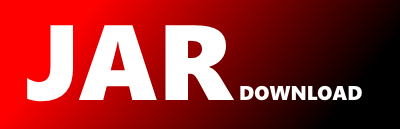
grpc.gateway.protoc_gen_openapiv2.options.OperationKt.kt Maven / Gradle / Ivy
//Generated by the protocol buffer compiler. DO NOT EDIT!
// source: protoc-gen-openapiv2/options/openapiv2.proto
package grpc.gateway.protoc_gen_openapiv2.options;
@kotlin.jvm.JvmName("-initializeoperation")
public inline fun operation(block: grpc.gateway.protoc_gen_openapiv2.options.OperationKt.Dsl.() -> kotlin.Unit): grpc.gateway.protoc_gen_openapiv2.options.Openapiv2.Operation =
grpc.gateway.protoc_gen_openapiv2.options.OperationKt.Dsl._create(grpc.gateway.protoc_gen_openapiv2.options.Openapiv2.Operation.newBuilder()).apply { block() }._build()
public object OperationKt {
@kotlin.OptIn(com.google.protobuf.kotlin.OnlyForUseByGeneratedProtoCode::class)
@com.google.protobuf.kotlin.ProtoDslMarker
public class Dsl private constructor(
private val _builder: grpc.gateway.protoc_gen_openapiv2.options.Openapiv2.Operation.Builder
) {
public companion object {
@kotlin.jvm.JvmSynthetic
@kotlin.PublishedApi
internal fun _create(builder: grpc.gateway.protoc_gen_openapiv2.options.Openapiv2.Operation.Builder): Dsl = Dsl(builder)
}
@kotlin.jvm.JvmSynthetic
@kotlin.PublishedApi
internal fun _build(): grpc.gateway.protoc_gen_openapiv2.options.Openapiv2.Operation = _builder.build()
/**
* An uninstantiable, behaviorless type to represent the field in
* generics.
*/
@kotlin.OptIn(com.google.protobuf.kotlin.OnlyForUseByGeneratedProtoCode::class)
public class TagsProxy private constructor() : com.google.protobuf.kotlin.DslProxy()
/**
*
* A list of tags for API documentation control. Tags can be used for logical
* grouping of operations by resources or any other qualifier.
*
*
* repeated string tags = 1;
* @return A list containing the tags.
*/
public val tags: com.google.protobuf.kotlin.DslList
@kotlin.jvm.JvmSynthetic
get() = com.google.protobuf.kotlin.DslList(
_builder.getTagsList()
)
/**
*
* A list of tags for API documentation control. Tags can be used for logical
* grouping of operations by resources or any other qualifier.
*
*
* repeated string tags = 1;
* @param value The tags to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("addTags")
public fun com.google.protobuf.kotlin.DslList.add(value: kotlin.String) {
_builder.addTags(value)
}
/**
*
* A list of tags for API documentation control. Tags can be used for logical
* grouping of operations by resources or any other qualifier.
*
*
* repeated string tags = 1;
* @param value The tags to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("plusAssignTags")
@Suppress("NOTHING_TO_INLINE")
public inline operator fun com.google.protobuf.kotlin.DslList.plusAssign(value: kotlin.String) {
add(value)
}
/**
*
* A list of tags for API documentation control. Tags can be used for logical
* grouping of operations by resources or any other qualifier.
*
*
* repeated string tags = 1;
* @param values The tags to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("addAllTags")
public fun com.google.protobuf.kotlin.DslList.addAll(values: kotlin.collections.Iterable) {
_builder.addAllTags(values)
}
/**
*
* A list of tags for API documentation control. Tags can be used for logical
* grouping of operations by resources or any other qualifier.
*
*
* repeated string tags = 1;
* @param values The tags to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("plusAssignAllTags")
@Suppress("NOTHING_TO_INLINE")
public inline operator fun com.google.protobuf.kotlin.DslList.plusAssign(values: kotlin.collections.Iterable) {
addAll(values)
}
/**
*
* A list of tags for API documentation control. Tags can be used for logical
* grouping of operations by resources or any other qualifier.
