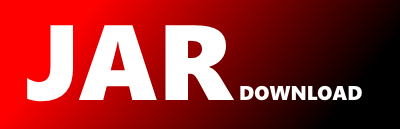
io.envoyproxy.pgv.validate.AnyRulesKt.kt Maven / Gradle / Ivy
The newest version!
//Generated by the protocol buffer compiler. DO NOT EDIT!
// source: validate/validate.proto
package io.envoyproxy.pgv.validate;
@kotlin.jvm.JvmName("-initializeanyRules")
public inline fun anyRules(block: io.envoyproxy.pgv.validate.AnyRulesKt.Dsl.() -> kotlin.Unit): io.envoyproxy.pgv.validate.Validate.AnyRules =
io.envoyproxy.pgv.validate.AnyRulesKt.Dsl._create(io.envoyproxy.pgv.validate.Validate.AnyRules.newBuilder()).apply { block() }._build()
public object AnyRulesKt {
@kotlin.OptIn(com.google.protobuf.kotlin.OnlyForUseByGeneratedProtoCode::class)
@com.google.protobuf.kotlin.ProtoDslMarker
public class Dsl private constructor(
private val _builder: io.envoyproxy.pgv.validate.Validate.AnyRules.Builder
) {
public companion object {
@kotlin.jvm.JvmSynthetic
@kotlin.PublishedApi
internal fun _create(builder: io.envoyproxy.pgv.validate.Validate.AnyRules.Builder): Dsl = Dsl(builder)
}
@kotlin.jvm.JvmSynthetic
@kotlin.PublishedApi
internal fun _build(): io.envoyproxy.pgv.validate.Validate.AnyRules = _builder.build()
/**
*
* Required specifies that this field must be set
*
*
* optional bool required = 1;
*/
public var required: kotlin.Boolean
@JvmName("getRequired")
get() = _builder.getRequired()
@JvmName("setRequired")
set(value) {
_builder.setRequired(value)
}
/**
*
* Required specifies that this field must be set
*
*
* optional bool required = 1;
*/
public fun clearRequired() {
_builder.clearRequired()
}
/**
*
* Required specifies that this field must be set
*
*
* optional bool required = 1;
* @return Whether the required field is set.
*/
public fun hasRequired(): kotlin.Boolean {
return _builder.hasRequired()
}
/**
* An uninstantiable, behaviorless type to represent the field in
* generics.
*/
@kotlin.OptIn(com.google.protobuf.kotlin.OnlyForUseByGeneratedProtoCode::class)
public class In_Proxy private constructor() : com.google.protobuf.kotlin.DslProxy()
/**
*
* In specifies that this field's `type_url` must be equal to one of the
* specified values.
*
*
* repeated string in = 2;
* @return A list containing the in.
*/
public val in_: com.google.protobuf.kotlin.DslList
@kotlin.jvm.JvmSynthetic
get() = com.google.protobuf.kotlin.DslList(
_builder.getInList()
)
/**
*
* In specifies that this field's `type_url` must be equal to one of the
* specified values.
*
*
* repeated string in = 2;
* @param value The in to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("addIn_")
public fun com.google.protobuf.kotlin.DslList.add(value: kotlin.String) {
_builder.addIn(value)
}
/**
*
* In specifies that this field's `type_url` must be equal to one of the
* specified values.
*
*
* repeated string in = 2;
* @param value The in to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("plusAssignIn_")
@Suppress("NOTHING_TO_INLINE")
public inline operator fun com.google.protobuf.kotlin.DslList.plusAssign(value: kotlin.String) {
add(value)
}
/**
*
* In specifies that this field's `type_url` must be equal to one of the
* specified values.
*
*
* repeated string in = 2;
* @param values The in to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("addAllIn_")
public fun com.google.protobuf.kotlin.DslList.addAll(values: kotlin.collections.Iterable) {
_builder.addAllIn(values)
}
/**
*
* In specifies that this field's `type_url` must be equal to one of the
* specified values.
