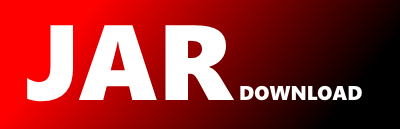
io.envoyproxy.pgv.validate.TimestampRulesKt.kt Maven / Gradle / Ivy
The newest version!
//Generated by the protocol buffer compiler. DO NOT EDIT!
// source: validate/validate.proto
package io.envoyproxy.pgv.validate;
@kotlin.jvm.JvmName("-initializetimestampRules")
public inline fun timestampRules(block: io.envoyproxy.pgv.validate.TimestampRulesKt.Dsl.() -> kotlin.Unit): io.envoyproxy.pgv.validate.Validate.TimestampRules =
io.envoyproxy.pgv.validate.TimestampRulesKt.Dsl._create(io.envoyproxy.pgv.validate.Validate.TimestampRules.newBuilder()).apply { block() }._build()
public object TimestampRulesKt {
@kotlin.OptIn(com.google.protobuf.kotlin.OnlyForUseByGeneratedProtoCode::class)
@com.google.protobuf.kotlin.ProtoDslMarker
public class Dsl private constructor(
private val _builder: io.envoyproxy.pgv.validate.Validate.TimestampRules.Builder
) {
public companion object {
@kotlin.jvm.JvmSynthetic
@kotlin.PublishedApi
internal fun _create(builder: io.envoyproxy.pgv.validate.Validate.TimestampRules.Builder): Dsl = Dsl(builder)
}
@kotlin.jvm.JvmSynthetic
@kotlin.PublishedApi
internal fun _build(): io.envoyproxy.pgv.validate.Validate.TimestampRules = _builder.build()
/**
*
* Required specifies that this field must be set
*
*
* optional bool required = 1;
*/
public var required: kotlin.Boolean
@JvmName("getRequired")
get() = _builder.getRequired()
@JvmName("setRequired")
set(value) {
_builder.setRequired(value)
}
/**
*
* Required specifies that this field must be set
*
*
* optional bool required = 1;
*/
public fun clearRequired() {
_builder.clearRequired()
}
/**
*
* Required specifies that this field must be set
*
*
* optional bool required = 1;
* @return Whether the required field is set.
*/
public fun hasRequired(): kotlin.Boolean {
return _builder.hasRequired()
}
/**
*
* Const specifies that this field must be exactly the specified value
*
*
* optional .google.protobuf.Timestamp const = 2;
*/
public var const: com.google.protobuf.Timestamp
@JvmName("getConst")
get() = _builder.getConst()
@JvmName("setConst")
set(value) {
_builder.setConst(value)
}
/**
*
* Const specifies that this field must be exactly the specified value
*
*
* optional .google.protobuf.Timestamp const = 2;
*/
public fun clearConst() {
_builder.clearConst()
}
/**
*
* Const specifies that this field must be exactly the specified value
*
*
* optional .google.protobuf.Timestamp const = 2;
* @return Whether the const field is set.
*/
public fun hasConst(): kotlin.Boolean {
return _builder.hasConst()
}
public val TimestampRulesKt.Dsl.constOrNull: com.google.protobuf.Timestamp?
get() = _builder.constOrNull
/**
*
* Lt specifies that this field must be less than the specified value,
* exclusive
*
*
* optional .google.protobuf.Timestamp lt = 3;
*/
public var lt: com.google.protobuf.Timestamp
@JvmName("getLt")
get() = _builder.getLt()
@JvmName("setLt")
set(value) {
_builder.setLt(value)
}
/**
*
* Lt specifies that this field must be less than the specified value,
* exclusive
*
*
* optional .google.protobuf.Timestamp lt = 3;
*/
public fun clearLt() {
_builder.clearLt()
}
/**
*
* Lt specifies that this field must be less than the specified value,
* exclusive
*
*
* optional .google.protobuf.Timestamp lt = 3;
* @return Whether the lt field is set.
*/
public fun hasLt(): kotlin.Boolean {
return _builder.hasLt()
}
public val TimestampRulesKt.Dsl.ltOrNull: com.google.protobuf.Timestamp?
get() = _builder.ltOrNull
/**
*
* Lte specifies that this field must be less than the specified value,
* inclusive
*
*
* optional .google.protobuf.Timestamp lte = 4;
*/
public var lte: com.google.protobuf.Timestamp
@JvmName("getLte")
get() = _builder.getLte()
@JvmName("setLte")
set(value) {
_builder.setLte(value)
}
/**
*
* Lte specifies that this field must be less than the specified value,
* inclusive
*
*
* optional .google.protobuf.Timestamp lte = 4;
*/
public fun clearLte() {
_builder.clearLte()
}
/**
*
* Lte specifies that this field must be less than the specified value,
* inclusive
*
*
* optional .google.protobuf.Timestamp lte = 4;
* @return Whether the lte field is set.
*/
public fun hasLte(): kotlin.Boolean {
return _builder.hasLte()
}
public val TimestampRulesKt.Dsl.lteOrNull: com.google.protobuf.Timestamp?
get() = _builder.lteOrNull
/**
*
* Gt specifies that this field must be greater than the specified value,
* exclusive
*
*
* optional .google.protobuf.Timestamp gt = 5;
*/
public var gt: com.google.protobuf.Timestamp
@JvmName("getGt")
get() = _builder.getGt()
@JvmName("setGt")
set(value) {
_builder.setGt(value)
}
/**
*
* Gt specifies that this field must be greater than the specified value,
* exclusive
*
*
* optional .google.protobuf.Timestamp gt = 5;
*/
public fun clearGt() {
_builder.clearGt()
}
/**
*
* Gt specifies that this field must be greater than the specified value,
* exclusive
*
*
* optional .google.protobuf.Timestamp gt = 5;
* @return Whether the gt field is set.
