.api.v1.PermissionService.RelationshipFilter getRelationshipFilter();
/**
* .authzed.api.v1.RelationshipFilter relationship_filter = 2 [(.validate.rules) = { ... }
*/
com.authzed.api.v1.PermissionService.RelationshipFilterOrBuilder getRelationshipFilterOrBuilder();
}
/**
*
* ReadRelationshipsRequest specifies one or more filters used to read matching
* relationships within the system.
*
*
* Protobuf type {@code authzed.api.v1.ReadRelationshipsRequest}
*/
public static final class ReadRelationshipsRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:authzed.api.v1.ReadRelationshipsRequest)
ReadRelationshipsRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use ReadRelationshipsRequest.newBuilder() to construct.
private ReadRelationshipsRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ReadRelationshipsRequest() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ReadRelationshipsRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_ReadRelationshipsRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_ReadRelationshipsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.PermissionService.ReadRelationshipsRequest.class, com.authzed.api.v1.PermissionService.ReadRelationshipsRequest.Builder.class);
}
public static final int CONSISTENCY_FIELD_NUMBER = 1;
private com.authzed.api.v1.PermissionService.Consistency consistency_;
/**
* .authzed.api.v1.Consistency consistency = 1;
* @return Whether the consistency field is set.
*/
@java.lang.Override
public boolean hasConsistency() {
return consistency_ != null;
}
/**
* .authzed.api.v1.Consistency consistency = 1;
* @return The consistency.
*/
@java.lang.Override
public com.authzed.api.v1.PermissionService.Consistency getConsistency() {
return consistency_ == null ? com.authzed.api.v1.PermissionService.Consistency.getDefaultInstance() : consistency_;
}
/**
* .authzed.api.v1.Consistency consistency = 1;
*/
@java.lang.Override
public com.authzed.api.v1.PermissionService.ConsistencyOrBuilder getConsistencyOrBuilder() {
return consistency_ == null ? com.authzed.api.v1.PermissionService.Consistency.getDefaultInstance() : consistency_;
}
public static final int RELATIONSHIP_FILTER_FIELD_NUMBER = 2;
private com.authzed.api.v1.PermissionService.RelationshipFilter relationshipFilter_;
/**
* .authzed.api.v1.RelationshipFilter relationship_filter = 2 [(.validate.rules) = { ... }
* @return Whether the relationshipFilter field is set.
*/
@java.lang.Override
public boolean hasRelationshipFilter() {
return relationshipFilter_ != null;
}
/**
* .authzed.api.v1.RelationshipFilter relationship_filter = 2 [(.validate.rules) = { ... }
* @return The relationshipFilter.
*/
@java.lang.Override
public com.authzed.api.v1.PermissionService.RelationshipFilter getRelationshipFilter() {
return relationshipFilter_ == null ? com.authzed.api.v1.PermissionService.RelationshipFilter.getDefaultInstance() : relationshipFilter_;
}
/**
* .authzed.api.v1.RelationshipFilter relationship_filter = 2 [(.validate.rules) = { ... }
*/
@java.lang.Override
public com.authzed.api.v1.PermissionService.RelationshipFilterOrBuilder getRelationshipFilterOrBuilder() {
return relationshipFilter_ == null ? com.authzed.api.v1.PermissionService.RelationshipFilter.getDefaultInstance() : relationshipFilter_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (consistency_ != null) {
output.writeMessage(1, getConsistency());
}
if (relationshipFilter_ != null) {
output.writeMessage(2, getRelationshipFilter());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (consistency_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getConsistency());
}
if (relationshipFilter_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getRelationshipFilter());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.authzed.api.v1.PermissionService.ReadRelationshipsRequest)) {
return super.equals(obj);
}
com.authzed.api.v1.PermissionService.ReadRelationshipsRequest other = (com.authzed.api.v1.PermissionService.ReadRelationshipsRequest) obj;
if (hasConsistency() != other.hasConsistency()) return false;
if (hasConsistency()) {
if (!getConsistency()
.equals(other.getConsistency())) return false;
}
if (hasRelationshipFilter() != other.hasRelationshipFilter()) return false;
if (hasRelationshipFilter()) {
if (!getRelationshipFilter()
.equals(other.getRelationshipFilter())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasConsistency()) {
hash = (37 * hash) + CONSISTENCY_FIELD_NUMBER;
hash = (53 * hash) + getConsistency().hashCode();
}
if (hasRelationshipFilter()) {
hash = (37 * hash) + RELATIONSHIP_FILTER_FIELD_NUMBER;
hash = (53 * hash) + getRelationshipFilter().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.authzed.api.v1.PermissionService.ReadRelationshipsRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.ReadRelationshipsRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.ReadRelationshipsRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.ReadRelationshipsRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.ReadRelationshipsRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.ReadRelationshipsRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.ReadRelationshipsRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.ReadRelationshipsRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.ReadRelationshipsRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.ReadRelationshipsRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.ReadRelationshipsRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.ReadRelationshipsRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.authzed.api.v1.PermissionService.ReadRelationshipsRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* ReadRelationshipsRequest specifies one or more filters used to read matching
* relationships within the system.
*
*
* Protobuf type {@code authzed.api.v1.ReadRelationshipsRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:authzed.api.v1.ReadRelationshipsRequest)
com.authzed.api.v1.PermissionService.ReadRelationshipsRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_ReadRelationshipsRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_ReadRelationshipsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.PermissionService.ReadRelationshipsRequest.class, com.authzed.api.v1.PermissionService.ReadRelationshipsRequest.Builder.class);
}
// Construct using com.authzed.api.v1.PermissionService.ReadRelationshipsRequest.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
consistency_ = null;
if (consistencyBuilder_ != null) {
consistencyBuilder_.dispose();
consistencyBuilder_ = null;
}
relationshipFilter_ = null;
if (relationshipFilterBuilder_ != null) {
relationshipFilterBuilder_.dispose();
relationshipFilterBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_ReadRelationshipsRequest_descriptor;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.ReadRelationshipsRequest getDefaultInstanceForType() {
return com.authzed.api.v1.PermissionService.ReadRelationshipsRequest.getDefaultInstance();
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.ReadRelationshipsRequest build() {
com.authzed.api.v1.PermissionService.ReadRelationshipsRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.ReadRelationshipsRequest buildPartial() {
com.authzed.api.v1.PermissionService.ReadRelationshipsRequest result = new com.authzed.api.v1.PermissionService.ReadRelationshipsRequest(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.authzed.api.v1.PermissionService.ReadRelationshipsRequest result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.consistency_ = consistencyBuilder_ == null
? consistency_
: consistencyBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.relationshipFilter_ = relationshipFilterBuilder_ == null
? relationshipFilter_
: relationshipFilterBuilder_.build();
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.authzed.api.v1.PermissionService.ReadRelationshipsRequest) {
return mergeFrom((com.authzed.api.v1.PermissionService.ReadRelationshipsRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.authzed.api.v1.PermissionService.ReadRelationshipsRequest other) {
if (other == com.authzed.api.v1.PermissionService.ReadRelationshipsRequest.getDefaultInstance()) return this;
if (other.hasConsistency()) {
mergeConsistency(other.getConsistency());
}
if (other.hasRelationshipFilter()) {
mergeRelationshipFilter(other.getRelationshipFilter());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
input.readMessage(
getConsistencyFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
input.readMessage(
getRelationshipFilterFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000002;
break;
} // case 18
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.authzed.api.v1.PermissionService.Consistency consistency_;
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.PermissionService.Consistency, com.authzed.api.v1.PermissionService.Consistency.Builder, com.authzed.api.v1.PermissionService.ConsistencyOrBuilder> consistencyBuilder_;
/**
* .authzed.api.v1.Consistency consistency = 1;
* @return Whether the consistency field is set.
*/
public boolean hasConsistency() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* .authzed.api.v1.Consistency consistency = 1;
* @return The consistency.
*/
public com.authzed.api.v1.PermissionService.Consistency getConsistency() {
if (consistencyBuilder_ == null) {
return consistency_ == null ? com.authzed.api.v1.PermissionService.Consistency.getDefaultInstance() : consistency_;
} else {
return consistencyBuilder_.getMessage();
}
}
/**
* .authzed.api.v1.Consistency consistency = 1;
*/
public Builder setConsistency(com.authzed.api.v1.PermissionService.Consistency value) {
if (consistencyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
consistency_ = value;
} else {
consistencyBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .authzed.api.v1.Consistency consistency = 1;
*/
public Builder setConsistency(
com.authzed.api.v1.PermissionService.Consistency.Builder builderForValue) {
if (consistencyBuilder_ == null) {
consistency_ = builderForValue.build();
} else {
consistencyBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .authzed.api.v1.Consistency consistency = 1;
*/
public Builder mergeConsistency(com.authzed.api.v1.PermissionService.Consistency value) {
if (consistencyBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0) &&
consistency_ != null &&
consistency_ != com.authzed.api.v1.PermissionService.Consistency.getDefaultInstance()) {
getConsistencyBuilder().mergeFrom(value);
} else {
consistency_ = value;
}
} else {
consistencyBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .authzed.api.v1.Consistency consistency = 1;
*/
public Builder clearConsistency() {
bitField0_ = (bitField0_ & ~0x00000001);
consistency_ = null;
if (consistencyBuilder_ != null) {
consistencyBuilder_.dispose();
consistencyBuilder_ = null;
}
onChanged();
return this;
}
/**
* .authzed.api.v1.Consistency consistency = 1;
*/
public com.authzed.api.v1.PermissionService.Consistency.Builder getConsistencyBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getConsistencyFieldBuilder().getBuilder();
}
/**
* .authzed.api.v1.Consistency consistency = 1;
*/
public com.authzed.api.v1.PermissionService.ConsistencyOrBuilder getConsistencyOrBuilder() {
if (consistencyBuilder_ != null) {
return consistencyBuilder_.getMessageOrBuilder();
} else {
return consistency_ == null ?
com.authzed.api.v1.PermissionService.Consistency.getDefaultInstance() : consistency_;
}
}
/**
* .authzed.api.v1.Consistency consistency = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.PermissionService.Consistency, com.authzed.api.v1.PermissionService.Consistency.Builder, com.authzed.api.v1.PermissionService.ConsistencyOrBuilder>
getConsistencyFieldBuilder() {
if (consistencyBuilder_ == null) {
consistencyBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.PermissionService.Consistency, com.authzed.api.v1.PermissionService.Consistency.Builder, com.authzed.api.v1.PermissionService.ConsistencyOrBuilder>(
getConsistency(),
getParentForChildren(),
isClean());
consistency_ = null;
}
return consistencyBuilder_;
}
private com.authzed.api.v1.PermissionService.RelationshipFilter relationshipFilter_;
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.PermissionService.RelationshipFilter, com.authzed.api.v1.PermissionService.RelationshipFilter.Builder, com.authzed.api.v1.PermissionService.RelationshipFilterOrBuilder> relationshipFilterBuilder_;
/**
* .authzed.api.v1.RelationshipFilter relationship_filter = 2 [(.validate.rules) = { ... }
* @return Whether the relationshipFilter field is set.
*/
public boolean hasRelationshipFilter() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* .authzed.api.v1.RelationshipFilter relationship_filter = 2 [(.validate.rules) = { ... }
* @return The relationshipFilter.
*/
public com.authzed.api.v1.PermissionService.RelationshipFilter getRelationshipFilter() {
if (relationshipFilterBuilder_ == null) {
return relationshipFilter_ == null ? com.authzed.api.v1.PermissionService.RelationshipFilter.getDefaultInstance() : relationshipFilter_;
} else {
return relationshipFilterBuilder_.getMessage();
}
}
/**
* .authzed.api.v1.RelationshipFilter relationship_filter = 2 [(.validate.rules) = { ... }
*/
public Builder setRelationshipFilter(com.authzed.api.v1.PermissionService.RelationshipFilter value) {
if (relationshipFilterBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
relationshipFilter_ = value;
} else {
relationshipFilterBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* .authzed.api.v1.RelationshipFilter relationship_filter = 2 [(.validate.rules) = { ... }
*/
public Builder setRelationshipFilter(
com.authzed.api.v1.PermissionService.RelationshipFilter.Builder builderForValue) {
if (relationshipFilterBuilder_ == null) {
relationshipFilter_ = builderForValue.build();
} else {
relationshipFilterBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* .authzed.api.v1.RelationshipFilter relationship_filter = 2 [(.validate.rules) = { ... }
*/
public Builder mergeRelationshipFilter(com.authzed.api.v1.PermissionService.RelationshipFilter value) {
if (relationshipFilterBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0) &&
relationshipFilter_ != null &&
relationshipFilter_ != com.authzed.api.v1.PermissionService.RelationshipFilter.getDefaultInstance()) {
getRelationshipFilterBuilder().mergeFrom(value);
} else {
relationshipFilter_ = value;
}
} else {
relationshipFilterBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* .authzed.api.v1.RelationshipFilter relationship_filter = 2 [(.validate.rules) = { ... }
*/
public Builder clearRelationshipFilter() {
bitField0_ = (bitField0_ & ~0x00000002);
relationshipFilter_ = null;
if (relationshipFilterBuilder_ != null) {
relationshipFilterBuilder_.dispose();
relationshipFilterBuilder_ = null;
}
onChanged();
return this;
}
/**
* .authzed.api.v1.RelationshipFilter relationship_filter = 2 [(.validate.rules) = { ... }
*/
public com.authzed.api.v1.PermissionService.RelationshipFilter.Builder getRelationshipFilterBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getRelationshipFilterFieldBuilder().getBuilder();
}
/**
* .authzed.api.v1.RelationshipFilter relationship_filter = 2 [(.validate.rules) = { ... }
*/
public com.authzed.api.v1.PermissionService.RelationshipFilterOrBuilder getRelationshipFilterOrBuilder() {
if (relationshipFilterBuilder_ != null) {
return relationshipFilterBuilder_.getMessageOrBuilder();
} else {
return relationshipFilter_ == null ?
com.authzed.api.v1.PermissionService.RelationshipFilter.getDefaultInstance() : relationshipFilter_;
}
}
/**
* .authzed.api.v1.RelationshipFilter relationship_filter = 2 [(.validate.rules) = { ... }
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.PermissionService.RelationshipFilter, com.authzed.api.v1.PermissionService.RelationshipFilter.Builder, com.authzed.api.v1.PermissionService.RelationshipFilterOrBuilder>
getRelationshipFilterFieldBuilder() {
if (relationshipFilterBuilder_ == null) {
relationshipFilterBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.PermissionService.RelationshipFilter, com.authzed.api.v1.PermissionService.RelationshipFilter.Builder, com.authzed.api.v1.PermissionService.RelationshipFilterOrBuilder>(
getRelationshipFilter(),
getParentForChildren(),
isClean());
relationshipFilter_ = null;
}
return relationshipFilterBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:authzed.api.v1.ReadRelationshipsRequest)
}
// @@protoc_insertion_point(class_scope:authzed.api.v1.ReadRelationshipsRequest)
private static final com.authzed.api.v1.PermissionService.ReadRelationshipsRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.authzed.api.v1.PermissionService.ReadRelationshipsRequest();
}
public static com.authzed.api.v1.PermissionService.ReadRelationshipsRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ReadRelationshipsRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.ReadRelationshipsRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ReadRelationshipsResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:authzed.api.v1.ReadRelationshipsResponse)
com.google.protobuf.MessageOrBuilder {
/**
* .authzed.api.v1.ZedToken read_at = 1 [(.validate.rules) = { ... }
* @return Whether the readAt field is set.
*/
boolean hasReadAt();
/**
* .authzed.api.v1.ZedToken read_at = 1 [(.validate.rules) = { ... }
* @return The readAt.
*/
com.authzed.api.v1.Core.ZedToken getReadAt();
/**
* .authzed.api.v1.ZedToken read_at = 1 [(.validate.rules) = { ... }
*/
com.authzed.api.v1.Core.ZedTokenOrBuilder getReadAtOrBuilder();
/**
* .authzed.api.v1.Relationship relationship = 2 [(.validate.rules) = { ... }
* @return Whether the relationship field is set.
*/
boolean hasRelationship();
/**
* .authzed.api.v1.Relationship relationship = 2 [(.validate.rules) = { ... }
* @return The relationship.
*/
com.authzed.api.v1.Core.Relationship getRelationship();
/**
* .authzed.api.v1.Relationship relationship = 2 [(.validate.rules) = { ... }
*/
com.authzed.api.v1.Core.RelationshipOrBuilder getRelationshipOrBuilder();
}
/**
*
* ReadRelationshipsResponse contains a Relationship found that matches the
* specified relationship filter(s). A instance of this response message will
* be streamed to the client for each relationship found.
*
*
* Protobuf type {@code authzed.api.v1.ReadRelationshipsResponse}
*/
public static final class ReadRelationshipsResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:authzed.api.v1.ReadRelationshipsResponse)
ReadRelationshipsResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use ReadRelationshipsResponse.newBuilder() to construct.
private ReadRelationshipsResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ReadRelationshipsResponse() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ReadRelationshipsResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_ReadRelationshipsResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_ReadRelationshipsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.PermissionService.ReadRelationshipsResponse.class, com.authzed.api.v1.PermissionService.ReadRelationshipsResponse.Builder.class);
}
public static final int READ_AT_FIELD_NUMBER = 1;
private com.authzed.api.v1.Core.ZedToken readAt_;
/**
* .authzed.api.v1.ZedToken read_at = 1 [(.validate.rules) = { ... }
* @return Whether the readAt field is set.
*/
@java.lang.Override
public boolean hasReadAt() {
return readAt_ != null;
}
/**
* .authzed.api.v1.ZedToken read_at = 1 [(.validate.rules) = { ... }
* @return The readAt.
*/
@java.lang.Override
public com.authzed.api.v1.Core.ZedToken getReadAt() {
return readAt_ == null ? com.authzed.api.v1.Core.ZedToken.getDefaultInstance() : readAt_;
}
/**
* .authzed.api.v1.ZedToken read_at = 1 [(.validate.rules) = { ... }
*/
@java.lang.Override
public com.authzed.api.v1.Core.ZedTokenOrBuilder getReadAtOrBuilder() {
return readAt_ == null ? com.authzed.api.v1.Core.ZedToken.getDefaultInstance() : readAt_;
}
public static final int RELATIONSHIP_FIELD_NUMBER = 2;
private com.authzed.api.v1.Core.Relationship relationship_;
/**
* .authzed.api.v1.Relationship relationship = 2 [(.validate.rules) = { ... }
* @return Whether the relationship field is set.
*/
@java.lang.Override
public boolean hasRelationship() {
return relationship_ != null;
}
/**
* .authzed.api.v1.Relationship relationship = 2 [(.validate.rules) = { ... }
* @return The relationship.
*/
@java.lang.Override
public com.authzed.api.v1.Core.Relationship getRelationship() {
return relationship_ == null ? com.authzed.api.v1.Core.Relationship.getDefaultInstance() : relationship_;
}
/**
* .authzed.api.v1.Relationship relationship = 2 [(.validate.rules) = { ... }
*/
@java.lang.Override
public com.authzed.api.v1.Core.RelationshipOrBuilder getRelationshipOrBuilder() {
return relationship_ == null ? com.authzed.api.v1.Core.Relationship.getDefaultInstance() : relationship_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (readAt_ != null) {
output.writeMessage(1, getReadAt());
}
if (relationship_ != null) {
output.writeMessage(2, getRelationship());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (readAt_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getReadAt());
}
if (relationship_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getRelationship());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.authzed.api.v1.PermissionService.ReadRelationshipsResponse)) {
return super.equals(obj);
}
com.authzed.api.v1.PermissionService.ReadRelationshipsResponse other = (com.authzed.api.v1.PermissionService.ReadRelationshipsResponse) obj;
if (hasReadAt() != other.hasReadAt()) return false;
if (hasReadAt()) {
if (!getReadAt()
.equals(other.getReadAt())) return false;
}
if (hasRelationship() != other.hasRelationship()) return false;
if (hasRelationship()) {
if (!getRelationship()
.equals(other.getRelationship())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasReadAt()) {
hash = (37 * hash) + READ_AT_FIELD_NUMBER;
hash = (53 * hash) + getReadAt().hashCode();
}
if (hasRelationship()) {
hash = (37 * hash) + RELATIONSHIP_FIELD_NUMBER;
hash = (53 * hash) + getRelationship().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.authzed.api.v1.PermissionService.ReadRelationshipsResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.ReadRelationshipsResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.ReadRelationshipsResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.ReadRelationshipsResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.ReadRelationshipsResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.ReadRelationshipsResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.ReadRelationshipsResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.ReadRelationshipsResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.ReadRelationshipsResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.ReadRelationshipsResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.ReadRelationshipsResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.ReadRelationshipsResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.authzed.api.v1.PermissionService.ReadRelationshipsResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* ReadRelationshipsResponse contains a Relationship found that matches the
* specified relationship filter(s). A instance of this response message will
* be streamed to the client for each relationship found.
*
*
* Protobuf type {@code authzed.api.v1.ReadRelationshipsResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:authzed.api.v1.ReadRelationshipsResponse)
com.authzed.api.v1.PermissionService.ReadRelationshipsResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_ReadRelationshipsResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_ReadRelationshipsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.PermissionService.ReadRelationshipsResponse.class, com.authzed.api.v1.PermissionService.ReadRelationshipsResponse.Builder.class);
}
// Construct using com.authzed.api.v1.PermissionService.ReadRelationshipsResponse.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
readAt_ = null;
if (readAtBuilder_ != null) {
readAtBuilder_.dispose();
readAtBuilder_ = null;
}
relationship_ = null;
if (relationshipBuilder_ != null) {
relationshipBuilder_.dispose();
relationshipBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_ReadRelationshipsResponse_descriptor;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.ReadRelationshipsResponse getDefaultInstanceForType() {
return com.authzed.api.v1.PermissionService.ReadRelationshipsResponse.getDefaultInstance();
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.ReadRelationshipsResponse build() {
com.authzed.api.v1.PermissionService.ReadRelationshipsResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.ReadRelationshipsResponse buildPartial() {
com.authzed.api.v1.PermissionService.ReadRelationshipsResponse result = new com.authzed.api.v1.PermissionService.ReadRelationshipsResponse(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.authzed.api.v1.PermissionService.ReadRelationshipsResponse result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.readAt_ = readAtBuilder_ == null
? readAt_
: readAtBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.relationship_ = relationshipBuilder_ == null
? relationship_
: relationshipBuilder_.build();
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.authzed.api.v1.PermissionService.ReadRelationshipsResponse) {
return mergeFrom((com.authzed.api.v1.PermissionService.ReadRelationshipsResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.authzed.api.v1.PermissionService.ReadRelationshipsResponse other) {
if (other == com.authzed.api.v1.PermissionService.ReadRelationshipsResponse.getDefaultInstance()) return this;
if (other.hasReadAt()) {
mergeReadAt(other.getReadAt());
}
if (other.hasRelationship()) {
mergeRelationship(other.getRelationship());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
input.readMessage(
getReadAtFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
input.readMessage(
getRelationshipFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000002;
break;
} // case 18
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.authzed.api.v1.Core.ZedToken readAt_;
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.ZedToken, com.authzed.api.v1.Core.ZedToken.Builder, com.authzed.api.v1.Core.ZedTokenOrBuilder> readAtBuilder_;
/**
* .authzed.api.v1.ZedToken read_at = 1 [(.validate.rules) = { ... }
* @return Whether the readAt field is set.
*/
public boolean hasReadAt() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* .authzed.api.v1.ZedToken read_at = 1 [(.validate.rules) = { ... }
* @return The readAt.
*/
public com.authzed.api.v1.Core.ZedToken getReadAt() {
if (readAtBuilder_ == null) {
return readAt_ == null ? com.authzed.api.v1.Core.ZedToken.getDefaultInstance() : readAt_;
} else {
return readAtBuilder_.getMessage();
}
}
/**
* .authzed.api.v1.ZedToken read_at = 1 [(.validate.rules) = { ... }
*/
public Builder setReadAt(com.authzed.api.v1.Core.ZedToken value) {
if (readAtBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
readAt_ = value;
} else {
readAtBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .authzed.api.v1.ZedToken read_at = 1 [(.validate.rules) = { ... }
*/
public Builder setReadAt(
com.authzed.api.v1.Core.ZedToken.Builder builderForValue) {
if (readAtBuilder_ == null) {
readAt_ = builderForValue.build();
} else {
readAtBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .authzed.api.v1.ZedToken read_at = 1 [(.validate.rules) = { ... }
*/
public Builder mergeReadAt(com.authzed.api.v1.Core.ZedToken value) {
if (readAtBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0) &&
readAt_ != null &&
readAt_ != com.authzed.api.v1.Core.ZedToken.getDefaultInstance()) {
getReadAtBuilder().mergeFrom(value);
} else {
readAt_ = value;
}
} else {
readAtBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .authzed.api.v1.ZedToken read_at = 1 [(.validate.rules) = { ... }
*/
public Builder clearReadAt() {
bitField0_ = (bitField0_ & ~0x00000001);
readAt_ = null;
if (readAtBuilder_ != null) {
readAtBuilder_.dispose();
readAtBuilder_ = null;
}
onChanged();
return this;
}
/**
* .authzed.api.v1.ZedToken read_at = 1 [(.validate.rules) = { ... }
*/
public com.authzed.api.v1.Core.ZedToken.Builder getReadAtBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getReadAtFieldBuilder().getBuilder();
}
/**
* .authzed.api.v1.ZedToken read_at = 1 [(.validate.rules) = { ... }
*/
public com.authzed.api.v1.Core.ZedTokenOrBuilder getReadAtOrBuilder() {
if (readAtBuilder_ != null) {
return readAtBuilder_.getMessageOrBuilder();
} else {
return readAt_ == null ?
com.authzed.api.v1.Core.ZedToken.getDefaultInstance() : readAt_;
}
}
/**
* .authzed.api.v1.ZedToken read_at = 1 [(.validate.rules) = { ... }
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.ZedToken, com.authzed.api.v1.Core.ZedToken.Builder, com.authzed.api.v1.Core.ZedTokenOrBuilder>
getReadAtFieldBuilder() {
if (readAtBuilder_ == null) {
readAtBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.ZedToken, com.authzed.api.v1.Core.ZedToken.Builder, com.authzed.api.v1.Core.ZedTokenOrBuilder>(
getReadAt(),
getParentForChildren(),
isClean());
readAt_ = null;
}
return readAtBuilder_;
}
private com.authzed.api.v1.Core.Relationship relationship_;
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.Relationship, com.authzed.api.v1.Core.Relationship.Builder, com.authzed.api.v1.Core.RelationshipOrBuilder> relationshipBuilder_;
/**
* .authzed.api.v1.Relationship relationship = 2 [(.validate.rules) = { ... }
* @return Whether the relationship field is set.
*/
public boolean hasRelationship() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* .authzed.api.v1.Relationship relationship = 2 [(.validate.rules) = { ... }
* @return The relationship.
*/
public com.authzed.api.v1.Core.Relationship getRelationship() {
if (relationshipBuilder_ == null) {
return relationship_ == null ? com.authzed.api.v1.Core.Relationship.getDefaultInstance() : relationship_;
} else {
return relationshipBuilder_.getMessage();
}
}
/**
* .authzed.api.v1.Relationship relationship = 2 [(.validate.rules) = { ... }
*/
public Builder setRelationship(com.authzed.api.v1.Core.Relationship value) {
if (relationshipBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
relationship_ = value;
} else {
relationshipBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* .authzed.api.v1.Relationship relationship = 2 [(.validate.rules) = { ... }
*/
public Builder setRelationship(
com.authzed.api.v1.Core.Relationship.Builder builderForValue) {
if (relationshipBuilder_ == null) {
relationship_ = builderForValue.build();
} else {
relationshipBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* .authzed.api.v1.Relationship relationship = 2 [(.validate.rules) = { ... }
*/
public Builder mergeRelationship(com.authzed.api.v1.Core.Relationship value) {
if (relationshipBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0) &&
relationship_ != null &&
relationship_ != com.authzed.api.v1.Core.Relationship.getDefaultInstance()) {
getRelationshipBuilder().mergeFrom(value);
} else {
relationship_ = value;
}
} else {
relationshipBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* .authzed.api.v1.Relationship relationship = 2 [(.validate.rules) = { ... }
*/
public Builder clearRelationship() {
bitField0_ = (bitField0_ & ~0x00000002);
relationship_ = null;
if (relationshipBuilder_ != null) {
relationshipBuilder_.dispose();
relationshipBuilder_ = null;
}
onChanged();
return this;
}
/**
* .authzed.api.v1.Relationship relationship = 2 [(.validate.rules) = { ... }
*/
public com.authzed.api.v1.Core.Relationship.Builder getRelationshipBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getRelationshipFieldBuilder().getBuilder();
}
/**
* .authzed.api.v1.Relationship relationship = 2 [(.validate.rules) = { ... }
*/
public com.authzed.api.v1.Core.RelationshipOrBuilder getRelationshipOrBuilder() {
if (relationshipBuilder_ != null) {
return relationshipBuilder_.getMessageOrBuilder();
} else {
return relationship_ == null ?
com.authzed.api.v1.Core.Relationship.getDefaultInstance() : relationship_;
}
}
/**
* .authzed.api.v1.Relationship relationship = 2 [(.validate.rules) = { ... }
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.Relationship, com.authzed.api.v1.Core.Relationship.Builder, com.authzed.api.v1.Core.RelationshipOrBuilder>
getRelationshipFieldBuilder() {
if (relationshipBuilder_ == null) {
relationshipBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.Relationship, com.authzed.api.v1.Core.Relationship.Builder, com.authzed.api.v1.Core.RelationshipOrBuilder>(
getRelationship(),
getParentForChildren(),
isClean());
relationship_ = null;
}
return relationshipBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:authzed.api.v1.ReadRelationshipsResponse)
}
// @@protoc_insertion_point(class_scope:authzed.api.v1.ReadRelationshipsResponse)
private static final com.authzed.api.v1.PermissionService.ReadRelationshipsResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.authzed.api.v1.PermissionService.ReadRelationshipsResponse();
}
public static com.authzed.api.v1.PermissionService.ReadRelationshipsResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ReadRelationshipsResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.ReadRelationshipsResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PreconditionOrBuilder extends
// @@protoc_insertion_point(interface_extends:authzed.api.v1.Precondition)
com.google.protobuf.MessageOrBuilder {
/**
* .authzed.api.v1.Precondition.Operation operation = 1 [(.validate.rules) = { ... }
* @return The enum numeric value on the wire for operation.
*/
int getOperationValue();
/**
* .authzed.api.v1.Precondition.Operation operation = 1 [(.validate.rules) = { ... }
* @return The operation.
*/
com.authzed.api.v1.PermissionService.Precondition.Operation getOperation();
/**
* .authzed.api.v1.RelationshipFilter filter = 2 [(.validate.rules) = { ... }
* @return Whether the filter field is set.
*/
boolean hasFilter();
/**
* .authzed.api.v1.RelationshipFilter filter = 2 [(.validate.rules) = { ... }
* @return The filter.
*/
com.authzed.api.v1.PermissionService.RelationshipFilter getFilter();
/**
* .authzed.api.v1.RelationshipFilter filter = 2 [(.validate.rules) = { ... }
*/
com.authzed.api.v1.PermissionService.RelationshipFilterOrBuilder getFilterOrBuilder();
}
/**
*
* Precondition specifies how and the existence or absence of certain
* relationships as expressed through the accompanying filter should affect
* whether or not the operation proceeds.
* MUST_NOT_MATCH will fail the parent request if any relationships match the
* relationships filter.
* MUST_MATCH will fail the parent request if there are no
* relationships that match the filter.
*
*
* Protobuf type {@code authzed.api.v1.Precondition}
*/
public static final class Precondition extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:authzed.api.v1.Precondition)
PreconditionOrBuilder {
private static final long serialVersionUID = 0L;
// Use Precondition.newBuilder() to construct.
private Precondition(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Precondition() {
operation_ = 0;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Precondition();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_Precondition_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_Precondition_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.PermissionService.Precondition.class, com.authzed.api.v1.PermissionService.Precondition.Builder.class);
}
/**
* Protobuf enum {@code authzed.api.v1.Precondition.Operation}
*/
public enum Operation
implements com.google.protobuf.ProtocolMessageEnum {
/**
* OPERATION_UNSPECIFIED = 0;
*/
OPERATION_UNSPECIFIED(0),
/**
* OPERATION_MUST_NOT_MATCH = 1;
*/
OPERATION_MUST_NOT_MATCH(1),
/**
* OPERATION_MUST_MATCH = 2;
*/
OPERATION_MUST_MATCH(2),
UNRECOGNIZED(-1),
;
/**
* OPERATION_UNSPECIFIED = 0;
*/
public static final int OPERATION_UNSPECIFIED_VALUE = 0;
/**
* OPERATION_MUST_NOT_MATCH = 1;
*/
public static final int OPERATION_MUST_NOT_MATCH_VALUE = 1;
/**
* OPERATION_MUST_MATCH = 2;
*/
public static final int OPERATION_MUST_MATCH_VALUE = 2;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Operation valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static Operation forNumber(int value) {
switch (value) {
case 0: return OPERATION_UNSPECIFIED;
case 1: return OPERATION_MUST_NOT_MATCH;
case 2: return OPERATION_MUST_MATCH;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
Operation> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Operation findValueByNumber(int number) {
return Operation.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.authzed.api.v1.PermissionService.Precondition.getDescriptor().getEnumTypes().get(0);
}
private static final Operation[] VALUES = values();
public static Operation valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private Operation(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:authzed.api.v1.Precondition.Operation)
}
public static final int OPERATION_FIELD_NUMBER = 1;
private int operation_ = 0;
/**
* .authzed.api.v1.Precondition.Operation operation = 1 [(.validate.rules) = { ... }
* @return The enum numeric value on the wire for operation.
*/
@java.lang.Override public int getOperationValue() {
return operation_;
}
/**
* .authzed.api.v1.Precondition.Operation operation = 1 [(.validate.rules) = { ... }
* @return The operation.
*/
@java.lang.Override public com.authzed.api.v1.PermissionService.Precondition.Operation getOperation() {
com.authzed.api.v1.PermissionService.Precondition.Operation result = com.authzed.api.v1.PermissionService.Precondition.Operation.forNumber(operation_);
return result == null ? com.authzed.api.v1.PermissionService.Precondition.Operation.UNRECOGNIZED : result;
}
public static final int FILTER_FIELD_NUMBER = 2;
private com.authzed.api.v1.PermissionService.RelationshipFilter filter_;
/**
* .authzed.api.v1.RelationshipFilter filter = 2 [(.validate.rules) = { ... }
* @return Whether the filter field is set.
*/
@java.lang.Override
public boolean hasFilter() {
return filter_ != null;
}
/**
* .authzed.api.v1.RelationshipFilter filter = 2 [(.validate.rules) = { ... }
* @return The filter.
*/
@java.lang.Override
public com.authzed.api.v1.PermissionService.RelationshipFilter getFilter() {
return filter_ == null ? com.authzed.api.v1.PermissionService.RelationshipFilter.getDefaultInstance() : filter_;
}
/**
* .authzed.api.v1.RelationshipFilter filter = 2 [(.validate.rules) = { ... }
*/
@java.lang.Override
public com.authzed.api.v1.PermissionService.RelationshipFilterOrBuilder getFilterOrBuilder() {
return filter_ == null ? com.authzed.api.v1.PermissionService.RelationshipFilter.getDefaultInstance() : filter_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (operation_ != com.authzed.api.v1.PermissionService.Precondition.Operation.OPERATION_UNSPECIFIED.getNumber()) {
output.writeEnum(1, operation_);
}
if (filter_ != null) {
output.writeMessage(2, getFilter());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (operation_ != com.authzed.api.v1.PermissionService.Precondition.Operation.OPERATION_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, operation_);
}
if (filter_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getFilter());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.authzed.api.v1.PermissionService.Precondition)) {
return super.equals(obj);
}
com.authzed.api.v1.PermissionService.Precondition other = (com.authzed.api.v1.PermissionService.Precondition) obj;
if (operation_ != other.operation_) return false;
if (hasFilter() != other.hasFilter()) return false;
if (hasFilter()) {
if (!getFilter()
.equals(other.getFilter())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + OPERATION_FIELD_NUMBER;
hash = (53 * hash) + operation_;
if (hasFilter()) {
hash = (37 * hash) + FILTER_FIELD_NUMBER;
hash = (53 * hash) + getFilter().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.authzed.api.v1.PermissionService.Precondition parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.Precondition parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.Precondition parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.Precondition parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.Precondition parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.Precondition parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.Precondition parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.Precondition parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.Precondition parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.Precondition parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.Precondition parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.Precondition parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.authzed.api.v1.PermissionService.Precondition prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Precondition specifies how and the existence or absence of certain
* relationships as expressed through the accompanying filter should affect
* whether or not the operation proceeds.
* MUST_NOT_MATCH will fail the parent request if any relationships match the
* relationships filter.
* MUST_MATCH will fail the parent request if there are no
* relationships that match the filter.
*
*
* Protobuf type {@code authzed.api.v1.Precondition}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:authzed.api.v1.Precondition)
com.authzed.api.v1.PermissionService.PreconditionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_Precondition_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_Precondition_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.PermissionService.Precondition.class, com.authzed.api.v1.PermissionService.Precondition.Builder.class);
}
// Construct using com.authzed.api.v1.PermissionService.Precondition.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
operation_ = 0;
filter_ = null;
if (filterBuilder_ != null) {
filterBuilder_.dispose();
filterBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_Precondition_descriptor;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.Precondition getDefaultInstanceForType() {
return com.authzed.api.v1.PermissionService.Precondition.getDefaultInstance();
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.Precondition build() {
com.authzed.api.v1.PermissionService.Precondition result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.Precondition buildPartial() {
com.authzed.api.v1.PermissionService.Precondition result = new com.authzed.api.v1.PermissionService.Precondition(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.authzed.api.v1.PermissionService.Precondition result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.operation_ = operation_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.filter_ = filterBuilder_ == null
? filter_
: filterBuilder_.build();
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.authzed.api.v1.PermissionService.Precondition) {
return mergeFrom((com.authzed.api.v1.PermissionService.Precondition)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.authzed.api.v1.PermissionService.Precondition other) {
if (other == com.authzed.api.v1.PermissionService.Precondition.getDefaultInstance()) return this;
if (other.operation_ != 0) {
setOperationValue(other.getOperationValue());
}
if (other.hasFilter()) {
mergeFilter(other.getFilter());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
operation_ = input.readEnum();
bitField0_ |= 0x00000001;
break;
} // case 8
case 18: {
input.readMessage(
getFilterFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000002;
break;
} // case 18
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int operation_ = 0;
/**
* .authzed.api.v1.Precondition.Operation operation = 1 [(.validate.rules) = { ... }
* @return The enum numeric value on the wire for operation.
*/
@java.lang.Override public int getOperationValue() {
return operation_;
}
/**
* .authzed.api.v1.Precondition.Operation operation = 1 [(.validate.rules) = { ... }
* @param value The enum numeric value on the wire for operation to set.
* @return This builder for chaining.
*/
public Builder setOperationValue(int value) {
operation_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .authzed.api.v1.Precondition.Operation operation = 1 [(.validate.rules) = { ... }
* @return The operation.
*/
@java.lang.Override
public com.authzed.api.v1.PermissionService.Precondition.Operation getOperation() {
com.authzed.api.v1.PermissionService.Precondition.Operation result = com.authzed.api.v1.PermissionService.Precondition.Operation.forNumber(operation_);
return result == null ? com.authzed.api.v1.PermissionService.Precondition.Operation.UNRECOGNIZED : result;
}
/**
* .authzed.api.v1.Precondition.Operation operation = 1 [(.validate.rules) = { ... }
* @param value The operation to set.
* @return This builder for chaining.
*/
public Builder setOperation(com.authzed.api.v1.PermissionService.Precondition.Operation value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
operation_ = value.getNumber();
onChanged();
return this;
}
/**
* .authzed.api.v1.Precondition.Operation operation = 1 [(.validate.rules) = { ... }
* @return This builder for chaining.
*/
public Builder clearOperation() {
bitField0_ = (bitField0_ & ~0x00000001);
operation_ = 0;
onChanged();
return this;
}
private com.authzed.api.v1.PermissionService.RelationshipFilter filter_;
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.PermissionService.RelationshipFilter, com.authzed.api.v1.PermissionService.RelationshipFilter.Builder, com.authzed.api.v1.PermissionService.RelationshipFilterOrBuilder> filterBuilder_;
/**
* .authzed.api.v1.RelationshipFilter filter = 2 [(.validate.rules) = { ... }
* @return Whether the filter field is set.
*/
public boolean hasFilter() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* .authzed.api.v1.RelationshipFilter filter = 2 [(.validate.rules) = { ... }
* @return The filter.
*/
public com.authzed.api.v1.PermissionService.RelationshipFilter getFilter() {
if (filterBuilder_ == null) {
return filter_ == null ? com.authzed.api.v1.PermissionService.RelationshipFilter.getDefaultInstance() : filter_;
} else {
return filterBuilder_.getMessage();
}
}
/**
* .authzed.api.v1.RelationshipFilter filter = 2 [(.validate.rules) = { ... }
*/
public Builder setFilter(com.authzed.api.v1.PermissionService.RelationshipFilter value) {
if (filterBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
filter_ = value;
} else {
filterBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* .authzed.api.v1.RelationshipFilter filter = 2 [(.validate.rules) = { ... }
*/
public Builder setFilter(
com.authzed.api.v1.PermissionService.RelationshipFilter.Builder builderForValue) {
if (filterBuilder_ == null) {
filter_ = builderForValue.build();
} else {
filterBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* .authzed.api.v1.RelationshipFilter filter = 2 [(.validate.rules) = { ... }
*/
public Builder mergeFilter(com.authzed.api.v1.PermissionService.RelationshipFilter value) {
if (filterBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0) &&
filter_ != null &&
filter_ != com.authzed.api.v1.PermissionService.RelationshipFilter.getDefaultInstance()) {
getFilterBuilder().mergeFrom(value);
} else {
filter_ = value;
}
} else {
filterBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* .authzed.api.v1.RelationshipFilter filter = 2 [(.validate.rules) = { ... }
*/
public Builder clearFilter() {
bitField0_ = (bitField0_ & ~0x00000002);
filter_ = null;
if (filterBuilder_ != null) {
filterBuilder_.dispose();
filterBuilder_ = null;
}
onChanged();
return this;
}
/**
* .authzed.api.v1.RelationshipFilter filter = 2 [(.validate.rules) = { ... }
*/
public com.authzed.api.v1.PermissionService.RelationshipFilter.Builder getFilterBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getFilterFieldBuilder().getBuilder();
}
/**
* .authzed.api.v1.RelationshipFilter filter = 2 [(.validate.rules) = { ... }
*/
public com.authzed.api.v1.PermissionService.RelationshipFilterOrBuilder getFilterOrBuilder() {
if (filterBuilder_ != null) {
return filterBuilder_.getMessageOrBuilder();
} else {
return filter_ == null ?
com.authzed.api.v1.PermissionService.RelationshipFilter.getDefaultInstance() : filter_;
}
}
/**
* .authzed.api.v1.RelationshipFilter filter = 2 [(.validate.rules) = { ... }
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.PermissionService.RelationshipFilter, com.authzed.api.v1.PermissionService.RelationshipFilter.Builder, com.authzed.api.v1.PermissionService.RelationshipFilterOrBuilder>
getFilterFieldBuilder() {
if (filterBuilder_ == null) {
filterBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.PermissionService.RelationshipFilter, com.authzed.api.v1.PermissionService.RelationshipFilter.Builder, com.authzed.api.v1.PermissionService.RelationshipFilterOrBuilder>(
getFilter(),
getParentForChildren(),
isClean());
filter_ = null;
}
return filterBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:authzed.api.v1.Precondition)
}
// @@protoc_insertion_point(class_scope:authzed.api.v1.Precondition)
private static final com.authzed.api.v1.PermissionService.Precondition DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.authzed.api.v1.PermissionService.Precondition();
}
public static com.authzed.api.v1.PermissionService.Precondition getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Precondition parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.Precondition getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface WriteRelationshipsRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:authzed.api.v1.WriteRelationshipsRequest)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .authzed.api.v1.RelationshipUpdate updates = 1 [(.validate.rules) = { ... }
*/
java.util.List
getUpdatesList();
/**
* repeated .authzed.api.v1.RelationshipUpdate updates = 1 [(.validate.rules) = { ... }
*/
com.authzed.api.v1.Core.RelationshipUpdate getUpdates(int index);
/**
* repeated .authzed.api.v1.RelationshipUpdate updates = 1 [(.validate.rules) = { ... }
*/
int getUpdatesCount();
/**
* repeated .authzed.api.v1.RelationshipUpdate updates = 1 [(.validate.rules) = { ... }
*/
java.util.List extends com.authzed.api.v1.Core.RelationshipUpdateOrBuilder>
getUpdatesOrBuilderList();
/**
* repeated .authzed.api.v1.RelationshipUpdate updates = 1 [(.validate.rules) = { ... }
*/
com.authzed.api.v1.Core.RelationshipUpdateOrBuilder getUpdatesOrBuilder(
int index);
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
java.util.List
getOptionalPreconditionsList();
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
com.authzed.api.v1.PermissionService.Precondition getOptionalPreconditions(int index);
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
int getOptionalPreconditionsCount();
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
java.util.List extends com.authzed.api.v1.PermissionService.PreconditionOrBuilder>
getOptionalPreconditionsOrBuilderList();
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
com.authzed.api.v1.PermissionService.PreconditionOrBuilder getOptionalPreconditionsOrBuilder(
int index);
}
/**
*
* WriteRelationshipsRequest contains a list of Relationship mutations that
* should be applied to the service. If the optional_preconditions parameter
* is included, all of the specified preconditions must also be satisfied before
* the write will be committed.
*
*
* Protobuf type {@code authzed.api.v1.WriteRelationshipsRequest}
*/
public static final class WriteRelationshipsRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:authzed.api.v1.WriteRelationshipsRequest)
WriteRelationshipsRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use WriteRelationshipsRequest.newBuilder() to construct.
private WriteRelationshipsRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private WriteRelationshipsRequest() {
updates_ = java.util.Collections.emptyList();
optionalPreconditions_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new WriteRelationshipsRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_WriteRelationshipsRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_WriteRelationshipsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.PermissionService.WriteRelationshipsRequest.class, com.authzed.api.v1.PermissionService.WriteRelationshipsRequest.Builder.class);
}
public static final int UPDATES_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private java.util.List updates_;
/**
* repeated .authzed.api.v1.RelationshipUpdate updates = 1 [(.validate.rules) = { ... }
*/
@java.lang.Override
public java.util.List getUpdatesList() {
return updates_;
}
/**
* repeated .authzed.api.v1.RelationshipUpdate updates = 1 [(.validate.rules) = { ... }
*/
@java.lang.Override
public java.util.List extends com.authzed.api.v1.Core.RelationshipUpdateOrBuilder>
getUpdatesOrBuilderList() {
return updates_;
}
/**
* repeated .authzed.api.v1.RelationshipUpdate updates = 1 [(.validate.rules) = { ... }
*/
@java.lang.Override
public int getUpdatesCount() {
return updates_.size();
}
/**
* repeated .authzed.api.v1.RelationshipUpdate updates = 1 [(.validate.rules) = { ... }
*/
@java.lang.Override
public com.authzed.api.v1.Core.RelationshipUpdate getUpdates(int index) {
return updates_.get(index);
}
/**
* repeated .authzed.api.v1.RelationshipUpdate updates = 1 [(.validate.rules) = { ... }
*/
@java.lang.Override
public com.authzed.api.v1.Core.RelationshipUpdateOrBuilder getUpdatesOrBuilder(
int index) {
return updates_.get(index);
}
public static final int OPTIONAL_PRECONDITIONS_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private java.util.List optionalPreconditions_;
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
@java.lang.Override
public java.util.List getOptionalPreconditionsList() {
return optionalPreconditions_;
}
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
@java.lang.Override
public java.util.List extends com.authzed.api.v1.PermissionService.PreconditionOrBuilder>
getOptionalPreconditionsOrBuilderList() {
return optionalPreconditions_;
}
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
@java.lang.Override
public int getOptionalPreconditionsCount() {
return optionalPreconditions_.size();
}
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
@java.lang.Override
public com.authzed.api.v1.PermissionService.Precondition getOptionalPreconditions(int index) {
return optionalPreconditions_.get(index);
}
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
@java.lang.Override
public com.authzed.api.v1.PermissionService.PreconditionOrBuilder getOptionalPreconditionsOrBuilder(
int index) {
return optionalPreconditions_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < updates_.size(); i++) {
output.writeMessage(1, updates_.get(i));
}
for (int i = 0; i < optionalPreconditions_.size(); i++) {
output.writeMessage(2, optionalPreconditions_.get(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < updates_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, updates_.get(i));
}
for (int i = 0; i < optionalPreconditions_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, optionalPreconditions_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.authzed.api.v1.PermissionService.WriteRelationshipsRequest)) {
return super.equals(obj);
}
com.authzed.api.v1.PermissionService.WriteRelationshipsRequest other = (com.authzed.api.v1.PermissionService.WriteRelationshipsRequest) obj;
if (!getUpdatesList()
.equals(other.getUpdatesList())) return false;
if (!getOptionalPreconditionsList()
.equals(other.getOptionalPreconditionsList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getUpdatesCount() > 0) {
hash = (37 * hash) + UPDATES_FIELD_NUMBER;
hash = (53 * hash) + getUpdatesList().hashCode();
}
if (getOptionalPreconditionsCount() > 0) {
hash = (37 * hash) + OPTIONAL_PRECONDITIONS_FIELD_NUMBER;
hash = (53 * hash) + getOptionalPreconditionsList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.authzed.api.v1.PermissionService.WriteRelationshipsRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.WriteRelationshipsRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.WriteRelationshipsRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.WriteRelationshipsRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.WriteRelationshipsRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.WriteRelationshipsRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.WriteRelationshipsRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.WriteRelationshipsRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.WriteRelationshipsRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.WriteRelationshipsRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.WriteRelationshipsRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.WriteRelationshipsRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.authzed.api.v1.PermissionService.WriteRelationshipsRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* WriteRelationshipsRequest contains a list of Relationship mutations that
* should be applied to the service. If the optional_preconditions parameter
* is included, all of the specified preconditions must also be satisfied before
* the write will be committed.
*
*
* Protobuf type {@code authzed.api.v1.WriteRelationshipsRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:authzed.api.v1.WriteRelationshipsRequest)
com.authzed.api.v1.PermissionService.WriteRelationshipsRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_WriteRelationshipsRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_WriteRelationshipsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.PermissionService.WriteRelationshipsRequest.class, com.authzed.api.v1.PermissionService.WriteRelationshipsRequest.Builder.class);
}
// Construct using com.authzed.api.v1.PermissionService.WriteRelationshipsRequest.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
if (updatesBuilder_ == null) {
updates_ = java.util.Collections.emptyList();
} else {
updates_ = null;
updatesBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
if (optionalPreconditionsBuilder_ == null) {
optionalPreconditions_ = java.util.Collections.emptyList();
} else {
optionalPreconditions_ = null;
optionalPreconditionsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_WriteRelationshipsRequest_descriptor;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.WriteRelationshipsRequest getDefaultInstanceForType() {
return com.authzed.api.v1.PermissionService.WriteRelationshipsRequest.getDefaultInstance();
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.WriteRelationshipsRequest build() {
com.authzed.api.v1.PermissionService.WriteRelationshipsRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.WriteRelationshipsRequest buildPartial() {
com.authzed.api.v1.PermissionService.WriteRelationshipsRequest result = new com.authzed.api.v1.PermissionService.WriteRelationshipsRequest(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.authzed.api.v1.PermissionService.WriteRelationshipsRequest result) {
if (updatesBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
updates_ = java.util.Collections.unmodifiableList(updates_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.updates_ = updates_;
} else {
result.updates_ = updatesBuilder_.build();
}
if (optionalPreconditionsBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0)) {
optionalPreconditions_ = java.util.Collections.unmodifiableList(optionalPreconditions_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.optionalPreconditions_ = optionalPreconditions_;
} else {
result.optionalPreconditions_ = optionalPreconditionsBuilder_.build();
}
}
private void buildPartial0(com.authzed.api.v1.PermissionService.WriteRelationshipsRequest result) {
int from_bitField0_ = bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.authzed.api.v1.PermissionService.WriteRelationshipsRequest) {
return mergeFrom((com.authzed.api.v1.PermissionService.WriteRelationshipsRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.authzed.api.v1.PermissionService.WriteRelationshipsRequest other) {
if (other == com.authzed.api.v1.PermissionService.WriteRelationshipsRequest.getDefaultInstance()) return this;
if (updatesBuilder_ == null) {
if (!other.updates_.isEmpty()) {
if (updates_.isEmpty()) {
updates_ = other.updates_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureUpdatesIsMutable();
updates_.addAll(other.updates_);
}
onChanged();
}
} else {
if (!other.updates_.isEmpty()) {
if (updatesBuilder_.isEmpty()) {
updatesBuilder_.dispose();
updatesBuilder_ = null;
updates_ = other.updates_;
bitField0_ = (bitField0_ & ~0x00000001);
updatesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getUpdatesFieldBuilder() : null;
} else {
updatesBuilder_.addAllMessages(other.updates_);
}
}
}
if (optionalPreconditionsBuilder_ == null) {
if (!other.optionalPreconditions_.isEmpty()) {
if (optionalPreconditions_.isEmpty()) {
optionalPreconditions_ = other.optionalPreconditions_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureOptionalPreconditionsIsMutable();
optionalPreconditions_.addAll(other.optionalPreconditions_);
}
onChanged();
}
} else {
if (!other.optionalPreconditions_.isEmpty()) {
if (optionalPreconditionsBuilder_.isEmpty()) {
optionalPreconditionsBuilder_.dispose();
optionalPreconditionsBuilder_ = null;
optionalPreconditions_ = other.optionalPreconditions_;
bitField0_ = (bitField0_ & ~0x00000002);
optionalPreconditionsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getOptionalPreconditionsFieldBuilder() : null;
} else {
optionalPreconditionsBuilder_.addAllMessages(other.optionalPreconditions_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.authzed.api.v1.Core.RelationshipUpdate m =
input.readMessage(
com.authzed.api.v1.Core.RelationshipUpdate.parser(),
extensionRegistry);
if (updatesBuilder_ == null) {
ensureUpdatesIsMutable();
updates_.add(m);
} else {
updatesBuilder_.addMessage(m);
}
break;
} // case 10
case 18: {
com.authzed.api.v1.PermissionService.Precondition m =
input.readMessage(
com.authzed.api.v1.PermissionService.Precondition.parser(),
extensionRegistry);
if (optionalPreconditionsBuilder_ == null) {
ensureOptionalPreconditionsIsMutable();
optionalPreconditions_.add(m);
} else {
optionalPreconditionsBuilder_.addMessage(m);
}
break;
} // case 18
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.util.List updates_ =
java.util.Collections.emptyList();
private void ensureUpdatesIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
updates_ = new java.util.ArrayList(updates_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.authzed.api.v1.Core.RelationshipUpdate, com.authzed.api.v1.Core.RelationshipUpdate.Builder, com.authzed.api.v1.Core.RelationshipUpdateOrBuilder> updatesBuilder_;
/**
* repeated .authzed.api.v1.RelationshipUpdate updates = 1 [(.validate.rules) = { ... }
*/
public java.util.List getUpdatesList() {
if (updatesBuilder_ == null) {
return java.util.Collections.unmodifiableList(updates_);
} else {
return updatesBuilder_.getMessageList();
}
}
/**
* repeated .authzed.api.v1.RelationshipUpdate updates = 1 [(.validate.rules) = { ... }
*/
public int getUpdatesCount() {
if (updatesBuilder_ == null) {
return updates_.size();
} else {
return updatesBuilder_.getCount();
}
}
/**
* repeated .authzed.api.v1.RelationshipUpdate updates = 1 [(.validate.rules) = { ... }
*/
public com.authzed.api.v1.Core.RelationshipUpdate getUpdates(int index) {
if (updatesBuilder_ == null) {
return updates_.get(index);
} else {
return updatesBuilder_.getMessage(index);
}
}
/**
* repeated .authzed.api.v1.RelationshipUpdate updates = 1 [(.validate.rules) = { ... }
*/
public Builder setUpdates(
int index, com.authzed.api.v1.Core.RelationshipUpdate value) {
if (updatesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureUpdatesIsMutable();
updates_.set(index, value);
onChanged();
} else {
updatesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .authzed.api.v1.RelationshipUpdate updates = 1 [(.validate.rules) = { ... }
*/
public Builder setUpdates(
int index, com.authzed.api.v1.Core.RelationshipUpdate.Builder builderForValue) {
if (updatesBuilder_ == null) {
ensureUpdatesIsMutable();
updates_.set(index, builderForValue.build());
onChanged();
} else {
updatesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .authzed.api.v1.RelationshipUpdate updates = 1 [(.validate.rules) = { ... }
*/
public Builder addUpdates(com.authzed.api.v1.Core.RelationshipUpdate value) {
if (updatesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureUpdatesIsMutable();
updates_.add(value);
onChanged();
} else {
updatesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .authzed.api.v1.RelationshipUpdate updates = 1 [(.validate.rules) = { ... }
*/
public Builder addUpdates(
int index, com.authzed.api.v1.Core.RelationshipUpdate value) {
if (updatesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureUpdatesIsMutable();
updates_.add(index, value);
onChanged();
} else {
updatesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .authzed.api.v1.RelationshipUpdate updates = 1 [(.validate.rules) = { ... }
*/
public Builder addUpdates(
com.authzed.api.v1.Core.RelationshipUpdate.Builder builderForValue) {
if (updatesBuilder_ == null) {
ensureUpdatesIsMutable();
updates_.add(builderForValue.build());
onChanged();
} else {
updatesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .authzed.api.v1.RelationshipUpdate updates = 1 [(.validate.rules) = { ... }
*/
public Builder addUpdates(
int index, com.authzed.api.v1.Core.RelationshipUpdate.Builder builderForValue) {
if (updatesBuilder_ == null) {
ensureUpdatesIsMutable();
updates_.add(index, builderForValue.build());
onChanged();
} else {
updatesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .authzed.api.v1.RelationshipUpdate updates = 1 [(.validate.rules) = { ... }
*/
public Builder addAllUpdates(
java.lang.Iterable extends com.authzed.api.v1.Core.RelationshipUpdate> values) {
if (updatesBuilder_ == null) {
ensureUpdatesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, updates_);
onChanged();
} else {
updatesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .authzed.api.v1.RelationshipUpdate updates = 1 [(.validate.rules) = { ... }
*/
public Builder clearUpdates() {
if (updatesBuilder_ == null) {
updates_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
updatesBuilder_.clear();
}
return this;
}
/**
* repeated .authzed.api.v1.RelationshipUpdate updates = 1 [(.validate.rules) = { ... }
*/
public Builder removeUpdates(int index) {
if (updatesBuilder_ == null) {
ensureUpdatesIsMutable();
updates_.remove(index);
onChanged();
} else {
updatesBuilder_.remove(index);
}
return this;
}
/**
* repeated .authzed.api.v1.RelationshipUpdate updates = 1 [(.validate.rules) = { ... }
*/
public com.authzed.api.v1.Core.RelationshipUpdate.Builder getUpdatesBuilder(
int index) {
return getUpdatesFieldBuilder().getBuilder(index);
}
/**
* repeated .authzed.api.v1.RelationshipUpdate updates = 1 [(.validate.rules) = { ... }
*/
public com.authzed.api.v1.Core.RelationshipUpdateOrBuilder getUpdatesOrBuilder(
int index) {
if (updatesBuilder_ == null) {
return updates_.get(index); } else {
return updatesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .authzed.api.v1.RelationshipUpdate updates = 1 [(.validate.rules) = { ... }
*/
public java.util.List extends com.authzed.api.v1.Core.RelationshipUpdateOrBuilder>
getUpdatesOrBuilderList() {
if (updatesBuilder_ != null) {
return updatesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(updates_);
}
}
/**
* repeated .authzed.api.v1.RelationshipUpdate updates = 1 [(.validate.rules) = { ... }
*/
public com.authzed.api.v1.Core.RelationshipUpdate.Builder addUpdatesBuilder() {
return getUpdatesFieldBuilder().addBuilder(
com.authzed.api.v1.Core.RelationshipUpdate.getDefaultInstance());
}
/**
* repeated .authzed.api.v1.RelationshipUpdate updates = 1 [(.validate.rules) = { ... }
*/
public com.authzed.api.v1.Core.RelationshipUpdate.Builder addUpdatesBuilder(
int index) {
return getUpdatesFieldBuilder().addBuilder(
index, com.authzed.api.v1.Core.RelationshipUpdate.getDefaultInstance());
}
/**
* repeated .authzed.api.v1.RelationshipUpdate updates = 1 [(.validate.rules) = { ... }
*/
public java.util.List
getUpdatesBuilderList() {
return getUpdatesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.authzed.api.v1.Core.RelationshipUpdate, com.authzed.api.v1.Core.RelationshipUpdate.Builder, com.authzed.api.v1.Core.RelationshipUpdateOrBuilder>
getUpdatesFieldBuilder() {
if (updatesBuilder_ == null) {
updatesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.authzed.api.v1.Core.RelationshipUpdate, com.authzed.api.v1.Core.RelationshipUpdate.Builder, com.authzed.api.v1.Core.RelationshipUpdateOrBuilder>(
updates_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
updates_ = null;
}
return updatesBuilder_;
}
private java.util.List optionalPreconditions_ =
java.util.Collections.emptyList();
private void ensureOptionalPreconditionsIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
optionalPreconditions_ = new java.util.ArrayList(optionalPreconditions_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.authzed.api.v1.PermissionService.Precondition, com.authzed.api.v1.PermissionService.Precondition.Builder, com.authzed.api.v1.PermissionService.PreconditionOrBuilder> optionalPreconditionsBuilder_;
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
public java.util.List getOptionalPreconditionsList() {
if (optionalPreconditionsBuilder_ == null) {
return java.util.Collections.unmodifiableList(optionalPreconditions_);
} else {
return optionalPreconditionsBuilder_.getMessageList();
}
}
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
public int getOptionalPreconditionsCount() {
if (optionalPreconditionsBuilder_ == null) {
return optionalPreconditions_.size();
} else {
return optionalPreconditionsBuilder_.getCount();
}
}
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
public com.authzed.api.v1.PermissionService.Precondition getOptionalPreconditions(int index) {
if (optionalPreconditionsBuilder_ == null) {
return optionalPreconditions_.get(index);
} else {
return optionalPreconditionsBuilder_.getMessage(index);
}
}
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
public Builder setOptionalPreconditions(
int index, com.authzed.api.v1.PermissionService.Precondition value) {
if (optionalPreconditionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOptionalPreconditionsIsMutable();
optionalPreconditions_.set(index, value);
onChanged();
} else {
optionalPreconditionsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
public Builder setOptionalPreconditions(
int index, com.authzed.api.v1.PermissionService.Precondition.Builder builderForValue) {
if (optionalPreconditionsBuilder_ == null) {
ensureOptionalPreconditionsIsMutable();
optionalPreconditions_.set(index, builderForValue.build());
onChanged();
} else {
optionalPreconditionsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
public Builder addOptionalPreconditions(com.authzed.api.v1.PermissionService.Precondition value) {
if (optionalPreconditionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOptionalPreconditionsIsMutable();
optionalPreconditions_.add(value);
onChanged();
} else {
optionalPreconditionsBuilder_.addMessage(value);
}
return this;
}
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
public Builder addOptionalPreconditions(
int index, com.authzed.api.v1.PermissionService.Precondition value) {
if (optionalPreconditionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOptionalPreconditionsIsMutable();
optionalPreconditions_.add(index, value);
onChanged();
} else {
optionalPreconditionsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
public Builder addOptionalPreconditions(
com.authzed.api.v1.PermissionService.Precondition.Builder builderForValue) {
if (optionalPreconditionsBuilder_ == null) {
ensureOptionalPreconditionsIsMutable();
optionalPreconditions_.add(builderForValue.build());
onChanged();
} else {
optionalPreconditionsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
public Builder addOptionalPreconditions(
int index, com.authzed.api.v1.PermissionService.Precondition.Builder builderForValue) {
if (optionalPreconditionsBuilder_ == null) {
ensureOptionalPreconditionsIsMutable();
optionalPreconditions_.add(index, builderForValue.build());
onChanged();
} else {
optionalPreconditionsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
public Builder addAllOptionalPreconditions(
java.lang.Iterable extends com.authzed.api.v1.PermissionService.Precondition> values) {
if (optionalPreconditionsBuilder_ == null) {
ensureOptionalPreconditionsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, optionalPreconditions_);
onChanged();
} else {
optionalPreconditionsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
public Builder clearOptionalPreconditions() {
if (optionalPreconditionsBuilder_ == null) {
optionalPreconditions_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
optionalPreconditionsBuilder_.clear();
}
return this;
}
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
public Builder removeOptionalPreconditions(int index) {
if (optionalPreconditionsBuilder_ == null) {
ensureOptionalPreconditionsIsMutable();
optionalPreconditions_.remove(index);
onChanged();
} else {
optionalPreconditionsBuilder_.remove(index);
}
return this;
}
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
public com.authzed.api.v1.PermissionService.Precondition.Builder getOptionalPreconditionsBuilder(
int index) {
return getOptionalPreconditionsFieldBuilder().getBuilder(index);
}
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
public com.authzed.api.v1.PermissionService.PreconditionOrBuilder getOptionalPreconditionsOrBuilder(
int index) {
if (optionalPreconditionsBuilder_ == null) {
return optionalPreconditions_.get(index); } else {
return optionalPreconditionsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
public java.util.List extends com.authzed.api.v1.PermissionService.PreconditionOrBuilder>
getOptionalPreconditionsOrBuilderList() {
if (optionalPreconditionsBuilder_ != null) {
return optionalPreconditionsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(optionalPreconditions_);
}
}
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
public com.authzed.api.v1.PermissionService.Precondition.Builder addOptionalPreconditionsBuilder() {
return getOptionalPreconditionsFieldBuilder().addBuilder(
com.authzed.api.v1.PermissionService.Precondition.getDefaultInstance());
}
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
public com.authzed.api.v1.PermissionService.Precondition.Builder addOptionalPreconditionsBuilder(
int index) {
return getOptionalPreconditionsFieldBuilder().addBuilder(
index, com.authzed.api.v1.PermissionService.Precondition.getDefaultInstance());
}
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
public java.util.List
getOptionalPreconditionsBuilderList() {
return getOptionalPreconditionsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.authzed.api.v1.PermissionService.Precondition, com.authzed.api.v1.PermissionService.Precondition.Builder, com.authzed.api.v1.PermissionService.PreconditionOrBuilder>
getOptionalPreconditionsFieldBuilder() {
if (optionalPreconditionsBuilder_ == null) {
optionalPreconditionsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.authzed.api.v1.PermissionService.Precondition, com.authzed.api.v1.PermissionService.Precondition.Builder, com.authzed.api.v1.PermissionService.PreconditionOrBuilder>(
optionalPreconditions_,
((bitField0_ & 0x00000002) != 0),
getParentForChildren(),
isClean());
optionalPreconditions_ = null;
}
return optionalPreconditionsBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:authzed.api.v1.WriteRelationshipsRequest)
}
// @@protoc_insertion_point(class_scope:authzed.api.v1.WriteRelationshipsRequest)
private static final com.authzed.api.v1.PermissionService.WriteRelationshipsRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.authzed.api.v1.PermissionService.WriteRelationshipsRequest();
}
public static com.authzed.api.v1.PermissionService.WriteRelationshipsRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public WriteRelationshipsRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.WriteRelationshipsRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface WriteRelationshipsResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:authzed.api.v1.WriteRelationshipsResponse)
com.google.protobuf.MessageOrBuilder {
/**
* .authzed.api.v1.ZedToken written_at = 1;
* @return Whether the writtenAt field is set.
*/
boolean hasWrittenAt();
/**
* .authzed.api.v1.ZedToken written_at = 1;
* @return The writtenAt.
*/
com.authzed.api.v1.Core.ZedToken getWrittenAt();
/**
* .authzed.api.v1.ZedToken written_at = 1;
*/
com.authzed.api.v1.Core.ZedTokenOrBuilder getWrittenAtOrBuilder();
}
/**
* Protobuf type {@code authzed.api.v1.WriteRelationshipsResponse}
*/
public static final class WriteRelationshipsResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:authzed.api.v1.WriteRelationshipsResponse)
WriteRelationshipsResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use WriteRelationshipsResponse.newBuilder() to construct.
private WriteRelationshipsResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private WriteRelationshipsResponse() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new WriteRelationshipsResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_WriteRelationshipsResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_WriteRelationshipsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.PermissionService.WriteRelationshipsResponse.class, com.authzed.api.v1.PermissionService.WriteRelationshipsResponse.Builder.class);
}
public static final int WRITTEN_AT_FIELD_NUMBER = 1;
private com.authzed.api.v1.Core.ZedToken writtenAt_;
/**
* .authzed.api.v1.ZedToken written_at = 1;
* @return Whether the writtenAt field is set.
*/
@java.lang.Override
public boolean hasWrittenAt() {
return writtenAt_ != null;
}
/**
* .authzed.api.v1.ZedToken written_at = 1;
* @return The writtenAt.
*/
@java.lang.Override
public com.authzed.api.v1.Core.ZedToken getWrittenAt() {
return writtenAt_ == null ? com.authzed.api.v1.Core.ZedToken.getDefaultInstance() : writtenAt_;
}
/**
* .authzed.api.v1.ZedToken written_at = 1;
*/
@java.lang.Override
public com.authzed.api.v1.Core.ZedTokenOrBuilder getWrittenAtOrBuilder() {
return writtenAt_ == null ? com.authzed.api.v1.Core.ZedToken.getDefaultInstance() : writtenAt_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (writtenAt_ != null) {
output.writeMessage(1, getWrittenAt());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (writtenAt_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getWrittenAt());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.authzed.api.v1.PermissionService.WriteRelationshipsResponse)) {
return super.equals(obj);
}
com.authzed.api.v1.PermissionService.WriteRelationshipsResponse other = (com.authzed.api.v1.PermissionService.WriteRelationshipsResponse) obj;
if (hasWrittenAt() != other.hasWrittenAt()) return false;
if (hasWrittenAt()) {
if (!getWrittenAt()
.equals(other.getWrittenAt())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasWrittenAt()) {
hash = (37 * hash) + WRITTEN_AT_FIELD_NUMBER;
hash = (53 * hash) + getWrittenAt().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.authzed.api.v1.PermissionService.WriteRelationshipsResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.WriteRelationshipsResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.WriteRelationshipsResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.WriteRelationshipsResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.WriteRelationshipsResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.WriteRelationshipsResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.WriteRelationshipsResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.WriteRelationshipsResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.WriteRelationshipsResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.WriteRelationshipsResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.WriteRelationshipsResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.WriteRelationshipsResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.authzed.api.v1.PermissionService.WriteRelationshipsResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code authzed.api.v1.WriteRelationshipsResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:authzed.api.v1.WriteRelationshipsResponse)
com.authzed.api.v1.PermissionService.WriteRelationshipsResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_WriteRelationshipsResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_WriteRelationshipsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.PermissionService.WriteRelationshipsResponse.class, com.authzed.api.v1.PermissionService.WriteRelationshipsResponse.Builder.class);
}
// Construct using com.authzed.api.v1.PermissionService.WriteRelationshipsResponse.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
writtenAt_ = null;
if (writtenAtBuilder_ != null) {
writtenAtBuilder_.dispose();
writtenAtBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_WriteRelationshipsResponse_descriptor;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.WriteRelationshipsResponse getDefaultInstanceForType() {
return com.authzed.api.v1.PermissionService.WriteRelationshipsResponse.getDefaultInstance();
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.WriteRelationshipsResponse build() {
com.authzed.api.v1.PermissionService.WriteRelationshipsResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.WriteRelationshipsResponse buildPartial() {
com.authzed.api.v1.PermissionService.WriteRelationshipsResponse result = new com.authzed.api.v1.PermissionService.WriteRelationshipsResponse(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.authzed.api.v1.PermissionService.WriteRelationshipsResponse result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.writtenAt_ = writtenAtBuilder_ == null
? writtenAt_
: writtenAtBuilder_.build();
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.authzed.api.v1.PermissionService.WriteRelationshipsResponse) {
return mergeFrom((com.authzed.api.v1.PermissionService.WriteRelationshipsResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.authzed.api.v1.PermissionService.WriteRelationshipsResponse other) {
if (other == com.authzed.api.v1.PermissionService.WriteRelationshipsResponse.getDefaultInstance()) return this;
if (other.hasWrittenAt()) {
mergeWrittenAt(other.getWrittenAt());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
input.readMessage(
getWrittenAtFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case 10
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.authzed.api.v1.Core.ZedToken writtenAt_;
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.ZedToken, com.authzed.api.v1.Core.ZedToken.Builder, com.authzed.api.v1.Core.ZedTokenOrBuilder> writtenAtBuilder_;
/**
* .authzed.api.v1.ZedToken written_at = 1;
* @return Whether the writtenAt field is set.
*/
public boolean hasWrittenAt() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* .authzed.api.v1.ZedToken written_at = 1;
* @return The writtenAt.
*/
public com.authzed.api.v1.Core.ZedToken getWrittenAt() {
if (writtenAtBuilder_ == null) {
return writtenAt_ == null ? com.authzed.api.v1.Core.ZedToken.getDefaultInstance() : writtenAt_;
} else {
return writtenAtBuilder_.getMessage();
}
}
/**
* .authzed.api.v1.ZedToken written_at = 1;
*/
public Builder setWrittenAt(com.authzed.api.v1.Core.ZedToken value) {
if (writtenAtBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
writtenAt_ = value;
} else {
writtenAtBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .authzed.api.v1.ZedToken written_at = 1;
*/
public Builder setWrittenAt(
com.authzed.api.v1.Core.ZedToken.Builder builderForValue) {
if (writtenAtBuilder_ == null) {
writtenAt_ = builderForValue.build();
} else {
writtenAtBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .authzed.api.v1.ZedToken written_at = 1;
*/
public Builder mergeWrittenAt(com.authzed.api.v1.Core.ZedToken value) {
if (writtenAtBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0) &&
writtenAt_ != null &&
writtenAt_ != com.authzed.api.v1.Core.ZedToken.getDefaultInstance()) {
getWrittenAtBuilder().mergeFrom(value);
} else {
writtenAt_ = value;
}
} else {
writtenAtBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .authzed.api.v1.ZedToken written_at = 1;
*/
public Builder clearWrittenAt() {
bitField0_ = (bitField0_ & ~0x00000001);
writtenAt_ = null;
if (writtenAtBuilder_ != null) {
writtenAtBuilder_.dispose();
writtenAtBuilder_ = null;
}
onChanged();
return this;
}
/**
* .authzed.api.v1.ZedToken written_at = 1;
*/
public com.authzed.api.v1.Core.ZedToken.Builder getWrittenAtBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getWrittenAtFieldBuilder().getBuilder();
}
/**
* .authzed.api.v1.ZedToken written_at = 1;
*/
public com.authzed.api.v1.Core.ZedTokenOrBuilder getWrittenAtOrBuilder() {
if (writtenAtBuilder_ != null) {
return writtenAtBuilder_.getMessageOrBuilder();
} else {
return writtenAt_ == null ?
com.authzed.api.v1.Core.ZedToken.getDefaultInstance() : writtenAt_;
}
}
/**
* .authzed.api.v1.ZedToken written_at = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.ZedToken, com.authzed.api.v1.Core.ZedToken.Builder, com.authzed.api.v1.Core.ZedTokenOrBuilder>
getWrittenAtFieldBuilder() {
if (writtenAtBuilder_ == null) {
writtenAtBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.ZedToken, com.authzed.api.v1.Core.ZedToken.Builder, com.authzed.api.v1.Core.ZedTokenOrBuilder>(
getWrittenAt(),
getParentForChildren(),
isClean());
writtenAt_ = null;
}
return writtenAtBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:authzed.api.v1.WriteRelationshipsResponse)
}
// @@protoc_insertion_point(class_scope:authzed.api.v1.WriteRelationshipsResponse)
private static final com.authzed.api.v1.PermissionService.WriteRelationshipsResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.authzed.api.v1.PermissionService.WriteRelationshipsResponse();
}
public static com.authzed.api.v1.PermissionService.WriteRelationshipsResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public WriteRelationshipsResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.WriteRelationshipsResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface DeleteRelationshipsRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:authzed.api.v1.DeleteRelationshipsRequest)
com.google.protobuf.MessageOrBuilder {
/**
* .authzed.api.v1.RelationshipFilter relationship_filter = 1 [(.validate.rules) = { ... }
* @return Whether the relationshipFilter field is set.
*/
boolean hasRelationshipFilter();
/**
* .authzed.api.v1.RelationshipFilter relationship_filter = 1 [(.validate.rules) = { ... }
* @return The relationshipFilter.
*/
com.authzed.api.v1.PermissionService.RelationshipFilter getRelationshipFilter();
/**
* .authzed.api.v1.RelationshipFilter relationship_filter = 1 [(.validate.rules) = { ... }
*/
com.authzed.api.v1.PermissionService.RelationshipFilterOrBuilder getRelationshipFilterOrBuilder();
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
java.util.List
getOptionalPreconditionsList();
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
com.authzed.api.v1.PermissionService.Precondition getOptionalPreconditions(int index);
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
int getOptionalPreconditionsCount();
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
java.util.List extends com.authzed.api.v1.PermissionService.PreconditionOrBuilder>
getOptionalPreconditionsOrBuilderList();
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
com.authzed.api.v1.PermissionService.PreconditionOrBuilder getOptionalPreconditionsOrBuilder(
int index);
}
/**
*
* DeleteRelationshipsRequest specifies which Relationships should be deleted,
* requesting the delete of *ALL* relationships that match the specified
* filters. If the optional_preconditions parameter is included, all of the
* specified preconditions must also be satisfied before the delete will be
* executed.
*
*
* Protobuf type {@code authzed.api.v1.DeleteRelationshipsRequest}
*/
public static final class DeleteRelationshipsRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:authzed.api.v1.DeleteRelationshipsRequest)
DeleteRelationshipsRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use DeleteRelationshipsRequest.newBuilder() to construct.
private DeleteRelationshipsRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private DeleteRelationshipsRequest() {
optionalPreconditions_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new DeleteRelationshipsRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_DeleteRelationshipsRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_DeleteRelationshipsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.PermissionService.DeleteRelationshipsRequest.class, com.authzed.api.v1.PermissionService.DeleteRelationshipsRequest.Builder.class);
}
public static final int RELATIONSHIP_FILTER_FIELD_NUMBER = 1;
private com.authzed.api.v1.PermissionService.RelationshipFilter relationshipFilter_;
/**
* .authzed.api.v1.RelationshipFilter relationship_filter = 1 [(.validate.rules) = { ... }
* @return Whether the relationshipFilter field is set.
*/
@java.lang.Override
public boolean hasRelationshipFilter() {
return relationshipFilter_ != null;
}
/**
* .authzed.api.v1.RelationshipFilter relationship_filter = 1 [(.validate.rules) = { ... }
* @return The relationshipFilter.
*/
@java.lang.Override
public com.authzed.api.v1.PermissionService.RelationshipFilter getRelationshipFilter() {
return relationshipFilter_ == null ? com.authzed.api.v1.PermissionService.RelationshipFilter.getDefaultInstance() : relationshipFilter_;
}
/**
* .authzed.api.v1.RelationshipFilter relationship_filter = 1 [(.validate.rules) = { ... }
*/
@java.lang.Override
public com.authzed.api.v1.PermissionService.RelationshipFilterOrBuilder getRelationshipFilterOrBuilder() {
return relationshipFilter_ == null ? com.authzed.api.v1.PermissionService.RelationshipFilter.getDefaultInstance() : relationshipFilter_;
}
public static final int OPTIONAL_PRECONDITIONS_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private java.util.List optionalPreconditions_;
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
@java.lang.Override
public java.util.List getOptionalPreconditionsList() {
return optionalPreconditions_;
}
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
@java.lang.Override
public java.util.List extends com.authzed.api.v1.PermissionService.PreconditionOrBuilder>
getOptionalPreconditionsOrBuilderList() {
return optionalPreconditions_;
}
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
@java.lang.Override
public int getOptionalPreconditionsCount() {
return optionalPreconditions_.size();
}
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
@java.lang.Override
public com.authzed.api.v1.PermissionService.Precondition getOptionalPreconditions(int index) {
return optionalPreconditions_.get(index);
}
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
@java.lang.Override
public com.authzed.api.v1.PermissionService.PreconditionOrBuilder getOptionalPreconditionsOrBuilder(
int index) {
return optionalPreconditions_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (relationshipFilter_ != null) {
output.writeMessage(1, getRelationshipFilter());
}
for (int i = 0; i < optionalPreconditions_.size(); i++) {
output.writeMessage(2, optionalPreconditions_.get(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (relationshipFilter_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getRelationshipFilter());
}
for (int i = 0; i < optionalPreconditions_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, optionalPreconditions_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.authzed.api.v1.PermissionService.DeleteRelationshipsRequest)) {
return super.equals(obj);
}
com.authzed.api.v1.PermissionService.DeleteRelationshipsRequest other = (com.authzed.api.v1.PermissionService.DeleteRelationshipsRequest) obj;
if (hasRelationshipFilter() != other.hasRelationshipFilter()) return false;
if (hasRelationshipFilter()) {
if (!getRelationshipFilter()
.equals(other.getRelationshipFilter())) return false;
}
if (!getOptionalPreconditionsList()
.equals(other.getOptionalPreconditionsList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasRelationshipFilter()) {
hash = (37 * hash) + RELATIONSHIP_FILTER_FIELD_NUMBER;
hash = (53 * hash) + getRelationshipFilter().hashCode();
}
if (getOptionalPreconditionsCount() > 0) {
hash = (37 * hash) + OPTIONAL_PRECONDITIONS_FIELD_NUMBER;
hash = (53 * hash) + getOptionalPreconditionsList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.authzed.api.v1.PermissionService.DeleteRelationshipsRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.DeleteRelationshipsRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.DeleteRelationshipsRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.DeleteRelationshipsRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.DeleteRelationshipsRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.DeleteRelationshipsRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.DeleteRelationshipsRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.DeleteRelationshipsRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.DeleteRelationshipsRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.DeleteRelationshipsRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.DeleteRelationshipsRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.DeleteRelationshipsRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.authzed.api.v1.PermissionService.DeleteRelationshipsRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* DeleteRelationshipsRequest specifies which Relationships should be deleted,
* requesting the delete of *ALL* relationships that match the specified
* filters. If the optional_preconditions parameter is included, all of the
* specified preconditions must also be satisfied before the delete will be
* executed.
*
*
* Protobuf type {@code authzed.api.v1.DeleteRelationshipsRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:authzed.api.v1.DeleteRelationshipsRequest)
com.authzed.api.v1.PermissionService.DeleteRelationshipsRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_DeleteRelationshipsRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_DeleteRelationshipsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.PermissionService.DeleteRelationshipsRequest.class, com.authzed.api.v1.PermissionService.DeleteRelationshipsRequest.Builder.class);
}
// Construct using com.authzed.api.v1.PermissionService.DeleteRelationshipsRequest.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
relationshipFilter_ = null;
if (relationshipFilterBuilder_ != null) {
relationshipFilterBuilder_.dispose();
relationshipFilterBuilder_ = null;
}
if (optionalPreconditionsBuilder_ == null) {
optionalPreconditions_ = java.util.Collections.emptyList();
} else {
optionalPreconditions_ = null;
optionalPreconditionsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_DeleteRelationshipsRequest_descriptor;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.DeleteRelationshipsRequest getDefaultInstanceForType() {
return com.authzed.api.v1.PermissionService.DeleteRelationshipsRequest.getDefaultInstance();
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.DeleteRelationshipsRequest build() {
com.authzed.api.v1.PermissionService.DeleteRelationshipsRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.DeleteRelationshipsRequest buildPartial() {
com.authzed.api.v1.PermissionService.DeleteRelationshipsRequest result = new com.authzed.api.v1.PermissionService.DeleteRelationshipsRequest(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.authzed.api.v1.PermissionService.DeleteRelationshipsRequest result) {
if (optionalPreconditionsBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0)) {
optionalPreconditions_ = java.util.Collections.unmodifiableList(optionalPreconditions_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.optionalPreconditions_ = optionalPreconditions_;
} else {
result.optionalPreconditions_ = optionalPreconditionsBuilder_.build();
}
}
private void buildPartial0(com.authzed.api.v1.PermissionService.DeleteRelationshipsRequest result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.relationshipFilter_ = relationshipFilterBuilder_ == null
? relationshipFilter_
: relationshipFilterBuilder_.build();
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.authzed.api.v1.PermissionService.DeleteRelationshipsRequest) {
return mergeFrom((com.authzed.api.v1.PermissionService.DeleteRelationshipsRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.authzed.api.v1.PermissionService.DeleteRelationshipsRequest other) {
if (other == com.authzed.api.v1.PermissionService.DeleteRelationshipsRequest.getDefaultInstance()) return this;
if (other.hasRelationshipFilter()) {
mergeRelationshipFilter(other.getRelationshipFilter());
}
if (optionalPreconditionsBuilder_ == null) {
if (!other.optionalPreconditions_.isEmpty()) {
if (optionalPreconditions_.isEmpty()) {
optionalPreconditions_ = other.optionalPreconditions_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureOptionalPreconditionsIsMutable();
optionalPreconditions_.addAll(other.optionalPreconditions_);
}
onChanged();
}
} else {
if (!other.optionalPreconditions_.isEmpty()) {
if (optionalPreconditionsBuilder_.isEmpty()) {
optionalPreconditionsBuilder_.dispose();
optionalPreconditionsBuilder_ = null;
optionalPreconditions_ = other.optionalPreconditions_;
bitField0_ = (bitField0_ & ~0x00000002);
optionalPreconditionsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getOptionalPreconditionsFieldBuilder() : null;
} else {
optionalPreconditionsBuilder_.addAllMessages(other.optionalPreconditions_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
input.readMessage(
getRelationshipFilterFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
com.authzed.api.v1.PermissionService.Precondition m =
input.readMessage(
com.authzed.api.v1.PermissionService.Precondition.parser(),
extensionRegistry);
if (optionalPreconditionsBuilder_ == null) {
ensureOptionalPreconditionsIsMutable();
optionalPreconditions_.add(m);
} else {
optionalPreconditionsBuilder_.addMessage(m);
}
break;
} // case 18
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.authzed.api.v1.PermissionService.RelationshipFilter relationshipFilter_;
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.PermissionService.RelationshipFilter, com.authzed.api.v1.PermissionService.RelationshipFilter.Builder, com.authzed.api.v1.PermissionService.RelationshipFilterOrBuilder> relationshipFilterBuilder_;
/**
* .authzed.api.v1.RelationshipFilter relationship_filter = 1 [(.validate.rules) = { ... }
* @return Whether the relationshipFilter field is set.
*/
public boolean hasRelationshipFilter() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* .authzed.api.v1.RelationshipFilter relationship_filter = 1 [(.validate.rules) = { ... }
* @return The relationshipFilter.
*/
public com.authzed.api.v1.PermissionService.RelationshipFilter getRelationshipFilter() {
if (relationshipFilterBuilder_ == null) {
return relationshipFilter_ == null ? com.authzed.api.v1.PermissionService.RelationshipFilter.getDefaultInstance() : relationshipFilter_;
} else {
return relationshipFilterBuilder_.getMessage();
}
}
/**
* .authzed.api.v1.RelationshipFilter relationship_filter = 1 [(.validate.rules) = { ... }
*/
public Builder setRelationshipFilter(com.authzed.api.v1.PermissionService.RelationshipFilter value) {
if (relationshipFilterBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
relationshipFilter_ = value;
} else {
relationshipFilterBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .authzed.api.v1.RelationshipFilter relationship_filter = 1 [(.validate.rules) = { ... }
*/
public Builder setRelationshipFilter(
com.authzed.api.v1.PermissionService.RelationshipFilter.Builder builderForValue) {
if (relationshipFilterBuilder_ == null) {
relationshipFilter_ = builderForValue.build();
} else {
relationshipFilterBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .authzed.api.v1.RelationshipFilter relationship_filter = 1 [(.validate.rules) = { ... }
*/
public Builder mergeRelationshipFilter(com.authzed.api.v1.PermissionService.RelationshipFilter value) {
if (relationshipFilterBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0) &&
relationshipFilter_ != null &&
relationshipFilter_ != com.authzed.api.v1.PermissionService.RelationshipFilter.getDefaultInstance()) {
getRelationshipFilterBuilder().mergeFrom(value);
} else {
relationshipFilter_ = value;
}
} else {
relationshipFilterBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .authzed.api.v1.RelationshipFilter relationship_filter = 1 [(.validate.rules) = { ... }
*/
public Builder clearRelationshipFilter() {
bitField0_ = (bitField0_ & ~0x00000001);
relationshipFilter_ = null;
if (relationshipFilterBuilder_ != null) {
relationshipFilterBuilder_.dispose();
relationshipFilterBuilder_ = null;
}
onChanged();
return this;
}
/**
* .authzed.api.v1.RelationshipFilter relationship_filter = 1 [(.validate.rules) = { ... }
*/
public com.authzed.api.v1.PermissionService.RelationshipFilter.Builder getRelationshipFilterBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getRelationshipFilterFieldBuilder().getBuilder();
}
/**
* .authzed.api.v1.RelationshipFilter relationship_filter = 1 [(.validate.rules) = { ... }
*/
public com.authzed.api.v1.PermissionService.RelationshipFilterOrBuilder getRelationshipFilterOrBuilder() {
if (relationshipFilterBuilder_ != null) {
return relationshipFilterBuilder_.getMessageOrBuilder();
} else {
return relationshipFilter_ == null ?
com.authzed.api.v1.PermissionService.RelationshipFilter.getDefaultInstance() : relationshipFilter_;
}
}
/**
* .authzed.api.v1.RelationshipFilter relationship_filter = 1 [(.validate.rules) = { ... }
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.PermissionService.RelationshipFilter, com.authzed.api.v1.PermissionService.RelationshipFilter.Builder, com.authzed.api.v1.PermissionService.RelationshipFilterOrBuilder>
getRelationshipFilterFieldBuilder() {
if (relationshipFilterBuilder_ == null) {
relationshipFilterBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.PermissionService.RelationshipFilter, com.authzed.api.v1.PermissionService.RelationshipFilter.Builder, com.authzed.api.v1.PermissionService.RelationshipFilterOrBuilder>(
getRelationshipFilter(),
getParentForChildren(),
isClean());
relationshipFilter_ = null;
}
return relationshipFilterBuilder_;
}
private java.util.List optionalPreconditions_ =
java.util.Collections.emptyList();
private void ensureOptionalPreconditionsIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
optionalPreconditions_ = new java.util.ArrayList(optionalPreconditions_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.authzed.api.v1.PermissionService.Precondition, com.authzed.api.v1.PermissionService.Precondition.Builder, com.authzed.api.v1.PermissionService.PreconditionOrBuilder> optionalPreconditionsBuilder_;
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
public java.util.List getOptionalPreconditionsList() {
if (optionalPreconditionsBuilder_ == null) {
return java.util.Collections.unmodifiableList(optionalPreconditions_);
} else {
return optionalPreconditionsBuilder_.getMessageList();
}
}
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
public int getOptionalPreconditionsCount() {
if (optionalPreconditionsBuilder_ == null) {
return optionalPreconditions_.size();
} else {
return optionalPreconditionsBuilder_.getCount();
}
}
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
public com.authzed.api.v1.PermissionService.Precondition getOptionalPreconditions(int index) {
if (optionalPreconditionsBuilder_ == null) {
return optionalPreconditions_.get(index);
} else {
return optionalPreconditionsBuilder_.getMessage(index);
}
}
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
public Builder setOptionalPreconditions(
int index, com.authzed.api.v1.PermissionService.Precondition value) {
if (optionalPreconditionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOptionalPreconditionsIsMutable();
optionalPreconditions_.set(index, value);
onChanged();
} else {
optionalPreconditionsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
public Builder setOptionalPreconditions(
int index, com.authzed.api.v1.PermissionService.Precondition.Builder builderForValue) {
if (optionalPreconditionsBuilder_ == null) {
ensureOptionalPreconditionsIsMutable();
optionalPreconditions_.set(index, builderForValue.build());
onChanged();
} else {
optionalPreconditionsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
public Builder addOptionalPreconditions(com.authzed.api.v1.PermissionService.Precondition value) {
if (optionalPreconditionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOptionalPreconditionsIsMutable();
optionalPreconditions_.add(value);
onChanged();
} else {
optionalPreconditionsBuilder_.addMessage(value);
}
return this;
}
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
public Builder addOptionalPreconditions(
int index, com.authzed.api.v1.PermissionService.Precondition value) {
if (optionalPreconditionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOptionalPreconditionsIsMutable();
optionalPreconditions_.add(index, value);
onChanged();
} else {
optionalPreconditionsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
public Builder addOptionalPreconditions(
com.authzed.api.v1.PermissionService.Precondition.Builder builderForValue) {
if (optionalPreconditionsBuilder_ == null) {
ensureOptionalPreconditionsIsMutable();
optionalPreconditions_.add(builderForValue.build());
onChanged();
} else {
optionalPreconditionsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
public Builder addOptionalPreconditions(
int index, com.authzed.api.v1.PermissionService.Precondition.Builder builderForValue) {
if (optionalPreconditionsBuilder_ == null) {
ensureOptionalPreconditionsIsMutable();
optionalPreconditions_.add(index, builderForValue.build());
onChanged();
} else {
optionalPreconditionsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
public Builder addAllOptionalPreconditions(
java.lang.Iterable extends com.authzed.api.v1.PermissionService.Precondition> values) {
if (optionalPreconditionsBuilder_ == null) {
ensureOptionalPreconditionsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, optionalPreconditions_);
onChanged();
} else {
optionalPreconditionsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
public Builder clearOptionalPreconditions() {
if (optionalPreconditionsBuilder_ == null) {
optionalPreconditions_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
optionalPreconditionsBuilder_.clear();
}
return this;
}
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
public Builder removeOptionalPreconditions(int index) {
if (optionalPreconditionsBuilder_ == null) {
ensureOptionalPreconditionsIsMutable();
optionalPreconditions_.remove(index);
onChanged();
} else {
optionalPreconditionsBuilder_.remove(index);
}
return this;
}
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
public com.authzed.api.v1.PermissionService.Precondition.Builder getOptionalPreconditionsBuilder(
int index) {
return getOptionalPreconditionsFieldBuilder().getBuilder(index);
}
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
public com.authzed.api.v1.PermissionService.PreconditionOrBuilder getOptionalPreconditionsOrBuilder(
int index) {
if (optionalPreconditionsBuilder_ == null) {
return optionalPreconditions_.get(index); } else {
return optionalPreconditionsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
public java.util.List extends com.authzed.api.v1.PermissionService.PreconditionOrBuilder>
getOptionalPreconditionsOrBuilderList() {
if (optionalPreconditionsBuilder_ != null) {
return optionalPreconditionsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(optionalPreconditions_);
}
}
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
public com.authzed.api.v1.PermissionService.Precondition.Builder addOptionalPreconditionsBuilder() {
return getOptionalPreconditionsFieldBuilder().addBuilder(
com.authzed.api.v1.PermissionService.Precondition.getDefaultInstance());
}
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
public com.authzed.api.v1.PermissionService.Precondition.Builder addOptionalPreconditionsBuilder(
int index) {
return getOptionalPreconditionsFieldBuilder().addBuilder(
index, com.authzed.api.v1.PermissionService.Precondition.getDefaultInstance());
}
/**
*
* To be bounded by configuration
*
*
* repeated .authzed.api.v1.Precondition optional_preconditions = 2 [(.validate.rules) = { ... }
*/
public java.util.List
getOptionalPreconditionsBuilderList() {
return getOptionalPreconditionsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.authzed.api.v1.PermissionService.Precondition, com.authzed.api.v1.PermissionService.Precondition.Builder, com.authzed.api.v1.PermissionService.PreconditionOrBuilder>
getOptionalPreconditionsFieldBuilder() {
if (optionalPreconditionsBuilder_ == null) {
optionalPreconditionsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.authzed.api.v1.PermissionService.Precondition, com.authzed.api.v1.PermissionService.Precondition.Builder, com.authzed.api.v1.PermissionService.PreconditionOrBuilder>(
optionalPreconditions_,
((bitField0_ & 0x00000002) != 0),
getParentForChildren(),
isClean());
optionalPreconditions_ = null;
}
return optionalPreconditionsBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:authzed.api.v1.DeleteRelationshipsRequest)
}
// @@protoc_insertion_point(class_scope:authzed.api.v1.DeleteRelationshipsRequest)
private static final com.authzed.api.v1.PermissionService.DeleteRelationshipsRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.authzed.api.v1.PermissionService.DeleteRelationshipsRequest();
}
public static com.authzed.api.v1.PermissionService.DeleteRelationshipsRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public DeleteRelationshipsRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.DeleteRelationshipsRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface DeleteRelationshipsResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:authzed.api.v1.DeleteRelationshipsResponse)
com.google.protobuf.MessageOrBuilder {
/**
* .authzed.api.v1.ZedToken deleted_at = 1;
* @return Whether the deletedAt field is set.
