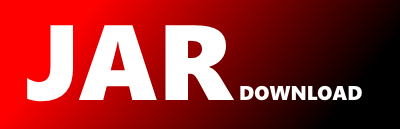
io.envoyproxy.pgv.validate.StringRulesKt.kt Maven / Gradle / Ivy
//Generated by the protocol buffer compiler. DO NOT EDIT!
// source: validate/validate.proto
package io.envoyproxy.pgv.validate;
@kotlin.jvm.JvmName("-initializestringRules")
public inline fun stringRules(block: io.envoyproxy.pgv.validate.StringRulesKt.Dsl.() -> kotlin.Unit): io.envoyproxy.pgv.validate.Validate.StringRules =
io.envoyproxy.pgv.validate.StringRulesKt.Dsl._create(io.envoyproxy.pgv.validate.Validate.StringRules.newBuilder()).apply { block() }._build()
public object StringRulesKt {
@kotlin.OptIn(com.google.protobuf.kotlin.OnlyForUseByGeneratedProtoCode::class)
@com.google.protobuf.kotlin.ProtoDslMarker
public class Dsl private constructor(
private val _builder: io.envoyproxy.pgv.validate.Validate.StringRules.Builder
) {
public companion object {
@kotlin.jvm.JvmSynthetic
@kotlin.PublishedApi
internal fun _create(builder: io.envoyproxy.pgv.validate.Validate.StringRules.Builder): Dsl = Dsl(builder)
}
@kotlin.jvm.JvmSynthetic
@kotlin.PublishedApi
internal fun _build(): io.envoyproxy.pgv.validate.Validate.StringRules = _builder.build()
/**
*
* Const specifies that this field must be exactly the specified value
*
*
* optional string const = 1;
*/
public var const: kotlin.String
@JvmName("getConst")
get() = _builder.getConst()
@JvmName("setConst")
set(value) {
_builder.setConst(value)
}
/**
*
* Const specifies that this field must be exactly the specified value
*
*
* optional string const = 1;
*/
public fun clearConst() {
_builder.clearConst()
}
/**
*
* Const specifies that this field must be exactly the specified value
*
*
* optional string const = 1;
* @return Whether the const field is set.
*/
public fun hasConst(): kotlin.Boolean {
return _builder.hasConst()
}
/**
*
* Len specifies that this field must be the specified number of
* characters (Unicode code points). Note that the number of
* characters may differ from the number of bytes in the string.
*
*
* optional uint64 len = 19;
*/
public var len: kotlin.Long
@JvmName("getLen")
get() = _builder.getLen()
@JvmName("setLen")
set(value) {
_builder.setLen(value)
}
/**
*
* Len specifies that this field must be the specified number of
* characters (Unicode code points). Note that the number of
* characters may differ from the number of bytes in the string.
*
*
* optional uint64 len = 19;
*/
public fun clearLen() {
_builder.clearLen()
}
/**
*
* Len specifies that this field must be the specified number of
* characters (Unicode code points). Note that the number of
* characters may differ from the number of bytes in the string.
*
*
* optional uint64 len = 19;
* @return Whether the len field is set.
*/
public fun hasLen(): kotlin.Boolean {
return _builder.hasLen()
}
/**
*
* MinLen specifies that this field must be the specified number of
* characters (Unicode code points) at a minimum. Note that the number of
* characters may differ from the number of bytes in the string.
*
*
* optional uint64 min_len = 2;
*/
public var minLen: kotlin.Long
@JvmName("getMinLen")
get() = _builder.getMinLen()
@JvmName("setMinLen")
set(value) {
_builder.setMinLen(value)
}
/**
*
* MinLen specifies that this field must be the specified number of
* characters (Unicode code points) at a minimum. Note that the number of
* characters may differ from the number of bytes in the string.
*
*
* optional uint64 min_len = 2;
*/
public fun clearMinLen() {
_builder.clearMinLen()
}
/**
*
* MinLen specifies that this field must be the specified number of
* characters (Unicode code points) at a minimum. Note that the number of
* characters may differ from the number of bytes in the string.
*
*
* optional uint64 min_len = 2;
* @return Whether the minLen field is set.
*/
public fun hasMinLen(): kotlin.Boolean {
return _builder.hasMinLen()
}
/**
*
* MaxLen specifies that this field must be the specified number of
* characters (Unicode code points) at a maximum. Note that the number of
* characters may differ from the number of bytes in the string.
*
*
* optional uint64 max_len = 3;
*/
public var maxLen: kotlin.Long
@JvmName("getMaxLen")
get() = _builder.getMaxLen()
@JvmName("setMaxLen")
set(value) {
_builder.setMaxLen(value)
}
/**
*
* MaxLen specifies that this field must be the specified number of
* characters (Unicode code points) at a maximum. Note that the number of
* characters may differ from the number of bytes in the string.
*
*
* optional uint64 max_len = 3;
*/
public fun clearMaxLen() {
_builder.clearMaxLen()
}
/**
*
* MaxLen specifies that this field must be the specified number of
* characters (Unicode code points) at a maximum. Note that the number of
* characters may differ from the number of bytes in the string.
*
*
* optional uint64 max_len = 3;
* @return Whether the maxLen field is set.
*/
public fun hasMaxLen(): kotlin.Boolean {
return _builder.hasMaxLen()
}
/**
*
* LenBytes specifies that this field must be the specified number of bytes
*
*
* optional uint64 len_bytes = 20;
*/
public var lenBytes: kotlin.Long
@JvmName("getLenBytes")
get() = _builder.getLenBytes()
@JvmName("setLenBytes")
set(value) {
_builder.setLenBytes(value)
}
/**
*
* LenBytes specifies that this field must be the specified number of bytes
*
*
* optional uint64 len_bytes = 20;
*/
public fun clearLenBytes() {
_builder.clearLenBytes()
}
/**
*
* LenBytes specifies that this field must be the specified number of bytes
*
*
* optional uint64 len_bytes = 20;
* @return Whether the lenBytes field is set.
*/
public fun hasLenBytes(): kotlin.Boolean {
return _builder.hasLenBytes()
}
/**
*
* MinBytes specifies that this field must be the specified number of bytes
* at a minimum
*
*
* optional uint64 min_bytes = 4;
*/
public var minBytes: kotlin.Long
@JvmName("getMinBytes")
get() = _builder.getMinBytes()
@JvmName("setMinBytes")
set(value) {
_builder.setMinBytes(value)
}
/**
*
* MinBytes specifies that this field must be the specified number of bytes
* at a minimum
*
*
* optional uint64 min_bytes = 4;
*/
public fun clearMinBytes() {
_builder.clearMinBytes()
}
/**
*
* MinBytes specifies that this field must be the specified number of bytes
* at a minimum
*
*
* optional uint64 min_bytes = 4;
* @return Whether the minBytes field is set.
*/
public fun hasMinBytes(): kotlin.Boolean {
return _builder.hasMinBytes()
}
/**
*
* MaxBytes specifies that this field must be the specified number of bytes
* at a maximum
*
*
* optional uint64 max_bytes = 5;
*/
public var maxBytes: kotlin.Long
@JvmName("getMaxBytes")
get() = _builder.getMaxBytes()
@JvmName("setMaxBytes")
set(value) {
_builder.setMaxBytes(value)
}
/**
*
* MaxBytes specifies that this field must be the specified number of bytes
* at a maximum
*
*
* optional uint64 max_bytes = 5;
*/
public fun clearMaxBytes() {
_builder.clearMaxBytes()
}
/**
*
* MaxBytes specifies that this field must be the specified number of bytes
* at a maximum
*
*
* optional uint64 max_bytes = 5;
* @return Whether the maxBytes field is set.
*/
public fun hasMaxBytes(): kotlin.Boolean {
return _builder.hasMaxBytes()
}
/**
*
* Pattern specifes that this field must match against the specified
* regular expression (RE2 syntax). The included expression should elide
* any delimiters.
*
*
* optional string pattern = 6;
*/
public var pattern: kotlin.String
@JvmName("getPattern")
get() = _builder.getPattern()
@JvmName("setPattern")
set(value) {
_builder.setPattern(value)
}
/**
*
* Pattern specifes that this field must match against the specified
* regular expression (RE2 syntax). The included expression should elide
* any delimiters.
*
*
* optional string pattern = 6;
*/
public fun clearPattern() {
_builder.clearPattern()
}
/**
*
* Pattern specifes that this field must match against the specified
* regular expression (RE2 syntax). The included expression should elide
* any delimiters.
*
*
* optional string pattern = 6;
* @return Whether the pattern field is set.
*/
public fun hasPattern(): kotlin.Boolean {
return _builder.hasPattern()
}
/**
*
* Prefix specifies that this field must have the specified substring at
* the beginning of the string.
*
*
* optional string prefix = 7;
*/
public var prefix: kotlin.String
@JvmName("getPrefix")
get() = _builder.getPrefix()
@JvmName("setPrefix")
set(value) {
_builder.setPrefix(value)
}
/**
*
* Prefix specifies that this field must have the specified substring at
* the beginning of the string.
*
*
* optional string prefix = 7;
*/
public fun clearPrefix() {
_builder.clearPrefix()
}
/**
*
* Prefix specifies that this field must have the specified substring at
* the beginning of the string.
*
*
* optional string prefix = 7;
* @return Whether the prefix field is set.
*/
public fun hasPrefix(): kotlin.Boolean {
return _builder.hasPrefix()
}
/**
*
* Suffix specifies that this field must have the specified substring at
* the end of the string.
*
*
* optional string suffix = 8;
*/
public var suffix: kotlin.String
@JvmName("getSuffix")
get() = _builder.getSuffix()
@JvmName("setSuffix")
set(value) {
_builder.setSuffix(value)
}
/**
*
* Suffix specifies that this field must have the specified substring at
* the end of the string.
*
*
* optional string suffix = 8;
*/
public fun clearSuffix() {
_builder.clearSuffix()
}
/**
*
* Suffix specifies that this field must have the specified substring at
* the end of the string.
*
*
* optional string suffix = 8;
* @return Whether the suffix field is set.
*/
public fun hasSuffix(): kotlin.Boolean {
return _builder.hasSuffix()
}
/**
*
* Contains specifies that this field must have the specified substring
* anywhere in the string.
*
*
* optional string contains = 9;
*/
public var contains: kotlin.String
@JvmName("getContains")
get() = _builder.getContains()
@JvmName("setContains")
set(value) {
_builder.setContains(value)
}
/**
*
* Contains specifies that this field must have the specified substring
* anywhere in the string.
*
*
* optional string contains = 9;
*/
public fun clearContains() {
_builder.clearContains()
}
/**
*
* Contains specifies that this field must have the specified substring
* anywhere in the string.
*
*
* optional string contains = 9;
* @return Whether the contains field is set.
*/
public fun hasContains(): kotlin.Boolean {
return _builder.hasContains()
}
/**
*
* NotContains specifies that this field cannot have the specified substring
* anywhere in the string.
*
*
* optional string not_contains = 23;
*/
public var notContains: kotlin.String
@JvmName("getNotContains")
get() = _builder.getNotContains()
@JvmName("setNotContains")
set(value) {
_builder.setNotContains(value)
}
/**
*
* NotContains specifies that this field cannot have the specified substring
* anywhere in the string.
*
*
* optional string not_contains = 23;
*/
public fun clearNotContains() {
_builder.clearNotContains()
}
/**
*
* NotContains specifies that this field cannot have the specified substring
* anywhere in the string.
*
*
* optional string not_contains = 23;
* @return Whether the notContains field is set.
*/
public fun hasNotContains(): kotlin.Boolean {
return _builder.hasNotContains()
}
/**
* An uninstantiable, behaviorless type to represent the field in
* generics.
*/
@kotlin.OptIn(com.google.protobuf.kotlin.OnlyForUseByGeneratedProtoCode::class)
public class In_Proxy private constructor() : com.google.protobuf.kotlin.DslProxy()
/**
*
* In specifies that this field must be equal to one of the specified
* values
*
*
* repeated string in = 10;
* @return A list containing the in.