*
*
* repeated string tags = 1;
* @param index The index to set the value at.
* @param value The tags to set.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("setTags")
public operator fun com.google.protobuf.kotlin.DslList.set(index: kotlin.Int, value: kotlin.String) {
_builder.setTags(index, value)
}/**
*
* A list of tags for API documentation control. Tags can be used for logical
* grouping of operations by resources or any other qualifier.
*
*
* repeated string tags = 1;
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("clearTags")
public fun com.google.protobuf.kotlin.DslList.clear() {
_builder.clearTags()
}
/**
*
* A short summary of what the operation does. For maximum readability in the
* swagger-ui, this field SHOULD be less than 120 characters.
*
*
* string summary = 2;
*/
public var summary: kotlin.String
@JvmName("getSummary")
get() = _builder.getSummary()
@JvmName("setSummary")
set(value) {
_builder.setSummary(value)
}
/**
*
* A short summary of what the operation does. For maximum readability in the
* swagger-ui, this field SHOULD be less than 120 characters.
*
*
* string summary = 2;
*/
public fun clearSummary() {
_builder.clearSummary()
}
/**
*
* A verbose explanation of the operation behavior. GFM syntax can be used for
* rich text representation.
*
*
* string description = 3;
*/
public var description: kotlin.String
@JvmName("getDescription")
get() = _builder.getDescription()
@JvmName("setDescription")
set(value) {
_builder.setDescription(value)
}
/**
*
* A verbose explanation of the operation behavior. GFM syntax can be used for
* rich text representation.
*
*
* string description = 3;
*/
public fun clearDescription() {
_builder.clearDescription()
}
/**
*
* Additional external documentation for this operation.
*
*
* .grpc.gateway.protoc_gen_openapiv2.options.ExternalDocumentation external_docs = 4;
*/
public var externalDocs: grpc.gateway.protoc_gen_openapiv2.options.Openapiv2.ExternalDocumentation
@JvmName("getExternalDocs")
get() = _builder.getExternalDocs()
@JvmName("setExternalDocs")
set(value) {
_builder.setExternalDocs(value)
}
/**
*
* Additional external documentation for this operation.
*
*
* .grpc.gateway.protoc_gen_openapiv2.options.ExternalDocumentation external_docs = 4;
*/
public fun clearExternalDocs() {
_builder.clearExternalDocs()
}
/**
*
* Additional external documentation for this operation.
*
*
* .grpc.gateway.protoc_gen_openapiv2.options.ExternalDocumentation external_docs = 4;
* @return Whether the externalDocs field is set.
*/
public fun hasExternalDocs(): kotlin.Boolean {
return _builder.hasExternalDocs()
}
/**
*
* Unique string used to identify the operation. The id MUST be unique among
* all operations described in the API. Tools and libraries MAY use the
* operationId to uniquely identify an operation, therefore, it is recommended
* to follow common programming naming conventions.
*
*
* string operation_id = 5;
*/
public var operationId: kotlin.String
@JvmName("getOperationId")
get() = _builder.getOperationId()
@JvmName("setOperationId")
set(value) {
_builder.setOperationId(value)
}
/**
*
* Unique string used to identify the operation. The id MUST be unique among
* all operations described in the API. Tools and libraries MAY use the
* operationId to uniquely identify an operation, therefore, it is recommended
* to follow common programming naming conventions.
*
*
* string operation_id = 5;
*/
public fun clearOperationId() {
_builder.clearOperationId()
}
/**
* An uninstantiable, behaviorless type to represent the field in
* generics.
*/
@kotlin.OptIn(com.google.protobuf.kotlin.OnlyForUseByGeneratedProtoCode::class)
public class ConsumesProxy private constructor() : com.google.protobuf.kotlin.DslProxy()
/**
*
* A list of MIME types the operation can consume. This overrides the consumes
* definition at the OpenAPI Object. An empty value MAY be used to clear the
* global definition. Value MUST be as described under Mime Types.