*/
boolean hasDeletedAt();
/**
* .authzed.api.v1.ZedToken deleted_at = 1;
* @return The deletedAt.
*/
com.authzed.api.v1.Core.ZedToken getDeletedAt();
/**
* .authzed.api.v1.ZedToken deleted_at = 1;
*/
com.authzed.api.v1.Core.ZedTokenOrBuilder getDeletedAtOrBuilder();
}
/**
* Protobuf type {@code authzed.api.v1.DeleteRelationshipsResponse}
*/
public static final class DeleteRelationshipsResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:authzed.api.v1.DeleteRelationshipsResponse)
DeleteRelationshipsResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use DeleteRelationshipsResponse.newBuilder() to construct.
private DeleteRelationshipsResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private DeleteRelationshipsResponse() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new DeleteRelationshipsResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_DeleteRelationshipsResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_DeleteRelationshipsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.PermissionService.DeleteRelationshipsResponse.class, com.authzed.api.v1.PermissionService.DeleteRelationshipsResponse.Builder.class);
}
public static final int DELETED_AT_FIELD_NUMBER = 1;
private com.authzed.api.v1.Core.ZedToken deletedAt_;
/**
* .authzed.api.v1.ZedToken deleted_at = 1;
* @return Whether the deletedAt field is set.
*/
@java.lang.Override
public boolean hasDeletedAt() {
return deletedAt_ != null;
}
/**
* .authzed.api.v1.ZedToken deleted_at = 1;
* @return The deletedAt.
*/
@java.lang.Override
public com.authzed.api.v1.Core.ZedToken getDeletedAt() {
return deletedAt_ == null ? com.authzed.api.v1.Core.ZedToken.getDefaultInstance() : deletedAt_;
}
/**
* .authzed.api.v1.ZedToken deleted_at = 1;
*/
@java.lang.Override
public com.authzed.api.v1.Core.ZedTokenOrBuilder getDeletedAtOrBuilder() {
return deletedAt_ == null ? com.authzed.api.v1.Core.ZedToken.getDefaultInstance() : deletedAt_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (deletedAt_ != null) {
output.writeMessage(1, getDeletedAt());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (deletedAt_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getDeletedAt());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.authzed.api.v1.PermissionService.DeleteRelationshipsResponse)) {
return super.equals(obj);
}
com.authzed.api.v1.PermissionService.DeleteRelationshipsResponse other = (com.authzed.api.v1.PermissionService.DeleteRelationshipsResponse) obj;
if (hasDeletedAt() != other.hasDeletedAt()) return false;
if (hasDeletedAt()) {
if (!getDeletedAt()
.equals(other.getDeletedAt())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasDeletedAt()) {
hash = (37 * hash) + DELETED_AT_FIELD_NUMBER;
hash = (53 * hash) + getDeletedAt().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.authzed.api.v1.PermissionService.DeleteRelationshipsResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.DeleteRelationshipsResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.DeleteRelationshipsResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.DeleteRelationshipsResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.DeleteRelationshipsResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.DeleteRelationshipsResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.DeleteRelationshipsResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.DeleteRelationshipsResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.DeleteRelationshipsResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.DeleteRelationshipsResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.DeleteRelationshipsResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.DeleteRelationshipsResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.authzed.api.v1.PermissionService.DeleteRelationshipsResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code authzed.api.v1.DeleteRelationshipsResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:authzed.api.v1.DeleteRelationshipsResponse)
com.authzed.api.v1.PermissionService.DeleteRelationshipsResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_DeleteRelationshipsResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_DeleteRelationshipsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.PermissionService.DeleteRelationshipsResponse.class, com.authzed.api.v1.PermissionService.DeleteRelationshipsResponse.Builder.class);
}
// Construct using com.authzed.api.v1.PermissionService.DeleteRelationshipsResponse.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
deletedAt_ = null;
if (deletedAtBuilder_ != null) {
deletedAtBuilder_.dispose();
deletedAtBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_DeleteRelationshipsResponse_descriptor;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.DeleteRelationshipsResponse getDefaultInstanceForType() {
return com.authzed.api.v1.PermissionService.DeleteRelationshipsResponse.getDefaultInstance();
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.DeleteRelationshipsResponse build() {
com.authzed.api.v1.PermissionService.DeleteRelationshipsResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.DeleteRelationshipsResponse buildPartial() {
com.authzed.api.v1.PermissionService.DeleteRelationshipsResponse result = new com.authzed.api.v1.PermissionService.DeleteRelationshipsResponse(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.authzed.api.v1.PermissionService.DeleteRelationshipsResponse result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.deletedAt_ = deletedAtBuilder_ == null
? deletedAt_
: deletedAtBuilder_.build();
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.authzed.api.v1.PermissionService.DeleteRelationshipsResponse) {
return mergeFrom((com.authzed.api.v1.PermissionService.DeleteRelationshipsResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.authzed.api.v1.PermissionService.DeleteRelationshipsResponse other) {
if (other == com.authzed.api.v1.PermissionService.DeleteRelationshipsResponse.getDefaultInstance()) return this;
if (other.hasDeletedAt()) {
mergeDeletedAt(other.getDeletedAt());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
input.readMessage(
getDeletedAtFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case 10
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.authzed.api.v1.Core.ZedToken deletedAt_;
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.ZedToken, com.authzed.api.v1.Core.ZedToken.Builder, com.authzed.api.v1.Core.ZedTokenOrBuilder> deletedAtBuilder_;
/**
* .authzed.api.v1.ZedToken deleted_at = 1;
* @return Whether the deletedAt field is set.
*/
public boolean hasDeletedAt() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* .authzed.api.v1.ZedToken deleted_at = 1;
* @return The deletedAt.
*/
public com.authzed.api.v1.Core.ZedToken getDeletedAt() {
if (deletedAtBuilder_ == null) {
return deletedAt_ == null ? com.authzed.api.v1.Core.ZedToken.getDefaultInstance() : deletedAt_;
} else {
return deletedAtBuilder_.getMessage();
}
}
/**
* .authzed.api.v1.ZedToken deleted_at = 1;
*/
public Builder setDeletedAt(com.authzed.api.v1.Core.ZedToken value) {
if (deletedAtBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
deletedAt_ = value;
} else {
deletedAtBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .authzed.api.v1.ZedToken deleted_at = 1;
*/
public Builder setDeletedAt(
com.authzed.api.v1.Core.ZedToken.Builder builderForValue) {
if (deletedAtBuilder_ == null) {
deletedAt_ = builderForValue.build();
} else {
deletedAtBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .authzed.api.v1.ZedToken deleted_at = 1;
*/
public Builder mergeDeletedAt(com.authzed.api.v1.Core.ZedToken value) {
if (deletedAtBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0) &&
deletedAt_ != null &&
deletedAt_ != com.authzed.api.v1.Core.ZedToken.getDefaultInstance()) {
getDeletedAtBuilder().mergeFrom(value);
} else {
deletedAt_ = value;
}
} else {
deletedAtBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .authzed.api.v1.ZedToken deleted_at = 1;
*/
public Builder clearDeletedAt() {
bitField0_ = (bitField0_ & ~0x00000001);
deletedAt_ = null;
if (deletedAtBuilder_ != null) {
deletedAtBuilder_.dispose();
deletedAtBuilder_ = null;
}
onChanged();
return this;
}
/**
* .authzed.api.v1.ZedToken deleted_at = 1;
*/
public com.authzed.api.v1.Core.ZedToken.Builder getDeletedAtBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getDeletedAtFieldBuilder().getBuilder();
}
/**
* .authzed.api.v1.ZedToken deleted_at = 1;
*/
public com.authzed.api.v1.Core.ZedTokenOrBuilder getDeletedAtOrBuilder() {
if (deletedAtBuilder_ != null) {
return deletedAtBuilder_.getMessageOrBuilder();
} else {
return deletedAt_ == null ?
com.authzed.api.v1.Core.ZedToken.getDefaultInstance() : deletedAt_;
}
}
/**
* .authzed.api.v1.ZedToken deleted_at = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.ZedToken, com.authzed.api.v1.Core.ZedToken.Builder, com.authzed.api.v1.Core.ZedTokenOrBuilder>
getDeletedAtFieldBuilder() {
if (deletedAtBuilder_ == null) {
deletedAtBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.ZedToken, com.authzed.api.v1.Core.ZedToken.Builder, com.authzed.api.v1.Core.ZedTokenOrBuilder>(
getDeletedAt(),
getParentForChildren(),
isClean());
deletedAt_ = null;
}
return deletedAtBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:authzed.api.v1.DeleteRelationshipsResponse)
}
// @@protoc_insertion_point(class_scope:authzed.api.v1.DeleteRelationshipsResponse)
private static final com.authzed.api.v1.PermissionService.DeleteRelationshipsResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.authzed.api.v1.PermissionService.DeleteRelationshipsResponse();
}
public static com.authzed.api.v1.PermissionService.DeleteRelationshipsResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public DeleteRelationshipsResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.DeleteRelationshipsResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CheckPermissionRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:authzed.api.v1.CheckPermissionRequest)
com.google.protobuf.MessageOrBuilder {
/**
* .authzed.api.v1.Consistency consistency = 1;
* @return Whether the consistency field is set.
*/
boolean hasConsistency();
/**
* .authzed.api.v1.Consistency consistency = 1;
* @return The consistency.
*/
com.authzed.api.v1.PermissionService.Consistency getConsistency();
/**
* .authzed.api.v1.Consistency consistency = 1;
*/
com.authzed.api.v1.PermissionService.ConsistencyOrBuilder getConsistencyOrBuilder();
/**
*
* resource is the resource on which to check the permission or relation.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
* @return Whether the resource field is set.
*/
boolean hasResource();
/**
*
* resource is the resource on which to check the permission or relation.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
* @return The resource.
*/
com.authzed.api.v1.Core.ObjectReference getResource();
/**
*
* resource is the resource on which to check the permission or relation.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
*/
com.authzed.api.v1.Core.ObjectReferenceOrBuilder getResourceOrBuilder();
/**
*
* permission is the name of the permission (or relation) on which to execute
* the check.
*
*
* string permission = 3 [(.validate.rules) = { ... }
* @return The permission.
*/
java.lang.String getPermission();
/**
*
* permission is the name of the permission (or relation) on which to execute
* the check.
*
*
* string permission = 3 [(.validate.rules) = { ... }
* @return The bytes for permission.
*/
com.google.protobuf.ByteString
getPermissionBytes();
/**
*
* subject is the subject that will be checked for the permission or relation.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
* @return Whether the subject field is set.
*/
boolean hasSubject();
/**
*
* subject is the subject that will be checked for the permission or relation.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
* @return The subject.
*/
com.authzed.api.v1.Core.SubjectReference getSubject();
/**
*
* subject is the subject that will be checked for the permission or relation.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
*/
com.authzed.api.v1.Core.SubjectReferenceOrBuilder getSubjectOrBuilder();
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 5 [(.validate.rules) = { ... }
* @return Whether the context field is set.
*/
boolean hasContext();
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 5 [(.validate.rules) = { ... }
* @return The context.
*/
com.google.protobuf.Struct getContext();
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 5 [(.validate.rules) = { ... }
*/
com.google.protobuf.StructOrBuilder getContextOrBuilder();
}
/**
*
* CheckPermissionRequest issues a check on whether a subject has a permission
* or is a member of a relation, on a specific resource.
*
*
* Protobuf type {@code authzed.api.v1.CheckPermissionRequest}
*/
public static final class CheckPermissionRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:authzed.api.v1.CheckPermissionRequest)
CheckPermissionRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use CheckPermissionRequest.newBuilder() to construct.
private CheckPermissionRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CheckPermissionRequest() {
permission_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CheckPermissionRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_CheckPermissionRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_CheckPermissionRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.PermissionService.CheckPermissionRequest.class, com.authzed.api.v1.PermissionService.CheckPermissionRequest.Builder.class);
}
public static final int CONSISTENCY_FIELD_NUMBER = 1;
private com.authzed.api.v1.PermissionService.Consistency consistency_;
/**
* .authzed.api.v1.Consistency consistency = 1;
* @return Whether the consistency field is set.
*/
@java.lang.Override
public boolean hasConsistency() {
return consistency_ != null;
}
/**
* .authzed.api.v1.Consistency consistency = 1;
* @return The consistency.
*/
@java.lang.Override
public com.authzed.api.v1.PermissionService.Consistency getConsistency() {
return consistency_ == null ? com.authzed.api.v1.PermissionService.Consistency.getDefaultInstance() : consistency_;
}
/**
* .authzed.api.v1.Consistency consistency = 1;
*/
@java.lang.Override
public com.authzed.api.v1.PermissionService.ConsistencyOrBuilder getConsistencyOrBuilder() {
return consistency_ == null ? com.authzed.api.v1.PermissionService.Consistency.getDefaultInstance() : consistency_;
}
public static final int RESOURCE_FIELD_NUMBER = 2;
private com.authzed.api.v1.Core.ObjectReference resource_;
/**
*
* resource is the resource on which to check the permission or relation.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
* @return Whether the resource field is set.
*/
@java.lang.Override
public boolean hasResource() {
return resource_ != null;
}
/**
*
* resource is the resource on which to check the permission or relation.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
* @return The resource.
*/
@java.lang.Override
public com.authzed.api.v1.Core.ObjectReference getResource() {
return resource_ == null ? com.authzed.api.v1.Core.ObjectReference.getDefaultInstance() : resource_;
}
/**
*
* resource is the resource on which to check the permission or relation.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
*/
@java.lang.Override
public com.authzed.api.v1.Core.ObjectReferenceOrBuilder getResourceOrBuilder() {
return resource_ == null ? com.authzed.api.v1.Core.ObjectReference.getDefaultInstance() : resource_;
}
public static final int PERMISSION_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private volatile java.lang.Object permission_ = "";
/**
*
* permission is the name of the permission (or relation) on which to execute
* the check.
*
*
* string permission = 3 [(.validate.rules) = { ... }
* @return The permission.
*/
@java.lang.Override
public java.lang.String getPermission() {
java.lang.Object ref = permission_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
permission_ = s;
return s;
}
}
/**
*
* permission is the name of the permission (or relation) on which to execute
* the check.
*
*
* string permission = 3 [(.validate.rules) = { ... }
* @return The bytes for permission.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getPermissionBytes() {
java.lang.Object ref = permission_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
permission_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SUBJECT_FIELD_NUMBER = 4;
private com.authzed.api.v1.Core.SubjectReference subject_;
/**
*
* subject is the subject that will be checked for the permission or relation.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
* @return Whether the subject field is set.
*/
@java.lang.Override
public boolean hasSubject() {
return subject_ != null;
}
/**
*
* subject is the subject that will be checked for the permission or relation.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
* @return The subject.
*/
@java.lang.Override
public com.authzed.api.v1.Core.SubjectReference getSubject() {
return subject_ == null ? com.authzed.api.v1.Core.SubjectReference.getDefaultInstance() : subject_;
}
/**
*
* subject is the subject that will be checked for the permission or relation.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
*/
@java.lang.Override
public com.authzed.api.v1.Core.SubjectReferenceOrBuilder getSubjectOrBuilder() {
return subject_ == null ? com.authzed.api.v1.Core.SubjectReference.getDefaultInstance() : subject_;
}
public static final int CONTEXT_FIELD_NUMBER = 5;
private com.google.protobuf.Struct context_;
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 5 [(.validate.rules) = { ... }
* @return Whether the context field is set.
*/
@java.lang.Override
public boolean hasContext() {
return context_ != null;
}
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 5 [(.validate.rules) = { ... }
* @return The context.
*/
@java.lang.Override
public com.google.protobuf.Struct getContext() {
return context_ == null ? com.google.protobuf.Struct.getDefaultInstance() : context_;
}
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 5 [(.validate.rules) = { ... }
*/
@java.lang.Override
public com.google.protobuf.StructOrBuilder getContextOrBuilder() {
return context_ == null ? com.google.protobuf.Struct.getDefaultInstance() : context_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (consistency_ != null) {
output.writeMessage(1, getConsistency());
}
if (resource_ != null) {
output.writeMessage(2, getResource());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(permission_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, permission_);
}
if (subject_ != null) {
output.writeMessage(4, getSubject());
}
if (context_ != null) {
output.writeMessage(5, getContext());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (consistency_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getConsistency());
}
if (resource_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getResource());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(permission_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, permission_);
}
if (subject_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getSubject());
}
if (context_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, getContext());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.authzed.api.v1.PermissionService.CheckPermissionRequest)) {
return super.equals(obj);
}
com.authzed.api.v1.PermissionService.CheckPermissionRequest other = (com.authzed.api.v1.PermissionService.CheckPermissionRequest) obj;
if (hasConsistency() != other.hasConsistency()) return false;
if (hasConsistency()) {
if (!getConsistency()
.equals(other.getConsistency())) return false;
}
if (hasResource() != other.hasResource()) return false;
if (hasResource()) {
if (!getResource()
.equals(other.getResource())) return false;
}
if (!getPermission()
.equals(other.getPermission())) return false;
if (hasSubject() != other.hasSubject()) return false;
if (hasSubject()) {
if (!getSubject()
.equals(other.getSubject())) return false;
}
if (hasContext() != other.hasContext()) return false;
if (hasContext()) {
if (!getContext()
.equals(other.getContext())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasConsistency()) {
hash = (37 * hash) + CONSISTENCY_FIELD_NUMBER;
hash = (53 * hash) + getConsistency().hashCode();
}
if (hasResource()) {
hash = (37 * hash) + RESOURCE_FIELD_NUMBER;
hash = (53 * hash) + getResource().hashCode();
}
hash = (37 * hash) + PERMISSION_FIELD_NUMBER;
hash = (53 * hash) + getPermission().hashCode();
if (hasSubject()) {
hash = (37 * hash) + SUBJECT_FIELD_NUMBER;
hash = (53 * hash) + getSubject().hashCode();
}
if (hasContext()) {
hash = (37 * hash) + CONTEXT_FIELD_NUMBER;
hash = (53 * hash) + getContext().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.authzed.api.v1.PermissionService.CheckPermissionRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.CheckPermissionRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.CheckPermissionRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.CheckPermissionRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.CheckPermissionRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.CheckPermissionRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.CheckPermissionRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.CheckPermissionRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.CheckPermissionRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.CheckPermissionRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.CheckPermissionRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.CheckPermissionRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.authzed.api.v1.PermissionService.CheckPermissionRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* CheckPermissionRequest issues a check on whether a subject has a permission
* or is a member of a relation, on a specific resource.
*
*
* Protobuf type {@code authzed.api.v1.CheckPermissionRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:authzed.api.v1.CheckPermissionRequest)
com.authzed.api.v1.PermissionService.CheckPermissionRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_CheckPermissionRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_CheckPermissionRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.PermissionService.CheckPermissionRequest.class, com.authzed.api.v1.PermissionService.CheckPermissionRequest.Builder.class);
}
// Construct using com.authzed.api.v1.PermissionService.CheckPermissionRequest.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
consistency_ = null;
if (consistencyBuilder_ != null) {
consistencyBuilder_.dispose();
consistencyBuilder_ = null;
}
resource_ = null;
if (resourceBuilder_ != null) {
resourceBuilder_.dispose();
resourceBuilder_ = null;
}
permission_ = "";
subject_ = null;
if (subjectBuilder_ != null) {
subjectBuilder_.dispose();
subjectBuilder_ = null;
}
context_ = null;
if (contextBuilder_ != null) {
contextBuilder_.dispose();
contextBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_CheckPermissionRequest_descriptor;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.CheckPermissionRequest getDefaultInstanceForType() {
return com.authzed.api.v1.PermissionService.CheckPermissionRequest.getDefaultInstance();
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.CheckPermissionRequest build() {
com.authzed.api.v1.PermissionService.CheckPermissionRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.CheckPermissionRequest buildPartial() {
com.authzed.api.v1.PermissionService.CheckPermissionRequest result = new com.authzed.api.v1.PermissionService.CheckPermissionRequest(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.authzed.api.v1.PermissionService.CheckPermissionRequest result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.consistency_ = consistencyBuilder_ == null
? consistency_
: consistencyBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.resource_ = resourceBuilder_ == null
? resource_
: resourceBuilder_.build();
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.permission_ = permission_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.subject_ = subjectBuilder_ == null
? subject_
: subjectBuilder_.build();
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.context_ = contextBuilder_ == null
? context_
: contextBuilder_.build();
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.authzed.api.v1.PermissionService.CheckPermissionRequest) {
return mergeFrom((com.authzed.api.v1.PermissionService.CheckPermissionRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.authzed.api.v1.PermissionService.CheckPermissionRequest other) {
if (other == com.authzed.api.v1.PermissionService.CheckPermissionRequest.getDefaultInstance()) return this;
if (other.hasConsistency()) {
mergeConsistency(other.getConsistency());
}
if (other.hasResource()) {
mergeResource(other.getResource());
}
if (!other.getPermission().isEmpty()) {
permission_ = other.permission_;
bitField0_ |= 0x00000004;
onChanged();
}
if (other.hasSubject()) {
mergeSubject(other.getSubject());
}
if (other.hasContext()) {
mergeContext(other.getContext());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
input.readMessage(
getConsistencyFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
input.readMessage(
getResourceFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000002;
break;
} // case 18
case 26: {
permission_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000004;
break;
} // case 26
case 34: {
input.readMessage(
getSubjectFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000008;
break;
} // case 34
case 42: {
input.readMessage(
getContextFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000010;
break;
} // case 42
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.authzed.api.v1.PermissionService.Consistency consistency_;
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.PermissionService.Consistency, com.authzed.api.v1.PermissionService.Consistency.Builder, com.authzed.api.v1.PermissionService.ConsistencyOrBuilder> consistencyBuilder_;
/**
* .authzed.api.v1.Consistency consistency = 1;
* @return Whether the consistency field is set.
*/
public boolean hasConsistency() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* .authzed.api.v1.Consistency consistency = 1;
* @return The consistency.
*/
public com.authzed.api.v1.PermissionService.Consistency getConsistency() {
if (consistencyBuilder_ == null) {
return consistency_ == null ? com.authzed.api.v1.PermissionService.Consistency.getDefaultInstance() : consistency_;
} else {
return consistencyBuilder_.getMessage();
}
}
/**
* .authzed.api.v1.Consistency consistency = 1;
*/
public Builder setConsistency(com.authzed.api.v1.PermissionService.Consistency value) {
if (consistencyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
consistency_ = value;
} else {
consistencyBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .authzed.api.v1.Consistency consistency = 1;
*/
public Builder setConsistency(
com.authzed.api.v1.PermissionService.Consistency.Builder builderForValue) {
if (consistencyBuilder_ == null) {
consistency_ = builderForValue.build();
} else {
consistencyBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .authzed.api.v1.Consistency consistency = 1;
*/
public Builder mergeConsistency(com.authzed.api.v1.PermissionService.Consistency value) {
if (consistencyBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0) &&
consistency_ != null &&
consistency_ != com.authzed.api.v1.PermissionService.Consistency.getDefaultInstance()) {
getConsistencyBuilder().mergeFrom(value);
} else {
consistency_ = value;
}
} else {
consistencyBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .authzed.api.v1.Consistency consistency = 1;
*/
public Builder clearConsistency() {
bitField0_ = (bitField0_ & ~0x00000001);
consistency_ = null;
if (consistencyBuilder_ != null) {
consistencyBuilder_.dispose();
consistencyBuilder_ = null;
}
onChanged();
return this;
}
/**
* .authzed.api.v1.Consistency consistency = 1;
*/
public com.authzed.api.v1.PermissionService.Consistency.Builder getConsistencyBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getConsistencyFieldBuilder().getBuilder();
}
/**
* .authzed.api.v1.Consistency consistency = 1;
*/
public com.authzed.api.v1.PermissionService.ConsistencyOrBuilder getConsistencyOrBuilder() {
if (consistencyBuilder_ != null) {
return consistencyBuilder_.getMessageOrBuilder();
} else {
return consistency_ == null ?
com.authzed.api.v1.PermissionService.Consistency.getDefaultInstance() : consistency_;
}
}
/**
* .authzed.api.v1.Consistency consistency = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.PermissionService.Consistency, com.authzed.api.v1.PermissionService.Consistency.Builder, com.authzed.api.v1.PermissionService.ConsistencyOrBuilder>
getConsistencyFieldBuilder() {
if (consistencyBuilder_ == null) {
consistencyBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.PermissionService.Consistency, com.authzed.api.v1.PermissionService.Consistency.Builder, com.authzed.api.v1.PermissionService.ConsistencyOrBuilder>(
getConsistency(),
getParentForChildren(),
isClean());
consistency_ = null;
}
return consistencyBuilder_;
}
private com.authzed.api.v1.Core.ObjectReference resource_;
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.ObjectReference, com.authzed.api.v1.Core.ObjectReference.Builder, com.authzed.api.v1.Core.ObjectReferenceOrBuilder> resourceBuilder_;
/**
*
* resource is the resource on which to check the permission or relation.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
* @return Whether the resource field is set.
*/
public boolean hasResource() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* resource is the resource on which to check the permission or relation.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
* @return The resource.
*/
public com.authzed.api.v1.Core.ObjectReference getResource() {
if (resourceBuilder_ == null) {
return resource_ == null ? com.authzed.api.v1.Core.ObjectReference.getDefaultInstance() : resource_;
} else {
return resourceBuilder_.getMessage();
}
}
/**
*
* resource is the resource on which to check the permission or relation.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
*/
public Builder setResource(com.authzed.api.v1.Core.ObjectReference value) {
if (resourceBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
resource_ = value;
} else {
resourceBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* resource is the resource on which to check the permission or relation.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
*/
public Builder setResource(
com.authzed.api.v1.Core.ObjectReference.Builder builderForValue) {
if (resourceBuilder_ == null) {
resource_ = builderForValue.build();
} else {
resourceBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* resource is the resource on which to check the permission or relation.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
*/
public Builder mergeResource(com.authzed.api.v1.Core.ObjectReference value) {
if (resourceBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0) &&
resource_ != null &&
resource_ != com.authzed.api.v1.Core.ObjectReference.getDefaultInstance()) {
getResourceBuilder().mergeFrom(value);
} else {
resource_ = value;
}
} else {
resourceBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* resource is the resource on which to check the permission or relation.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
*/
public Builder clearResource() {
bitField0_ = (bitField0_ & ~0x00000002);
resource_ = null;
if (resourceBuilder_ != null) {
resourceBuilder_.dispose();
resourceBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* resource is the resource on which to check the permission or relation.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
*/
public com.authzed.api.v1.Core.ObjectReference.Builder getResourceBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getResourceFieldBuilder().getBuilder();
}
/**
*
* resource is the resource on which to check the permission or relation.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
*/
public com.authzed.api.v1.Core.ObjectReferenceOrBuilder getResourceOrBuilder() {
if (resourceBuilder_ != null) {
return resourceBuilder_.getMessageOrBuilder();
} else {
return resource_ == null ?
com.authzed.api.v1.Core.ObjectReference.getDefaultInstance() : resource_;
}
}
/**
*
* resource is the resource on which to check the permission or relation.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.ObjectReference, com.authzed.api.v1.Core.ObjectReference.Builder, com.authzed.api.v1.Core.ObjectReferenceOrBuilder>
getResourceFieldBuilder() {
if (resourceBuilder_ == null) {
resourceBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.ObjectReference, com.authzed.api.v1.Core.ObjectReference.Builder, com.authzed.api.v1.Core.ObjectReferenceOrBuilder>(
getResource(),
getParentForChildren(),
isClean());
resource_ = null;
}
return resourceBuilder_;
}
private java.lang.Object permission_ = "";
/**
*
* permission is the name of the permission (or relation) on which to execute
* the check.
*
*
* string permission = 3 [(.validate.rules) = { ... }
* @return The permission.
*/
public java.lang.String getPermission() {
java.lang.Object ref = permission_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
permission_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* permission is the name of the permission (or relation) on which to execute
* the check.
*
*
* string permission = 3 [(.validate.rules) = { ... }
* @return The bytes for permission.
*/
public com.google.protobuf.ByteString
getPermissionBytes() {
java.lang.Object ref = permission_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
permission_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* permission is the name of the permission (or relation) on which to execute
* the check.
*
*
* string permission = 3 [(.validate.rules) = { ... }
* @param value The permission to set.
* @return This builder for chaining.
*/
public Builder setPermission(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
permission_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* permission is the name of the permission (or relation) on which to execute
* the check.
*
*
* string permission = 3 [(.validate.rules) = { ... }
* @return This builder for chaining.
*/
public Builder clearPermission() {
permission_ = getDefaultInstance().getPermission();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
*
* permission is the name of the permission (or relation) on which to execute
* the check.
*
*
* string permission = 3 [(.validate.rules) = { ... }
* @param value The bytes for permission to set.
* @return This builder for chaining.
*/
public Builder setPermissionBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
permission_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
private com.authzed.api.v1.Core.SubjectReference subject_;
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.SubjectReference, com.authzed.api.v1.Core.SubjectReference.Builder, com.authzed.api.v1.Core.SubjectReferenceOrBuilder> subjectBuilder_;
/**
*
* subject is the subject that will be checked for the permission or relation.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
* @return Whether the subject field is set.
*/
public boolean hasSubject() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* subject is the subject that will be checked for the permission or relation.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
* @return The subject.
*/
public com.authzed.api.v1.Core.SubjectReference getSubject() {
if (subjectBuilder_ == null) {
return subject_ == null ? com.authzed.api.v1.Core.SubjectReference.getDefaultInstance() : subject_;
} else {
return subjectBuilder_.getMessage();
}
}
/**
*
* subject is the subject that will be checked for the permission or relation.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
*/
public Builder setSubject(com.authzed.api.v1.Core.SubjectReference value) {
if (subjectBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
subject_ = value;
} else {
subjectBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* subject is the subject that will be checked for the permission or relation.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
*/
public Builder setSubject(
com.authzed.api.v1.Core.SubjectReference.Builder builderForValue) {
if (subjectBuilder_ == null) {
subject_ = builderForValue.build();
} else {
subjectBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* subject is the subject that will be checked for the permission or relation.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
*/
public Builder mergeSubject(com.authzed.api.v1.Core.SubjectReference value) {
if (subjectBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0) &&
subject_ != null &&
subject_ != com.authzed.api.v1.Core.SubjectReference.getDefaultInstance()) {
getSubjectBuilder().mergeFrom(value);
} else {
subject_ = value;
}
} else {
subjectBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* subject is the subject that will be checked for the permission or relation.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
*/
public Builder clearSubject() {
bitField0_ = (bitField0_ & ~0x00000008);
subject_ = null;
if (subjectBuilder_ != null) {
subjectBuilder_.dispose();
subjectBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* subject is the subject that will be checked for the permission or relation.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
*/
public com.authzed.api.v1.Core.SubjectReference.Builder getSubjectBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getSubjectFieldBuilder().getBuilder();
}
/**
*
* subject is the subject that will be checked for the permission or relation.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
*/
public com.authzed.api.v1.Core.SubjectReferenceOrBuilder getSubjectOrBuilder() {
if (subjectBuilder_ != null) {
return subjectBuilder_.getMessageOrBuilder();
} else {
return subject_ == null ?
com.authzed.api.v1.Core.SubjectReference.getDefaultInstance() : subject_;
}
}
/**
*
* subject is the subject that will be checked for the permission or relation.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.SubjectReference, com.authzed.api.v1.Core.SubjectReference.Builder, com.authzed.api.v1.Core.SubjectReferenceOrBuilder>
getSubjectFieldBuilder() {
if (subjectBuilder_ == null) {
subjectBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.SubjectReference, com.authzed.api.v1.Core.SubjectReference.Builder, com.authzed.api.v1.Core.SubjectReferenceOrBuilder>(
getSubject(),
getParentForChildren(),
isClean());
subject_ = null;
}
return subjectBuilder_;
}
private com.google.protobuf.Struct context_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Struct, com.google.protobuf.Struct.Builder, com.google.protobuf.StructOrBuilder> contextBuilder_;
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 5 [(.validate.rules) = { ... }
* @return Whether the context field is set.
*/
public boolean hasContext() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 5 [(.validate.rules) = { ... }
* @return The context.
*/
public com.google.protobuf.Struct getContext() {
if (contextBuilder_ == null) {
return context_ == null ? com.google.protobuf.Struct.getDefaultInstance() : context_;
} else {
return contextBuilder_.getMessage();
}
}
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 5 [(.validate.rules) = { ... }
*/
public Builder setContext(com.google.protobuf.Struct value) {
if (contextBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
context_ = value;
} else {
contextBuilder_.setMessage(value);
}
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 5 [(.validate.rules) = { ... }
*/
public Builder setContext(
com.google.protobuf.Struct.Builder builderForValue) {
if (contextBuilder_ == null) {
context_ = builderForValue.build();
} else {
contextBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 5 [(.validate.rules) = { ... }
*/
public Builder mergeContext(com.google.protobuf.Struct value) {
if (contextBuilder_ == null) {
if (((bitField0_ & 0x00000010) != 0) &&
context_ != null &&
context_ != com.google.protobuf.Struct.getDefaultInstance()) {
getContextBuilder().mergeFrom(value);
} else {
context_ = value;
}
} else {
contextBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 5 [(.validate.rules) = { ... }
*/
public Builder clearContext() {
bitField0_ = (bitField0_ & ~0x00000010);
context_ = null;
if (contextBuilder_ != null) {
contextBuilder_.dispose();
contextBuilder_ = null;
}
onChanged();
return this;
}
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 5 [(.validate.rules) = { ... }
*/
public com.google.protobuf.Struct.Builder getContextBuilder() {
bitField0_ |= 0x00000010;
onChanged();
return getContextFieldBuilder().getBuilder();
}
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 5 [(.validate.rules) = { ... }
*/
public com.google.protobuf.StructOrBuilder getContextOrBuilder() {
if (contextBuilder_ != null) {
return contextBuilder_.getMessageOrBuilder();
} else {
return context_ == null ?
com.google.protobuf.Struct.getDefaultInstance() : context_;
}
}
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 5 [(.validate.rules) = { ... }
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Struct, com.google.protobuf.Struct.Builder, com.google.protobuf.StructOrBuilder>
getContextFieldBuilder() {
if (contextBuilder_ == null) {
contextBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Struct, com.google.protobuf.Struct.Builder, com.google.protobuf.StructOrBuilder>(
getContext(),
getParentForChildren(),
isClean());
context_ = null;
}
return contextBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:authzed.api.v1.CheckPermissionRequest)
}
// @@protoc_insertion_point(class_scope:authzed.api.v1.CheckPermissionRequest)
private static final com.authzed.api.v1.PermissionService.CheckPermissionRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.authzed.api.v1.PermissionService.CheckPermissionRequest();
}
public static com.authzed.api.v1.PermissionService.CheckPermissionRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CheckPermissionRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.CheckPermissionRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CheckPermissionResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:authzed.api.v1.CheckPermissionResponse)
com.google.protobuf.MessageOrBuilder {
/**
* .authzed.api.v1.ZedToken checked_at = 1 [(.validate.rules) = { ... }
* @return Whether the checkedAt field is set.
*/
boolean hasCheckedAt();
/**
* .authzed.api.v1.ZedToken checked_at = 1 [(.validate.rules) = { ... }
* @return The checkedAt.
*/
com.authzed.api.v1.Core.ZedToken getCheckedAt();
/**
* .authzed.api.v1.ZedToken checked_at = 1 [(.validate.rules) = { ... }
*/
com.authzed.api.v1.Core.ZedTokenOrBuilder getCheckedAtOrBuilder();
/**
*
* Permissionship communicates whether or not the subject has the requested
* permission or has a relationship with the given resource, over the given
* relation.
* This value will be authzed.api.v1.PERMISSIONSHIP_HAS_PERMISSION if the
* requested subject is a member of the computed permission set or there
* exists a relationship with the requested relation from the given resource
* to the given subject.
*
*
* .authzed.api.v1.CheckPermissionResponse.Permissionship permissionship = 2 [(.validate.rules) = { ... }
* @return The enum numeric value on the wire for permissionship.
*/
int getPermissionshipValue();
/**
*
* Permissionship communicates whether or not the subject has the requested
* permission or has a relationship with the given resource, over the given
* relation.
* This value will be authzed.api.v1.PERMISSIONSHIP_HAS_PERMISSION if the
* requested subject is a member of the computed permission set or there
* exists a relationship with the requested relation from the given resource
* to the given subject.
*
*
* .authzed.api.v1.CheckPermissionResponse.Permissionship permissionship = 2 [(.validate.rules) = { ... }
* @return The permissionship.
*/
com.authzed.api.v1.PermissionService.CheckPermissionResponse.Permissionship getPermissionship();
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 3 [(.validate.rules) = { ... }
* @return Whether the partialCaveatInfo field is set.
*/
boolean hasPartialCaveatInfo();
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 3 [(.validate.rules) = { ... }
* @return The partialCaveatInfo.
*/
com.authzed.api.v1.Core.PartialCaveatInfo getPartialCaveatInfo();
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 3 [(.validate.rules) = { ... }
*/
com.authzed.api.v1.Core.PartialCaveatInfoOrBuilder getPartialCaveatInfoOrBuilder();
}
/**
* Protobuf type {@code authzed.api.v1.CheckPermissionResponse}
*/
public static final class CheckPermissionResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:authzed.api.v1.CheckPermissionResponse)
CheckPermissionResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use CheckPermissionResponse.newBuilder() to construct.
private CheckPermissionResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CheckPermissionResponse() {
permissionship_ = 0;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CheckPermissionResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_CheckPermissionResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_CheckPermissionResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.PermissionService.CheckPermissionResponse.class, com.authzed.api.v1.PermissionService.CheckPermissionResponse.Builder.class);
}
/**
* Protobuf enum {@code authzed.api.v1.CheckPermissionResponse.Permissionship}
*/
public enum Permissionship
implements com.google.protobuf.ProtocolMessageEnum {
/**
* PERMISSIONSHIP_UNSPECIFIED = 0;
*/
PERMISSIONSHIP_UNSPECIFIED(0),
/**
* PERMISSIONSHIP_NO_PERMISSION = 1;
*/
PERMISSIONSHIP_NO_PERMISSION(1),
/**
* PERMISSIONSHIP_HAS_PERMISSION = 2;
*/
PERMISSIONSHIP_HAS_PERMISSION(2),
/**
* PERMISSIONSHIP_CONDITIONAL_PERMISSION = 3;
*/
PERMISSIONSHIP_CONDITIONAL_PERMISSION(3),
UNRECOGNIZED(-1),
;
/**
* PERMISSIONSHIP_UNSPECIFIED = 0;
*/
public static final int PERMISSIONSHIP_UNSPECIFIED_VALUE = 0;
/**
* PERMISSIONSHIP_NO_PERMISSION = 1;
*/
public static final int PERMISSIONSHIP_NO_PERMISSION_VALUE = 1;
/**
* PERMISSIONSHIP_HAS_PERMISSION = 2;
*/
public static final int PERMISSIONSHIP_HAS_PERMISSION_VALUE = 2;
/**
* PERMISSIONSHIP_CONDITIONAL_PERMISSION = 3;
*/
public static final int PERMISSIONSHIP_CONDITIONAL_PERMISSION_VALUE = 3;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Permissionship valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static Permissionship forNumber(int value) {
switch (value) {
case 0: return PERMISSIONSHIP_UNSPECIFIED;
case 1: return PERMISSIONSHIP_NO_PERMISSION;
case 2: return PERMISSIONSHIP_HAS_PERMISSION;
case 3: return PERMISSIONSHIP_CONDITIONAL_PERMISSION;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
Permissionship> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Permissionship findValueByNumber(int number) {
return Permissionship.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.authzed.api.v1.PermissionService.CheckPermissionResponse.getDescriptor().getEnumTypes().get(0);
}
private static final Permissionship[] VALUES = values();
public static Permissionship valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private Permissionship(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:authzed.api.v1.CheckPermissionResponse.Permissionship)
}
public static final int CHECKED_AT_FIELD_NUMBER = 1;
private com.authzed.api.v1.Core.ZedToken checkedAt_;
/**
* .authzed.api.v1.ZedToken checked_at = 1 [(.validate.rules) = { ... }
* @return Whether the checkedAt field is set.
*/
@java.lang.Override
public boolean hasCheckedAt() {
return checkedAt_ != null;
}
/**
* .authzed.api.v1.ZedToken checked_at = 1 [(.validate.rules) = { ... }
* @return The checkedAt.
*/
@java.lang.Override
public com.authzed.api.v1.Core.ZedToken getCheckedAt() {
return checkedAt_ == null ? com.authzed.api.v1.Core.ZedToken.getDefaultInstance() : checkedAt_;
}
/**
* .authzed.api.v1.ZedToken checked_at = 1 [(.validate.rules) = { ... }
*/
@java.lang.Override
public com.authzed.api.v1.Core.ZedTokenOrBuilder getCheckedAtOrBuilder() {
return checkedAt_ == null ? com.authzed.api.v1.Core.ZedToken.getDefaultInstance() : checkedAt_;
}
public static final int PERMISSIONSHIP_FIELD_NUMBER = 2;
private int permissionship_ = 0;
/**
*
* Permissionship communicates whether or not the subject has the requested
* permission or has a relationship with the given resource, over the given
* relation.
* This value will be authzed.api.v1.PERMISSIONSHIP_HAS_PERMISSION if the
* requested subject is a member of the computed permission set or there
* exists a relationship with the requested relation from the given resource
* to the given subject.
*
*
* .authzed.api.v1.CheckPermissionResponse.Permissionship permissionship = 2 [(.validate.rules) = { ... }
* @return The enum numeric value on the wire for permissionship.
*/
@java.lang.Override public int getPermissionshipValue() {
return permissionship_;
}
/**
*
* Permissionship communicates whether or not the subject has the requested
* permission or has a relationship with the given resource, over the given
* relation.
* This value will be authzed.api.v1.PERMISSIONSHIP_HAS_PERMISSION if the
* requested subject is a member of the computed permission set or there
* exists a relationship with the requested relation from the given resource
* to the given subject.
*
*
* .authzed.api.v1.CheckPermissionResponse.Permissionship permissionship = 2 [(.validate.rules) = { ... }
* @return The permissionship.
*/
@java.lang.Override public com.authzed.api.v1.PermissionService.CheckPermissionResponse.Permissionship getPermissionship() {
com.authzed.api.v1.PermissionService.CheckPermissionResponse.Permissionship result = com.authzed.api.v1.PermissionService.CheckPermissionResponse.Permissionship.forNumber(permissionship_);
return result == null ? com.authzed.api.v1.PermissionService.CheckPermissionResponse.Permissionship.UNRECOGNIZED : result;
}
public static final int PARTIAL_CAVEAT_INFO_FIELD_NUMBER = 3;
private com.authzed.api.v1.Core.PartialCaveatInfo partialCaveatInfo_;
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 3 [(.validate.rules) = { ... }
* @return Whether the partialCaveatInfo field is set.