*/
public val in_: com.google.protobuf.kotlin.DslList
@kotlin.jvm.JvmSynthetic
get() = com.google.protobuf.kotlin.DslList(
_builder.getInList()
)
/**
*
* In specifies that this field must be equal to one of the specified
* values
*
*
* repeated string in = 10;
* @param value The in to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("addIn_")
public fun com.google.protobuf.kotlin.DslList.add(value: kotlin.String) {
_builder.addIn(value)
}
/**
*
* In specifies that this field must be equal to one of the specified
* values
*
*
* repeated string in = 10;
* @param value The in to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("plusAssignIn_")
@Suppress("NOTHING_TO_INLINE")
public inline operator fun com.google.protobuf.kotlin.DslList.plusAssign(value: kotlin.String) {
add(value)
}
/**
*
* In specifies that this field must be equal to one of the specified
* values
*
*
* repeated string in = 10;
* @param values The in to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("addAllIn_")
public fun com.google.protobuf.kotlin.DslList.addAll(values: kotlin.collections.Iterable) {
_builder.addAllIn(values)
}
/**
*
* In specifies that this field must be equal to one of the specified
* values
*
*
* repeated string in = 10;
* @param values The in to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("plusAssignAllIn_")
@Suppress("NOTHING_TO_INLINE")
public inline operator fun com.google.protobuf.kotlin.DslList.plusAssign(values: kotlin.collections.Iterable) {
addAll(values)
}
/**
*
* In specifies that this field must be equal to one of the specified
* values
*
*
* repeated string in = 10;
* @param index The index to set the value at.
* @param value The in to set.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("setIn_")
public operator fun com.google.protobuf.kotlin.DslList.set(index: kotlin.Int, value: kotlin.String) {
_builder.setIn(index, value)
}/**
*
* In specifies that this field must be equal to one of the specified
* values
*
*
* repeated string in = 10;
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("clearIn_")
public fun com.google.protobuf.kotlin.DslList.clear() {
_builder.clearIn()
}
/**
* An uninstantiable, behaviorless type to represent the field in
* generics.
*/
@kotlin.OptIn(com.google.protobuf.kotlin.OnlyForUseByGeneratedProtoCode::class)
public class NotInProxy private constructor() : com.google.protobuf.kotlin.DslProxy()
/**
*
* NotIn specifies that this field cannot be equal to one of the specified
* values
*
*
* repeated string not_in = 11;
* @return A list containing the notIn.
*/
public val notIn: com.google.protobuf.kotlin.DslList
@kotlin.jvm.JvmSynthetic
get() = com.google.protobuf.kotlin.DslList(
_builder.getNotInList()
)
/**
*
* NotIn specifies that this field cannot be equal to one of the specified
* values
*
*
* repeated string not_in = 11;
* @param value The notIn to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("addNotIn")
public fun com.google.protobuf.kotlin.DslList.add(value: kotlin.String) {
_builder.addNotIn(value)
}
/**
*
* NotIn specifies that this field cannot be equal to one of the specified
* values
*
*
* repeated string not_in = 11;
* @param value The notIn to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("plusAssignNotIn")
@Suppress("NOTHING_TO_INLINE")
public inline operator fun com.google.protobuf.kotlin.DslList.plusAssign(value: kotlin.String) {
add(value)
}
/**
*
* NotIn specifies that this field cannot be equal to one of the specified
* values
*
*
* repeated string not_in = 11;
* @param values The notIn to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("addAllNotIn")
public fun com.google.protobuf.kotlin.DslList.addAll(values: kotlin.collections.Iterable) {
_builder.addAllNotIn(values)
}
/**
*
* NotIn specifies that this field cannot be equal to one of the specified
* values
*
*
* repeated string not_in = 11;
* @param values The notIn to add.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("plusAssignAllNotIn")
@Suppress("NOTHING_TO_INLINE")
public inline operator fun com.google.protobuf.kotlin.DslList.plusAssign(values: kotlin.collections.Iterable) {
addAll(values)
}
/**
*
* NotIn specifies that this field cannot be equal to one of the specified
* values
*
*
* repeated string not_in = 11;
* @param index The index to set the value at.
* @param value The notIn to set.