*
*
* repeated string consumes = 6;
* @return A list containing the consumes.
*/
public val consumes: com.google.protobuf.kotlin.DslList
@kotlin.jvm.JvmSynthetic
get() = com.google.protobuf.kotlin.DslList(
_builder.getConsumesList()
)
/**
*
* A list of MIME types the operation can consume. This overrides the consumes
* definition at the OpenAPI Object. An empty value MAY be used to clear the
* global definition. Value MUST be as described under Mime Types.
*
*
* repeated string consumes = 6;
* @param value The consumes to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("addConsumes")
public fun com.google.protobuf.kotlin.DslList.add(value: kotlin.String) {
_builder.addConsumes(value)
}
/**
*
* A list of MIME types the operation can consume. This overrides the consumes
* definition at the OpenAPI Object. An empty value MAY be used to clear the
* global definition. Value MUST be as described under Mime Types.
*
*
* repeated string consumes = 6;
* @param value The consumes to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("plusAssignConsumes")
@Suppress("NOTHING_TO_INLINE")
public inline operator fun com.google.protobuf.kotlin.DslList.plusAssign(value: kotlin.String) {
add(value)
}
/**
*
* A list of MIME types the operation can consume. This overrides the consumes
* definition at the OpenAPI Object. An empty value MAY be used to clear the
* global definition. Value MUST be as described under Mime Types.
*
*
* repeated string consumes = 6;
* @param values The consumes to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("addAllConsumes")
public fun com.google.protobuf.kotlin.DslList.addAll(values: kotlin.collections.Iterable) {
_builder.addAllConsumes(values)
}
/**
*
* A list of MIME types the operation can consume. This overrides the consumes
* definition at the OpenAPI Object. An empty value MAY be used to clear the
* global definition. Value MUST be as described under Mime Types.
*
*
* repeated string consumes = 6;
* @param values The consumes to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("plusAssignAllConsumes")
@Suppress("NOTHING_TO_INLINE")
public inline operator fun com.google.protobuf.kotlin.DslList.plusAssign(values: kotlin.collections.Iterable) {
addAll(values)
}
/**
*
* A list of MIME types the operation can consume. This overrides the consumes
* definition at the OpenAPI Object. An empty value MAY be used to clear the
* global definition. Value MUST be as described under Mime Types.
*
*
* repeated string consumes = 6;
* @param index The index to set the value at.
* @param value The consumes to set.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("setConsumes")
public operator fun com.google.protobuf.kotlin.DslList.set(index: kotlin.Int, value: kotlin.String) {
_builder.setConsumes(index, value)
}/**
*
* A list of MIME types the operation can consume. This overrides the consumes
* definition at the OpenAPI Object. An empty value MAY be used to clear the
* global definition. Value MUST be as described under Mime Types.
*
*
* repeated string consumes = 6;
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("clearConsumes")
public fun com.google.protobuf.kotlin.DslList.clear() {
_builder.clearConsumes()
}
/**
* An uninstantiable, behaviorless type to represent the field in
* generics.
*/
@kotlin.OptIn(com.google.protobuf.kotlin.OnlyForUseByGeneratedProtoCode::class)
public class ProducesProxy private constructor() : com.google.protobuf.kotlin.DslProxy()
/**
*
* A list of MIME types the operation can produce. This overrides the produces
* definition at the OpenAPI Object. An empty value MAY be used to clear the
* global definition. Value MUST be as described under Mime Types.
*
*
* repeated string produces = 7;
* @return A list containing the produces.
*/
public val produces: com.google.protobuf.kotlin.DslList
@kotlin.jvm.JvmSynthetic
get() = com.google.protobuf.kotlin.DslList(
_builder.getProducesList()
)
/**
*
* A list of MIME types the operation can produce. This overrides the produces
* definition at the OpenAPI Object. An empty value MAY be used to clear the
* global definition. Value MUST be as described under Mime Types.