*/
@java.lang.Override
public boolean hasPartialCaveatInfo() {
return partialCaveatInfo_ != null;
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 3 [(.validate.rules) = { ... }
* @return The partialCaveatInfo.
*/
@java.lang.Override
public com.authzed.api.v1.Core.PartialCaveatInfo getPartialCaveatInfo() {
return partialCaveatInfo_ == null ? com.authzed.api.v1.Core.PartialCaveatInfo.getDefaultInstance() : partialCaveatInfo_;
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 3 [(.validate.rules) = { ... }
*/
@java.lang.Override
public com.authzed.api.v1.Core.PartialCaveatInfoOrBuilder getPartialCaveatInfoOrBuilder() {
return partialCaveatInfo_ == null ? com.authzed.api.v1.Core.PartialCaveatInfo.getDefaultInstance() : partialCaveatInfo_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (checkedAt_ != null) {
output.writeMessage(1, getCheckedAt());
}
if (permissionship_ != com.authzed.api.v1.PermissionService.CheckPermissionResponse.Permissionship.PERMISSIONSHIP_UNSPECIFIED.getNumber()) {
output.writeEnum(2, permissionship_);
}
if (partialCaveatInfo_ != null) {
output.writeMessage(3, getPartialCaveatInfo());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (checkedAt_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getCheckedAt());
}
if (permissionship_ != com.authzed.api.v1.PermissionService.CheckPermissionResponse.Permissionship.PERMISSIONSHIP_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, permissionship_);
}
if (partialCaveatInfo_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getPartialCaveatInfo());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.authzed.api.v1.PermissionService.CheckPermissionResponse)) {
return super.equals(obj);
}
com.authzed.api.v1.PermissionService.CheckPermissionResponse other = (com.authzed.api.v1.PermissionService.CheckPermissionResponse) obj;
if (hasCheckedAt() != other.hasCheckedAt()) return false;
if (hasCheckedAt()) {
if (!getCheckedAt()
.equals(other.getCheckedAt())) return false;
}
if (permissionship_ != other.permissionship_) return false;
if (hasPartialCaveatInfo() != other.hasPartialCaveatInfo()) return false;
if (hasPartialCaveatInfo()) {
if (!getPartialCaveatInfo()
.equals(other.getPartialCaveatInfo())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasCheckedAt()) {
hash = (37 * hash) + CHECKED_AT_FIELD_NUMBER;
hash = (53 * hash) + getCheckedAt().hashCode();
}
hash = (37 * hash) + PERMISSIONSHIP_FIELD_NUMBER;
hash = (53 * hash) + permissionship_;
if (hasPartialCaveatInfo()) {
hash = (37 * hash) + PARTIAL_CAVEAT_INFO_FIELD_NUMBER;
hash = (53 * hash) + getPartialCaveatInfo().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.authzed.api.v1.PermissionService.CheckPermissionResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.CheckPermissionResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.CheckPermissionResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.CheckPermissionResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.CheckPermissionResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.CheckPermissionResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.CheckPermissionResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.CheckPermissionResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.CheckPermissionResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.CheckPermissionResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.CheckPermissionResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.CheckPermissionResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.authzed.api.v1.PermissionService.CheckPermissionResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code authzed.api.v1.CheckPermissionResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:authzed.api.v1.CheckPermissionResponse)
com.authzed.api.v1.PermissionService.CheckPermissionResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_CheckPermissionResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_CheckPermissionResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.PermissionService.CheckPermissionResponse.class, com.authzed.api.v1.PermissionService.CheckPermissionResponse.Builder.class);
}
// Construct using com.authzed.api.v1.PermissionService.CheckPermissionResponse.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
checkedAt_ = null;
if (checkedAtBuilder_ != null) {
checkedAtBuilder_.dispose();
checkedAtBuilder_ = null;
}
permissionship_ = 0;
partialCaveatInfo_ = null;
if (partialCaveatInfoBuilder_ != null) {
partialCaveatInfoBuilder_.dispose();
partialCaveatInfoBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_CheckPermissionResponse_descriptor;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.CheckPermissionResponse getDefaultInstanceForType() {
return com.authzed.api.v1.PermissionService.CheckPermissionResponse.getDefaultInstance();
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.CheckPermissionResponse build() {
com.authzed.api.v1.PermissionService.CheckPermissionResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.CheckPermissionResponse buildPartial() {
com.authzed.api.v1.PermissionService.CheckPermissionResponse result = new com.authzed.api.v1.PermissionService.CheckPermissionResponse(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.authzed.api.v1.PermissionService.CheckPermissionResponse result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.checkedAt_ = checkedAtBuilder_ == null
? checkedAt_
: checkedAtBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.permissionship_ = permissionship_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.partialCaveatInfo_ = partialCaveatInfoBuilder_ == null
? partialCaveatInfo_
: partialCaveatInfoBuilder_.build();
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.authzed.api.v1.PermissionService.CheckPermissionResponse) {
return mergeFrom((com.authzed.api.v1.PermissionService.CheckPermissionResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.authzed.api.v1.PermissionService.CheckPermissionResponse other) {
if (other == com.authzed.api.v1.PermissionService.CheckPermissionResponse.getDefaultInstance()) return this;
if (other.hasCheckedAt()) {
mergeCheckedAt(other.getCheckedAt());
}
if (other.permissionship_ != 0) {
setPermissionshipValue(other.getPermissionshipValue());
}
if (other.hasPartialCaveatInfo()) {
mergePartialCaveatInfo(other.getPartialCaveatInfo());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
input.readMessage(
getCheckedAtFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case 10
case 16: {
permissionship_ = input.readEnum();
bitField0_ |= 0x00000002;
break;
} // case 16
case 26: {
input.readMessage(
getPartialCaveatInfoFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000004;
break;
} // case 26
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.authzed.api.v1.Core.ZedToken checkedAt_;
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.ZedToken, com.authzed.api.v1.Core.ZedToken.Builder, com.authzed.api.v1.Core.ZedTokenOrBuilder> checkedAtBuilder_;
/**
* .authzed.api.v1.ZedToken checked_at = 1 [(.validate.rules) = { ... }
* @return Whether the checkedAt field is set.
*/
public boolean hasCheckedAt() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* .authzed.api.v1.ZedToken checked_at = 1 [(.validate.rules) = { ... }
* @return The checkedAt.
*/
public com.authzed.api.v1.Core.ZedToken getCheckedAt() {
if (checkedAtBuilder_ == null) {
return checkedAt_ == null ? com.authzed.api.v1.Core.ZedToken.getDefaultInstance() : checkedAt_;
} else {
return checkedAtBuilder_.getMessage();
}
}
/**
* .authzed.api.v1.ZedToken checked_at = 1 [(.validate.rules) = { ... }
*/
public Builder setCheckedAt(com.authzed.api.v1.Core.ZedToken value) {
if (checkedAtBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
checkedAt_ = value;
} else {
checkedAtBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .authzed.api.v1.ZedToken checked_at = 1 [(.validate.rules) = { ... }
*/
public Builder setCheckedAt(
com.authzed.api.v1.Core.ZedToken.Builder builderForValue) {
if (checkedAtBuilder_ == null) {
checkedAt_ = builderForValue.build();
} else {
checkedAtBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .authzed.api.v1.ZedToken checked_at = 1 [(.validate.rules) = { ... }
*/
public Builder mergeCheckedAt(com.authzed.api.v1.Core.ZedToken value) {
if (checkedAtBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0) &&
checkedAt_ != null &&
checkedAt_ != com.authzed.api.v1.Core.ZedToken.getDefaultInstance()) {
getCheckedAtBuilder().mergeFrom(value);
} else {
checkedAt_ = value;
}
} else {
checkedAtBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .authzed.api.v1.ZedToken checked_at = 1 [(.validate.rules) = { ... }
*/
public Builder clearCheckedAt() {
bitField0_ = (bitField0_ & ~0x00000001);
checkedAt_ = null;
if (checkedAtBuilder_ != null) {
checkedAtBuilder_.dispose();
checkedAtBuilder_ = null;
}
onChanged();
return this;
}
/**
* .authzed.api.v1.ZedToken checked_at = 1 [(.validate.rules) = { ... }
*/
public com.authzed.api.v1.Core.ZedToken.Builder getCheckedAtBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getCheckedAtFieldBuilder().getBuilder();
}
/**
* .authzed.api.v1.ZedToken checked_at = 1 [(.validate.rules) = { ... }
*/
public com.authzed.api.v1.Core.ZedTokenOrBuilder getCheckedAtOrBuilder() {
if (checkedAtBuilder_ != null) {
return checkedAtBuilder_.getMessageOrBuilder();
} else {
return checkedAt_ == null ?
com.authzed.api.v1.Core.ZedToken.getDefaultInstance() : checkedAt_;
}
}
/**
* .authzed.api.v1.ZedToken checked_at = 1 [(.validate.rules) = { ... }
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.ZedToken, com.authzed.api.v1.Core.ZedToken.Builder, com.authzed.api.v1.Core.ZedTokenOrBuilder>
getCheckedAtFieldBuilder() {
if (checkedAtBuilder_ == null) {
checkedAtBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.ZedToken, com.authzed.api.v1.Core.ZedToken.Builder, com.authzed.api.v1.Core.ZedTokenOrBuilder>(
getCheckedAt(),
getParentForChildren(),
isClean());
checkedAt_ = null;
}
return checkedAtBuilder_;
}
private int permissionship_ = 0;
/**
*
* Permissionship communicates whether or not the subject has the requested
* permission or has a relationship with the given resource, over the given
* relation.
* This value will be authzed.api.v1.PERMISSIONSHIP_HAS_PERMISSION if the
* requested subject is a member of the computed permission set or there
* exists a relationship with the requested relation from the given resource
* to the given subject.
*
*
* .authzed.api.v1.CheckPermissionResponse.Permissionship permissionship = 2 [(.validate.rules) = { ... }
* @return The enum numeric value on the wire for permissionship.
*/
@java.lang.Override public int getPermissionshipValue() {
return permissionship_;
}
/**
*
* Permissionship communicates whether or not the subject has the requested
* permission or has a relationship with the given resource, over the given
* relation.
* This value will be authzed.api.v1.PERMISSIONSHIP_HAS_PERMISSION if the
* requested subject is a member of the computed permission set or there
* exists a relationship with the requested relation from the given resource
* to the given subject.
*
*
* .authzed.api.v1.CheckPermissionResponse.Permissionship permissionship = 2 [(.validate.rules) = { ... }
* @param value The enum numeric value on the wire for permissionship to set.
* @return This builder for chaining.
*/
public Builder setPermissionshipValue(int value) {
permissionship_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* Permissionship communicates whether or not the subject has the requested
* permission or has a relationship with the given resource, over the given
* relation.
* This value will be authzed.api.v1.PERMISSIONSHIP_HAS_PERMISSION if the
* requested subject is a member of the computed permission set or there
* exists a relationship with the requested relation from the given resource
* to the given subject.
*
*
* .authzed.api.v1.CheckPermissionResponse.Permissionship permissionship = 2 [(.validate.rules) = { ... }
* @return The permissionship.
*/
@java.lang.Override
public com.authzed.api.v1.PermissionService.CheckPermissionResponse.Permissionship getPermissionship() {
com.authzed.api.v1.PermissionService.CheckPermissionResponse.Permissionship result = com.authzed.api.v1.PermissionService.CheckPermissionResponse.Permissionship.forNumber(permissionship_);
return result == null ? com.authzed.api.v1.PermissionService.CheckPermissionResponse.Permissionship.UNRECOGNIZED : result;
}
/**
*
* Permissionship communicates whether or not the subject has the requested
* permission or has a relationship with the given resource, over the given
* relation.
* This value will be authzed.api.v1.PERMISSIONSHIP_HAS_PERMISSION if the
* requested subject is a member of the computed permission set or there
* exists a relationship with the requested relation from the given resource
* to the given subject.
*
*
* .authzed.api.v1.CheckPermissionResponse.Permissionship permissionship = 2 [(.validate.rules) = { ... }
* @param value The permissionship to set.
* @return This builder for chaining.
*/
public Builder setPermissionship(com.authzed.api.v1.PermissionService.CheckPermissionResponse.Permissionship value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
permissionship_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Permissionship communicates whether or not the subject has the requested
* permission or has a relationship with the given resource, over the given
* relation.
* This value will be authzed.api.v1.PERMISSIONSHIP_HAS_PERMISSION if the
* requested subject is a member of the computed permission set or there
* exists a relationship with the requested relation from the given resource
* to the given subject.
*
*
* .authzed.api.v1.CheckPermissionResponse.Permissionship permissionship = 2 [(.validate.rules) = { ... }
* @return This builder for chaining.
*/
public Builder clearPermissionship() {
bitField0_ = (bitField0_ & ~0x00000002);
permissionship_ = 0;
onChanged();
return this;
}
private com.authzed.api.v1.Core.PartialCaveatInfo partialCaveatInfo_;
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.PartialCaveatInfo, com.authzed.api.v1.Core.PartialCaveatInfo.Builder, com.authzed.api.v1.Core.PartialCaveatInfoOrBuilder> partialCaveatInfoBuilder_;
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 3 [(.validate.rules) = { ... }
* @return Whether the partialCaveatInfo field is set.
*/
public boolean hasPartialCaveatInfo() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 3 [(.validate.rules) = { ... }
* @return The partialCaveatInfo.
*/
public com.authzed.api.v1.Core.PartialCaveatInfo getPartialCaveatInfo() {
if (partialCaveatInfoBuilder_ == null) {
return partialCaveatInfo_ == null ? com.authzed.api.v1.Core.PartialCaveatInfo.getDefaultInstance() : partialCaveatInfo_;
} else {
return partialCaveatInfoBuilder_.getMessage();
}
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 3 [(.validate.rules) = { ... }
*/
public Builder setPartialCaveatInfo(com.authzed.api.v1.Core.PartialCaveatInfo value) {
if (partialCaveatInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
partialCaveatInfo_ = value;
} else {
partialCaveatInfoBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 3 [(.validate.rules) = { ... }
*/
public Builder setPartialCaveatInfo(
com.authzed.api.v1.Core.PartialCaveatInfo.Builder builderForValue) {
if (partialCaveatInfoBuilder_ == null) {
partialCaveatInfo_ = builderForValue.build();
} else {
partialCaveatInfoBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 3 [(.validate.rules) = { ... }
*/
public Builder mergePartialCaveatInfo(com.authzed.api.v1.Core.PartialCaveatInfo value) {
if (partialCaveatInfoBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0) &&
partialCaveatInfo_ != null &&
partialCaveatInfo_ != com.authzed.api.v1.Core.PartialCaveatInfo.getDefaultInstance()) {
getPartialCaveatInfoBuilder().mergeFrom(value);
} else {
partialCaveatInfo_ = value;
}
} else {
partialCaveatInfoBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 3 [(.validate.rules) = { ... }
*/
public Builder clearPartialCaveatInfo() {
bitField0_ = (bitField0_ & ~0x00000004);
partialCaveatInfo_ = null;
if (partialCaveatInfoBuilder_ != null) {
partialCaveatInfoBuilder_.dispose();
partialCaveatInfoBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 3 [(.validate.rules) = { ... }
*/
public com.authzed.api.v1.Core.PartialCaveatInfo.Builder getPartialCaveatInfoBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getPartialCaveatInfoFieldBuilder().getBuilder();
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 3 [(.validate.rules) = { ... }
*/
public com.authzed.api.v1.Core.PartialCaveatInfoOrBuilder getPartialCaveatInfoOrBuilder() {
if (partialCaveatInfoBuilder_ != null) {
return partialCaveatInfoBuilder_.getMessageOrBuilder();
} else {
return partialCaveatInfo_ == null ?
com.authzed.api.v1.Core.PartialCaveatInfo.getDefaultInstance() : partialCaveatInfo_;
}
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 3 [(.validate.rules) = { ... }
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.PartialCaveatInfo, com.authzed.api.v1.Core.PartialCaveatInfo.Builder, com.authzed.api.v1.Core.PartialCaveatInfoOrBuilder>
getPartialCaveatInfoFieldBuilder() {
if (partialCaveatInfoBuilder_ == null) {
partialCaveatInfoBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.PartialCaveatInfo, com.authzed.api.v1.Core.PartialCaveatInfo.Builder, com.authzed.api.v1.Core.PartialCaveatInfoOrBuilder>(
getPartialCaveatInfo(),
getParentForChildren(),
isClean());
partialCaveatInfo_ = null;
}
return partialCaveatInfoBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:authzed.api.v1.CheckPermissionResponse)
}
// @@protoc_insertion_point(class_scope:authzed.api.v1.CheckPermissionResponse)
private static final com.authzed.api.v1.PermissionService.CheckPermissionResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.authzed.api.v1.PermissionService.CheckPermissionResponse();
}
public static com.authzed.api.v1.PermissionService.CheckPermissionResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CheckPermissionResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.CheckPermissionResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ExpandPermissionTreeRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:authzed.api.v1.ExpandPermissionTreeRequest)
com.google.protobuf.MessageOrBuilder {
/**
* .authzed.api.v1.Consistency consistency = 1;
* @return Whether the consistency field is set.
*/
boolean hasConsistency();
/**
* .authzed.api.v1.Consistency consistency = 1;
* @return The consistency.
*/
com.authzed.api.v1.PermissionService.Consistency getConsistency();
/**
* .authzed.api.v1.Consistency consistency = 1;
*/
com.authzed.api.v1.PermissionService.ConsistencyOrBuilder getConsistencyOrBuilder();
/**
*
* resource is the resource over which to run the expansion.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
* @return Whether the resource field is set.
*/
boolean hasResource();
/**
*
* resource is the resource over which to run the expansion.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
* @return The resource.
*/
com.authzed.api.v1.Core.ObjectReference getResource();
/**
*
* resource is the resource over which to run the expansion.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
*/
com.authzed.api.v1.Core.ObjectReferenceOrBuilder getResourceOrBuilder();
/**
*
* permission is the name of the permission or relation over which to run the
* expansion for the resource.
*
*
* string permission = 3 [(.validate.rules) = { ... }
* @return The permission.
*/
java.lang.String getPermission();
/**
*
* permission is the name of the permission or relation over which to run the
* expansion for the resource.
*
*
* string permission = 3 [(.validate.rules) = { ... }
* @return The bytes for permission.
*/
com.google.protobuf.ByteString
getPermissionBytes();
}
/**
*
* ExpandPermissionTreeRequest returns a tree representing the expansion of all
* relationships found accessible from a permission or relation on a particular
* resource.
* ExpandPermissionTreeRequest is typically used to determine the full set of
* subjects with a permission, along with the relationships that grant said
* access.
*
*
* Protobuf type {@code authzed.api.v1.ExpandPermissionTreeRequest}
*/
public static final class ExpandPermissionTreeRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:authzed.api.v1.ExpandPermissionTreeRequest)
ExpandPermissionTreeRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use ExpandPermissionTreeRequest.newBuilder() to construct.
private ExpandPermissionTreeRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ExpandPermissionTreeRequest() {
permission_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ExpandPermissionTreeRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_ExpandPermissionTreeRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_ExpandPermissionTreeRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.PermissionService.ExpandPermissionTreeRequest.class, com.authzed.api.v1.PermissionService.ExpandPermissionTreeRequest.Builder.class);
}
public static final int CONSISTENCY_FIELD_NUMBER = 1;
private com.authzed.api.v1.PermissionService.Consistency consistency_;
/**
* .authzed.api.v1.Consistency consistency = 1;
* @return Whether the consistency field is set.
*/
@java.lang.Override
public boolean hasConsistency() {
return consistency_ != null;
}
/**
* .authzed.api.v1.Consistency consistency = 1;
* @return The consistency.
*/
@java.lang.Override
public com.authzed.api.v1.PermissionService.Consistency getConsistency() {
return consistency_ == null ? com.authzed.api.v1.PermissionService.Consistency.getDefaultInstance() : consistency_;
}
/**
* .authzed.api.v1.Consistency consistency = 1;
*/
@java.lang.Override
public com.authzed.api.v1.PermissionService.ConsistencyOrBuilder getConsistencyOrBuilder() {
return consistency_ == null ? com.authzed.api.v1.PermissionService.Consistency.getDefaultInstance() : consistency_;
}
public static final int RESOURCE_FIELD_NUMBER = 2;
private com.authzed.api.v1.Core.ObjectReference resource_;
/**
*
* resource is the resource over which to run the expansion.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
* @return Whether the resource field is set.
*/
@java.lang.Override
public boolean hasResource() {
return resource_ != null;
}
/**
*
* resource is the resource over which to run the expansion.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
* @return The resource.
*/
@java.lang.Override
public com.authzed.api.v1.Core.ObjectReference getResource() {
return resource_ == null ? com.authzed.api.v1.Core.ObjectReference.getDefaultInstance() : resource_;
}
/**
*
* resource is the resource over which to run the expansion.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
*/
@java.lang.Override
public com.authzed.api.v1.Core.ObjectReferenceOrBuilder getResourceOrBuilder() {
return resource_ == null ? com.authzed.api.v1.Core.ObjectReference.getDefaultInstance() : resource_;
}
public static final int PERMISSION_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private volatile java.lang.Object permission_ = "";
/**
*
* permission is the name of the permission or relation over which to run the
* expansion for the resource.
*
*
* string permission = 3 [(.validate.rules) = { ... }
* @return The permission.
*/
@java.lang.Override
public java.lang.String getPermission() {
java.lang.Object ref = permission_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
permission_ = s;
return s;
}
}
/**
*
* permission is the name of the permission or relation over which to run the
* expansion for the resource.
*
*
* string permission = 3 [(.validate.rules) = { ... }
* @return The bytes for permission.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getPermissionBytes() {
java.lang.Object ref = permission_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
permission_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (consistency_ != null) {
output.writeMessage(1, getConsistency());
}
if (resource_ != null) {
output.writeMessage(2, getResource());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(permission_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, permission_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (consistency_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getConsistency());
}
if (resource_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getResource());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(permission_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, permission_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.authzed.api.v1.PermissionService.ExpandPermissionTreeRequest)) {
return super.equals(obj);
}
com.authzed.api.v1.PermissionService.ExpandPermissionTreeRequest other = (com.authzed.api.v1.PermissionService.ExpandPermissionTreeRequest) obj;
if (hasConsistency() != other.hasConsistency()) return false;
if (hasConsistency()) {
if (!getConsistency()
.equals(other.getConsistency())) return false;
}
if (hasResource() != other.hasResource()) return false;
if (hasResource()) {
if (!getResource()
.equals(other.getResource())) return false;
}
if (!getPermission()
.equals(other.getPermission())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasConsistency()) {
hash = (37 * hash) + CONSISTENCY_FIELD_NUMBER;
hash = (53 * hash) + getConsistency().hashCode();
}
if (hasResource()) {
hash = (37 * hash) + RESOURCE_FIELD_NUMBER;
hash = (53 * hash) + getResource().hashCode();
}
hash = (37 * hash) + PERMISSION_FIELD_NUMBER;
hash = (53 * hash) + getPermission().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.authzed.api.v1.PermissionService.ExpandPermissionTreeRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.ExpandPermissionTreeRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.ExpandPermissionTreeRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.ExpandPermissionTreeRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.ExpandPermissionTreeRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.ExpandPermissionTreeRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.ExpandPermissionTreeRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.ExpandPermissionTreeRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.ExpandPermissionTreeRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.ExpandPermissionTreeRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.ExpandPermissionTreeRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.ExpandPermissionTreeRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.authzed.api.v1.PermissionService.ExpandPermissionTreeRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* ExpandPermissionTreeRequest returns a tree representing the expansion of all
* relationships found accessible from a permission or relation on a particular
* resource.
* ExpandPermissionTreeRequest is typically used to determine the full set of
* subjects with a permission, along with the relationships that grant said
* access.
*
*
* Protobuf type {@code authzed.api.v1.ExpandPermissionTreeRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:authzed.api.v1.ExpandPermissionTreeRequest)
com.authzed.api.v1.PermissionService.ExpandPermissionTreeRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_ExpandPermissionTreeRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_ExpandPermissionTreeRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.PermissionService.ExpandPermissionTreeRequest.class, com.authzed.api.v1.PermissionService.ExpandPermissionTreeRequest.Builder.class);
}
// Construct using com.authzed.api.v1.PermissionService.ExpandPermissionTreeRequest.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
consistency_ = null;
if (consistencyBuilder_ != null) {
consistencyBuilder_.dispose();
consistencyBuilder_ = null;
}
resource_ = null;
if (resourceBuilder_ != null) {
resourceBuilder_.dispose();
resourceBuilder_ = null;
}
permission_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_ExpandPermissionTreeRequest_descriptor;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.ExpandPermissionTreeRequest getDefaultInstanceForType() {
return com.authzed.api.v1.PermissionService.ExpandPermissionTreeRequest.getDefaultInstance();
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.ExpandPermissionTreeRequest build() {
com.authzed.api.v1.PermissionService.ExpandPermissionTreeRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.ExpandPermissionTreeRequest buildPartial() {
com.authzed.api.v1.PermissionService.ExpandPermissionTreeRequest result = new com.authzed.api.v1.PermissionService.ExpandPermissionTreeRequest(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.authzed.api.v1.PermissionService.ExpandPermissionTreeRequest result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.consistency_ = consistencyBuilder_ == null
? consistency_
: consistencyBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.resource_ = resourceBuilder_ == null
? resource_
: resourceBuilder_.build();
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.permission_ = permission_;
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.authzed.api.v1.PermissionService.ExpandPermissionTreeRequest) {
return mergeFrom((com.authzed.api.v1.PermissionService.ExpandPermissionTreeRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.authzed.api.v1.PermissionService.ExpandPermissionTreeRequest other) {
if (other == com.authzed.api.v1.PermissionService.ExpandPermissionTreeRequest.getDefaultInstance()) return this;
if (other.hasConsistency()) {
mergeConsistency(other.getConsistency());
}
if (other.hasResource()) {
mergeResource(other.getResource());
}
if (!other.getPermission().isEmpty()) {
permission_ = other.permission_;
bitField0_ |= 0x00000004;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
input.readMessage(
getConsistencyFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
input.readMessage(
getResourceFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000002;
break;
} // case 18
case 26: {
permission_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000004;
break;
} // case 26
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.authzed.api.v1.PermissionService.Consistency consistency_;
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.PermissionService.Consistency, com.authzed.api.v1.PermissionService.Consistency.Builder, com.authzed.api.v1.PermissionService.ConsistencyOrBuilder> consistencyBuilder_;
/**
* .authzed.api.v1.Consistency consistency = 1;
* @return Whether the consistency field is set.
*/
public boolean hasConsistency() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* .authzed.api.v1.Consistency consistency = 1;
* @return The consistency.
*/
public com.authzed.api.v1.PermissionService.Consistency getConsistency() {
if (consistencyBuilder_ == null) {
return consistency_ == null ? com.authzed.api.v1.PermissionService.Consistency.getDefaultInstance() : consistency_;
} else {
return consistencyBuilder_.getMessage();
}
}
/**
* .authzed.api.v1.Consistency consistency = 1;
*/
public Builder setConsistency(com.authzed.api.v1.PermissionService.Consistency value) {
if (consistencyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
consistency_ = value;
} else {
consistencyBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .authzed.api.v1.Consistency consistency = 1;
*/
public Builder setConsistency(
com.authzed.api.v1.PermissionService.Consistency.Builder builderForValue) {
if (consistencyBuilder_ == null) {
consistency_ = builderForValue.build();
} else {
consistencyBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .authzed.api.v1.Consistency consistency = 1;
*/
public Builder mergeConsistency(com.authzed.api.v1.PermissionService.Consistency value) {
if (consistencyBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0) &&
consistency_ != null &&
consistency_ != com.authzed.api.v1.PermissionService.Consistency.getDefaultInstance()) {
getConsistencyBuilder().mergeFrom(value);
} else {
consistency_ = value;
}
} else {
consistencyBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .authzed.api.v1.Consistency consistency = 1;
*/
public Builder clearConsistency() {
bitField0_ = (bitField0_ & ~0x00000001);
consistency_ = null;
if (consistencyBuilder_ != null) {
consistencyBuilder_.dispose();
consistencyBuilder_ = null;
}
onChanged();
return this;
}
/**
* .authzed.api.v1.Consistency consistency = 1;
*/
public com.authzed.api.v1.PermissionService.Consistency.Builder getConsistencyBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getConsistencyFieldBuilder().getBuilder();
}
/**
* .authzed.api.v1.Consistency consistency = 1;
*/
public com.authzed.api.v1.PermissionService.ConsistencyOrBuilder getConsistencyOrBuilder() {
if (consistencyBuilder_ != null) {
return consistencyBuilder_.getMessageOrBuilder();
} else {
return consistency_ == null ?
com.authzed.api.v1.PermissionService.Consistency.getDefaultInstance() : consistency_;
}
}
/**
* .authzed.api.v1.Consistency consistency = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.PermissionService.Consistency, com.authzed.api.v1.PermissionService.Consistency.Builder, com.authzed.api.v1.PermissionService.ConsistencyOrBuilder>
getConsistencyFieldBuilder() {
if (consistencyBuilder_ == null) {
consistencyBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.PermissionService.Consistency, com.authzed.api.v1.PermissionService.Consistency.Builder, com.authzed.api.v1.PermissionService.ConsistencyOrBuilder>(
getConsistency(),
getParentForChildren(),
isClean());
consistency_ = null;
}
return consistencyBuilder_;
}
private com.authzed.api.v1.Core.ObjectReference resource_;
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.ObjectReference, com.authzed.api.v1.Core.ObjectReference.Builder, com.authzed.api.v1.Core.ObjectReferenceOrBuilder> resourceBuilder_;
/**
*
* resource is the resource over which to run the expansion.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
* @return Whether the resource field is set.
*/
public boolean hasResource() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* resource is the resource over which to run the expansion.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
* @return The resource.
*/
public com.authzed.api.v1.Core.ObjectReference getResource() {
if (resourceBuilder_ == null) {
return resource_ == null ? com.authzed.api.v1.Core.ObjectReference.getDefaultInstance() : resource_;
} else {
return resourceBuilder_.getMessage();
}
}
/**
*
* resource is the resource over which to run the expansion.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
*/
public Builder setResource(com.authzed.api.v1.Core.ObjectReference value) {
if (resourceBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
resource_ = value;
} else {
resourceBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* resource is the resource over which to run the expansion.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
*/
public Builder setResource(
com.authzed.api.v1.Core.ObjectReference.Builder builderForValue) {
if (resourceBuilder_ == null) {
resource_ = builderForValue.build();
} else {
resourceBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* resource is the resource over which to run the expansion.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
*/
public Builder mergeResource(com.authzed.api.v1.Core.ObjectReference value) {
if (resourceBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0) &&
resource_ != null &&
resource_ != com.authzed.api.v1.Core.ObjectReference.getDefaultInstance()) {
getResourceBuilder().mergeFrom(value);
} else {
resource_ = value;
}
} else {
resourceBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* resource is the resource over which to run the expansion.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
*/
public Builder clearResource() {
bitField0_ = (bitField0_ & ~0x00000002);
resource_ = null;
if (resourceBuilder_ != null) {
resourceBuilder_.dispose();
resourceBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* resource is the resource over which to run the expansion.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
*/
public com.authzed.api.v1.Core.ObjectReference.Builder getResourceBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getResourceFieldBuilder().getBuilder();
}
/**
*
* resource is the resource over which to run the expansion.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
*/
public com.authzed.api.v1.Core.ObjectReferenceOrBuilder getResourceOrBuilder() {
if (resourceBuilder_ != null) {
return resourceBuilder_.getMessageOrBuilder();
} else {
return resource_ == null ?
com.authzed.api.v1.Core.ObjectReference.getDefaultInstance() : resource_;
}
}
/**
*
* resource is the resource over which to run the expansion.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.ObjectReference, com.authzed.api.v1.Core.ObjectReference.Builder, com.authzed.api.v1.Core.ObjectReferenceOrBuilder>
getResourceFieldBuilder() {
if (resourceBuilder_ == null) {
resourceBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.ObjectReference, com.authzed.api.v1.Core.ObjectReference.Builder, com.authzed.api.v1.Core.ObjectReferenceOrBuilder>(
getResource(),
getParentForChildren(),
isClean());
resource_ = null;
}
return resourceBuilder_;
}
private java.lang.Object permission_ = "";
/**
*
* permission is the name of the permission or relation over which to run the
* expansion for the resource.
*
*
* string permission = 3 [(.validate.rules) = { ... }
* @return The permission.
*/
public java.lang.String getPermission() {
java.lang.Object ref = permission_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
permission_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* permission is the name of the permission or relation over which to run the
* expansion for the resource.
*
*
* string permission = 3 [(.validate.rules) = { ... }
* @return The bytes for permission.
*/
public com.google.protobuf.ByteString
getPermissionBytes() {
java.lang.Object ref = permission_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
permission_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* permission is the name of the permission or relation over which to run the
* expansion for the resource.
*
*
* string permission = 3 [(.validate.rules) = { ... }
* @param value The permission to set.
* @return This builder for chaining.
*/
public Builder setPermission(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
permission_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* permission is the name of the permission or relation over which to run the
* expansion for the resource.
*
*
* string permission = 3 [(.validate.rules) = { ... }
* @return This builder for chaining.
*/
public Builder clearPermission() {
permission_ = getDefaultInstance().getPermission();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
*
* permission is the name of the permission or relation over which to run the
* expansion for the resource.
*
*
* string permission = 3 [(.validate.rules) = { ... }
* @param value The bytes for permission to set.
* @return This builder for chaining.
*/
public Builder setPermissionBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
permission_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:authzed.api.v1.ExpandPermissionTreeRequest)
}
// @@protoc_insertion_point(class_scope:authzed.api.v1.ExpandPermissionTreeRequest)
private static final com.authzed.api.v1.PermissionService.ExpandPermissionTreeRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.authzed.api.v1.PermissionService.ExpandPermissionTreeRequest();
}
public static com.authzed.api.v1.PermissionService.ExpandPermissionTreeRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ExpandPermissionTreeRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.ExpandPermissionTreeRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ExpandPermissionTreeResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:authzed.api.v1.ExpandPermissionTreeResponse)
com.google.protobuf.MessageOrBuilder {
/**
* .authzed.api.v1.ZedToken expanded_at = 1;
* @return Whether the expandedAt field is set.
*/
boolean hasExpandedAt();
/**
* .authzed.api.v1.ZedToken expanded_at = 1;
* @return The expandedAt.
*/
com.authzed.api.v1.Core.ZedToken getExpandedAt();
/**
* .authzed.api.v1.ZedToken expanded_at = 1;
*/
com.authzed.api.v1.Core.ZedTokenOrBuilder getExpandedAtOrBuilder();
/**
*
* tree_root is a tree structure whose leaf nodes are subjects, and
* intermediate nodes represent the various operations (union, intersection,
* exclusion) to reach those subjects.
*
*
* .authzed.api.v1.PermissionRelationshipTree tree_root = 2;
* @return Whether the treeRoot field is set.
*/
boolean hasTreeRoot();
/**
*
* tree_root is a tree structure whose leaf nodes are subjects, and
* intermediate nodes represent the various operations (union, intersection,
* exclusion) to reach those subjects.
*
*
* .authzed.api.v1.PermissionRelationshipTree tree_root = 2;
* @return The treeRoot.
*/
com.authzed.api.v1.Core.PermissionRelationshipTree getTreeRoot();
/**
*
* tree_root is a tree structure whose leaf nodes are subjects, and
* intermediate nodes represent the various operations (union, intersection,
* exclusion) to reach those subjects.
*
*
* .authzed.api.v1.PermissionRelationshipTree tree_root = 2;
*/
com.authzed.api.v1.Core.PermissionRelationshipTreeOrBuilder getTreeRootOrBuilder();
}
/**
* Protobuf type {@code authzed.api.v1.ExpandPermissionTreeResponse}
*/
public static final class ExpandPermissionTreeResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:authzed.api.v1.ExpandPermissionTreeResponse)
ExpandPermissionTreeResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use ExpandPermissionTreeResponse.newBuilder() to construct.
private ExpandPermissionTreeResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ExpandPermissionTreeResponse() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ExpandPermissionTreeResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_ExpandPermissionTreeResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_ExpandPermissionTreeResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.PermissionService.ExpandPermissionTreeResponse.class, com.authzed.api.v1.PermissionService.ExpandPermissionTreeResponse.Builder.class);
}
public static final int EXPANDED_AT_FIELD_NUMBER = 1;
private com.authzed.api.v1.Core.ZedToken expandedAt_;
/**
* .authzed.api.v1.ZedToken expanded_at = 1;
* @return Whether the expandedAt field is set.
*/
@java.lang.Override
public boolean hasExpandedAt() {
return expandedAt_ != null;
}
/**
* .authzed.api.v1.ZedToken expanded_at = 1;
* @return The expandedAt.
*/
@java.lang.Override
public com.authzed.api.v1.Core.ZedToken getExpandedAt() {
return expandedAt_ == null ? com.authzed.api.v1.Core.ZedToken.getDefaultInstance() : expandedAt_;
}
/**
* .authzed.api.v1.ZedToken expanded_at = 1;
*/
@java.lang.Override
public com.authzed.api.v1.Core.ZedTokenOrBuilder getExpandedAtOrBuilder() {
return expandedAt_ == null ? com.authzed.api.v1.Core.ZedToken.getDefaultInstance() : expandedAt_;
}
public static final int TREE_ROOT_FIELD_NUMBER = 2;
private com.authzed.api.v1.Core.PermissionRelationshipTree treeRoot_;
/**
*
* tree_root is a tree structure whose leaf nodes are subjects, and
* intermediate nodes represent the various operations (union, intersection,
* exclusion) to reach those subjects.
*
*
* .authzed.api.v1.PermissionRelationshipTree tree_root = 2;
* @return Whether the treeRoot field is set.
*/
@java.lang.Override
public boolean hasTreeRoot() {
return treeRoot_ != null;
}
/**
*
* tree_root is a tree structure whose leaf nodes are subjects, and
* intermediate nodes represent the various operations (union, intersection,
* exclusion) to reach those subjects.
*
*
* .authzed.api.v1.PermissionRelationshipTree tree_root = 2;
* @return The treeRoot.
*/
@java.lang.Override
public com.authzed.api.v1.Core.PermissionRelationshipTree getTreeRoot() {
return treeRoot_ == null ? com.authzed.api.v1.Core.PermissionRelationshipTree.getDefaultInstance() : treeRoot_;
}
/**
*
* tree_root is a tree structure whose leaf nodes are subjects, and
* intermediate nodes represent the various operations (union, intersection,
* exclusion) to reach those subjects.
*
*
* .authzed.api.v1.PermissionRelationshipTree tree_root = 2;
*/
@java.lang.Override
public com.authzed.api.v1.Core.PermissionRelationshipTreeOrBuilder getTreeRootOrBuilder() {
return treeRoot_ == null ? com.authzed.api.v1.Core.PermissionRelationshipTree.getDefaultInstance() : treeRoot_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (expandedAt_ != null) {
output.writeMessage(1, getExpandedAt());
}
if (treeRoot_ != null) {
output.writeMessage(2, getTreeRoot());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (expandedAt_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getExpandedAt());
}
if (treeRoot_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getTreeRoot());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.authzed.api.v1.PermissionService.ExpandPermissionTreeResponse)) {
return super.equals(obj);
}
com.authzed.api.v1.PermissionService.ExpandPermissionTreeResponse other = (com.authzed.api.v1.PermissionService.ExpandPermissionTreeResponse) obj;
if (hasExpandedAt() != other.hasExpandedAt()) return false;
if (hasExpandedAt()) {
if (!getExpandedAt()
.equals(other.getExpandedAt())) return false;
}
if (hasTreeRoot() != other.hasTreeRoot()) return false;
if (hasTreeRoot()) {
if (!getTreeRoot()
.equals(other.getTreeRoot())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasExpandedAt()) {
hash = (37 * hash) + EXPANDED_AT_FIELD_NUMBER;
hash = (53 * hash) + getExpandedAt().hashCode();
}
if (hasTreeRoot()) {
hash = (37 * hash) + TREE_ROOT_FIELD_NUMBER;
hash = (53 * hash) + getTreeRoot().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.authzed.api.v1.PermissionService.ExpandPermissionTreeResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.ExpandPermissionTreeResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.ExpandPermissionTreeResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.ExpandPermissionTreeResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.ExpandPermissionTreeResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.ExpandPermissionTreeResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.ExpandPermissionTreeResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.ExpandPermissionTreeResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.ExpandPermissionTreeResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.ExpandPermissionTreeResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.ExpandPermissionTreeResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.ExpandPermissionTreeResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.authzed.api.v1.PermissionService.ExpandPermissionTreeResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code authzed.api.v1.ExpandPermissionTreeResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:authzed.api.v1.ExpandPermissionTreeResponse)
com.authzed.api.v1.PermissionService.ExpandPermissionTreeResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_ExpandPermissionTreeResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_ExpandPermissionTreeResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.PermissionService.ExpandPermissionTreeResponse.class, com.authzed.api.v1.PermissionService.ExpandPermissionTreeResponse.Builder.class);
}
// Construct using com.authzed.api.v1.PermissionService.ExpandPermissionTreeResponse.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
expandedAt_ = null;
if (expandedAtBuilder_ != null) {
expandedAtBuilder_.dispose();
expandedAtBuilder_ = null;
}
treeRoot_ = null;
if (treeRootBuilder_ != null) {
treeRootBuilder_.dispose();
treeRootBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_ExpandPermissionTreeResponse_descriptor;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.ExpandPermissionTreeResponse getDefaultInstanceForType() {
return com.authzed.api.v1.PermissionService.ExpandPermissionTreeResponse.getDefaultInstance();
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.ExpandPermissionTreeResponse build() {
com.authzed.api.v1.PermissionService.ExpandPermissionTreeResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.ExpandPermissionTreeResponse buildPartial() {
com.authzed.api.v1.PermissionService.ExpandPermissionTreeResponse result = new com.authzed.api.v1.PermissionService.ExpandPermissionTreeResponse(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.authzed.api.v1.PermissionService.ExpandPermissionTreeResponse result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.expandedAt_ = expandedAtBuilder_ == null
? expandedAt_
: expandedAtBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.treeRoot_ = treeRootBuilder_ == null
? treeRoot_
: treeRootBuilder_.build();
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.authzed.api.v1.PermissionService.ExpandPermissionTreeResponse) {
return mergeFrom((com.authzed.api.v1.PermissionService.ExpandPermissionTreeResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.authzed.api.v1.PermissionService.ExpandPermissionTreeResponse other) {
if (other == com.authzed.api.v1.PermissionService.ExpandPermissionTreeResponse.getDefaultInstance()) return this;
if (other.hasExpandedAt()) {
mergeExpandedAt(other.getExpandedAt());
}
if (other.hasTreeRoot()) {
mergeTreeRoot(other.getTreeRoot());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
input.readMessage(
getExpandedAtFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
input.readMessage(
getTreeRootFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000002;
break;
} // case 18
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.authzed.api.v1.Core.ZedToken expandedAt_;
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.ZedToken, com.authzed.api.v1.Core.ZedToken.Builder, com.authzed.api.v1.Core.ZedTokenOrBuilder> expandedAtBuilder_;
/**
* .authzed.api.v1.ZedToken expanded_at = 1;
* @return Whether the expandedAt field is set.
*/
public boolean hasExpandedAt() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* .authzed.api.v1.ZedToken expanded_at = 1;
* @return The expandedAt.
*/
public com.authzed.api.v1.Core.ZedToken getExpandedAt() {
if (expandedAtBuilder_ == null) {
return expandedAt_ == null ? com.authzed.api.v1.Core.ZedToken.getDefaultInstance() : expandedAt_;
} else {
return expandedAtBuilder_.getMessage();
}
}
/**
* .authzed.api.v1.ZedToken expanded_at = 1;
*/
public Builder setExpandedAt(com.authzed.api.v1.Core.ZedToken value) {
if (expandedAtBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
expandedAt_ = value;
} else {
expandedAtBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .authzed.api.v1.ZedToken expanded_at = 1;
*/
public Builder setExpandedAt(
com.authzed.api.v1.Core.ZedToken.Builder builderForValue) {
if (expandedAtBuilder_ == null) {
expandedAt_ = builderForValue.build();
} else {
expandedAtBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .authzed.api.v1.ZedToken expanded_at = 1;
*/
public Builder mergeExpandedAt(com.authzed.api.v1.Core.ZedToken value) {
if (expandedAtBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0) &&
expandedAt_ != null &&
expandedAt_ != com.authzed.api.v1.Core.ZedToken.getDefaultInstance()) {
getExpandedAtBuilder().mergeFrom(value);
} else {
expandedAt_ = value;
}
} else {
expandedAtBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .authzed.api.v1.ZedToken expanded_at = 1;
*/
public Builder clearExpandedAt() {
bitField0_ = (bitField0_ & ~0x00000001);
expandedAt_ = null;
if (expandedAtBuilder_ != null) {
expandedAtBuilder_.dispose();
expandedAtBuilder_ = null;
}
onChanged();
return this;
}
/**
* .authzed.api.v1.ZedToken expanded_at = 1;
*/
public com.authzed.api.v1.Core.ZedToken.Builder getExpandedAtBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getExpandedAtFieldBuilder().getBuilder();
}
/**
* .authzed.api.v1.ZedToken expanded_at = 1;
*/
public com.authzed.api.v1.Core.ZedTokenOrBuilder getExpandedAtOrBuilder() {
if (expandedAtBuilder_ != null) {
return expandedAtBuilder_.getMessageOrBuilder();
} else {
return expandedAt_ == null ?
com.authzed.api.v1.Core.ZedToken.getDefaultInstance() : expandedAt_;
}
}
/**
* .authzed.api.v1.ZedToken expanded_at = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.ZedToken, com.authzed.api.v1.Core.ZedToken.Builder, com.authzed.api.v1.Core.ZedTokenOrBuilder>
getExpandedAtFieldBuilder() {
if (expandedAtBuilder_ == null) {
expandedAtBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.ZedToken, com.authzed.api.v1.Core.ZedToken.Builder, com.authzed.api.v1.Core.ZedTokenOrBuilder>(
getExpandedAt(),
getParentForChildren(),
isClean());
expandedAt_ = null;
}
return expandedAtBuilder_;
}
private com.authzed.api.v1.Core.PermissionRelationshipTree treeRoot_;
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.PermissionRelationshipTree, com.authzed.api.v1.Core.PermissionRelationshipTree.Builder, com.authzed.api.v1.Core.PermissionRelationshipTreeOrBuilder> treeRootBuilder_;
/**
*
* tree_root is a tree structure whose leaf nodes are subjects, and
* intermediate nodes represent the various operations (union, intersection,
* exclusion) to reach those subjects.
