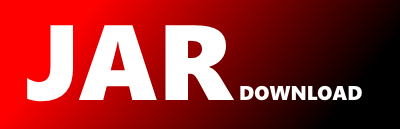
io.envoyproxy.pgv.validate.MapRulesKt.kt Maven / Gradle / Ivy
The newest version!
//Generated by the protocol buffer compiler. DO NOT EDIT!
// source: validate/validate.proto
package io.envoyproxy.pgv.validate;
@kotlin.jvm.JvmName("-initializemapRules")
public inline fun mapRules(block: io.envoyproxy.pgv.validate.MapRulesKt.Dsl.() -> kotlin.Unit): io.envoyproxy.pgv.validate.Validate.MapRules =
io.envoyproxy.pgv.validate.MapRulesKt.Dsl._create(io.envoyproxy.pgv.validate.Validate.MapRules.newBuilder()).apply { block() }._build()
public object MapRulesKt {
@kotlin.OptIn(com.google.protobuf.kotlin.OnlyForUseByGeneratedProtoCode::class)
@com.google.protobuf.kotlin.ProtoDslMarker
public class Dsl private constructor(
private val _builder: io.envoyproxy.pgv.validate.Validate.MapRules.Builder
) {
public companion object {
@kotlin.jvm.JvmSynthetic
@kotlin.PublishedApi
internal fun _create(builder: io.envoyproxy.pgv.validate.Validate.MapRules.Builder): Dsl = Dsl(builder)
}
@kotlin.jvm.JvmSynthetic
@kotlin.PublishedApi
internal fun _build(): io.envoyproxy.pgv.validate.Validate.MapRules = _builder.build()
/**
*
* MinPairs specifies that this field must have the specified number of
* KVs at a minimum
*
*
* optional uint64 min_pairs = 1;
*/
public var minPairs: kotlin.Long
@JvmName("getMinPairs")
get() = _builder.getMinPairs()
@JvmName("setMinPairs")
set(value) {
_builder.setMinPairs(value)
}
/**
*
* MinPairs specifies that this field must have the specified number of
* KVs at a minimum
*
*
* optional uint64 min_pairs = 1;
*/
public fun clearMinPairs() {
_builder.clearMinPairs()
}
/**
*
* MinPairs specifies that this field must have the specified number of
* KVs at a minimum
*
*
* optional uint64 min_pairs = 1;
* @return Whether the minPairs field is set.
*/
public fun hasMinPairs(): kotlin.Boolean {
return _builder.hasMinPairs()
}
/**
*
* MaxPairs specifies that this field must have the specified number of
* KVs at a maximum
*
*
* optional uint64 max_pairs = 2;
*/
public var maxPairs: kotlin.Long
@JvmName("getMaxPairs")
get() = _builder.getMaxPairs()
@JvmName("setMaxPairs")
set(value) {
_builder.setMaxPairs(value)
}
/**
*
* MaxPairs specifies that this field must have the specified number of
* KVs at a maximum
*
*
* optional uint64 max_pairs = 2;
*/
public fun clearMaxPairs() {
_builder.clearMaxPairs()
}
/**
*
* MaxPairs specifies that this field must have the specified number of
* KVs at a maximum
*
*
* optional uint64 max_pairs = 2;
* @return Whether the maxPairs field is set.
*/
public fun hasMaxPairs(): kotlin.Boolean {
return _builder.hasMaxPairs()
}
/**
*
* NoSparse specifies values in this field cannot be unset. This only
* applies to map's with message value types.
*
*
* optional bool no_sparse = 3;
*/
public var noSparse: kotlin.Boolean
@JvmName("getNoSparse")
get() = _builder.getNoSparse()
@JvmName("setNoSparse")
set(value) {
_builder.setNoSparse(value)
}
/**
*
* NoSparse specifies values in this field cannot be unset. This only
* applies to map's with message value types.
*
*
* optional bool no_sparse = 3;
*/
public fun clearNoSparse() {
_builder.clearNoSparse()
}
/**
*
* NoSparse specifies values in this field cannot be unset. This only
* applies to map's with message value types.
*
*
* optional bool no_sparse = 3;
* @return Whether the noSparse field is set.