*
*
* repeated string in = 2;
* @param values The in to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("plusAssignAllIn_")
@Suppress("NOTHING_TO_INLINE")
public inline operator fun com.google.protobuf.kotlin.DslList.plusAssign(values: kotlin.collections.Iterable) {
addAll(values)
}
/**
*
* In specifies that this field's `type_url` must be equal to one of the
* specified values.
*
*
* repeated string in = 2;
* @param index The index to set the value at.
* @param value The in to set.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("setIn_")
public operator fun com.google.protobuf.kotlin.DslList.set(index: kotlin.Int, value: kotlin.String) {
_builder.setIn(index, value)
}/**
*
* In specifies that this field's `type_url` must be equal to one of the
* specified values.
*
*
* repeated string in = 2;
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("clearIn_")
public fun com.google.protobuf.kotlin.DslList.clear() {
_builder.clearIn()
}
/**
* An uninstantiable, behaviorless type to represent the field in
* generics.
*/
@kotlin.OptIn(com.google.protobuf.kotlin.OnlyForUseByGeneratedProtoCode::class)
public class NotInProxy private constructor() : com.google.protobuf.kotlin.DslProxy()
/**
*
* NotIn specifies that this field's `type_url` must not be equal to any of
* the specified values.
*
*
* repeated string not_in = 3;
* @return A list containing the notIn.
*/
public val notIn: com.google.protobuf.kotlin.DslList
@kotlin.jvm.JvmSynthetic
get() = com.google.protobuf.kotlin.DslList(
_builder.getNotInList()
)
/**
*
* NotIn specifies that this field's `type_url` must not be equal to any of
* the specified values.
*
*
* repeated string not_in = 3;
* @param value The notIn to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("addNotIn")
public fun com.google.protobuf.kotlin.DslList.add(value: kotlin.String) {
_builder.addNotIn(value)
}
/**
*
* NotIn specifies that this field's `type_url` must not be equal to any of
* the specified values.
*
*
* repeated string not_in = 3;
* @param value The notIn to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("plusAssignNotIn")
@Suppress("NOTHING_TO_INLINE")
public inline operator fun com.google.protobuf.kotlin.DslList.plusAssign(value: kotlin.String) {
add(value)
}
/**
*
* NotIn specifies that this field's `type_url` must not be equal to any of
* the specified values.
*
*
* repeated string not_in = 3;
* @param values The notIn to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("addAllNotIn")
public fun com.google.protobuf.kotlin.DslList.addAll(values: kotlin.collections.Iterable) {
_builder.addAllNotIn(values)
}
/**
*
* NotIn specifies that this field's `type_url` must not be equal to any of
* the specified values.
*
*
* repeated string not_in = 3;
* @param values The notIn to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("plusAssignAllNotIn")
@Suppress("NOTHING_TO_INLINE")
public inline operator fun com.google.protobuf.kotlin.DslList.plusAssign(values: kotlin.collections.Iterable) {
addAll(values)
}
/**
*
* NotIn specifies that this field's `type_url` must not be equal to any of
* the specified values.
*
*
* repeated string not_in = 3;
* @param index The index to set the value at.
* @param value The notIn to set.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("setNotIn")
public operator fun com.google.protobuf.kotlin.DslList.set(index: kotlin.Int, value: kotlin.String) {
_builder.setNotIn(index, value)
}/**
*
* NotIn specifies that this field's `type_url` must not be equal to any of
* the specified values.
*
*
* repeated string not_in = 3;
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("clearNotIn")
public fun com.google.protobuf.kotlin.DslList.clear() {
_builder.clearNotIn()
}}
}
@kotlin.jvm.JvmSynthetic
public inline fun io.envoyproxy.pgv.validate.Validate.AnyRules.copy(block: io.envoyproxy.pgv.validate.AnyRulesKt.Dsl.() -> kotlin.Unit): io.envoyproxy.pgv.validate.Validate.AnyRules =
io.envoyproxy.pgv.validate.AnyRulesKt.Dsl._create(this.toBuilder()).apply { block() }._build()
© 2015 - 2025 Weber Informatics LLC | Privacy Policy