*
*
* repeated string produces = 7;
* @param value The produces to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("addProduces")
public fun com.google.protobuf.kotlin.DslList.add(value: kotlin.String) {
_builder.addProduces(value)
}
/**
*
* A list of MIME types the operation can produce. This overrides the produces
* definition at the OpenAPI Object. An empty value MAY be used to clear the
* global definition. Value MUST be as described under Mime Types.
*
*
* repeated string produces = 7;
* @param value The produces to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("plusAssignProduces")
@Suppress("NOTHING_TO_INLINE")
public inline operator fun com.google.protobuf.kotlin.DslList.plusAssign(value: kotlin.String) {
add(value)
}
/**
*
* A list of MIME types the operation can produce. This overrides the produces
* definition at the OpenAPI Object. An empty value MAY be used to clear the
* global definition. Value MUST be as described under Mime Types.
*
*
* repeated string produces = 7;
* @param values The produces to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("addAllProduces")
public fun com.google.protobuf.kotlin.DslList.addAll(values: kotlin.collections.Iterable) {
_builder.addAllProduces(values)
}
/**
*
* A list of MIME types the operation can produce. This overrides the produces
* definition at the OpenAPI Object. An empty value MAY be used to clear the
* global definition. Value MUST be as described under Mime Types.
*
*
* repeated string produces = 7;
* @param values The produces to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("plusAssignAllProduces")
@Suppress("NOTHING_TO_INLINE")
public inline operator fun com.google.protobuf.kotlin.DslList.plusAssign(values: kotlin.collections.Iterable) {
addAll(values)
}
/**
*
* A list of MIME types the operation can produce. This overrides the produces
* definition at the OpenAPI Object. An empty value MAY be used to clear the
* global definition. Value MUST be as described under Mime Types.
*
*
* repeated string produces = 7;
* @param index The index to set the value at.
* @param value The produces to set.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("setProduces")
public operator fun com.google.protobuf.kotlin.DslList.set(index: kotlin.Int, value: kotlin.String) {
_builder.setProduces(index, value)
}/**
*
* A list of MIME types the operation can produce. This overrides the produces
* definition at the OpenAPI Object. An empty value MAY be used to clear the
* global definition. Value MUST be as described under Mime Types.
*
*
* repeated string produces = 7;
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("clearProduces")
public fun com.google.protobuf.kotlin.DslList.clear() {
_builder.clearProduces()
}
/**
* An uninstantiable, behaviorless type to represent the field in
* generics.
*/
@kotlin.OptIn(com.google.protobuf.kotlin.OnlyForUseByGeneratedProtoCode::class)
public class ResponsesProxy private constructor() : com.google.protobuf.kotlin.DslProxy()
/**
*
* The list of possible responses as they are returned from executing this
* operation.
*
*
* map<string, .grpc.gateway.protoc_gen_openapiv2.options.Response> responses = 9;
*/
public val responses: com.google.protobuf.kotlin.DslMap
@kotlin.jvm.JvmSynthetic
@JvmName("getResponsesMap")
get() = com.google.protobuf.kotlin.DslMap(
_builder.getResponsesMap()
)
/**
*
* The list of possible responses as they are returned from executing this
* operation.
*
*
* map<string, .grpc.gateway.protoc_gen_openapiv2.options.Response> responses = 9;
*/
@JvmName("putResponses")
public fun com.google.protobuf.kotlin.DslMap
.put(key: kotlin.String, value: grpc.gateway.protoc_gen_openapiv2.options.Openapiv2.Response) {
_builder.putResponses(key, value)
}
/**
*
* The list of possible responses as they are returned from executing this
* operation.
*
*
* map<string, .grpc.gateway.protoc_gen_openapiv2.options.Response> responses = 9;
*/
@kotlin.jvm.JvmSynthetic
@JvmName("setResponses")
@Suppress("NOTHING_TO_INLINE")
public inline operator fun com.google.protobuf.kotlin.DslMap
.set(key: kotlin.String, value: grpc.gateway.protoc_gen_openapiv2.options.Openapiv2.Response) {
put(key, value)
}
/**
*
* The list of possible responses as they are returned from executing this
* operation.
