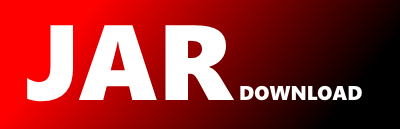
io.envoyproxy.pgv.validate.SFixed32RulesKt.kt Maven / Gradle / Ivy
The newest version!
//Generated by the protocol buffer compiler. DO NOT EDIT!
// source: validate/validate.proto
package io.envoyproxy.pgv.validate;
@kotlin.jvm.JvmName("-initializesFixed32Rules")
public inline fun sFixed32Rules(block: io.envoyproxy.pgv.validate.SFixed32RulesKt.Dsl.() -> kotlin.Unit): io.envoyproxy.pgv.validate.Validate.SFixed32Rules =
io.envoyproxy.pgv.validate.SFixed32RulesKt.Dsl._create(io.envoyproxy.pgv.validate.Validate.SFixed32Rules.newBuilder()).apply { block() }._build()
public object SFixed32RulesKt {
@kotlin.OptIn(com.google.protobuf.kotlin.OnlyForUseByGeneratedProtoCode::class)
@com.google.protobuf.kotlin.ProtoDslMarker
public class Dsl private constructor(
private val _builder: io.envoyproxy.pgv.validate.Validate.SFixed32Rules.Builder
) {
public companion object {
@kotlin.jvm.JvmSynthetic
@kotlin.PublishedApi
internal fun _create(builder: io.envoyproxy.pgv.validate.Validate.SFixed32Rules.Builder): Dsl = Dsl(builder)
}
@kotlin.jvm.JvmSynthetic
@kotlin.PublishedApi
internal fun _build(): io.envoyproxy.pgv.validate.Validate.SFixed32Rules = _builder.build()
/**
*
* Const specifies that this field must be exactly the specified value
*
*
* optional sfixed32 const = 1;
*/
public var const: kotlin.Int
@JvmName("getConst")
get() = _builder.getConst()
@JvmName("setConst")
set(value) {
_builder.setConst(value)
}
/**
*
* Const specifies that this field must be exactly the specified value
*
*
* optional sfixed32 const = 1;
*/
public fun clearConst() {
_builder.clearConst()
}
/**
*
* Const specifies that this field must be exactly the specified value
*
*
* optional sfixed32 const = 1;
* @return Whether the const field is set.
*/
public fun hasConst(): kotlin.Boolean {
return _builder.hasConst()
}
/**
*
* Lt specifies that this field must be less than the specified value,
* exclusive
*
*
* optional sfixed32 lt = 2;
*/
public var lt: kotlin.Int
@JvmName("getLt")
get() = _builder.getLt()
@JvmName("setLt")
set(value) {
_builder.setLt(value)
}
/**
*
* Lt specifies that this field must be less than the specified value,
* exclusive
*
*
* optional sfixed32 lt = 2;
*/
public fun clearLt() {
_builder.clearLt()
}
/**
*
* Lt specifies that this field must be less than the specified value,
* exclusive
*
*
* optional sfixed32 lt = 2;
* @return Whether the lt field is set.
*/
public fun hasLt(): kotlin.Boolean {
return _builder.hasLt()
}
/**
*
* Lte specifies that this field must be less than or equal to the
* specified value, inclusive
*
*
* optional sfixed32 lte = 3;
*/
public var lte: kotlin.Int
@JvmName("getLte")
get() = _builder.getLte()
@JvmName("setLte")
set(value) {
_builder.setLte(value)
}
/**
*
* Lte specifies that this field must be less than or equal to the
* specified value, inclusive
*
*
* optional sfixed32 lte = 3;
*/
public fun clearLte() {
_builder.clearLte()
}
/**
*
* Lte specifies that this field must be less than or equal to the
* specified value, inclusive
*
*
* optional sfixed32 lte = 3;
* @return Whether the lte field is set.
*/
public fun hasLte(): kotlin.Boolean {
return _builder.hasLte()
}
/**
*
* Gt specifies that this field must be greater than the specified value,
* exclusive. If the value of Gt is larger than a specified Lt or Lte, the
* range is reversed.
*
*
* optional sfixed32 gt = 4;
*/
public var gt: kotlin.Int
@JvmName("getGt")
get() = _builder.getGt()
@JvmName("setGt")
set(value) {
_builder.setGt(value)
}
/**
*
* Gt specifies that this field must be greater than the specified value,
* exclusive. If the value of Gt is larger than a specified Lt or Lte, the
* range is reversed.
*
*
* optional sfixed32 gt = 4;
*/
public fun clearGt() {
_builder.clearGt()
}
/**
*
* Gt specifies that this field must be greater than the specified value,
* exclusive. If the value of Gt is larger than a specified Lt or Lte, the
* range is reversed.
*
*
* optional sfixed32 gt = 4;
* @return Whether the gt field is set.
*/
public fun hasGt(): kotlin.Boolean {
return _builder.hasGt()
}
/**
*
* Gte specifies that this field must be greater than or equal to the
* specified value, inclusive. If the value of Gte is larger than a
* specified Lt or Lte, the range is reversed.
*
*
* optional sfixed32 gte = 5;
*/
public var gte: kotlin.Int
@JvmName("getGte")
get() = _builder.getGte()
@JvmName("setGte")
set(value) {
_builder.setGte(value)
}
/**
*
* Gte specifies that this field must be greater than or equal to the
* specified value, inclusive. If the value of Gte is larger than a
* specified Lt or Lte, the range is reversed.
*
*
* optional sfixed32 gte = 5;
*/
public fun clearGte() {
_builder.clearGte()
}
/**
*
* Gte specifies that this field must be greater than or equal to the
* specified value, inclusive. If the value of Gte is larger than a
* specified Lt or Lte, the range is reversed.
*
*
* optional sfixed32 gte = 5;
* @return Whether the gte field is set.
*/
public fun hasGte(): kotlin.Boolean {
return _builder.hasGte()
}
/**
* An uninstantiable, behaviorless type to represent the field in
* generics.
*/
@kotlin.OptIn(com.google.protobuf.kotlin.OnlyForUseByGeneratedProtoCode::class)
public class In_Proxy private constructor() : com.google.protobuf.kotlin.DslProxy()
/**
*
* In specifies that this field must be equal to one of the specified
* values
*
*
* repeated sfixed32 in = 6;
*/
public val in_: com.google.protobuf.kotlin.DslList
@kotlin.jvm.JvmSynthetic
get() = com.google.protobuf.kotlin.DslList(
_builder.getInList()
)
/**
*
* In specifies that this field must be equal to one of the specified
* values
*
*
* repeated sfixed32 in = 6;
* @param value The in to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("addIn_")
public fun com.google.protobuf.kotlin.DslList.add(value: kotlin.Int) {
_builder.addIn(value)
}/**
*
* In specifies that this field must be equal to one of the specified
* values
*
*
* repeated sfixed32 in = 6;
* @param value The in to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("plusAssignIn_")
@Suppress("NOTHING_TO_INLINE")
public inline operator fun com.google.protobuf.kotlin.DslList.plusAssign(value: kotlin.Int) {
add(value)
}/**
*
* In specifies that this field must be equal to one of the specified
* values
*
*
* repeated sfixed32 in = 6;
* @param values The in to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("addAllIn_")
public fun com.google.protobuf.kotlin.DslList.addAll(values: kotlin.collections.Iterable) {
_builder.addAllIn(values)
}/**
*
* In specifies that this field must be equal to one of the specified
* values
*
*
* repeated sfixed32 in = 6;
* @param values The in to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("plusAssignAllIn_")
@Suppress("NOTHING_TO_INLINE")
public inline operator fun com.google.protobuf.kotlin.DslList.plusAssign(values: kotlin.collections.Iterable) {
addAll(values)
}/**
*
* In specifies that this field must be equal to one of the specified
* values
*
*
* repeated sfixed32 in = 6;
* @param index The index to set the value at.
* @param value The in to set.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("setIn_")
public operator fun com.google.protobuf.kotlin.DslList.set(index: kotlin.Int, value: kotlin.Int) {
_builder.setIn(index, value)
}/**
*
* In specifies that this field must be equal to one of the specified
* values
*
*
* repeated sfixed32 in = 6;
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("clearIn_")
public fun com.google.protobuf.kotlin.DslList.clear() {
_builder.clearIn()
}
/**
* An uninstantiable, behaviorless type to represent the field in
* generics.