*
*
* .authzed.api.v1.PermissionRelationshipTree tree_root = 2;
* @return Whether the treeRoot field is set.
*/
public boolean hasTreeRoot() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* tree_root is a tree structure whose leaf nodes are subjects, and
* intermediate nodes represent the various operations (union, intersection,
* exclusion) to reach those subjects.
*
*
* .authzed.api.v1.PermissionRelationshipTree tree_root = 2;
* @return The treeRoot.
*/
public com.authzed.api.v1.Core.PermissionRelationshipTree getTreeRoot() {
if (treeRootBuilder_ == null) {
return treeRoot_ == null ? com.authzed.api.v1.Core.PermissionRelationshipTree.getDefaultInstance() : treeRoot_;
} else {
return treeRootBuilder_.getMessage();
}
}
/**
*
* tree_root is a tree structure whose leaf nodes are subjects, and
* intermediate nodes represent the various operations (union, intersection,
* exclusion) to reach those subjects.
*
*
* .authzed.api.v1.PermissionRelationshipTree tree_root = 2;
*/
public Builder setTreeRoot(com.authzed.api.v1.Core.PermissionRelationshipTree value) {
if (treeRootBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
treeRoot_ = value;
} else {
treeRootBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* tree_root is a tree structure whose leaf nodes are subjects, and
* intermediate nodes represent the various operations (union, intersection,
* exclusion) to reach those subjects.
*
*
* .authzed.api.v1.PermissionRelationshipTree tree_root = 2;
*/
public Builder setTreeRoot(
com.authzed.api.v1.Core.PermissionRelationshipTree.Builder builderForValue) {
if (treeRootBuilder_ == null) {
treeRoot_ = builderForValue.build();
} else {
treeRootBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* tree_root is a tree structure whose leaf nodes are subjects, and
* intermediate nodes represent the various operations (union, intersection,
* exclusion) to reach those subjects.
*
*
* .authzed.api.v1.PermissionRelationshipTree tree_root = 2;
*/
public Builder mergeTreeRoot(com.authzed.api.v1.Core.PermissionRelationshipTree value) {
if (treeRootBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0) &&
treeRoot_ != null &&
treeRoot_ != com.authzed.api.v1.Core.PermissionRelationshipTree.getDefaultInstance()) {
getTreeRootBuilder().mergeFrom(value);
} else {
treeRoot_ = value;
}
} else {
treeRootBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* tree_root is a tree structure whose leaf nodes are subjects, and
* intermediate nodes represent the various operations (union, intersection,
* exclusion) to reach those subjects.
*
*
* .authzed.api.v1.PermissionRelationshipTree tree_root = 2;
*/
public Builder clearTreeRoot() {
bitField0_ = (bitField0_ & ~0x00000002);
treeRoot_ = null;
if (treeRootBuilder_ != null) {
treeRootBuilder_.dispose();
treeRootBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* tree_root is a tree structure whose leaf nodes are subjects, and
* intermediate nodes represent the various operations (union, intersection,
* exclusion) to reach those subjects.
*
*
* .authzed.api.v1.PermissionRelationshipTree tree_root = 2;
*/
public com.authzed.api.v1.Core.PermissionRelationshipTree.Builder getTreeRootBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getTreeRootFieldBuilder().getBuilder();
}
/**
*
* tree_root is a tree structure whose leaf nodes are subjects, and
* intermediate nodes represent the various operations (union, intersection,
* exclusion) to reach those subjects.
*
*
* .authzed.api.v1.PermissionRelationshipTree tree_root = 2;
*/
public com.authzed.api.v1.Core.PermissionRelationshipTreeOrBuilder getTreeRootOrBuilder() {
if (treeRootBuilder_ != null) {
return treeRootBuilder_.getMessageOrBuilder();
} else {
return treeRoot_ == null ?
com.authzed.api.v1.Core.PermissionRelationshipTree.getDefaultInstance() : treeRoot_;
}
}
/**
*
* tree_root is a tree structure whose leaf nodes are subjects, and
* intermediate nodes represent the various operations (union, intersection,
* exclusion) to reach those subjects.
*
*
* .authzed.api.v1.PermissionRelationshipTree tree_root = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.PermissionRelationshipTree, com.authzed.api.v1.Core.PermissionRelationshipTree.Builder, com.authzed.api.v1.Core.PermissionRelationshipTreeOrBuilder>
getTreeRootFieldBuilder() {
if (treeRootBuilder_ == null) {
treeRootBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.PermissionRelationshipTree, com.authzed.api.v1.Core.PermissionRelationshipTree.Builder, com.authzed.api.v1.Core.PermissionRelationshipTreeOrBuilder>(
getTreeRoot(),
getParentForChildren(),
isClean());
treeRoot_ = null;
}
return treeRootBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:authzed.api.v1.ExpandPermissionTreeResponse)
}
// @@protoc_insertion_point(class_scope:authzed.api.v1.ExpandPermissionTreeResponse)
private static final com.authzed.api.v1.PermissionService.ExpandPermissionTreeResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.authzed.api.v1.PermissionService.ExpandPermissionTreeResponse();
}
public static com.authzed.api.v1.PermissionService.ExpandPermissionTreeResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ExpandPermissionTreeResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.ExpandPermissionTreeResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface LookupResourcesRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:authzed.api.v1.LookupResourcesRequest)
com.google.protobuf.MessageOrBuilder {
/**
* .authzed.api.v1.Consistency consistency = 1;
* @return Whether the consistency field is set.
*/
boolean hasConsistency();
/**
* .authzed.api.v1.Consistency consistency = 1;
* @return The consistency.
*/
com.authzed.api.v1.PermissionService.Consistency getConsistency();
/**
* .authzed.api.v1.Consistency consistency = 1;
*/
com.authzed.api.v1.PermissionService.ConsistencyOrBuilder getConsistencyOrBuilder();
/**
*
* resource_object_type is the type of resource object for which the IDs will
* be returned.
*
*
* string resource_object_type = 2 [(.validate.rules) = { ... }
* @return The resourceObjectType.
*/
java.lang.String getResourceObjectType();
/**
*
* resource_object_type is the type of resource object for which the IDs will
* be returned.
*
*
* string resource_object_type = 2 [(.validate.rules) = { ... }
* @return The bytes for resourceObjectType.
*/
com.google.protobuf.ByteString
getResourceObjectTypeBytes();
/**
*
* permission is the name of the permission or relation for which the subject
* must Check.
*
*
* string permission = 3 [(.validate.rules) = { ... }
* @return The permission.
*/
java.lang.String getPermission();
/**
*
* permission is the name of the permission or relation for which the subject
* must Check.
*
*
* string permission = 3 [(.validate.rules) = { ... }
* @return The bytes for permission.
*/
com.google.protobuf.ByteString
getPermissionBytes();
/**
*
* subject is the subject with access to the resources.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
* @return Whether the subject field is set.
*/
boolean hasSubject();
/**
*
* subject is the subject with access to the resources.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
* @return The subject.
*/
com.authzed.api.v1.Core.SubjectReference getSubject();
/**
*
* subject is the subject with access to the resources.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
*/
com.authzed.api.v1.Core.SubjectReferenceOrBuilder getSubjectOrBuilder();
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 5 [(.validate.rules) = { ... }
* @return Whether the context field is set.
*/
boolean hasContext();
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 5 [(.validate.rules) = { ... }
* @return The context.
*/
com.google.protobuf.Struct getContext();
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 5 [(.validate.rules) = { ... }
*/
com.google.protobuf.StructOrBuilder getContextOrBuilder();
}
/**
*
* LookupResourcesRequest performs a lookup of all resources of a particular
* kind on which the subject has the specified permission or the relation in
* which the subject exists, streaming back the IDs of those resources.
*
*
* Protobuf type {@code authzed.api.v1.LookupResourcesRequest}
*/
public static final class LookupResourcesRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:authzed.api.v1.LookupResourcesRequest)
LookupResourcesRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use LookupResourcesRequest.newBuilder() to construct.
private LookupResourcesRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private LookupResourcesRequest() {
resourceObjectType_ = "";
permission_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new LookupResourcesRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_LookupResourcesRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_LookupResourcesRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.PermissionService.LookupResourcesRequest.class, com.authzed.api.v1.PermissionService.LookupResourcesRequest.Builder.class);
}
public static final int CONSISTENCY_FIELD_NUMBER = 1;
private com.authzed.api.v1.PermissionService.Consistency consistency_;
/**
* .authzed.api.v1.Consistency consistency = 1;
* @return Whether the consistency field is set.
*/
@java.lang.Override
public boolean hasConsistency() {
return consistency_ != null;
}
/**
* .authzed.api.v1.Consistency consistency = 1;
* @return The consistency.
*/
@java.lang.Override
public com.authzed.api.v1.PermissionService.Consistency getConsistency() {
return consistency_ == null ? com.authzed.api.v1.PermissionService.Consistency.getDefaultInstance() : consistency_;
}
/**
* .authzed.api.v1.Consistency consistency = 1;
*/
@java.lang.Override
public com.authzed.api.v1.PermissionService.ConsistencyOrBuilder getConsistencyOrBuilder() {
return consistency_ == null ? com.authzed.api.v1.PermissionService.Consistency.getDefaultInstance() : consistency_;
}
public static final int RESOURCE_OBJECT_TYPE_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object resourceObjectType_ = "";
/**
*
* resource_object_type is the type of resource object for which the IDs will
* be returned.
*
*
* string resource_object_type = 2 [(.validate.rules) = { ... }
* @return The resourceObjectType.
*/
@java.lang.Override
public java.lang.String getResourceObjectType() {
java.lang.Object ref = resourceObjectType_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
resourceObjectType_ = s;
return s;
}
}
/**
*
* resource_object_type is the type of resource object for which the IDs will
* be returned.
*
*
* string resource_object_type = 2 [(.validate.rules) = { ... }
* @return The bytes for resourceObjectType.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getResourceObjectTypeBytes() {
java.lang.Object ref = resourceObjectType_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
resourceObjectType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PERMISSION_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private volatile java.lang.Object permission_ = "";
/**
*
* permission is the name of the permission or relation for which the subject
* must Check.
*
*
* string permission = 3 [(.validate.rules) = { ... }
* @return The permission.
*/
@java.lang.Override
public java.lang.String getPermission() {
java.lang.Object ref = permission_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
permission_ = s;
return s;
}
}
/**
*
* permission is the name of the permission or relation for which the subject
* must Check.
*
*
* string permission = 3 [(.validate.rules) = { ... }
* @return The bytes for permission.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getPermissionBytes() {
java.lang.Object ref = permission_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
permission_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SUBJECT_FIELD_NUMBER = 4;
private com.authzed.api.v1.Core.SubjectReference subject_;
/**
*
* subject is the subject with access to the resources.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
* @return Whether the subject field is set.
*/
@java.lang.Override
public boolean hasSubject() {
return subject_ != null;
}
/**
*
* subject is the subject with access to the resources.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
* @return The subject.
*/
@java.lang.Override
public com.authzed.api.v1.Core.SubjectReference getSubject() {
return subject_ == null ? com.authzed.api.v1.Core.SubjectReference.getDefaultInstance() : subject_;
}
/**
*
* subject is the subject with access to the resources.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
*/
@java.lang.Override
public com.authzed.api.v1.Core.SubjectReferenceOrBuilder getSubjectOrBuilder() {
return subject_ == null ? com.authzed.api.v1.Core.SubjectReference.getDefaultInstance() : subject_;
}
public static final int CONTEXT_FIELD_NUMBER = 5;
private com.google.protobuf.Struct context_;
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 5 [(.validate.rules) = { ... }
* @return Whether the context field is set.
*/
@java.lang.Override
public boolean hasContext() {
return context_ != null;
}
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 5 [(.validate.rules) = { ... }
* @return The context.
*/
@java.lang.Override
public com.google.protobuf.Struct getContext() {
return context_ == null ? com.google.protobuf.Struct.getDefaultInstance() : context_;
}
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 5 [(.validate.rules) = { ... }
*/
@java.lang.Override
public com.google.protobuf.StructOrBuilder getContextOrBuilder() {
return context_ == null ? com.google.protobuf.Struct.getDefaultInstance() : context_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (consistency_ != null) {
output.writeMessage(1, getConsistency());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(resourceObjectType_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, resourceObjectType_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(permission_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, permission_);
}
if (subject_ != null) {
output.writeMessage(4, getSubject());
}
if (context_ != null) {
output.writeMessage(5, getContext());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (consistency_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getConsistency());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(resourceObjectType_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, resourceObjectType_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(permission_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, permission_);
}
if (subject_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getSubject());
}
if (context_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, getContext());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.authzed.api.v1.PermissionService.LookupResourcesRequest)) {
return super.equals(obj);
}
com.authzed.api.v1.PermissionService.LookupResourcesRequest other = (com.authzed.api.v1.PermissionService.LookupResourcesRequest) obj;
if (hasConsistency() != other.hasConsistency()) return false;
if (hasConsistency()) {
if (!getConsistency()
.equals(other.getConsistency())) return false;
}
if (!getResourceObjectType()
.equals(other.getResourceObjectType())) return false;
if (!getPermission()
.equals(other.getPermission())) return false;
if (hasSubject() != other.hasSubject()) return false;
if (hasSubject()) {
if (!getSubject()
.equals(other.getSubject())) return false;
}
if (hasContext() != other.hasContext()) return false;
if (hasContext()) {
if (!getContext()
.equals(other.getContext())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasConsistency()) {
hash = (37 * hash) + CONSISTENCY_FIELD_NUMBER;
hash = (53 * hash) + getConsistency().hashCode();
}
hash = (37 * hash) + RESOURCE_OBJECT_TYPE_FIELD_NUMBER;
hash = (53 * hash) + getResourceObjectType().hashCode();
hash = (37 * hash) + PERMISSION_FIELD_NUMBER;
hash = (53 * hash) + getPermission().hashCode();
if (hasSubject()) {
hash = (37 * hash) + SUBJECT_FIELD_NUMBER;
hash = (53 * hash) + getSubject().hashCode();
}
if (hasContext()) {
hash = (37 * hash) + CONTEXT_FIELD_NUMBER;
hash = (53 * hash) + getContext().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.authzed.api.v1.PermissionService.LookupResourcesRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.LookupResourcesRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.LookupResourcesRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.LookupResourcesRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.LookupResourcesRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.LookupResourcesRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.LookupResourcesRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.LookupResourcesRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.LookupResourcesRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.LookupResourcesRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.LookupResourcesRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.LookupResourcesRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.authzed.api.v1.PermissionService.LookupResourcesRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* LookupResourcesRequest performs a lookup of all resources of a particular
* kind on which the subject has the specified permission or the relation in
* which the subject exists, streaming back the IDs of those resources.
*
*
* Protobuf type {@code authzed.api.v1.LookupResourcesRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:authzed.api.v1.LookupResourcesRequest)
com.authzed.api.v1.PermissionService.LookupResourcesRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_LookupResourcesRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_LookupResourcesRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.PermissionService.LookupResourcesRequest.class, com.authzed.api.v1.PermissionService.LookupResourcesRequest.Builder.class);
}
// Construct using com.authzed.api.v1.PermissionService.LookupResourcesRequest.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
consistency_ = null;
if (consistencyBuilder_ != null) {
consistencyBuilder_.dispose();
consistencyBuilder_ = null;
}
resourceObjectType_ = "";
permission_ = "";
subject_ = null;
if (subjectBuilder_ != null) {
subjectBuilder_.dispose();
subjectBuilder_ = null;
}
context_ = null;
if (contextBuilder_ != null) {
contextBuilder_.dispose();
contextBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_LookupResourcesRequest_descriptor;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.LookupResourcesRequest getDefaultInstanceForType() {
return com.authzed.api.v1.PermissionService.LookupResourcesRequest.getDefaultInstance();
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.LookupResourcesRequest build() {
com.authzed.api.v1.PermissionService.LookupResourcesRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.LookupResourcesRequest buildPartial() {
com.authzed.api.v1.PermissionService.LookupResourcesRequest result = new com.authzed.api.v1.PermissionService.LookupResourcesRequest(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.authzed.api.v1.PermissionService.LookupResourcesRequest result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.consistency_ = consistencyBuilder_ == null
? consistency_
: consistencyBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.resourceObjectType_ = resourceObjectType_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.permission_ = permission_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.subject_ = subjectBuilder_ == null
? subject_
: subjectBuilder_.build();
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.context_ = contextBuilder_ == null
? context_
: contextBuilder_.build();
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.authzed.api.v1.PermissionService.LookupResourcesRequest) {
return mergeFrom((com.authzed.api.v1.PermissionService.LookupResourcesRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.authzed.api.v1.PermissionService.LookupResourcesRequest other) {
if (other == com.authzed.api.v1.PermissionService.LookupResourcesRequest.getDefaultInstance()) return this;
if (other.hasConsistency()) {
mergeConsistency(other.getConsistency());
}
if (!other.getResourceObjectType().isEmpty()) {
resourceObjectType_ = other.resourceObjectType_;
bitField0_ |= 0x00000002;
onChanged();
}
if (!other.getPermission().isEmpty()) {
permission_ = other.permission_;
bitField0_ |= 0x00000004;
onChanged();
}
if (other.hasSubject()) {
mergeSubject(other.getSubject());
}
if (other.hasContext()) {
mergeContext(other.getContext());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
input.readMessage(
getConsistencyFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
resourceObjectType_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 18
case 26: {
permission_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000004;
break;
} // case 26
case 34: {
input.readMessage(
getSubjectFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000008;
break;
} // case 34
case 42: {
input.readMessage(
getContextFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000010;
break;
} // case 42
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.authzed.api.v1.PermissionService.Consistency consistency_;
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.PermissionService.Consistency, com.authzed.api.v1.PermissionService.Consistency.Builder, com.authzed.api.v1.PermissionService.ConsistencyOrBuilder> consistencyBuilder_;
/**
* .authzed.api.v1.Consistency consistency = 1;
* @return Whether the consistency field is set.
*/
public boolean hasConsistency() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* .authzed.api.v1.Consistency consistency = 1;
* @return The consistency.
*/
public com.authzed.api.v1.PermissionService.Consistency getConsistency() {
if (consistencyBuilder_ == null) {
return consistency_ == null ? com.authzed.api.v1.PermissionService.Consistency.getDefaultInstance() : consistency_;
} else {
return consistencyBuilder_.getMessage();
}
}
/**
* .authzed.api.v1.Consistency consistency = 1;
*/
public Builder setConsistency(com.authzed.api.v1.PermissionService.Consistency value) {
if (consistencyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
consistency_ = value;
} else {
consistencyBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .authzed.api.v1.Consistency consistency = 1;
*/
public Builder setConsistency(
com.authzed.api.v1.PermissionService.Consistency.Builder builderForValue) {
if (consistencyBuilder_ == null) {
consistency_ = builderForValue.build();
} else {
consistencyBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .authzed.api.v1.Consistency consistency = 1;
*/
public Builder mergeConsistency(com.authzed.api.v1.PermissionService.Consistency value) {
if (consistencyBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0) &&
consistency_ != null &&
consistency_ != com.authzed.api.v1.PermissionService.Consistency.getDefaultInstance()) {
getConsistencyBuilder().mergeFrom(value);
} else {
consistency_ = value;
}
} else {
consistencyBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .authzed.api.v1.Consistency consistency = 1;
*/
public Builder clearConsistency() {
bitField0_ = (bitField0_ & ~0x00000001);
consistency_ = null;
if (consistencyBuilder_ != null) {
consistencyBuilder_.dispose();
consistencyBuilder_ = null;
}
onChanged();
return this;
}
/**
* .authzed.api.v1.Consistency consistency = 1;
*/
public com.authzed.api.v1.PermissionService.Consistency.Builder getConsistencyBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getConsistencyFieldBuilder().getBuilder();
}
/**
* .authzed.api.v1.Consistency consistency = 1;
*/
public com.authzed.api.v1.PermissionService.ConsistencyOrBuilder getConsistencyOrBuilder() {
if (consistencyBuilder_ != null) {
return consistencyBuilder_.getMessageOrBuilder();
} else {
return consistency_ == null ?
com.authzed.api.v1.PermissionService.Consistency.getDefaultInstance() : consistency_;
}
}
/**
* .authzed.api.v1.Consistency consistency = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.PermissionService.Consistency, com.authzed.api.v1.PermissionService.Consistency.Builder, com.authzed.api.v1.PermissionService.ConsistencyOrBuilder>
getConsistencyFieldBuilder() {
if (consistencyBuilder_ == null) {
consistencyBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.PermissionService.Consistency, com.authzed.api.v1.PermissionService.Consistency.Builder, com.authzed.api.v1.PermissionService.ConsistencyOrBuilder>(
getConsistency(),
getParentForChildren(),
isClean());
consistency_ = null;
}
return consistencyBuilder_;
}
private java.lang.Object resourceObjectType_ = "";
/**
*
* resource_object_type is the type of resource object for which the IDs will
* be returned.
*
*
* string resource_object_type = 2 [(.validate.rules) = { ... }
* @return The resourceObjectType.
*/
public java.lang.String getResourceObjectType() {
java.lang.Object ref = resourceObjectType_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
resourceObjectType_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* resource_object_type is the type of resource object for which the IDs will
* be returned.
*
*
* string resource_object_type = 2 [(.validate.rules) = { ... }
* @return The bytes for resourceObjectType.
*/
public com.google.protobuf.ByteString
getResourceObjectTypeBytes() {
java.lang.Object ref = resourceObjectType_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
resourceObjectType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* resource_object_type is the type of resource object for which the IDs will
* be returned.
*
*
* string resource_object_type = 2 [(.validate.rules) = { ... }
* @param value The resourceObjectType to set.
* @return This builder for chaining.
*/
public Builder setResourceObjectType(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
resourceObjectType_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* resource_object_type is the type of resource object for which the IDs will
* be returned.
*
*
* string resource_object_type = 2 [(.validate.rules) = { ... }
* @return This builder for chaining.
*/
public Builder clearResourceObjectType() {
resourceObjectType_ = getDefaultInstance().getResourceObjectType();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
* resource_object_type is the type of resource object for which the IDs will
* be returned.
*
*
* string resource_object_type = 2 [(.validate.rules) = { ... }
* @param value The bytes for resourceObjectType to set.
* @return This builder for chaining.
*/
public Builder setResourceObjectTypeBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
resourceObjectType_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private java.lang.Object permission_ = "";
/**
*
* permission is the name of the permission or relation for which the subject
* must Check.
*
*
* string permission = 3 [(.validate.rules) = { ... }
* @return The permission.
*/
public java.lang.String getPermission() {
java.lang.Object ref = permission_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
permission_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* permission is the name of the permission or relation for which the subject
* must Check.
*
*
* string permission = 3 [(.validate.rules) = { ... }
* @return The bytes for permission.
*/
public com.google.protobuf.ByteString
getPermissionBytes() {
java.lang.Object ref = permission_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
permission_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* permission is the name of the permission or relation for which the subject
* must Check.
*
*
* string permission = 3 [(.validate.rules) = { ... }
* @param value The permission to set.
* @return This builder for chaining.
*/
public Builder setPermission(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
permission_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* permission is the name of the permission or relation for which the subject
* must Check.
*
*
* string permission = 3 [(.validate.rules) = { ... }
* @return This builder for chaining.
*/
public Builder clearPermission() {
permission_ = getDefaultInstance().getPermission();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
*
* permission is the name of the permission or relation for which the subject
* must Check.
*
*
* string permission = 3 [(.validate.rules) = { ... }
* @param value The bytes for permission to set.
* @return This builder for chaining.
*/
public Builder setPermissionBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
permission_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
private com.authzed.api.v1.Core.SubjectReference subject_;
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.SubjectReference, com.authzed.api.v1.Core.SubjectReference.Builder, com.authzed.api.v1.Core.SubjectReferenceOrBuilder> subjectBuilder_;
/**
*
* subject is the subject with access to the resources.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
* @return Whether the subject field is set.
*/
public boolean hasSubject() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* subject is the subject with access to the resources.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
* @return The subject.
*/
public com.authzed.api.v1.Core.SubjectReference getSubject() {
if (subjectBuilder_ == null) {
return subject_ == null ? com.authzed.api.v1.Core.SubjectReference.getDefaultInstance() : subject_;
} else {
return subjectBuilder_.getMessage();
}
}
/**
*
* subject is the subject with access to the resources.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
*/
public Builder setSubject(com.authzed.api.v1.Core.SubjectReference value) {
if (subjectBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
subject_ = value;
} else {
subjectBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* subject is the subject with access to the resources.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
*/
public Builder setSubject(
com.authzed.api.v1.Core.SubjectReference.Builder builderForValue) {
if (subjectBuilder_ == null) {
subject_ = builderForValue.build();
} else {
subjectBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* subject is the subject with access to the resources.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
*/
public Builder mergeSubject(com.authzed.api.v1.Core.SubjectReference value) {
if (subjectBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0) &&
subject_ != null &&
subject_ != com.authzed.api.v1.Core.SubjectReference.getDefaultInstance()) {
getSubjectBuilder().mergeFrom(value);
} else {
subject_ = value;
}
} else {
subjectBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* subject is the subject with access to the resources.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
*/
public Builder clearSubject() {
bitField0_ = (bitField0_ & ~0x00000008);
subject_ = null;
if (subjectBuilder_ != null) {
subjectBuilder_.dispose();
subjectBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* subject is the subject with access to the resources.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
*/
public com.authzed.api.v1.Core.SubjectReference.Builder getSubjectBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getSubjectFieldBuilder().getBuilder();
}
/**
*
* subject is the subject with access to the resources.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
*/
public com.authzed.api.v1.Core.SubjectReferenceOrBuilder getSubjectOrBuilder() {
if (subjectBuilder_ != null) {
return subjectBuilder_.getMessageOrBuilder();
} else {
return subject_ == null ?
com.authzed.api.v1.Core.SubjectReference.getDefaultInstance() : subject_;
}
}
/**
*
* subject is the subject with access to the resources.
*
*
* .authzed.api.v1.SubjectReference subject = 4 [(.validate.rules) = { ... }
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.SubjectReference, com.authzed.api.v1.Core.SubjectReference.Builder, com.authzed.api.v1.Core.SubjectReferenceOrBuilder>
getSubjectFieldBuilder() {
if (subjectBuilder_ == null) {
subjectBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.SubjectReference, com.authzed.api.v1.Core.SubjectReference.Builder, com.authzed.api.v1.Core.SubjectReferenceOrBuilder>(
getSubject(),
getParentForChildren(),
isClean());
subject_ = null;
}
return subjectBuilder_;
}
private com.google.protobuf.Struct context_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Struct, com.google.protobuf.Struct.Builder, com.google.protobuf.StructOrBuilder> contextBuilder_;
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 5 [(.validate.rules) = { ... }
* @return Whether the context field is set.
*/
public boolean hasContext() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 5 [(.validate.rules) = { ... }
* @return The context.
*/
public com.google.protobuf.Struct getContext() {
if (contextBuilder_ == null) {
return context_ == null ? com.google.protobuf.Struct.getDefaultInstance() : context_;
} else {
return contextBuilder_.getMessage();
}
}
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 5 [(.validate.rules) = { ... }
*/
public Builder setContext(com.google.protobuf.Struct value) {
if (contextBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
context_ = value;
} else {
contextBuilder_.setMessage(value);
}
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 5 [(.validate.rules) = { ... }
*/
public Builder setContext(
com.google.protobuf.Struct.Builder builderForValue) {
if (contextBuilder_ == null) {
context_ = builderForValue.build();
} else {
contextBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 5 [(.validate.rules) = { ... }
*/
public Builder mergeContext(com.google.protobuf.Struct value) {
if (contextBuilder_ == null) {
if (((bitField0_ & 0x00000010) != 0) &&
context_ != null &&
context_ != com.google.protobuf.Struct.getDefaultInstance()) {
getContextBuilder().mergeFrom(value);
} else {
context_ = value;
}
} else {
contextBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 5 [(.validate.rules) = { ... }
*/
public Builder clearContext() {
bitField0_ = (bitField0_ & ~0x00000010);
context_ = null;
if (contextBuilder_ != null) {
contextBuilder_.dispose();
contextBuilder_ = null;
}
onChanged();
return this;
}
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 5 [(.validate.rules) = { ... }
*/
public com.google.protobuf.Struct.Builder getContextBuilder() {
bitField0_ |= 0x00000010;
onChanged();
return getContextFieldBuilder().getBuilder();
}
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 5 [(.validate.rules) = { ... }
*/
public com.google.protobuf.StructOrBuilder getContextOrBuilder() {
if (contextBuilder_ != null) {
return contextBuilder_.getMessageOrBuilder();
} else {
return context_ == null ?
com.google.protobuf.Struct.getDefaultInstance() : context_;
}
}
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 5 [(.validate.rules) = { ... }
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Struct, com.google.protobuf.Struct.Builder, com.google.protobuf.StructOrBuilder>
getContextFieldBuilder() {
if (contextBuilder_ == null) {
contextBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Struct, com.google.protobuf.Struct.Builder, com.google.protobuf.StructOrBuilder>(
getContext(),
getParentForChildren(),
isClean());
context_ = null;
}
return contextBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:authzed.api.v1.LookupResourcesRequest)
}
// @@protoc_insertion_point(class_scope:authzed.api.v1.LookupResourcesRequest)
private static final com.authzed.api.v1.PermissionService.LookupResourcesRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.authzed.api.v1.PermissionService.LookupResourcesRequest();
}
public static com.authzed.api.v1.PermissionService.LookupResourcesRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public LookupResourcesRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.LookupResourcesRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface LookupResourcesResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:authzed.api.v1.LookupResourcesResponse)
com.google.protobuf.MessageOrBuilder {
/**
* .authzed.api.v1.ZedToken looked_up_at = 1;
* @return Whether the lookedUpAt field is set.
*/
boolean hasLookedUpAt();
/**
* .authzed.api.v1.ZedToken looked_up_at = 1;
* @return The lookedUpAt.
*/
com.authzed.api.v1.Core.ZedToken getLookedUpAt();
/**
* .authzed.api.v1.ZedToken looked_up_at = 1;
*/
com.authzed.api.v1.Core.ZedTokenOrBuilder getLookedUpAtOrBuilder();
/**
* string resource_object_id = 2;
* @return The resourceObjectId.
*/
java.lang.String getResourceObjectId();
/**
* string resource_object_id = 2;
* @return The bytes for resourceObjectId.
*/
com.google.protobuf.ByteString
getResourceObjectIdBytes();
/**
*
* permissionship indicates whether the response was partially evaluated or not
*
*
* .authzed.api.v1.LookupPermissionship permissionship = 3 [(.validate.rules) = { ... }
* @return The enum numeric value on the wire for permissionship.
*/
int getPermissionshipValue();
/**
*
* permissionship indicates whether the response was partially evaluated or not
*
*
* .authzed.api.v1.LookupPermissionship permissionship = 3 [(.validate.rules) = { ... }
* @return The permissionship.
*/
com.authzed.api.v1.PermissionService.LookupPermissionship getPermissionship();
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 4 [(.validate.rules) = { ... }
* @return Whether the partialCaveatInfo field is set.
*/
boolean hasPartialCaveatInfo();
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 4 [(.validate.rules) = { ... }
* @return The partialCaveatInfo.
*/
com.authzed.api.v1.Core.PartialCaveatInfo getPartialCaveatInfo();
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 4 [(.validate.rules) = { ... }
*/
com.authzed.api.v1.Core.PartialCaveatInfoOrBuilder getPartialCaveatInfoOrBuilder();
}
/**
*
* LookupResourcesResponse contains a single matching resource object ID for the
* requested object type, permission, and subject.
*
*
* Protobuf type {@code authzed.api.v1.LookupResourcesResponse}
*/
public static final class LookupResourcesResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:authzed.api.v1.LookupResourcesResponse)
LookupResourcesResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use LookupResourcesResponse.newBuilder() to construct.
private LookupResourcesResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private LookupResourcesResponse() {
resourceObjectId_ = "";
permissionship_ = 0;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new LookupResourcesResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_LookupResourcesResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_LookupResourcesResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.PermissionService.LookupResourcesResponse.class, com.authzed.api.v1.PermissionService.LookupResourcesResponse.Builder.class);
}
public static final int LOOKED_UP_AT_FIELD_NUMBER = 1;
private com.authzed.api.v1.Core.ZedToken lookedUpAt_;
/**
* .authzed.api.v1.ZedToken looked_up_at = 1;
* @return Whether the lookedUpAt field is set.
*/
@java.lang.Override
public boolean hasLookedUpAt() {
return lookedUpAt_ != null;
}
/**
* .authzed.api.v1.ZedToken looked_up_at = 1;
* @return The lookedUpAt.
*/
@java.lang.Override
public com.authzed.api.v1.Core.ZedToken getLookedUpAt() {
return lookedUpAt_ == null ? com.authzed.api.v1.Core.ZedToken.getDefaultInstance() : lookedUpAt_;
}
/**
* .authzed.api.v1.ZedToken looked_up_at = 1;
*/
@java.lang.Override
public com.authzed.api.v1.Core.ZedTokenOrBuilder getLookedUpAtOrBuilder() {
return lookedUpAt_ == null ? com.authzed.api.v1.Core.ZedToken.getDefaultInstance() : lookedUpAt_;
}
public static final int RESOURCE_OBJECT_ID_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object resourceObjectId_ = "";
/**
* string resource_object_id = 2;
* @return The resourceObjectId.
*/
@java.lang.Override
public java.lang.String getResourceObjectId() {
java.lang.Object ref = resourceObjectId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
resourceObjectId_ = s;
return s;
}
}
/**
* string resource_object_id = 2;
* @return The bytes for resourceObjectId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getResourceObjectIdBytes() {
java.lang.Object ref = resourceObjectId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
resourceObjectId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PERMISSIONSHIP_FIELD_NUMBER = 3;
private int permissionship_ = 0;
/**
*
* permissionship indicates whether the response was partially evaluated or not
*
*
* .authzed.api.v1.LookupPermissionship permissionship = 3 [(.validate.rules) = { ... }
* @return The enum numeric value on the wire for permissionship.
*/
@java.lang.Override public int getPermissionshipValue() {
return permissionship_;
}
/**
*
* permissionship indicates whether the response was partially evaluated or not
*
*
* .authzed.api.v1.LookupPermissionship permissionship = 3 [(.validate.rules) = { ... }
* @return The permissionship.
*/
@java.lang.Override public com.authzed.api.v1.PermissionService.LookupPermissionship getPermissionship() {
com.authzed.api.v1.PermissionService.LookupPermissionship result = com.authzed.api.v1.PermissionService.LookupPermissionship.forNumber(permissionship_);
return result == null ? com.authzed.api.v1.PermissionService.LookupPermissionship.UNRECOGNIZED : result;
}
public static final int PARTIAL_CAVEAT_INFO_FIELD_NUMBER = 4;
private com.authzed.api.v1.Core.PartialCaveatInfo partialCaveatInfo_;
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 4 [(.validate.rules) = { ... }
* @return Whether the partialCaveatInfo field is set.
*/
@java.lang.Override
public boolean hasPartialCaveatInfo() {
return partialCaveatInfo_ != null;
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 4 [(.validate.rules) = { ... }
* @return The partialCaveatInfo.
*/
@java.lang.Override
public com.authzed.api.v1.Core.PartialCaveatInfo getPartialCaveatInfo() {
return partialCaveatInfo_ == null ? com.authzed.api.v1.Core.PartialCaveatInfo.getDefaultInstance() : partialCaveatInfo_;
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 4 [(.validate.rules) = { ... }
*/
@java.lang.Override
public com.authzed.api.v1.Core.PartialCaveatInfoOrBuilder getPartialCaveatInfoOrBuilder() {
return partialCaveatInfo_ == null ? com.authzed.api.v1.Core.PartialCaveatInfo.getDefaultInstance() : partialCaveatInfo_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (lookedUpAt_ != null) {
output.writeMessage(1, getLookedUpAt());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(resourceObjectId_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, resourceObjectId_);
}
if (permissionship_ != com.authzed.api.v1.PermissionService.LookupPermissionship.LOOKUP_PERMISSIONSHIP_UNSPECIFIED.getNumber()) {
output.writeEnum(3, permissionship_);
}
if (partialCaveatInfo_ != null) {
output.writeMessage(4, getPartialCaveatInfo());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (lookedUpAt_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getLookedUpAt());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(resourceObjectId_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, resourceObjectId_);
}
if (permissionship_ != com.authzed.api.v1.PermissionService.LookupPermissionship.LOOKUP_PERMISSIONSHIP_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(3, permissionship_);
}
if (partialCaveatInfo_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getPartialCaveatInfo());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.authzed.api.v1.PermissionService.LookupResourcesResponse)) {
return super.equals(obj);
}
com.authzed.api.v1.PermissionService.LookupResourcesResponse other = (com.authzed.api.v1.PermissionService.LookupResourcesResponse) obj;
if (hasLookedUpAt() != other.hasLookedUpAt()) return false;
if (hasLookedUpAt()) {
if (!getLookedUpAt()
.equals(other.getLookedUpAt())) return false;
}
if (!getResourceObjectId()
.equals(other.getResourceObjectId())) return false;
if (permissionship_ != other.permissionship_) return false;
if (hasPartialCaveatInfo() != other.hasPartialCaveatInfo()) return false;
if (hasPartialCaveatInfo()) {
if (!getPartialCaveatInfo()
.equals(other.getPartialCaveatInfo())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasLookedUpAt()) {
hash = (37 * hash) + LOOKED_UP_AT_FIELD_NUMBER;
hash = (53 * hash) + getLookedUpAt().hashCode();
}
hash = (37 * hash) + RESOURCE_OBJECT_ID_FIELD_NUMBER;
hash = (53 * hash) + getResourceObjectId().hashCode();
hash = (37 * hash) + PERMISSIONSHIP_FIELD_NUMBER;
hash = (53 * hash) + permissionship_;
if (hasPartialCaveatInfo()) {
hash = (37 * hash) + PARTIAL_CAVEAT_INFO_FIELD_NUMBER;
hash = (53 * hash) + getPartialCaveatInfo().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.authzed.api.v1.PermissionService.LookupResourcesResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.LookupResourcesResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.LookupResourcesResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.LookupResourcesResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.LookupResourcesResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.LookupResourcesResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.LookupResourcesResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.LookupResourcesResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.LookupResourcesResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.LookupResourcesResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.LookupResourcesResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.LookupResourcesResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.authzed.api.v1.PermissionService.LookupResourcesResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* LookupResourcesResponse contains a single matching resource object ID for the
* requested object type, permission, and subject.
*
*
* Protobuf type {@code authzed.api.v1.LookupResourcesResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:authzed.api.v1.LookupResourcesResponse)
com.authzed.api.v1.PermissionService.LookupResourcesResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_LookupResourcesResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_LookupResourcesResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.PermissionService.LookupResourcesResponse.class, com.authzed.api.v1.PermissionService.LookupResourcesResponse.Builder.class);
}
// Construct using com.authzed.api.v1.PermissionService.LookupResourcesResponse.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
lookedUpAt_ = null;
if (lookedUpAtBuilder_ != null) {
lookedUpAtBuilder_.dispose();
lookedUpAtBuilder_ = null;
}
resourceObjectId_ = "";
permissionship_ = 0;
partialCaveatInfo_ = null;
if (partialCaveatInfoBuilder_ != null) {
partialCaveatInfoBuilder_.dispose();
partialCaveatInfoBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_LookupResourcesResponse_descriptor;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.LookupResourcesResponse getDefaultInstanceForType() {
return com.authzed.api.v1.PermissionService.LookupResourcesResponse.getDefaultInstance();
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.LookupResourcesResponse build() {
com.authzed.api.v1.PermissionService.LookupResourcesResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.LookupResourcesResponse buildPartial() {
com.authzed.api.v1.PermissionService.LookupResourcesResponse result = new com.authzed.api.v1.PermissionService.LookupResourcesResponse(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.authzed.api.v1.PermissionService.LookupResourcesResponse result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.lookedUpAt_ = lookedUpAtBuilder_ == null
? lookedUpAt_
: lookedUpAtBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.resourceObjectId_ = resourceObjectId_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.permissionship_ = permissionship_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.partialCaveatInfo_ = partialCaveatInfoBuilder_ == null
? partialCaveatInfo_
: partialCaveatInfoBuilder_.build();
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.authzed.api.v1.PermissionService.LookupResourcesResponse) {
return mergeFrom((com.authzed.api.v1.PermissionService.LookupResourcesResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.authzed.api.v1.PermissionService.LookupResourcesResponse other) {
if (other == com.authzed.api.v1.PermissionService.LookupResourcesResponse.getDefaultInstance()) return this;
if (other.hasLookedUpAt()) {
mergeLookedUpAt(other.getLookedUpAt());
}
if (!other.getResourceObjectId().isEmpty()) {
resourceObjectId_ = other.resourceObjectId_;
bitField0_ |= 0x00000002;
onChanged();
}
if (other.permissionship_ != 0) {
setPermissionshipValue(other.getPermissionshipValue());
}
if (other.hasPartialCaveatInfo()) {
mergePartialCaveatInfo(other.getPartialCaveatInfo());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
input.readMessage(
getLookedUpAtFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
resourceObjectId_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 18
case 24: {
permissionship_ = input.readEnum();
bitField0_ |= 0x00000004;
break;
} // case 24
case 34: {
input.readMessage(
getPartialCaveatInfoFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000008;
break;
} // case 34
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.authzed.api.v1.Core.ZedToken lookedUpAt_;
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.ZedToken, com.authzed.api.v1.Core.ZedToken.Builder, com.authzed.api.v1.Core.ZedTokenOrBuilder> lookedUpAtBuilder_;
/**
* .authzed.api.v1.ZedToken looked_up_at = 1;
* @return Whether the lookedUpAt field is set.
*/
public boolean hasLookedUpAt() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* .authzed.api.v1.ZedToken looked_up_at = 1;
* @return The lookedUpAt.
*/
public com.authzed.api.v1.Core.ZedToken getLookedUpAt() {
if (lookedUpAtBuilder_ == null) {
return lookedUpAt_ == null ? com.authzed.api.v1.Core.ZedToken.getDefaultInstance() : lookedUpAt_;
} else {
return lookedUpAtBuilder_.getMessage();
}
}
/**
* .authzed.api.v1.ZedToken looked_up_at = 1;
*/
public Builder setLookedUpAt(com.authzed.api.v1.Core.ZedToken value) {
if (lookedUpAtBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
lookedUpAt_ = value;
} else {
lookedUpAtBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .authzed.api.v1.ZedToken looked_up_at = 1;
*/
public Builder setLookedUpAt(
com.authzed.api.v1.Core.ZedToken.Builder builderForValue) {
if (lookedUpAtBuilder_ == null) {
lookedUpAt_ = builderForValue.build();
} else {
lookedUpAtBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .authzed.api.v1.ZedToken looked_up_at = 1;
*/
public Builder mergeLookedUpAt(com.authzed.api.v1.Core.ZedToken value) {
if (lookedUpAtBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0) &&
lookedUpAt_ != null &&
lookedUpAt_ != com.authzed.api.v1.Core.ZedToken.getDefaultInstance()) {
getLookedUpAtBuilder().mergeFrom(value);
} else {
lookedUpAt_ = value;
}
} else {
lookedUpAtBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .authzed.api.v1.ZedToken looked_up_at = 1;
*/
public Builder clearLookedUpAt() {
bitField0_ = (bitField0_ & ~0x00000001);
lookedUpAt_ = null;
if (lookedUpAtBuilder_ != null) {
lookedUpAtBuilder_.dispose();
lookedUpAtBuilder_ = null;
}
onChanged();
return this;
}
/**
* .authzed.api.v1.ZedToken looked_up_at = 1;
*/
public com.authzed.api.v1.Core.ZedToken.Builder getLookedUpAtBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getLookedUpAtFieldBuilder().getBuilder();
}
/**
* .authzed.api.v1.ZedToken looked_up_at = 1;
*/
public com.authzed.api.v1.Core.ZedTokenOrBuilder getLookedUpAtOrBuilder() {
if (lookedUpAtBuilder_ != null) {
return lookedUpAtBuilder_.getMessageOrBuilder();
} else {
return lookedUpAt_ == null ?
com.authzed.api.v1.Core.ZedToken.getDefaultInstance() : lookedUpAt_;
}
}
/**
* .authzed.api.v1.ZedToken looked_up_at = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.ZedToken, com.authzed.api.v1.Core.ZedToken.Builder, com.authzed.api.v1.Core.ZedTokenOrBuilder>
getLookedUpAtFieldBuilder() {
if (lookedUpAtBuilder_ == null) {
lookedUpAtBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.ZedToken, com.authzed.api.v1.Core.ZedToken.Builder, com.authzed.api.v1.Core.ZedTokenOrBuilder>(
getLookedUpAt(),
getParentForChildren(),
isClean());
lookedUpAt_ = null;
}
return lookedUpAtBuilder_;
}
private java.lang.Object resourceObjectId_ = "";
/**
* string resource_object_id = 2;
* @return The resourceObjectId.
*/
public java.lang.String getResourceObjectId() {
java.lang.Object ref = resourceObjectId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
resourceObjectId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string resource_object_id = 2;
* @return The bytes for resourceObjectId.
*/
public com.google.protobuf.ByteString
getResourceObjectIdBytes() {
java.lang.Object ref = resourceObjectId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
resourceObjectId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string resource_object_id = 2;
* @param value The resourceObjectId to set.
* @return This builder for chaining.
*/
public Builder setResourceObjectId(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
resourceObjectId_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* string resource_object_id = 2;
* @return This builder for chaining.
*/
public Builder clearResourceObjectId() {
resourceObjectId_ = getDefaultInstance().getResourceObjectId();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
* string resource_object_id = 2;
* @param value The bytes for resourceObjectId to set.
* @return This builder for chaining.
*/
public Builder setResourceObjectIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
resourceObjectId_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private int permissionship_ = 0;
/**
*
* permissionship indicates whether the response was partially evaluated or not
*
*
* .authzed.api.v1.LookupPermissionship permissionship = 3 [(.validate.rules) = { ... }
* @return The enum numeric value on the wire for permissionship.
*/
@java.lang.Override public int getPermissionshipValue() {
return permissionship_;
}
/**
*
* permissionship indicates whether the response was partially evaluated or not
*
*
* .authzed.api.v1.LookupPermissionship permissionship = 3 [(.validate.rules) = { ... }
* @param value The enum numeric value on the wire for permissionship to set.
* @return This builder for chaining.