*
*
* map<string, .grpc.gateway.protoc_gen_openapiv2.options.Response> responses = 9;
*/
@kotlin.jvm.JvmSynthetic
@JvmName("removeResponses")
public fun com.google.protobuf.kotlin.DslMap
.remove(key: kotlin.String) {
_builder.removeResponses(key)
}
/**
*
* The list of possible responses as they are returned from executing this
* operation.
*
*
* map<string, .grpc.gateway.protoc_gen_openapiv2.options.Response> responses = 9;
*/
@kotlin.jvm.JvmSynthetic
@JvmName("putAllResponses")
public fun com.google.protobuf.kotlin.DslMap
.putAll(map: kotlin.collections.Map) {
_builder.putAllResponses(map)
}
/**
*
* The list of possible responses as they are returned from executing this
* operation.
*
*
* map<string, .grpc.gateway.protoc_gen_openapiv2.options.Response> responses = 9;
*/
@kotlin.jvm.JvmSynthetic
@JvmName("clearResponses")
public fun com.google.protobuf.kotlin.DslMap
.clear() {
_builder.clearResponses()
}
/**
* An uninstantiable, behaviorless type to represent the field in
* generics.
*/
@kotlin.OptIn(com.google.protobuf.kotlin.OnlyForUseByGeneratedProtoCode::class)
public class SchemesProxy private constructor() : com.google.protobuf.kotlin.DslProxy()
/**
*
* The transfer protocol for the operation. Values MUST be from the list:
* "http", "https", "ws", "wss". The value overrides the OpenAPI Object
* schemes definition.
*
*
* repeated .grpc.gateway.protoc_gen_openapiv2.options.Scheme schemes = 10;
*/
public val schemes: com.google.protobuf.kotlin.DslList
@kotlin.jvm.JvmSynthetic
get() = com.google.protobuf.kotlin.DslList(
_builder.getSchemesList()
)
/**
*
* The transfer protocol for the operation. Values MUST be from the list:
* "http", "https", "ws", "wss". The value overrides the OpenAPI Object
* schemes definition.
*
*
* repeated .grpc.gateway.protoc_gen_openapiv2.options.Scheme schemes = 10;
* @param value The schemes to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("addSchemes")
public fun com.google.protobuf.kotlin.DslList.add(value: grpc.gateway.protoc_gen_openapiv2.options.Openapiv2.Scheme) {
_builder.addSchemes(value)
}/**
*
* The transfer protocol for the operation. Values MUST be from the list:
* "http", "https", "ws", "wss". The value overrides the OpenAPI Object
* schemes definition.
*
*
* repeated .grpc.gateway.protoc_gen_openapiv2.options.Scheme schemes = 10;
* @param value The schemes to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("plusAssignSchemes")
@Suppress("NOTHING_TO_INLINE")
public inline operator fun com.google.protobuf.kotlin.DslList.plusAssign(value: grpc.gateway.protoc_gen_openapiv2.options.Openapiv2.Scheme) {
add(value)
}/**
*
* The transfer protocol for the operation. Values MUST be from the list:
* "http", "https", "ws", "wss". The value overrides the OpenAPI Object
* schemes definition.
*
*
* repeated .grpc.gateway.protoc_gen_openapiv2.options.Scheme schemes = 10;
* @param values The schemes to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("addAllSchemes")
public fun com.google.protobuf.kotlin.DslList.addAll(values: kotlin.collections.Iterable) {
_builder.addAllSchemes(values)
}/**
*
* The transfer protocol for the operation. Values MUST be from the list:
* "http", "https", "ws", "wss". The value overrides the OpenAPI Object
* schemes definition.
*
*
* repeated .grpc.gateway.protoc_gen_openapiv2.options.Scheme schemes = 10;
* @param values The schemes to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("plusAssignAllSchemes")
@Suppress("NOTHING_TO_INLINE")
public inline operator fun com.google.protobuf.kotlin.DslList.plusAssign(values: kotlin.collections.Iterable) {
addAll(values)
}/**
*
* The transfer protocol for the operation. Values MUST be from the list:
* "http", "https", "ws", "wss". The value overrides the OpenAPI Object
* schemes definition.
*
*
* repeated .grpc.gateway.protoc_gen_openapiv2.options.Scheme schemes = 10;
* @param index The index to set the value at.
* @param value The schemes to set.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("setSchemes")
public operator fun com.google.protobuf.kotlin.DslList.set(index: kotlin.Int, value: grpc.gateway.protoc_gen_openapiv2.options.Openapiv2.Scheme) {
_builder.setSchemes(index, value)
}/**
*
* The transfer protocol for the operation. Values MUST be from the list:
* "http", "https", "ws", "wss". The value overrides the OpenAPI Object
* schemes definition.
*
*
* repeated .grpc.gateway.protoc_gen_openapiv2.options.Scheme schemes = 10;
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("clearSchemes")
public fun com.google.protobuf.kotlin.DslList.clear() {
_builder.clearSchemes()
}
/**
*
* Declares this operation to be deprecated. Usage of the declared operation
* should be refrained. Default value is false.
*
*
* bool deprecated = 11;
*/
public var deprecated: kotlin.Boolean
@JvmName("getDeprecated")
get() = _builder.getDeprecated()
@JvmName("setDeprecated")
set(value) {
_builder.setDeprecated(value)
}
/**
*
* Declares this operation to be deprecated. Usage of the declared operation
* should be refrained. Default value is false.
*
*
* bool deprecated = 11;
*/
public fun clearDeprecated() {
_builder.clearDeprecated()
}
/**
* An uninstantiable, behaviorless type to represent the field in
* generics.
*/
@kotlin.OptIn(com.google.protobuf.kotlin.OnlyForUseByGeneratedProtoCode::class)
public class SecurityProxy private constructor() : com.google.protobuf.kotlin.DslProxy()
/**
*
* A declaration of which security schemes are applied for this operation. The
* list of values describes alternative security schemes that can be used
* (that is, there is a logical OR between the security requirements). This
* definition overrides any declared top-level security. To remove a top-level
* security declaration, an empty array can be used.
*
*
* repeated .grpc.gateway.protoc_gen_openapiv2.options.SecurityRequirement security = 12;
*/
public val security: com.google.protobuf.kotlin.DslList
@kotlin.jvm.JvmSynthetic
get() = com.google.protobuf.kotlin.DslList(
_builder.getSecurityList()
)
/**
*
* A declaration of which security schemes are applied for this operation. The
* list of values describes alternative security schemes that can be used
* (that is, there is a logical OR between the security requirements). This
* definition overrides any declared top-level security. To remove a top-level
* security declaration, an empty array can be used.
*
*
* repeated .grpc.gateway.protoc_gen_openapiv2.options.SecurityRequirement security = 12;
* @param value The security to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("addSecurity")
public fun com.google.protobuf.kotlin.DslList.add(value: grpc.gateway.protoc_gen_openapiv2.options.Openapiv2.SecurityRequirement) {
_builder.addSecurity(value)
}
/**
*
* A declaration of which security schemes are applied for this operation. The
* list of values describes alternative security schemes that can be used
* (that is, there is a logical OR between the security requirements). This
* definition overrides any declared top-level security. To remove a top-level
* security declaration, an empty array can be used.
*
*
* repeated .grpc.gateway.protoc_gen_openapiv2.options.SecurityRequirement security = 12;
* @param value The security to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("plusAssignSecurity")
@Suppress("NOTHING_TO_INLINE")
public inline operator fun com.google.protobuf.kotlin.DslList.plusAssign(value: grpc.gateway.protoc_gen_openapiv2.options.Openapiv2.SecurityRequirement) {
add(value)
}
/**
*
* A declaration of which security schemes are applied for this operation. The
* list of values describes alternative security schemes that can be used
* (that is, there is a logical OR between the security requirements). This
* definition overrides any declared top-level security. To remove a top-level
* security declaration, an empty array can be used.
*
*
* repeated .grpc.gateway.protoc_gen_openapiv2.options.SecurityRequirement security = 12;
* @param values The security to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("addAllSecurity")
public fun com.google.protobuf.kotlin.DslList.addAll(values: kotlin.collections.Iterable) {
_builder.addAllSecurity(values)
}
/**
*
* A declaration of which security schemes are applied for this operation. The
* list of values describes alternative security schemes that can be used
* (that is, there is a logical OR between the security requirements). This
* definition overrides any declared top-level security. To remove a top-level
* security declaration, an empty array can be used.
*
*
* repeated .grpc.gateway.protoc_gen_openapiv2.options.SecurityRequirement security = 12;
* @param values The security to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("plusAssignAllSecurity")
@Suppress("NOTHING_TO_INLINE")
public inline operator fun com.google.protobuf.kotlin.DslList.plusAssign(values: kotlin.collections.Iterable) {
addAll(values)
}
/**
*
* A declaration of which security schemes are applied for this operation. The
* list of values describes alternative security schemes that can be used
* (that is, there is a logical OR between the security requirements). This
* definition overrides any declared top-level security. To remove a top-level
* security declaration, an empty array can be used.
*
*
* repeated .grpc.gateway.protoc_gen_openapiv2.options.SecurityRequirement security = 12;
* @param index The index to set the value at.
* @param value The security to set.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("setSecurity")
public operator fun com.google.protobuf.kotlin.DslList.set(index: kotlin.Int, value: grpc.gateway.protoc_gen_openapiv2.options.Openapiv2.SecurityRequirement) {
_builder.setSecurity(index, value)
}
/**
*
* A declaration of which security schemes are applied for this operation. The
* list of values describes alternative security schemes that can be used
* (that is, there is a logical OR between the security requirements). This
* definition overrides any declared top-level security. To remove a top-level
* security declaration, an empty array can be used.
*
*
* repeated .grpc.gateway.protoc_gen_openapiv2.options.SecurityRequirement security = 12;
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("clearSecurity")
public fun com.google.protobuf.kotlin.DslList.clear() {
_builder.clearSecurity()
}
/**
* An uninstantiable, behaviorless type to represent the field in
* generics.
*/
@kotlin.OptIn(com.google.protobuf.kotlin.OnlyForUseByGeneratedProtoCode::class)
public class ExtensionsProxy private constructor() : com.google.protobuf.kotlin.DslProxy()
/**
*
* Custom properties that start with "x-" such as "x-foo" used to describe
* extra functionality that is not covered by the standard OpenAPI Specification.
* See: https://swagger.io/docs/specification/2-0/swagger-extensions/
*
*
* map<string, .google.protobuf.Value> extensions = 13;
*/
public val extensions: com.google.protobuf.kotlin.DslMap
@kotlin.jvm.JvmSynthetic
@JvmName("getExtensionsMap")
get() = com.google.protobuf.kotlin.DslMap(
_builder.getExtensionsMap()
)
/**
*
* Custom properties that start with "x-" such as "x-foo" used to describe
* extra functionality that is not covered by the standard OpenAPI Specification.
* See: https://swagger.io/docs/specification/2-0/swagger-extensions/
*
*
* map<string, .google.protobuf.Value> extensions = 13;
*/
@JvmName("putExtensions")
public fun com.google.protobuf.kotlin.DslMap
.put(key: kotlin.String, value: com.google.protobuf.Value) {
_builder.putExtensions(key, value)
}
/**
*
* Custom properties that start with "x-" such as "x-foo" used to describe
* extra functionality that is not covered by the standard OpenAPI Specification.
* See: https://swagger.io/docs/specification/2-0/swagger-extensions/
*
*
* map<string, .google.protobuf.Value> extensions = 13;
*/
@kotlin.jvm.JvmSynthetic
@JvmName("setExtensions")
@Suppress("NOTHING_TO_INLINE")
public inline operator fun com.google.protobuf.kotlin.DslMap
.set(key: kotlin.String, value: com.google.protobuf.Value) {
put(key, value)
}
/**
*
* Custom properties that start with "x-" such as "x-foo" used to describe
* extra functionality that is not covered by the standard OpenAPI Specification.
* See: https://swagger.io/docs/specification/2-0/swagger-extensions/
*
*
* map<string, .google.protobuf.Value> extensions = 13;
*/
@kotlin.jvm.JvmSynthetic
@JvmName("removeExtensions")
public fun com.google.protobuf.kotlin.DslMap
.remove(key: kotlin.String) {
_builder.removeExtensions(key)
}
/**
*
* Custom properties that start with "x-" such as "x-foo" used to describe
* extra functionality that is not covered by the standard OpenAPI Specification.
* See: https://swagger.io/docs/specification/2-0/swagger-extensions/
*
*
* map<string, .google.protobuf.Value> extensions = 13;
*/
@kotlin.jvm.JvmSynthetic
@JvmName("putAllExtensions")
public fun com.google.protobuf.kotlin.DslMap
.putAll(map: kotlin.collections.Map) {
_builder.putAllExtensions(map)
}
/**
*
* Custom properties that start with "x-" such as "x-foo" used to describe
* extra functionality that is not covered by the standard OpenAPI Specification.
* See: https://swagger.io/docs/specification/2-0/swagger-extensions/
*
*
* map<string, .google.protobuf.Value> extensions = 13;
*/
@kotlin.jvm.JvmSynthetic
@JvmName("clearExtensions")
public fun com.google.protobuf.kotlin.DslMap
.clear() {
_builder.clearExtensions()
}
/**
*
* Custom parameters such as HTTP request headers.
* See: https://swagger.io/docs/specification/2-0/describing-parameters/
* and https://swagger.io/specification/v2/#parameter-object.
*
*
* .grpc.gateway.protoc_gen_openapiv2.options.Parameters parameters = 14;
*/
public var parameters: grpc.gateway.protoc_gen_openapiv2.options.Openapiv2.Parameters
@JvmName("getParameters")
get() = _builder.getParameters()
@JvmName("setParameters")
set(value) {
_builder.setParameters(value)
}
/**
*
* Custom parameters such as HTTP request headers.
* See: https://swagger.io/docs/specification/2-0/describing-parameters/
* and https://swagger.io/specification/v2/#parameter-object.
*
*
* .grpc.gateway.protoc_gen_openapiv2.options.Parameters parameters = 14;
*/
public fun clearParameters() {
_builder.clearParameters()
}
/**
*
* Custom parameters such as HTTP request headers.
* See: https://swagger.io/docs/specification/2-0/describing-parameters/
* and https://swagger.io/specification/v2/#parameter-object.
*
*
* .grpc.gateway.protoc_gen_openapiv2.options.Parameters parameters = 14;
* @return Whether the parameters field is set.
*/
public fun hasParameters(): kotlin.Boolean {
return _builder.hasParameters()
}
}
}
@kotlin.jvm.JvmSynthetic
public inline fun grpc.gateway.protoc_gen_openapiv2.options.Openapiv2.Operation.copy(block: grpc.gateway.protoc_gen_openapiv2.options.OperationKt.Dsl.() -> kotlin.Unit): grpc.gateway.protoc_gen_openapiv2.options.Openapiv2.Operation =
grpc.gateway.protoc_gen_openapiv2.options.OperationKt.Dsl._create(this.toBuilder()).apply { block() }._build()
public val grpc.gateway.protoc_gen_openapiv2.options.Openapiv2.OperationOrBuilder.externalDocsOrNull: grpc.gateway.protoc_gen_openapiv2.options.Openapiv2.ExternalDocumentation?
get() = if (hasExternalDocs()) getExternalDocs() else null
public val grpc.gateway.protoc_gen_openapiv2.options.Openapiv2.OperationOrBuilder.parametersOrNull: grpc.gateway.protoc_gen_openapiv2.options.Openapiv2.Parameters?
get() = if (hasParameters()) getParameters() else null
© 2015 - 2025 Weber Informatics LLC | Privacy Policy