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("setNotIn")
public operator fun com.google.protobuf.kotlin.DslList.set(index: kotlin.Int, value: kotlin.String) {
_builder.setNotIn(index, value)
}/**
*
* NotIn specifies that this field cannot be equal to one of the specified
* values
*
*
* repeated string not_in = 11;
*/
@kotlin.jvm.JvmSynthetic
@kotlin.jvm.JvmName("clearNotIn")
public fun com.google.protobuf.kotlin.DslList.clear() {
_builder.clearNotIn()
}
/**
*
* Email specifies that the field must be a valid email address as
* defined by RFC 5322
*
*
* bool email = 12;
*/
public var email: kotlin.Boolean
@JvmName("getEmail")
get() = _builder.getEmail()
@JvmName("setEmail")
set(value) {
_builder.setEmail(value)
}
/**
*
* Email specifies that the field must be a valid email address as
* defined by RFC 5322
*
*
* bool email = 12;
*/
public fun clearEmail() {
_builder.clearEmail()
}
/**
*
* Email specifies that the field must be a valid email address as
* defined by RFC 5322
*
*
* bool email = 12;
* @return Whether the email field is set.
*/
public fun hasEmail(): kotlin.Boolean {
return _builder.hasEmail()
}
/**
*
* Hostname specifies that the field must be a valid hostname as
* defined by RFC 1034. This constraint does not support
* internationalized domain names (IDNs).
*
*
* bool hostname = 13;
*/
public var hostname: kotlin.Boolean
@JvmName("getHostname")
get() = _builder.getHostname()
@JvmName("setHostname")
set(value) {
_builder.setHostname(value)
}
/**
*
* Hostname specifies that the field must be a valid hostname as
* defined by RFC 1034. This constraint does not support
* internationalized domain names (IDNs).
*
*
* bool hostname = 13;
*/
public fun clearHostname() {
_builder.clearHostname()
}
/**
*
* Hostname specifies that the field must be a valid hostname as
* defined by RFC 1034. This constraint does not support
* internationalized domain names (IDNs).
*
*
* bool hostname = 13;
* @return Whether the hostname field is set.
*/
public fun hasHostname(): kotlin.Boolean {
return _builder.hasHostname()
}
/**
*
* Ip specifies that the field must be a valid IP (v4 or v6) address.
* Valid IPv6 addresses should not include surrounding square brackets.
*
*
* bool ip = 14;
*/
public var ip: kotlin.Boolean
@JvmName("getIp")
get() = _builder.getIp()
@JvmName("setIp")
set(value) {
_builder.setIp(value)
}
/**
*
* Ip specifies that the field must be a valid IP (v4 or v6) address.
* Valid IPv6 addresses should not include surrounding square brackets.
*
*
* bool ip = 14;
*/
public fun clearIp() {
_builder.clearIp()
}
/**
*
* Ip specifies that the field must be a valid IP (v4 or v6) address.
* Valid IPv6 addresses should not include surrounding square brackets.
*
*
* bool ip = 14;
* @return Whether the ip field is set.
*/
public fun hasIp(): kotlin.Boolean {
return _builder.hasIp()
}
/**
*
* Ipv4 specifies that the field must be a valid IPv4 address.
*
*
* bool ipv4 = 15;
*/
public var ipv4: kotlin.Boolean
@JvmName("getIpv4")
get() = _builder.getIpv4()
@JvmName("setIpv4")
set(value) {
_builder.setIpv4(value)
}
/**
*
* Ipv4 specifies that the field must be a valid IPv4 address.
*
*
* bool ipv4 = 15;
*/
public fun clearIpv4() {
_builder.clearIpv4()
}
/**
*
* Ipv4 specifies that the field must be a valid IPv4 address.
*
*
* bool ipv4 = 15;
* @return Whether the ipv4 field is set.
*/
public fun hasIpv4(): kotlin.Boolean {
return _builder.hasIpv4()
}
/**
*
* Ipv6 specifies that the field must be a valid IPv6 address. Valid
* IPv6 addresses should not include surrounding square brackets.
*
*
* bool ipv6 = 16;
*/
public var ipv6: kotlin.Boolean
@JvmName("getIpv6")
get() = _builder.getIpv6()
@JvmName("setIpv6")
set(value) {
_builder.setIpv6(value)
}
/**
*
* Ipv6 specifies that the field must be a valid IPv6 address. Valid
* IPv6 addresses should not include surrounding square brackets.
*
*
* bool ipv6 = 16;
*/
public fun clearIpv6() {
_builder.clearIpv6()
}
/**
*
* Ipv6 specifies that the field must be a valid IPv6 address. Valid
* IPv6 addresses should not include surrounding square brackets.
*
*
* bool ipv6 = 16;
* @return Whether the ipv6 field is set.
*/
public fun hasIpv6(): kotlin.Boolean {
return _builder.hasIpv6()
}
/**
*
* Uri specifies that the field must be a valid, absolute URI as defined
* by RFC 3986
*
*
* bool uri = 17;
*/
public var uri: kotlin.Boolean
@JvmName("getUri")
get() = _builder.getUri()
@JvmName("setUri")
set(value) {
_builder.setUri(value)
}
/**
*
* Uri specifies that the field must be a valid, absolute URI as defined
* by RFC 3986
*
*
* bool uri = 17;
*/
public fun clearUri() {
_builder.clearUri()
}
/**
*
* Uri specifies that the field must be a valid, absolute URI as defined
* by RFC 3986
*
*
* bool uri = 17;
* @return Whether the uri field is set.
*/
public fun hasUri(): kotlin.Boolean {
return _builder.hasUri()
}
/**
*
* UriRef specifies that the field must be a valid URI as defined by RFC
* 3986 and may be relative or absolute.
*
*
* bool uri_ref = 18;
*/
public var uriRef: kotlin.Boolean
@JvmName("getUriRef")
get() = _builder.getUriRef()
@JvmName("setUriRef")
set(value) {
_builder.setUriRef(value)
}
/**
*
* UriRef specifies that the field must be a valid URI as defined by RFC
* 3986 and may be relative or absolute.
*
*
* bool uri_ref = 18;
*/
public fun clearUriRef() {
_builder.clearUriRef()
}
/**
*
* UriRef specifies that the field must be a valid URI as defined by RFC
* 3986 and may be relative or absolute.
*
*
* bool uri_ref = 18;
* @return Whether the uriRef field is set.
*/
public fun hasUriRef(): kotlin.Boolean {
return _builder.hasUriRef()
}
/**
*
* Address specifies that the field must be either a valid hostname as
* defined by RFC 1034 (which does not support internationalized domain
* names or IDNs), or it can be a valid IP (v4 or v6).
*
*
* bool address = 21;
*/
public var address: kotlin.Boolean
@JvmName("getAddress")
get() = _builder.getAddress()
@JvmName("setAddress")
set(value) {
_builder.setAddress(value)
}
/**
*
* Address specifies that the field must be either a valid hostname as
* defined by RFC 1034 (which does not support internationalized domain
* names or IDNs), or it can be a valid IP (v4 or v6).
*
*
* bool address = 21;
*/
public fun clearAddress() {
_builder.clearAddress()
}
/**
*
* Address specifies that the field must be either a valid hostname as
* defined by RFC 1034 (which does not support internationalized domain
* names or IDNs), or it can be a valid IP (v4 or v6).
*
*
* bool address = 21;
* @return Whether the address field is set.
*/
public fun hasAddress(): kotlin.Boolean {
return _builder.hasAddress()
}
/**
*
* Uuid specifies that the field must be a valid UUID as defined by
* RFC 4122
*
*
* bool uuid = 22;
*/
public var uuid: kotlin.Boolean
@JvmName("getUuid")
get() = _builder.getUuid()
@JvmName("setUuid")
set(value) {
_builder.setUuid(value)
}
/**
*
* Uuid specifies that the field must be a valid UUID as defined by
* RFC 4122
*
*
* bool uuid = 22;
*/
public fun clearUuid() {
_builder.clearUuid()
}
/**
*
* Uuid specifies that the field must be a valid UUID as defined by
* RFC 4122
*
*
* bool uuid = 22;
* @return Whether the uuid field is set.
*/
public fun hasUuid(): kotlin.Boolean {
return _builder.hasUuid()
}
/**
*
* WellKnownRegex specifies a common well known pattern defined as a regex.
*
*
* .validate.KnownRegex well_known_regex = 24;
*/
public var wellKnownRegex: io.envoyproxy.pgv.validate.Validate.KnownRegex
@JvmName("getWellKnownRegex")
get() = _builder.getWellKnownRegex()
@JvmName("setWellKnownRegex")
set(value) {
_builder.setWellKnownRegex(value)
}
/**
*
* WellKnownRegex specifies a common well known pattern defined as a regex.
*
*
* .validate.KnownRegex well_known_regex = 24;
*/
public fun clearWellKnownRegex() {
_builder.clearWellKnownRegex()
}
/**
*
* WellKnownRegex specifies a common well known pattern defined as a regex.
*
*
* .validate.KnownRegex well_known_regex = 24;
* @return Whether the wellKnownRegex field is set.
*/
public fun hasWellKnownRegex(): kotlin.Boolean {
return _builder.hasWellKnownRegex()
}
/**
*
* This applies to regexes HTTP_HEADER_NAME and HTTP_HEADER_VALUE to enable
* strict header validation.
* By default, this is true, and HTTP header validations are RFC-compliant.
* Setting to false will enable a looser validations that only disallows
* \r\n\0 characters, which can be used to bypass header matching rules.
*
*
* optional bool strict = 25 [default = true];
*/
public var strict: kotlin.Boolean
@JvmName("getStrict")
get() = _builder.getStrict()
@JvmName("setStrict")
set(value) {
_builder.setStrict(value)
}
/**
*
* This applies to regexes HTTP_HEADER_NAME and HTTP_HEADER_VALUE to enable
* strict header validation.
* By default, this is true, and HTTP header validations are RFC-compliant.
* Setting to false will enable a looser validations that only disallows
* \r\n\0 characters, which can be used to bypass header matching rules.
*
*
* optional bool strict = 25 [default = true];
*/
public fun clearStrict() {
_builder.clearStrict()
}
/**
*
* This applies to regexes HTTP_HEADER_NAME and HTTP_HEADER_VALUE to enable
* strict header validation.
* By default, this is true, and HTTP header validations are RFC-compliant.
* Setting to false will enable a looser validations that only disallows
* \r\n\0 characters, which can be used to bypass header matching rules.
*
*
* optional bool strict = 25 [default = true];
* @return Whether the strict field is set.
*/
public fun hasStrict(): kotlin.Boolean {
return _builder.hasStrict()
}
/**
*
* IgnoreEmpty specifies that the validation rules of this field should be
* evaluated only if the field is not empty
*
*
* optional bool ignore_empty = 26;
*/
public var ignoreEmpty: kotlin.Boolean
@JvmName("getIgnoreEmpty")
get() = _builder.getIgnoreEmpty()
@JvmName("setIgnoreEmpty")
set(value) {
_builder.setIgnoreEmpty(value)
}
/**
*
* IgnoreEmpty specifies that the validation rules of this field should be
* evaluated only if the field is not empty
*
*
* optional bool ignore_empty = 26;
*/
public fun clearIgnoreEmpty() {
_builder.clearIgnoreEmpty()
}
/**
*
* IgnoreEmpty specifies that the validation rules of this field should be
* evaluated only if the field is not empty
*
*
* optional bool ignore_empty = 26;
* @return Whether the ignoreEmpty field is set.
*/
public fun hasIgnoreEmpty(): kotlin.Boolean {
return _builder.hasIgnoreEmpty()
}
public val wellKnownCase: io.envoyproxy.pgv.validate.Validate.StringRules.WellKnownCase
@JvmName("getWellKnownCase")
get() = _builder.getWellKnownCase()
public fun clearWellKnown() {
_builder.clearWellKnown()
}
}
}
@kotlin.jvm.JvmSynthetic
public inline fun io.envoyproxy.pgv.validate.Validate.StringRules.copy(block: io.envoyproxy.pgv.validate.StringRulesKt.Dsl.() -> kotlin.Unit): io.envoyproxy.pgv.validate.Validate.StringRules =
io.envoyproxy.pgv.validate.StringRulesKt.Dsl._create(this.toBuilder()).apply { block() }._build()
© 2015 - 2025 Weber Informatics LLC | Privacy Policy