*/
public Builder setPermissionshipValue(int value) {
permissionship_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* permissionship indicates whether the response was partially evaluated or not
*
*
* .authzed.api.v1.LookupPermissionship permissionship = 3 [(.validate.rules) = { ... }
* @return The permissionship.
*/
@java.lang.Override
public com.authzed.api.v1.PermissionService.LookupPermissionship getPermissionship() {
com.authzed.api.v1.PermissionService.LookupPermissionship result = com.authzed.api.v1.PermissionService.LookupPermissionship.forNumber(permissionship_);
return result == null ? com.authzed.api.v1.PermissionService.LookupPermissionship.UNRECOGNIZED : result;
}
/**
*
* permissionship indicates whether the response was partially evaluated or not
*
*
* .authzed.api.v1.LookupPermissionship permissionship = 3 [(.validate.rules) = { ... }
* @param value The permissionship to set.
* @return This builder for chaining.
*/
public Builder setPermissionship(com.authzed.api.v1.PermissionService.LookupPermissionship value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
permissionship_ = value.getNumber();
onChanged();
return this;
}
/**
*
* permissionship indicates whether the response was partially evaluated or not
*
*
* .authzed.api.v1.LookupPermissionship permissionship = 3 [(.validate.rules) = { ... }
* @return This builder for chaining.
*/
public Builder clearPermissionship() {
bitField0_ = (bitField0_ & ~0x00000004);
permissionship_ = 0;
onChanged();
return this;
}
private com.authzed.api.v1.Core.PartialCaveatInfo partialCaveatInfo_;
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.PartialCaveatInfo, com.authzed.api.v1.Core.PartialCaveatInfo.Builder, com.authzed.api.v1.Core.PartialCaveatInfoOrBuilder> partialCaveatInfoBuilder_;
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 4 [(.validate.rules) = { ... }
* @return Whether the partialCaveatInfo field is set.
*/
public boolean hasPartialCaveatInfo() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 4 [(.validate.rules) = { ... }
* @return The partialCaveatInfo.
*/
public com.authzed.api.v1.Core.PartialCaveatInfo getPartialCaveatInfo() {
if (partialCaveatInfoBuilder_ == null) {
return partialCaveatInfo_ == null ? com.authzed.api.v1.Core.PartialCaveatInfo.getDefaultInstance() : partialCaveatInfo_;
} else {
return partialCaveatInfoBuilder_.getMessage();
}
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 4 [(.validate.rules) = { ... }
*/
public Builder setPartialCaveatInfo(com.authzed.api.v1.Core.PartialCaveatInfo value) {
if (partialCaveatInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
partialCaveatInfo_ = value;
} else {
partialCaveatInfoBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 4 [(.validate.rules) = { ... }
*/
public Builder setPartialCaveatInfo(
com.authzed.api.v1.Core.PartialCaveatInfo.Builder builderForValue) {
if (partialCaveatInfoBuilder_ == null) {
partialCaveatInfo_ = builderForValue.build();
} else {
partialCaveatInfoBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 4 [(.validate.rules) = { ... }
*/
public Builder mergePartialCaveatInfo(com.authzed.api.v1.Core.PartialCaveatInfo value) {
if (partialCaveatInfoBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0) &&
partialCaveatInfo_ != null &&
partialCaveatInfo_ != com.authzed.api.v1.Core.PartialCaveatInfo.getDefaultInstance()) {
getPartialCaveatInfoBuilder().mergeFrom(value);
} else {
partialCaveatInfo_ = value;
}
} else {
partialCaveatInfoBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 4 [(.validate.rules) = { ... }
*/
public Builder clearPartialCaveatInfo() {
bitField0_ = (bitField0_ & ~0x00000008);
partialCaveatInfo_ = null;
if (partialCaveatInfoBuilder_ != null) {
partialCaveatInfoBuilder_.dispose();
partialCaveatInfoBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 4 [(.validate.rules) = { ... }
*/
public com.authzed.api.v1.Core.PartialCaveatInfo.Builder getPartialCaveatInfoBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getPartialCaveatInfoFieldBuilder().getBuilder();
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 4 [(.validate.rules) = { ... }
*/
public com.authzed.api.v1.Core.PartialCaveatInfoOrBuilder getPartialCaveatInfoOrBuilder() {
if (partialCaveatInfoBuilder_ != null) {
return partialCaveatInfoBuilder_.getMessageOrBuilder();
} else {
return partialCaveatInfo_ == null ?
com.authzed.api.v1.Core.PartialCaveatInfo.getDefaultInstance() : partialCaveatInfo_;
}
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 4 [(.validate.rules) = { ... }
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.PartialCaveatInfo, com.authzed.api.v1.Core.PartialCaveatInfo.Builder, com.authzed.api.v1.Core.PartialCaveatInfoOrBuilder>
getPartialCaveatInfoFieldBuilder() {
if (partialCaveatInfoBuilder_ == null) {
partialCaveatInfoBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.PartialCaveatInfo, com.authzed.api.v1.Core.PartialCaveatInfo.Builder, com.authzed.api.v1.Core.PartialCaveatInfoOrBuilder>(
getPartialCaveatInfo(),
getParentForChildren(),
isClean());
partialCaveatInfo_ = null;
}
return partialCaveatInfoBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:authzed.api.v1.LookupResourcesResponse)
}
// @@protoc_insertion_point(class_scope:authzed.api.v1.LookupResourcesResponse)
private static final com.authzed.api.v1.PermissionService.LookupResourcesResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.authzed.api.v1.PermissionService.LookupResourcesResponse();
}
public static com.authzed.api.v1.PermissionService.LookupResourcesResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public LookupResourcesResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.LookupResourcesResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface LookupSubjectsRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:authzed.api.v1.LookupSubjectsRequest)
com.google.protobuf.MessageOrBuilder {
/**
* .authzed.api.v1.Consistency consistency = 1;
* @return Whether the consistency field is set.
*/
boolean hasConsistency();
/**
* .authzed.api.v1.Consistency consistency = 1;
* @return The consistency.
*/
com.authzed.api.v1.PermissionService.Consistency getConsistency();
/**
* .authzed.api.v1.Consistency consistency = 1;
*/
com.authzed.api.v1.PermissionService.ConsistencyOrBuilder getConsistencyOrBuilder();
/**
*
* resource is the resource for which all matching subjects for the permission
* or relation will be returned.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
* @return Whether the resource field is set.
*/
boolean hasResource();
/**
*
* resource is the resource for which all matching subjects for the permission
* or relation will be returned.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
* @return The resource.
*/
com.authzed.api.v1.Core.ObjectReference getResource();
/**
*
* resource is the resource for which all matching subjects for the permission
* or relation will be returned.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
*/
com.authzed.api.v1.Core.ObjectReferenceOrBuilder getResourceOrBuilder();
/**
*
* permission is the name of the permission (or relation) for which to find
* the subjects.
*
*
* string permission = 3 [(.validate.rules) = { ... }
* @return The permission.
*/
java.lang.String getPermission();
/**
*
* permission is the name of the permission (or relation) for which to find
* the subjects.
*
*
* string permission = 3 [(.validate.rules) = { ... }
* @return The bytes for permission.
*/
com.google.protobuf.ByteString
getPermissionBytes();
/**
*
* subject_object_type is the type of subject object for which the IDs will
* be returned.
*
*
* string subject_object_type = 4 [(.validate.rules) = { ... }
* @return The subjectObjectType.
*/
java.lang.String getSubjectObjectType();
/**
*
* subject_object_type is the type of subject object for which the IDs will
* be returned.
*
*
* string subject_object_type = 4 [(.validate.rules) = { ... }
* @return The bytes for subjectObjectType.
*/
com.google.protobuf.ByteString
getSubjectObjectTypeBytes();
/**
*
* optional_subject_relation is the optional relation for the subject.
*
*
* string optional_subject_relation = 5 [(.validate.rules) = { ... }
* @return The optionalSubjectRelation.
*/
java.lang.String getOptionalSubjectRelation();
/**
*
* optional_subject_relation is the optional relation for the subject.
*
*
* string optional_subject_relation = 5 [(.validate.rules) = { ... }
* @return The bytes for optionalSubjectRelation.
*/
com.google.protobuf.ByteString
getOptionalSubjectRelationBytes();
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 6 [(.validate.rules) = { ... }
* @return Whether the context field is set.
*/
boolean hasContext();
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 6 [(.validate.rules) = { ... }
* @return The context.
*/
com.google.protobuf.Struct getContext();
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 6 [(.validate.rules) = { ... }
*/
com.google.protobuf.StructOrBuilder getContextOrBuilder();
}
/**
*
* LookupSubjectsRequest performs a lookup of all subjects of a particular
* kind for which the subject has the specified permission or the relation in
* which the subject exists, streaming back the IDs of those subjects.
*
*
* Protobuf type {@code authzed.api.v1.LookupSubjectsRequest}
*/
public static final class LookupSubjectsRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:authzed.api.v1.LookupSubjectsRequest)
LookupSubjectsRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use LookupSubjectsRequest.newBuilder() to construct.
private LookupSubjectsRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private LookupSubjectsRequest() {
permission_ = "";
subjectObjectType_ = "";
optionalSubjectRelation_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new LookupSubjectsRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_LookupSubjectsRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_LookupSubjectsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.PermissionService.LookupSubjectsRequest.class, com.authzed.api.v1.PermissionService.LookupSubjectsRequest.Builder.class);
}
public static final int CONSISTENCY_FIELD_NUMBER = 1;
private com.authzed.api.v1.PermissionService.Consistency consistency_;
/**
* .authzed.api.v1.Consistency consistency = 1;
* @return Whether the consistency field is set.
*/
@java.lang.Override
public boolean hasConsistency() {
return consistency_ != null;
}
/**
* .authzed.api.v1.Consistency consistency = 1;
* @return The consistency.
*/
@java.lang.Override
public com.authzed.api.v1.PermissionService.Consistency getConsistency() {
return consistency_ == null ? com.authzed.api.v1.PermissionService.Consistency.getDefaultInstance() : consistency_;
}
/**
* .authzed.api.v1.Consistency consistency = 1;
*/
@java.lang.Override
public com.authzed.api.v1.PermissionService.ConsistencyOrBuilder getConsistencyOrBuilder() {
return consistency_ == null ? com.authzed.api.v1.PermissionService.Consistency.getDefaultInstance() : consistency_;
}
public static final int RESOURCE_FIELD_NUMBER = 2;
private com.authzed.api.v1.Core.ObjectReference resource_;
/**
*
* resource is the resource for which all matching subjects for the permission
* or relation will be returned.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
* @return Whether the resource field is set.
*/
@java.lang.Override
public boolean hasResource() {
return resource_ != null;
}
/**
*
* resource is the resource for which all matching subjects for the permission
* or relation will be returned.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
* @return The resource.
*/
@java.lang.Override
public com.authzed.api.v1.Core.ObjectReference getResource() {
return resource_ == null ? com.authzed.api.v1.Core.ObjectReference.getDefaultInstance() : resource_;
}
/**
*
* resource is the resource for which all matching subjects for the permission
* or relation will be returned.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
*/
@java.lang.Override
public com.authzed.api.v1.Core.ObjectReferenceOrBuilder getResourceOrBuilder() {
return resource_ == null ? com.authzed.api.v1.Core.ObjectReference.getDefaultInstance() : resource_;
}
public static final int PERMISSION_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private volatile java.lang.Object permission_ = "";
/**
*
* permission is the name of the permission (or relation) for which to find
* the subjects.
*
*
* string permission = 3 [(.validate.rules) = { ... }
* @return The permission.
*/
@java.lang.Override
public java.lang.String getPermission() {
java.lang.Object ref = permission_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
permission_ = s;
return s;
}
}
/**
*
* permission is the name of the permission (or relation) for which to find
* the subjects.
*
*
* string permission = 3 [(.validate.rules) = { ... }
* @return The bytes for permission.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getPermissionBytes() {
java.lang.Object ref = permission_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
permission_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SUBJECT_OBJECT_TYPE_FIELD_NUMBER = 4;
@SuppressWarnings("serial")
private volatile java.lang.Object subjectObjectType_ = "";
/**
*
* subject_object_type is the type of subject object for which the IDs will
* be returned.
*
*
* string subject_object_type = 4 [(.validate.rules) = { ... }
* @return The subjectObjectType.
*/
@java.lang.Override
public java.lang.String getSubjectObjectType() {
java.lang.Object ref = subjectObjectType_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
subjectObjectType_ = s;
return s;
}
}
/**
*
* subject_object_type is the type of subject object for which the IDs will
* be returned.
*
*
* string subject_object_type = 4 [(.validate.rules) = { ... }
* @return The bytes for subjectObjectType.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getSubjectObjectTypeBytes() {
java.lang.Object ref = subjectObjectType_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
subjectObjectType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int OPTIONAL_SUBJECT_RELATION_FIELD_NUMBER = 5;
@SuppressWarnings("serial")
private volatile java.lang.Object optionalSubjectRelation_ = "";
/**
*
* optional_subject_relation is the optional relation for the subject.
*
*
* string optional_subject_relation = 5 [(.validate.rules) = { ... }
* @return The optionalSubjectRelation.
*/
@java.lang.Override
public java.lang.String getOptionalSubjectRelation() {
java.lang.Object ref = optionalSubjectRelation_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
optionalSubjectRelation_ = s;
return s;
}
}
/**
*
* optional_subject_relation is the optional relation for the subject.
*
*
* string optional_subject_relation = 5 [(.validate.rules) = { ... }
* @return The bytes for optionalSubjectRelation.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getOptionalSubjectRelationBytes() {
java.lang.Object ref = optionalSubjectRelation_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
optionalSubjectRelation_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CONTEXT_FIELD_NUMBER = 6;
private com.google.protobuf.Struct context_;
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 6 [(.validate.rules) = { ... }
* @return Whether the context field is set.
*/
@java.lang.Override
public boolean hasContext() {
return context_ != null;
}
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 6 [(.validate.rules) = { ... }
* @return The context.
*/
@java.lang.Override
public com.google.protobuf.Struct getContext() {
return context_ == null ? com.google.protobuf.Struct.getDefaultInstance() : context_;
}
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 6 [(.validate.rules) = { ... }
*/
@java.lang.Override
public com.google.protobuf.StructOrBuilder getContextOrBuilder() {
return context_ == null ? com.google.protobuf.Struct.getDefaultInstance() : context_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (consistency_ != null) {
output.writeMessage(1, getConsistency());
}
if (resource_ != null) {
output.writeMessage(2, getResource());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(permission_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, permission_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(subjectObjectType_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, subjectObjectType_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(optionalSubjectRelation_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, optionalSubjectRelation_);
}
if (context_ != null) {
output.writeMessage(6, getContext());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (consistency_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getConsistency());
}
if (resource_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getResource());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(permission_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, permission_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(subjectObjectType_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, subjectObjectType_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(optionalSubjectRelation_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, optionalSubjectRelation_);
}
if (context_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, getContext());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.authzed.api.v1.PermissionService.LookupSubjectsRequest)) {
return super.equals(obj);
}
com.authzed.api.v1.PermissionService.LookupSubjectsRequest other = (com.authzed.api.v1.PermissionService.LookupSubjectsRequest) obj;
if (hasConsistency() != other.hasConsistency()) return false;
if (hasConsistency()) {
if (!getConsistency()
.equals(other.getConsistency())) return false;
}
if (hasResource() != other.hasResource()) return false;
if (hasResource()) {
if (!getResource()
.equals(other.getResource())) return false;
}
if (!getPermission()
.equals(other.getPermission())) return false;
if (!getSubjectObjectType()
.equals(other.getSubjectObjectType())) return false;
if (!getOptionalSubjectRelation()
.equals(other.getOptionalSubjectRelation())) return false;
if (hasContext() != other.hasContext()) return false;
if (hasContext()) {
if (!getContext()
.equals(other.getContext())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasConsistency()) {
hash = (37 * hash) + CONSISTENCY_FIELD_NUMBER;
hash = (53 * hash) + getConsistency().hashCode();
}
if (hasResource()) {
hash = (37 * hash) + RESOURCE_FIELD_NUMBER;
hash = (53 * hash) + getResource().hashCode();
}
hash = (37 * hash) + PERMISSION_FIELD_NUMBER;
hash = (53 * hash) + getPermission().hashCode();
hash = (37 * hash) + SUBJECT_OBJECT_TYPE_FIELD_NUMBER;
hash = (53 * hash) + getSubjectObjectType().hashCode();
hash = (37 * hash) + OPTIONAL_SUBJECT_RELATION_FIELD_NUMBER;
hash = (53 * hash) + getOptionalSubjectRelation().hashCode();
if (hasContext()) {
hash = (37 * hash) + CONTEXT_FIELD_NUMBER;
hash = (53 * hash) + getContext().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.authzed.api.v1.PermissionService.LookupSubjectsRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.LookupSubjectsRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.LookupSubjectsRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.LookupSubjectsRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.LookupSubjectsRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.LookupSubjectsRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.LookupSubjectsRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.LookupSubjectsRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.LookupSubjectsRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.LookupSubjectsRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.LookupSubjectsRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.LookupSubjectsRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.authzed.api.v1.PermissionService.LookupSubjectsRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* LookupSubjectsRequest performs a lookup of all subjects of a particular
* kind for which the subject has the specified permission or the relation in
* which the subject exists, streaming back the IDs of those subjects.
*
*
* Protobuf type {@code authzed.api.v1.LookupSubjectsRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:authzed.api.v1.LookupSubjectsRequest)
com.authzed.api.v1.PermissionService.LookupSubjectsRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_LookupSubjectsRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_LookupSubjectsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.PermissionService.LookupSubjectsRequest.class, com.authzed.api.v1.PermissionService.LookupSubjectsRequest.Builder.class);
}
// Construct using com.authzed.api.v1.PermissionService.LookupSubjectsRequest.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
consistency_ = null;
if (consistencyBuilder_ != null) {
consistencyBuilder_.dispose();
consistencyBuilder_ = null;
}
resource_ = null;
if (resourceBuilder_ != null) {
resourceBuilder_.dispose();
resourceBuilder_ = null;
}
permission_ = "";
subjectObjectType_ = "";
optionalSubjectRelation_ = "";
context_ = null;
if (contextBuilder_ != null) {
contextBuilder_.dispose();
contextBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_LookupSubjectsRequest_descriptor;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.LookupSubjectsRequest getDefaultInstanceForType() {
return com.authzed.api.v1.PermissionService.LookupSubjectsRequest.getDefaultInstance();
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.LookupSubjectsRequest build() {
com.authzed.api.v1.PermissionService.LookupSubjectsRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.LookupSubjectsRequest buildPartial() {
com.authzed.api.v1.PermissionService.LookupSubjectsRequest result = new com.authzed.api.v1.PermissionService.LookupSubjectsRequest(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.authzed.api.v1.PermissionService.LookupSubjectsRequest result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.consistency_ = consistencyBuilder_ == null
? consistency_
: consistencyBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.resource_ = resourceBuilder_ == null
? resource_
: resourceBuilder_.build();
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.permission_ = permission_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.subjectObjectType_ = subjectObjectType_;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.optionalSubjectRelation_ = optionalSubjectRelation_;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.context_ = contextBuilder_ == null
? context_
: contextBuilder_.build();
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.authzed.api.v1.PermissionService.LookupSubjectsRequest) {
return mergeFrom((com.authzed.api.v1.PermissionService.LookupSubjectsRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.authzed.api.v1.PermissionService.LookupSubjectsRequest other) {
if (other == com.authzed.api.v1.PermissionService.LookupSubjectsRequest.getDefaultInstance()) return this;
if (other.hasConsistency()) {
mergeConsistency(other.getConsistency());
}
if (other.hasResource()) {
mergeResource(other.getResource());
}
if (!other.getPermission().isEmpty()) {
permission_ = other.permission_;
bitField0_ |= 0x00000004;
onChanged();
}
if (!other.getSubjectObjectType().isEmpty()) {
subjectObjectType_ = other.subjectObjectType_;
bitField0_ |= 0x00000008;
onChanged();
}
if (!other.getOptionalSubjectRelation().isEmpty()) {
optionalSubjectRelation_ = other.optionalSubjectRelation_;
bitField0_ |= 0x00000010;
onChanged();
}
if (other.hasContext()) {
mergeContext(other.getContext());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
input.readMessage(
getConsistencyFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
input.readMessage(
getResourceFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000002;
break;
} // case 18
case 26: {
permission_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000004;
break;
} // case 26
case 34: {
subjectObjectType_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000008;
break;
} // case 34
case 42: {
optionalSubjectRelation_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000010;
break;
} // case 42
case 50: {
input.readMessage(
getContextFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000020;
break;
} // case 50
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.authzed.api.v1.PermissionService.Consistency consistency_;
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.PermissionService.Consistency, com.authzed.api.v1.PermissionService.Consistency.Builder, com.authzed.api.v1.PermissionService.ConsistencyOrBuilder> consistencyBuilder_;
/**
* .authzed.api.v1.Consistency consistency = 1;
* @return Whether the consistency field is set.
*/
public boolean hasConsistency() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* .authzed.api.v1.Consistency consistency = 1;
* @return The consistency.
*/
public com.authzed.api.v1.PermissionService.Consistency getConsistency() {
if (consistencyBuilder_ == null) {
return consistency_ == null ? com.authzed.api.v1.PermissionService.Consistency.getDefaultInstance() : consistency_;
} else {
return consistencyBuilder_.getMessage();
}
}
/**
* .authzed.api.v1.Consistency consistency = 1;
*/
public Builder setConsistency(com.authzed.api.v1.PermissionService.Consistency value) {
if (consistencyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
consistency_ = value;
} else {
consistencyBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .authzed.api.v1.Consistency consistency = 1;
*/
public Builder setConsistency(
com.authzed.api.v1.PermissionService.Consistency.Builder builderForValue) {
if (consistencyBuilder_ == null) {
consistency_ = builderForValue.build();
} else {
consistencyBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .authzed.api.v1.Consistency consistency = 1;
*/
public Builder mergeConsistency(com.authzed.api.v1.PermissionService.Consistency value) {
if (consistencyBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0) &&
consistency_ != null &&
consistency_ != com.authzed.api.v1.PermissionService.Consistency.getDefaultInstance()) {
getConsistencyBuilder().mergeFrom(value);
} else {
consistency_ = value;
}
} else {
consistencyBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .authzed.api.v1.Consistency consistency = 1;
*/
public Builder clearConsistency() {
bitField0_ = (bitField0_ & ~0x00000001);
consistency_ = null;
if (consistencyBuilder_ != null) {
consistencyBuilder_.dispose();
consistencyBuilder_ = null;
}
onChanged();
return this;
}
/**
* .authzed.api.v1.Consistency consistency = 1;
*/
public com.authzed.api.v1.PermissionService.Consistency.Builder getConsistencyBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getConsistencyFieldBuilder().getBuilder();
}
/**
* .authzed.api.v1.Consistency consistency = 1;
*/
public com.authzed.api.v1.PermissionService.ConsistencyOrBuilder getConsistencyOrBuilder() {
if (consistencyBuilder_ != null) {
return consistencyBuilder_.getMessageOrBuilder();
} else {
return consistency_ == null ?
com.authzed.api.v1.PermissionService.Consistency.getDefaultInstance() : consistency_;
}
}
/**
* .authzed.api.v1.Consistency consistency = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.PermissionService.Consistency, com.authzed.api.v1.PermissionService.Consistency.Builder, com.authzed.api.v1.PermissionService.ConsistencyOrBuilder>
getConsistencyFieldBuilder() {
if (consistencyBuilder_ == null) {
consistencyBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.PermissionService.Consistency, com.authzed.api.v1.PermissionService.Consistency.Builder, com.authzed.api.v1.PermissionService.ConsistencyOrBuilder>(
getConsistency(),
getParentForChildren(),
isClean());
consistency_ = null;
}
return consistencyBuilder_;
}
private com.authzed.api.v1.Core.ObjectReference resource_;
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.ObjectReference, com.authzed.api.v1.Core.ObjectReference.Builder, com.authzed.api.v1.Core.ObjectReferenceOrBuilder> resourceBuilder_;
/**
*
* resource is the resource for which all matching subjects for the permission
* or relation will be returned.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
* @return Whether the resource field is set.
*/
public boolean hasResource() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* resource is the resource for which all matching subjects for the permission
* or relation will be returned.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
* @return The resource.
*/
public com.authzed.api.v1.Core.ObjectReference getResource() {
if (resourceBuilder_ == null) {
return resource_ == null ? com.authzed.api.v1.Core.ObjectReference.getDefaultInstance() : resource_;
} else {
return resourceBuilder_.getMessage();
}
}
/**
*
* resource is the resource for which all matching subjects for the permission
* or relation will be returned.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
*/
public Builder setResource(com.authzed.api.v1.Core.ObjectReference value) {
if (resourceBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
resource_ = value;
} else {
resourceBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* resource is the resource for which all matching subjects for the permission
* or relation will be returned.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
*/
public Builder setResource(
com.authzed.api.v1.Core.ObjectReference.Builder builderForValue) {
if (resourceBuilder_ == null) {
resource_ = builderForValue.build();
} else {
resourceBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* resource is the resource for which all matching subjects for the permission
* or relation will be returned.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
*/
public Builder mergeResource(com.authzed.api.v1.Core.ObjectReference value) {
if (resourceBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0) &&
resource_ != null &&
resource_ != com.authzed.api.v1.Core.ObjectReference.getDefaultInstance()) {
getResourceBuilder().mergeFrom(value);
} else {
resource_ = value;
}
} else {
resourceBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* resource is the resource for which all matching subjects for the permission
* or relation will be returned.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
*/
public Builder clearResource() {
bitField0_ = (bitField0_ & ~0x00000002);
resource_ = null;
if (resourceBuilder_ != null) {
resourceBuilder_.dispose();
resourceBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* resource is the resource for which all matching subjects for the permission
* or relation will be returned.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
*/
public com.authzed.api.v1.Core.ObjectReference.Builder getResourceBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getResourceFieldBuilder().getBuilder();
}
/**
*
* resource is the resource for which all matching subjects for the permission
* or relation will be returned.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
*/
public com.authzed.api.v1.Core.ObjectReferenceOrBuilder getResourceOrBuilder() {
if (resourceBuilder_ != null) {
return resourceBuilder_.getMessageOrBuilder();
} else {
return resource_ == null ?
com.authzed.api.v1.Core.ObjectReference.getDefaultInstance() : resource_;
}
}
/**
*
* resource is the resource for which all matching subjects for the permission
* or relation will be returned.
*
*
* .authzed.api.v1.ObjectReference resource = 2 [(.validate.rules) = { ... }
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.ObjectReference, com.authzed.api.v1.Core.ObjectReference.Builder, com.authzed.api.v1.Core.ObjectReferenceOrBuilder>
getResourceFieldBuilder() {
if (resourceBuilder_ == null) {
resourceBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.ObjectReference, com.authzed.api.v1.Core.ObjectReference.Builder, com.authzed.api.v1.Core.ObjectReferenceOrBuilder>(
getResource(),
getParentForChildren(),
isClean());
resource_ = null;
}
return resourceBuilder_;
}
private java.lang.Object permission_ = "";
/**
*
* permission is the name of the permission (or relation) for which to find
* the subjects.
*
*
* string permission = 3 [(.validate.rules) = { ... }
* @return The permission.
*/
public java.lang.String getPermission() {
java.lang.Object ref = permission_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
permission_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* permission is the name of the permission (or relation) for which to find
* the subjects.
*
*
* string permission = 3 [(.validate.rules) = { ... }
* @return The bytes for permission.
*/
public com.google.protobuf.ByteString
getPermissionBytes() {
java.lang.Object ref = permission_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
permission_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* permission is the name of the permission (or relation) for which to find
* the subjects.
*
*
* string permission = 3 [(.validate.rules) = { ... }
* @param value The permission to set.
* @return This builder for chaining.
*/
public Builder setPermission(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
permission_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* permission is the name of the permission (or relation) for which to find
* the subjects.
*
*
* string permission = 3 [(.validate.rules) = { ... }
* @return This builder for chaining.
*/
public Builder clearPermission() {
permission_ = getDefaultInstance().getPermission();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
*
* permission is the name of the permission (or relation) for which to find
* the subjects.
*
*
* string permission = 3 [(.validate.rules) = { ... }
* @param value The bytes for permission to set.
* @return This builder for chaining.
*/
public Builder setPermissionBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
permission_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
private java.lang.Object subjectObjectType_ = "";
/**
*
* subject_object_type is the type of subject object for which the IDs will
* be returned.
*
*
* string subject_object_type = 4 [(.validate.rules) = { ... }
* @return The subjectObjectType.
*/
public java.lang.String getSubjectObjectType() {
java.lang.Object ref = subjectObjectType_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
subjectObjectType_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* subject_object_type is the type of subject object for which the IDs will
* be returned.
*
*
* string subject_object_type = 4 [(.validate.rules) = { ... }
* @return The bytes for subjectObjectType.
*/
public com.google.protobuf.ByteString
getSubjectObjectTypeBytes() {
java.lang.Object ref = subjectObjectType_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
subjectObjectType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* subject_object_type is the type of subject object for which the IDs will
* be returned.
*
*
* string subject_object_type = 4 [(.validate.rules) = { ... }
* @param value The subjectObjectType to set.
* @return This builder for chaining.
*/
public Builder setSubjectObjectType(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
subjectObjectType_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* subject_object_type is the type of subject object for which the IDs will
* be returned.
*
*
* string subject_object_type = 4 [(.validate.rules) = { ... }
* @return This builder for chaining.
*/
public Builder clearSubjectObjectType() {
subjectObjectType_ = getDefaultInstance().getSubjectObjectType();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
return this;
}
/**
*
* subject_object_type is the type of subject object for which the IDs will
* be returned.
*
*
* string subject_object_type = 4 [(.validate.rules) = { ... }
* @param value The bytes for subjectObjectType to set.
* @return This builder for chaining.
*/
public Builder setSubjectObjectTypeBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
subjectObjectType_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
private java.lang.Object optionalSubjectRelation_ = "";
/**
*
* optional_subject_relation is the optional relation for the subject.
*
*
* string optional_subject_relation = 5 [(.validate.rules) = { ... }
* @return The optionalSubjectRelation.
*/
public java.lang.String getOptionalSubjectRelation() {
java.lang.Object ref = optionalSubjectRelation_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
optionalSubjectRelation_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* optional_subject_relation is the optional relation for the subject.
*
*
* string optional_subject_relation = 5 [(.validate.rules) = { ... }
* @return The bytes for optionalSubjectRelation.
*/
public com.google.protobuf.ByteString
getOptionalSubjectRelationBytes() {
java.lang.Object ref = optionalSubjectRelation_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
optionalSubjectRelation_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* optional_subject_relation is the optional relation for the subject.
*
*
* string optional_subject_relation = 5 [(.validate.rules) = { ... }
* @param value The optionalSubjectRelation to set.
* @return This builder for chaining.
*/
public Builder setOptionalSubjectRelation(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
optionalSubjectRelation_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
* optional_subject_relation is the optional relation for the subject.
*
*
* string optional_subject_relation = 5 [(.validate.rules) = { ... }
* @return This builder for chaining.
*/
public Builder clearOptionalSubjectRelation() {
optionalSubjectRelation_ = getDefaultInstance().getOptionalSubjectRelation();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
return this;
}
/**
*
* optional_subject_relation is the optional relation for the subject.
*
*
* string optional_subject_relation = 5 [(.validate.rules) = { ... }
* @param value The bytes for optionalSubjectRelation to set.
* @return This builder for chaining.
*/
public Builder setOptionalSubjectRelationBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
optionalSubjectRelation_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
private com.google.protobuf.Struct context_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Struct, com.google.protobuf.Struct.Builder, com.google.protobuf.StructOrBuilder> contextBuilder_;
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 6 [(.validate.rules) = { ... }
* @return Whether the context field is set.
*/
public boolean hasContext() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 6 [(.validate.rules) = { ... }
* @return The context.
*/
public com.google.protobuf.Struct getContext() {
if (contextBuilder_ == null) {
return context_ == null ? com.google.protobuf.Struct.getDefaultInstance() : context_;
} else {
return contextBuilder_.getMessage();
}
}
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 6 [(.validate.rules) = { ... }
*/
public Builder setContext(com.google.protobuf.Struct value) {
if (contextBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
context_ = value;
} else {
contextBuilder_.setMessage(value);
}
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 6 [(.validate.rules) = { ... }
*/
public Builder setContext(
com.google.protobuf.Struct.Builder builderForValue) {
if (contextBuilder_ == null) {
context_ = builderForValue.build();
} else {
contextBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 6 [(.validate.rules) = { ... }
*/
public Builder mergeContext(com.google.protobuf.Struct value) {
if (contextBuilder_ == null) {
if (((bitField0_ & 0x00000020) != 0) &&
context_ != null &&
context_ != com.google.protobuf.Struct.getDefaultInstance()) {
getContextBuilder().mergeFrom(value);
} else {
context_ = value;
}
} else {
contextBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 6 [(.validate.rules) = { ... }
*/
public Builder clearContext() {
bitField0_ = (bitField0_ & ~0x00000020);
context_ = null;
if (contextBuilder_ != null) {
contextBuilder_.dispose();
contextBuilder_ = null;
}
onChanged();
return this;
}
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 6 [(.validate.rules) = { ... }
*/
public com.google.protobuf.Struct.Builder getContextBuilder() {
bitField0_ |= 0x00000020;
onChanged();
return getContextFieldBuilder().getBuilder();
}
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 6 [(.validate.rules) = { ... }
*/
public com.google.protobuf.StructOrBuilder getContextOrBuilder() {
if (contextBuilder_ != null) {
return contextBuilder_.getMessageOrBuilder();
} else {
return context_ == null ?
com.google.protobuf.Struct.getDefaultInstance() : context_;
}
}
/**
*
** context consists of named values that are injected into the caveat evaluation context *
*
*
* .google.protobuf.Struct context = 6 [(.validate.rules) = { ... }
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Struct, com.google.protobuf.Struct.Builder, com.google.protobuf.StructOrBuilder>
getContextFieldBuilder() {
if (contextBuilder_ == null) {
contextBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Struct, com.google.protobuf.Struct.Builder, com.google.protobuf.StructOrBuilder>(
getContext(),
getParentForChildren(),
isClean());
context_ = null;
}
return contextBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:authzed.api.v1.LookupSubjectsRequest)
}
// @@protoc_insertion_point(class_scope:authzed.api.v1.LookupSubjectsRequest)
private static final com.authzed.api.v1.PermissionService.LookupSubjectsRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.authzed.api.v1.PermissionService.LookupSubjectsRequest();
}
public static com.authzed.api.v1.PermissionService.LookupSubjectsRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public LookupSubjectsRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.LookupSubjectsRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface LookupSubjectsResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:authzed.api.v1.LookupSubjectsResponse)
com.google.protobuf.MessageOrBuilder {
/**
* .authzed.api.v1.ZedToken looked_up_at = 1;
* @return Whether the lookedUpAt field is set.
*/
boolean hasLookedUpAt();
/**
* .authzed.api.v1.ZedToken looked_up_at = 1;
* @return The lookedUpAt.
*/
com.authzed.api.v1.Core.ZedToken getLookedUpAt();
/**
* .authzed.api.v1.ZedToken looked_up_at = 1;
*/
com.authzed.api.v1.Core.ZedTokenOrBuilder getLookedUpAtOrBuilder();
/**
*
* subject_object_id is the Object ID of the subject found. May be a `*` if
* a wildcard was found.
* deprecated: use `subject`
*
*
* string subject_object_id = 2 [deprecated = true];
* @deprecated authzed.api.v1.LookupSubjectsResponse.subject_object_id is deprecated.
* See authzed/api/v1/permission_service.proto;l=401
* @return The subjectObjectId.
*/
@java.lang.Deprecated java.lang.String getSubjectObjectId();
/**
*
* subject_object_id is the Object ID of the subject found. May be a `*` if
* a wildcard was found.
* deprecated: use `subject`
*
*
* string subject_object_id = 2 [deprecated = true];
* @deprecated authzed.api.v1.LookupSubjectsResponse.subject_object_id is deprecated.
* See authzed/api/v1/permission_service.proto;l=401
* @return The bytes for subjectObjectId.
*/
@java.lang.Deprecated com.google.protobuf.ByteString
getSubjectObjectIdBytes();
/**
*
* excluded_subject_ids are the Object IDs of the subjects excluded. This list
* will only contain object IDs if `subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
* deprecated: use `excluded_subjects`
*
*
* repeated string excluded_subject_ids = 3 [deprecated = true];
* @deprecated authzed.api.v1.LookupSubjectsResponse.excluded_subject_ids is deprecated.
* See authzed/api/v1/permission_service.proto;l=407
* @return A list containing the excludedSubjectIds.
*/
@java.lang.Deprecated java.util.List
getExcludedSubjectIdsList();
/**
*
* excluded_subject_ids are the Object IDs of the subjects excluded. This list
* will only contain object IDs if `subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
* deprecated: use `excluded_subjects`
*
*
* repeated string excluded_subject_ids = 3 [deprecated = true];
* @deprecated authzed.api.v1.LookupSubjectsResponse.excluded_subject_ids is deprecated.
* See authzed/api/v1/permission_service.proto;l=407
* @return The count of excludedSubjectIds.
*/
@java.lang.Deprecated int getExcludedSubjectIdsCount();
/**
*
* excluded_subject_ids are the Object IDs of the subjects excluded. This list
* will only contain object IDs if `subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
* deprecated: use `excluded_subjects`
*
*
* repeated string excluded_subject_ids = 3 [deprecated = true];
* @deprecated authzed.api.v1.LookupSubjectsResponse.excluded_subject_ids is deprecated.
* See authzed/api/v1/permission_service.proto;l=407
* @param index The index of the element to return.
* @return The excludedSubjectIds at the given index.
*/
@java.lang.Deprecated java.lang.String getExcludedSubjectIds(int index);
/**
*
* excluded_subject_ids are the Object IDs of the subjects excluded. This list
* will only contain object IDs if `subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
* deprecated: use `excluded_subjects`
*
*
* repeated string excluded_subject_ids = 3 [deprecated = true];
* @deprecated authzed.api.v1.LookupSubjectsResponse.excluded_subject_ids is deprecated.
* See authzed/api/v1/permission_service.proto;l=407
* @param index The index of the value to return.
* @return The bytes of the excludedSubjectIds at the given index.
*/
@java.lang.Deprecated com.google.protobuf.ByteString
getExcludedSubjectIdsBytes(int index);
/**
*
* permissionship indicates whether the response was partially evaluated or not
* deprecated: use `subject.permissionship`
*
*
* .authzed.api.v1.LookupPermissionship permissionship = 4 [deprecated = true, (.validate.rules) = { ... }
* @deprecated authzed.api.v1.LookupSubjectsResponse.permissionship is deprecated.
* See authzed/api/v1/permission_service.proto;l=411
* @return The enum numeric value on the wire for permissionship.
*/
@java.lang.Deprecated int getPermissionshipValue();
/**
*
* permissionship indicates whether the response was partially evaluated or not
* deprecated: use `subject.permissionship`
*
*
* .authzed.api.v1.LookupPermissionship permissionship = 4 [deprecated = true, (.validate.rules) = { ... }
* @deprecated authzed.api.v1.LookupSubjectsResponse.permissionship is deprecated.
* See authzed/api/v1/permission_service.proto;l=411
* @return The permissionship.
*/
@java.lang.Deprecated com.authzed.api.v1.PermissionService.LookupPermissionship getPermissionship();
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
* deprecated: use `subject.partial_caveat_info`
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 5 [deprecated = true, (.validate.rules) = { ... }
* @deprecated authzed.api.v1.LookupSubjectsResponse.partial_caveat_info is deprecated.
* See authzed/api/v1/permission_service.proto;l=415
* @return Whether the partialCaveatInfo field is set.
*/
@java.lang.Deprecated boolean hasPartialCaveatInfo();
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
* deprecated: use `subject.partial_caveat_info`
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 5 [deprecated = true, (.validate.rules) = { ... }
* @deprecated authzed.api.v1.LookupSubjectsResponse.partial_caveat_info is deprecated.
* See authzed/api/v1/permission_service.proto;l=415
* @return The partialCaveatInfo.
*/
@java.lang.Deprecated com.authzed.api.v1.Core.PartialCaveatInfo getPartialCaveatInfo();
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
* deprecated: use `subject.partial_caveat_info`
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 5 [deprecated = true, (.validate.rules) = { ... }
*/
@java.lang.Deprecated com.authzed.api.v1.Core.PartialCaveatInfoOrBuilder getPartialCaveatInfoOrBuilder();
/**
*
* subject is the subject found, along with its permissionship.
*
*
* .authzed.api.v1.ResolvedSubject subject = 6;
* @return Whether the subject field is set.
*/
boolean hasSubject();
/**
*
* subject is the subject found, along with its permissionship.
*
*
* .authzed.api.v1.ResolvedSubject subject = 6;
* @return The subject.
*/
com.authzed.api.v1.PermissionService.ResolvedSubject getSubject();
/**
*
* subject is the subject found, along with its permissionship.
*
*
* .authzed.api.v1.ResolvedSubject subject = 6;
*/
com.authzed.api.v1.PermissionService.ResolvedSubjectOrBuilder getSubjectOrBuilder();
/**
*
* excluded_subjects are the subjects excluded. This list
* will only contain subjects if `subject.subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
*
*
* repeated .authzed.api.v1.ResolvedSubject excluded_subjects = 7;
*/
java.util.List
getExcludedSubjectsList();
/**
*
* excluded_subjects are the subjects excluded. This list
* will only contain subjects if `subject.subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
*
*
* repeated .authzed.api.v1.ResolvedSubject excluded_subjects = 7;
*/
com.authzed.api.v1.PermissionService.ResolvedSubject getExcludedSubjects(int index);
/**
*
* excluded_subjects are the subjects excluded. This list
* will only contain subjects if `subject.subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
*
*
* repeated .authzed.api.v1.ResolvedSubject excluded_subjects = 7;
*/
int getExcludedSubjectsCount();
/**
*
* excluded_subjects are the subjects excluded. This list
* will only contain subjects if `subject.subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
*
*
* repeated .authzed.api.v1.ResolvedSubject excluded_subjects = 7;
*/
java.util.List extends com.authzed.api.v1.PermissionService.ResolvedSubjectOrBuilder>
getExcludedSubjectsOrBuilderList();
/**
*
* excluded_subjects are the subjects excluded. This list
* will only contain subjects if `subject.subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
*
*
* repeated .authzed.api.v1.ResolvedSubject excluded_subjects = 7;
*/
com.authzed.api.v1.PermissionService.ResolvedSubjectOrBuilder getExcludedSubjectsOrBuilder(
int index);
}
/**
*
* LookupSubjectsResponse contains a single matching subject object ID for the
* requested subject object type on the permission or relation.
*
*
* Protobuf type {@code authzed.api.v1.LookupSubjectsResponse}
*/
public static final class LookupSubjectsResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:authzed.api.v1.LookupSubjectsResponse)
LookupSubjectsResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use LookupSubjectsResponse.newBuilder() to construct.
private LookupSubjectsResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private LookupSubjectsResponse() {
subjectObjectId_ = "";
excludedSubjectIds_ = com.google.protobuf.LazyStringArrayList.EMPTY;
permissionship_ = 0;
excludedSubjects_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new LookupSubjectsResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_LookupSubjectsResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_LookupSubjectsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.PermissionService.LookupSubjectsResponse.class, com.authzed.api.v1.PermissionService.LookupSubjectsResponse.Builder.class);
}
public static final int LOOKED_UP_AT_FIELD_NUMBER = 1;
private com.authzed.api.v1.Core.ZedToken lookedUpAt_;
/**
* .authzed.api.v1.ZedToken looked_up_at = 1;
* @return Whether the lookedUpAt field is set.
*/
@java.lang.Override
public boolean hasLookedUpAt() {
return lookedUpAt_ != null;
}
/**
* .authzed.api.v1.ZedToken looked_up_at = 1;
* @return The lookedUpAt.
*/
@java.lang.Override
public com.authzed.api.v1.Core.ZedToken getLookedUpAt() {
return lookedUpAt_ == null ? com.authzed.api.v1.Core.ZedToken.getDefaultInstance() : lookedUpAt_;
}
/**
* .authzed.api.v1.ZedToken looked_up_at = 1;
*/
@java.lang.Override
public com.authzed.api.v1.Core.ZedTokenOrBuilder getLookedUpAtOrBuilder() {
return lookedUpAt_ == null ? com.authzed.api.v1.Core.ZedToken.getDefaultInstance() : lookedUpAt_;
}
public static final int SUBJECT_OBJECT_ID_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object subjectObjectId_ = "";
/**
*
* subject_object_id is the Object ID of the subject found. May be a `*` if
* a wildcard was found.
* deprecated: use `subject`
*
*
* string subject_object_id = 2 [deprecated = true];
* @deprecated authzed.api.v1.LookupSubjectsResponse.subject_object_id is deprecated.
* See authzed/api/v1/permission_service.proto;l=401
* @return The subjectObjectId.
*/
@java.lang.Override
@java.lang.Deprecated public java.lang.String getSubjectObjectId() {
java.lang.Object ref = subjectObjectId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
subjectObjectId_ = s;
return s;
}
}
/**
*
* subject_object_id is the Object ID of the subject found. May be a `*` if
* a wildcard was found.
* deprecated: use `subject`
*
*
* string subject_object_id = 2 [deprecated = true];
* @deprecated authzed.api.v1.LookupSubjectsResponse.subject_object_id is deprecated.
* See authzed/api/v1/permission_service.proto;l=401
* @return The bytes for subjectObjectId.
*/
@java.lang.Override
@java.lang.Deprecated public com.google.protobuf.ByteString
getSubjectObjectIdBytes() {
java.lang.Object ref = subjectObjectId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
subjectObjectId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int EXCLUDED_SUBJECT_IDS_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringList excludedSubjectIds_;
/**
*
* excluded_subject_ids are the Object IDs of the subjects excluded. This list
* will only contain object IDs if `subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
* deprecated: use `excluded_subjects`
*
*
* repeated string excluded_subject_ids = 3 [deprecated = true];
* @deprecated authzed.api.v1.LookupSubjectsResponse.excluded_subject_ids is deprecated.
* See authzed/api/v1/permission_service.proto;l=407
* @return A list containing the excludedSubjectIds.
*/
@java.lang.Deprecated public com.google.protobuf.ProtocolStringList
getExcludedSubjectIdsList() {
return excludedSubjectIds_;
}
/**
*
* excluded_subject_ids are the Object IDs of the subjects excluded. This list
* will only contain object IDs if `subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
* deprecated: use `excluded_subjects`
*
*
* repeated string excluded_subject_ids = 3 [deprecated = true];
* @deprecated authzed.api.v1.LookupSubjectsResponse.excluded_subject_ids is deprecated.