*/
public fun hasGt(): kotlin.Boolean {
return _builder.hasGt()
}
public val TimestampRulesKt.Dsl.gtOrNull: com.google.protobuf.Timestamp?
get() = _builder.gtOrNull
/**
*
* Gte specifies that this field must be greater than the specified value,
* inclusive
*
*
* optional .google.protobuf.Timestamp gte = 6;
*/
public var gte: com.google.protobuf.Timestamp
@JvmName("getGte")
get() = _builder.getGte()
@JvmName("setGte")
set(value) {
_builder.setGte(value)
}
/**
*
* Gte specifies that this field must be greater than the specified value,
* inclusive
*
*
* optional .google.protobuf.Timestamp gte = 6;
*/
public fun clearGte() {
_builder.clearGte()
}
/**
*
* Gte specifies that this field must be greater than the specified value,
* inclusive
*
*
* optional .google.protobuf.Timestamp gte = 6;
* @return Whether the gte field is set.
*/
public fun hasGte(): kotlin.Boolean {
return _builder.hasGte()
}
public val TimestampRulesKt.Dsl.gteOrNull: com.google.protobuf.Timestamp?
get() = _builder.gteOrNull
/**
*
* LtNow specifies that this must be less than the current time. LtNow
* can only be used with the Within rule.
*
*
* optional bool lt_now = 7;
*/
public var ltNow: kotlin.Boolean
@JvmName("getLtNow")
get() = _builder.getLtNow()
@JvmName("setLtNow")
set(value) {
_builder.setLtNow(value)
}
/**
*
* LtNow specifies that this must be less than the current time. LtNow
* can only be used with the Within rule.
*
*
* optional bool lt_now = 7;
*/
public fun clearLtNow() {
_builder.clearLtNow()
}
/**
*
* LtNow specifies that this must be less than the current time. LtNow
* can only be used with the Within rule.
*
*
* optional bool lt_now = 7;
* @return Whether the ltNow field is set.
*/
public fun hasLtNow(): kotlin.Boolean {
return _builder.hasLtNow()
}
/**
*
* GtNow specifies that this must be greater than the current time. GtNow
* can only be used with the Within rule.
*
*
* optional bool gt_now = 8;
*/
public var gtNow: kotlin.Boolean
@JvmName("getGtNow")
get() = _builder.getGtNow()
@JvmName("setGtNow")
set(value) {
_builder.setGtNow(value)
}
/**
*
* GtNow specifies that this must be greater than the current time. GtNow
* can only be used with the Within rule.
*
*
* optional bool gt_now = 8;
*/
public fun clearGtNow() {
_builder.clearGtNow()
}
/**
*
* GtNow specifies that this must be greater than the current time. GtNow
* can only be used with the Within rule.
*
*
* optional bool gt_now = 8;
* @return Whether the gtNow field is set.
*/
public fun hasGtNow(): kotlin.Boolean {
return _builder.hasGtNow()
}
/**
*
* Within specifies that this field must be within this duration of the
* current time. This constraint can be used alone or with the LtNow and
* GtNow rules.
*
*
* optional .google.protobuf.Duration within = 9;
*/
public var within: com.google.protobuf.Duration
@JvmName("getWithin")
get() = _builder.getWithin()
@JvmName("setWithin")
set(value) {
_builder.setWithin(value)
}
/**
*
* Within specifies that this field must be within this duration of the
* current time. This constraint can be used alone or with the LtNow and
* GtNow rules.
*
*
* optional .google.protobuf.Duration within = 9;
*/
public fun clearWithin() {
_builder.clearWithin()
}
/**
*
* Within specifies that this field must be within this duration of the
* current time. This constraint can be used alone or with the LtNow and
* GtNow rules.
*
*
* optional .google.protobuf.Duration within = 9;
* @return Whether the within field is set.
*/
public fun hasWithin(): kotlin.Boolean {
return _builder.hasWithin()
}
public val TimestampRulesKt.Dsl.withinOrNull: com.google.protobuf.Duration?
get() = _builder.withinOrNull
}
}
@kotlin.jvm.JvmSynthetic
public inline fun io.envoyproxy.pgv.validate.Validate.TimestampRules.copy(block: io.envoyproxy.pgv.validate.TimestampRulesKt.Dsl.() -> kotlin.Unit): io.envoyproxy.pgv.validate.Validate.TimestampRules =
io.envoyproxy.pgv.validate.TimestampRulesKt.Dsl._create(this.toBuilder()).apply { block() }._build()
public val io.envoyproxy.pgv.validate.Validate.TimestampRulesOrBuilder.constOrNull: com.google.protobuf.Timestamp?
get() = if (hasConst()) getConst() else null
public val io.envoyproxy.pgv.validate.Validate.TimestampRulesOrBuilder.ltOrNull: com.google.protobuf.Timestamp?
get() = if (hasLt()) getLt() else null
public val io.envoyproxy.pgv.validate.Validate.TimestampRulesOrBuilder.lteOrNull: com.google.protobuf.Timestamp?
get() = if (hasLte()) getLte() else null
public val io.envoyproxy.pgv.validate.Validate.TimestampRulesOrBuilder.gtOrNull: com.google.protobuf.Timestamp?
get() = if (hasGt()) getGt() else null
public val io.envoyproxy.pgv.validate.Validate.TimestampRulesOrBuilder.gteOrNull: com.google.protobuf.Timestamp?
get() = if (hasGte()) getGte() else null
public val io.envoyproxy.pgv.validate.Validate.TimestampRulesOrBuilder.withinOrNull: com.google.protobuf.Duration?
get() = if (hasWithin()) getWithin() else null
© 2015 - 2025 Weber Informatics LLC | Privacy Policy