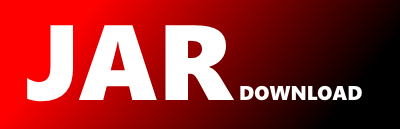
grpc.gateway.protoc_gen_openapiv2.options.SchemaKt.kt Maven / Gradle / Ivy
The newest version!
//Generated by the protocol buffer compiler. DO NOT EDIT!
// source: protoc-gen-openapiv2/options/openapiv2.proto
package grpc.gateway.protoc_gen_openapiv2.options;
@kotlin.jvm.JvmName("-initializeschema")
public inline fun schema(block: grpc.gateway.protoc_gen_openapiv2.options.SchemaKt.Dsl.() -> kotlin.Unit): grpc.gateway.protoc_gen_openapiv2.options.Openapiv2.Schema =
grpc.gateway.protoc_gen_openapiv2.options.SchemaKt.Dsl._create(grpc.gateway.protoc_gen_openapiv2.options.Openapiv2.Schema.newBuilder()).apply { block() }._build()
public object SchemaKt {
@kotlin.OptIn(com.google.protobuf.kotlin.OnlyForUseByGeneratedProtoCode::class)
@com.google.protobuf.kotlin.ProtoDslMarker
public class Dsl private constructor(
private val _builder: grpc.gateway.protoc_gen_openapiv2.options.Openapiv2.Schema.Builder
) {
public companion object {
@kotlin.jvm.JvmSynthetic
@kotlin.PublishedApi
internal fun _create(builder: grpc.gateway.protoc_gen_openapiv2.options.Openapiv2.Schema.Builder): Dsl = Dsl(builder)
}
@kotlin.jvm.JvmSynthetic
@kotlin.PublishedApi
internal fun _build(): grpc.gateway.protoc_gen_openapiv2.options.Openapiv2.Schema = _builder.build()
/**
* .grpc.gateway.protoc_gen_openapiv2.options.JSONSchema json_schema = 1;
*/
public var jsonSchema: grpc.gateway.protoc_gen_openapiv2.options.Openapiv2.JSONSchema
@JvmName("getJsonSchema")
get() = _builder.getJsonSchema()
@JvmName("setJsonSchema")
set(value) {
_builder.setJsonSchema(value)
}
/**
* .grpc.gateway.protoc_gen_openapiv2.options.JSONSchema json_schema = 1;
*/
public fun clearJsonSchema() {
_builder.clearJsonSchema()
}
/**
* .grpc.gateway.protoc_gen_openapiv2.options.JSONSchema json_schema = 1;
* @return Whether the jsonSchema field is set.
*/
public fun hasJsonSchema(): kotlin.Boolean {
return _builder.hasJsonSchema()
}
/**
*
* Adds support for polymorphism. The discriminator is the schema property
* name that is used to differentiate between other schema that inherit this
* schema. The property name used MUST be defined at this schema and it MUST
* be in the required property list. When used, the value MUST be the name of
* this schema or any schema that inherits it.
*
*
* string discriminator = 2;
*/
public var discriminator: kotlin.String
@JvmName("getDiscriminator")
get() = _builder.getDiscriminator()
@JvmName("setDiscriminator")
set(value) {
_builder.setDiscriminator(value)
}
/**
*
* Adds support for polymorphism. The discriminator is the schema property
* name that is used to differentiate between other schema that inherit this
* schema. The property name used MUST be defined at this schema and it MUST
* be in the required property list. When used, the value MUST be the name of
* this schema or any schema that inherits it.
*
*
* string discriminator = 2;
*/
public fun clearDiscriminator() {
_builder.clearDiscriminator()
}
/**
*
* Relevant only for Schema "properties" definitions. Declares the property as
* "read only". This means that it MAY be sent as part of a response but MUST
* NOT be sent as part of the request. Properties marked as readOnly being
* true SHOULD NOT be in the required list of the defined schema. Default
* value is false.
*
*
* bool read_only = 3;
*/
public var readOnly: kotlin.Boolean
@JvmName("getReadOnly")
get() = _builder.getReadOnly()
@JvmName("setReadOnly")
set(value) {
_builder.setReadOnly(value)
}
/**
*
* Relevant only for Schema "properties" definitions. Declares the property as
* "read only". This means that it MAY be sent as part of a response but MUST
* NOT be sent as part of the request. Properties marked as readOnly being
* true SHOULD NOT be in the required list of the defined schema. Default
* value is false.
*
*
* bool read_only = 3;
*/
public fun clearReadOnly() {
_builder.clearReadOnly()
}
/**
*
* Additional external documentation for this schema.
*
*
* .grpc.gateway.protoc_gen_openapiv2.options.ExternalDocumentation external_docs = 5;
*/
public var externalDocs: grpc.gateway.protoc_gen_openapiv2.options.Openapiv2.ExternalDocumentation
@JvmName("getExternalDocs")
get() = _builder.getExternalDocs()
@JvmName("setExternalDocs")
set(value) {
_builder.setExternalDocs(value)
}
/**
*
* Additional external documentation for this schema.
*
*
* .grpc.gateway.protoc_gen_openapiv2.options.ExternalDocumentation external_docs = 5;
*/
public fun clearExternalDocs() {
_builder.clearExternalDocs()
}
/**
*
* Additional external documentation for this schema.
*
*
* .grpc.gateway.protoc_gen_openapiv2.options.ExternalDocumentation external_docs = 5;
* @return Whether the externalDocs field is set.
*/
public fun hasExternalDocs(): kotlin.Boolean {
return _builder.hasExternalDocs()
}
/**
*
* A free-form property to include an example of an instance for this schema in JSON.
* This is copied verbatim to the output.
*
*
* string example = 6;
*/
public var example: kotlin.String
@JvmName("getExample")
get() = _builder.getExample()
@JvmName("setExample")
set(value) {
_builder.setExample(value)
}
/**
*
* A free-form property to include an example of an instance for this schema in JSON.
* This is copied verbatim to the output.
*
*
* string example = 6;
*/
public fun clearExample() {
_builder.clearExample()
}
}
}
@kotlin.jvm.JvmSynthetic
public inline fun grpc.gateway.protoc_gen_openapiv2.options.Openapiv2.Schema.copy(block: grpc.gateway.protoc_gen_openapiv2.options.SchemaKt.Dsl.() -> kotlin.Unit): grpc.gateway.protoc_gen_openapiv2.options.Openapiv2.Schema =
grpc.gateway.protoc_gen_openapiv2.options.SchemaKt.Dsl._create(this.toBuilder()).apply { block() }._build()
public val grpc.gateway.protoc_gen_openapiv2.options.Openapiv2.SchemaOrBuilder.jsonSchemaOrNull: grpc.gateway.protoc_gen_openapiv2.options.Openapiv2.JSONSchema?
get() = if (hasJsonSchema()) getJsonSchema() else null
public val grpc.gateway.protoc_gen_openapiv2.options.Openapiv2.SchemaOrBuilder.externalDocsOrNull: grpc.gateway.protoc_gen_openapiv2.options.Openapiv2.ExternalDocumentation?
get() = if (hasExternalDocs()) getExternalDocs() else null
© 2015 - 2025 Weber Informatics LLC | Privacy Policy