* See authzed/api/v1/permission_service.proto;l=407
* @return The count of excludedSubjectIds.
*/
@java.lang.Deprecated public int getExcludedSubjectIdsCount() {
return excludedSubjectIds_.size();
}
/**
*
* excluded_subject_ids are the Object IDs of the subjects excluded. This list
* will only contain object IDs if `subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
* deprecated: use `excluded_subjects`
*
*
* repeated string excluded_subject_ids = 3 [deprecated = true];
* @deprecated authzed.api.v1.LookupSubjectsResponse.excluded_subject_ids is deprecated.
* See authzed/api/v1/permission_service.proto;l=407
* @param index The index of the element to return.
* @return The excludedSubjectIds at the given index.
*/
@java.lang.Deprecated public java.lang.String getExcludedSubjectIds(int index) {
return excludedSubjectIds_.get(index);
}
/**
*
* excluded_subject_ids are the Object IDs of the subjects excluded. This list
* will only contain object IDs if `subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
* deprecated: use `excluded_subjects`
*
*
* repeated string excluded_subject_ids = 3 [deprecated = true];
* @deprecated authzed.api.v1.LookupSubjectsResponse.excluded_subject_ids is deprecated.
* See authzed/api/v1/permission_service.proto;l=407
* @param index The index of the value to return.
* @return The bytes of the excludedSubjectIds at the given index.
*/
@java.lang.Deprecated public com.google.protobuf.ByteString
getExcludedSubjectIdsBytes(int index) {
return excludedSubjectIds_.getByteString(index);
}
public static final int PERMISSIONSHIP_FIELD_NUMBER = 4;
private int permissionship_ = 0;
/**
*
* permissionship indicates whether the response was partially evaluated or not
* deprecated: use `subject.permissionship`
*
*
* .authzed.api.v1.LookupPermissionship permissionship = 4 [deprecated = true, (.validate.rules) = { ... }
* @deprecated authzed.api.v1.LookupSubjectsResponse.permissionship is deprecated.
* See authzed/api/v1/permission_service.proto;l=411
* @return The enum numeric value on the wire for permissionship.
*/
@java.lang.Override @java.lang.Deprecated public int getPermissionshipValue() {
return permissionship_;
}
/**
*
* permissionship indicates whether the response was partially evaluated or not
* deprecated: use `subject.permissionship`
*
*
* .authzed.api.v1.LookupPermissionship permissionship = 4 [deprecated = true, (.validate.rules) = { ... }
* @deprecated authzed.api.v1.LookupSubjectsResponse.permissionship is deprecated.
* See authzed/api/v1/permission_service.proto;l=411
* @return The permissionship.
*/
@java.lang.Override @java.lang.Deprecated public com.authzed.api.v1.PermissionService.LookupPermissionship getPermissionship() {
com.authzed.api.v1.PermissionService.LookupPermissionship result = com.authzed.api.v1.PermissionService.LookupPermissionship.forNumber(permissionship_);
return result == null ? com.authzed.api.v1.PermissionService.LookupPermissionship.UNRECOGNIZED : result;
}
public static final int PARTIAL_CAVEAT_INFO_FIELD_NUMBER = 5;
private com.authzed.api.v1.Core.PartialCaveatInfo partialCaveatInfo_;
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
* deprecated: use `subject.partial_caveat_info`
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 5 [deprecated = true, (.validate.rules) = { ... }
* @deprecated authzed.api.v1.LookupSubjectsResponse.partial_caveat_info is deprecated.
* See authzed/api/v1/permission_service.proto;l=415
* @return Whether the partialCaveatInfo field is set.
*/
@java.lang.Override
@java.lang.Deprecated public boolean hasPartialCaveatInfo() {
return partialCaveatInfo_ != null;
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
* deprecated: use `subject.partial_caveat_info`
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 5 [deprecated = true, (.validate.rules) = { ... }
* @deprecated authzed.api.v1.LookupSubjectsResponse.partial_caveat_info is deprecated.
* See authzed/api/v1/permission_service.proto;l=415
* @return The partialCaveatInfo.
*/
@java.lang.Override
@java.lang.Deprecated public com.authzed.api.v1.Core.PartialCaveatInfo getPartialCaveatInfo() {
return partialCaveatInfo_ == null ? com.authzed.api.v1.Core.PartialCaveatInfo.getDefaultInstance() : partialCaveatInfo_;
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
* deprecated: use `subject.partial_caveat_info`
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 5 [deprecated = true, (.validate.rules) = { ... }
*/
@java.lang.Override
@java.lang.Deprecated public com.authzed.api.v1.Core.PartialCaveatInfoOrBuilder getPartialCaveatInfoOrBuilder() {
return partialCaveatInfo_ == null ? com.authzed.api.v1.Core.PartialCaveatInfo.getDefaultInstance() : partialCaveatInfo_;
}
public static final int SUBJECT_FIELD_NUMBER = 6;
private com.authzed.api.v1.PermissionService.ResolvedSubject subject_;
/**
*
* subject is the subject found, along with its permissionship.
*
*
* .authzed.api.v1.ResolvedSubject subject = 6;
* @return Whether the subject field is set.
*/
@java.lang.Override
public boolean hasSubject() {
return subject_ != null;
}
/**
*
* subject is the subject found, along with its permissionship.
*
*
* .authzed.api.v1.ResolvedSubject subject = 6;
* @return The subject.
*/
@java.lang.Override
public com.authzed.api.v1.PermissionService.ResolvedSubject getSubject() {
return subject_ == null ? com.authzed.api.v1.PermissionService.ResolvedSubject.getDefaultInstance() : subject_;
}
/**
*
* subject is the subject found, along with its permissionship.
*
*
* .authzed.api.v1.ResolvedSubject subject = 6;
*/
@java.lang.Override
public com.authzed.api.v1.PermissionService.ResolvedSubjectOrBuilder getSubjectOrBuilder() {
return subject_ == null ? com.authzed.api.v1.PermissionService.ResolvedSubject.getDefaultInstance() : subject_;
}
public static final int EXCLUDED_SUBJECTS_FIELD_NUMBER = 7;
@SuppressWarnings("serial")
private java.util.List excludedSubjects_;
/**
*
* excluded_subjects are the subjects excluded. This list
* will only contain subjects if `subject.subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
*
*
* repeated .authzed.api.v1.ResolvedSubject excluded_subjects = 7;
*/
@java.lang.Override
public java.util.List getExcludedSubjectsList() {
return excludedSubjects_;
}
/**
*
* excluded_subjects are the subjects excluded. This list
* will only contain subjects if `subject.subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
*
*
* repeated .authzed.api.v1.ResolvedSubject excluded_subjects = 7;
*/
@java.lang.Override
public java.util.List extends com.authzed.api.v1.PermissionService.ResolvedSubjectOrBuilder>
getExcludedSubjectsOrBuilderList() {
return excludedSubjects_;
}
/**
*
* excluded_subjects are the subjects excluded. This list
* will only contain subjects if `subject.subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
*
*
* repeated .authzed.api.v1.ResolvedSubject excluded_subjects = 7;
*/
@java.lang.Override
public int getExcludedSubjectsCount() {
return excludedSubjects_.size();
}
/**
*
* excluded_subjects are the subjects excluded. This list
* will only contain subjects if `subject.subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
*
*
* repeated .authzed.api.v1.ResolvedSubject excluded_subjects = 7;
*/
@java.lang.Override
public com.authzed.api.v1.PermissionService.ResolvedSubject getExcludedSubjects(int index) {
return excludedSubjects_.get(index);
}
/**
*
* excluded_subjects are the subjects excluded. This list
* will only contain subjects if `subject.subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
*
*
* repeated .authzed.api.v1.ResolvedSubject excluded_subjects = 7;
*/
@java.lang.Override
public com.authzed.api.v1.PermissionService.ResolvedSubjectOrBuilder getExcludedSubjectsOrBuilder(
int index) {
return excludedSubjects_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (lookedUpAt_ != null) {
output.writeMessage(1, getLookedUpAt());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(subjectObjectId_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, subjectObjectId_);
}
for (int i = 0; i < excludedSubjectIds_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, excludedSubjectIds_.getRaw(i));
}
if (permissionship_ != com.authzed.api.v1.PermissionService.LookupPermissionship.LOOKUP_PERMISSIONSHIP_UNSPECIFIED.getNumber()) {
output.writeEnum(4, permissionship_);
}
if (partialCaveatInfo_ != null) {
output.writeMessage(5, getPartialCaveatInfo());
}
if (subject_ != null) {
output.writeMessage(6, getSubject());
}
for (int i = 0; i < excludedSubjects_.size(); i++) {
output.writeMessage(7, excludedSubjects_.get(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (lookedUpAt_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getLookedUpAt());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(subjectObjectId_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, subjectObjectId_);
}
{
int dataSize = 0;
for (int i = 0; i < excludedSubjectIds_.size(); i++) {
dataSize += computeStringSizeNoTag(excludedSubjectIds_.getRaw(i));
}
size += dataSize;
size += 1 * getExcludedSubjectIdsList().size();
}
if (permissionship_ != com.authzed.api.v1.PermissionService.LookupPermissionship.LOOKUP_PERMISSIONSHIP_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(4, permissionship_);
}
if (partialCaveatInfo_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, getPartialCaveatInfo());
}
if (subject_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, getSubject());
}
for (int i = 0; i < excludedSubjects_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, excludedSubjects_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.authzed.api.v1.PermissionService.LookupSubjectsResponse)) {
return super.equals(obj);
}
com.authzed.api.v1.PermissionService.LookupSubjectsResponse other = (com.authzed.api.v1.PermissionService.LookupSubjectsResponse) obj;
if (hasLookedUpAt() != other.hasLookedUpAt()) return false;
if (hasLookedUpAt()) {
if (!getLookedUpAt()
.equals(other.getLookedUpAt())) return false;
}
if (!getSubjectObjectId()
.equals(other.getSubjectObjectId())) return false;
if (!getExcludedSubjectIdsList()
.equals(other.getExcludedSubjectIdsList())) return false;
if (permissionship_ != other.permissionship_) return false;
if (hasPartialCaveatInfo() != other.hasPartialCaveatInfo()) return false;
if (hasPartialCaveatInfo()) {
if (!getPartialCaveatInfo()
.equals(other.getPartialCaveatInfo())) return false;
}
if (hasSubject() != other.hasSubject()) return false;
if (hasSubject()) {
if (!getSubject()
.equals(other.getSubject())) return false;
}
if (!getExcludedSubjectsList()
.equals(other.getExcludedSubjectsList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasLookedUpAt()) {
hash = (37 * hash) + LOOKED_UP_AT_FIELD_NUMBER;
hash = (53 * hash) + getLookedUpAt().hashCode();
}
hash = (37 * hash) + SUBJECT_OBJECT_ID_FIELD_NUMBER;
hash = (53 * hash) + getSubjectObjectId().hashCode();
if (getExcludedSubjectIdsCount() > 0) {
hash = (37 * hash) + EXCLUDED_SUBJECT_IDS_FIELD_NUMBER;
hash = (53 * hash) + getExcludedSubjectIdsList().hashCode();
}
hash = (37 * hash) + PERMISSIONSHIP_FIELD_NUMBER;
hash = (53 * hash) + permissionship_;
if (hasPartialCaveatInfo()) {
hash = (37 * hash) + PARTIAL_CAVEAT_INFO_FIELD_NUMBER;
hash = (53 * hash) + getPartialCaveatInfo().hashCode();
}
if (hasSubject()) {
hash = (37 * hash) + SUBJECT_FIELD_NUMBER;
hash = (53 * hash) + getSubject().hashCode();
}
if (getExcludedSubjectsCount() > 0) {
hash = (37 * hash) + EXCLUDED_SUBJECTS_FIELD_NUMBER;
hash = (53 * hash) + getExcludedSubjectsList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.authzed.api.v1.PermissionService.LookupSubjectsResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.LookupSubjectsResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.LookupSubjectsResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.LookupSubjectsResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.LookupSubjectsResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.LookupSubjectsResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.LookupSubjectsResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.LookupSubjectsResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.LookupSubjectsResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.LookupSubjectsResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.LookupSubjectsResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.LookupSubjectsResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.authzed.api.v1.PermissionService.LookupSubjectsResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* LookupSubjectsResponse contains a single matching subject object ID for the
* requested subject object type on the permission or relation.
*
*
* Protobuf type {@code authzed.api.v1.LookupSubjectsResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:authzed.api.v1.LookupSubjectsResponse)
com.authzed.api.v1.PermissionService.LookupSubjectsResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_LookupSubjectsResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_LookupSubjectsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.PermissionService.LookupSubjectsResponse.class, com.authzed.api.v1.PermissionService.LookupSubjectsResponse.Builder.class);
}
// Construct using com.authzed.api.v1.PermissionService.LookupSubjectsResponse.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
lookedUpAt_ = null;
if (lookedUpAtBuilder_ != null) {
lookedUpAtBuilder_.dispose();
lookedUpAtBuilder_ = null;
}
subjectObjectId_ = "";
excludedSubjectIds_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000004);
permissionship_ = 0;
partialCaveatInfo_ = null;
if (partialCaveatInfoBuilder_ != null) {
partialCaveatInfoBuilder_.dispose();
partialCaveatInfoBuilder_ = null;
}
subject_ = null;
if (subjectBuilder_ != null) {
subjectBuilder_.dispose();
subjectBuilder_ = null;
}
if (excludedSubjectsBuilder_ == null) {
excludedSubjects_ = java.util.Collections.emptyList();
} else {
excludedSubjects_ = null;
excludedSubjectsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000040);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_LookupSubjectsResponse_descriptor;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.LookupSubjectsResponse getDefaultInstanceForType() {
return com.authzed.api.v1.PermissionService.LookupSubjectsResponse.getDefaultInstance();
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.LookupSubjectsResponse build() {
com.authzed.api.v1.PermissionService.LookupSubjectsResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.LookupSubjectsResponse buildPartial() {
com.authzed.api.v1.PermissionService.LookupSubjectsResponse result = new com.authzed.api.v1.PermissionService.LookupSubjectsResponse(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.authzed.api.v1.PermissionService.LookupSubjectsResponse result) {
if (((bitField0_ & 0x00000004) != 0)) {
excludedSubjectIds_ = excludedSubjectIds_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000004);
}
result.excludedSubjectIds_ = excludedSubjectIds_;
if (excludedSubjectsBuilder_ == null) {
if (((bitField0_ & 0x00000040) != 0)) {
excludedSubjects_ = java.util.Collections.unmodifiableList(excludedSubjects_);
bitField0_ = (bitField0_ & ~0x00000040);
}
result.excludedSubjects_ = excludedSubjects_;
} else {
result.excludedSubjects_ = excludedSubjectsBuilder_.build();
}
}
private void buildPartial0(com.authzed.api.v1.PermissionService.LookupSubjectsResponse result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.lookedUpAt_ = lookedUpAtBuilder_ == null
? lookedUpAt_
: lookedUpAtBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.subjectObjectId_ = subjectObjectId_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.permissionship_ = permissionship_;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.partialCaveatInfo_ = partialCaveatInfoBuilder_ == null
? partialCaveatInfo_
: partialCaveatInfoBuilder_.build();
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.subject_ = subjectBuilder_ == null
? subject_
: subjectBuilder_.build();
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.authzed.api.v1.PermissionService.LookupSubjectsResponse) {
return mergeFrom((com.authzed.api.v1.PermissionService.LookupSubjectsResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.authzed.api.v1.PermissionService.LookupSubjectsResponse other) {
if (other == com.authzed.api.v1.PermissionService.LookupSubjectsResponse.getDefaultInstance()) return this;
if (other.hasLookedUpAt()) {
mergeLookedUpAt(other.getLookedUpAt());
}
if (!other.getSubjectObjectId().isEmpty()) {
subjectObjectId_ = other.subjectObjectId_;
bitField0_ |= 0x00000002;
onChanged();
}
if (!other.excludedSubjectIds_.isEmpty()) {
if (excludedSubjectIds_.isEmpty()) {
excludedSubjectIds_ = other.excludedSubjectIds_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureExcludedSubjectIdsIsMutable();
excludedSubjectIds_.addAll(other.excludedSubjectIds_);
}
onChanged();
}
if (other.permissionship_ != 0) {
setPermissionshipValue(other.getPermissionshipValue());
}
if (other.hasPartialCaveatInfo()) {
mergePartialCaveatInfo(other.getPartialCaveatInfo());
}
if (other.hasSubject()) {
mergeSubject(other.getSubject());
}
if (excludedSubjectsBuilder_ == null) {
if (!other.excludedSubjects_.isEmpty()) {
if (excludedSubjects_.isEmpty()) {
excludedSubjects_ = other.excludedSubjects_;
bitField0_ = (bitField0_ & ~0x00000040);
} else {
ensureExcludedSubjectsIsMutable();
excludedSubjects_.addAll(other.excludedSubjects_);
}
onChanged();
}
} else {
if (!other.excludedSubjects_.isEmpty()) {
if (excludedSubjectsBuilder_.isEmpty()) {
excludedSubjectsBuilder_.dispose();
excludedSubjectsBuilder_ = null;
excludedSubjects_ = other.excludedSubjects_;
bitField0_ = (bitField0_ & ~0x00000040);
excludedSubjectsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getExcludedSubjectsFieldBuilder() : null;
} else {
excludedSubjectsBuilder_.addAllMessages(other.excludedSubjects_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
input.readMessage(
getLookedUpAtFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
subjectObjectId_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 18
case 26: {
java.lang.String s = input.readStringRequireUtf8();
ensureExcludedSubjectIdsIsMutable();
excludedSubjectIds_.add(s);
break;
} // case 26
case 32: {
permissionship_ = input.readEnum();
bitField0_ |= 0x00000008;
break;
} // case 32
case 42: {
input.readMessage(
getPartialCaveatInfoFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000010;
break;
} // case 42
case 50: {
input.readMessage(
getSubjectFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000020;
break;
} // case 50
case 58: {
com.authzed.api.v1.PermissionService.ResolvedSubject m =
input.readMessage(
com.authzed.api.v1.PermissionService.ResolvedSubject.parser(),
extensionRegistry);
if (excludedSubjectsBuilder_ == null) {
ensureExcludedSubjectsIsMutable();
excludedSubjects_.add(m);
} else {
excludedSubjectsBuilder_.addMessage(m);
}
break;
} // case 58
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.authzed.api.v1.Core.ZedToken lookedUpAt_;
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.ZedToken, com.authzed.api.v1.Core.ZedToken.Builder, com.authzed.api.v1.Core.ZedTokenOrBuilder> lookedUpAtBuilder_;
/**
* .authzed.api.v1.ZedToken looked_up_at = 1;
* @return Whether the lookedUpAt field is set.
*/
public boolean hasLookedUpAt() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* .authzed.api.v1.ZedToken looked_up_at = 1;
* @return The lookedUpAt.
*/
public com.authzed.api.v1.Core.ZedToken getLookedUpAt() {
if (lookedUpAtBuilder_ == null) {
return lookedUpAt_ == null ? com.authzed.api.v1.Core.ZedToken.getDefaultInstance() : lookedUpAt_;
} else {
return lookedUpAtBuilder_.getMessage();
}
}
/**
* .authzed.api.v1.ZedToken looked_up_at = 1;
*/
public Builder setLookedUpAt(com.authzed.api.v1.Core.ZedToken value) {
if (lookedUpAtBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
lookedUpAt_ = value;
} else {
lookedUpAtBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .authzed.api.v1.ZedToken looked_up_at = 1;
*/
public Builder setLookedUpAt(
com.authzed.api.v1.Core.ZedToken.Builder builderForValue) {
if (lookedUpAtBuilder_ == null) {
lookedUpAt_ = builderForValue.build();
} else {
lookedUpAtBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .authzed.api.v1.ZedToken looked_up_at = 1;
*/
public Builder mergeLookedUpAt(com.authzed.api.v1.Core.ZedToken value) {
if (lookedUpAtBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0) &&
lookedUpAt_ != null &&
lookedUpAt_ != com.authzed.api.v1.Core.ZedToken.getDefaultInstance()) {
getLookedUpAtBuilder().mergeFrom(value);
} else {
lookedUpAt_ = value;
}
} else {
lookedUpAtBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .authzed.api.v1.ZedToken looked_up_at = 1;
*/
public Builder clearLookedUpAt() {
bitField0_ = (bitField0_ & ~0x00000001);
lookedUpAt_ = null;
if (lookedUpAtBuilder_ != null) {
lookedUpAtBuilder_.dispose();
lookedUpAtBuilder_ = null;
}
onChanged();
return this;
}
/**
* .authzed.api.v1.ZedToken looked_up_at = 1;
*/
public com.authzed.api.v1.Core.ZedToken.Builder getLookedUpAtBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getLookedUpAtFieldBuilder().getBuilder();
}
/**
* .authzed.api.v1.ZedToken looked_up_at = 1;
*/
public com.authzed.api.v1.Core.ZedTokenOrBuilder getLookedUpAtOrBuilder() {
if (lookedUpAtBuilder_ != null) {
return lookedUpAtBuilder_.getMessageOrBuilder();
} else {
return lookedUpAt_ == null ?
com.authzed.api.v1.Core.ZedToken.getDefaultInstance() : lookedUpAt_;
}
}
/**
* .authzed.api.v1.ZedToken looked_up_at = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.ZedToken, com.authzed.api.v1.Core.ZedToken.Builder, com.authzed.api.v1.Core.ZedTokenOrBuilder>
getLookedUpAtFieldBuilder() {
if (lookedUpAtBuilder_ == null) {
lookedUpAtBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.ZedToken, com.authzed.api.v1.Core.ZedToken.Builder, com.authzed.api.v1.Core.ZedTokenOrBuilder>(
getLookedUpAt(),
getParentForChildren(),
isClean());
lookedUpAt_ = null;
}
return lookedUpAtBuilder_;
}
private java.lang.Object subjectObjectId_ = "";
/**
*
* subject_object_id is the Object ID of the subject found. May be a `*` if
* a wildcard was found.
* deprecated: use `subject`
*
*
* string subject_object_id = 2 [deprecated = true];
* @deprecated authzed.api.v1.LookupSubjectsResponse.subject_object_id is deprecated.
* See authzed/api/v1/permission_service.proto;l=401
* @return The subjectObjectId.
*/
@java.lang.Deprecated public java.lang.String getSubjectObjectId() {
java.lang.Object ref = subjectObjectId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
subjectObjectId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* subject_object_id is the Object ID of the subject found. May be a `*` if
* a wildcard was found.
* deprecated: use `subject`
*
*
* string subject_object_id = 2 [deprecated = true];
* @deprecated authzed.api.v1.LookupSubjectsResponse.subject_object_id is deprecated.
* See authzed/api/v1/permission_service.proto;l=401
* @return The bytes for subjectObjectId.
*/
@java.lang.Deprecated public com.google.protobuf.ByteString
getSubjectObjectIdBytes() {
java.lang.Object ref = subjectObjectId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
subjectObjectId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* subject_object_id is the Object ID of the subject found. May be a `*` if
* a wildcard was found.
* deprecated: use `subject`
*
*
* string subject_object_id = 2 [deprecated = true];
* @deprecated authzed.api.v1.LookupSubjectsResponse.subject_object_id is deprecated.
* See authzed/api/v1/permission_service.proto;l=401
* @param value The subjectObjectId to set.
* @return This builder for chaining.
*/
@java.lang.Deprecated public Builder setSubjectObjectId(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
subjectObjectId_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* subject_object_id is the Object ID of the subject found. May be a `*` if
* a wildcard was found.
* deprecated: use `subject`
*
*
* string subject_object_id = 2 [deprecated = true];
* @deprecated authzed.api.v1.LookupSubjectsResponse.subject_object_id is deprecated.
* See authzed/api/v1/permission_service.proto;l=401
* @return This builder for chaining.
*/
@java.lang.Deprecated public Builder clearSubjectObjectId() {
subjectObjectId_ = getDefaultInstance().getSubjectObjectId();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
* subject_object_id is the Object ID of the subject found. May be a `*` if
* a wildcard was found.
* deprecated: use `subject`
*
*
* string subject_object_id = 2 [deprecated = true];
* @deprecated authzed.api.v1.LookupSubjectsResponse.subject_object_id is deprecated.
* See authzed/api/v1/permission_service.proto;l=401
* @param value The bytes for subjectObjectId to set.
* @return This builder for chaining.
*/
@java.lang.Deprecated public Builder setSubjectObjectIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
subjectObjectId_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList excludedSubjectIds_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureExcludedSubjectIdsIsMutable() {
if (!((bitField0_ & 0x00000004) != 0)) {
excludedSubjectIds_ = new com.google.protobuf.LazyStringArrayList(excludedSubjectIds_);
bitField0_ |= 0x00000004;
}
}
/**
*
* excluded_subject_ids are the Object IDs of the subjects excluded. This list
* will only contain object IDs if `subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
* deprecated: use `excluded_subjects`
*
*
* repeated string excluded_subject_ids = 3 [deprecated = true];
* @deprecated authzed.api.v1.LookupSubjectsResponse.excluded_subject_ids is deprecated.
* See authzed/api/v1/permission_service.proto;l=407
* @return A list containing the excludedSubjectIds.
*/
@java.lang.Deprecated public com.google.protobuf.ProtocolStringList
getExcludedSubjectIdsList() {
return excludedSubjectIds_.getUnmodifiableView();
}
/**
*
* excluded_subject_ids are the Object IDs of the subjects excluded. This list
* will only contain object IDs if `subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
* deprecated: use `excluded_subjects`
*
*
* repeated string excluded_subject_ids = 3 [deprecated = true];
* @deprecated authzed.api.v1.LookupSubjectsResponse.excluded_subject_ids is deprecated.
* See authzed/api/v1/permission_service.proto;l=407
* @return The count of excludedSubjectIds.
*/
@java.lang.Deprecated public int getExcludedSubjectIdsCount() {
return excludedSubjectIds_.size();
}
/**
*
* excluded_subject_ids are the Object IDs of the subjects excluded. This list
* will only contain object IDs if `subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
* deprecated: use `excluded_subjects`
*
*
* repeated string excluded_subject_ids = 3 [deprecated = true];
* @deprecated authzed.api.v1.LookupSubjectsResponse.excluded_subject_ids is deprecated.
* See authzed/api/v1/permission_service.proto;l=407
* @param index The index of the element to return.
* @return The excludedSubjectIds at the given index.
*/
@java.lang.Deprecated public java.lang.String getExcludedSubjectIds(int index) {
return excludedSubjectIds_.get(index);
}
/**
*
* excluded_subject_ids are the Object IDs of the subjects excluded. This list
* will only contain object IDs if `subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
* deprecated: use `excluded_subjects`
*
*
* repeated string excluded_subject_ids = 3 [deprecated = true];
* @deprecated authzed.api.v1.LookupSubjectsResponse.excluded_subject_ids is deprecated.
* See authzed/api/v1/permission_service.proto;l=407
* @param index The index of the value to return.
* @return The bytes of the excludedSubjectIds at the given index.
*/
@java.lang.Deprecated public com.google.protobuf.ByteString
getExcludedSubjectIdsBytes(int index) {
return excludedSubjectIds_.getByteString(index);
}
/**
*
* excluded_subject_ids are the Object IDs of the subjects excluded. This list
* will only contain object IDs if `subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
* deprecated: use `excluded_subjects`
*
*
* repeated string excluded_subject_ids = 3 [deprecated = true];
* @deprecated authzed.api.v1.LookupSubjectsResponse.excluded_subject_ids is deprecated.
* See authzed/api/v1/permission_service.proto;l=407
* @param index The index to set the value at.
* @param value The excludedSubjectIds to set.
* @return This builder for chaining.
*/
@java.lang.Deprecated public Builder setExcludedSubjectIds(
int index, java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureExcludedSubjectIdsIsMutable();
excludedSubjectIds_.set(index, value);
onChanged();
return this;
}
/**
*
* excluded_subject_ids are the Object IDs of the subjects excluded. This list
* will only contain object IDs if `subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
* deprecated: use `excluded_subjects`
*
*
* repeated string excluded_subject_ids = 3 [deprecated = true];
* @deprecated authzed.api.v1.LookupSubjectsResponse.excluded_subject_ids is deprecated.
* See authzed/api/v1/permission_service.proto;l=407
* @param value The excludedSubjectIds to add.
* @return This builder for chaining.
*/
@java.lang.Deprecated public Builder addExcludedSubjectIds(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureExcludedSubjectIdsIsMutable();
excludedSubjectIds_.add(value);
onChanged();
return this;
}
/**
*
* excluded_subject_ids are the Object IDs of the subjects excluded. This list
* will only contain object IDs if `subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
* deprecated: use `excluded_subjects`
*
*
* repeated string excluded_subject_ids = 3 [deprecated = true];
* @deprecated authzed.api.v1.LookupSubjectsResponse.excluded_subject_ids is deprecated.
* See authzed/api/v1/permission_service.proto;l=407
* @param values The excludedSubjectIds to add.
* @return This builder for chaining.
*/
@java.lang.Deprecated public Builder addAllExcludedSubjectIds(
java.lang.Iterable values) {
ensureExcludedSubjectIdsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, excludedSubjectIds_);
onChanged();
return this;
}
/**
*
* excluded_subject_ids are the Object IDs of the subjects excluded. This list
* will only contain object IDs if `subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
* deprecated: use `excluded_subjects`
*
*
* repeated string excluded_subject_ids = 3 [deprecated = true];
* @deprecated authzed.api.v1.LookupSubjectsResponse.excluded_subject_ids is deprecated.
* See authzed/api/v1/permission_service.proto;l=407
* @return This builder for chaining.
*/
@java.lang.Deprecated public Builder clearExcludedSubjectIds() {
excludedSubjectIds_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
*
* excluded_subject_ids are the Object IDs of the subjects excluded. This list
* will only contain object IDs if `subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
* deprecated: use `excluded_subjects`
*
*
* repeated string excluded_subject_ids = 3 [deprecated = true];
* @deprecated authzed.api.v1.LookupSubjectsResponse.excluded_subject_ids is deprecated.
* See authzed/api/v1/permission_service.proto;l=407
* @param value The bytes of the excludedSubjectIds to add.
* @return This builder for chaining.
*/
@java.lang.Deprecated public Builder addExcludedSubjectIdsBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
ensureExcludedSubjectIdsIsMutable();
excludedSubjectIds_.add(value);
onChanged();
return this;
}
private int permissionship_ = 0;
/**
*
* permissionship indicates whether the response was partially evaluated or not
* deprecated: use `subject.permissionship`
*
*
* .authzed.api.v1.LookupPermissionship permissionship = 4 [deprecated = true, (.validate.rules) = { ... }
* @deprecated authzed.api.v1.LookupSubjectsResponse.permissionship is deprecated.
* See authzed/api/v1/permission_service.proto;l=411
* @return The enum numeric value on the wire for permissionship.
*/
@java.lang.Override @java.lang.Deprecated public int getPermissionshipValue() {
return permissionship_;
}
/**
*
* permissionship indicates whether the response was partially evaluated or not
* deprecated: use `subject.permissionship`
*
*
* .authzed.api.v1.LookupPermissionship permissionship = 4 [deprecated = true, (.validate.rules) = { ... }
* @deprecated authzed.api.v1.LookupSubjectsResponse.permissionship is deprecated.
* See authzed/api/v1/permission_service.proto;l=411
* @param value The enum numeric value on the wire for permissionship to set.
* @return This builder for chaining.
*/
@java.lang.Deprecated public Builder setPermissionshipValue(int value) {
permissionship_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* permissionship indicates whether the response was partially evaluated or not
* deprecated: use `subject.permissionship`
*
*
* .authzed.api.v1.LookupPermissionship permissionship = 4 [deprecated = true, (.validate.rules) = { ... }
* @deprecated authzed.api.v1.LookupSubjectsResponse.permissionship is deprecated.
* See authzed/api/v1/permission_service.proto;l=411
* @return The permissionship.
*/
@java.lang.Override
@java.lang.Deprecated public com.authzed.api.v1.PermissionService.LookupPermissionship getPermissionship() {
com.authzed.api.v1.PermissionService.LookupPermissionship result = com.authzed.api.v1.PermissionService.LookupPermissionship.forNumber(permissionship_);
return result == null ? com.authzed.api.v1.PermissionService.LookupPermissionship.UNRECOGNIZED : result;
}
/**
*
* permissionship indicates whether the response was partially evaluated or not
* deprecated: use `subject.permissionship`
*
*
* .authzed.api.v1.LookupPermissionship permissionship = 4 [deprecated = true, (.validate.rules) = { ... }
* @deprecated authzed.api.v1.LookupSubjectsResponse.permissionship is deprecated.
* See authzed/api/v1/permission_service.proto;l=411
* @param value The permissionship to set.
* @return This builder for chaining.
*/
@java.lang.Deprecated public Builder setPermissionship(com.authzed.api.v1.PermissionService.LookupPermissionship value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
permissionship_ = value.getNumber();
onChanged();
return this;
}
/**
*
* permissionship indicates whether the response was partially evaluated or not
* deprecated: use `subject.permissionship`
*
*
* .authzed.api.v1.LookupPermissionship permissionship = 4 [deprecated = true, (.validate.rules) = { ... }
* @deprecated authzed.api.v1.LookupSubjectsResponse.permissionship is deprecated.
* See authzed/api/v1/permission_service.proto;l=411
* @return This builder for chaining.
*/
@java.lang.Deprecated public Builder clearPermissionship() {
bitField0_ = (bitField0_ & ~0x00000008);
permissionship_ = 0;
onChanged();
return this;
}
private com.authzed.api.v1.Core.PartialCaveatInfo partialCaveatInfo_;
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.PartialCaveatInfo, com.authzed.api.v1.Core.PartialCaveatInfo.Builder, com.authzed.api.v1.Core.PartialCaveatInfoOrBuilder> partialCaveatInfoBuilder_;
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
* deprecated: use `subject.partial_caveat_info`
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 5 [deprecated = true, (.validate.rules) = { ... }
* @deprecated authzed.api.v1.LookupSubjectsResponse.partial_caveat_info is deprecated.
* See authzed/api/v1/permission_service.proto;l=415
* @return Whether the partialCaveatInfo field is set.
*/
@java.lang.Deprecated public boolean hasPartialCaveatInfo() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
* deprecated: use `subject.partial_caveat_info`
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 5 [deprecated = true, (.validate.rules) = { ... }
* @deprecated authzed.api.v1.LookupSubjectsResponse.partial_caveat_info is deprecated.
* See authzed/api/v1/permission_service.proto;l=415
* @return The partialCaveatInfo.
*/
@java.lang.Deprecated public com.authzed.api.v1.Core.PartialCaveatInfo getPartialCaveatInfo() {
if (partialCaveatInfoBuilder_ == null) {
return partialCaveatInfo_ == null ? com.authzed.api.v1.Core.PartialCaveatInfo.getDefaultInstance() : partialCaveatInfo_;
} else {
return partialCaveatInfoBuilder_.getMessage();
}
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
* deprecated: use `subject.partial_caveat_info`
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 5 [deprecated = true, (.validate.rules) = { ... }
*/
@java.lang.Deprecated public Builder setPartialCaveatInfo(com.authzed.api.v1.Core.PartialCaveatInfo value) {
if (partialCaveatInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
partialCaveatInfo_ = value;
} else {
partialCaveatInfoBuilder_.setMessage(value);
}
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
* deprecated: use `subject.partial_caveat_info`
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 5 [deprecated = true, (.validate.rules) = { ... }
*/
@java.lang.Deprecated public Builder setPartialCaveatInfo(
com.authzed.api.v1.Core.PartialCaveatInfo.Builder builderForValue) {
if (partialCaveatInfoBuilder_ == null) {
partialCaveatInfo_ = builderForValue.build();
} else {
partialCaveatInfoBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
* deprecated: use `subject.partial_caveat_info`
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 5 [deprecated = true, (.validate.rules) = { ... }
*/
@java.lang.Deprecated public Builder mergePartialCaveatInfo(com.authzed.api.v1.Core.PartialCaveatInfo value) {
if (partialCaveatInfoBuilder_ == null) {
if (((bitField0_ & 0x00000010) != 0) &&
partialCaveatInfo_ != null &&
partialCaveatInfo_ != com.authzed.api.v1.Core.PartialCaveatInfo.getDefaultInstance()) {
getPartialCaveatInfoBuilder().mergeFrom(value);
} else {
partialCaveatInfo_ = value;
}
} else {
partialCaveatInfoBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
* deprecated: use `subject.partial_caveat_info`
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 5 [deprecated = true, (.validate.rules) = { ... }
*/
@java.lang.Deprecated public Builder clearPartialCaveatInfo() {
bitField0_ = (bitField0_ & ~0x00000010);
partialCaveatInfo_ = null;
if (partialCaveatInfoBuilder_ != null) {
partialCaveatInfoBuilder_.dispose();
partialCaveatInfoBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
* deprecated: use `subject.partial_caveat_info`
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 5 [deprecated = true, (.validate.rules) = { ... }
*/
@java.lang.Deprecated public com.authzed.api.v1.Core.PartialCaveatInfo.Builder getPartialCaveatInfoBuilder() {
bitField0_ |= 0x00000010;
onChanged();
return getPartialCaveatInfoFieldBuilder().getBuilder();
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
* deprecated: use `subject.partial_caveat_info`
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 5 [deprecated = true, (.validate.rules) = { ... }
*/
@java.lang.Deprecated public com.authzed.api.v1.Core.PartialCaveatInfoOrBuilder getPartialCaveatInfoOrBuilder() {
if (partialCaveatInfoBuilder_ != null) {
return partialCaveatInfoBuilder_.getMessageOrBuilder();
} else {
return partialCaveatInfo_ == null ?
com.authzed.api.v1.Core.PartialCaveatInfo.getDefaultInstance() : partialCaveatInfo_;
}
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
* deprecated: use `subject.partial_caveat_info`
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 5 [deprecated = true, (.validate.rules) = { ... }
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.PartialCaveatInfo, com.authzed.api.v1.Core.PartialCaveatInfo.Builder, com.authzed.api.v1.Core.PartialCaveatInfoOrBuilder>
getPartialCaveatInfoFieldBuilder() {
if (partialCaveatInfoBuilder_ == null) {
partialCaveatInfoBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.PartialCaveatInfo, com.authzed.api.v1.Core.PartialCaveatInfo.Builder, com.authzed.api.v1.Core.PartialCaveatInfoOrBuilder>(
getPartialCaveatInfo(),
getParentForChildren(),
isClean());
partialCaveatInfo_ = null;
}
return partialCaveatInfoBuilder_;
}
private com.authzed.api.v1.PermissionService.ResolvedSubject subject_;
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.PermissionService.ResolvedSubject, com.authzed.api.v1.PermissionService.ResolvedSubject.Builder, com.authzed.api.v1.PermissionService.ResolvedSubjectOrBuilder> subjectBuilder_;
/**
*
* subject is the subject found, along with its permissionship.
*
*
* .authzed.api.v1.ResolvedSubject subject = 6;
* @return Whether the subject field is set.
*/
public boolean hasSubject() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* subject is the subject found, along with its permissionship.
*
*
* .authzed.api.v1.ResolvedSubject subject = 6;
* @return The subject.
*/
public com.authzed.api.v1.PermissionService.ResolvedSubject getSubject() {
if (subjectBuilder_ == null) {
return subject_ == null ? com.authzed.api.v1.PermissionService.ResolvedSubject.getDefaultInstance() : subject_;
} else {
return subjectBuilder_.getMessage();
}
}
/**
*
* subject is the subject found, along with its permissionship.
*
*
* .authzed.api.v1.ResolvedSubject subject = 6;
*/
public Builder setSubject(com.authzed.api.v1.PermissionService.ResolvedSubject value) {
if (subjectBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
subject_ = value;
} else {
subjectBuilder_.setMessage(value);
}
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
* subject is the subject found, along with its permissionship.
*
*
* .authzed.api.v1.ResolvedSubject subject = 6;
*/
public Builder setSubject(
com.authzed.api.v1.PermissionService.ResolvedSubject.Builder builderForValue) {
if (subjectBuilder_ == null) {
subject_ = builderForValue.build();
} else {
subjectBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
* subject is the subject found, along with its permissionship.
*
*
* .authzed.api.v1.ResolvedSubject subject = 6;
*/
public Builder mergeSubject(com.authzed.api.v1.PermissionService.ResolvedSubject value) {
if (subjectBuilder_ == null) {
if (((bitField0_ & 0x00000020) != 0) &&
subject_ != null &&
subject_ != com.authzed.api.v1.PermissionService.ResolvedSubject.getDefaultInstance()) {
getSubjectBuilder().mergeFrom(value);
} else {
subject_ = value;
}
} else {
subjectBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
* subject is the subject found, along with its permissionship.
*
*
* .authzed.api.v1.ResolvedSubject subject = 6;
*/
public Builder clearSubject() {
bitField0_ = (bitField0_ & ~0x00000020);
subject_ = null;
if (subjectBuilder_ != null) {
subjectBuilder_.dispose();
subjectBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* subject is the subject found, along with its permissionship.
*
*
* .authzed.api.v1.ResolvedSubject subject = 6;
*/
public com.authzed.api.v1.PermissionService.ResolvedSubject.Builder getSubjectBuilder() {
bitField0_ |= 0x00000020;
onChanged();
return getSubjectFieldBuilder().getBuilder();
}
/**
*
* subject is the subject found, along with its permissionship.
*
*
* .authzed.api.v1.ResolvedSubject subject = 6;
*/
public com.authzed.api.v1.PermissionService.ResolvedSubjectOrBuilder getSubjectOrBuilder() {
if (subjectBuilder_ != null) {
return subjectBuilder_.getMessageOrBuilder();
} else {
return subject_ == null ?
com.authzed.api.v1.PermissionService.ResolvedSubject.getDefaultInstance() : subject_;
}
}
/**
*
* subject is the subject found, along with its permissionship.
*
*
* .authzed.api.v1.ResolvedSubject subject = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.PermissionService.ResolvedSubject, com.authzed.api.v1.PermissionService.ResolvedSubject.Builder, com.authzed.api.v1.PermissionService.ResolvedSubjectOrBuilder>
getSubjectFieldBuilder() {
if (subjectBuilder_ == null) {
subjectBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.PermissionService.ResolvedSubject, com.authzed.api.v1.PermissionService.ResolvedSubject.Builder, com.authzed.api.v1.PermissionService.ResolvedSubjectOrBuilder>(
getSubject(),
getParentForChildren(),
isClean());
subject_ = null;
}
return subjectBuilder_;
}
private java.util.List excludedSubjects_ =
java.util.Collections.emptyList();
private void ensureExcludedSubjectsIsMutable() {
if (!((bitField0_ & 0x00000040) != 0)) {
excludedSubjects_ = new java.util.ArrayList(excludedSubjects_);
bitField0_ |= 0x00000040;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.authzed.api.v1.PermissionService.ResolvedSubject, com.authzed.api.v1.PermissionService.ResolvedSubject.Builder, com.authzed.api.v1.PermissionService.ResolvedSubjectOrBuilder> excludedSubjectsBuilder_;
/**
*
* excluded_subjects are the subjects excluded. This list
* will only contain subjects if `subject.subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
*
*
* repeated .authzed.api.v1.ResolvedSubject excluded_subjects = 7;
*/
public java.util.List getExcludedSubjectsList() {
if (excludedSubjectsBuilder_ == null) {
return java.util.Collections.unmodifiableList(excludedSubjects_);
} else {
return excludedSubjectsBuilder_.getMessageList();
}
}
/**
*
* excluded_subjects are the subjects excluded. This list
* will only contain subjects if `subject.subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
*
*
* repeated .authzed.api.v1.ResolvedSubject excluded_subjects = 7;
*/
public int getExcludedSubjectsCount() {
if (excludedSubjectsBuilder_ == null) {
return excludedSubjects_.size();
} else {
return excludedSubjectsBuilder_.getCount();
}
}
/**
*
* excluded_subjects are the subjects excluded. This list
* will only contain subjects if `subject.subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
*
*
* repeated .authzed.api.v1.ResolvedSubject excluded_subjects = 7;
*/
public com.authzed.api.v1.PermissionService.ResolvedSubject getExcludedSubjects(int index) {
if (excludedSubjectsBuilder_ == null) {
return excludedSubjects_.get(index);
} else {
return excludedSubjectsBuilder_.getMessage(index);
}
}
/**
*
* excluded_subjects are the subjects excluded. This list
* will only contain subjects if `subject.subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
*
*
* repeated .authzed.api.v1.ResolvedSubject excluded_subjects = 7;
*/
public Builder setExcludedSubjects(
int index, com.authzed.api.v1.PermissionService.ResolvedSubject value) {
if (excludedSubjectsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureExcludedSubjectsIsMutable();
excludedSubjects_.set(index, value);
onChanged();
} else {
excludedSubjectsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* excluded_subjects are the subjects excluded. This list
* will only contain subjects if `subject.subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
*
*
* repeated .authzed.api.v1.ResolvedSubject excluded_subjects = 7;
*/
public Builder setExcludedSubjects(
int index, com.authzed.api.v1.PermissionService.ResolvedSubject.Builder builderForValue) {
if (excludedSubjectsBuilder_ == null) {
ensureExcludedSubjectsIsMutable();
excludedSubjects_.set(index, builderForValue.build());
onChanged();
} else {
excludedSubjectsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* excluded_subjects are the subjects excluded. This list
* will only contain subjects if `subject.subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
*
*
* repeated .authzed.api.v1.ResolvedSubject excluded_subjects = 7;
*/
public Builder addExcludedSubjects(com.authzed.api.v1.PermissionService.ResolvedSubject value) {
if (excludedSubjectsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureExcludedSubjectsIsMutable();
excludedSubjects_.add(value);
onChanged();
} else {
excludedSubjectsBuilder_.addMessage(value);
}
return this;
}
/**
*
* excluded_subjects are the subjects excluded. This list
* will only contain subjects if `subject.subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
*
*
* repeated .authzed.api.v1.ResolvedSubject excluded_subjects = 7;
*/
public Builder addExcludedSubjects(
int index, com.authzed.api.v1.PermissionService.ResolvedSubject value) {
if (excludedSubjectsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureExcludedSubjectsIsMutable();
excludedSubjects_.add(index, value);
onChanged();
} else {
excludedSubjectsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* excluded_subjects are the subjects excluded. This list
* will only contain subjects if `subject.subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
*
*
* repeated .authzed.api.v1.ResolvedSubject excluded_subjects = 7;
*/
public Builder addExcludedSubjects(
com.authzed.api.v1.PermissionService.ResolvedSubject.Builder builderForValue) {
if (excludedSubjectsBuilder_ == null) {
ensureExcludedSubjectsIsMutable();
excludedSubjects_.add(builderForValue.build());
onChanged();
} else {
excludedSubjectsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* excluded_subjects are the subjects excluded. This list
* will only contain subjects if `subject.subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
*
*
* repeated .authzed.api.v1.ResolvedSubject excluded_subjects = 7;
*/
public Builder addExcludedSubjects(
int index, com.authzed.api.v1.PermissionService.ResolvedSubject.Builder builderForValue) {
if (excludedSubjectsBuilder_ == null) {
ensureExcludedSubjectsIsMutable();
excludedSubjects_.add(index, builderForValue.build());
onChanged();
} else {
excludedSubjectsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* excluded_subjects are the subjects excluded. This list
* will only contain subjects if `subject.subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
*
*
* repeated .authzed.api.v1.ResolvedSubject excluded_subjects = 7;
*/
public Builder addAllExcludedSubjects(
java.lang.Iterable extends com.authzed.api.v1.PermissionService.ResolvedSubject> values) {
if (excludedSubjectsBuilder_ == null) {
ensureExcludedSubjectsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, excludedSubjects_);
onChanged();
} else {
excludedSubjectsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* excluded_subjects are the subjects excluded. This list
* will only contain subjects if `subject.subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
*
*
* repeated .authzed.api.v1.ResolvedSubject excluded_subjects = 7;
*/
public Builder clearExcludedSubjects() {
if (excludedSubjectsBuilder_ == null) {
excludedSubjects_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
} else {
excludedSubjectsBuilder_.clear();
}
return this;
}
/**
*
* excluded_subjects are the subjects excluded. This list
* will only contain subjects if `subject.subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
*
*
* repeated .authzed.api.v1.ResolvedSubject excluded_subjects = 7;
*/
public Builder removeExcludedSubjects(int index) {
if (excludedSubjectsBuilder_ == null) {
ensureExcludedSubjectsIsMutable();
excludedSubjects_.remove(index);
onChanged();
} else {
excludedSubjectsBuilder_.remove(index);
}
return this;
}
/**
*
* excluded_subjects are the subjects excluded. This list
* will only contain subjects if `subject.subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
*
*
* repeated .authzed.api.v1.ResolvedSubject excluded_subjects = 7;
*/
public com.authzed.api.v1.PermissionService.ResolvedSubject.Builder getExcludedSubjectsBuilder(
int index) {
return getExcludedSubjectsFieldBuilder().getBuilder(index);
}
/**
*
* excluded_subjects are the subjects excluded. This list
* will only contain subjects if `subject.subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
*
*
* repeated .authzed.api.v1.ResolvedSubject excluded_subjects = 7;
*/
public com.authzed.api.v1.PermissionService.ResolvedSubjectOrBuilder getExcludedSubjectsOrBuilder(
int index) {
if (excludedSubjectsBuilder_ == null) {
return excludedSubjects_.get(index); } else {
return excludedSubjectsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* excluded_subjects are the subjects excluded. This list
* will only contain subjects if `subject.subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
*
*
* repeated .authzed.api.v1.ResolvedSubject excluded_subjects = 7;
*/
public java.util.List extends com.authzed.api.v1.PermissionService.ResolvedSubjectOrBuilder>
getExcludedSubjectsOrBuilderList() {
if (excludedSubjectsBuilder_ != null) {
return excludedSubjectsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(excludedSubjects_);
}
}
/**
*
* excluded_subjects are the subjects excluded. This list
* will only contain subjects if `subject.subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
*
*
* repeated .authzed.api.v1.ResolvedSubject excluded_subjects = 7;
*/
public com.authzed.api.v1.PermissionService.ResolvedSubject.Builder addExcludedSubjectsBuilder() {
return getExcludedSubjectsFieldBuilder().addBuilder(
com.authzed.api.v1.PermissionService.ResolvedSubject.getDefaultInstance());
}
/**
*
* excluded_subjects are the subjects excluded. This list
* will only contain subjects if `subject.subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
*
*
* repeated .authzed.api.v1.ResolvedSubject excluded_subjects = 7;
*/
public com.authzed.api.v1.PermissionService.ResolvedSubject.Builder addExcludedSubjectsBuilder(
int index) {
return getExcludedSubjectsFieldBuilder().addBuilder(
index, com.authzed.api.v1.PermissionService.ResolvedSubject.getDefaultInstance());
}
/**
*
* excluded_subjects are the subjects excluded. This list
* will only contain subjects if `subject.subject_object_id` is a wildcard (`*`) and
* will only be populated if exclusions exist from the wildcard.