*/
@kotlin.OptIn(com.google.protobuf.kotlin.OnlyForUseByGeneratedProtoCode::class)
public class NotInProxy private constructor() : com.google.protobuf.kotlin.DslProxy()
/**
*
* NotIn specifies that this field cannot be equal to one of the specified
* values
*
*
* repeated sfixed32 not_in = 7;
*/
public val notIn: com.google.protobuf.kotlin.DslList
@kotlin.jvm.JvmSynthetic
get() = com.google.protobuf.kotlin.DslList(
_builder.getNotInList()
)
/**
*
* NotIn specifies that this field cannot be equal to one of the specified
* values
*
*
* repeated sfixed32 not_in = 7;
* @param value The notIn to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("addNotIn")
public fun com.google.protobuf.kotlin.DslList.add(value: kotlin.Int) {
_builder.addNotIn(value)
}/**
*
* NotIn specifies that this field cannot be equal to one of the specified
* values
*
*
* repeated sfixed32 not_in = 7;
* @param value The notIn to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("plusAssignNotIn")
@Suppress("NOTHING_TO_INLINE")
public inline operator fun com.google.protobuf.kotlin.DslList.plusAssign(value: kotlin.Int) {
add(value)
}/**
*
* NotIn specifies that this field cannot be equal to one of the specified
* values
*
*
* repeated sfixed32 not_in = 7;
* @param values The notIn to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("addAllNotIn")
public fun com.google.protobuf.kotlin.DslList.addAll(values: kotlin.collections.Iterable) {
_builder.addAllNotIn(values)
}/**
*
* NotIn specifies that this field cannot be equal to one of the specified
* values
*
*
* repeated sfixed32 not_in = 7;
* @param values The notIn to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("plusAssignAllNotIn")
@Suppress("NOTHING_TO_INLINE")
public inline operator fun com.google.protobuf.kotlin.DslList.plusAssign(values: kotlin.collections.Iterable) {
addAll(values)
}/**
*
* NotIn specifies that this field cannot be equal to one of the specified
* values
*
*
* repeated sfixed32 not_in = 7;
* @param index The index to set the value at.
* @param value The notIn to set.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("setNotIn")
public operator fun com.google.protobuf.kotlin.DslList.set(index: kotlin.Int, value: kotlin.Int) {
_builder.setNotIn(index, value)
}/**
*
* NotIn specifies that this field cannot be equal to one of the specified
* values
*
*
* repeated sfixed32 not_in = 7;
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("clearNotIn")
public fun com.google.protobuf.kotlin.DslList.clear() {
_builder.clearNotIn()
}
/**
*
* IgnoreEmpty specifies that the validation rules of this field should be
* evaluated only if the field is not empty
*
*
* optional bool ignore_empty = 8;
*/
public var ignoreEmpty: kotlin.Boolean
@JvmName("getIgnoreEmpty")
get() = _builder.getIgnoreEmpty()
@JvmName("setIgnoreEmpty")
set(value) {
_builder.setIgnoreEmpty(value)
}
/**
*
* IgnoreEmpty specifies that the validation rules of this field should be
* evaluated only if the field is not empty
*
*
* optional bool ignore_empty = 8;
*/
public fun clearIgnoreEmpty() {
_builder.clearIgnoreEmpty()
}
/**
*
* IgnoreEmpty specifies that the validation rules of this field should be
* evaluated only if the field is not empty
*
*
* optional bool ignore_empty = 8;
* @return Whether the ignoreEmpty field is set.
*/
public fun hasIgnoreEmpty(): kotlin.Boolean {
return _builder.hasIgnoreEmpty()
}
}
}
@kotlin.jvm.JvmSynthetic
public inline fun io.envoyproxy.pgv.validate.Validate.SFixed32Rules.copy(block: io.envoyproxy.pgv.validate.SFixed32RulesKt.Dsl.() -> kotlin.Unit): io.envoyproxy.pgv.validate.Validate.SFixed32Rules =
io.envoyproxy.pgv.validate.SFixed32RulesKt.Dsl._create(this.toBuilder()).apply { block() }._build()
© 2015 - 2025 Weber Informatics LLC | Privacy Policy