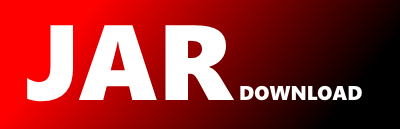
io.envoyproxy.pgv.validate.RepeatedRulesKt.kt Maven / Gradle / Ivy
The newest version!
//Generated by the protocol buffer compiler. DO NOT EDIT!
// source: validate/validate.proto
package io.envoyproxy.pgv.validate;
@kotlin.jvm.JvmName("-initializerepeatedRules")
public inline fun repeatedRules(block: io.envoyproxy.pgv.validate.RepeatedRulesKt.Dsl.() -> kotlin.Unit): io.envoyproxy.pgv.validate.Validate.RepeatedRules =
io.envoyproxy.pgv.validate.RepeatedRulesKt.Dsl._create(io.envoyproxy.pgv.validate.Validate.RepeatedRules.newBuilder()).apply { block() }._build()
public object RepeatedRulesKt {
@kotlin.OptIn(com.google.protobuf.kotlin.OnlyForUseByGeneratedProtoCode::class)
@com.google.protobuf.kotlin.ProtoDslMarker
public class Dsl private constructor(
private val _builder: io.envoyproxy.pgv.validate.Validate.RepeatedRules.Builder
) {
public companion object {
@kotlin.jvm.JvmSynthetic
@kotlin.PublishedApi
internal fun _create(builder: io.envoyproxy.pgv.validate.Validate.RepeatedRules.Builder): Dsl = Dsl(builder)
}
@kotlin.jvm.JvmSynthetic
@kotlin.PublishedApi
internal fun _build(): io.envoyproxy.pgv.validate.Validate.RepeatedRules = _builder.build()
/**
*
* MinItems specifies that this field must have the specified number of
* items at a minimum
*
*
* optional uint64 min_items = 1;
*/
public var minItems: kotlin.Long
@JvmName("getMinItems")
get() = _builder.getMinItems()
@JvmName("setMinItems")
set(value) {
_builder.setMinItems(value)
}
/**
*
* MinItems specifies that this field must have the specified number of
* items at a minimum
*
*
* optional uint64 min_items = 1;
*/
public fun clearMinItems() {
_builder.clearMinItems()
}
/**
*
* MinItems specifies that this field must have the specified number of
* items at a minimum
*
*
* optional uint64 min_items = 1;
* @return Whether the minItems field is set.
*/
public fun hasMinItems(): kotlin.Boolean {
return _builder.hasMinItems()
}
/**
*
* MaxItems specifies that this field must have the specified number of
* items at a maximum
*
*
* optional uint64 max_items = 2;
*/
public var maxItems: kotlin.Long
@JvmName("getMaxItems")
get() = _builder.getMaxItems()
@JvmName("setMaxItems")
set(value) {
_builder.setMaxItems(value)
}
/**
*
* MaxItems specifies that this field must have the specified number of
* items at a maximum
*
*
* optional uint64 max_items = 2;
*/
public fun clearMaxItems() {
_builder.clearMaxItems()
}
/**
*
* MaxItems specifies that this field must have the specified number of
* items at a maximum
*
*
* optional uint64 max_items = 2;
* @return Whether the maxItems field is set.
*/
public fun hasMaxItems(): kotlin.Boolean {
return _builder.hasMaxItems()
}
/**
*
* Unique specifies that all elements in this field must be unique. This
* contraint is only applicable to scalar and enum types (messages are not
* supported).
*
*
* optional bool unique = 3;
*/
public var unique: kotlin.Boolean
@JvmName("getUnique")
get() = _builder.getUnique()
@JvmName("setUnique")
set(value) {
_builder.setUnique(value)
}
/**
*
* Unique specifies that all elements in this field must be unique. This
* contraint is only applicable to scalar and enum types (messages are not
* supported).
*
*
* optional bool unique = 3;
*/
public fun clearUnique() {
_builder.clearUnique()
}
/**
*
* Unique specifies that all elements in this field must be unique. This
* contraint is only applicable to scalar and enum types (messages are not
* supported).
*
*
* optional bool unique = 3;
* @return Whether the unique field is set.
*/
public fun hasUnique(): kotlin.Boolean {
return _builder.hasUnique()
}
/**
*
* Items specifies the contraints to be applied to each item in the field.
* Repeated message fields will still execute validation against each item
* unless skip is specified here.
*
*
* optional .validate.FieldRules items = 4;
*/
public var items: io.envoyproxy.pgv.validate.Validate.FieldRules
@JvmName("getItems")
get() = _builder.getItems()
@JvmName("setItems")
set(value) {
_builder.setItems(value)
}
/**
*
* Items specifies the contraints to be applied to each item in the field.
* Repeated message fields will still execute validation against each item
* unless skip is specified here.
*
*
* optional .validate.FieldRules items = 4;
*/
public fun clearItems() {
_builder.clearItems()
}
/**
*
* Items specifies the contraints to be applied to each item in the field.
* Repeated message fields will still execute validation against each item
* unless skip is specified here.
*
*
* optional .validate.FieldRules items = 4;
* @return Whether the items field is set.
*/
public fun hasItems(): kotlin.Boolean {
return _builder.hasItems()
}
public val RepeatedRulesKt.Dsl.itemsOrNull: io.envoyproxy.pgv.validate.Validate.FieldRules?
get() = _builder.itemsOrNull
/**
*
* IgnoreEmpty specifies that the validation rules of this field should be
* evaluated only if the field is not empty
*
*
* optional bool ignore_empty = 5;
*/
public var ignoreEmpty: kotlin.Boolean
@JvmName("getIgnoreEmpty")
get() = _builder.getIgnoreEmpty()
@JvmName("setIgnoreEmpty")
set(value) {
_builder.setIgnoreEmpty(value)
}
/**
*
* IgnoreEmpty specifies that the validation rules of this field should be
* evaluated only if the field is not empty
*
*
* optional bool ignore_empty = 5;
*/
public fun clearIgnoreEmpty() {
_builder.clearIgnoreEmpty()
}
/**
*
* IgnoreEmpty specifies that the validation rules of this field should be
* evaluated only if the field is not empty
*
*
* optional bool ignore_empty = 5;
* @return Whether the ignoreEmpty field is set.
*/
public fun hasIgnoreEmpty(): kotlin.Boolean {
return _builder.hasIgnoreEmpty()
}
}
}
@kotlin.jvm.JvmSynthetic
public inline fun io.envoyproxy.pgv.validate.Validate.RepeatedRules.copy(block: io.envoyproxy.pgv.validate.RepeatedRulesKt.Dsl.() -> kotlin.Unit): io.envoyproxy.pgv.validate.Validate.RepeatedRules =
io.envoyproxy.pgv.validate.RepeatedRulesKt.Dsl._create(this.toBuilder()).apply { block() }._build()
public val io.envoyproxy.pgv.validate.Validate.RepeatedRulesOrBuilder.itemsOrNull: io.envoyproxy.pgv.validate.Validate.FieldRules?
get() = if (hasItems()) getItems() else null
© 2015 - 2025 Weber Informatics LLC | Privacy Policy