*/
public fun hasNoSparse(): kotlin.Boolean {
return _builder.hasNoSparse()
}
/**
*
* Keys specifies the constraints to be applied to each key in the field.
*
*
* optional .validate.FieldRules keys = 4;
*/
public var keys: io.envoyproxy.pgv.validate.Validate.FieldRules
@JvmName("getKeys")
get() = _builder.getKeys()
@JvmName("setKeys")
set(value) {
_builder.setKeys(value)
}
/**
*
* Keys specifies the constraints to be applied to each key in the field.
*
*
* optional .validate.FieldRules keys = 4;
*/
public fun clearKeys() {
_builder.clearKeys()
}
/**
*
* Keys specifies the constraints to be applied to each key in the field.
*
*
* optional .validate.FieldRules keys = 4;
* @return Whether the keys field is set.
*/
public fun hasKeys(): kotlin.Boolean {
return _builder.hasKeys()
}
public val MapRulesKt.Dsl.keysOrNull: io.envoyproxy.pgv.validate.Validate.FieldRules?
get() = _builder.keysOrNull
/**
*
* Values specifies the constraints to be applied to the value of each key
* in the field. Message values will still have their validations evaluated
* unless skip is specified here.
*
*
* optional .validate.FieldRules values = 5;
*/
public var values: io.envoyproxy.pgv.validate.Validate.FieldRules
@JvmName("getValues")
get() = _builder.getValues()
@JvmName("setValues")
set(value) {
_builder.setValues(value)
}
/**
*
* Values specifies the constraints to be applied to the value of each key
* in the field. Message values will still have their validations evaluated
* unless skip is specified here.
*
*
* optional .validate.FieldRules values = 5;
*/
public fun clearValues() {
_builder.clearValues()
}
/**
*
* Values specifies the constraints to be applied to the value of each key
* in the field. Message values will still have their validations evaluated
* unless skip is specified here.
*
*
* optional .validate.FieldRules values = 5;
* @return Whether the values field is set.
*/
public fun hasValues(): kotlin.Boolean {
return _builder.hasValues()
}
public val MapRulesKt.Dsl.valuesOrNull: io.envoyproxy.pgv.validate.Validate.FieldRules?
get() = _builder.valuesOrNull
/**
*
* IgnoreEmpty specifies that the validation rules of this field should be
* evaluated only if the field is not empty
*
*
* optional bool ignore_empty = 6;
*/
public var ignoreEmpty: kotlin.Boolean
@JvmName("getIgnoreEmpty")
get() = _builder.getIgnoreEmpty()
@JvmName("setIgnoreEmpty")
set(value) {
_builder.setIgnoreEmpty(value)
}
/**
*
* IgnoreEmpty specifies that the validation rules of this field should be
* evaluated only if the field is not empty
*
*
* optional bool ignore_empty = 6;
*/
public fun clearIgnoreEmpty() {
_builder.clearIgnoreEmpty()
}
/**
*
* IgnoreEmpty specifies that the validation rules of this field should be
* evaluated only if the field is not empty
*
*
* optional bool ignore_empty = 6;
* @return Whether the ignoreEmpty field is set.
*/
public fun hasIgnoreEmpty(): kotlin.Boolean {
return _builder.hasIgnoreEmpty()
}
}
}
@kotlin.jvm.JvmSynthetic
public inline fun io.envoyproxy.pgv.validate.Validate.MapRules.copy(block: io.envoyproxy.pgv.validate.MapRulesKt.Dsl.() -> kotlin.Unit): io.envoyproxy.pgv.validate.Validate.MapRules =
io.envoyproxy.pgv.validate.MapRulesKt.Dsl._create(this.toBuilder()).apply { block() }._build()
public val io.envoyproxy.pgv.validate.Validate.MapRulesOrBuilder.keysOrNull: io.envoyproxy.pgv.validate.Validate.FieldRules?
get() = if (hasKeys()) getKeys() else null
public val io.envoyproxy.pgv.validate.Validate.MapRulesOrBuilder.valuesOrNull: io.envoyproxy.pgv.validate.Validate.FieldRules?
get() = if (hasValues()) getValues() else null
© 2015 - 2025 Weber Informatics LLC | Privacy Policy