*
*
* repeated .authzed.api.v1.ResolvedSubject excluded_subjects = 7;
*/
public java.util.List
getExcludedSubjectsBuilderList() {
return getExcludedSubjectsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.authzed.api.v1.PermissionService.ResolvedSubject, com.authzed.api.v1.PermissionService.ResolvedSubject.Builder, com.authzed.api.v1.PermissionService.ResolvedSubjectOrBuilder>
getExcludedSubjectsFieldBuilder() {
if (excludedSubjectsBuilder_ == null) {
excludedSubjectsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.authzed.api.v1.PermissionService.ResolvedSubject, com.authzed.api.v1.PermissionService.ResolvedSubject.Builder, com.authzed.api.v1.PermissionService.ResolvedSubjectOrBuilder>(
excludedSubjects_,
((bitField0_ & 0x00000040) != 0),
getParentForChildren(),
isClean());
excludedSubjects_ = null;
}
return excludedSubjectsBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:authzed.api.v1.LookupSubjectsResponse)
}
// @@protoc_insertion_point(class_scope:authzed.api.v1.LookupSubjectsResponse)
private static final com.authzed.api.v1.PermissionService.LookupSubjectsResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.authzed.api.v1.PermissionService.LookupSubjectsResponse();
}
public static com.authzed.api.v1.PermissionService.LookupSubjectsResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public LookupSubjectsResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.LookupSubjectsResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ResolvedSubjectOrBuilder extends
// @@protoc_insertion_point(interface_extends:authzed.api.v1.ResolvedSubject)
com.google.protobuf.MessageOrBuilder {
/**
*
* subject_object_id is the Object ID of the subject found. May be a `*` if
* a wildcard was found.
*
*
* string subject_object_id = 1;
* @return The subjectObjectId.
*/
java.lang.String getSubjectObjectId();
/**
*
* subject_object_id is the Object ID of the subject found. May be a `*` if
* a wildcard was found.
*
*
* string subject_object_id = 1;
* @return The bytes for subjectObjectId.
*/
com.google.protobuf.ByteString
getSubjectObjectIdBytes();
/**
*
* permissionship indicates whether the response was partially evaluated or not
*
*
* .authzed.api.v1.LookupPermissionship permissionship = 2 [(.validate.rules) = { ... }
* @return The enum numeric value on the wire for permissionship.
*/
int getPermissionshipValue();
/**
*
* permissionship indicates whether the response was partially evaluated or not
*
*
* .authzed.api.v1.LookupPermissionship permissionship = 2 [(.validate.rules) = { ... }
* @return The permissionship.
*/
com.authzed.api.v1.PermissionService.LookupPermissionship getPermissionship();
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 3 [(.validate.rules) = { ... }
* @return Whether the partialCaveatInfo field is set.
*/
boolean hasPartialCaveatInfo();
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 3 [(.validate.rules) = { ... }
* @return The partialCaveatInfo.
*/
com.authzed.api.v1.Core.PartialCaveatInfo getPartialCaveatInfo();
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 3 [(.validate.rules) = { ... }
*/
com.authzed.api.v1.Core.PartialCaveatInfoOrBuilder getPartialCaveatInfoOrBuilder();
}
/**
*
* ResolvedSubject is a single subject resolved within LookupSubjects.
*
*
* Protobuf type {@code authzed.api.v1.ResolvedSubject}
*/
public static final class ResolvedSubject extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:authzed.api.v1.ResolvedSubject)
ResolvedSubjectOrBuilder {
private static final long serialVersionUID = 0L;
// Use ResolvedSubject.newBuilder() to construct.
private ResolvedSubject(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ResolvedSubject() {
subjectObjectId_ = "";
permissionship_ = 0;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ResolvedSubject();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_ResolvedSubject_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_ResolvedSubject_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.PermissionService.ResolvedSubject.class, com.authzed.api.v1.PermissionService.ResolvedSubject.Builder.class);
}
public static final int SUBJECT_OBJECT_ID_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object subjectObjectId_ = "";
/**
*
* subject_object_id is the Object ID of the subject found. May be a `*` if
* a wildcard was found.
*
*
* string subject_object_id = 1;
* @return The subjectObjectId.
*/
@java.lang.Override
public java.lang.String getSubjectObjectId() {
java.lang.Object ref = subjectObjectId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
subjectObjectId_ = s;
return s;
}
}
/**
*
* subject_object_id is the Object ID of the subject found. May be a `*` if
* a wildcard was found.
*
*
* string subject_object_id = 1;
* @return The bytes for subjectObjectId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getSubjectObjectIdBytes() {
java.lang.Object ref = subjectObjectId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
subjectObjectId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PERMISSIONSHIP_FIELD_NUMBER = 2;
private int permissionship_ = 0;
/**
*
* permissionship indicates whether the response was partially evaluated or not
*
*
* .authzed.api.v1.LookupPermissionship permissionship = 2 [(.validate.rules) = { ... }
* @return The enum numeric value on the wire for permissionship.
*/
@java.lang.Override public int getPermissionshipValue() {
return permissionship_;
}
/**
*
* permissionship indicates whether the response was partially evaluated or not
*
*
* .authzed.api.v1.LookupPermissionship permissionship = 2 [(.validate.rules) = { ... }
* @return The permissionship.
*/
@java.lang.Override public com.authzed.api.v1.PermissionService.LookupPermissionship getPermissionship() {
com.authzed.api.v1.PermissionService.LookupPermissionship result = com.authzed.api.v1.PermissionService.LookupPermissionship.forNumber(permissionship_);
return result == null ? com.authzed.api.v1.PermissionService.LookupPermissionship.UNRECOGNIZED : result;
}
public static final int PARTIAL_CAVEAT_INFO_FIELD_NUMBER = 3;
private com.authzed.api.v1.Core.PartialCaveatInfo partialCaveatInfo_;
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 3 [(.validate.rules) = { ... }
* @return Whether the partialCaveatInfo field is set.
*/
@java.lang.Override
public boolean hasPartialCaveatInfo() {
return partialCaveatInfo_ != null;
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 3 [(.validate.rules) = { ... }
* @return The partialCaveatInfo.
*/
@java.lang.Override
public com.authzed.api.v1.Core.PartialCaveatInfo getPartialCaveatInfo() {
return partialCaveatInfo_ == null ? com.authzed.api.v1.Core.PartialCaveatInfo.getDefaultInstance() : partialCaveatInfo_;
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 3 [(.validate.rules) = { ... }
*/
@java.lang.Override
public com.authzed.api.v1.Core.PartialCaveatInfoOrBuilder getPartialCaveatInfoOrBuilder() {
return partialCaveatInfo_ == null ? com.authzed.api.v1.Core.PartialCaveatInfo.getDefaultInstance() : partialCaveatInfo_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(subjectObjectId_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, subjectObjectId_);
}
if (permissionship_ != com.authzed.api.v1.PermissionService.LookupPermissionship.LOOKUP_PERMISSIONSHIP_UNSPECIFIED.getNumber()) {
output.writeEnum(2, permissionship_);
}
if (partialCaveatInfo_ != null) {
output.writeMessage(3, getPartialCaveatInfo());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(subjectObjectId_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, subjectObjectId_);
}
if (permissionship_ != com.authzed.api.v1.PermissionService.LookupPermissionship.LOOKUP_PERMISSIONSHIP_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, permissionship_);
}
if (partialCaveatInfo_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getPartialCaveatInfo());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.authzed.api.v1.PermissionService.ResolvedSubject)) {
return super.equals(obj);
}
com.authzed.api.v1.PermissionService.ResolvedSubject other = (com.authzed.api.v1.PermissionService.ResolvedSubject) obj;
if (!getSubjectObjectId()
.equals(other.getSubjectObjectId())) return false;
if (permissionship_ != other.permissionship_) return false;
if (hasPartialCaveatInfo() != other.hasPartialCaveatInfo()) return false;
if (hasPartialCaveatInfo()) {
if (!getPartialCaveatInfo()
.equals(other.getPartialCaveatInfo())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + SUBJECT_OBJECT_ID_FIELD_NUMBER;
hash = (53 * hash) + getSubjectObjectId().hashCode();
hash = (37 * hash) + PERMISSIONSHIP_FIELD_NUMBER;
hash = (53 * hash) + permissionship_;
if (hasPartialCaveatInfo()) {
hash = (37 * hash) + PARTIAL_CAVEAT_INFO_FIELD_NUMBER;
hash = (53 * hash) + getPartialCaveatInfo().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.authzed.api.v1.PermissionService.ResolvedSubject parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.ResolvedSubject parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.ResolvedSubject parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.ResolvedSubject parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.ResolvedSubject parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.authzed.api.v1.PermissionService.ResolvedSubject parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.ResolvedSubject parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.ResolvedSubject parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.ResolvedSubject parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.ResolvedSubject parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.authzed.api.v1.PermissionService.ResolvedSubject parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.authzed.api.v1.PermissionService.ResolvedSubject parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.authzed.api.v1.PermissionService.ResolvedSubject prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* ResolvedSubject is a single subject resolved within LookupSubjects.
*
*
* Protobuf type {@code authzed.api.v1.ResolvedSubject}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:authzed.api.v1.ResolvedSubject)
com.authzed.api.v1.PermissionService.ResolvedSubjectOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_ResolvedSubject_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_ResolvedSubject_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.authzed.api.v1.PermissionService.ResolvedSubject.class, com.authzed.api.v1.PermissionService.ResolvedSubject.Builder.class);
}
// Construct using com.authzed.api.v1.PermissionService.ResolvedSubject.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
subjectObjectId_ = "";
permissionship_ = 0;
partialCaveatInfo_ = null;
if (partialCaveatInfoBuilder_ != null) {
partialCaveatInfoBuilder_.dispose();
partialCaveatInfoBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.authzed.api.v1.PermissionService.internal_static_authzed_api_v1_ResolvedSubject_descriptor;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.ResolvedSubject getDefaultInstanceForType() {
return com.authzed.api.v1.PermissionService.ResolvedSubject.getDefaultInstance();
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.ResolvedSubject build() {
com.authzed.api.v1.PermissionService.ResolvedSubject result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.ResolvedSubject buildPartial() {
com.authzed.api.v1.PermissionService.ResolvedSubject result = new com.authzed.api.v1.PermissionService.ResolvedSubject(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.authzed.api.v1.PermissionService.ResolvedSubject result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.subjectObjectId_ = subjectObjectId_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.permissionship_ = permissionship_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.partialCaveatInfo_ = partialCaveatInfoBuilder_ == null
? partialCaveatInfo_
: partialCaveatInfoBuilder_.build();
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.authzed.api.v1.PermissionService.ResolvedSubject) {
return mergeFrom((com.authzed.api.v1.PermissionService.ResolvedSubject)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.authzed.api.v1.PermissionService.ResolvedSubject other) {
if (other == com.authzed.api.v1.PermissionService.ResolvedSubject.getDefaultInstance()) return this;
if (!other.getSubjectObjectId().isEmpty()) {
subjectObjectId_ = other.subjectObjectId_;
bitField0_ |= 0x00000001;
onChanged();
}
if (other.permissionship_ != 0) {
setPermissionshipValue(other.getPermissionshipValue());
}
if (other.hasPartialCaveatInfo()) {
mergePartialCaveatInfo(other.getPartialCaveatInfo());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
subjectObjectId_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 10
case 16: {
permissionship_ = input.readEnum();
bitField0_ |= 0x00000002;
break;
} // case 16
case 26: {
input.readMessage(
getPartialCaveatInfoFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000004;
break;
} // case 26
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object subjectObjectId_ = "";
/**
*
* subject_object_id is the Object ID of the subject found. May be a `*` if
* a wildcard was found.
*
*
* string subject_object_id = 1;
* @return The subjectObjectId.
*/
public java.lang.String getSubjectObjectId() {
java.lang.Object ref = subjectObjectId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
subjectObjectId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* subject_object_id is the Object ID of the subject found. May be a `*` if
* a wildcard was found.
*
*
* string subject_object_id = 1;
* @return The bytes for subjectObjectId.
*/
public com.google.protobuf.ByteString
getSubjectObjectIdBytes() {
java.lang.Object ref = subjectObjectId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
subjectObjectId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* subject_object_id is the Object ID of the subject found. May be a `*` if
* a wildcard was found.
*
*
* string subject_object_id = 1;
* @param value The subjectObjectId to set.
* @return This builder for chaining.
*/
public Builder setSubjectObjectId(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
subjectObjectId_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* subject_object_id is the Object ID of the subject found. May be a `*` if
* a wildcard was found.
*
*
* string subject_object_id = 1;
* @return This builder for chaining.
*/
public Builder clearSubjectObjectId() {
subjectObjectId_ = getDefaultInstance().getSubjectObjectId();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
* subject_object_id is the Object ID of the subject found. May be a `*` if
* a wildcard was found.
*
*
* string subject_object_id = 1;
* @param value The bytes for subjectObjectId to set.
* @return This builder for chaining.
*/
public Builder setSubjectObjectIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
subjectObjectId_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private int permissionship_ = 0;
/**
*
* permissionship indicates whether the response was partially evaluated or not
*
*
* .authzed.api.v1.LookupPermissionship permissionship = 2 [(.validate.rules) = { ... }
* @return The enum numeric value on the wire for permissionship.
*/
@java.lang.Override public int getPermissionshipValue() {
return permissionship_;
}
/**
*
* permissionship indicates whether the response was partially evaluated or not
*
*
* .authzed.api.v1.LookupPermissionship permissionship = 2 [(.validate.rules) = { ... }
* @param value The enum numeric value on the wire for permissionship to set.
* @return This builder for chaining.
*/
public Builder setPermissionshipValue(int value) {
permissionship_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* permissionship indicates whether the response was partially evaluated or not
*
*
* .authzed.api.v1.LookupPermissionship permissionship = 2 [(.validate.rules) = { ... }
* @return The permissionship.
*/
@java.lang.Override
public com.authzed.api.v1.PermissionService.LookupPermissionship getPermissionship() {
com.authzed.api.v1.PermissionService.LookupPermissionship result = com.authzed.api.v1.PermissionService.LookupPermissionship.forNumber(permissionship_);
return result == null ? com.authzed.api.v1.PermissionService.LookupPermissionship.UNRECOGNIZED : result;
}
/**
*
* permissionship indicates whether the response was partially evaluated or not
*
*
* .authzed.api.v1.LookupPermissionship permissionship = 2 [(.validate.rules) = { ... }
* @param value The permissionship to set.
* @return This builder for chaining.
*/
public Builder setPermissionship(com.authzed.api.v1.PermissionService.LookupPermissionship value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
permissionship_ = value.getNumber();
onChanged();
return this;
}
/**
*
* permissionship indicates whether the response was partially evaluated or not
*
*
* .authzed.api.v1.LookupPermissionship permissionship = 2 [(.validate.rules) = { ... }
* @return This builder for chaining.
*/
public Builder clearPermissionship() {
bitField0_ = (bitField0_ & ~0x00000002);
permissionship_ = 0;
onChanged();
return this;
}
private com.authzed.api.v1.Core.PartialCaveatInfo partialCaveatInfo_;
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.PartialCaveatInfo, com.authzed.api.v1.Core.PartialCaveatInfo.Builder, com.authzed.api.v1.Core.PartialCaveatInfoOrBuilder> partialCaveatInfoBuilder_;
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 3 [(.validate.rules) = { ... }
* @return Whether the partialCaveatInfo field is set.
*/
public boolean hasPartialCaveatInfo() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 3 [(.validate.rules) = { ... }
* @return The partialCaveatInfo.
*/
public com.authzed.api.v1.Core.PartialCaveatInfo getPartialCaveatInfo() {
if (partialCaveatInfoBuilder_ == null) {
return partialCaveatInfo_ == null ? com.authzed.api.v1.Core.PartialCaveatInfo.getDefaultInstance() : partialCaveatInfo_;
} else {
return partialCaveatInfoBuilder_.getMessage();
}
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 3 [(.validate.rules) = { ... }
*/
public Builder setPartialCaveatInfo(com.authzed.api.v1.Core.PartialCaveatInfo value) {
if (partialCaveatInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
partialCaveatInfo_ = value;
} else {
partialCaveatInfoBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 3 [(.validate.rules) = { ... }
*/
public Builder setPartialCaveatInfo(
com.authzed.api.v1.Core.PartialCaveatInfo.Builder builderForValue) {
if (partialCaveatInfoBuilder_ == null) {
partialCaveatInfo_ = builderForValue.build();
} else {
partialCaveatInfoBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 3 [(.validate.rules) = { ... }
*/
public Builder mergePartialCaveatInfo(com.authzed.api.v1.Core.PartialCaveatInfo value) {
if (partialCaveatInfoBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0) &&
partialCaveatInfo_ != null &&
partialCaveatInfo_ != com.authzed.api.v1.Core.PartialCaveatInfo.getDefaultInstance()) {
getPartialCaveatInfoBuilder().mergeFrom(value);
} else {
partialCaveatInfo_ = value;
}
} else {
partialCaveatInfoBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 3 [(.validate.rules) = { ... }
*/
public Builder clearPartialCaveatInfo() {
bitField0_ = (bitField0_ & ~0x00000004);
partialCaveatInfo_ = null;
if (partialCaveatInfoBuilder_ != null) {
partialCaveatInfoBuilder_.dispose();
partialCaveatInfoBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 3 [(.validate.rules) = { ... }
*/
public com.authzed.api.v1.Core.PartialCaveatInfo.Builder getPartialCaveatInfoBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getPartialCaveatInfoFieldBuilder().getBuilder();
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 3 [(.validate.rules) = { ... }
*/
public com.authzed.api.v1.Core.PartialCaveatInfoOrBuilder getPartialCaveatInfoOrBuilder() {
if (partialCaveatInfoBuilder_ != null) {
return partialCaveatInfoBuilder_.getMessageOrBuilder();
} else {
return partialCaveatInfo_ == null ?
com.authzed.api.v1.Core.PartialCaveatInfo.getDefaultInstance() : partialCaveatInfo_;
}
}
/**
*
* partial_caveat_info holds information of a partially-evaluated caveated response
*
*
* .authzed.api.v1.PartialCaveatInfo partial_caveat_info = 3 [(.validate.rules) = { ... }
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.PartialCaveatInfo, com.authzed.api.v1.Core.PartialCaveatInfo.Builder, com.authzed.api.v1.Core.PartialCaveatInfoOrBuilder>
getPartialCaveatInfoFieldBuilder() {
if (partialCaveatInfoBuilder_ == null) {
partialCaveatInfoBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.authzed.api.v1.Core.PartialCaveatInfo, com.authzed.api.v1.Core.PartialCaveatInfo.Builder, com.authzed.api.v1.Core.PartialCaveatInfoOrBuilder>(
getPartialCaveatInfo(),
getParentForChildren(),
isClean());
partialCaveatInfo_ = null;
}
return partialCaveatInfoBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:authzed.api.v1.ResolvedSubject)
}
// @@protoc_insertion_point(class_scope:authzed.api.v1.ResolvedSubject)
private static final com.authzed.api.v1.PermissionService.ResolvedSubject DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.authzed.api.v1.PermissionService.ResolvedSubject();
}
public static com.authzed.api.v1.PermissionService.ResolvedSubject getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ResolvedSubject parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.authzed.api.v1.PermissionService.ResolvedSubject getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_authzed_api_v1_Consistency_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_authzed_api_v1_Consistency_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_authzed_api_v1_RelationshipFilter_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_authzed_api_v1_RelationshipFilter_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_authzed_api_v1_SubjectFilter_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_authzed_api_v1_SubjectFilter_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_authzed_api_v1_SubjectFilter_RelationFilter_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_authzed_api_v1_SubjectFilter_RelationFilter_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_authzed_api_v1_ReadRelationshipsRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_authzed_api_v1_ReadRelationshipsRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_authzed_api_v1_ReadRelationshipsResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_authzed_api_v1_ReadRelationshipsResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_authzed_api_v1_Precondition_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_authzed_api_v1_Precondition_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_authzed_api_v1_WriteRelationshipsRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_authzed_api_v1_WriteRelationshipsRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_authzed_api_v1_WriteRelationshipsResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_authzed_api_v1_WriteRelationshipsResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_authzed_api_v1_DeleteRelationshipsRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_authzed_api_v1_DeleteRelationshipsRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_authzed_api_v1_DeleteRelationshipsResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_authzed_api_v1_DeleteRelationshipsResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_authzed_api_v1_CheckPermissionRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_authzed_api_v1_CheckPermissionRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_authzed_api_v1_CheckPermissionResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_authzed_api_v1_CheckPermissionResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_authzed_api_v1_ExpandPermissionTreeRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_authzed_api_v1_ExpandPermissionTreeRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_authzed_api_v1_ExpandPermissionTreeResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_authzed_api_v1_ExpandPermissionTreeResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_authzed_api_v1_LookupResourcesRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_authzed_api_v1_LookupResourcesRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_authzed_api_v1_LookupResourcesResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_authzed_api_v1_LookupResourcesResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_authzed_api_v1_LookupSubjectsRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_authzed_api_v1_LookupSubjectsRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_authzed_api_v1_LookupSubjectsResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_authzed_api_v1_LookupSubjectsResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_authzed_api_v1_ResolvedSubject_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_authzed_api_v1_ResolvedSubject_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\'authzed/api/v1/permission_service.prot" +
"o\022\016authzed.api.v1\032\034google/protobuf/struc" +
"t.proto\032\034google/api/annotations.proto\032\027v" +
"alidate/validate.proto\032\031authzed/api/v1/c" +
"ore.proto\"\331\001\n\013Consistency\022#\n\020minimize_la" +
"tency\030\001 \001(\010B\007\372B\004j\002\010\001H\000\0225\n\021at_least_as_fr" +
"esh\030\002 \001(\0132\030.authzed.api.v1.ZedTokenH\000\0225\n" +
"\021at_exact_snapshot\030\003 \001(\0132\030.authzed.api.v" +
"1.ZedTokenH\000\022#\n\020fully_consistent\030\004 \001(\010B\007" +
"\372B\004j\002\010\001H\000B\022\n\013requirement\022\003\370B\001\"\315\002\n\022Relati" +
"onshipFilter\022_\n\rresource_type\030\001 \001(\tBH\372BE" +
"rC(\200\0012>^([a-z][a-z0-9_]{1,61}[a-z0-9]/)?" +
"[a-z][a-z0-9_]{1,62}[a-z0-9]$\022O\n\024optiona" +
"l_resource_id\030\002 \001(\tB1\372B.r,(\200\0012\'^([a-zA-Z" +
"0-9_][a-zA-Z0-9/_|-]{0,127})?$\022E\n\021option" +
"al_relation\030\003 \001(\tB*\372B\'r%(@2!^([a-z][a-z0" +
"-9_]{1,62}[a-z0-9])?$\022>\n\027optional_subjec" +
"t_filter\030\004 \001(\0132\035.authzed.api.v1.SubjectF" +
"ilter\"\335\002\n\rSubjectFilter\022^\n\014subject_type\030" +
"\001 \001(\tBH\372BErC(\200\0012>^([a-z][a-z0-9_]{1,61}[" +
"a-z0-9]/)?[a-z][a-z0-9_]{1,62}[a-z0-9]$\022" +
"S\n\023optional_subject_id\030\002 \001(\tB6\372B3r1(\200\0012," +
"^(([a-zA-Z0-9_][a-zA-Z0-9/_|-]{0,127})|\\" +
"*)?$\022G\n\021optional_relation\030\003 \001(\0132,.authze" +
"d.api.v1.SubjectFilter.RelationFilter\032N\n" +
"\016RelationFilter\022<\n\010relation\030\001 \001(\tB*\372B\'r%" +
"(@2!^([a-z][a-z0-9_]{1,62}[a-z0-9])?$\"\227\001" +
"\n\030ReadRelationshipsRequest\0220\n\013consistenc" +
"y\030\001 \001(\0132\033.authzed.api.v1.Consistency\022I\n\023" +
"relationship_filter\030\002 \001(\0132\".authzed.api." +
"v1.RelationshipFilterB\010\372B\005\212\001\002\020\001\"\216\001\n\031Read" +
"RelationshipsResponse\0223\n\007read_at\030\001 \001(\0132\030" +
".authzed.api.v1.ZedTokenB\010\372B\005\212\001\002\020\001\022<\n\014re" +
"lationship\030\002 \001(\0132\034.authzed.api.v1.Relati" +
"onshipB\010\372B\005\212\001\002\020\001\"\363\001\n\014Precondition\022E\n\tope" +
"ration\030\001 \001(\0162&.authzed.api.v1.Preconditi" +
"on.OperationB\n\372B\007\202\001\004\020\001 \000\022<\n\006filter\030\002 \001(\013" +
"2\".authzed.api.v1.RelationshipFilterB\010\372B" +
"\005\212\001\002\020\001\"^\n\tOperation\022\031\n\025OPERATION_UNSPECI" +
"FIED\020\000\022\034\n\030OPERATION_MUST_NOT_MATCH\020\001\022\030\n\024" +
"OPERATION_MUST_MATCH\020\002\"\254\001\n\031WriteRelation" +
"shipsRequest\022B\n\007updates\030\001 \003(\0132\".authzed." +
"api.v1.RelationshipUpdateB\r\372B\n\222\001\007\"\005\212\001\002\020\001" +
"\022K\n\026optional_preconditions\030\002 \003(\0132\034.authz" +
"ed.api.v1.PreconditionB\r\372B\n\222\001\007\"\005\212\001\002\020\001\"J\n" +
"\032WriteRelationshipsResponse\022,\n\nwritten_a" +
"t\030\001 \001(\0132\030.authzed.api.v1.ZedToken\"\264\001\n\032De" +
"leteRelationshipsRequest\022I\n\023relationship" +
"_filter\030\001 \001(\0132\".authzed.api.v1.Relations" +
"hipFilterB\010\372B\005\212\001\002\020\001\022K\n\026optional_precondi" +
"tions\030\002 \003(\0132\034.authzed.api.v1.Preconditio" +
"nB\r\372B\n\222\001\007\"\005\212\001\002\020\001\"K\n\033DeleteRelationshipsR" +
"esponse\022,\n\ndeleted_at\030\001 \001(\0132\030.authzed.ap" +
"i.v1.ZedToken\"\270\002\n\026CheckPermissionRequest" +
"\0220\n\013consistency\030\001 \001(\0132\033.authzed.api.v1.C" +
"onsistency\022;\n\010resource\030\002 \001(\0132\037.authzed.a" +
"pi.v1.ObjectReferenceB\010\372B\005\212\001\002\020\001\022>\n\npermi" +
"ssion\030\003 \001(\tB*\372B\'r%(@2!^([a-z][a-z0-9_]{1" +
",62}[a-z0-9])?$\022;\n\007subject\030\004 \001(\0132 .authz" +
"ed.api.v1.SubjectReferenceB\010\372B\005\212\001\002\020\001\0222\n\007" +
"context\030\005 \001(\0132\027.google.protobuf.StructB\010" +
"\372B\005\212\001\002\020\000\"\232\003\n\027CheckPermissionResponse\0226\n\n" +
"checked_at\030\001 \001(\0132\030.authzed.api.v1.ZedTok" +
"enB\010\372B\005\212\001\002\020\000\022Z\n\016permissionship\030\002 \001(\01626.a" +
"uthzed.api.v1.CheckPermissionResponse.Pe" +
"rmissionshipB\n\372B\007\202\001\004\020\001 \000\022H\n\023partial_cave" +
"at_info\030\003 \001(\0132!.authzed.api.v1.PartialCa" +
"veatInfoB\010\372B\005\212\001\002\020\000\"\240\001\n\016Permissionship\022\036\n" +
"\032PERMISSIONSHIP_UNSPECIFIED\020\000\022 \n\034PERMISS" +
"IONSHIP_NO_PERMISSION\020\001\022!\n\035PERMISSIONSHI" +
"P_HAS_PERMISSION\020\002\022)\n%PERMISSIONSHIP_CON" +
"DITIONAL_PERMISSION\020\003\"\314\001\n\033ExpandPermissi" +
"onTreeRequest\0220\n\013consistency\030\001 \001(\0132\033.aut" +
"hzed.api.v1.Consistency\022;\n\010resource\030\002 \001(" +
"\0132\037.authzed.api.v1.ObjectReferenceB\010\372B\005\212" +
"\001\002\020\001\022>\n\npermission\030\003 \001(\tB*\372B\'r%(@2!^([a-" +
"z][a-z0-9_]{1,62}[a-z0-9])?$\"\214\001\n\034ExpandP" +
"ermissionTreeResponse\022-\n\013expanded_at\030\001 \001" +
"(\0132\030.authzed.api.v1.ZedToken\022=\n\ttree_roo" +
"t\030\002 \001(\0132*.authzed.api.v1.PermissionRelat" +
"ionshipTree\"\340\002\n\026LookupResourcesRequest\0220" +
"\n\013consistency\030\001 \001(\0132\033.authzed.api.v1.Con" +
"sistency\022f\n\024resource_object_type\030\002 \001(\tBH" +
"\372BErC(\200\0012>^([a-z][a-z0-9_]{1,61}[a-z0-9]" +
"/)?[a-z][a-z0-9_]{1,62}[a-z0-9]$\022;\n\nperm" +
"ission\030\003 \001(\tB\'\372B$r\"(@2\036^[a-z][a-z0-9_]{1" +
",62}[a-z0-9]$\022;\n\007subject\030\004 \001(\0132 .authzed" +
".api.v1.SubjectReferenceB\010\372B\005\212\001\002\020\001\0222\n\007co" +
"ntext\030\005 \001(\0132\027.google.protobuf.StructB\010\372B" +
"\005\212\001\002\020\000\"\371\001\n\027LookupResourcesResponse\022.\n\014lo" +
"oked_up_at\030\001 \001(\0132\030.authzed.api.v1.ZedTok" +
"en\022\032\n\022resource_object_id\030\002 \001(\t\022H\n\016permis" +
"sionship\030\003 \001(\0162$.authzed.api.v1.LookupPe" +
"rmissionshipB\n\372B\007\202\001\004\020\001 \000\022H\n\023partial_cave" +
"at_info\030\004 \001(\0132!.authzed.api.v1.PartialCa" +
"veatInfoB\010\372B\005\212\001\002\020\000\"\260\003\n\025LookupSubjectsReq" +
"uest\0220\n\013consistency\030\001 \001(\0132\033.authzed.api." +
"v1.Consistency\022;\n\010resource\030\002 \001(\0132\037.authz" +
"ed.api.v1.ObjectReferenceB\010\372B\005\212\001\002\020\001\022>\n\np" +
"ermission\030\003 \001(\tB*\372B\'r%(@2!^([a-z][a-z0-9" +
"_]{1,62}[a-z0-9])?$\022e\n\023subject_object_ty" +
"pe\030\004 \001(\tBH\372BErC(\200\0012>^([a-z][a-z0-9_]{1,6" +
"1}[a-z0-9]/)?[a-z][a-z0-9_]{1,62}[a-z0-9" +
"]$\022M\n\031optional_subject_relation\030\005 \001(\tB*\372" +
"B\'r%(@2!^([a-z][a-z0-9_]{1,62}[a-z0-9])?" +
"$\0222\n\007context\030\006 \001(\0132\027.google.protobuf.Str" +
"uctB\010\372B\005\212\001\002\020\000\"\217\003\n\026LookupSubjectsResponse" +
"\022.\n\014looked_up_at\030\001 \001(\0132\030.authzed.api.v1." +
"ZedToken\022\035\n\021subject_object_id\030\002 \001(\tB\002\030\001\022" +
" \n\024excluded_subject_ids\030\003 \003(\tB\002\030\001\022J\n\016per" +
"missionship\030\004 \001(\0162$.authzed.api.v1.Looku" +
"pPermissionshipB\014\030\001\372B\007\202\001\004\020\001 \000\022J\n\023partial" +
"_caveat_info\030\005 \001(\0132!.authzed.api.v1.Part" +
"ialCaveatInfoB\n\030\001\372B\005\212\001\002\020\000\0220\n\007subject\030\006 \001" +
"(\0132\037.authzed.api.v1.ResolvedSubject\022:\n\021e" +
"xcluded_subjects\030\007 \003(\0132\037.authzed.api.v1." +
"ResolvedSubject\"\300\001\n\017ResolvedSubject\022\031\n\021s" +
"ubject_object_id\030\001 \001(\t\022H\n\016permissionship" +
"\030\002 \001(\0162$.authzed.api.v1.LookupPermission" +
"shipB\n\372B\007\202\001\004\020\001 \000\022H\n\023partial_caveat_info\030" +
"\003 \001(\0132!.authzed.api.v1.PartialCaveatInfo" +
"B\010\372B\005\212\001\002\020\000*\231\001\n\024LookupPermissionship\022%\n!L" +
"OOKUP_PERMISSIONSHIP_UNSPECIFIED\020\000\022(\n$LO" +
"OKUP_PERMISSIONSHIP_HAS_PERMISSION\020\001\0220\n," +
"LOOKUP_PERMISSIONSHIP_CONDITIONAL_PERMIS" +
"SION\020\0022\200\010\n\022PermissionsService\022\215\001\n\021ReadRe" +
"lationships\022(.authzed.api.v1.ReadRelatio" +
"nshipsRequest\032).authzed.api.v1.ReadRelat" +
"ionshipsResponse\"!\202\323\344\223\002\033\"\026/v1/relationsh" +
"ips/read:\001*0\001\022\217\001\n\022WriteRelationships\022).a" +
"uthzed.api.v1.WriteRelationshipsRequest\032" +
"*.authzed.api.v1.WriteRelationshipsRespo" +
"nse\"\"\202\323\344\223\002\034\"\027/v1/relationships/write:\001*\022" +
"\223\001\n\023DeleteRelationships\022*.authzed.api.v1" +
".DeleteRelationshipsRequest\032+.authzed.ap" +
"i.v1.DeleteRelationshipsResponse\"#\202\323\344\223\002\035" +
"\"\030/v1/relationships/delete:\001*\022\204\001\n\017CheckP" +
"ermission\022&.authzed.api.v1.CheckPermissi" +
"onRequest\032\'.authzed.api.v1.CheckPermissi" +
"onResponse\" \202\323\344\223\002\032\"\025/v1/permissions/chec" +
"k:\001*\022\224\001\n\024ExpandPermissionTree\022+.authzed." +
"api.v1.ExpandPermissionTreeRequest\032,.aut" +
"hzed.api.v1.ExpandPermissionTreeResponse" +
"\"!\202\323\344\223\002\033\"\026/v1/permissions/expand:\001*\022\212\001\n\017" +
"LookupResources\022&.authzed.api.v1.LookupR" +
"esourcesRequest\032\'.authzed.api.v1.LookupR" +
"esourcesResponse\"$\202\323\344\223\002\036\"\031/v1/permission" +
"s/resources:\001*0\001\022\206\001\n\016LookupSubjects\022%.au" +
"thzed.api.v1.LookupSubjectsRequest\032&.aut" +
"hzed.api.v1.LookupSubjectsResponse\"#\202\323\344\223" +
"\002\035\"\030/v1/permissions/subjects:\001*0\001BH\n\022com" +
".authzed.api.v1Z2github.com/authzed/auth" +
"zed-go/proto/authzed/api/v1b\006proto3"
};
descriptor = com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
com.google.protobuf.StructProto.getDescriptor(),
com.google.api.AnnotationsProto.getDescriptor(),
io.envoyproxy.pgv.validate.Validate.getDescriptor(),
com.authzed.api.v1.Core.getDescriptor(),
});
internal_static_authzed_api_v1_Consistency_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_authzed_api_v1_Consistency_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_authzed_api_v1_Consistency_descriptor,
new java.lang.String[] { "MinimizeLatency", "AtLeastAsFresh", "AtExactSnapshot", "FullyConsistent", "Requirement", });
internal_static_authzed_api_v1_RelationshipFilter_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_authzed_api_v1_RelationshipFilter_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_authzed_api_v1_RelationshipFilter_descriptor,
new java.lang.String[] { "ResourceType", "OptionalResourceId", "OptionalRelation", "OptionalSubjectFilter", });
internal_static_authzed_api_v1_SubjectFilter_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_authzed_api_v1_SubjectFilter_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_authzed_api_v1_SubjectFilter_descriptor,
new java.lang.String[] { "SubjectType", "OptionalSubjectId", "OptionalRelation", });
internal_static_authzed_api_v1_SubjectFilter_RelationFilter_descriptor =
internal_static_authzed_api_v1_SubjectFilter_descriptor.getNestedTypes().get(0);
internal_static_authzed_api_v1_SubjectFilter_RelationFilter_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_authzed_api_v1_SubjectFilter_RelationFilter_descriptor,
new java.lang.String[] { "Relation", });
internal_static_authzed_api_v1_ReadRelationshipsRequest_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_authzed_api_v1_ReadRelationshipsRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_authzed_api_v1_ReadRelationshipsRequest_descriptor,
new java.lang.String[] { "Consistency", "RelationshipFilter", });
internal_static_authzed_api_v1_ReadRelationshipsResponse_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_authzed_api_v1_ReadRelationshipsResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_authzed_api_v1_ReadRelationshipsResponse_descriptor,
new java.lang.String[] { "ReadAt", "Relationship", });
internal_static_authzed_api_v1_Precondition_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_authzed_api_v1_Precondition_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_authzed_api_v1_Precondition_descriptor,
new java.lang.String[] { "Operation", "Filter", });
internal_static_authzed_api_v1_WriteRelationshipsRequest_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_authzed_api_v1_WriteRelationshipsRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_authzed_api_v1_WriteRelationshipsRequest_descriptor,
new java.lang.String[] { "Updates", "OptionalPreconditions", });
internal_static_authzed_api_v1_WriteRelationshipsResponse_descriptor =
getDescriptor().getMessageTypes().get(7);
internal_static_authzed_api_v1_WriteRelationshipsResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_authzed_api_v1_WriteRelationshipsResponse_descriptor,
new java.lang.String[] { "WrittenAt", });
internal_static_authzed_api_v1_DeleteRelationshipsRequest_descriptor =
getDescriptor().getMessageTypes().get(8);
internal_static_authzed_api_v1_DeleteRelationshipsRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_authzed_api_v1_DeleteRelationshipsRequest_descriptor,
new java.lang.String[] { "RelationshipFilter", "OptionalPreconditions", });
internal_static_authzed_api_v1_DeleteRelationshipsResponse_descriptor =
getDescriptor().getMessageTypes().get(9);
internal_static_authzed_api_v1_DeleteRelationshipsResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_authzed_api_v1_DeleteRelationshipsResponse_descriptor,
new java.lang.String[] { "DeletedAt", });
internal_static_authzed_api_v1_CheckPermissionRequest_descriptor =
getDescriptor().getMessageTypes().get(10);
internal_static_authzed_api_v1_CheckPermissionRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_authzed_api_v1_CheckPermissionRequest_descriptor,
new java.lang.String[] { "Consistency", "Resource", "Permission", "Subject", "Context", });
internal_static_authzed_api_v1_CheckPermissionResponse_descriptor =
getDescriptor().getMessageTypes().get(11);
internal_static_authzed_api_v1_CheckPermissionResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_authzed_api_v1_CheckPermissionResponse_descriptor,
new java.lang.String[] { "CheckedAt", "Permissionship", "PartialCaveatInfo", });
internal_static_authzed_api_v1_ExpandPermissionTreeRequest_descriptor =
getDescriptor().getMessageTypes().get(12);
internal_static_authzed_api_v1_ExpandPermissionTreeRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_authzed_api_v1_ExpandPermissionTreeRequest_descriptor,
new java.lang.String[] { "Consistency", "Resource", "Permission", });
internal_static_authzed_api_v1_ExpandPermissionTreeResponse_descriptor =
getDescriptor().getMessageTypes().get(13);
internal_static_authzed_api_v1_ExpandPermissionTreeResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_authzed_api_v1_ExpandPermissionTreeResponse_descriptor,
new java.lang.String[] { "ExpandedAt", "TreeRoot", });
internal_static_authzed_api_v1_LookupResourcesRequest_descriptor =
getDescriptor().getMessageTypes().get(14);
internal_static_authzed_api_v1_LookupResourcesRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_authzed_api_v1_LookupResourcesRequest_descriptor,
new java.lang.String[] { "Consistency", "ResourceObjectType", "Permission", "Subject", "Context", });
internal_static_authzed_api_v1_LookupResourcesResponse_descriptor =
getDescriptor().getMessageTypes().get(15);
internal_static_authzed_api_v1_LookupResourcesResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_authzed_api_v1_LookupResourcesResponse_descriptor,
new java.lang.String[] { "LookedUpAt", "ResourceObjectId", "Permissionship", "PartialCaveatInfo", });
internal_static_authzed_api_v1_LookupSubjectsRequest_descriptor =
getDescriptor().getMessageTypes().get(16);
internal_static_authzed_api_v1_LookupSubjectsRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_authzed_api_v1_LookupSubjectsRequest_descriptor,
new java.lang.String[] { "Consistency", "Resource", "Permission", "SubjectObjectType", "OptionalSubjectRelation", "Context", });
internal_static_authzed_api_v1_LookupSubjectsResponse_descriptor =
getDescriptor().getMessageTypes().get(17);
internal_static_authzed_api_v1_LookupSubjectsResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_authzed_api_v1_LookupSubjectsResponse_descriptor,
new java.lang.String[] { "LookedUpAt", "SubjectObjectId", "ExcludedSubjectIds", "Permissionship", "PartialCaveatInfo", "Subject", "ExcludedSubjects", });
internal_static_authzed_api_v1_ResolvedSubject_descriptor =
getDescriptor().getMessageTypes().get(18);
internal_static_authzed_api_v1_ResolvedSubject_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_authzed_api_v1_ResolvedSubject_descriptor,
new java.lang.String[] { "SubjectObjectId", "Permissionship", "PartialCaveatInfo", });
com.google.protobuf.ExtensionRegistry registry =
com.google.protobuf.ExtensionRegistry.newInstance();
registry.add(com.google.api.AnnotationsProto.http);
registry.add(io.envoyproxy.pgv.validate.Validate.required);
registry.add(io.envoyproxy.pgv.validate.Validate.rules);
com.google.protobuf.Descriptors.FileDescriptor
.internalUpdateFileDescriptor(descriptor, registry);
com.google.protobuf.StructProto.getDescriptor();
com.google.api.AnnotationsProto.getDescriptor();
io.envoyproxy.pgv.validate.Validate.getDescriptor();
com.authzed.api.v1.Core.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy