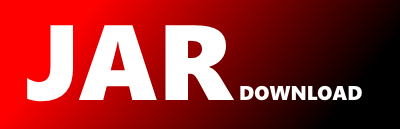
de.gold.scim.common.request.PatchRequestOperation Maven / Gradle / Ivy
The newest version!
package de.gold.scim.common.request;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import com.fasterxml.jackson.databind.JsonNode;
import de.gold.scim.common.constants.AttributeNames;
import de.gold.scim.common.constants.enums.PatchOp;
import de.gold.scim.common.resources.base.ScimObjectNode;
import lombok.Builder;
import lombok.NoArgsConstructor;
/**
* author Pascal Knueppel
* created at: 29.10.2019 - 08:32
*
* represents a single operation within a patch request
*/
@NoArgsConstructor
public class PatchRequestOperation extends ScimObjectNode
{
@Builder
public PatchRequestOperation(String path, PatchOp op, List values, JsonNode valueNode)
{
this();
setPath(path);
setOp(op);
if (values == null || values.isEmpty())
{
setValueNode(valueNode);
}
else
{
setValues(values);
}
}
/**
* The "path" attribute value is a String containing an attribute path describing the target of the
* operation.The "path" attribute is OPTIONAL for "add" and "replace" and is REQUIRED for "remove" operations.
*/
public Optional getPath()
{
return getStringAttribute(AttributeNames.RFC7643.PATH);
}
/**
* The "path" attribute value is a String containing an attribute path describing the target of the
* operation.The "path" attribute is OPTIONAL for "add" and "replace" and is REQUIRED for "remove" operations.
*/
public void setPath(String path)
{
setAttribute(AttributeNames.RFC7643.PATH, path);
}
/**
* Each PATCH operation object MUST have exactly one "op" member, whose value indicates the operation to
* perform and MAY be one of "add", "remove", or "replace"
* (This will never return null on server side for schema validation is executed before this method is called)
*/
public PatchOp getOp()
{
return getStringAttribute(AttributeNames.RFC7643.OP).map(PatchOp::getByValue).orElse(null);
}
/**
* Each PATCH operation object MUST have exactly one "op" member, whose value indicates the operation to
* perform and MAY be one of "add", "remove", or "replace"
*/
public void setOp(PatchOp patchOp)
{
setAttribute(AttributeNames.RFC7643.OP, patchOp == null ? null : patchOp.getValue());
}
/**
* the new value of the targeted attribute
* (This will never return null on server side for schema validation is executed before this method is called)
*/
public List getValues()
{
return getSimpleArrayAttribute(AttributeNames.RFC7643.VALUE);
}
/**
* the new value of the targeted attribute
*/
public void setValues(List value)
{
setAttributeList(AttributeNames.RFC7643.VALUE, value);
}
/**
* the new value of the targeted attribute. in this case the value is represented by the resource itself
*/
public void setValueNode(JsonNode value)
{
setAttribute(AttributeNames.RFC7643.VALUE, value == null ? null : Collections.singletonList(value));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy